To create a local Git repository, navigate to your project directory in the terminal and run the command `git init` to initialize an empty Git repository.
cd /path/to/your/project
git init
What You Need to Get Started
Understanding Git
Git is a version control system that allows developers to track changes in their code over time. It enables collaboration among multiple contributors, ensures that everyone is working on the most current version of the code, and allows for easy recovery of previous versions if needed. One of its most powerful features is the ability to create a local repository.
A local repository is the copy of a repository that resides on your machine. It's where you can make changes, experiment, and have complete control over your version of the project without affecting others until you're ready to share those changes.
Prerequisites
Before you start creating a local git repo, you need to ensure that you have the appropriate software installed:
-
Git: The first step is to download and install Git. You can find the installer on the [official Git site](https://git-scm.com/downloads). Follow the instructions based on your operating system (Windows, Mac, or Linux).
-
Command Line Interface (CLI): Familiarity with your terminal or command prompt is essential, as most Git operations are performed through these interfaces. Make sure you can open your terminal or command prompt and can execute commands.
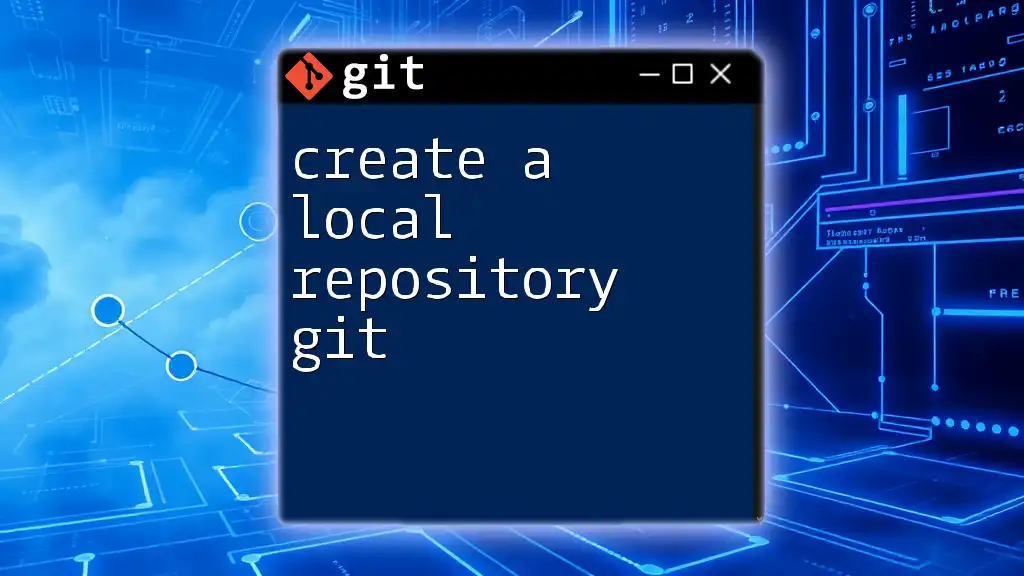
Setting Up Your Local Git Repository
Step 1: Installing Git
Windows Installation
To install Git on Windows, begin by downloading the installer from the official site. Once downloaded, launch the installer and follow the installation prompts. Make sure to select the option that allows you to use Git from the command prompt.
Mac Installation
For Mac users, the easiest way to install Git is through Homebrew. If you haven't installed Homebrew, you can do so with the following command:
/bin/bash -c "$(curl -fsSL https://raw.githubusercontent.com/Homebrew/install/HEAD/install.sh)"
Once Homebrew is installed, run:
brew install git
Linux Installation
On Linux, you can install Git using your distribution's package manager. For example, if you’re using Ubuntu, execute:
sudo apt-get install git
Step 2: Configuring Git
Setting Up Your Identity
Once Git is installed, it's essential to set up your identity so your contributions are correctly attributed to you. Use the following commands to configure your username and email:
git config --global user.name "Your Name"
git config --global user.email "your.email@example.com"
These configurations are stored globally, meaning they will apply to all your repositories. Having this information will play a crucial role in your commit history and collaboration with others.
Step 3: Creating a New Local Repository
Using the Command Line
Now that you have Git installed and configured, you can create a new local repository. Start by creating a directory for your project:
mkdir my-repo
cd my-repo
After navigating into your new project directory, initialize a new Git repository with:
git init
This command sets up the necessary files and folder structure for Git to track changes, allowing you to start managing versions of your project.
Step 4: Adding Files to Your Repository
Creating Files
With the repository initialized, it’s time to add files. For example, you may want to create a `README.md` file to describe your project:
touch README.md
Staging Files
Now that you have a file in your repository, you need to stage it for your first commit. Staging helps you prepare changes before finalizing them. To stage the `README.md` file, run:
git add README.md
Staging means you’re telling Git you want to include changes from this file in your next commit. This is a crucial step in the Git workflow.
Step 5: Making Your First Commit
The Commit Command
After staging files, you’ll want to commit the changes to your local repository. Use the following command:
git commit -m "Initial commit"
The `-m` option allows you to provide a short message describing your changes. It’s important to write a clear and meaningful commit message, as this will help others (and yourself) understand what changes were made and why.
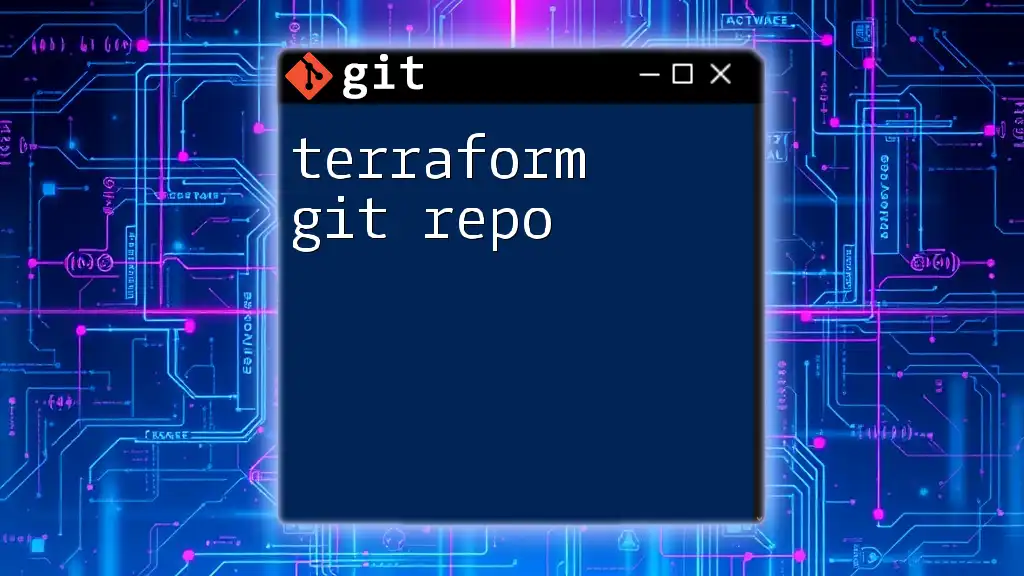
Working with Existing Projects
Cloning a Remote Repository
In some cases, you may want to work on a project that already exists online. This can be done by cloning a remote repository. Use the following command to clone a repository:
git clone https://github.com/user/repo.git
This command makes a complete copy of the selected repository on your local machine, including all its files, history, and branches, allowing you to start working on it right away.
Creating a Branch in Your Local Repository
Branches are essential for development workflows. They allow you to work on different features independently without affecting the main codebase. To create and switch to a new branch, use:
git checkout -b new-feature
This command creates a new branch called `new-feature` and switches you to it. Working with branches enhances collaboration and helps keep your main branch stable.
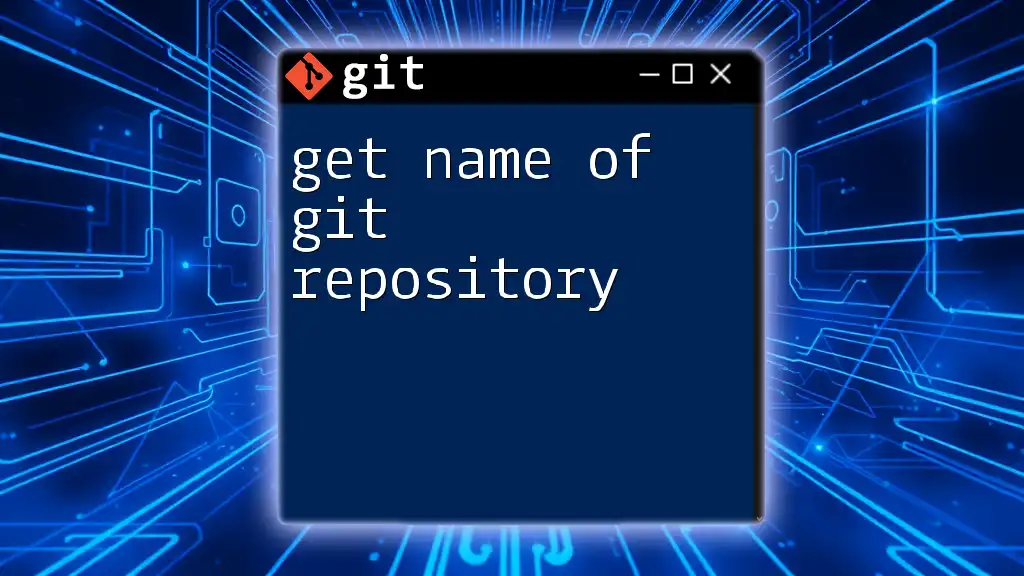
Common Git Commands for Local Repositories
Viewing the Status
To see the status of your files and whether they are staged for commit, you can use:
git status
This command provides valuable information about which files are being tracked, which are staged, and which have changes that haven’t been staged.
Viewing Commit History
To explore the history of your commits, use:
git log
This command displays a list of commits and can be essential for tracking changes in your project over time.
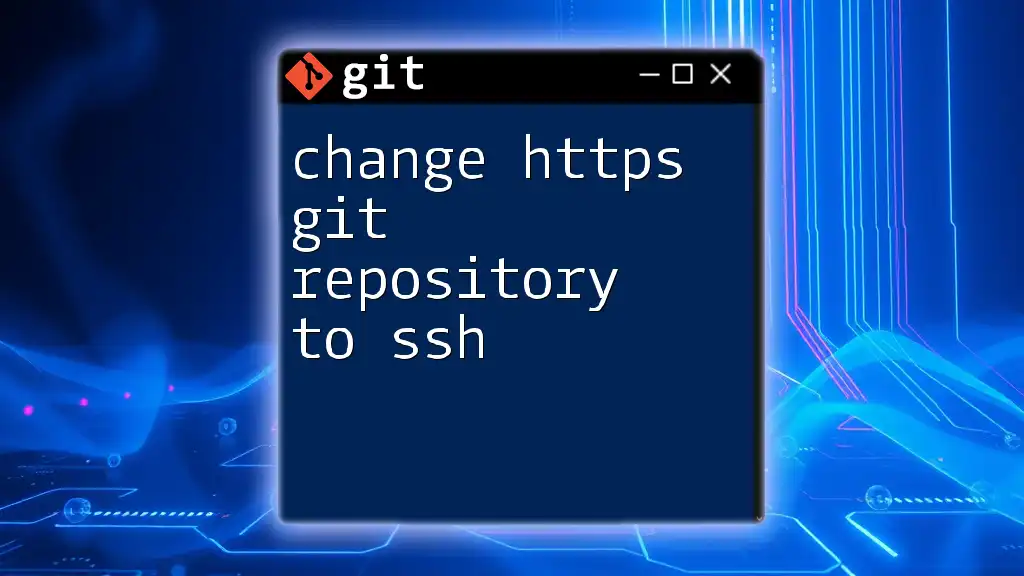
Troubleshooting
Common Issues and Solutions
While creating a local Git repository, you may encounter some issues. Here are a few common problems:
-
Git not found error: If your command line says that the `git` command is not recognized, check if Git is properly installed and that your system’s PATH variable includes the Git installation path.
-
Permissions issues: If you run into permission errors when trying to modify files in your repository directory, ensure that you have the required permissions for that directory. You might need administrative rights or to change the ownership of the directory.
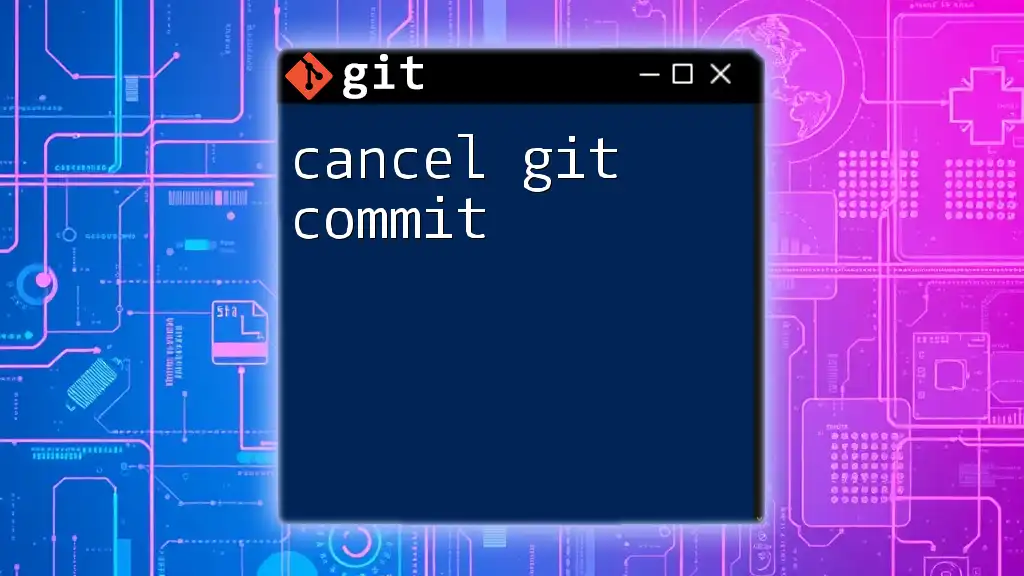
Conclusion
Creating a local Git repository is a fundamental skill for any developer. It allows you to manage your code efficiently, experiment safely, and contribute effectively to collaborative projects. By following the steps outlined in this guide, you're now equipped to set up your own local repository and start harnessing the benefits of version control with Git.
Take the opportunity to explore further commands and features in Git, as they can significantly enhance your workflow. Remember, practice makes perfect, and the more you use Git, the more comfortable you'll become with its commands and functionalities.
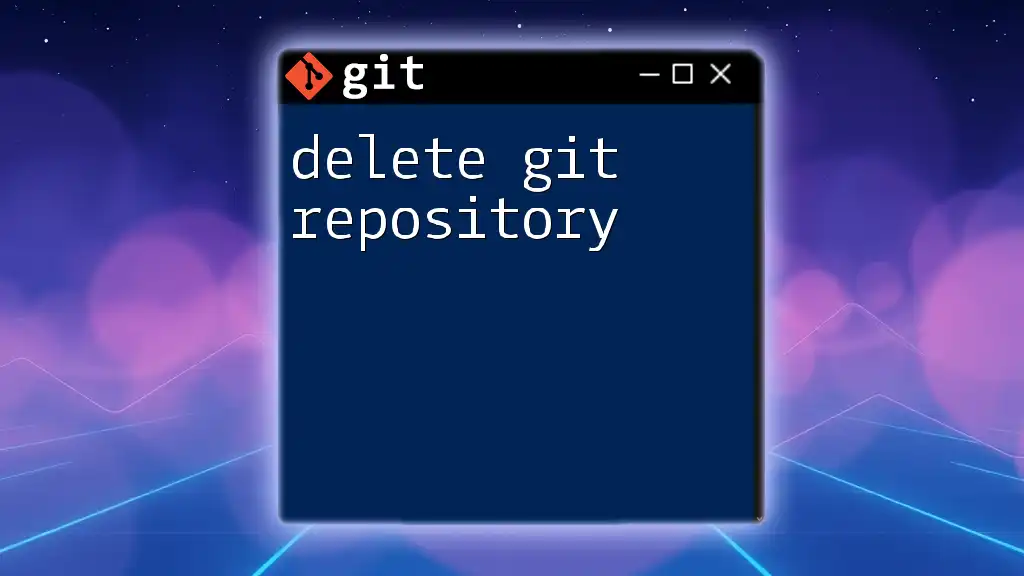
Call to Action
We’d love to hear about your experiences with Git and any questions you may have! Share your thoughts in the comments below, and don't forget to follow us for more concise tips and tricks related to Git and version control.
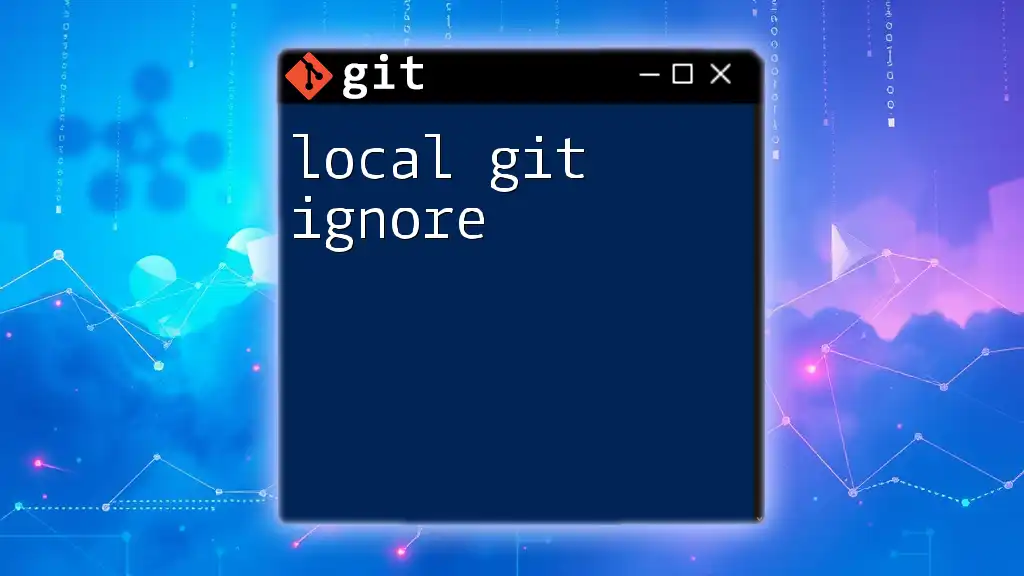
Additional Resources
For further exploration, consider checking out the official Git documentation, online tutorials specific to Git workflows, or Git GUI tools for those who prefer visual interfaces. These resources will help deepen your understanding and broaden your skills as you continue working with Git.