"Node.js Git" refers to using Git version control to manage Node.js project files and collaborate effectively on code development.
Here’s a simple Git command to initialize a new Node.js project:
git init my-nodejs-project
Understanding Git Basics
What is Git?
Git is a powerful version control system that allows developers to track changes in files and collaborate with others. It provides essential features like branching, merging, and rebasing, which facilitate efficient workflows in both individual and team projects.
The benefits of using Git include:
- Version History: Maintain a history of changes to your code, so you can revert if necessary.
- Collaboration: Multiple developers can work on different features simultaneously without overwriting each other's work.
- Branching: Test new ideas in isolated branches without affecting the main codebase.
Setting Up Git
To start using Git, you need to install it on your machine. Instructions vary depending on your operating system.
Installation steps:
- Windows: Download the Git installer from the official Git website, run the installer, and follow the prompts.
- macOS: Use Homebrew by running `brew install git` in the terminal.
- Linux: Install Git via your package manager, for example, with `sudo apt-get install git` for Ubuntu.
Once Git is installed, configure it with your user name and email:
git config --global user.name "Your Name"
git config --global user.email "youremail@example.com"
Essential Git Commands
Familiarize yourself with these essential commands to effectively manage your Node.js projects with Git:
- `git init`: This command initializes a new Git repository in your current directory.
- `git clone`: Use this command to create a local copy of an existing repository.
- `git add`: Stages file changes for the next commit. You can add individual files or all changes with `.`.
- `git commit`: Saves your changes to the local repository with a descriptive message.
- `git push`: Uploads your committed changes to a remote repository.
- `git pull`: Fetches and merges changes from a remote repository into your current branch.
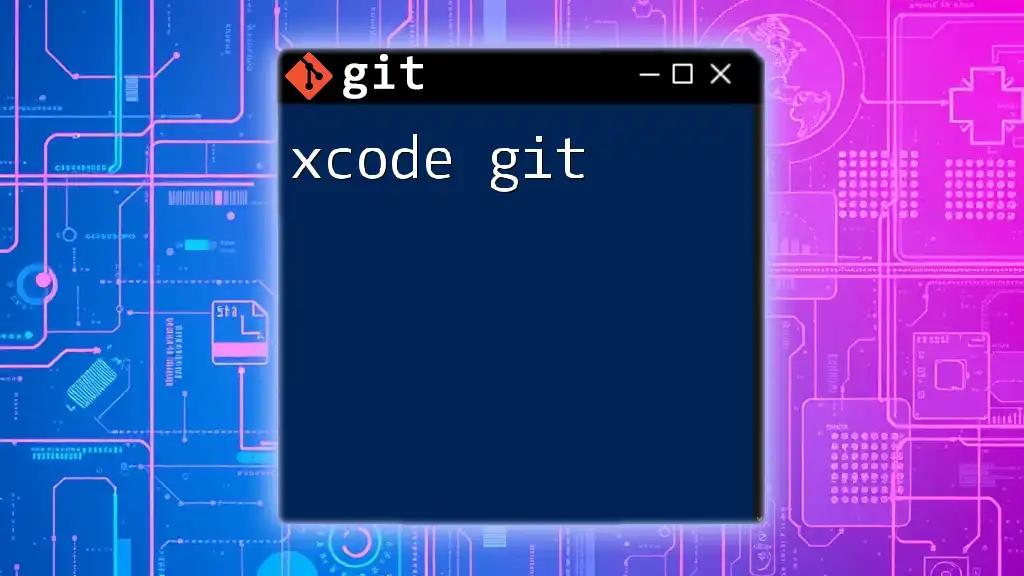
Integrating Git with Node.js Projects
Initializing a Node.js Project with Git
To start a new Node.js project and integrate Git, follow these steps:
- Create a new directory for your project and navigate into it:
mkdir my-node-app
cd my-node-app
- Initialize a new Node.js project with the following:
npm init -y
- Finally, initialize a Git repository in the project folder:
git init
At this point, you have a basic Node.js setup with Git ready to track your changes.
Creating a .gitignore File
Not all files should be tracked by Git. For a Node.js project, it's crucial to exclude certain files and directories that could clutter your repository or expose sensitive information.
Create a `.gitignore` file in your project directory and include the following lines:
node_modules/
npm-debug.log
.env
This configuration ensures that node_modules (which can be recreated with `npm install`), log files, and environment variables remain unstaged and hidden from your repository.
Committing Changes in a Node.js Project
When you're ready to save your progress, you need to stage and commit your changes.
- Stage all modified files:
git add .
- Commit the changes with a meaningful message that describes what you've done:
git commit -m "Initial commit: Set up project with basic structure"
This practice provides a clear history of changes and makes it easier to understand what was done at each stage.
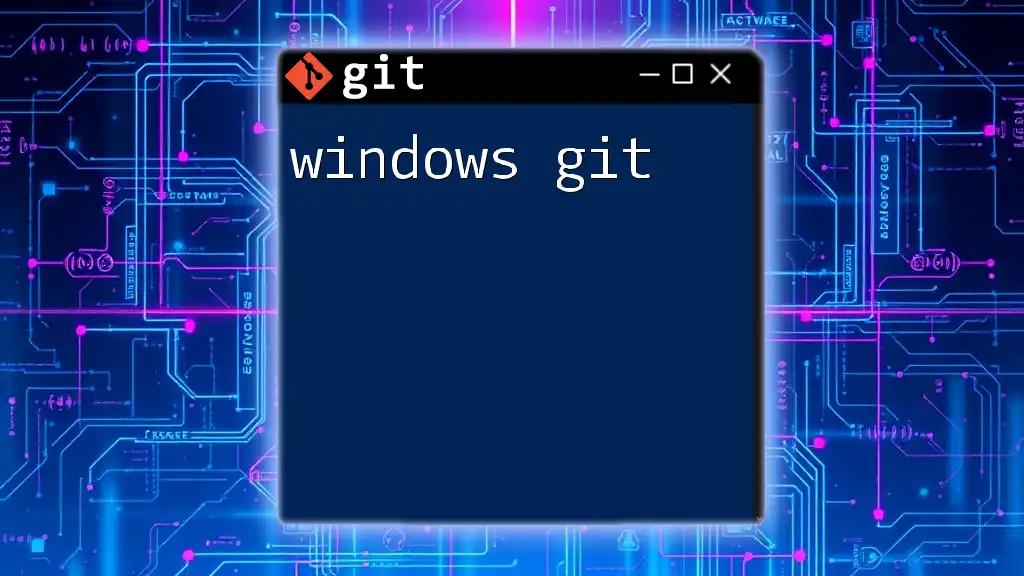
Branching in Git
What is Branching?
Branching in Git allows developers to diverge from the main code line and work independently on features, bug fixes, or experiments. This flexibility is essential when working in teams or managing complex projects.
Creating and Merging Branches
Creating a branch is straightforward. For example, when you're ready to develop a new feature, you can create a branch like this:
git checkout -b feature/new-feature
After developing your feature and committing the changes, you'll want to merge these changes back into the main branch. Switch back to the main branch and run:
git checkout main
git merge feature/new-feature
This command incorporates the changes from your feature branch into your main codebase.
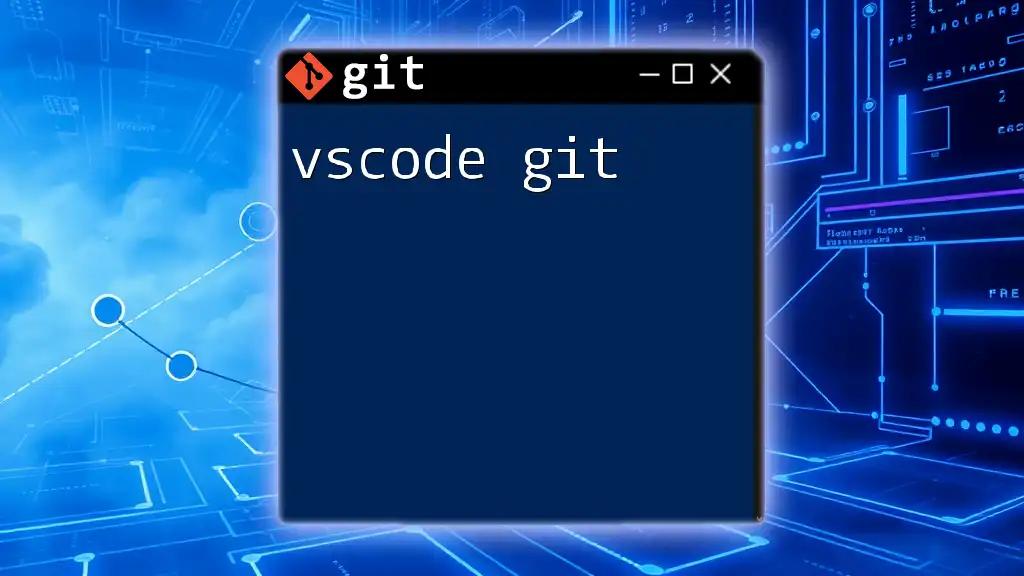
Collaboration with Git
Working with Remote Repositories
To collaborate with others on a Node.js project, you'll need a remote repository. Common platforms include GitHub and GitLab. Here's how to set up a remote repository after initializing your local repository:
git remote add origin https://github.com/username/my-node-app.git
Collaborating with Team Members
Team collaboration is enhanced using tools like pull requests and code reviews. When a team member wants to incorporate changes into the main branch, they create a pull request in the remote repository, prompting others for review.
Resolving Merge Conflicts
Merge conflicts occur when multiple changes are made to the same line of a file. Here’s how to deal with them:
- Identify conflicts: After merging, run:
git status
This shows you which files have conflicts.
- Use a merge tool: You can open a merge tool to resolve conflicts interactively:
git mergetool
Resolving conflicts is crucial to maintaining a coherent codebase.
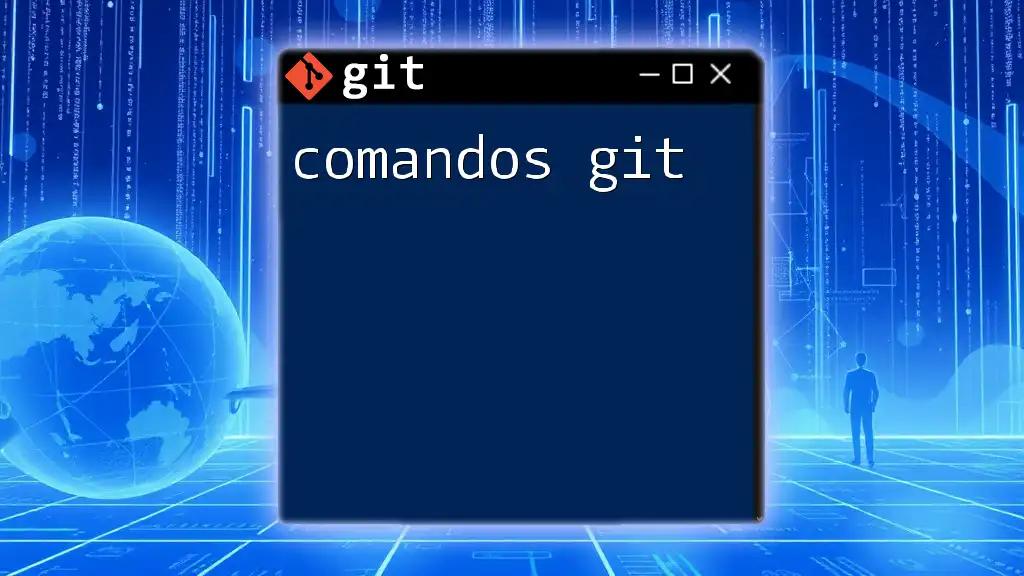
Best Practices for Using Git with Node.js
Writing Clear Commit Messages
Clear commit messages not only help you remember what changes were made but also assist others in understanding the progress of the project. For example, instead of writing "fixed bug," say "fixed null reference error in user authentication."
Keeping Your Repository Clean
Regularly tidy up your repository by deleting unused branches and ensuring your main branch remains stable. A clean repository improves collaboration and minimizes confusion.
Using Tags for Versioning
Tags help in marking specific points in your project’s history, such as releases. You can create a tagged version with the command:
git tag v1.0.0
This practice is beneficial for maintaining version control and deployment.
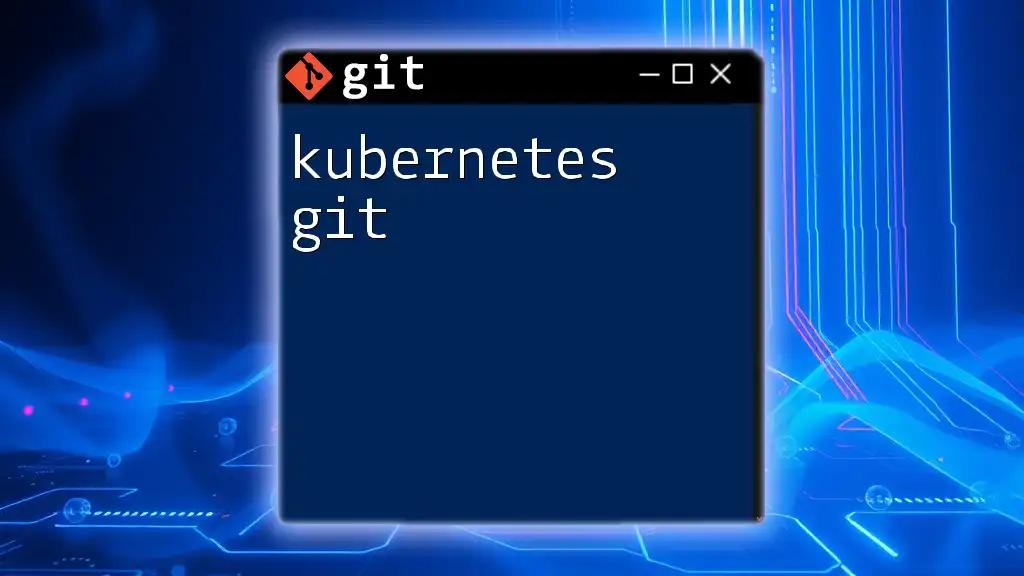
Troubleshooting Common Git Issues in Node.js
Common Errors and Solutions
One common issue developers face is merge conflicts, which can be resolved by carefully reviewing the affected files and using `git mergetool`. Another common error is a detached HEAD state, which occurs when you checkout a specific commit without being on a branch. To resolve this, simply create a new branch from that state.
Utilizing Git Documentation and Resources
Familiarize yourself with the official [Git documentation](https://git-scm.com/doc) and [Node.js resources](https://nodejs.org/en/docs/) to deepen your understanding of both tools.
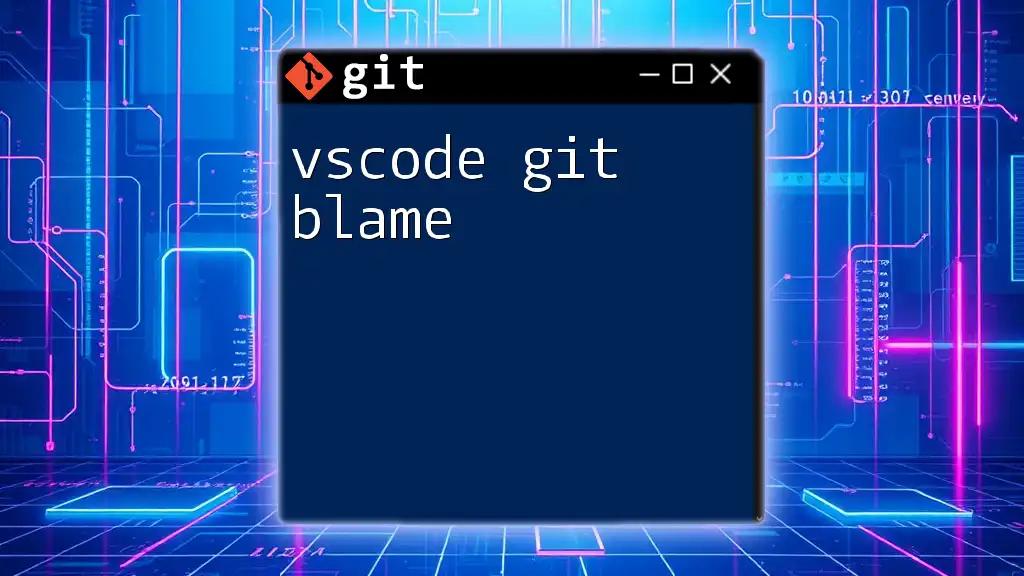
Conclusion
Mastering Node.js Git is essential for effective project management and collaboration in software development. By understanding core Git commands, setting up your Node.js projects correctly, and adhering to best practices, you will enhance your development workflow and contribute more efficiently to team projects. Continue practicing Git commands in your projects and explore further learning resources to refine your skills. We're excited for you to embark on this journey!