"CI Git" refers to the integration of Git with Continuous Integration (CI) tools, enabling automatic testing and deployment of code changes, typically using Git commands to manage version control in the CI workflow.
Here's a simple example of how to use Git commands in a CI pipeline to push changes:
git add .
git commit -m "Update code"
git push origin main
What is Git?
Git is a distributed version control system designed to handle everything from small to very large projects with speed and efficiency. At its core, Git allows developers to track changes in code, enabling them to collaborate on projects effectively. By versioning their work, developers can experiment with new features, revert to previous versions, and manage multiple branches of development without losing any progress.
Understanding key terms associated with Git will form the foundation of using it effectively, especially in CI/CD practices:
- Repository (repo): A storage space for your project where all the files and their history are kept.
- Commit: A snapshot of your project at a specific point in time, along with a message describing changes.
- Branch: A parallel version of the repository, allowing developers to work on features independently.
- Merge: The act of combining changes from different branches together.
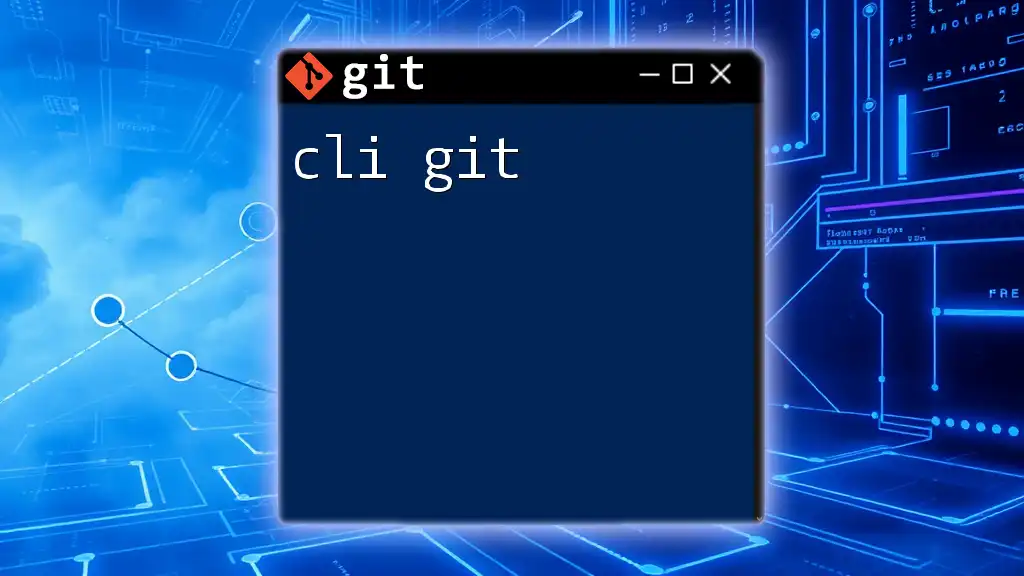
Integrating Git with CI/CD
The CI/CD Pipeline
The Continuous Integration (CI) and Continuous Deployment (CD) pipeline has become a vital part of modern development. CI refers to the practice of integrating code changes frequently, with automated builds and tests to ensure that errors are caught early in the development process. Continuous Deployment (CD) takes this a step further, automating the deployment of code to production once it has passed the necessary tests.
Each CI/CD pipeline typically includes three stages: Building, Testing, and Deployment. Understanding and mastering this pipeline with Git lays the groundwork for efficient development.
Setting Up a Git Repository for CI
Creating a new Git repository is the first step towards integrating CI into your workflow. You can do this quickly with the following command:
git init my-repo
cd my-repo
If you want to work on an existing project, you can clone a repository with:
git clone https://github.com/user/repo.git
This command allows you to access another developer's repo, fostering collaboration and knowledge sharing.
Choosing a CI Tool
Selecting the right CI tool is crucial for smooth integration with your Git repositories. Several popular CI tools are available, including:
- Jenkins: An open-source automation server that allows users to build, test, and deploy their applications.
- Travis CI: An integrated CI service used for GitHub projects, which is particularly user-friendly for new developers.
- CircleCI: Offers both cloud-hosted and on-premises solutions, with powerful insights.
- GitHub Actions: Built directly into GitHub, allowing you to automate workflows right from your repository.
Each tool has its strengths and weaknesses. While Jenkins offers customization, GitHub Actions may provide the most seamless experience for those already on GitHub.
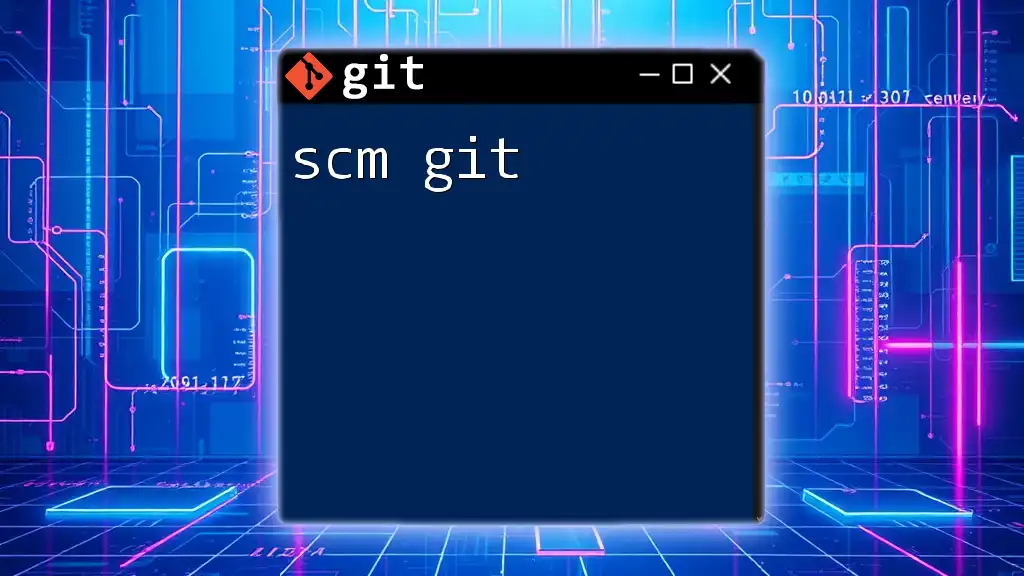
Configuring Git for CI
Basic Configuration for CI
Before diving deep into CI, proper Git configuration is essential. Ensure that your repository has a `.gitignore` file to avoid tracking unnecessary files, such as logs or node modules. The content might look like this:
# Ignore node_modules directory
node_modules/
This configuration helps maintain a clean repository, making CI operations smoother.
Setting Up a CI Configuration File
CI systems typically rely on configuration files that define the steps the CI tool should take upon detecting changes in the repository. For example, a configuration file for a Node.js project using Travis CI might look like:
language: node_js
node_js:
- "14"
script:
- npm install
- npm test
This configuration specifies the Node.js version and installs dependencies, followed by running tests, ensuring that your application is reliable.
Developing a CI Workflow
Writing Tests
One of the main purposes of CI is to ensure that your code is functioning as intended. Writing tests is crucial for this. For example, if you’re using the Jest framework for testing, you might write a simple test like this:
test('adds 1 + 2 to equal 3', () => {
expect(1 + 2).toBe(3);
});
This straightforward test checks basic functionality, and running tests automatically during CI will catch issues as soon as they arise.
Building the Application
After testing, building the application is essential to prepare it for deployment. A simple command to run your build script in a Node.js environment might be:
npm run build
This process often includes compiling code, optimizing assets, and preparing the application for production.
Running CI Jobs
CI jobs are automatically triggered by actions such as code pushes or pull requests. A successful job indicates that the code changes have passed all defined tests and checks. CI tools provide logs and status indicators, allowing developers to quickly assess build outcomes and identify any issues.
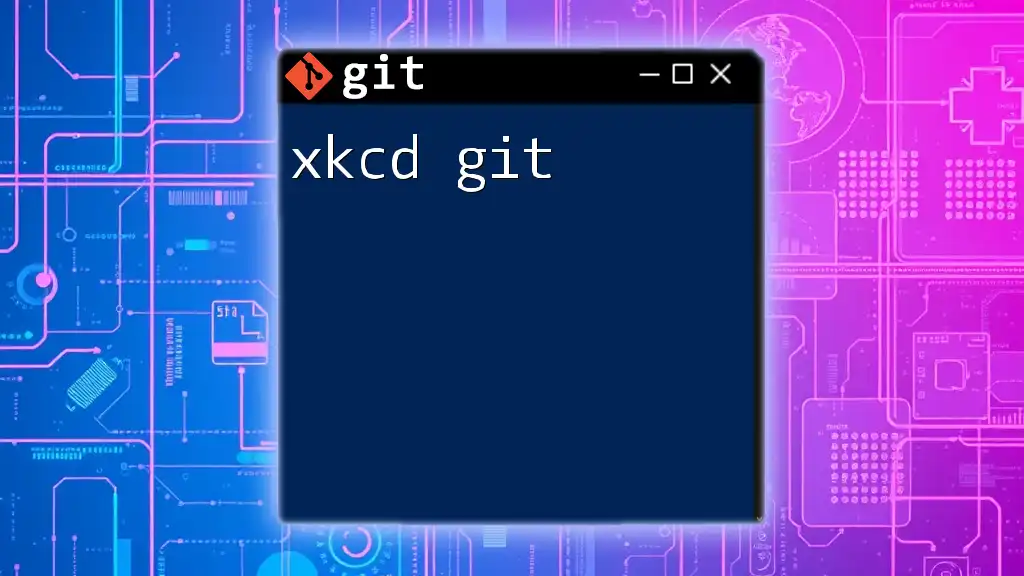
Common CI Workflows with Git
Integration with GitHub Actions
GitHub Actions provides a built-in mechanism to implement CI/CD workflows directly in your GitHub repository. A sample configuration file located at `.github/workflows/ci.yml` could look like this:
name: CI
on: [push, pull_request]
jobs:
build:
runs-on: ubuntu-latest
steps:
- name: Checkout code
uses: actions/checkout@v2
- name: Set up Node.js
uses: actions/setup-node@v2
with:
node-version: '14'
- name: Install Dependencies
run: npm install
- name: Run Tests
run: npm test
This concise workflow activates on any push or pull request, checks out the code, sets up the environment, installs the dependencies, and runs tests. This automation streamlines the development process.
Common Pitfalls in CI with Git
While using CI with Git has huge advantages, there are common pitfalls to be aware of:
- Misconfiguring CI files: Ensure that the syntax and commands are correct, as errors can stop the workflow.
- Not running tests: If tests are skipped, you may unknowingly integrate faulty code. Always configure CI to run tests.
- Ignoring dependencies: Failing to install or update dependencies can lead to compatibility issues in your application.
By being aware of these pitfalls, you can design a more effective CI process.
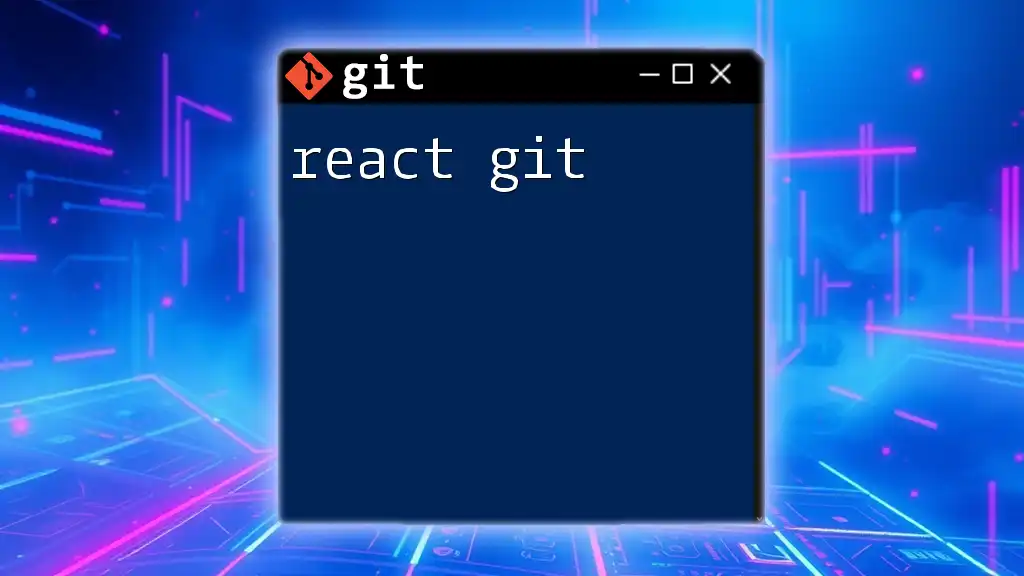
Best Practices for CI with Git
Version Control Principles
Following best practices is key to maximizing your CI efforts. Commit changes early and often to maintain a comprehensive history of your work. Keeping your branches small and focused allows for rapid code reviews and easier conflict resolution.
Maintaining a Clean CI Pipeline
Establishing a fast and reliable CI pipeline is crucial. Regularly monitor and refine your processes to identify bottlenecks or outdated practices. Prioritize failing builds to protect the quality of your code.
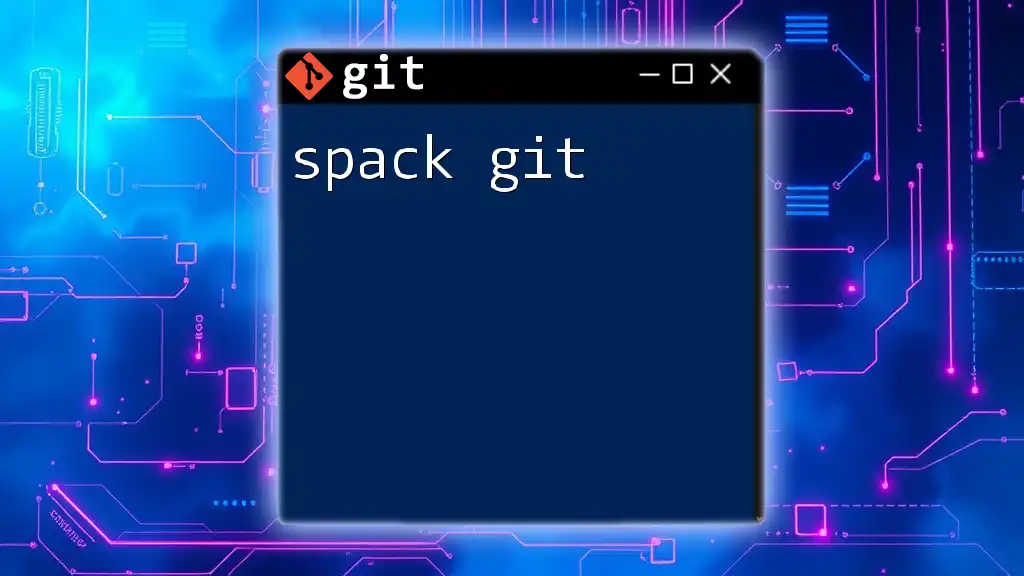
Conclusion
Embracing CI with Git symbolizes a commitment to quality and collaboration in software development. By integrating the CI process into your workflow, you enhance productivity, encourage more frequent deployments, and ultimately deliver higher-quality software products. Use the outlined strategies and insights to structure a robust CI pipeline that aligns with your team's goals.
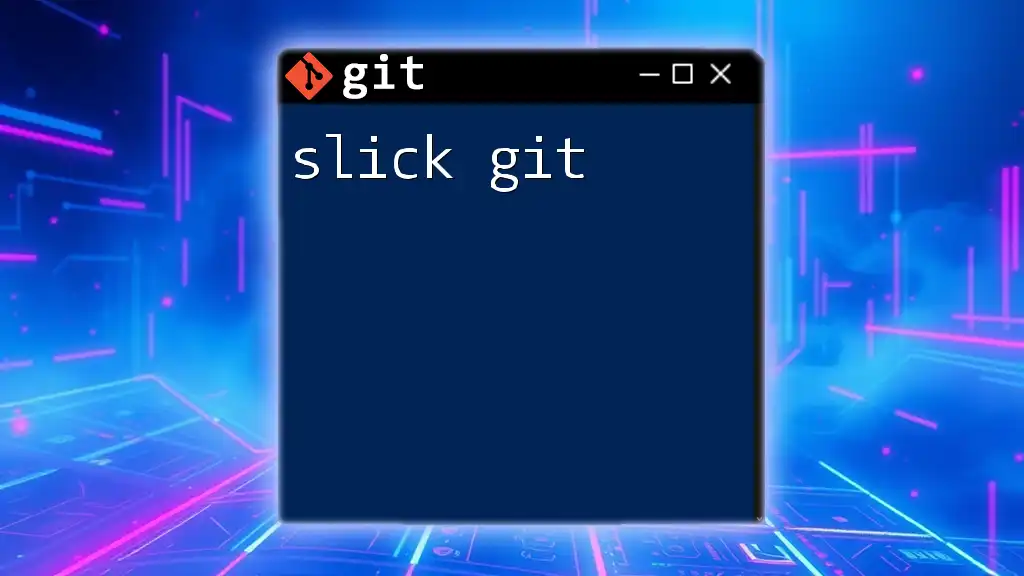
Additional Resources
To further deepen your understanding of CI with Git, explore the following resources:
- Official documentation for tools like Travis CI, Jenkins, CircleCI, and GitHub Actions.
- Online tutorials and courses focused on Git and CI/CD practices.
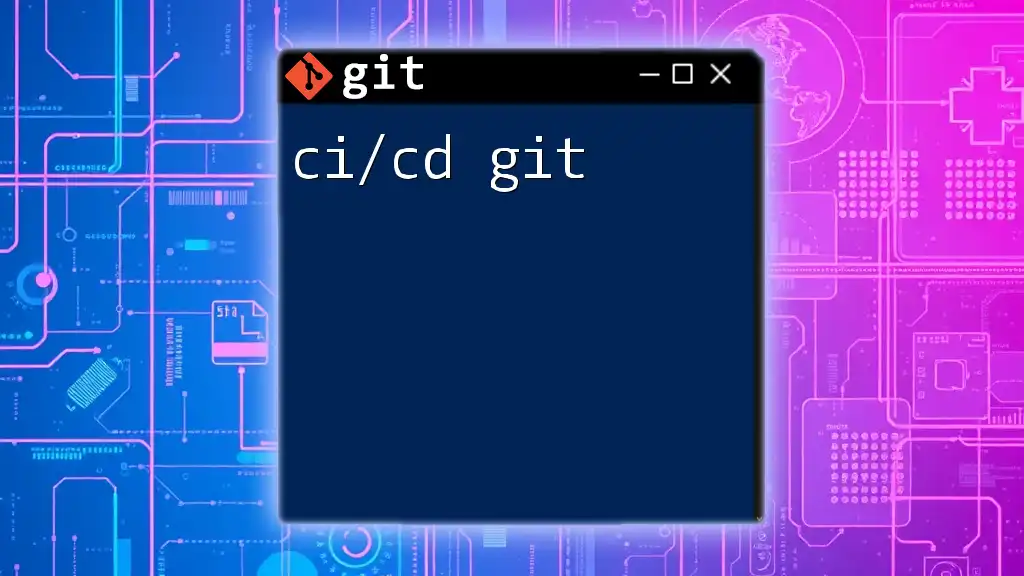
FAQs
What happens if a CI build fails?
If a CI build fails, it usually indicates an issue with the code or the configuration. Developers should promptly review the logs to identify problems, fix them, and re-run the build.
How can I test my configurations locally before pushing?
You can test configurations locally using tools like Docker or executing build scripts in a local environment, providing a safe way to verify everything is functioning as intended.
What are the costs associated with CI tools?
Many CI tools offer both free tiers and premium plans. Costs can vary based on usage, the number of builds, and features. Always check the pricing details for the specific tool you choose.