The `rm` command in Git is used to remove files and/or directories from your working directory and staging area, effectively marking them for deletion from the repository in the next commit.
Here's how to use it:
git rm <file_name>
Understanding the `rm` Command
What is `rm` in Git?
The `rm` command in Git is used to remove files from both the staging area and the working directory. It’s important to understand that the `git rm` command functions distinctly from the traditional Unix/Linux `rm` command, which deletes files directly from the filesystem. In Git, using `git rm` also informs Git about the removal, effectively staging the change for the next commit. This way, Git keeps track of the history of file deletions.
Why Use `rm` in Git?
Maintaining a clean project repository is crucial for effective version control. The `rm git` command becomes essential when you need to remove unnecessary or obsolete files from your project. Some scenarios where you might want to use `git rm` include:
- Deleting temporary files that are no longer needed.
- Removing backups or old versions of files that are cluttering the repository.
- Tidying up during code refactoring.
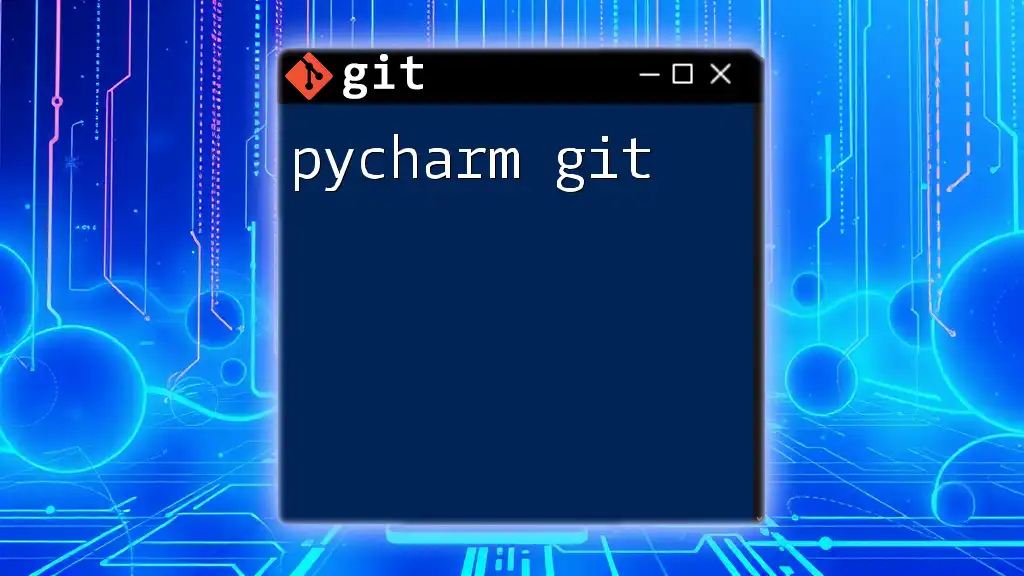
How to Use the `rm` Command
Basic Syntax of `git rm`
The general syntax for the `git rm` command is as follows:
git rm [options] <file>
This structure allows you to specify various options to customize the behavior of the command depending on your needs.
Removing a File with `git rm`
To remove a single file from your Git repository, you simply use the `git rm` command followed by the file's name. For instance:
git rm example.txt
Once executed, this command accomplishes two things: the file `example.txt` is removed from your working directory and staged for deletion in the next commit. Running `git status` after this command will show that the file has been deleted.
Removing Multiple Files
Using Wildcards
You can also remove multiple files at once using wildcards. For example, if you want to delete all log files in your directory, this command will do just that:
git rm *.log
Here, `*.log` acts as a wildcard that matches all files with a `.log` extension. Using wildcards can save you time and effort when dealing with many files that share a common naming pattern.
Case Study: Removing a Directory
How to Remove Entire Directories
To remove an entire directory and all its contents from your Git repository, you will need to use the `-r` (recursive) flag. Here is how you can do it:
git rm -r my_directory/
This command will delete `my_directory`, including all files and subdirectories within it. It’s worth noting that using the `-r` flag can lead to significant data loss if not used carefully, so always double-check before executing.
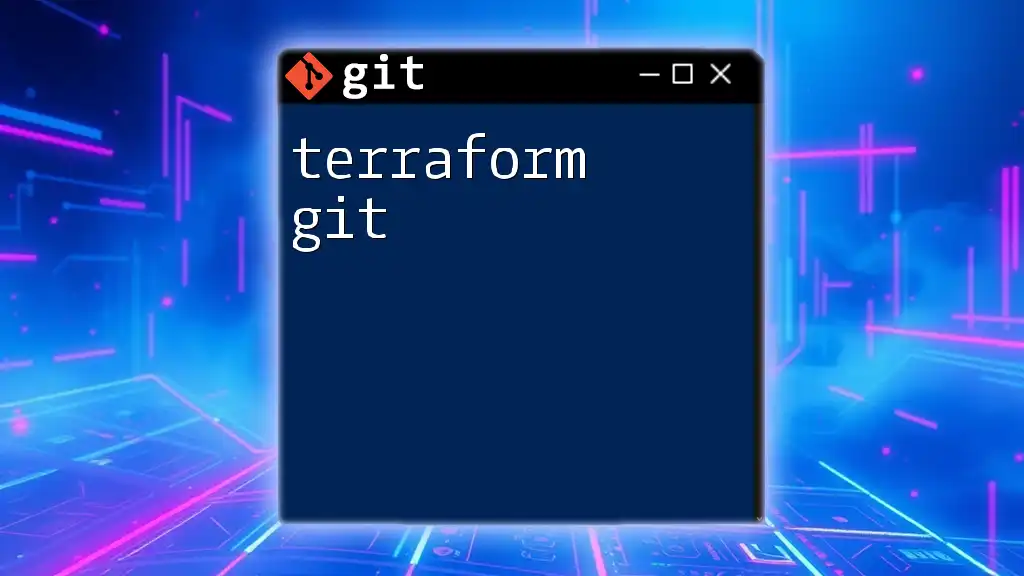
What Happens After Executing `git rm`
Staging the Changes
After you use `git rm`, the changes are staged automatically. To confirm the deletion, you can run:
git status
This command will show you the current state of your repository, with the removed files listed as changes ready to be committed.
Committing the Changes
Once you are satisfied with your changes, remember to commit them to finalize the removal. This is done using the commit command:
git commit -m "Removed unnecessary files"
Committing is essential because it saves the state of your repository. Best practices dictate that commit messages should be descriptive enough to convey the purpose of the changes made.
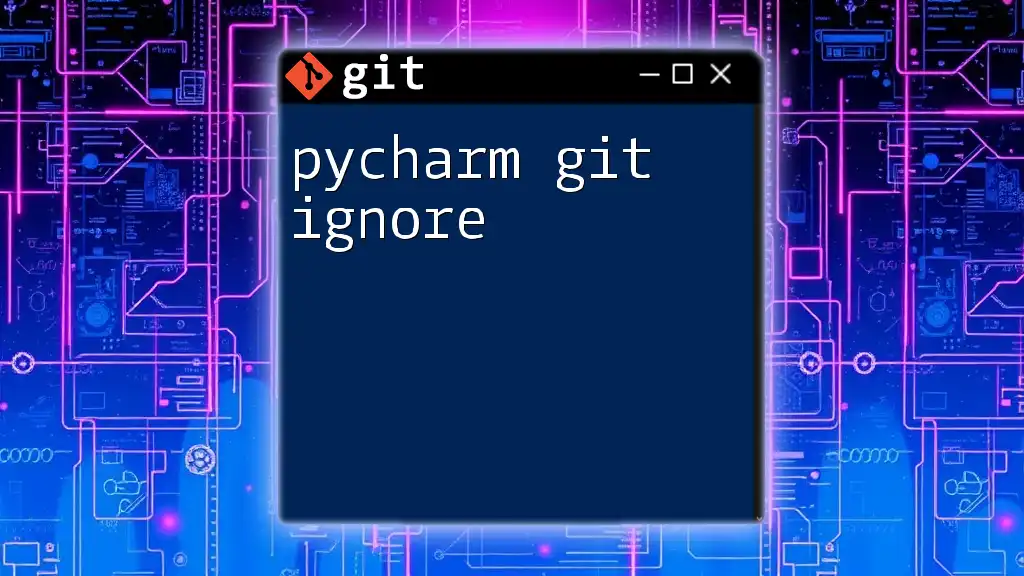
Common Options for `git rm`
Force Removal
If you encounter a scenario where you need to remove a file that has unsaved changes or is being tracked but not staged, you can use the `-f` (force) option:
git rm -f stubborn_file.txt
The `-f` flag instructs Git to disregard the changes and forcibly remove the file. Use this option with caution, as it may lead to loss of important data.
Caching Changes
In some cases, you might want to remove a file from version control without deleting it from your working directory. You can achieve this using the `--cached` option. Here’s how:
git rm --cached tracked_file.txt
This command stops tracking `tracked_file.txt` but leaves the file intact in your local filesystem. This can be particularly useful if you accidentally added a sensitive file to your Git history and want to remove it from tracking without losing the content.
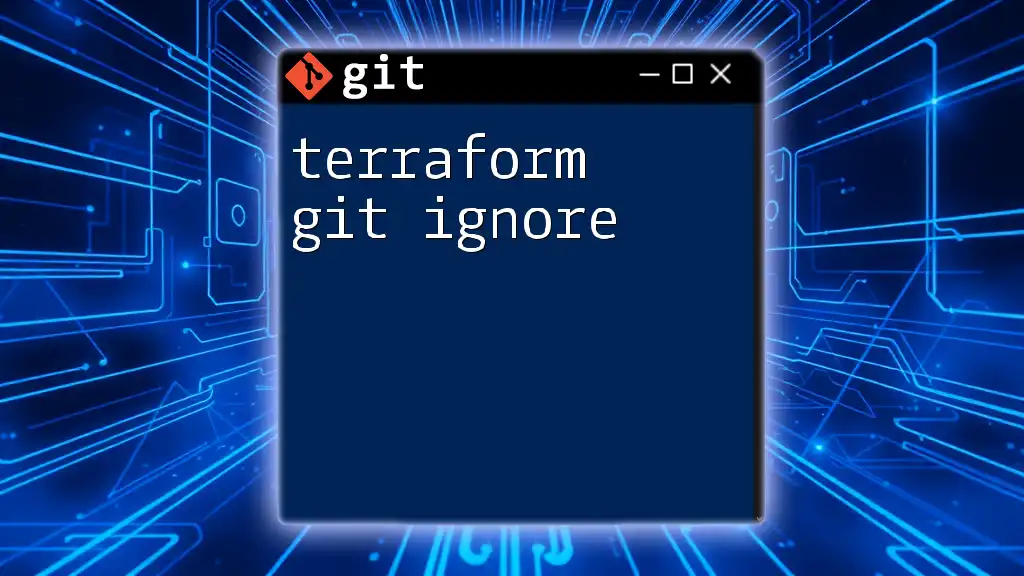
Undoing Changes After `git rm`
Recovering Deleted Files
If you realize that you’ve made a mistake after running `git rm`, there is a way to recover the deleted files. You can use the following command to restore the file from the last commit:
git checkout HEAD -- removed_file.txt
This command fetches the last committed version of `removed_file.txt` and places it back in your working directory. Practicing caution and double-checking your deletions can prevent many mistakes.
Best Practices to Avoid Mistakes
To ensure you don't accidentally remove crucial files, consider these best practices:
- Use `git status` before executing any `git rm` command to verify your current tracked files and changes.
- Back up important files in a different directory or branch before making deletions.
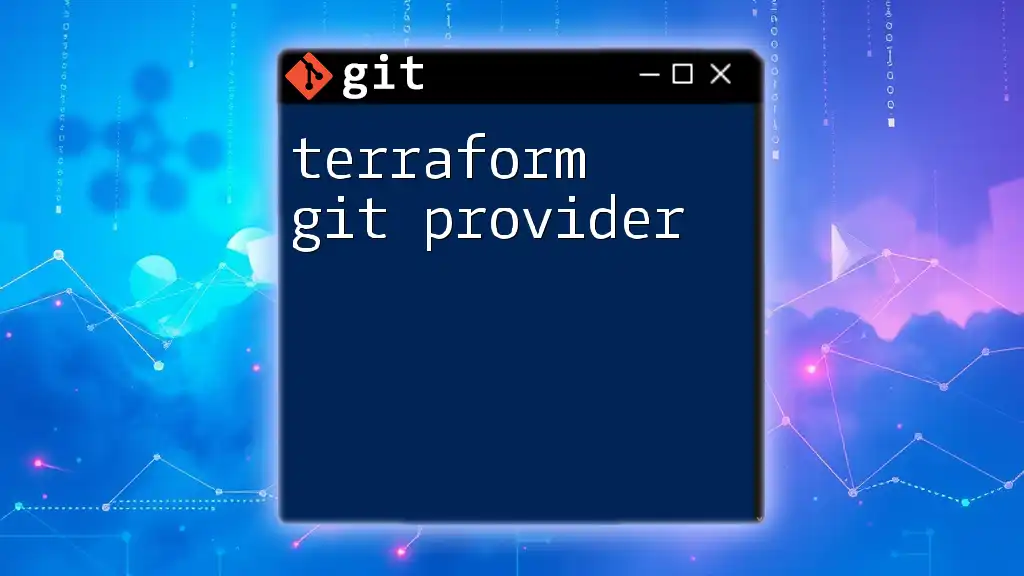
Conclusion
The `rm git` command is an essential tool for maintaining a clean and organized Git repository. By effectively using the command to manage files and directories, you can improve your workflow and maintain better version control practices. Practice its various options and features to become adept at using it in your daily versioning tasks.
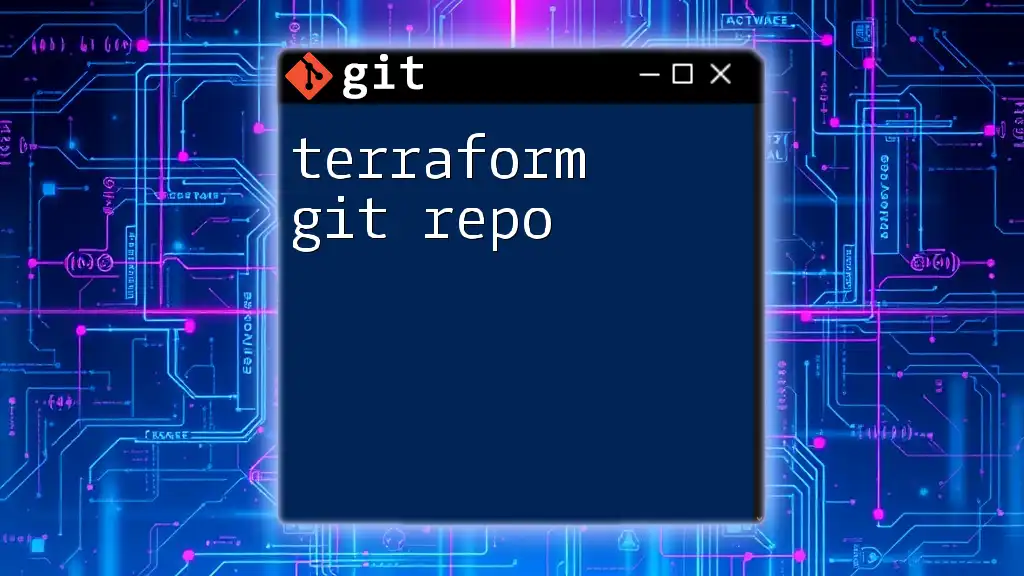
Additional Resources
For further mastery of Git commands, explore the official Git documentation and reputable online tutorials that offer deeper dives into version control principles.
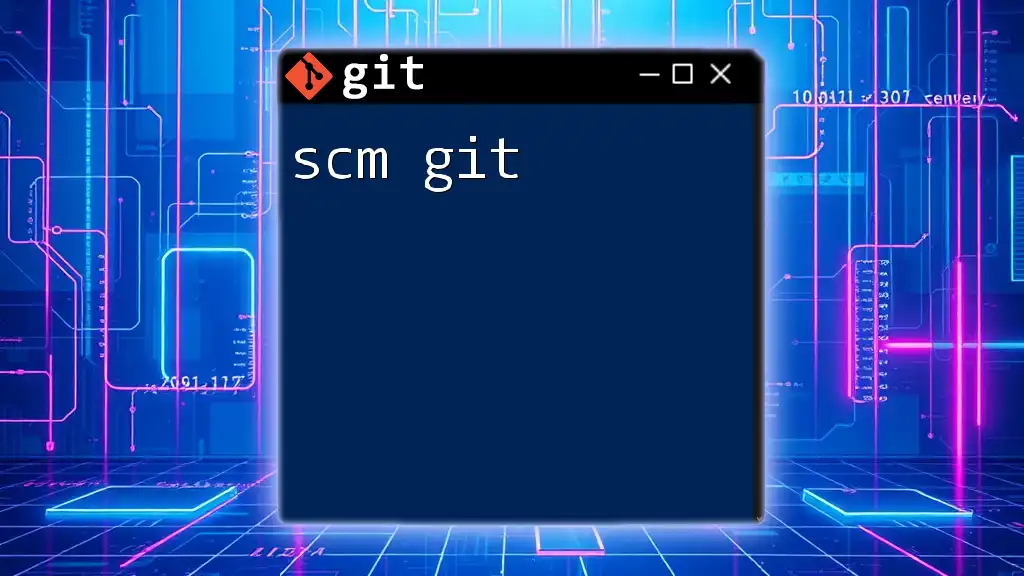
FAQs about `git rm`
Can I use `git rm` for files in the staging area?
Yes, you can use `git rm` for files that are currently staged. This command will remove them from both the working directory and the staging area.
What is the difference between `git rm` and `rm`?
The key difference is that `git rm` tracks the removal of files in the Git version history, whereas the Unix/Linux `rm` command merely deletes files from the filesystem without informing Git.
Is there a way to undo a `git rm` without losing the file?
Yes, by using the `git checkout` command, you can restore a file after using `git rm`.
What should I do if I accidentally removed a directory?
If you realize your mistake, use `git checkout HEAD -- path/to/directory` to restore the deleted directory and its contents from the last commit.