SCM Git (Source Control Management using Git) is a version control system that allows multiple developers to collaborate on projects efficiently by tracking changes in source code over time.
Here’s a basic Git command to initialize a new Git repository:
git init
What is SCM?
Software Configuration Management (SCM) encompasses the disciplines and practices used to manage changes in software development projects. SCM is crucial for ensuring that the software remains consistent and functional as modifications occur. It plays a pivotal role in tracking changes, managing versions, and facilitating collaborative efforts among development teams.
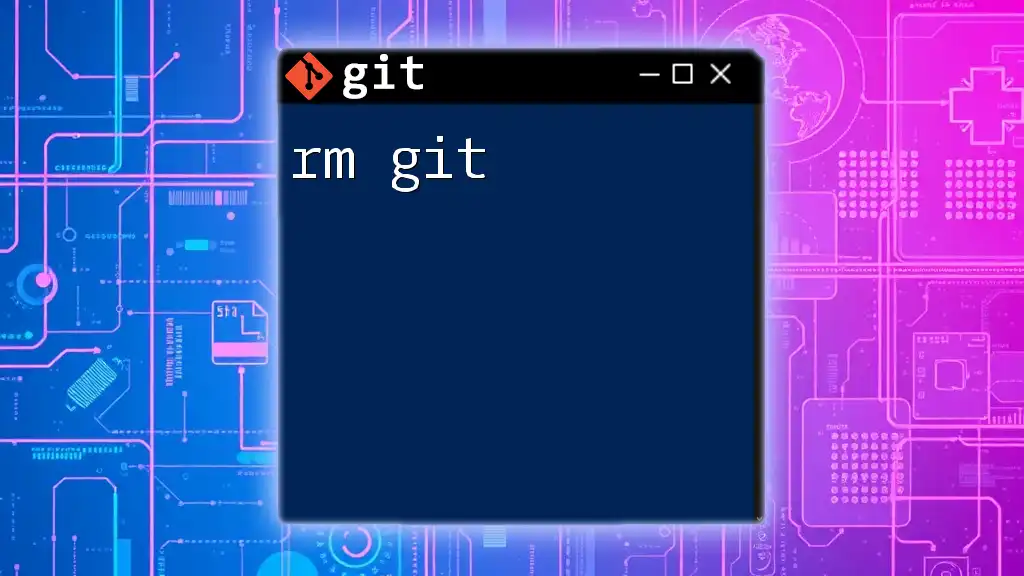
Overview of Git
Git is an open-source version control system designed to handle everything from small to very large projects with speed and efficiency. It was created in 2005 by Linus Torvalds, primarily for managing the Linux kernel source code. Unlike many other version control systems, Git allows developers to keep a complete history of their code, enabling easy collaboration and minimizing potential conflicts.
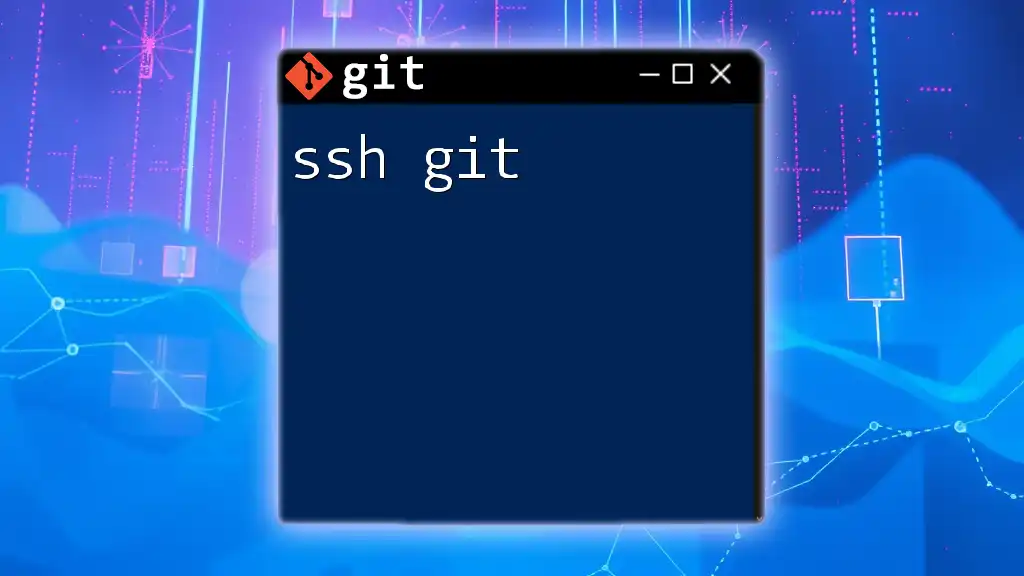
Understanding the Basics of Git
What is a Repository?
A repository is essentially a data structure that stores metadata for a set of files or directory structure. In Git, a repository serves as the heart of version control, containing all the versions of your code. There are two types of repositories:
- Local repositories are stored on your computer, providing quick access to the project history.
- Remote repositories are hosted on a server, allowing for collaboration when multiple people work on the same project.
Key Git Terminology
Familiarity with key Git terminology will assist you as you start to navigate this robust tool. Here are some terms to know:
- Commit: A snapshot of changes made to the files in a repository. Each commit has a unique ID.
- Branch: A separate line of development in your project. Branches allow you to work on features independently.
- Merge: The act of integrating changes from different branches, ensuring that all updates are combined.
- HEAD: A pointer to the current commit in your repository.
- Staging area: A temporary space where your changes are prepared before committing.
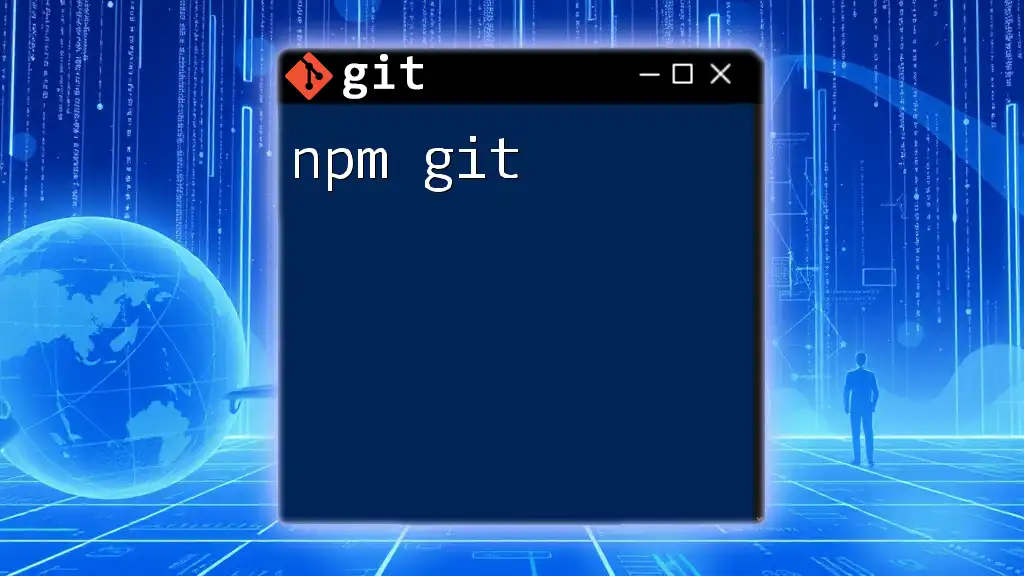
Setting Up Git
Installing Git
Installing Git is straightforward, and here are the steps depending on your operating system:
- Windows: Download and run the Git installer from the official Git website.
- macOS: Use Homebrew by running the command
brew install git
- Linux: For Debian-based systems, use:
sudo apt-get install git
After installation, you can verify it by checking the version:
git --version
Configuring Git
Configuring Git allows you to set your user information, which will be associated with your commits. This is particularly important for collaboration. Here’s how to do it:
Set your user name and email:
git config --global user.name "Your Name"
git config --global user.email "youremail@example.com"
To review your current settings:
git config --list
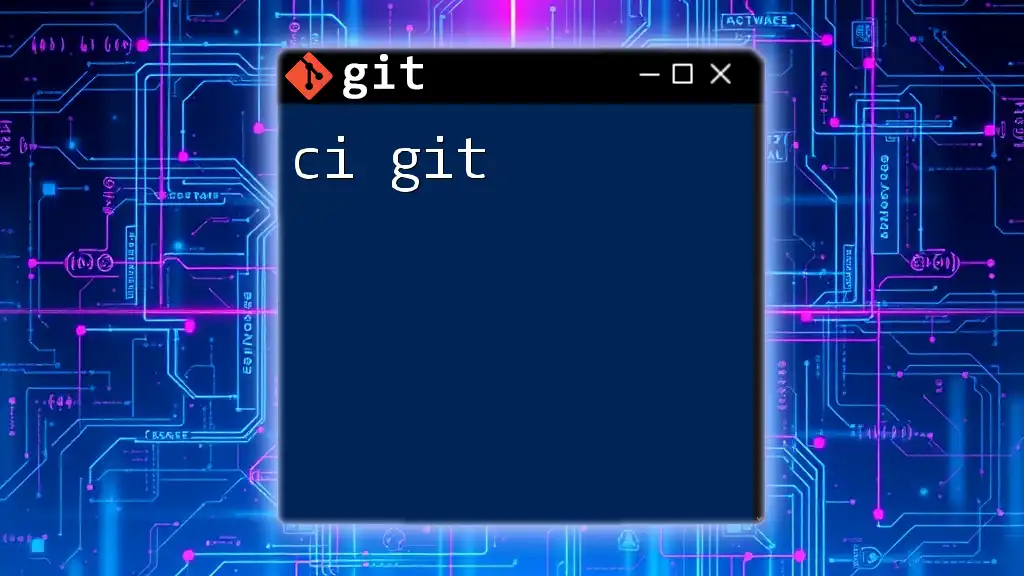
Creating Your First Repository
Creating a Local Repository
Once Git is installed and configured, you can create a local repository. Use the following command to initialize a new repository called `my-repo`:
git init my-repo
This command creates a `.git` directory where Git stores all the version control information for the project.
Cloning a Remote Repository
If you want to contribute to an existing project, you need to clone its remote repository. The command to clone a repository is as follows:
git clone https://github.com/user/repo.git
This command duplicates the entire repository, complete with all its version history, allowing you to work on the project locally.
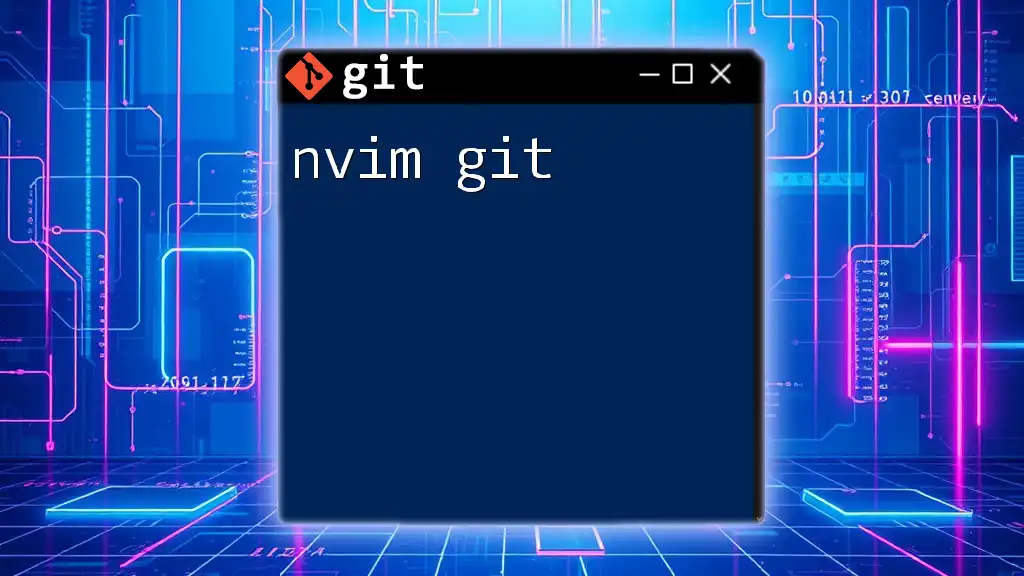
Fundamental Git Commands
Tracking Changes
To manage your project's version history, you must track changes to files using Git. The process involves staging and committing changes.
- Staging files for commit:
git add filename
This command adds changes to the staging area but does not commit them yet.
- Committing changes:
git commit -m "Commit message"
This command saves the staged changes in your repository along with a descriptive message.
Viewing History
To understand the evolution of your project, you’ll need to view the commit history. Use:
git log
This command displays a chronological list of all commits, along with their commit IDs, authors, and messages.
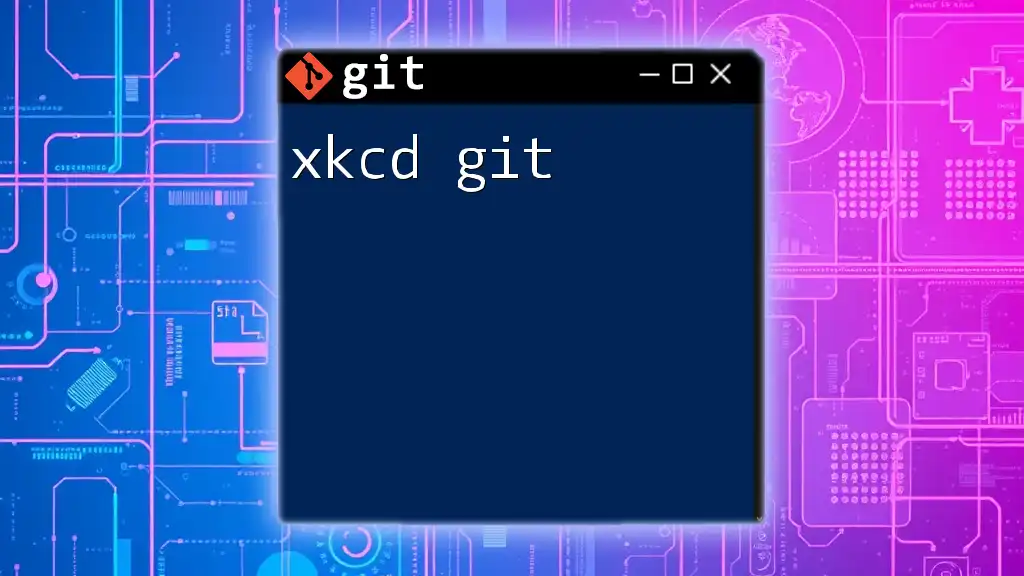
Working with Branches
Understanding Branches
Branches allow you to work independently on different lines of development within the same repository. This is especially useful for developing new features or fixing bugs without affecting the main project.
Creating and Switching Branches
To create a new branch, use:
git branch new-branch
To switch to that branch:
git checkout new-branch
Merging Branches
Once you've completed work on a branch, you can merge it back into the main branch (usually called `master` or `main`). While merging, you may sometimes encounter conflicts. This can occur when changes are made on the same lines in both branches. To merge branches, use:
git merge another-branch
If conflicts arise, Git will notify you, and you will need to resolve them before completing the merge.
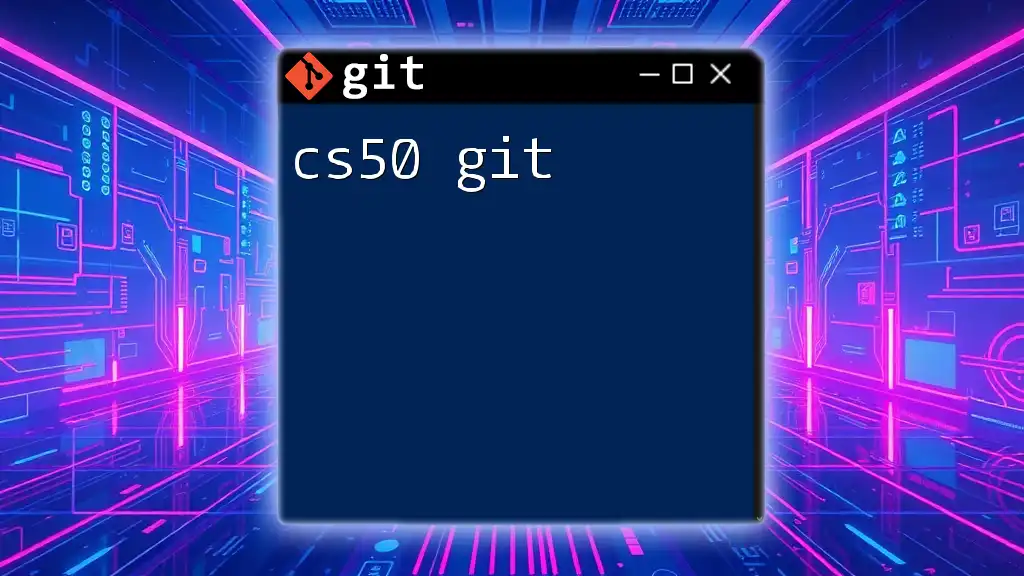
Remote Repositories and Collaboration
Working with Remote Repositories
Remote repositories are key for collaboration, allowing multiple developers to work on the same project. Understanding how to interact with these repositories is crucial for effective teamwork.
Common Remote Commands
Once you have content in your local repository, you’ll often need to push changes to the remote repository. Use this command to push your changes:
git push origin branch-name
To sync your local repository with updates from others, use:
git fetch origin
git pull origin master
Fetching simply downloads changes without integrating them, while pulling merges the downloaded changes into your current branch.
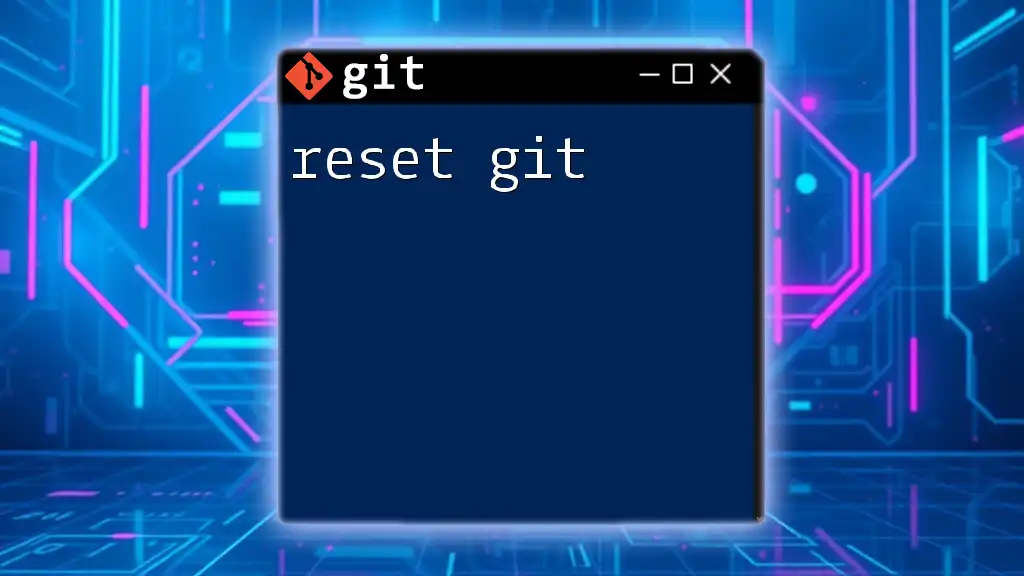
Managing Conflicts
Understanding Merge Conflicts
Merge conflicts arise when two branches have competing changes on the same lines of code. Such situations are inevitable when collaborating with others, and it’s essential to understand how to navigate these conflicts.
Resolving Conflicts
To resolve a merge conflict, follow these steps:
-
Identify conflicting files using:
git status
-
Edit the conflicting files to resolve disagreements between changes.
-
Once conflicts are resolved, mark the files as resolved:
git add filename
-
Finally, complete the merge with:
git commit
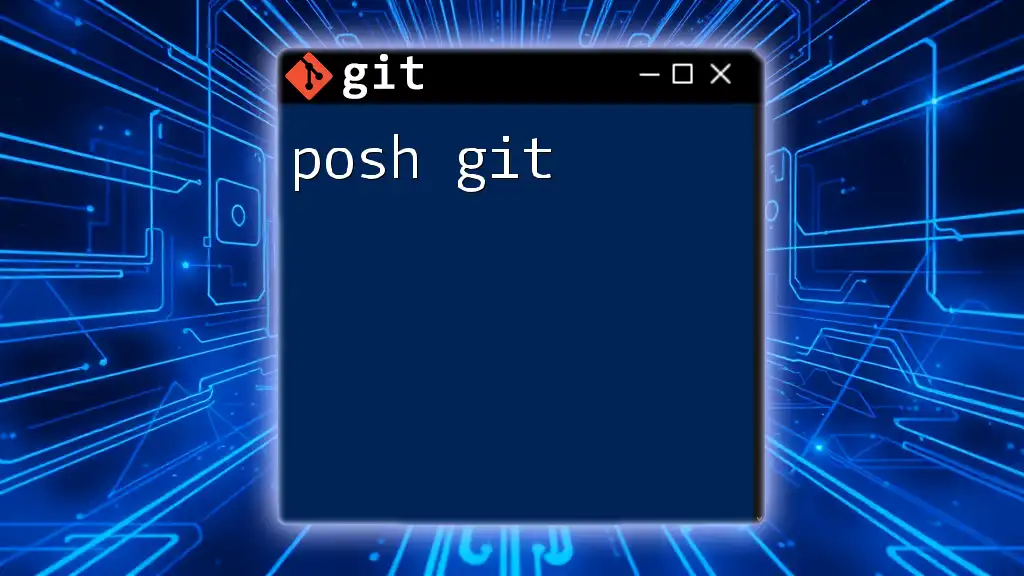
Best Practices for Using Git
Commit Messages Best Practices
Good commit messages are essential for clear project history. A well-structured commit message typically includes a brief summary of changes, followed by a more detailed explanation if necessary. Example:
Fix user login issue
- Updated authentication mechanism to improve user experience
- Refactored login page for better performance
Branch Naming Conventions
When creating branches, it's beneficial to adopt a naming convention that provides context about the changes. Common practices include using prefixes like `feature/`, `bugfix/`, or `hotfix/` to categorize your branches effectively.
Using Tags for Releases
Tags are useful for marking specific points in your project’s history, typically used for releases. To create a tag, use the following command:
git tag -a v1.0 -m "Version 1.0"
Tags help you easily reference and release particular versions of your software.
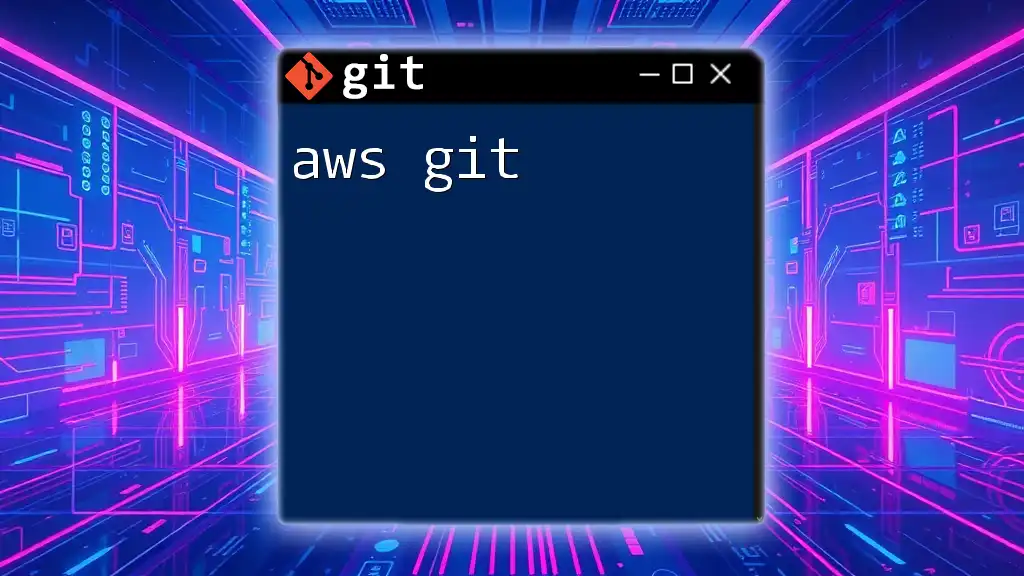
Conclusion
In this guide, we've explored the fundamental aspects of SCM Git, from its basic setup through the complexities of collaboration and conflict resolution. By understanding and leveraging these concepts, you're now better equipped to handle version control in your software projects. Start practicing these commands and techniques to master Git, and you'll soon appreciate the immense power and flexibility it offers for software development!