Windows Bash Git refers to using Git commands in the Bash shell environment on Windows, allowing users to manage version control seamlessly with concise syntax.
git clone https://github.com/username/repository.git
Setting Up Windows Bash for Git
Installing Git on Windows
To get started with Windows Bash Git, the first step is to install Git on your Windows machine. You can download the latest version from the [official Git website](https://git-scm.com/download/win).
Once you’ve downloaded the installer, follow these steps for a smooth installation:
- Run the downloaded executable and follow the installation prompts.
- Choose your preferred components. It’s generally best to leave the default options selected.
- Select your terminal emulator. Opt for "Git Bash" for an enhanced experience.
- Complete the installation by clicking "Finish".
After installation, it's crucial to configure your Git environment. Executing the following commands in Bash will set your user credentials globally:
git config --global user.name "Your Name"
git config --global user.email "your.email@example.com"
Using Windows Bash
What is Windows Bash?
Windows Bash is a command line interface that enables users to interact with their system in a way similar to Linux. This capability is particularly beneficial for developers who use Git as part of their workflow. It allows for powerful command-line functionalities and script editing.
How to Access Windows Bash
To access Windows Bash, you can launch the Command Prompt by searching for "cmd" in the start menu and typing `bash` to switch over to the Unix-like Bash shell.
Basic Bash Commands for Beginners
Familiarizing yourself with some basic Bash commands will help you navigate and create directories effectively. Common commands include:
- `ls`: List files and directories.
- `cd`: Change directories.
- `mkdir`: Create new directories.
These foundational commands can enhance your file management skills while using Git.
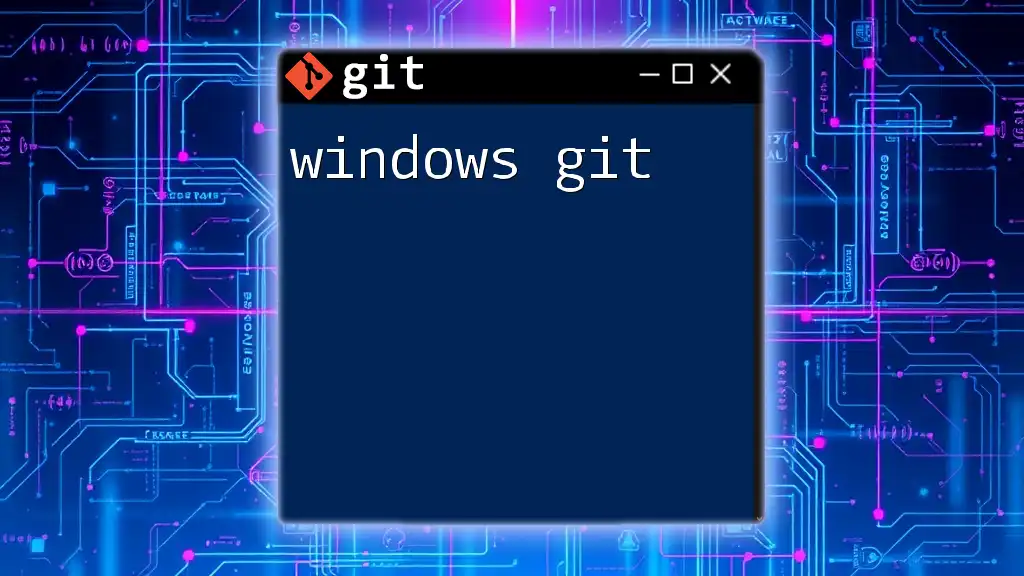
Basic Git Commands in Windows Bash
Cloning a Repository
Cloning a repository is often the first step in collaborating on a project. The command allows you to create a local copy of a remote repository on your machine. Execute the following command in Bash:
git clone https://github.com/username/repo.git
This command not only clones the repository but also sets the remote origin, making it easier to push and fetch changes.
Checking the Status
Before proceeding to commit changes, it’s essential to check the status of your Git repository. The command `git status` will provide detailed information about changed files, untracked files, and the state of your staging area.
git status
Staging Changes
Understanding the Staging Area
The staging area, or index, serves as a buffer before changes are committed to the repository. It's a snapshot of your files at a given time, which lets you control what gets included in your next commit.
Adding Files to Staging
To stage changes, use the `git add` command. You can specify a single file or add all changes at once:
git add filename.txt
git add .
The first command adds a specific file to the staging area, while the second command stages all modified files in the current directory.
Committing Changes
Once you’ve staged your changes, the next step is to commit them. A commit represents a saved snapshot of your project. It's crucial to write a meaningful message explaining the changes you've made.
Use the following command to commit your changes:
git commit -m "Your commit message"
Best Practices for Commit Messages
- Use the imperative mood (e.g., "Add feature" instead of "Added feature" or "Adding feature").
- Be concise yet descriptive.
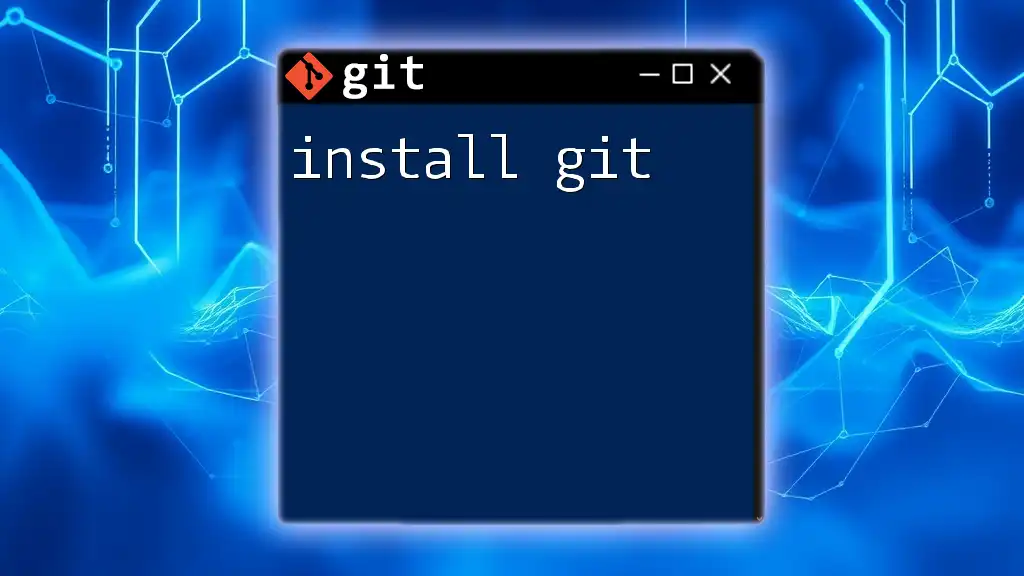
Working with Branches
The Concept of Branching
Branching is at the heart of Git’s power, allowing you to work on different features or fixes concurrently without affecting the main codebase. Each branch represents an isolated environment where you can make changes.
Creating and Switching Branches
Creating new branches is straightforward. Use the following command:
git branch branch-name
After creating a branch, you can switch to it with:
git checkout branch-name
Alternatively, Git offers a simplified command to switch branches:
git switch branch-name
This command is especially useful for users transitioning from different Git workflows.
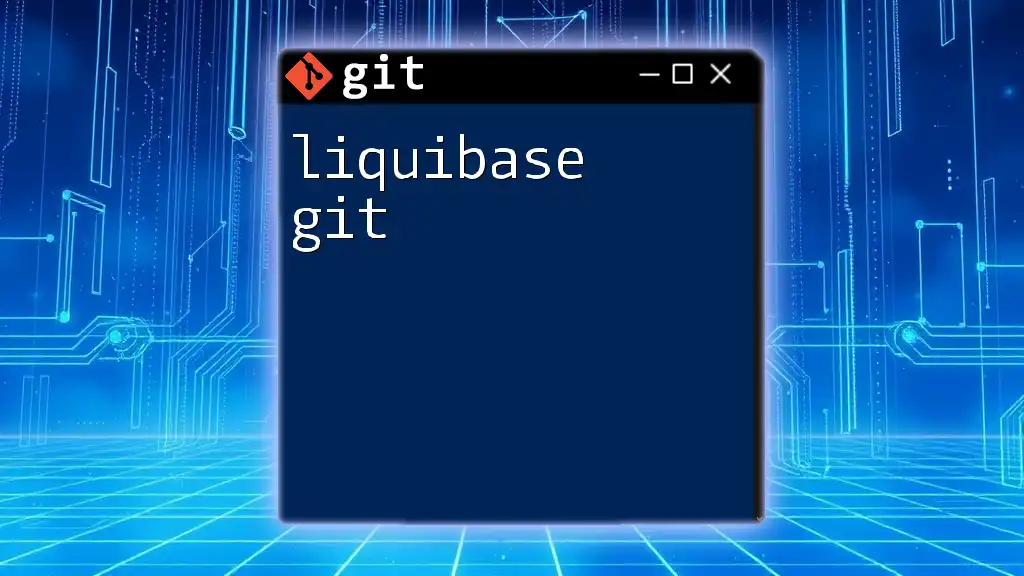
Merging and Resolving Conflicts
Merging Branches
Once your work in a branch is complete, merging allows you to integrate those changes back into the main branch (usually `master`). Use the following command:
git merge branch-name
This command combines the histories of the merged branches.
Handling Merge Conflicts
Merge conflicts arise when changes in one branch contradict those in another. It requires careful resolution to ensure code integrity.
- Open the files that have conflicts.
- Manually edit the sections indicated by Git.
- After resolving, you need to stage the resolved files:
git add resolved-file.txt
- Finally, commit the changes:
git commit -m "Resolved merge conflict"
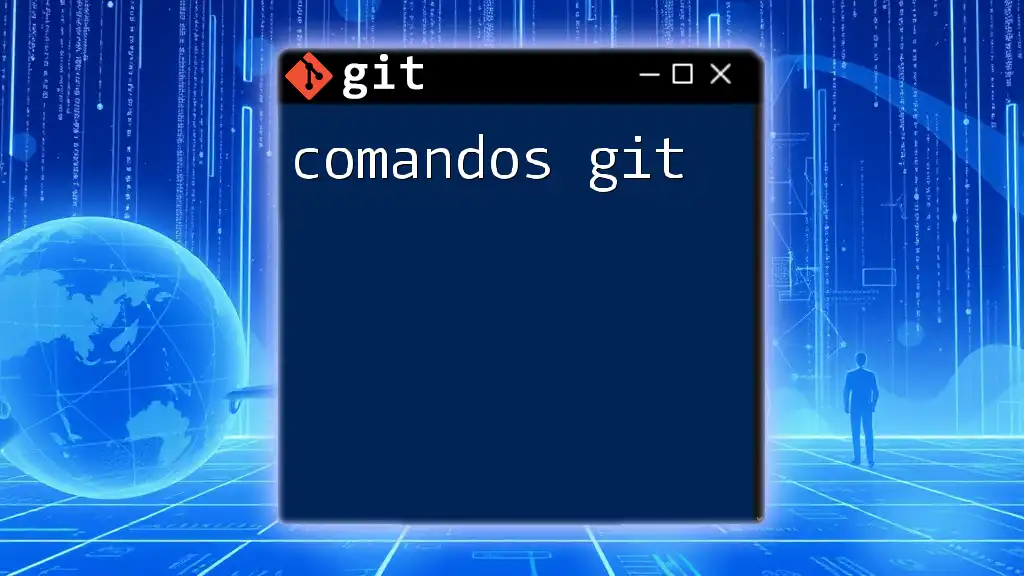
Remote Repositories and Collaboration
Adding a Remote Repository
To connect your local Git repository with a remote server, you need to define the remote repository URL:
git remote add origin https://github.com/username/repo.git
This command establishes a relationship between your local and remote repositories.
Pushing Changes to Remote
After committing your changes, you can share your commits with others by pushing them to the remote repository:
git push origin master
Pulling Changes from Remote
As collaborators work on the same project, it's vital to synchronize your local repository with changes from the remote. You can pull changes from the remote repository using:
git pull origin master
This command fetches and merges changes in one go.
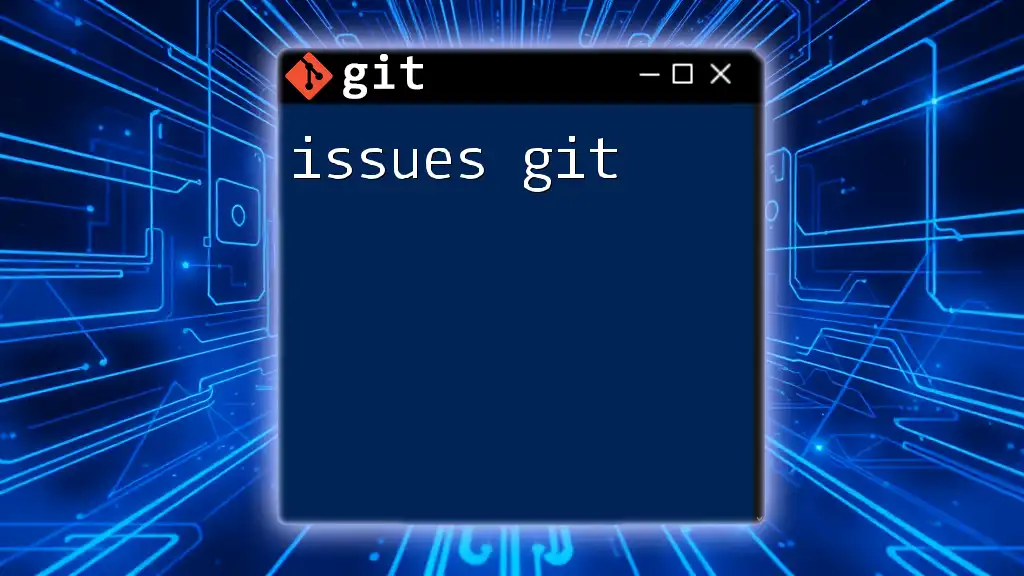
Advanced Git Commands in Windows Bash
Viewing Git History
Knowing how to view your project’s history is crucial for understanding past changes. You can use:
git log
This command displays a detailed log of commits made in the repository, including commit hashes, authorship, and timestamps.
Reverting Changes
Should you need to undo changes, Git provides powerful commands:
To discard local changes in a specific file, use:
git checkout -- filename.txt
If you need to revert to a previous commit, utilize:
git revert commit-hash
This command creates a new commit that reverts changes made by a specific commit, thereby preserving project history.
Stashing Changes
In cases where you need to switch branches but aren’t ready to commit changes, stashing allows you to temporarily save modifications:
git stash save "stash message"
When you're ready to restore the stashed changes, run:
git stash pop
This feature is incredibly useful for keeping your working directory clean without losing progress.
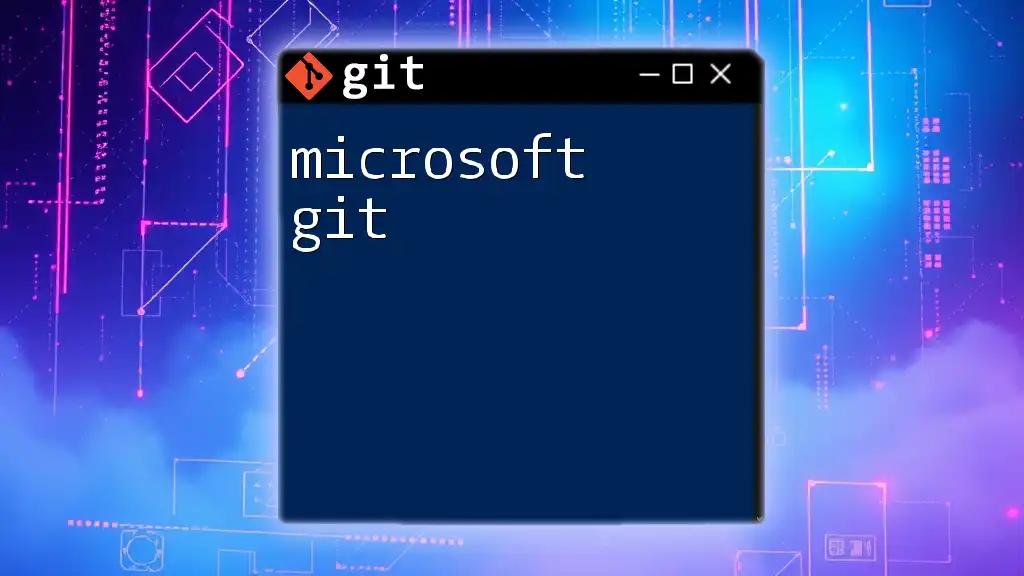
Conclusion
Navigating Windows Bash Git empowers you to manage your projects efficiently and collaboratively. By mastering basic commands and understanding more complex operations like branching and merging, you will enhance your workflow and collaborate effectively with others. Embrace these tools and practices, and take the next step in your Git journey through structured guidance available in our training program.
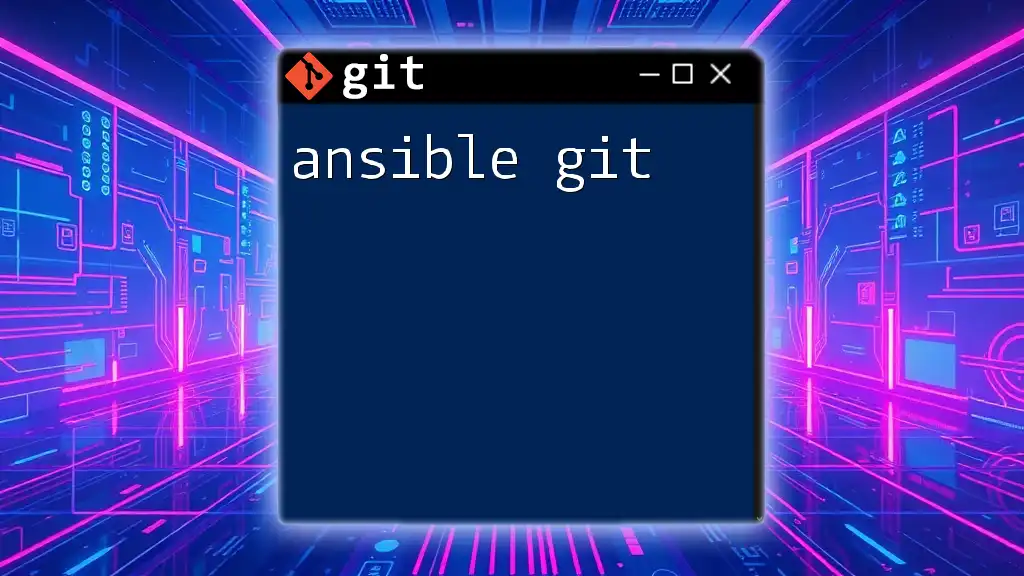
Additional Resources
For those looking to deepen their understanding, consider exploring the latest Git documentation, recommended tutorials, and community forums that provide additional support and guidance on using Git effectively.