AWS Git refers to the integration of Git version control with Amazon Web Services, allowing developers to manage code repositories and deploy applications seamlessly within the AWS ecosystem.
Here’s a simple command to clone a Git repository hosted on AWS CodeCommit:
git clone https://git-codecommit.us-east-1.amazonaws.com/v1/repos/MyRepo
Understanding Git Basics
What is Git?
Git is a distributed version control system that facilitates collaborative software development. It allows multiple developers to work on a project simultaneously without overwriting each other's changes. By maintaining a history of changes, Git enables teams to track and manage their code effectively.
Key Git Concepts
-
Repositories: At the core of Git's functionality is the repository (repo). A repository is a storage space where your project files and their version history reside. Repos can be hosted locally on your computer or remotely on platforms such as AWS CodeCommit.
-
Commits: A commit is a snapshot of changes made to your project at a given point in time. Each commit is identified by a unique SHA hash, ensuring that all modifications can be traced back to their origin. Writing clear and informative commit messages is crucial for maintaining a readable project history.
-
Branches: Branches are an essential feature of Git that allow you to diverge from the main line of development without affecting the production code. Creating separate branches for different features or bug fixes enables streamlined workflows and encourages experimentation without disrupting the main project.
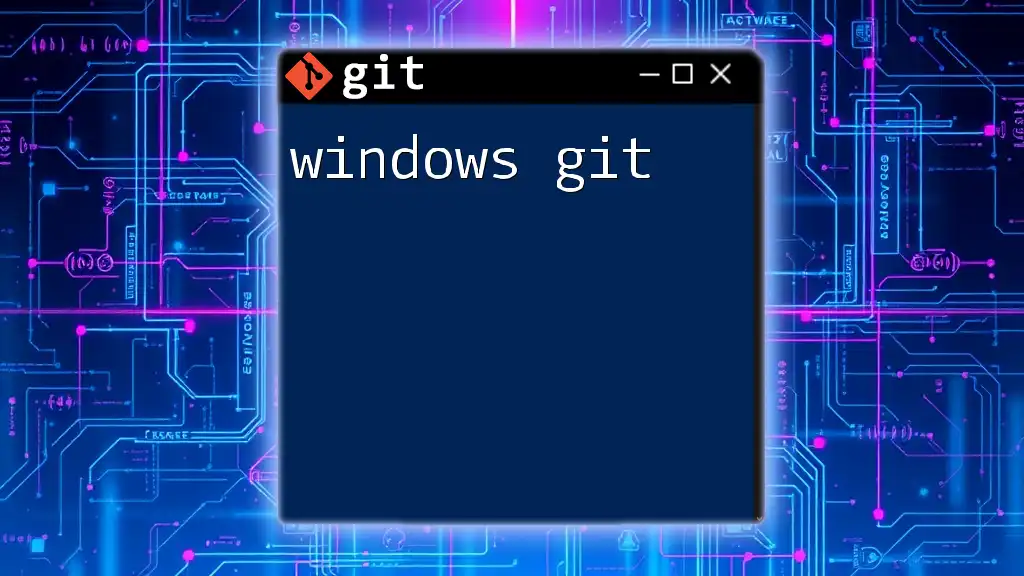
Setting Up AWS for Git
Prerequisites
Before diving into AWS Git, ensure you have the following:
-
AWS Account: To access AWS services, you'll need to create an AWS account. This process is straightforward; visit the AWS website and sign up.
-
Git Installation: Git installation varies by operating system. Follow the instructions for your chosen platform:
- Windows: Download and install Git from the official Git website.
- Mac: Use Homebrew to install Git with the command:
brew install git
- Linux: Most distributions offer Git in their package repositories. Install it using the command:
sudo apt-get install git
Creating an AWS CodeCommit Repository
What is AWS CodeCommit?
AWS CodeCommit is a fully managed source control service that hosts secure Git repositories. It integrates seamlessly with other AWS services, providing a robust system for version control and collaboration.
Step-by-Step Guide
Creating Your First Repository: Follow these steps to create a repository in AWS CodeCommit:
- Sign in to the AWS Management Console and navigate to the CodeCommit service.
- Click “Create repository.”
- Enter a name and optional description for your repository.
- Review the settings, and click “Create.”
Once your repository is created, you can clone it to your local machine.
Cloning the Repository: To clone your newly created repository, use the following command in your terminal:
git clone https://git-codecommit.us-east-1.amazonaws.com/v1/repos/YourRepoName
This command creates a local copy of your AWS CodeCommit repository on your machine, allowing you to start working with it immediately.
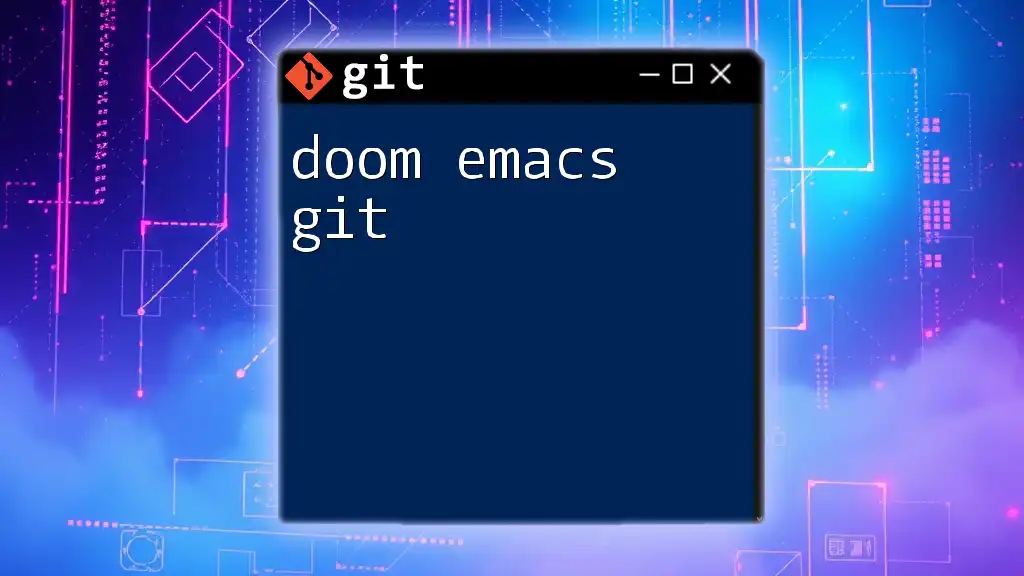
Common AWS Git Commands
Basic Commands
-
Adding Changes: To stage your changes, use:
git add .
This command stages all changes in your working directory.
-
Committing Changes: After staging, commit your changes with a meaningful message:
git commit -m "Your commit message"
Ensure your commit messages are informative; they help others understand the context of your changes.
Working with Branches
- Creating a Branch: To create a new branch, use the following command:
This command creates and switches to a new branch named "new-branch", enabling you to work on new features without affecting the main branch.git checkout -b new-branch
Pushing and Pulling
-
Pushing Code to AWS CodeCommit: To push your local changes back to AWS CodeCommit, use:
git push origin branch-name
Replace "branch-name" with the name of the branch you want to push. This command updates the remote repository with your local changes.
-
Pulling Changes from AWS CodeCommit: To fetch and merge changes from the remote repository into your local branch, execute:
git pull origin branch-name
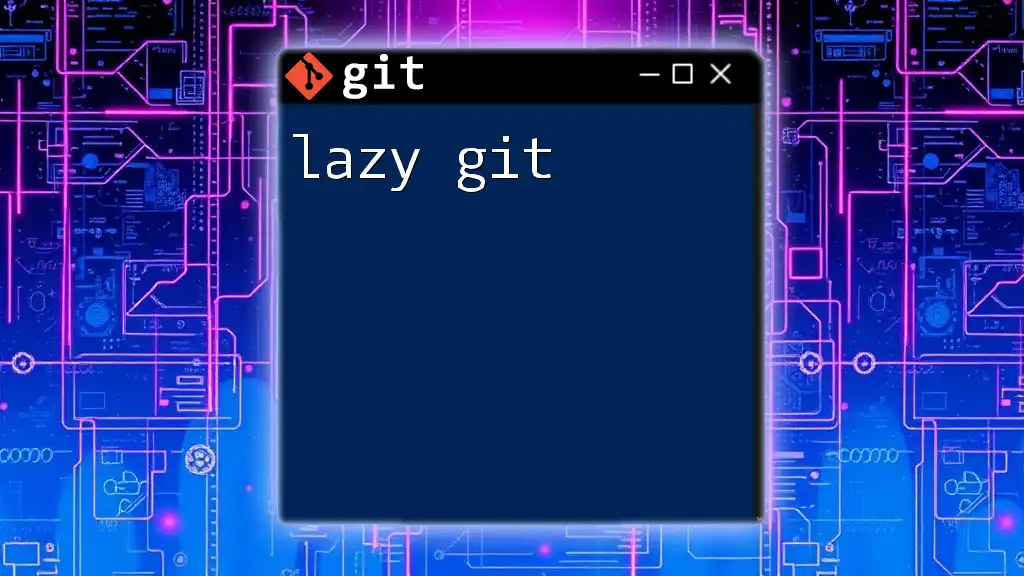
Integrating AWS Git with Other AWS Services
Using AWS CodePipeline
Overview of Continuous Integration/Continuous Deployment (CI/CD): CI/CD is an essential practice for automating the software delivery process. It allows developers to deploy code more efficiently and ensures high-quality software release.
Setting Up a Pipeline with CodeCommit
To create a CI/CD pipeline that integrates with AWS CodeCommit, follow these steps:
- In the AWS Management Console, navigate to CodePipeline.
- Click "Create Pipeline" and follow the prompts to define your pipeline settings.
- Select CodeCommit as your source provider and choose your repository.
- Configure additional stages — such as build and deploy — per your project needs.
Example Use Case
Imagine you are developing a web application. By setting up a CodePipeline that listens for changes in your CodeCommit repository, every time you push code, the pipeline will trigger, build the application, run tests, and deploy it to your AWS environment. This automation minimizes manual tasks and reduces the risk of human error.
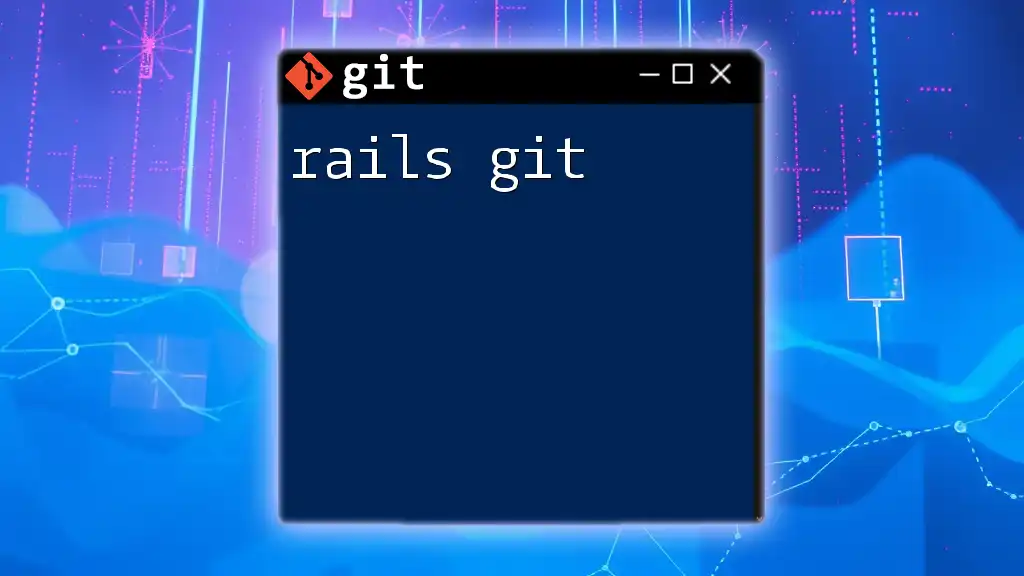
Best Practices for Using AWS Git
Commit Message Guidelines
Writing effective commit messages is a vital aspect of maintaining a clean project history. A good commit message should:
- Clearly describe the changes made.
- Use the imperative mood (e.g., "Fix bug" instead of "Fixed bug").
- Reference issue numbers or related requests, if applicable.
Branching Strategies
Choosing the right branching model enables teams to work efficiently. Common strategies include:
- Git Flow: A well-defined strategy including feature branches, releases, and hotfixes.
- Feature Branching: Creating separate branches for individual features.
Consider your team's workflows and select a branching model that minimizes conflicts and enhances collaboration.
Security Considerations
IAM Roles and Permissions: Use AWS Identity and Access Management (IAM) to configure permissions for accessing CodeCommit. A well-structured IAM policy ensures that only authorized users can access your repositories, adding an essential layer of security to your development process.
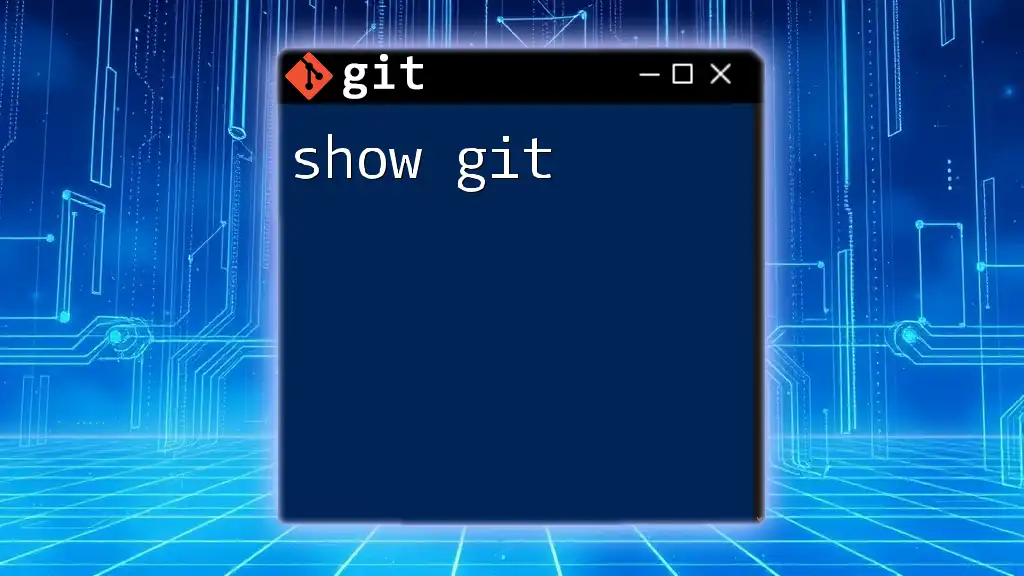
Common Challenges and Solutions
Troubleshooting Push/Pull Issues
Common errors you may encounter while pushing or pulling from your AWS CodeCommit repository include authentication failures and merge conflicts. Address these issues by ensuring your AWS CLI is properly configured and reviewing merge conflict resolutions.
Integrating Local Development with AWS Git
Managing your local Git repository in conjunction with AWS CodeCommit requires disciplined workflows. Regularly merge changes from the main branch into your feature branches to minimize conflicts. Keeping your local repository in sync with CodeCommit ensures smooth collaboration.
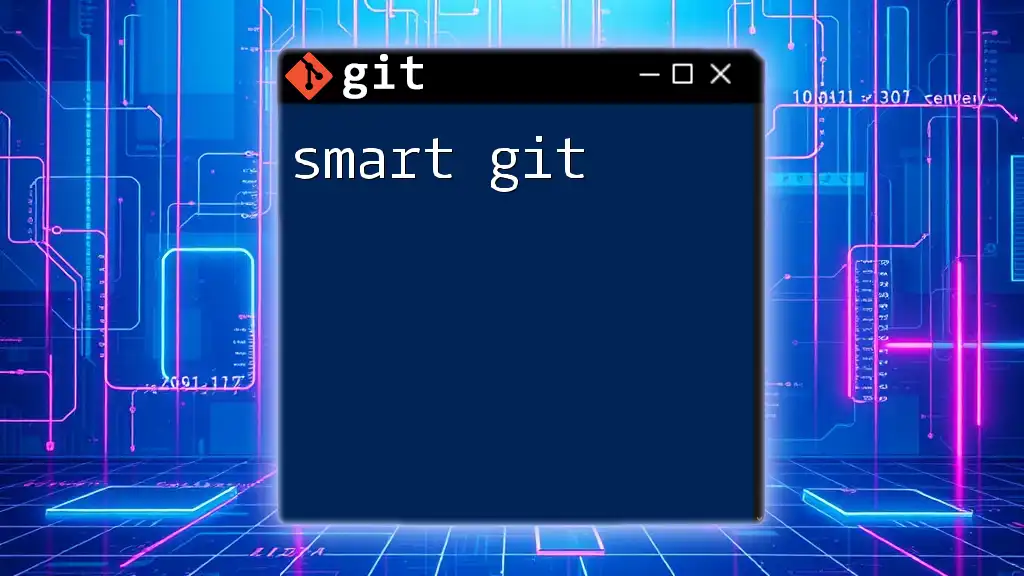
Conclusion
In summary, understanding how to leverage AWS Git effectively is crucial for streamlined software development. AWS CodeCommit, along with Git commands, serves as a powerful toolset for teams looking to enhance their version control and CI/CD practices. As AWS continues to innovate, staying informed about the evolving integration of AWS and Git will empower you to build and deploy high-quality applications with confidence.
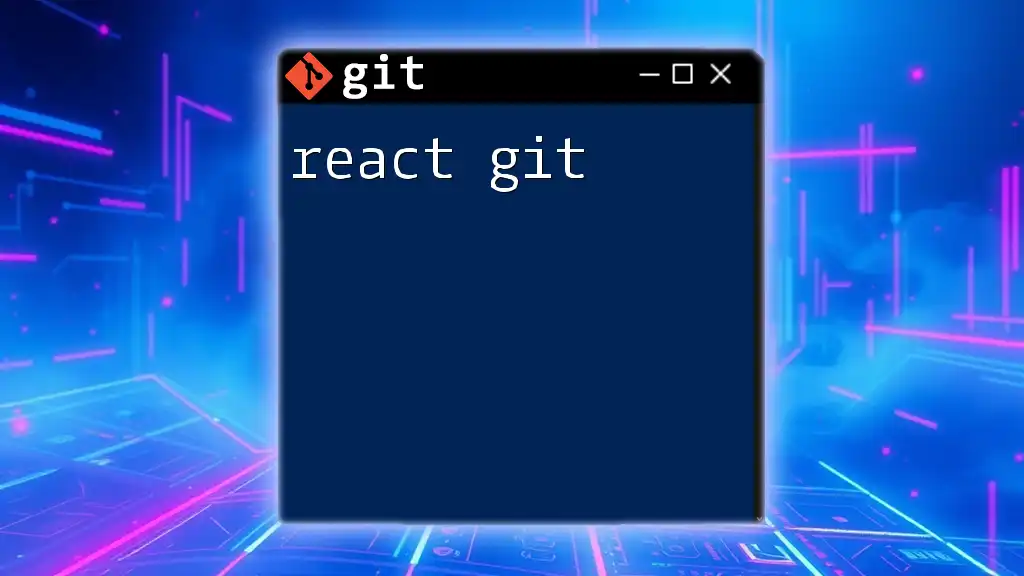
Additional Resources
For more in-depth learning, consider exploring the official AWS CodeCommit documentation, along with various tutorials and blog posts available online.