A Terraform Git repository is a centralized location where infrastructure as code templates are stored and versioned using Git commands, enabling collaboration and efficient management of cloud resources.
Here's a basic example of how to clone a Terraform Git repository:
git clone https://github.com/username/terraform-repo.git
Understanding Terraform
What is Terraform?
Terraform is an open-source tool designed for Infrastructure as Code (IaC)—allowing you to define and provision data center infrastructure using a declarative configuration language known as HashiCorp Configuration Language (HCL). Terraform enables organizations to automate the deployment and management of infrastructure at scale.
With features such as resource graphs and execution planning, Terraform offers visibility into infrastructure updates and dependencies that many traditional provisioning tools lack. This ensures that any infrastructure changes are performed accurately and efficiently, minimizing the risks of errors and downtime.
Why Use Git with Terraform?
Integrating Git with Terraform is vital for maintaining the integrity and continuity of your infrastructure management. The benefits include:
- Version Control Benefits: You can track changes made over time, facilitating easy rollbacks if something goes wrong.
- Team Collaboration: Multiple team members can work simultaneously on Terraform code without worrying about overwriting each other's work, thanks to branching and merging capabilities of Git.
- Audit Trail: Maintaining a history of all changes helps in compliance and accountability, especially in regulated environments.
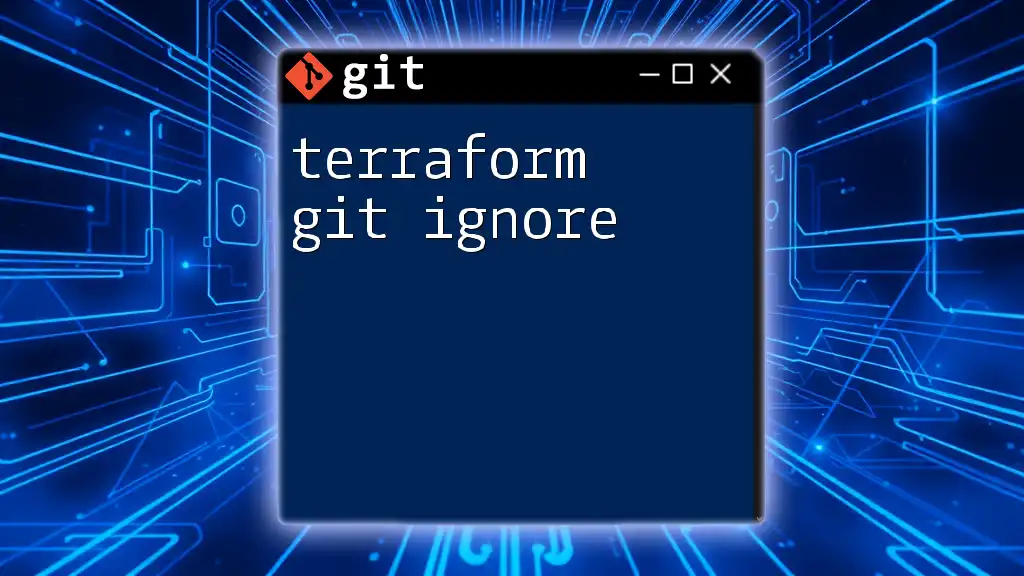
Setting Up Your Terraform Git Repository
Creating a New Git Repository
To begin, navigate to your project directory and initialize a Git repository with the following commands:
mkdir terraform-project
cd terraform-project
git init
This establishes a new Git repository where you can start tracking your Terraform files. As you build your repository, maintain a clear directory structure to improve organization and maintainability.
Basic File Structure for Terraform Projects
A well-defined structure for your Terraform project can significantly enhance readability and manageability. Here’s a recommended file structure:
terraform-project/
│
├── README.md # Project documentation
├── main.tf # Main Terraform configuration
├── variables.tf # Input variable definitions
├── outputs.tf # Output values
├── modules/ # Directory for reusable modules
│ ├── module_name/
│ ├── main.tf
│ ├── variables.tf
│ └── outputs.tf
└── environments/ # Configuration files for different environments
├── dev/
└── prod/
Adding Terraform Configuration Files
Adding your first Terraform configuration file is crucial for establishing your infrastructure. For example, to configure an AWS S3 bucket, you can start with the following code snippet in your `main.tf` file:
# main.tf
provider "aws" {
region = "us-east-1"
}
resource "aws_s3_bucket" "my_bucket" {
bucket = "my-unique-bucket-name"
}
This code defines an AWS provider and creates an S3 bucket. It's essential to tailor values to your project’s needs.
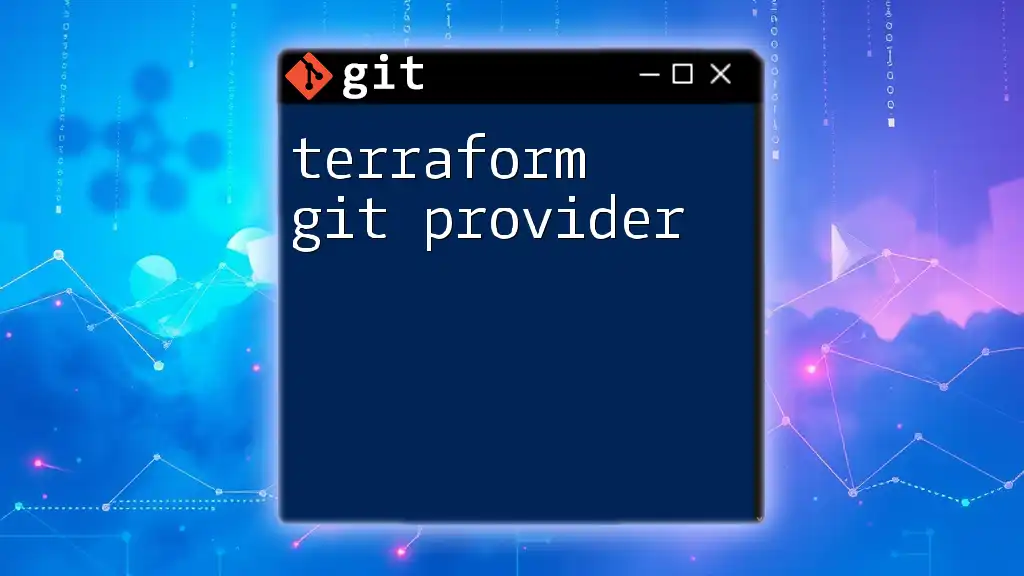
Using Git Commands with Terraform
Basic Git Commands for Initialization
Once you have your Terraform setup, it’s time to start version controlling your changes. Use the `git add` command to stage your files and the `git commit` command to save them:
git add .
git commit -m "Initialize Terraform project with S3 bucket"
Meaningful commit messages provide context for your changes, making it easier for team members (and future you) to understand the repository's history.
Workflow for Updating Terraform Code
As you develop and iterate on your Terraform files, adopting a consistent workflow is essential. Whenever you make changes to your configuration, follow these steps to ensure proper tracking:
- Make your changes to the `.tf` files.
- Stage the changes using `git add`.
- Create a commit with a clear, descriptive message.
For example, if you update your S3 bucket configuration to enable versioning, your commands might look like this:
git add .
git commit -m "Updated S3 bucket configuration to enable versioning"
Branching Strategies
Effective branching strategies can help manage features and fixes without disrupting your main codebase. One common approach is Feature Branching. Rather than making all changes directly to the `main` branch, create a new branch for each feature or update:
git checkout -b feature/add-s3-versioning
After implementing your changes and testing them, merge your feature branch back into `main`:
git checkout main
git merge feature/add-s3-versioning
This practice helps maintain a clean main branch while allowing for experimental work.
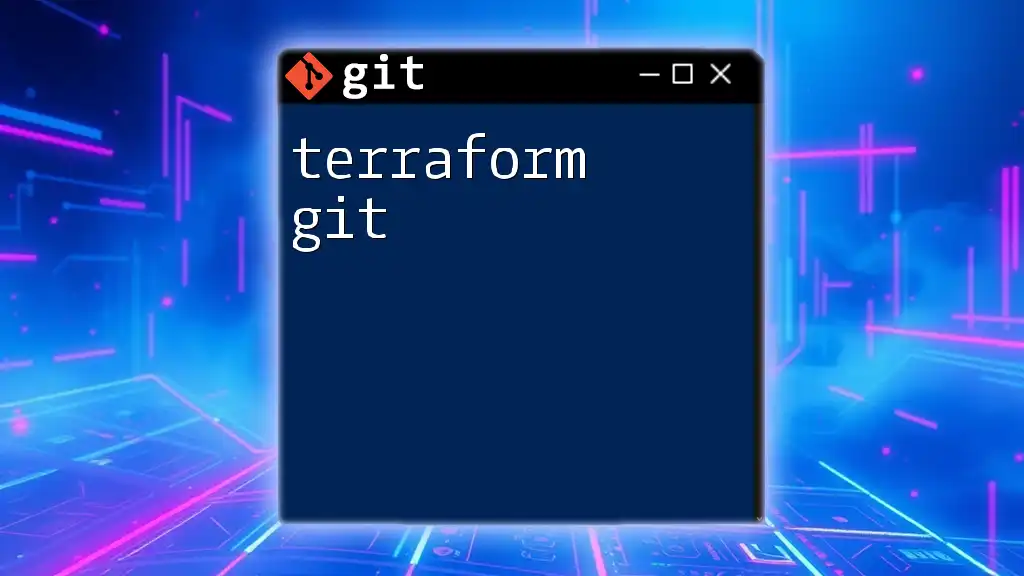
Managing Terraform State Files
Understanding Terraform State
Terraform keeps track of the resources it manages in a state file, which is critical for mapping the real-world infrastructure to your configurations. Understanding and managing this state is essential; it allows Terraform to accurately reflect the actual state of your infrastructure.
Avoid including `.tfstate` files in your Git repository, as they can contain sensitive information and can lead to conflicts when multiple users make changes.
Ignoring Sensitive Files
To prevent sensitive files from being tracked by Git, you should create a `.gitignore` file in your main repository directory. A typical `.gitignore` for a Terraform project might include:
*.tfstate
*.tfstate.backup
.terraform/
This ensures that your state files and temporary directories are excluded from version control.
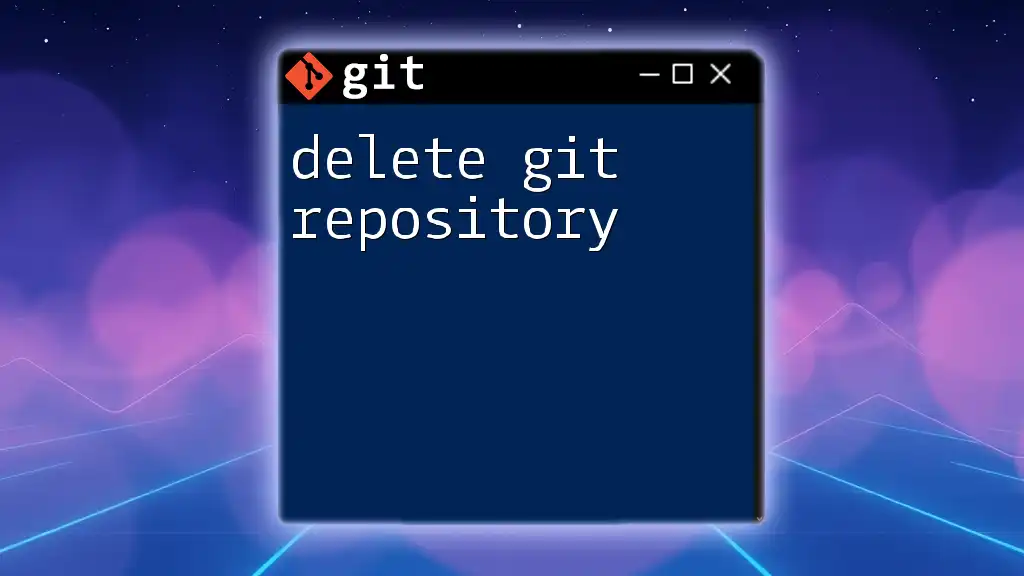
Collaboration with Git in Terraform Projects
Code Reviews
In any collaborative environment, code reviews are invaluable. When using Git, it's common to create pull requests (PRs) for your changes. This allows your team to review and discuss changes before they're merged into the main branch.
This practice not only helps catch potential issues before deployment but also fosters knowledge sharing among team members.
Handling Merge Conflicts
With multiple collaborators, it’s possible to encounter merge conflicts. These often occur when two or more team members modify the same line in the same file. In such cases, you can resolve conflicts by:
- Checking the status of your repository with `git status`.
- Using `git merge --abort` if you want to backtrack on the incomplete merge.
- Manually merging changes in the file and committing the resolution.
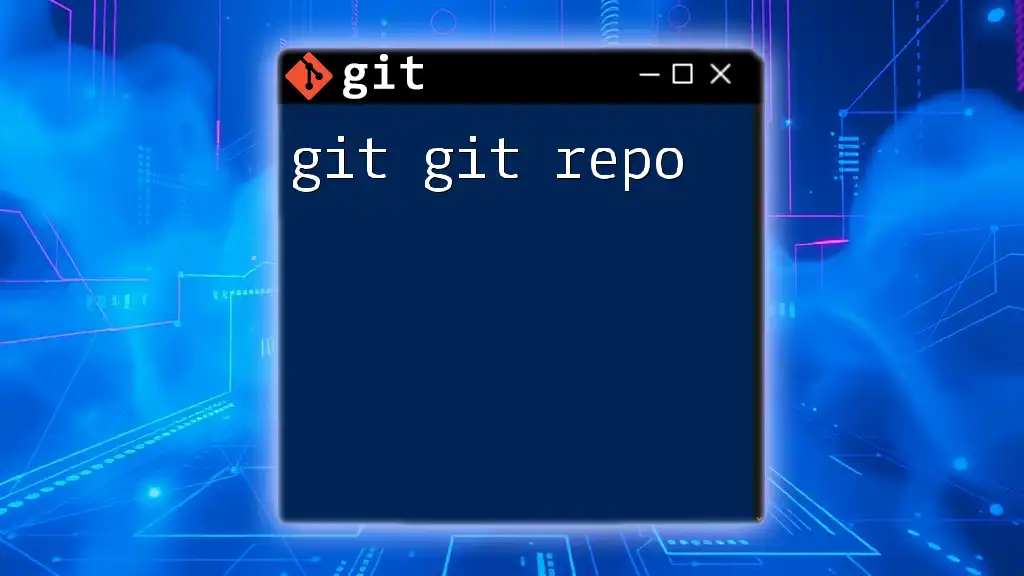
Best Practices for Terraform Git Workflow
Commit Often and Use Clear Messages
Regularly committing changes helps maintain a clear project timeline. Each commit should have a meaningful message that accurately summarizes the changes made. This practice not only aids your understanding of the project’s evolution but also assists others in navigating the commit history.
Documentation and Comments
Inline comments within your Terraform files improve readability and maintainability. Moreover, creating a `README.md` file at the root of your repository is essential for documenting your project. Include information such as:
- Project purpose
- Quick start guide
- Instructions for contributing
This documentation aids new team members and provides context for decisions made during development.
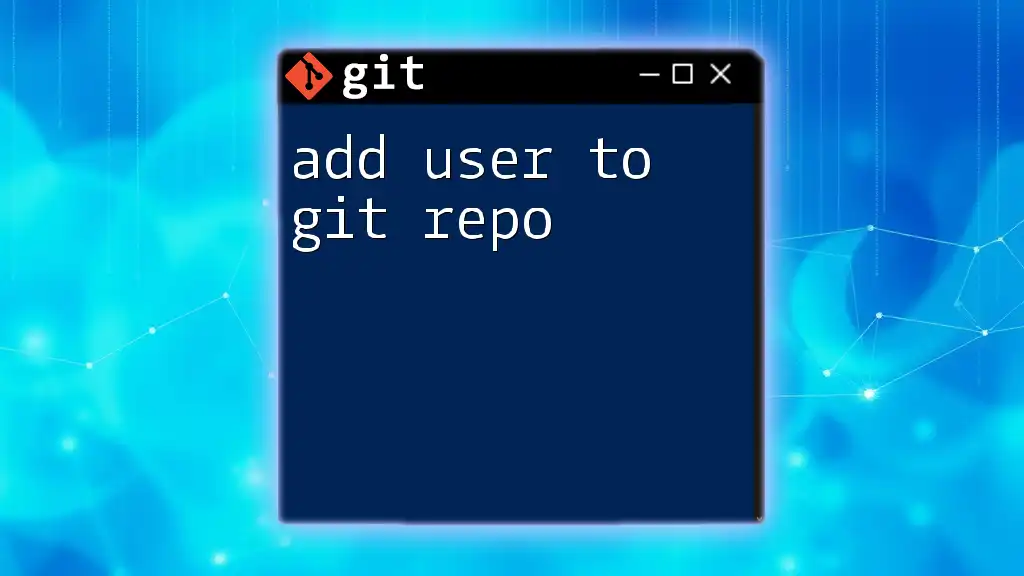
Conclusion
Using a Terraform Git repo effectively enables you to strengthen collaboration, streamline changes, and maintain robust infrastructure management practices. By implementing these workflows and best practices, you set your team up for success in managing infrastructure with ease and efficiency.
Now is the perfect time to apply these methods and enhance your infrastructure as code journey with Terraform and Git!
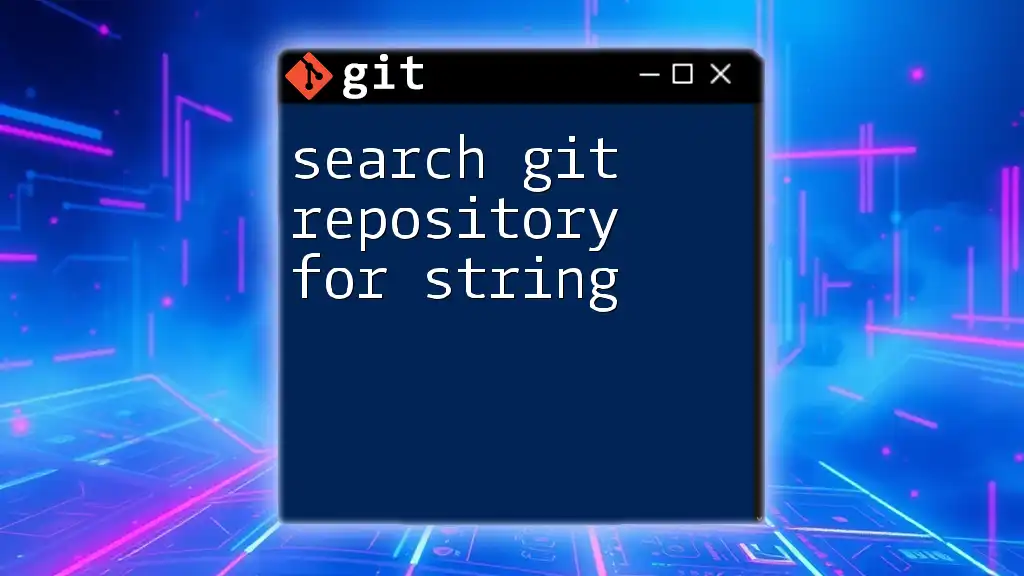
Additional Resources
- [Terraform Documentation](https://www.terraform.io/docs/index.html)
- [Git Documentation](https://git-scm.com/doc)
- Examples of well-structured Terraform Git repositories available on GitHub or other code hosting services.