The Terraform Git provider enables you to manage Git repositories and resources, allowing you to automate interactions with version control systems as part of your infrastructure as code workflow.
Here's a simple example of how to use the Git provider in your Terraform configuration:
terraform {
required_providers {
git = {
source = "someprovider/git"
version = "~> 1.0"
}
}
}
resource "git_repository" "example" {
uri = "https://github.com/yourusername/your-repo.git"
branch = "main"
clone_path = "/path/to/clone"
private_key = file("~/.ssh/id_rsa")
# Additional configuration options
}
Understanding the Terraform Git Provider
What is Terraform?
Terraform is an open-source tool that enables users to manage infrastructure as code (IaC). This means that you can create, update, and version your infrastructure using declarative configuration files. Instead of manually creating resources in cloud platforms like AWS, Azure, or Google Cloud, Terraform allows you to define your infrastructure in simple, readable syntax. The key features of Terraform include:
- Declarative Configuration: Allows you to specify the desired state of your infrastructure.
- Execution Plan: Terraform shows you what it will change before it does so, allowing for easier management.
- Resource Graph: It builds a dependency graph of your resources, optimizing the resource creation process.
What is the Git Provider?
The Terraform Git Provider serves as a bridge between Terraform and your Git repositories, allowing you to manage and interact with Git resources through Terraform configurations. With this provider, you can execute various Git operations directly from your Terraform scripts, enabling a more effective workflow when managing infrastructure.
The use cases for integrating the Git Provider with Terraform include:
- Automating Deployment Pipelines: Easily clone repositories, push changes, and keep configurations synchronized.
- Version Management: Utilize Git as a version control system for Terraform scripts, making rollbacks and collaboration seamless.
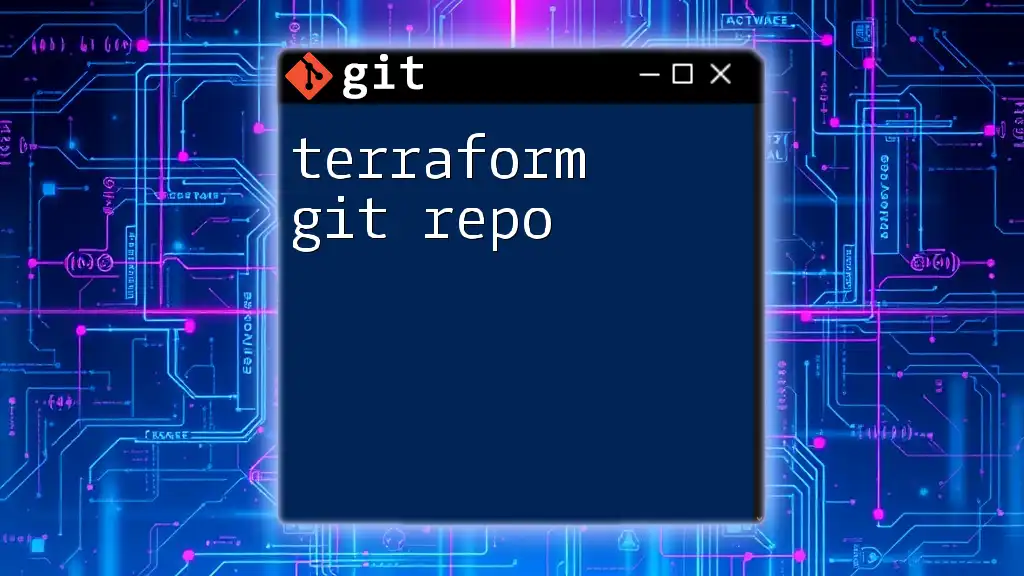
Setting Up the Terraform Git Provider
Prerequisites
Before you can dive into using the Terraform Git Provider, ensure you have the following set up on your local machine:
- Terraform Installation: Download and install Terraform from the official website.
- Git Installation: If you haven't done so already, install Git and configure your user settings.
Initial Setup
To begin working with the Terraform Git Provider, you need to create a new Terraform project and configure the Git Provider in your configuration file. First, create a new directory for your project:
mkdir terraform-git-provider-example
cd terraform-git-provider-example
Now, create a `main.tf` file and add the following configuration to set the Git Provider:
terraform {
required_providers {
git = {
source = "hashicorp/git"
version = "~> 1.0"
}
}
}
This code snippet sets the Git Provider as a required provider for your Terraform project, indicating that you will use features defined within this provider.
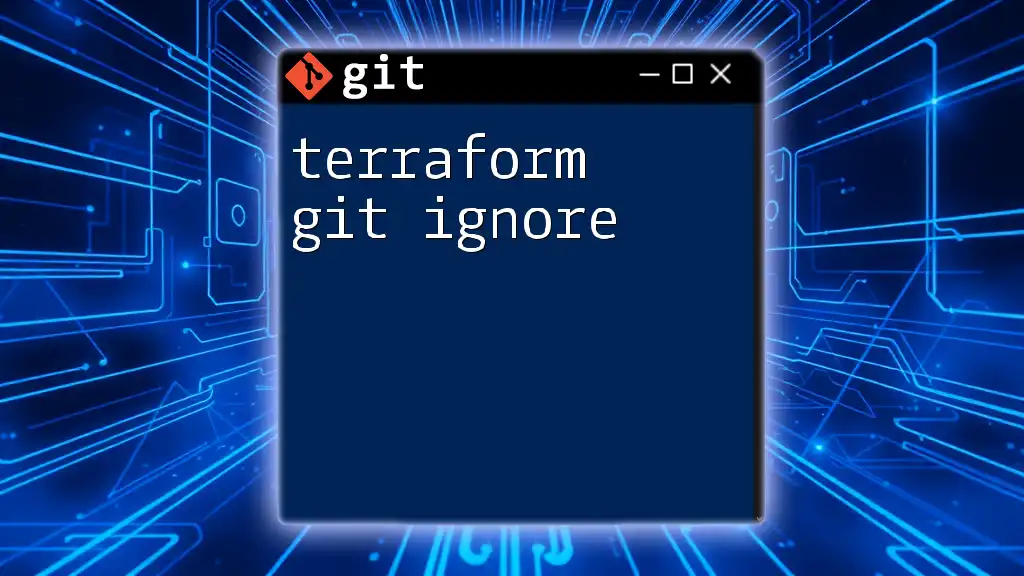
Working with Git Repositories in Terraform
Authenticating with Git
Authentication is crucial for accessing private repositories. There are two main methods available:
- SSH Keys: Generate an SSH key pair and add the public key to your Git provider (e.g., GitHub, GitLab). This allows you to clone and manage repositories securely.
- HTTPS: You can authenticate using username and password or personal access tokens.
For SSH authentication, you might use the following commands to create your keys:
ssh-keygen -t rsa -b 4096 -C "your_email@domain.com"
After generating your keys, remember to add the public key (`~/.ssh/id_rsa.pub`) to your Git provider's settings.
Resources Supported by the Git Provider
The Git Provider supports several resources, with the most commonly used being:
- `git_repository`: Represents a Git repository.
- `git_branch`: Represents branches within a Git repository.
- `git_commit`: Allows you to interact with commits in the repository.
An example of how to define a Git repository using Terraform would be:
resource "git_repository" "example" {
name = "example-repo"
owner = "example-user"
provider = git
}
This snippet creates a new Git repository resource, specifying the name and owner for clarity and ease of management.
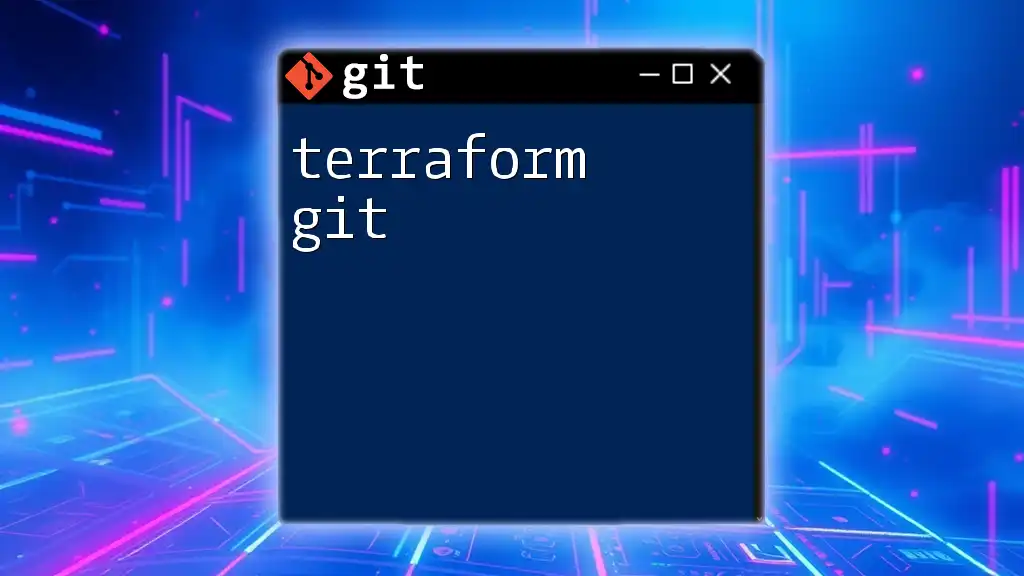
Key Terraform Commands for Git Operations
Cloning a Repository
To clone a repository using the Terraform Git Provider, you can set up the `git_clone` resource. This allows you to pull down a specific repository and manage it accordingly:
resource "git_clone" "example" {
repository = git_repository.example.id
}
Committing Changes
Managing commits can be particularly powerful. You can configure your Terraform project to facilitate commits directly within your Terraform scripts. A typical commit configuration may look like:
resource "git_commit" "new_commit" {
repository = git_repository.example.id
message = "Automated commit from Terraform"
changes = ["file1.txt", "file2.txt"]
}
Pushing Changes
When you need to push your updates back to the Git remote, you need to leverage Terraform’s functionality. Here’s an example of setting up a push operation:
resource "git_push" "push_changes" {
repository = git_repository.example.id
branch = "main"
}
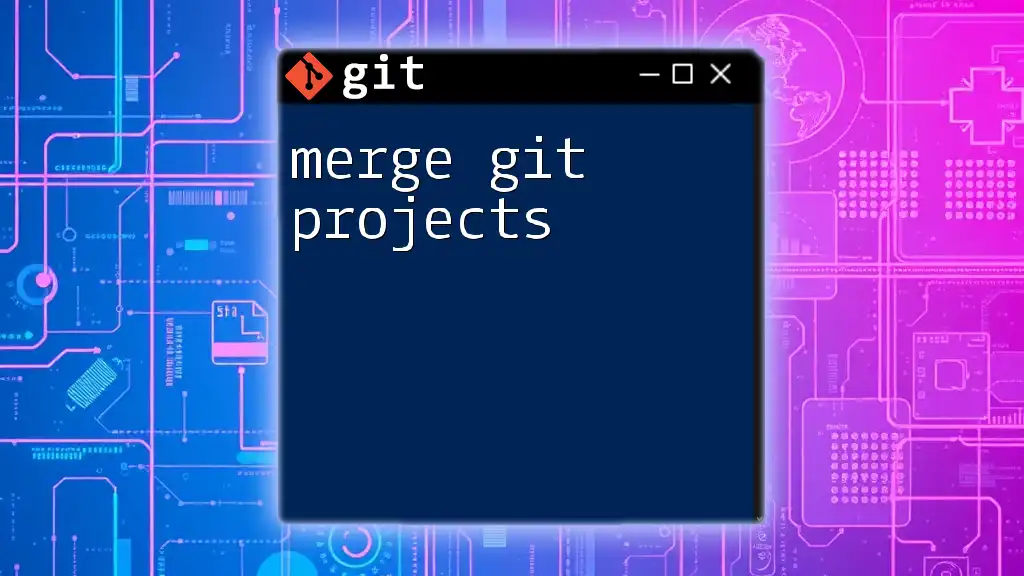
Best Practices for Using the Git Provider
Version Control with Terraform
One of the most significant advantages of integrating Git with Terraform is the ability to manage versioning effectively. Always tag important points in your infrastructure history, allowing you to refer back or roll back when necessary.
Consider implementing branching strategies, such as feature branches for new work or master/main for stable releases.
Collaboration and Workflow Management
When working in teams, establishing a common Git workflow is essential. The Git Flow model is an excellent strategy where development occurs in feature branches, and stable versions are merged into the main branch periodically.
Integrating Continuous Integration/Continuous Deployment (CI/CD) practices with Terraform and Git can enhance your deployment process. This approach allows automated tests to validate infrastructure changes before merging into the main branch.
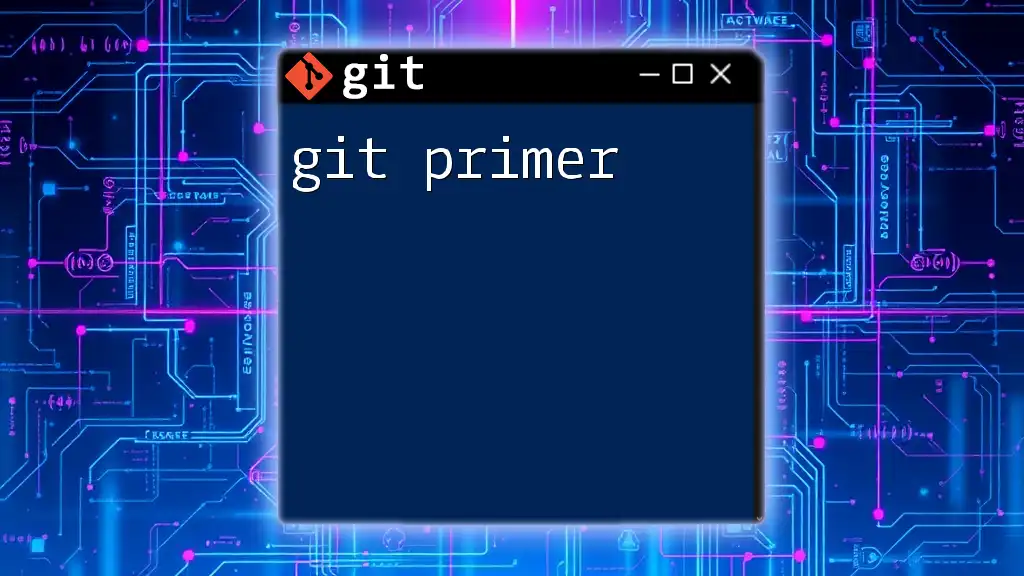
Troubleshooting Common Issues
Common Errors and Solutions
Throughout your experience with the Git Provider, you may encounter some common issues:
- Authentication Failures: Verify your SSH keys or access tokens, and ensure they hold the required permissions for the repository.
- Repository Access Errors: Ensure your repository has been created and that you are correctly referencing it in your Terraform files.
Debugging Terraform Configurations
Debugging can be simplified by utilizing Terraform's logging capabilities. You can enable debug logs to get more insight into what Terraform is processing by setting the environment variable before running your command:
export TF_LOG=DEBUG
This command helps you spot misconfigurations and understand how Terraform processes your operations.
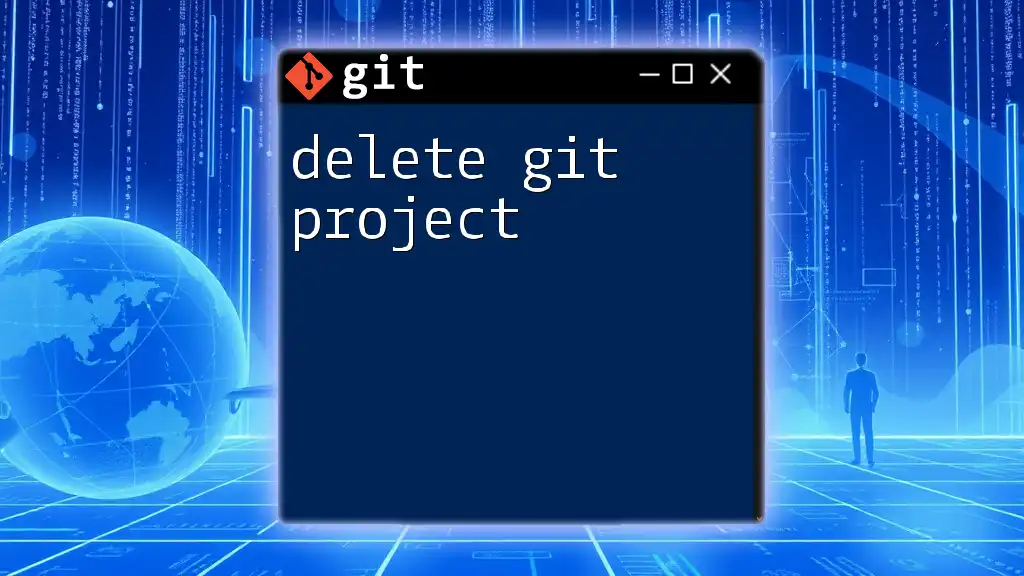
Conclusion
The Terraform Git Provider offers robust capabilities for managing Git resources directly from your Terraform configurations, streamlining your infrastructure automation. By integrating Git, you can leverage version control for your Terraform scripts, ensuring a smoother deployment process and better collaboration.
As you embark on your journey with the Terraform Git Provider, consider exploring further into advanced features and integrations that can enhance your Infrastructure as Code practices. Embrace this powerful tool and its capabilities to optimize your infrastructure management.
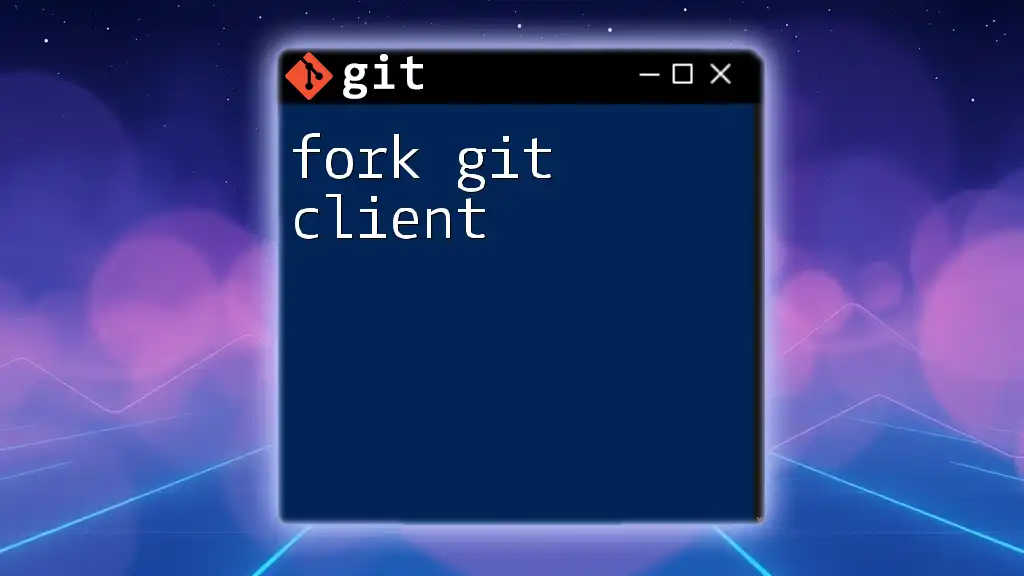
Additional Resources
For further learning, consider diving into:
- The official [Terraform documentation](https://www.terraform.io/docs)
- Tutorials on Git and Terraform workflows
- Community forums like Stack Overflow for troubleshooting and support
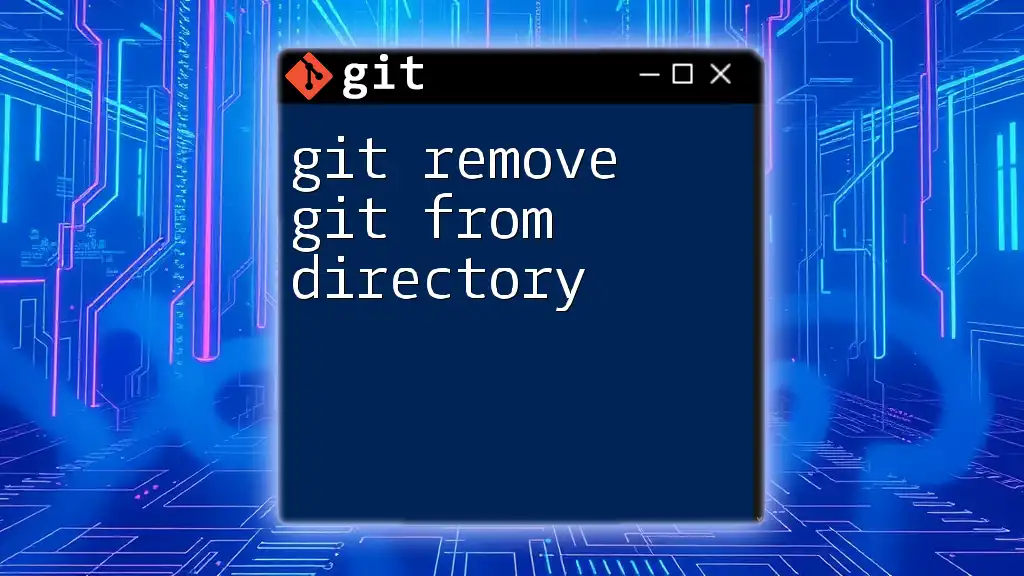
Call to Action
Equip yourself with the skills to harness Terraform and Git effectively. Sign up for our courses to enhance your understanding and application of these powerful tools in real-world scenarios!