The `cargo add` command allows you to add a dependency from a Git repository, specifying a sub-path within that repository for better organization and quick reference.
Here's how you can do it:
cargo add --git https://github.com/username/repo.git --sub-path path/to/directory
Understanding Cargo and Git Repositories
What is Cargo?
Cargo is the official package manager for the Rust programming language. It streamlines the process of managing Rust projects and their dependencies by handling downloading, compiling, and publishing packages. With Cargo, developers can define the dependencies in a simple configuration file, alleviating the complexity of tracking library versions and updates.
What is Git?
Git is a distributed version control system that enables multiple developers to work on a project simultaneously without stepping on each other's toes. A Git repository is a storage space where your project lives. It maintains the history of all changes made to the project, allowing for easy collaboration and version management.
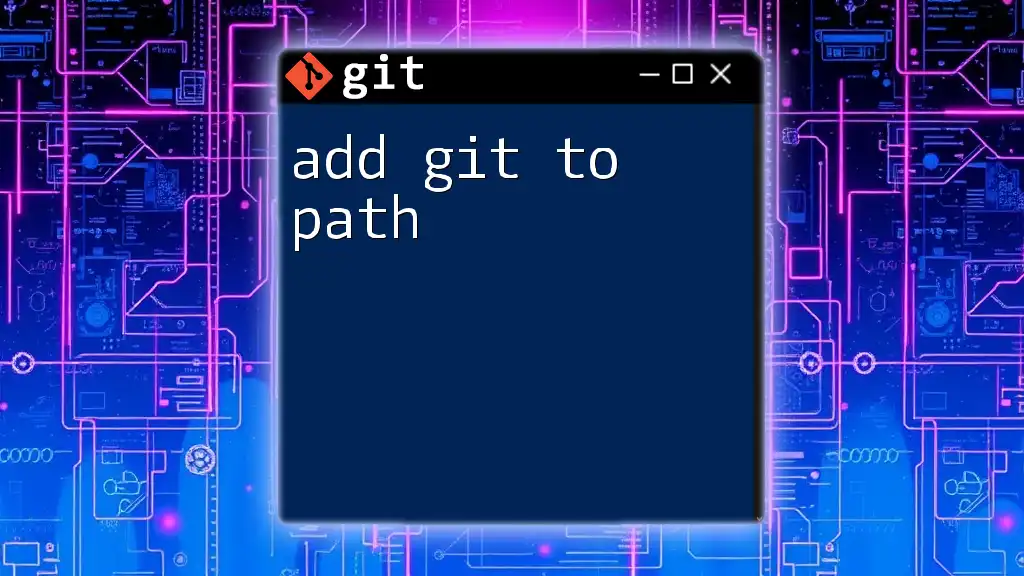
Introducing the Concept of Sub-path
What is a Sub-path in a Git Repository?
A sub-path refers to a specific directory within a Git repository. It allows you to target a particular portion of the repository, especially useful in large projects. Instead of pulling an entire repository, which may contain many unnecessary files, you can focus on the subset of files relevant to your needs.
Importance of Using Sub-paths
Utilizing sub-paths is crucial in several scenarios. For example, when working with large monorepos that might contain numerous packages, selecting a specific sub-path ensures that your project lightweight and specific to your requirements. This modular approach can substantially reduce project complexity and improve build times.
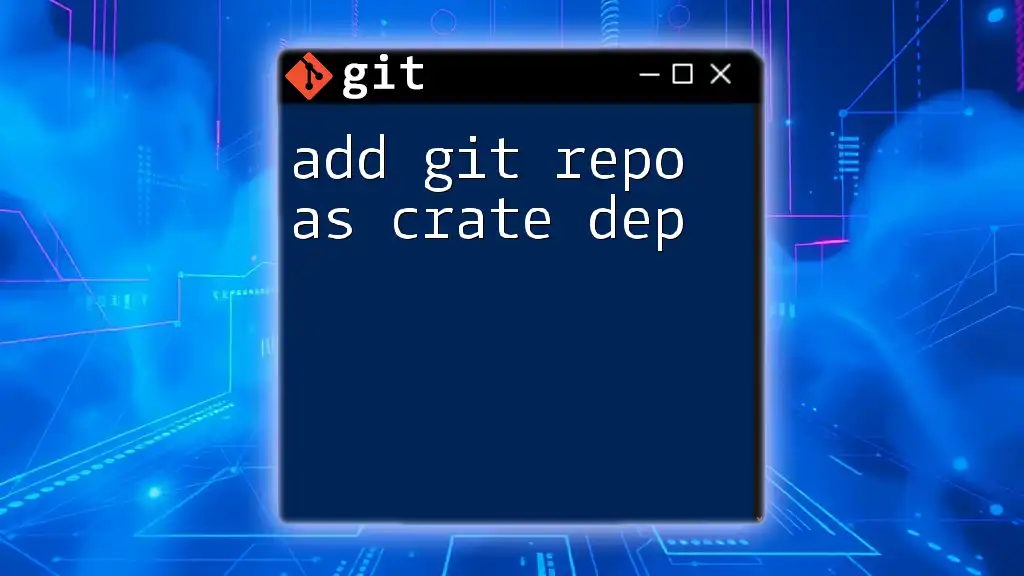
The Command: `cargo add git repo sub-path`
Basic Syntax of the Command
The command to add a dependency from a Git repository located in a sub-path is as follows:
cargo add --git <repository-url> --sub-path <sub-path>
This succinctly describes how to introduce a library into your Cargo project from a specific location within a Git repository.
Breaking Down the Command
- cargo add: This command adds dependencies to your project. It updates the project's `Cargo.toml` file accordingly.
- --git: This parameter specifies the Git repository URL from which you want to pull your dependency.
- --sub-path: This option allows you to target a specific directory within the provided repository rather than fetching everything. It's particularly useful for modular projects.
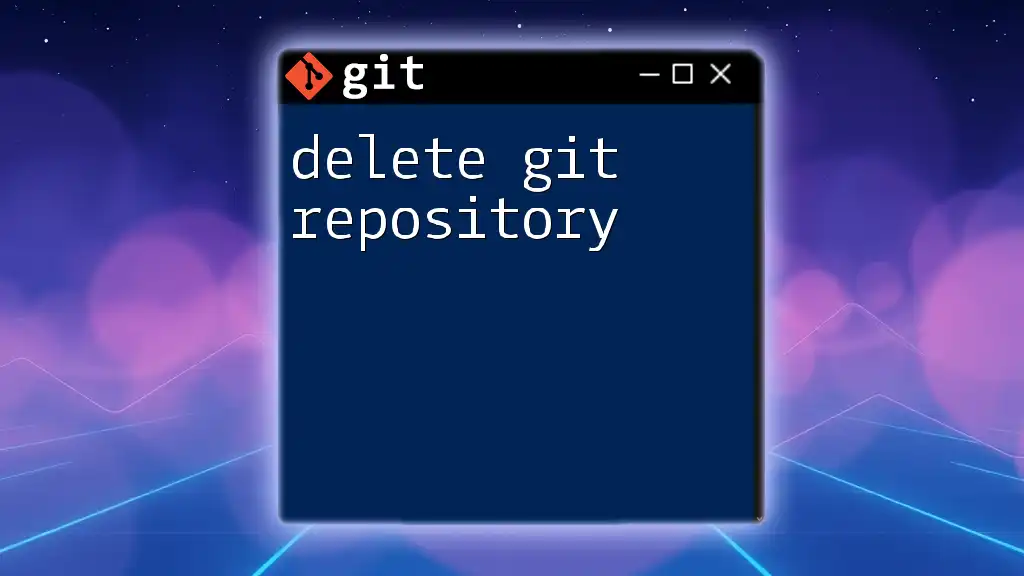
Setting Up Your Environment
Prerequisites
Before you can use Cargo and the `cargo add` command, you need to ensure that both Cargo and Git are installed on your system. You can install Cargo by following the official Rust installation guide, which includes both Rust and Cargo. Git can be downloaded from the official Git website. After installation, verify that they are installed correctly by running the following commands:
cargo --version
git --version
Creating a Sample Git Repository
To fully grasp how to use the `cargo add git repo sub-path` command, it's beneficial to create a simple Git repository. Start by initializing a new repository:
mkdir example_repo
cd example_repo
git init
Next, create a sub-path within your repository:
mkdir -p some_module
echo 'pub fn example() { println!("Hello from example!"); }' > some_module/lib.rs
git add .
git commit -m "Initial commit with some_module"
Now you have a basic repository structure where `some_module` serves as a sub-path that you can target later.
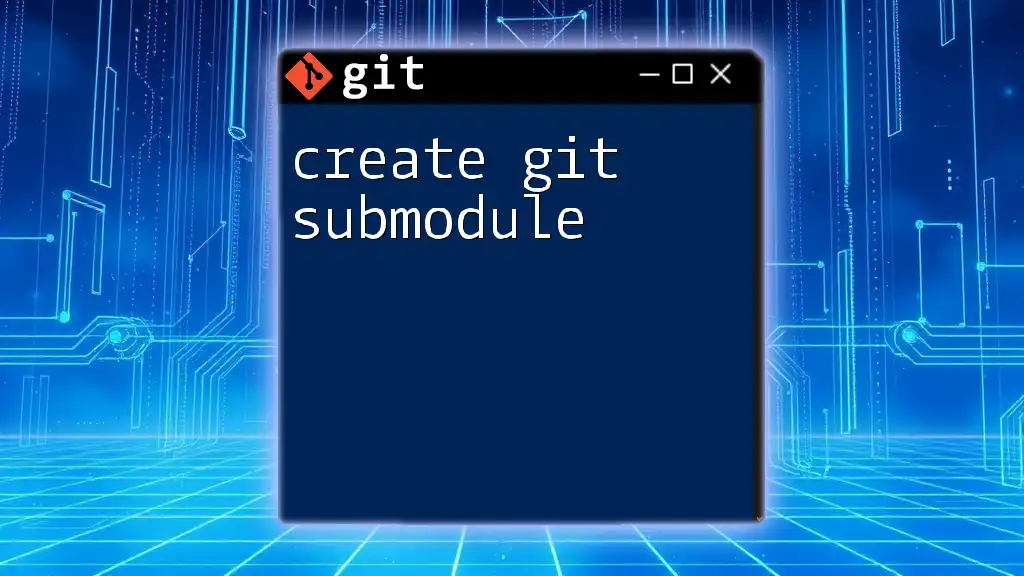
Practical Examples of `cargo add git repo sub-path`
Example 1: Adding a Dependency from a Sub-path
Let’s say you want to include the `some_module` library from your Git repository. You can use:
cargo add --git https://github.com/yourusername/example_repo.git --sub-path some_module
After executing this command, Cargo updates your project's `Cargo.toml` to include `some_module` as a dependency.
Example 2: Using a Private Repository
If your repository is private, you need to set up authentication. For example, you may use an SSH URL:
cargo add --git git@github.com:yourusername/private_repo.git --sub-path some_module
This command assumes that your SSH keys are properly configured for accessing your GitHub account.
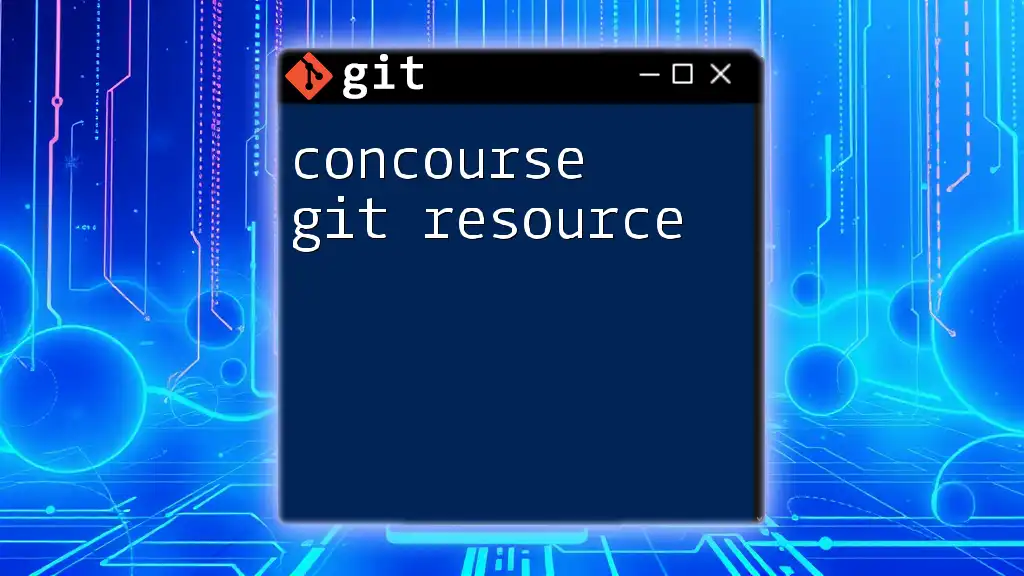
Troubleshooting and Best Practices
Common Issues
While using the `cargo add` command, you might encounter some common errors such as authentication failures or path issues. Ensure that you have the correct URL and the necessary permissions to access the repository. If problems persist, double-check your SSH or HTTPS settings as applicable.
Best Practices
To make the most of `cargo add git repo sub-path`, consider the following best practices:
- Keep your dependencies updated: Regularly check for updates to the libraries you are using from Git repositories to ensure you benefit from bug fixes and improvements.
- Use branches appropriately: If you're targeting a specific version or feature, specify the branch in your command to avoid inconsistencies.
- Monitor for changes in the sub-path: Keep an eye on the upstream repository for significant modifications in the targeted sub-path that could affect your project.
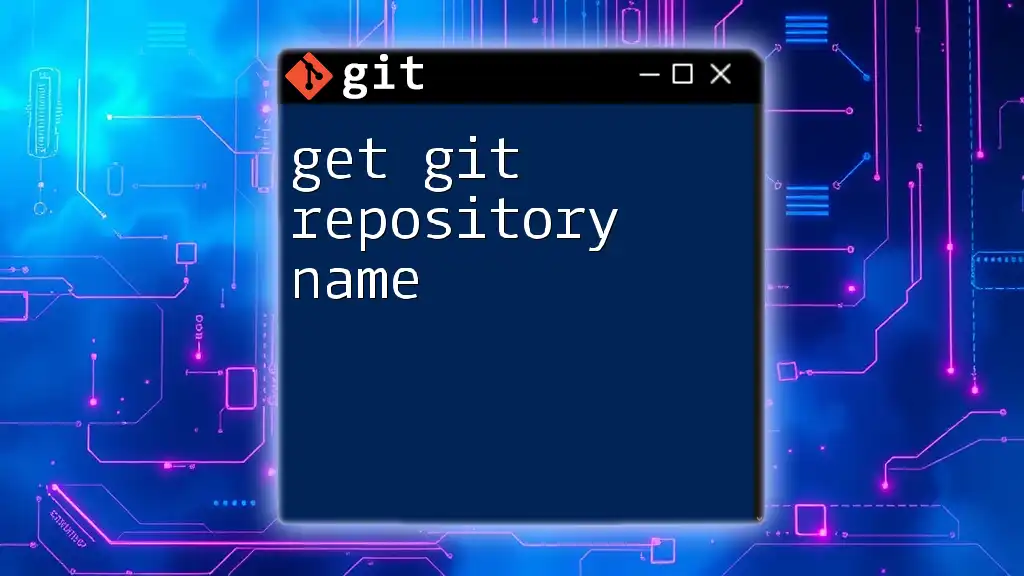
Conclusion
Summary of Key Takeaways
In this guide, we explored how to use the `cargo add git repo sub-path` command to add dependencies from Git repositories efficiently. This method enhances modularization and easy integration into Rust projects.
Further Reading and Resources
To deepen your understanding, refer to the [official Cargo documentation](https://doc.rust-lang.org/cargo/) and explore additional tutorials that outline using Cargo in versatile and innovative ways.
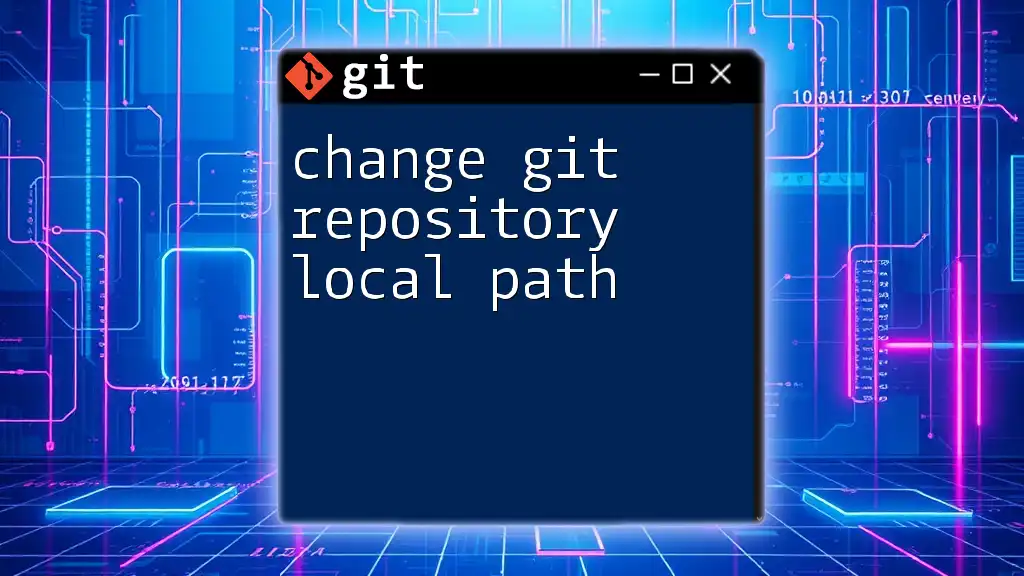
Call to Action
Start experimenting with your own projects by utilizing the `cargo add` command today. Don’t hesitate to reach out, subscribe, or follow for more quick and concise tips on mastering Git and Cargo!