The "error: unable to update local ref" during `git pull` typically occurs when there is a conflict between local and remote branch references, which can often be resolved by manually deleting the problematic references or performing a hard reset.
git fetch --prune origin
git reset --hard origin/main
Understanding the Basics
What is Git?
Git is a powerful version control system that allows developers to track changes in their codebase over time. It facilitates collaboration among multiple developers while maintaining a history of edits and updates. With Git, you can easily roll back to previous versions, create branches, and merge code, making it an essential tool in modern software development workflows.
What is a Git Pull?
The `git pull` command is a critical operation that fetches and integrates changes from a remote repository into your current branch. It effectively combines two actions: fetching the latest changes (`git fetch`) and automatically merging those changes into your local branch. While this command streamlines collaboration, its misuse or conflicts can lead to issues, including the notorious error lock ref during git pull.
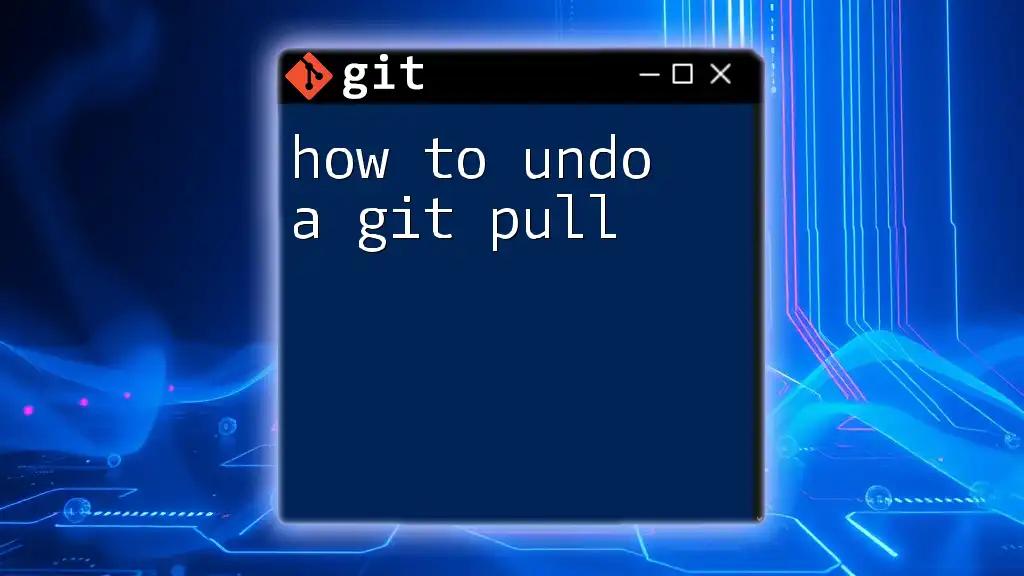
The Lock Ref Error: An Overview
What is the Lock Ref Error?
The lock ref error occurs due to Git's mechanism for avoiding simultaneous updates to the same reference. When you attempt a `git pull`, Git tries to update the references of branches but encounters a situation where one is already locked. This results in an error message indicating that Git could not create a lock file necessary for the operation.
Common Causes of the Lock Ref Error
There are several reasons why you might encounter the error lock ref during git pull, including:
- Filesystem Permissions Issues: If your user account lacks the right permissions for the Git repository, it can lead to lock files not being created or accessed.
- Simultaneous Git Operations: Running multiple Git processes at the same time, such as two `git pull` commands, can conflict and cause a lock.
- Corrupted Reference Files: Occasionally, the files in `.git/refs/heads/` can become corrupted, leading to the lock error.
Impact of the Error
Ignoring the lock ref error can severely disrupt collaborative workflows. It may prevent you from pulling the latest changes and could hinder your ability to push updates to the remote repository. Therefore, it is crucial to address this error promptly to maintain efficient project management and version control.
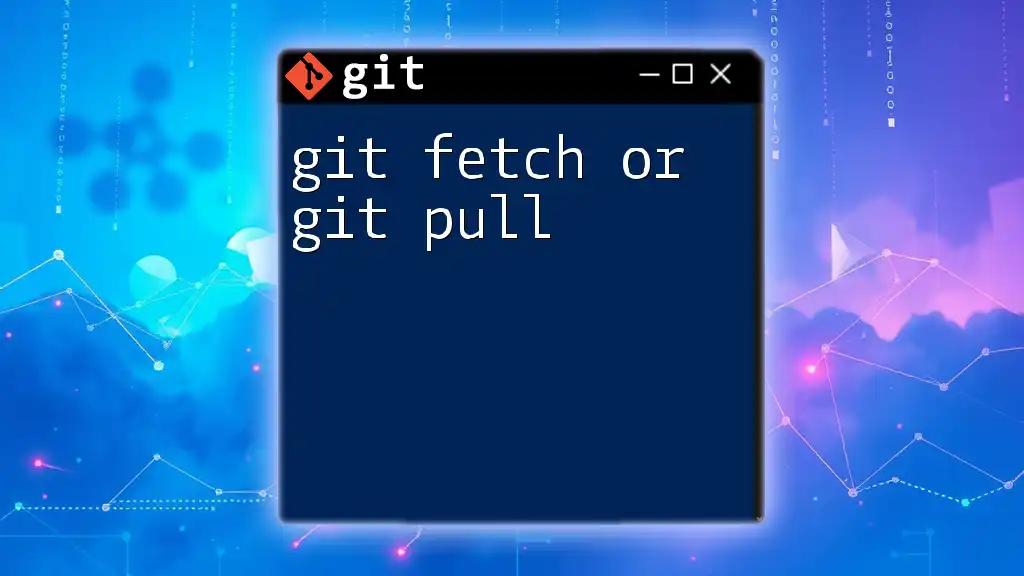
Diagnosing the Error
Identifying the Error
You will typically recognize the error lock ref during git pull when you see an error message similar to the following in your terminal:
fatal: Unable to update the ref 'refs/heads/branch_name': Unable to create '.../refs/heads/branch_name.lock': File exists.
This message is clear and indicates a problem with the reference file locking.
Logs and Debugging
To further diagnose the issue, you can inspect the Git logs. The following commands can help you troubleshoot:
- Check the reference logs:
git reflog
- Verify the integrity of your repository:
git fsck
Using these commands, you can identify any anomalies in your repository’s history or integrity that could contribute to the lock error.
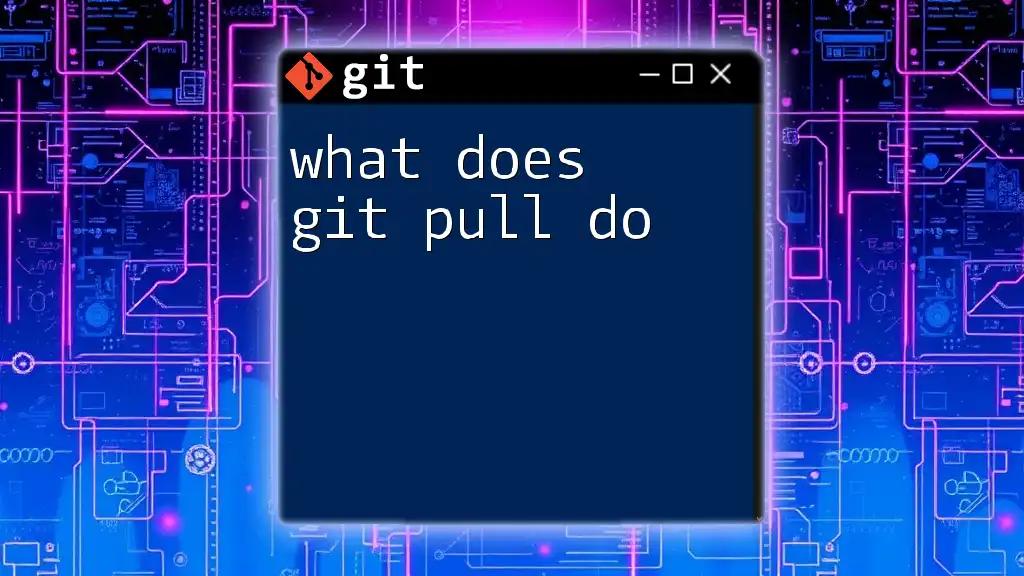
Solutions to the Lock Ref Error
Solution 1: Removing the Lock File
One of the most straightforward solutions is to remove the lock file manually. Locate the specific lock file that is causing the issue and delete it. You can usually find the lock files within the `.git/refs/heads/` directory. To do this, execute the following command:
rm -f .git/refs/heads/branch_name.lock
This operation frees the reference from its locked state, allowing you to proceed with your `git pull` again.
Solution 2: Checking Permissions
Sometimes, the lock ref error arises from permission issues. To resolve it, ensure you have the proper permissions on your repository directory. A quick way to grant write access is by running:
chmod -R u+w .git/refs/heads/
This command adjusts the permissions and should resolve any issues related to file accessibility.
Solution 3: Ensuring No Concurrent Git Processes
Running multiple Git processes simultaneously can lead to the lock ref error. To check for any active Git processes, use the `ps` command and identify any running Git activities. If you find any, make sure to terminate them safely before attempting another `git pull`.
Solution 4: Clone the Repository Again
If the previous solutions do not resolve the error, or if you continue to experience issues, consider cloning the repository again. This approach effectively starts you fresh, without any lingering problems from the original clone. Use the command:
git clone repository_url
Bear in mind that this may not be necessary for minor issues, but it serves as a last resort for persistent errors.

Preventing the Error
Best Practices for Using Git
To prevent encountering the error lock ref during git pull in the future, adhere to these best practices:
- Ensure that you are not performing multiple Git operations simultaneously, especially when collaborating with others.
- Regularly maintain your repository by cleaning up branches and unused references.
Regular Maintenance
Incorporate regular checks and cleanups into your workflow to maintain a healthy Git repository. Use the following commands:
git remote prune origin
This command will remove any old remote-tracking branches that no longer exist on the remote.
git gc
This command performs garbage collection on the repository, optimizing performance.
Collaborating with Others
When working in a team, communication is key. Ensure all developers are aware of ongoing operations to prevent simultaneous pulls. Consider setting guidelines that promote clarity on who is working on what, reducing the likelihood of conflicts.
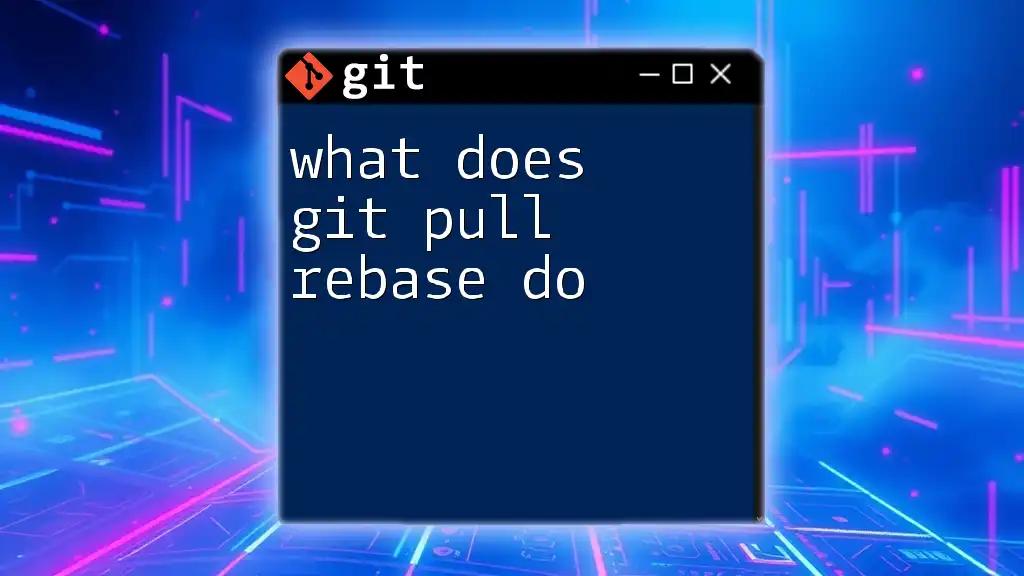
Conclusion
The error lock ref during git pull can hinder your workflow, but understanding its causes and solutions enables you to resolve it effectively. By adhering to best practices and performing regular maintenance, you can prevent its occurrence in the future. Collaboration in a team environment is critical; by communicating effectively, you ensure smoother operations and fewer disruptions.

Additional Resources
For further reading and comprehensive Git documentation, consider checking out:
- [Pro Git Book](https://git-scm.com/book/en/v2)
- [Official Git Documentation](https://git-scm.com/doc)
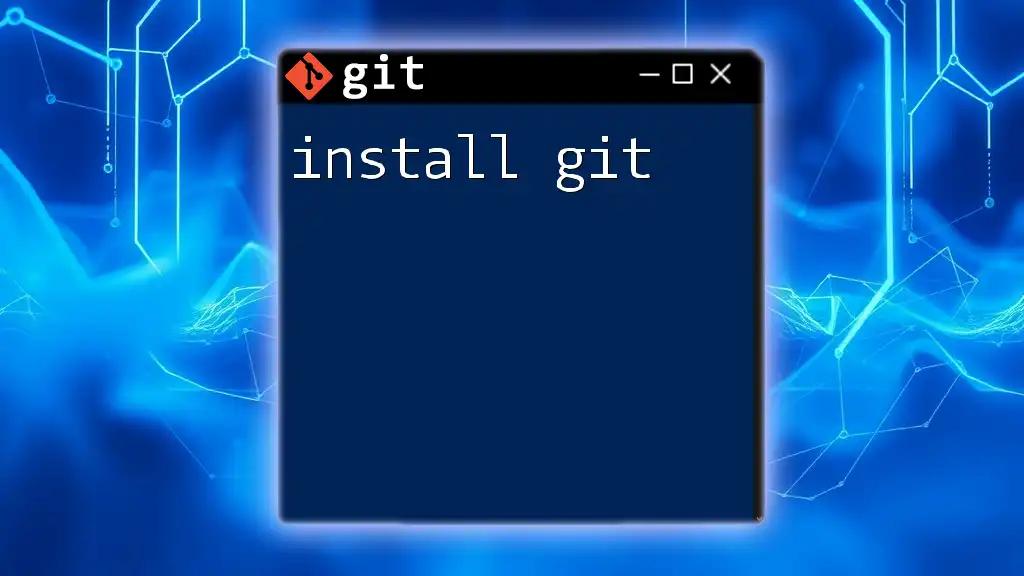
FAQ Section
What should I do if the error persists after trying the solutions?
If the error continues, investigate potential repository corruption, check your environment for conflicts, or consult Git’s community forums for assistance.
Can this error affect my commits?
Yes, the lock ref error can prevent you from pulling the latest changes, which may hinder your ability to perform subsequent commits correctly.
Are there any risks in manually deleting lock files?
While deleting lock files usually poses minimal risk, ensure that no other Git operations are ongoing to avoid data loss or corruption. Always double-check that you are removing the correct lock file before proceeding.