React Native Git refers to using Git version control to manage the source code and collaboration of React Native applications efficiently.
Here's a quick Git command snippet to initialize a new React Native project:
npx react-native init MyProject
cd MyProject
git init
git add .
git commit -m "Initial commit of React Native project"
Setting Up Your Environment
Prerequisites
Before diving into using React Native Git, ensure you have the following installed:
- Node.js: The JavaScript runtime used to run React Native.
- npm: The package manager that comes with Node.js, which will help you install the React Native CLI.
- React Native CLI: This command-line interface allows you to create and manage React Native projects.
Installing Git
Git is a critical tool for version control. Here’s how to install it depending on your operating system:
-
Windows:
- Download the installer from the [official Git website](https://git-scm.com/download/win) and follow the prompts.
-
macOS:
- You can install Git via Homebrew with the command:
brew install git
- You can install Git via Homebrew with the command:
-
Linux:
- Using apt package manager, run:
sudo apt-get install git
- Using apt package manager, run:
Setting Up a New React Native Project
After installing the required tools, create a new React Native project by running:
npx react-native init MyProject
This initializes a new React Native application in a folder named `MyProject`.
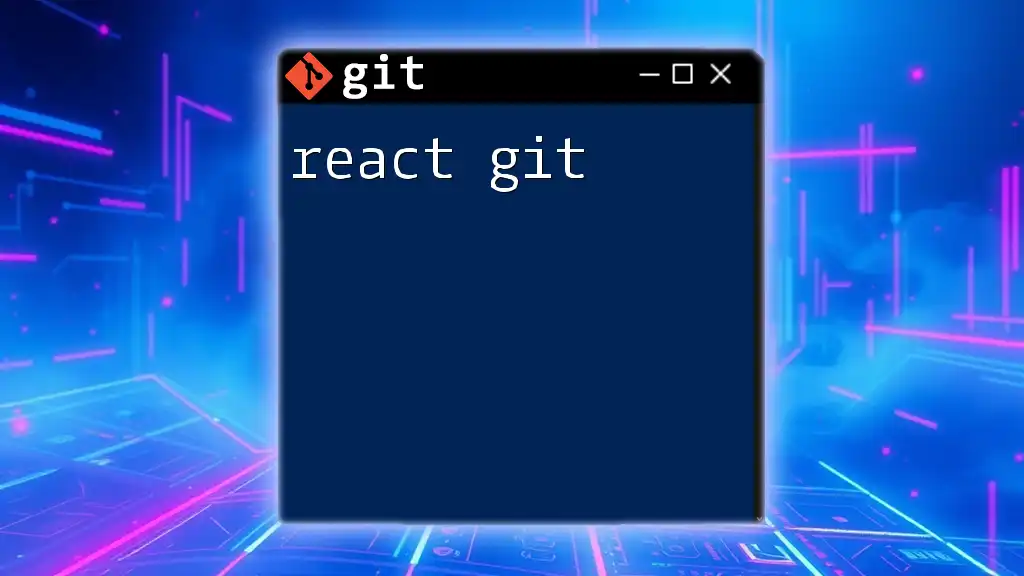
Basic Git Commands for React Native Development
Creating a New Repository
To start version control for your new project, navigate into the project directory and initialize a Git repository:
cd MyProject
git init
This command creates a hidden `.git` folder that holds all your version history.
Cloning an Existing Repository
If you want to work on an existing project, you can clone it from a remote repository using:
git clone <repository-url>
This action downloads all the project files and initializes a repository in your local directory.
Staging Files to Commit
Before you can save changes to your repository, you need to stage them. To add a particular file, use:
git add <file-name>
To stage all changes (both tracked and new files):
git add .
Staging allows you to selectively commit only the changes you want to include.
Committing Changes
Once you have staged your files, it's time to commit them. A commit is a snapshot of your project at a point in time. Use the following command:
git commit -m "Your commit message"
Choose a meaningful message that describes the changes made, which is crucial for project clarity.
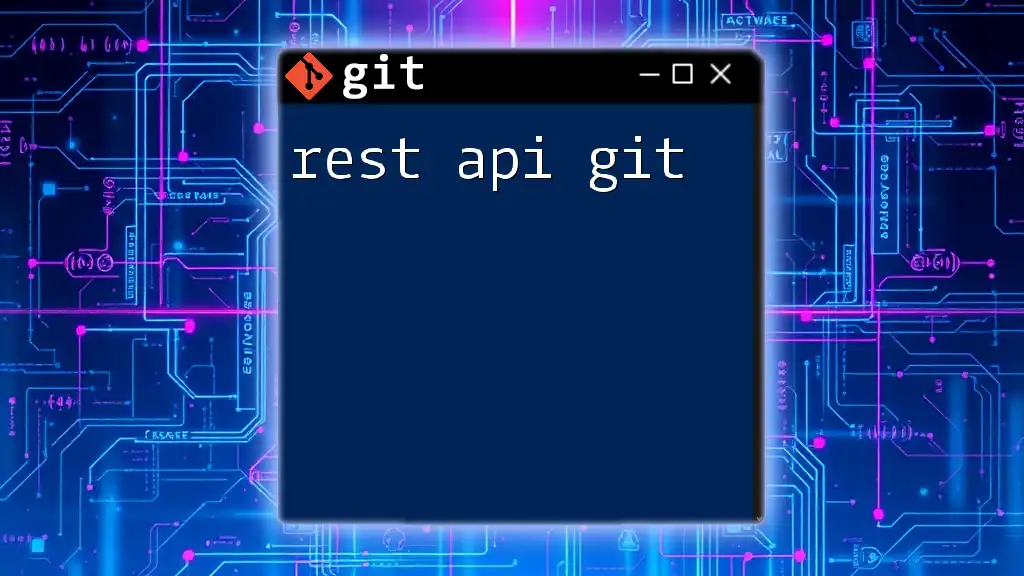
Branching and Merging
Understanding Branches
Branches are an essential concept in Git, enabling you to work on features without affecting the main project. The default branch is usually `main`.
Creating a New Branch
To create a new branch, use:
git branch <branch-name>
This command allows you to work independently without impacting the main branch until your changes are ready to be merged.
Switching Branches
To switch to your newly created branch, run:
git checkout <branch-name>
Now, you can work on your new feature or bug fix isolated from the main codebase.
Merging Branches
After completing your work in the feature branch, you may want to merge it back into the main branch. First, switch to the main branch:
git checkout main
Then, merge the changes using:
git merge <branch-name>
If there are any conflicts, Git will prompt you to resolve them.
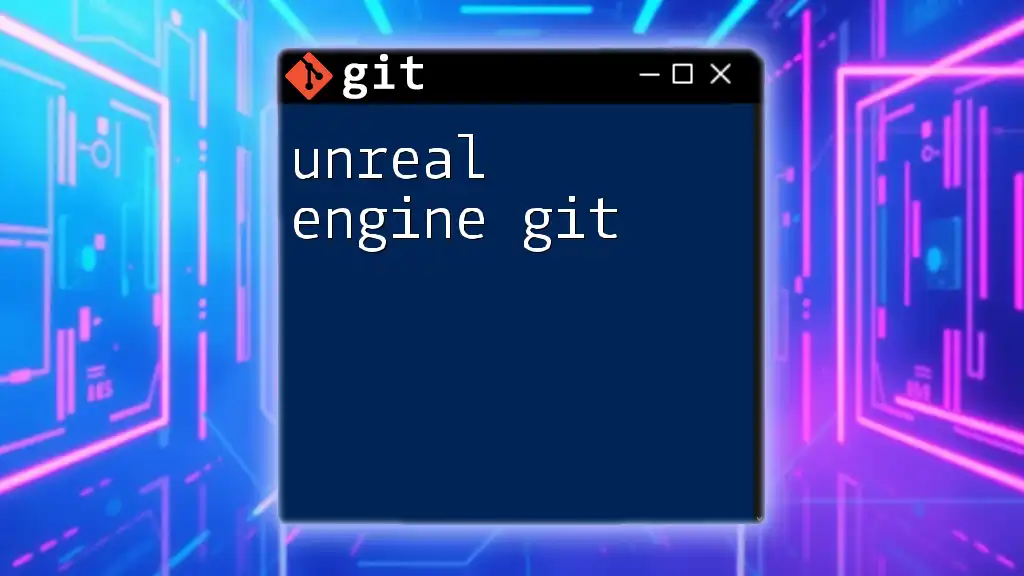
Working with Remote Repositories
Adding a Remote Repository
To connect your local Git repo to a remote one (like GitHub), run:
git remote add origin <repository-url>
This command sets up a name (`origin`) that refers to the remote repository.
Pushing Changes to Remote
Once you make local commits, push them to the remote repository with:
git push origin <branch-name>
This command uploads your committed changes and keeps the remote repository updated.
Pulling Changes from Remote
To fetch and merge changes made by others in the remote repository, use:
git pull origin <branch-name>
This action ensures that your local branch is consistent with the remote.
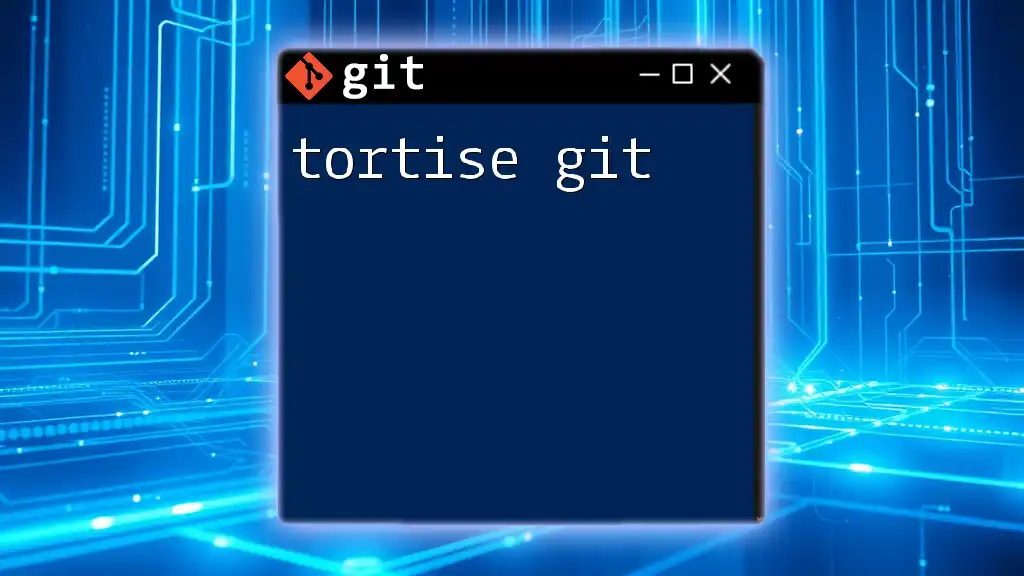
Handling Merge Conflicts in React Native
Merge conflicts arise when changes from different branches affect the same parts of a file. When you merge and Git encounters conflicts, it will mark the issue and prompt you to resolve it.
Identifying Merge Conflicts
You can identify conflicts with markers within the affected files, which will look something like this:
<<<<<<< HEAD
// Your changes
=======
// Changes from the other branch
>>>>>>> branch-name
Edit the file to combine the necessary changes and remove the conflict markers.
Resolving Merge Conflicts
After resolving, make sure to stage and commit the resolved files:
git add <conflicted-file>
git commit -m "Resolved merge conflicts"
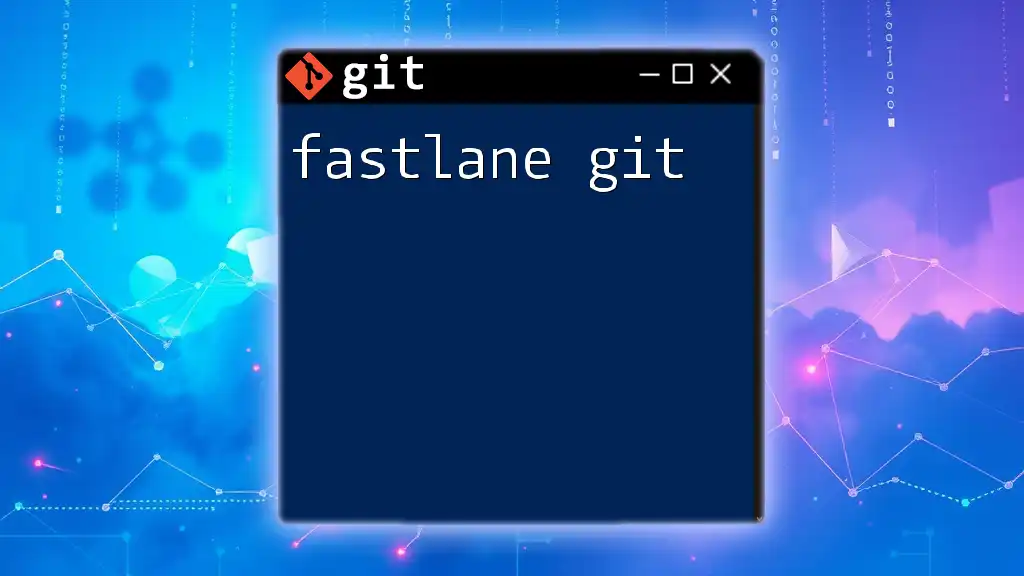
Best Practices for Git in React Native Projects
Writing Meaningful Commit Messages
A well-written commit message can improve your team's workflow. Follow this format:
- Short summary of changes (50 characters or less)
- Include additional details or rationale (if necessary)
Making Use of `.gitignore`
To prevent unnecessary files from being tracked, create a `.gitignore` file in your project root. For React Native, an example would be:
node_modules/
*.log
.env
This ensures that files like `node_modules` are excluded from version control, saving space and avoiding clutter.
Regularly Pulling Changes
Regularly pull changes from the main branch to minimize merge conflicts. This keeps your local repository up-to-date and reduces integration issues.
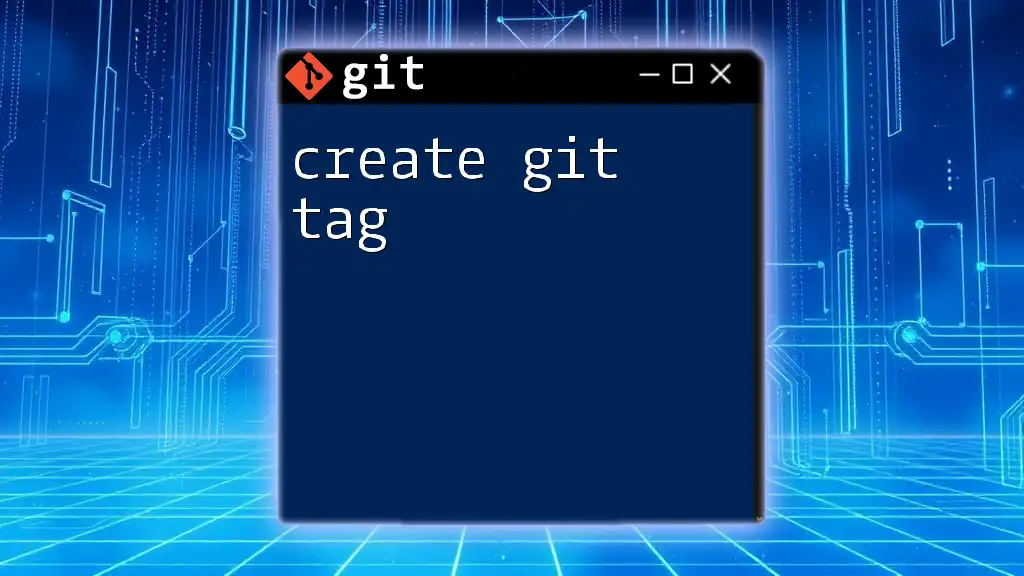
Advanced Git Techniques
Rebasing
Rebasing allows you to streamline your commit history by moving or combining commits from one branch onto another. Use this command to rebase:
git rebase <branch-name>
Rebasing is particularly useful when integrating feature branches before merging them to eliminate unnecessary merge commits.
Cherry-Picking Commits
Cherry-picking enables you to apply specific commits from one branch to another without merging the entire branch. Use:
git cherry-pick <commit-hash>
This is helpful when you want specific changes from another branch without merging all the changes.
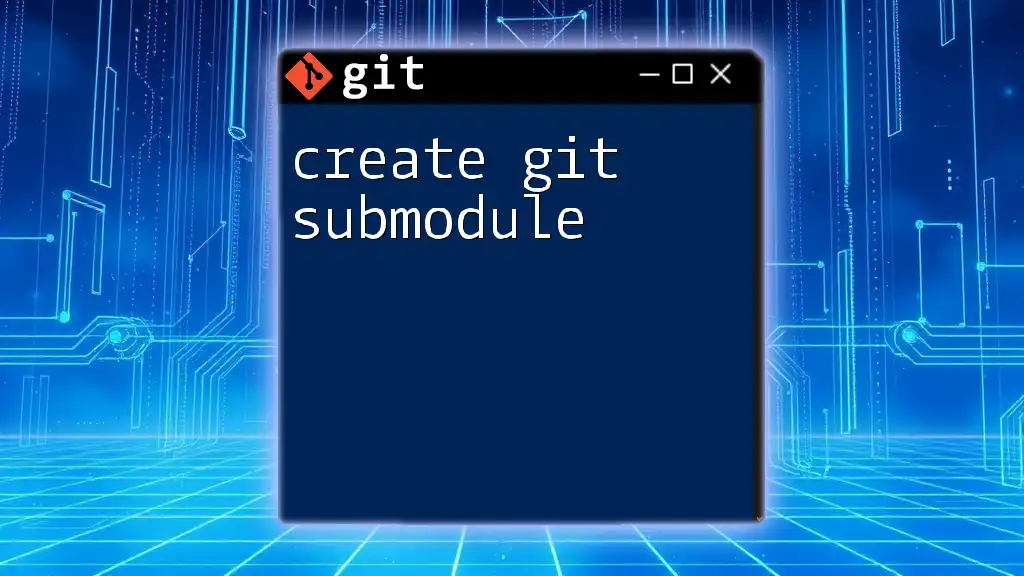
Conclusion
This guide provides a comprehensive overview of how to use React Native Git for effective version control in your mobile app projects. Emphasizing best practices and essential commands, you can streamline your development process and collaborate efficiently. Regularly revisiting and practicing these concepts will enhance your proficiency in Git.
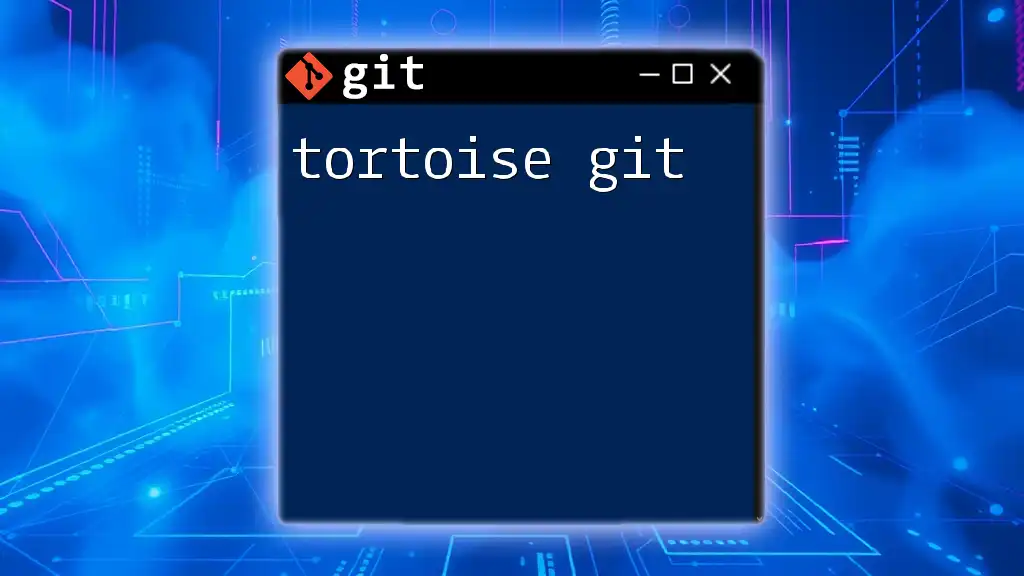
Frequently Asked Questions (FAQ)
What if I mess up my Git repository?
If things go wrong, you can always use:
git reset --hard HEAD
This command will revert your branch to the last committed state.
How can I undo a commit?
To undo your last commit but keep the changes, use:
git reset --soft HEAD~1
Can I use Git with other version control systems?
While Git is widely adopted, you can integrate it with other systems using various bridging tools. However, mastering Git is generally sufficient for most development needs.
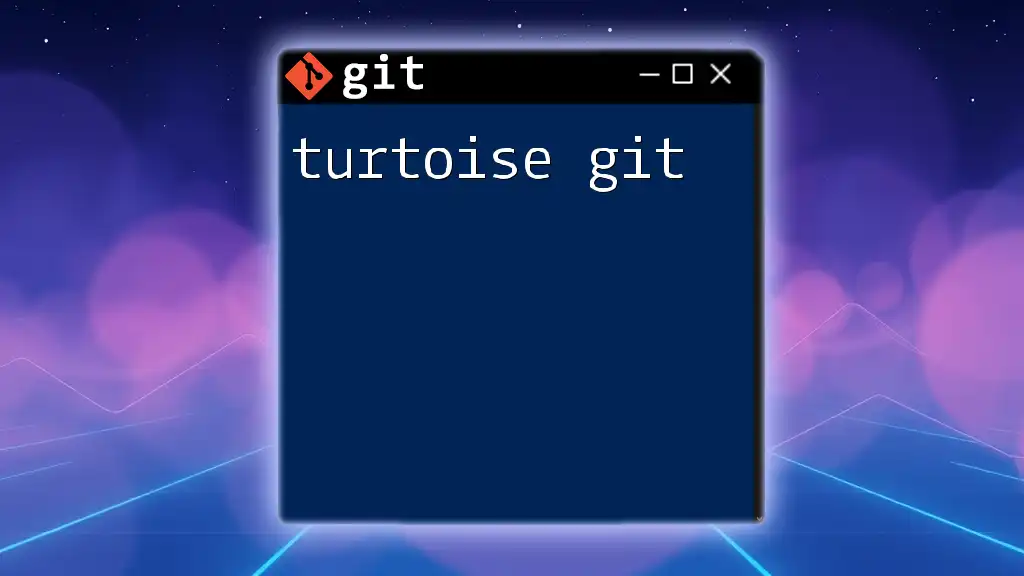
Additional Resources
For further learning, consider checking out:
- The official Git documentation.
- React Native development tutorials.
- Online courses focusing on version control and branching strategies.