A Git primer is an introductory guide that provides essential commands and concepts to help beginners effectively manage version control in their projects.
Here's a simple code snippet to get you started with Git:
# Initialize a new Git repository
git init
# Add a file to the staging area
git add filename.txt
# Commit changes with a message
git commit -m "Initial commit"
What is Git?
Git is a distributed version control system that allows developers to track changes in their code over time. It enables coordination among multiple collaborators, making it an essential tool for software development. Created by Linus Torvalds in 2005, Git has evolved into one of the most popular systems worldwide due to its speed, flexibility, and powerful features.
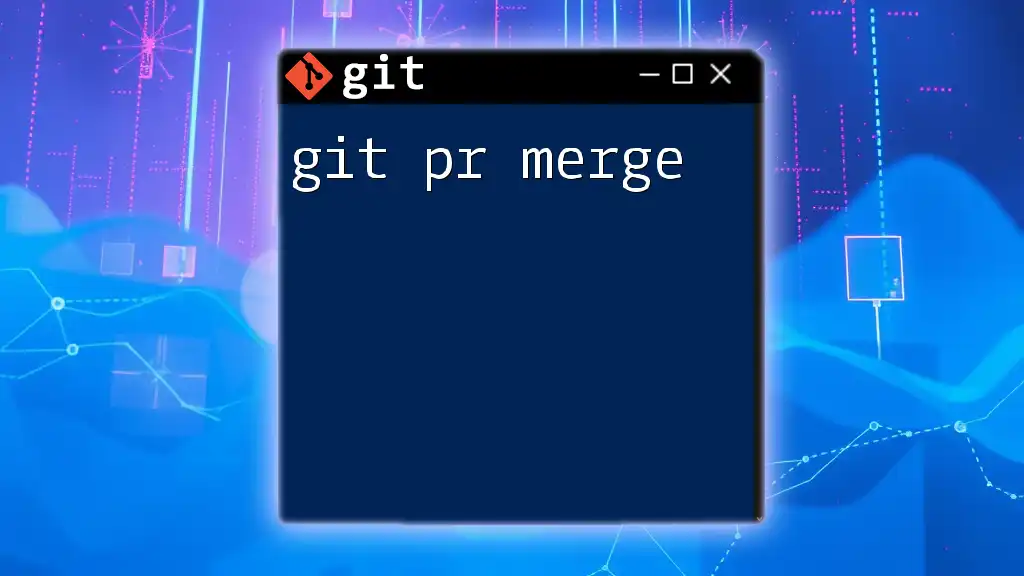
Why Use Git?
Using Git offers numerous advantages such as:
- Collaboration: Multiple developers can work on a project simultaneously without overwriting each other's work.
- Version Tracking: Git maintains a history of changes, allowing users to review past versions of their code easily.
- Branching and Merging: Developers can create separate branches of a project, experiment, and merge changes back into the main codebase seamlessly.
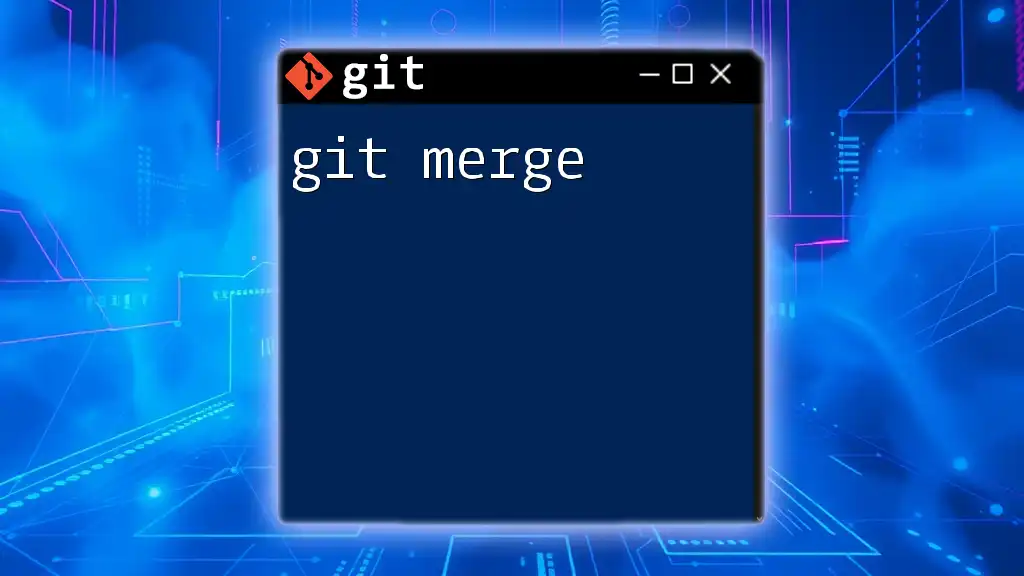
Getting Started with Git
Installing Git
To start using Git, you first need to install it on your system.
Downloading Git
You can download Git from its [official website](https://git-scm.com/downloads), which provides versions for Windows, macOS, and Linux.
Installation Process
-
Windows: Download the installer and follow the on-screen prompts.
-
macOS: You can install Git using Homebrew with the command:
brew install git
-
Linux: Use your package manager (e.g., `apt` for Debian-based systems) to install Git:
sudo apt-get install git
Configuration
After installation, it’s essential to configure Git with your personal information. This helps in tracking who made which changes. Use the following commands:
git config --global user.name "Your Name"
git config --global user.email "youremail@example.com"
This will set your name and email globally for all Git repositories on your machine.
Basic Concepts
Repositories
A repository (or repo) is where your project’s files and version history are stored. There are two types of repositories:
- Local Repository: This is on your machine and contains the files and history.
- Remote Repository: This is usually hosted on a server (e.g., GitHub, GitLab) and allows collaboration.
You can create a new local repository with:
git init my-repo
This initializes a new directory named `my-repo` as a Git repository.
Commits
Commits are snapshots of your project’s changes. Each commit records what changes were made, when, and by whom. You can make your first commit by staging files and committing them:
git add file.txt
git commit -m "Initial commit"
Make sure to write meaningful commit messages to document the changes clearly.
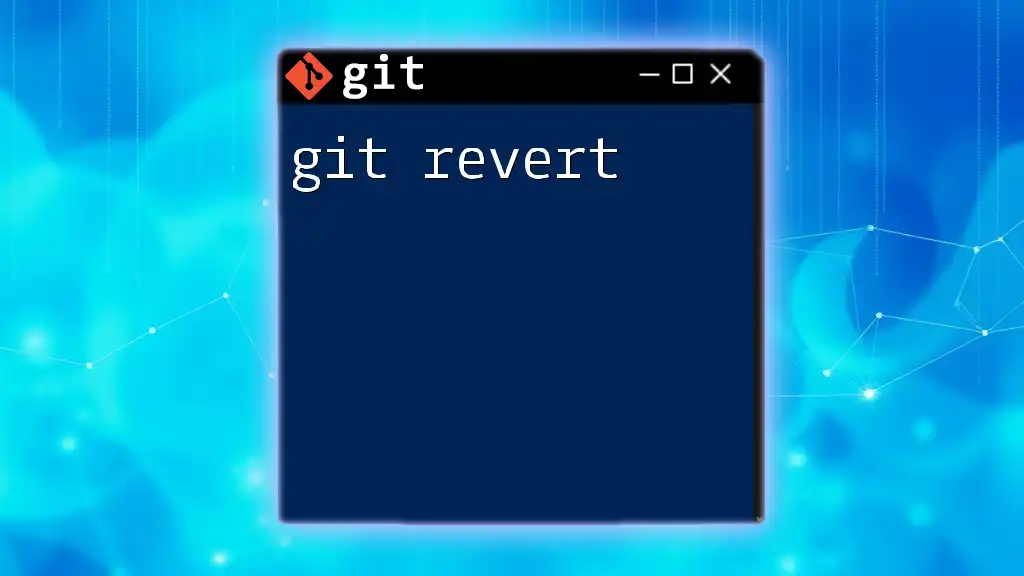
Essential Git Commands
Working with Repositories
Cloning a Repository
You can clone an existing remote repository using:
git clone https://github.com/user/repo.git
This command creates a local copy of the repository on your computer, including its full history.
Checking Repository Status
To see the current status of your repository, including staged, unstaged, and untracked files, use:
git status
This command is essential for understanding the state of your work before making commits.
Modifying Files
Adding Changes
The `git add` command stages modified files before committing. For example, to stage multiple files, you can use:
git add file1.txt file2.txt
Committing Changes
Commit your staged changes using the `git commit` command, making sure to include a clear message indicating the purpose of the commit.
git commit -m "Added new feature"
Branching and Merging
Understanding Branches
Branches allow you to diverge from the main codebase to work on new features without affecting the main project. This is especially useful when multiple features or bug fixes are being developed simultaneously.
Creating Branches
To create a new branch, use:
git branch new-feature
Switching Between Branches
You can switch to an existing branch with:
git checkout new-feature
With recent versions of Git, you can also use:
git switch new-feature
Merging Changes
After making changes to a branch, you can merge them back into the main branch (often called `main` or `master`) with:
git checkout main
git merge new-feature
This command applies the changes from `new-feature` to the `main` branch.
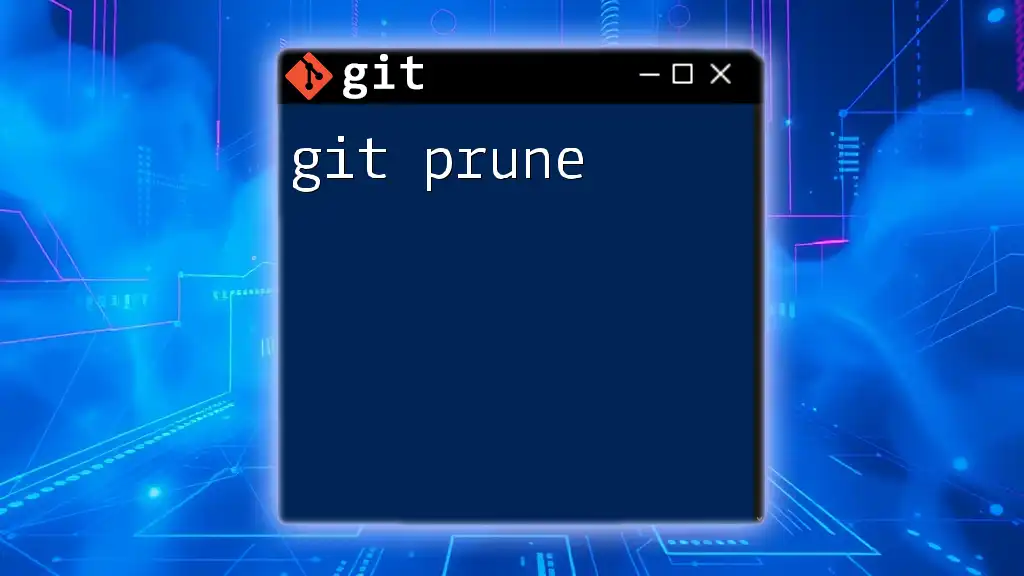
Collaboration with Git
Remote Repositories
Adding Remote Repositories
Link your local repository to a remote one by using:
git remote add origin https://github.com/user/repo.git
This sets `origin` as the name of your remote repository.
Pushing and Pulling Changes
Pushing Changes
To upload your commits to the remote repository, use:
git push origin main
This command sends your local changes to the `main` branch of the remote repo.
Pulling Changes
If you've collaborated with others, you need to fetch and integrate changes from the remote repository using:
git pull origin main
This command pulls the latest changes to your local branch.
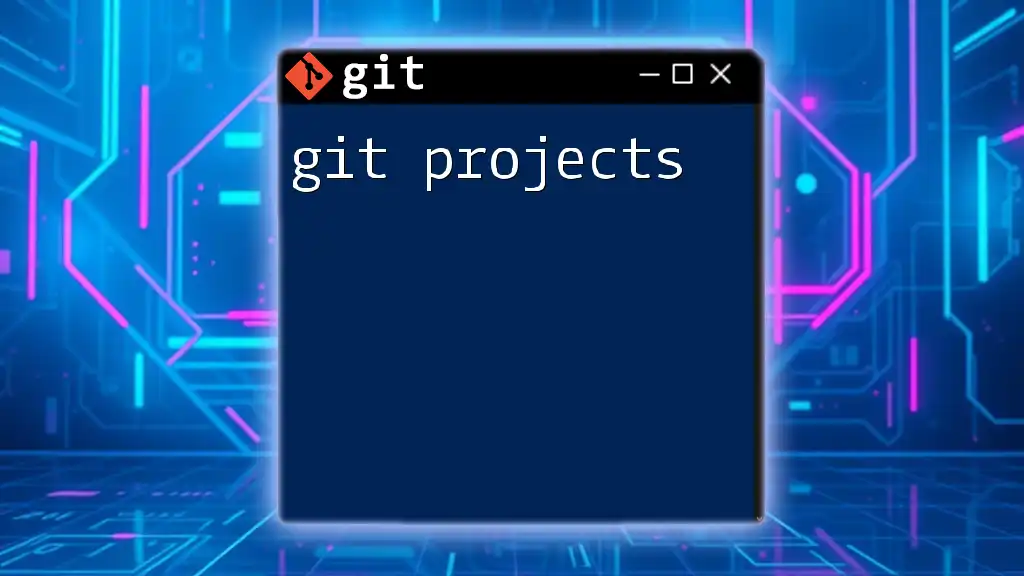
Advanced Git Features
Stashing Changes
What is Stashing?
Sometimes you may want to save your progress without committing. Stashing lets you temporarily set aside changes.
Using Git Stash
You can stash your changes using:
git stash
To apply the stashed changes later, use:
git stash apply
Handling Merge Conflicts
What is a Merge Conflict?
A merge conflict occurs when two branches have changes that conflict with each other. During merging, Git cannot automatically reconcile these changes.
Resolving Merge Conflicts
To resolve conflicts, Git will mark the conflicting files. You need to manually edit these files to resolve discrepancies, then stage the resolved files:
git add resolved-file.txt
git commit -m "Resolved merge conflict"
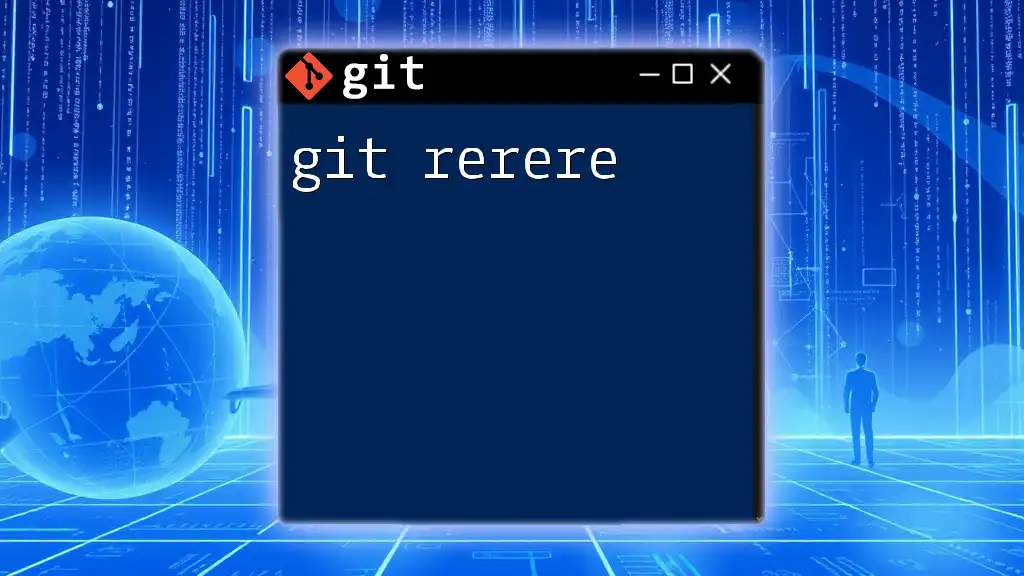
Best Practices for Using Git
Writing Clear Commit Messages
A good commit message clearly describes what changed and why. Consider using the imperative mood (e.g., "Fix typo") and keep them concise.
Keeping Commits Small and Focused
Smaller, focused commits make it easier for others (and yourself) to review changes. Aim to commit one logical change at a time.
Regularly Pushing Changes
Submit your changes to the remote repository frequently to prevent data loss and to keep your work backed up.
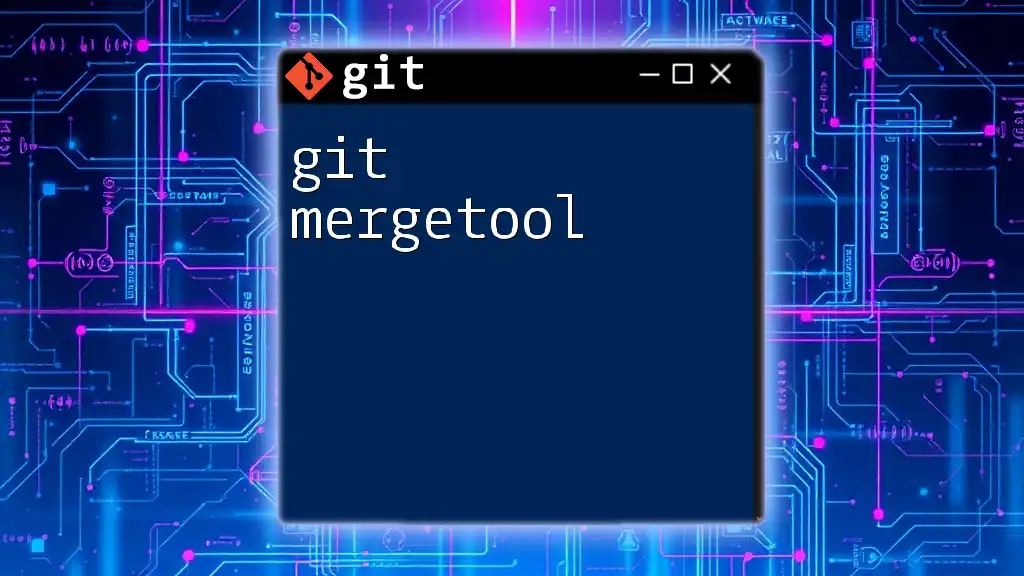
Conclusion
In this Git primer, we covered fundamental concepts and commands that are essential for managing projects with Git. By understanding how to initialize repositories, manage branches, and collaborate with others, you can leverage Git's power effectively.
Encouragement to practice these concepts using your projects will reinforce your understanding and make you proficient in Git.
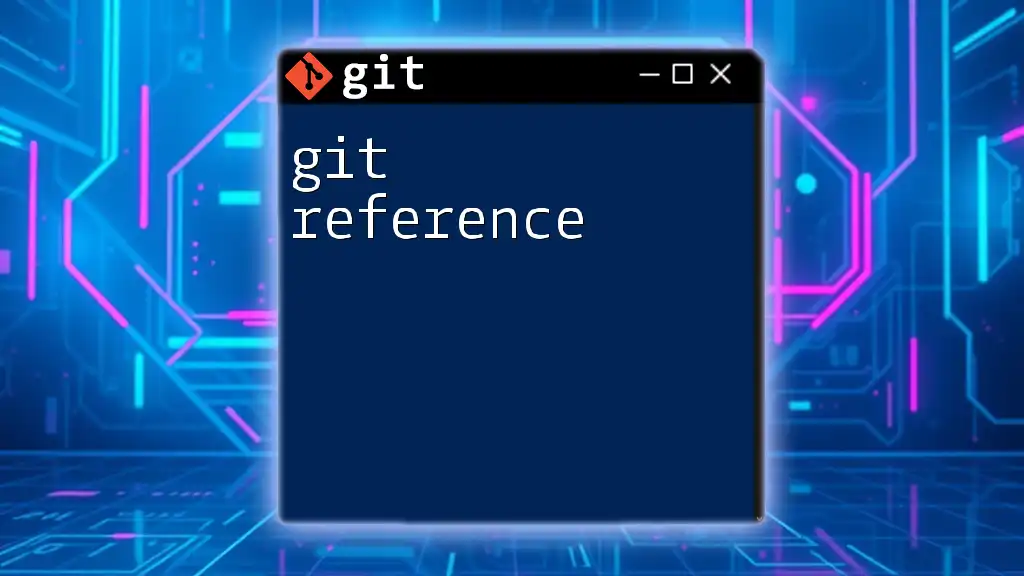
Additional Resources
For further learning, consider exploring the [official Git documentation](https://git-scm.com/doc), online tutorials, recommended books, and communities where you can ask questions and share knowledge.