To force a Git merge when you want to merge a branch but have conflicts that you want to override, use the `--strategy-option theirs` to resolve the conflicts in favor of the incoming branch.
Here’s the command you would use:
git merge <branch-name> --strategy-option theirs
Understanding Git Merging
What is Merging in Git?
Merging is a fundamental aspect of Git that enables you to combine changes from different branches into a single branch. It's an essential operation in collaborative environments where multiple developers contribute to the same project. By merging, you ensure that all the contributions are integrated and that the project remains up-to-date with everyone’s changes.
Types of Merging
Merging in Git generally falls into two categories: fast-forward merges and three-way merges.
Fast-Forward Merge
A fast-forward merge occurs when the branch being merged is ahead of the current branch with no divergent changes. In this scenario, Git simply moves the pointer of the current branch forward to the tip of the merged branch.
Here’s how to perform a fast-forward merge:
git merge feature-branch
This command will advance the pointer of your current branch to include all the new commits from `feature-branch`.
Three-Way Merge
A three-way merge is a more complex operation that occurs when the branches have diverged. This situation requires Git to analyze the last common ancestor of both branches to properly combine the changes. This is the most common scenario in collaborative workflows.
To initiate a three-way merge, you can use:
git merge branch-name
If conflicts arise, Git will require you to resolve them manually.
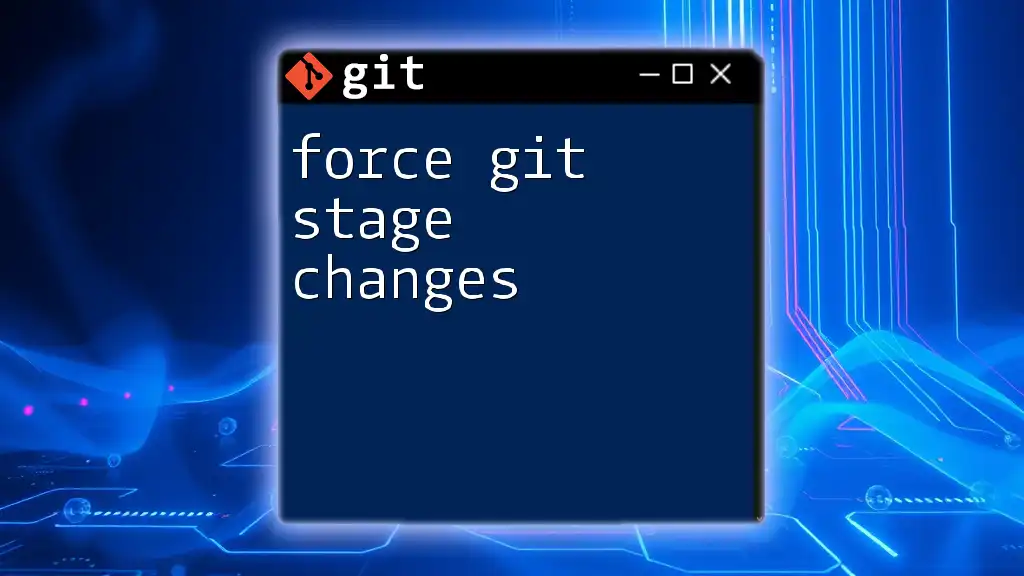
When to Use Force Merge
Situations Requiring a Force Merge
A force git merge may become necessary in several situations:
-
Resolving significant merge conflicts: When both branches have changes that conflict heavily, and you have decided which branch’s changes should prevail.
-
Overriding branch differences: In cases where one branch is significantly lagging in incorporating changes from the other branch.
Understanding when to force a merge helps in maintaining project integrity, but it’s crucial to proceed with extreme caution.
Risks of Using Force Merge
While a force git merge is a powerful tool, it comes with its risks:
-
Potential data loss: A careless force merge can overwrite committed changes from other branches without preserving them, leading to irretrievable data loss.
-
Compromising project integrity: Skipping the necessary review and resolution of conflicts can lead to a codebase that functions incorrectly or has bugs.
-
Importance of communication with team members: Failing to communicate could lead to confusion and conflicts amongst team members, impacting workflow and team morale.
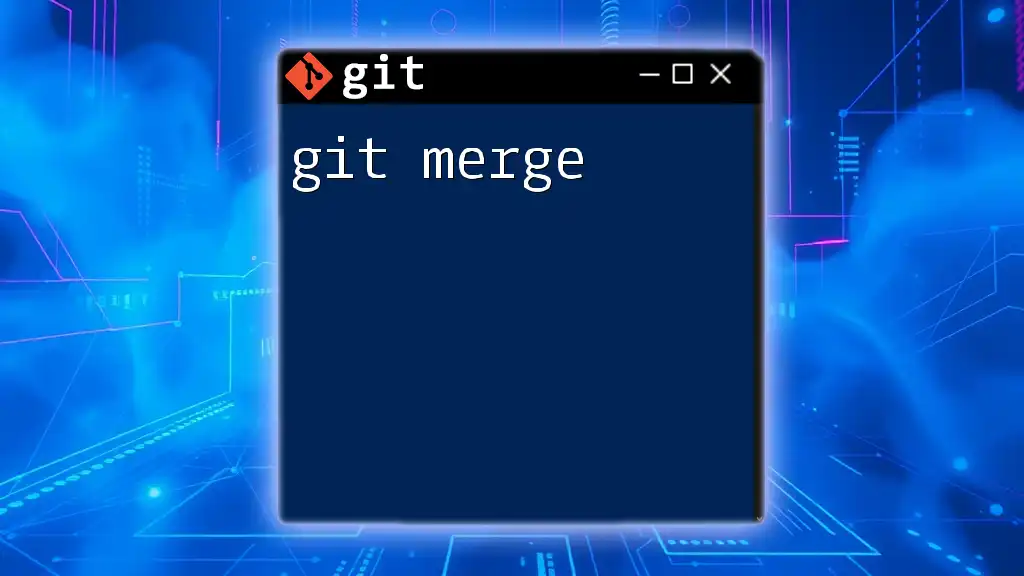
Performing a Force Merge in Git
Pre-Merge Preparation
Before you execute a force merge, it’s vital to backup your work. Creating a copy of your current branch can safeguard against accidental data loss during the merge process.
To set up a backup branch, use the following command:
git branch backup-branch
This creates a branch with all the current changes where you can revert back if needed.
Executing a Force Merge
Merging with `--no-ff` Option
The `--no-ff` option prevents Git from fast-forwarding during the merge. Instead, it creates a new commit for the merge, preserving the history of the merged branch. This is particularly useful for maintaining a clear project history.
To merge using the `--no-ff` option, use:
git merge --no-ff feature-branch
This command will create a merge commit even if a fast-forward merge is possible.
Using Git's `-X theirs` and `-X ours` Options
When you encounter conflicts during a merge, Git provides strategies to resolve these conflicts automatically:
- Using `-X theirs`: This strategy favors the changes from the branch you are merging in, effectively ignoring changes from the current branch where possible. This can be executed with:
git merge -X theirs conflicting-branch
- Using `-X ours`: Conversely, this option favors changes from your current branch, effectively overriding incoming changes. Use it with:
git merge -X ours conflicting-branch
These options can be immensely helpful in swiftly resolving conflicts without manual intervention.
Finalizing the Merge
Once the merge has been successfully applied, it’s time to commit the changes. Use the following command to confirm and document your merge:
git commit -m "Force merged with conflicting-branch"
After committing, it’s essential to verify that all changes have been integrated correctly into the codebase. Check your files, run test cases, and ensure everything is functioning as expected.
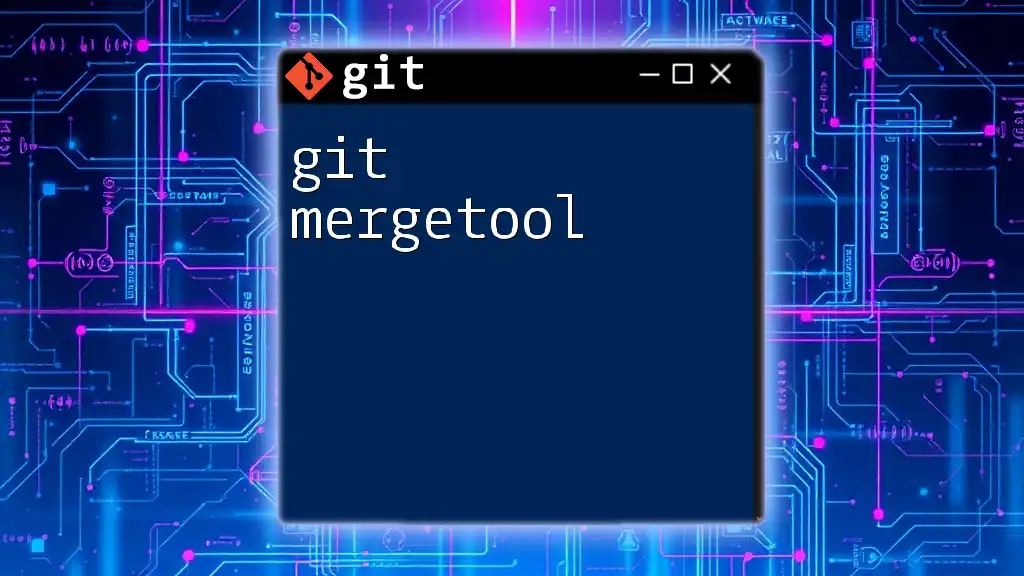
Best Practices for Force Merging
Communicating with Your Team
Effective communication is crucial when performing a force git merge. Discuss your intent to force merge with your team members beforehand to avoid any surprises or misunderstandings. Suggested channels for discussion include Slack, email, or team meetings.
Keeping a Clean Project History
Maintain a clean project history by using branches effectively. Regularly update your branches with the latest changes, and ensure that unnecessary branches are deleted after they have been merged.
Regular Code Reviews
Establish a process for code reviews to ensure code quality. Regularly reviewing changes helps catch potential issues early, making it easier to handle conflicts before they escalate. Tools like GitHub or GitLab can assist in the code review process.
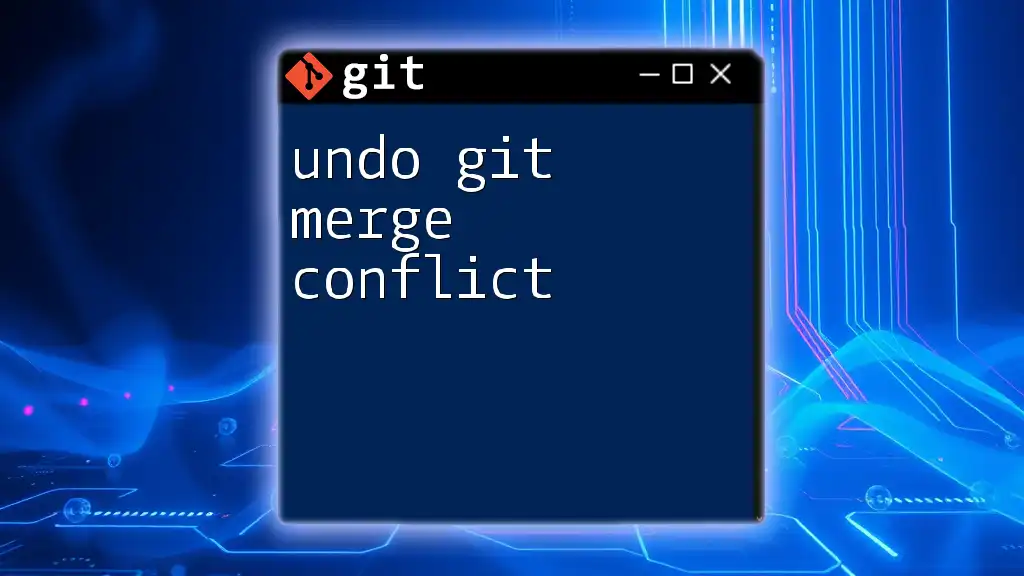
Conclusion
Understanding and responsibly executing a force git merge is vital for maintaining project health in collaborative environments. Always prioritize communication and backup measures when dealing with merges. Proper execution can lead to a smooth workflow, but awareness of risks and team dynamics is key to success.
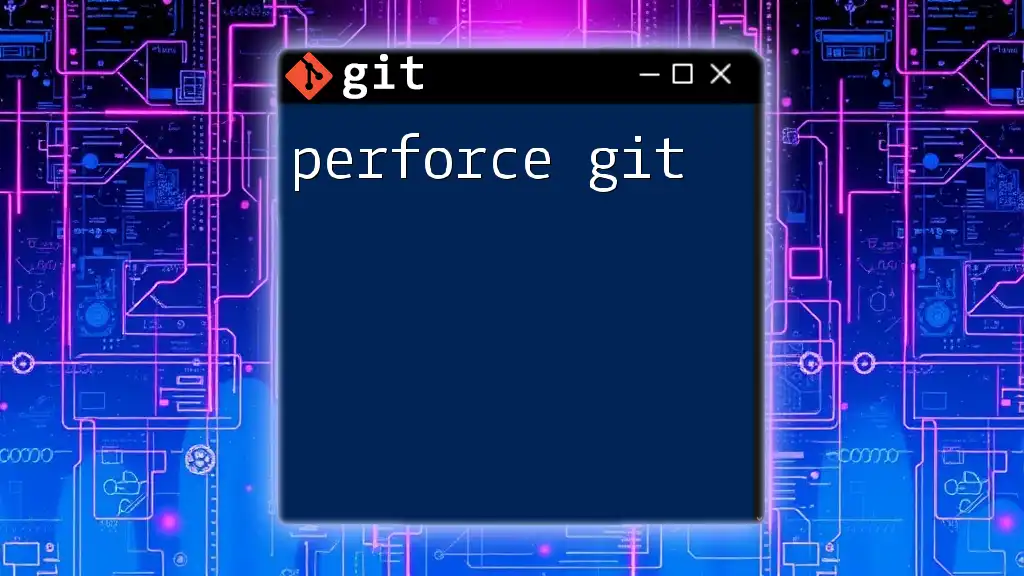
Additional Resources
For further reading and to deepen your understanding of Git, consider exploring the official Git documentation or online tutorials. Resources that cover version control best practices will enhance your skills in using Git effectively.