SSH (Secure Shell) in Git allows you to securely connect to remote repositories and perform operations like cloning and pushing changes without needing to enter your username and password each time.
Here's a basic command to clone a Git repository using SSH:
git clone git@github.com:username/repository.git
What is SSH?
SSH, or Secure Shell, is a cryptographic network protocol that enables secure data communication between computers. It allows users to securely access and manage devices over unsecured networks. One of the primary benefits of SSH is that it offers strong encryption, ensuring that even if data packets are intercepted, they cannot be easily read. This makes SSH indispensable for developers and system administrators who often need to connect to remote servers securely.
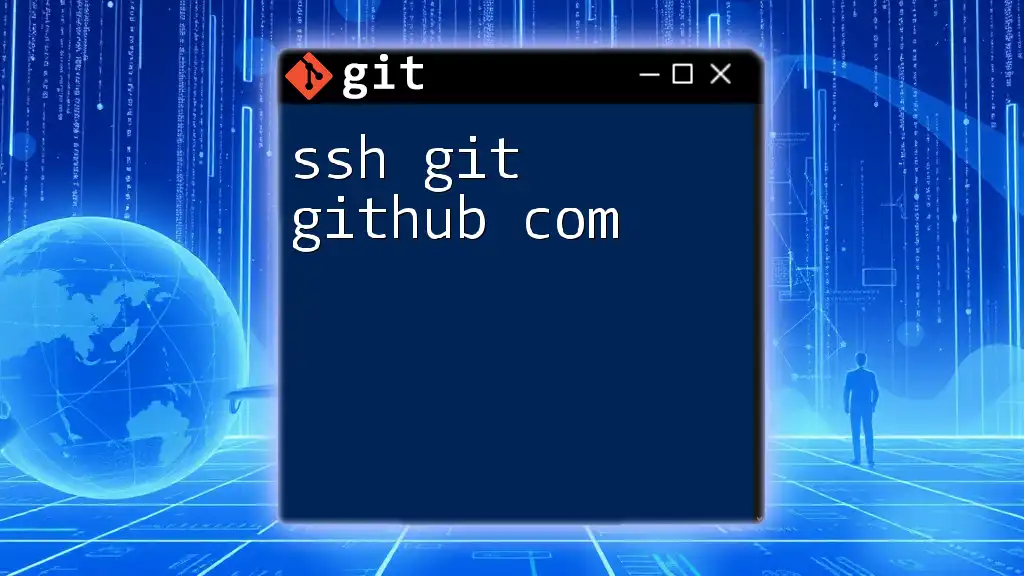
Why Use SSH with Git?
Using SSH with Git provides enhanced security for your repositories and makes interactions with remote servers smooth and efficient. When you use SSH keys for authentication instead of standard username/password combinations, you minimize the risk of password theft. Additionally, SSH simplifies workflows through seamless access to your projects without repeatedly entering credentials. With SSH, you can manage your repositories while knowing your data is transmitted securely.
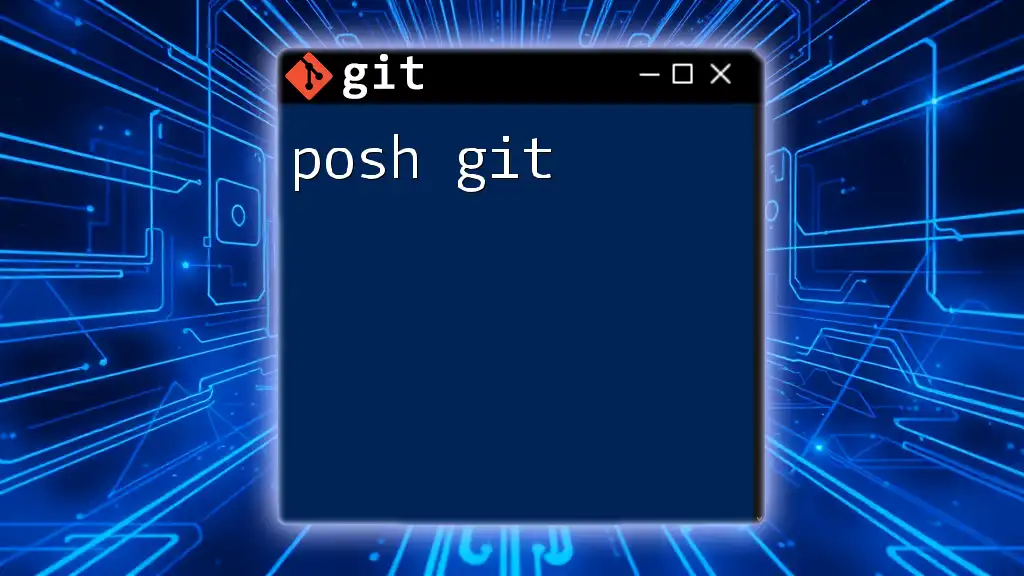
Setting Up SSH for Git
Generating an SSH Key
To start using SSH with Git, you first need an SSH key pair—comprising a public key and a private key. The public key is shared with services like GitHub, GitLab, or Bitbucket, while the private key remains on your local machine.
To generate an SSH key, execute the following command in your terminal:
ssh-keygen -t rsa -b 4096 -C "your_email@example.com"
Explanation of the command:
- `ssh-keygen`: The command for generating the SSH key.
- `-t rsa`: Specifies the type of key to create, RSA is a widely used method.
- `-b 4096`: Sets the bit length of the key; 4096 bits is recommended for security.
- `-C "your_email@example.com"`: Adds a label to your key for identification purposes.
When prompted, choose a file location (or press Enter to accept the default), and set a passphrase for added security if desired.
Adding the SSH Key to the SSH Agent
Once your SSH key is generated, you should add it to the SSH agent, which manages your keys and allows you to use them without having to enter your passphrase every time.
First, start the SSH agent with the following command:
eval "$(ssh-agent -s)"
Then, add your SSH private key to the agent:
ssh-add ~/.ssh/id_rsa
Explanation of the commands:
- `eval "$(ssh-agent -s)"`: Initializes the SSH agent in the current shell session.
- `ssh-add ~/.ssh/id_rsa`: Adds your SSH private key to the agent, allowing it to manage and use it securely.
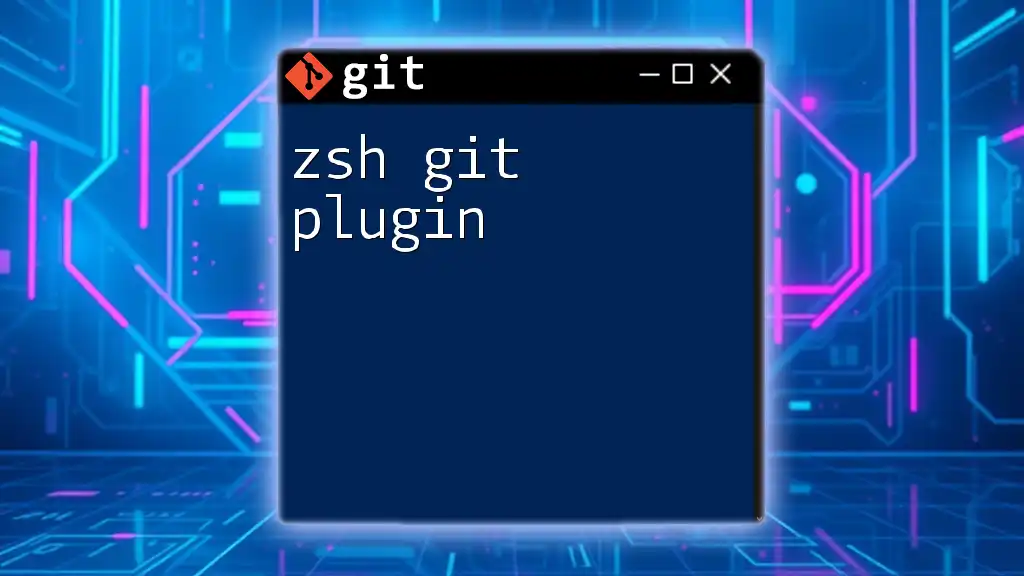
Configuring SSH for Git Hosting Services
GitHub
To use your SSH key with GitHub, you must add the public key to your GitHub account. First, display your public key using:
cat ~/.ssh/id_rsa.pub
Copy the displayed key, then log in to your GitHub account. Navigate to Settings > SSH and GPG keys and click on New SSH key. In the title field, you can use any descriptor (such as "My Laptop"), paste your public key, and hit Add SSH key.
GitLab
Similar to GitHub, you’ll need to add your SSH key to your GitLab account. Again, use the command to view your public key:
cat ~/.ssh/id_rsa.pub
Log in to GitLab, go to Settings > SSH Keys, and paste your public key into the designated field. Click on Add key to complete the process.
Bitbucket
For Bitbucket, the process is nearly the same. Copy your public key just like before and log in to your Bitbucket account. Navigate to Personal settings > SSH keys, then click on Add key, and paste your public key. Finally, confirm by hitting Save.
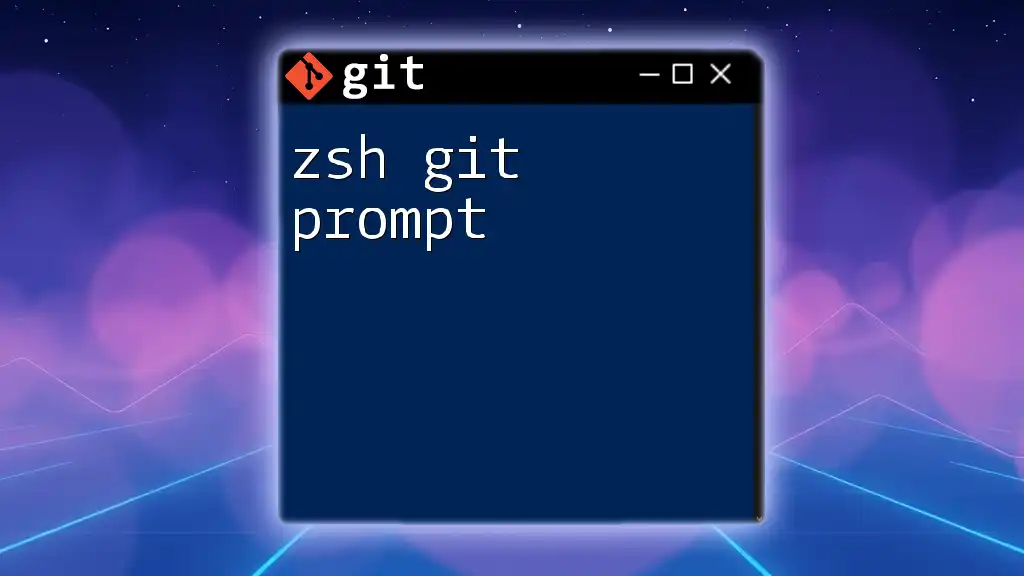
Testing Your SSH Connection
After configuring SSH with your Git hosting service, it’s crucial to verify that everything works as expected. To test your connection to GitHub, for instance, simply run:
ssh -T git@github.com
If this is your first time connecting, you may be prompted to confirm the authenticity of the host. Type "yes" to continue. A successful message such as "Hi username! You've successfully authenticated, but GitHub does not provide shell access." confirms that your SSH setup is functional.
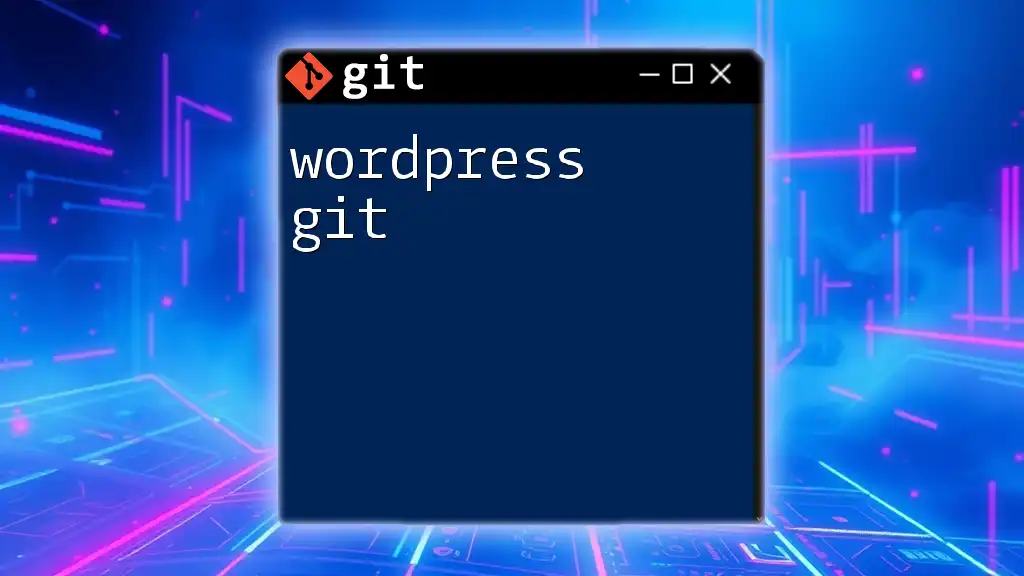
Using SSH with Git Commands
Cloning a Repository
To clone a repository using SSH, you’d use the SSH URL provided by the hosting service. An example command looks like this:
git clone git@github.com:username/repository.git
Replace `username` and `repository` with your actual GitHub username and repository name.
Pushing Changes
When you push changes to your repository, you’ll work through the usual Git commands. Here’s how you can prepare and push changes:
git add .
git commit -m "Your commit message"
git push origin main
Explanation of the process:
- `git add .`: Stages all changes in the current directory.
- `git commit -m "Your commit message"`: Commits the staged changes with a descriptive message.
- `git push origin main`: Sends your commits to the `main` branch of the remote repository.
Pulling Changes
To pull changes made by others to your local repository, use:
git pull origin main
This command fetches updates from the remote repository and merges them with your local repository.
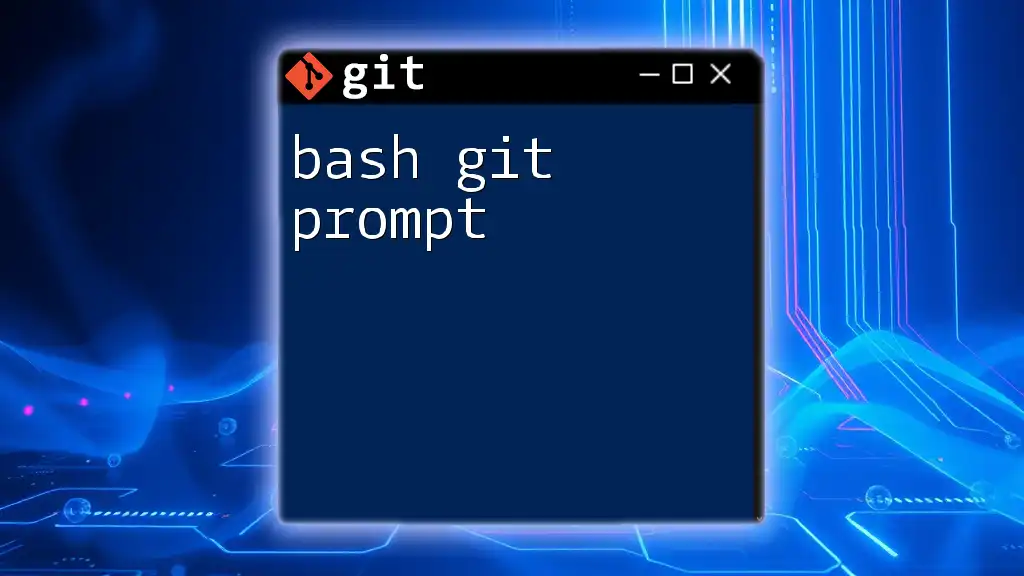
Troubleshooting Common SSH Issues with Git
Authentication Issues
If you encounter authentication issues when using SSH, double-check that you’ve added the correct public key to your hosting service. Also, ensure that your local SSH agent is running and that your key is properly loaded. Running `ssh-add -l` will list loaded keys.
Permission Denied Errors
"Permission denied" messages can occur if your SSH keys are misconfigured or if you're trying to clone/push to a repository without permission. Ensure that the SSH URL is correct, and that your public key is associated with the correct account on the hosting service.
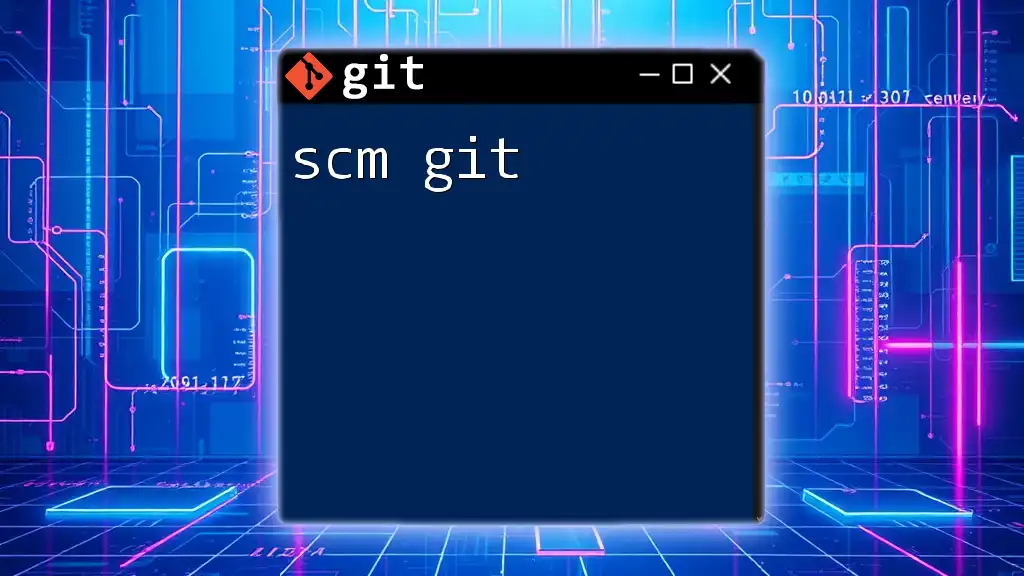
Best Practices for Using SSH with Git
To maintain the best security practices when using SSH with Git, keep your SSH keys secure. Generate strong keys and manage them securely. Regularly rotate your keys to avoid potential security vulnerabilities; changing them every few months is a good practice.
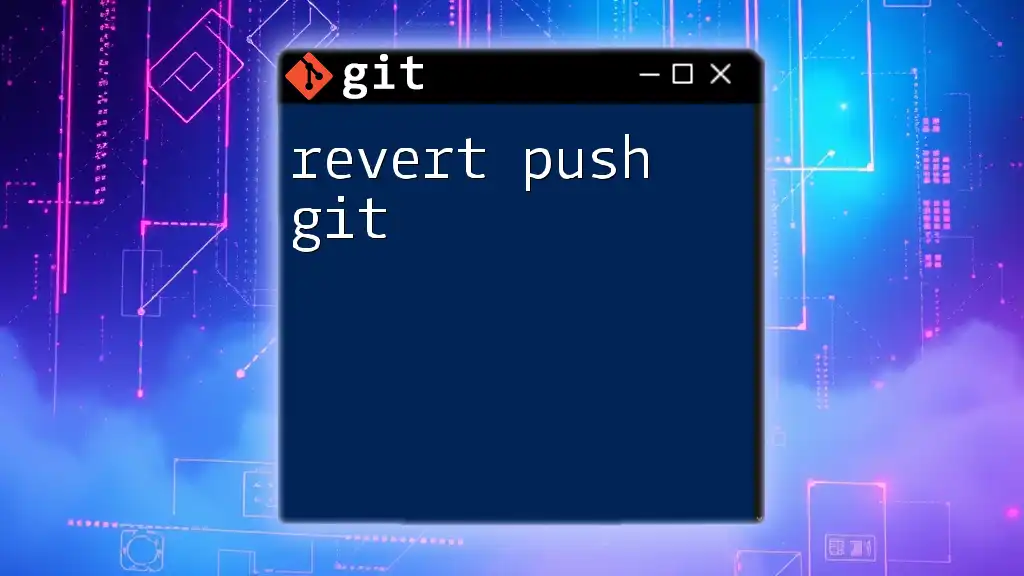
Conclusion
Establishing and using SSH with Git significantly improves your workflow while adding layers of security. Following this guide will not only help you set up SSH correctly but will also aid in troubleshooting common issues. Embracing SSH in your Git practices will ultimately enhance your development experience.
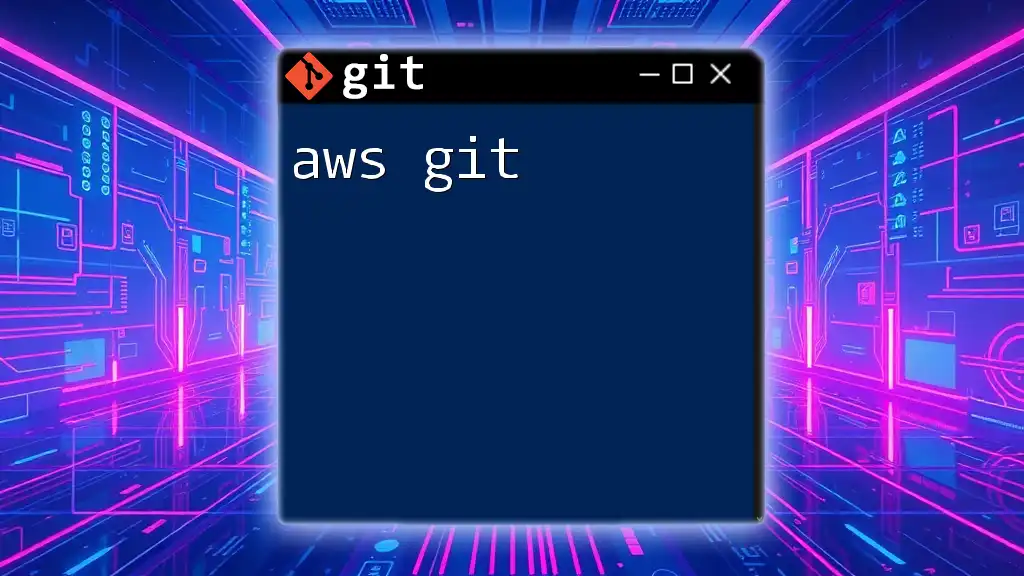
Additional Resources
For further reading on SSH and Git, consider exploring official documentation, online tutorials, and community forums for in-depth knowledge and troubleshooting tips.