To add a Git repository as a crate dependency in your Rust project's `Cargo.toml`, you can specify the repository URL under the `[dependencies]` section like this:
[dependencies]
your-crate-name = { git = "https://github.com/username/repo.git" }
Understanding Crates and Dependencies
What is a Crate?
A crate in Rust is a package of Rust code. It can be an entire library, an executable, or some combination of both. Crates are fundamental units of Rust’s modularity, allowing developers to share and maintain code effectively. They come in two varieties:
- Library Crates: These provide reusable code that can be included in other projects.
- Binary Crates: These contain the `main` function required to create an executable.
The Role of Dependencies in Rust Projects
Dependencies are pivotal in promoting modular programming, enabling developers to use external libraries without reinventing the wheel. Rust's package manager, Cargo, simplifies the management of these dependencies, ensuring that you can easily add, update, and configure them for your projects.
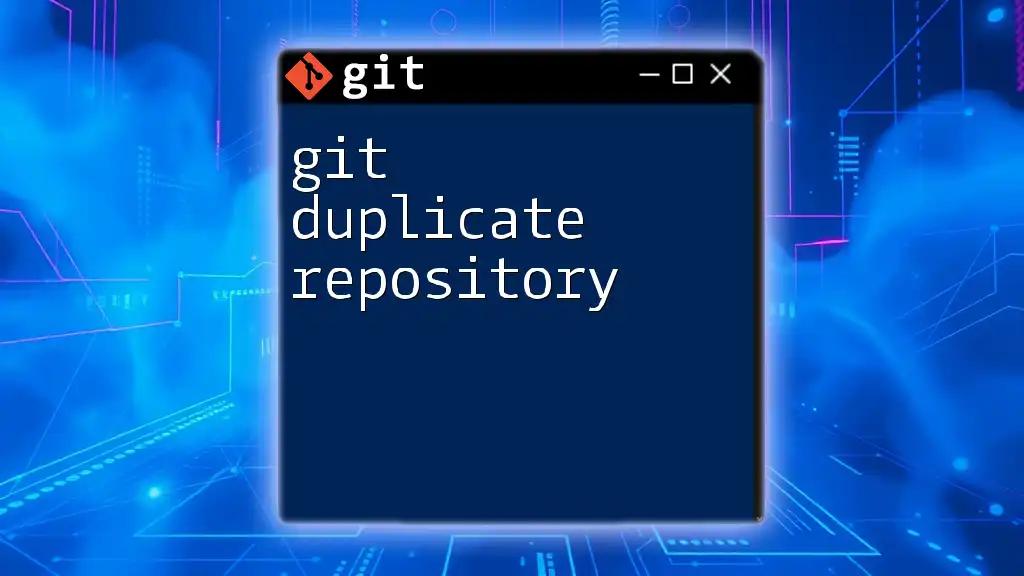
Adding a Git Repository as a Dependency
Why Use Git Repositories for Dependencies?
Using Git repositories as dependencies offers several advantages:
- Version Control: You can access specific versions of a crate that are maintained in the repository.
- Collaboration: Contributing to an open-source project or using code that isn’t published to `crates.io` becomes seamless.
- Latest Features: Utilizing a repository allows you to pull in the latest changes and features developed by the maintainers.
Choosing Git dependencies over traditional crates is prudent when you need more control over the code being used or if you're working on a project that is still in development.
Syntax for Adding a Git Dependency
The general format for specifying a Git dependency in your project’s `Cargo.toml` file is straightforward. Use the following syntax:
[dependencies]
my_crate = { git = "https://github.com/username/my_crate.git" }
This snippet informs Cargo that it should pull `my_crate` directly from the specified Git repository.
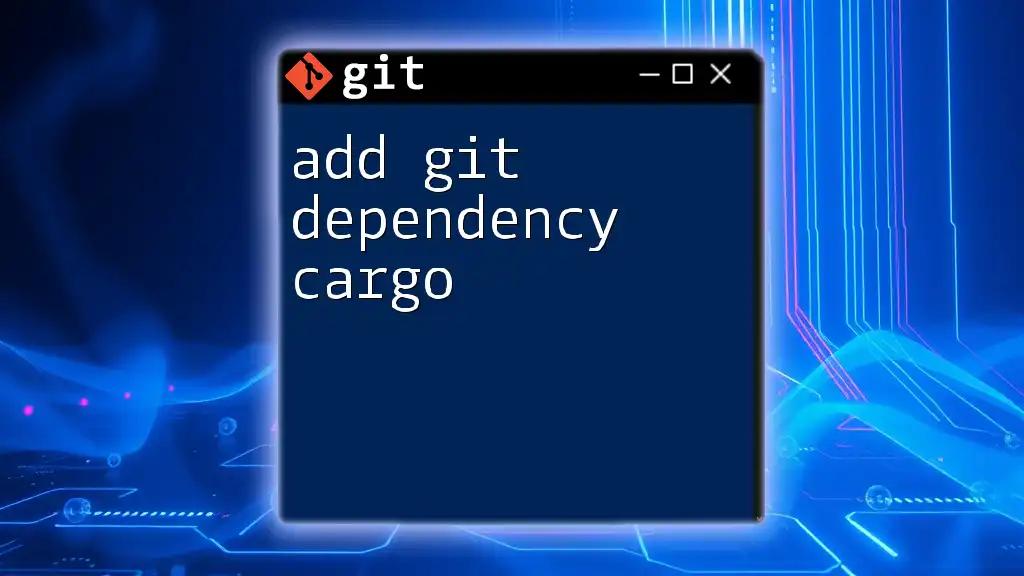
Step-by-Step Guide to Adding a Git Repo as a Dependency
Setting Up Your Rust Project
Before you can add any Git dependencies, you need a Rust project set up. You can create a new project using the following commands:
cargo new my_project
cd my_project
This will create a new Rust project named `my_project` with a basic directory structure.
Modifying the Cargo.toml File
After setting up your project, locate the `Cargo.toml` file in the root of your project directory. This file contains metadata about your project, including its dependencies.
To add a Git dependency, simply open the `Cargo.toml` file and add an entry under the `[dependencies]` section.
Example: Adding a Git Dependency
Let’s consider a real-world example of adding a popular crate from a Git repository, such as `serde`, a widely-used serialization library. You can specify it in your `Cargo.toml` like this:
[dependencies]
serde = { git = "https://github.com/serde-rs/serde.git" }
This line directs Cargo to fetch the latest version of `serde` directly from its Git repository.
Specifying a Branch or Tag
In some cases, you may want to use a specific branch or tag to ensure compatibility or to use stable versions of the code. Here’s how you specify them:
Specifying a Branch: If you want to use a branch that contains new features or fixes, you can include it as follows:
my_crate = { git = "https://github.com/username/my_crate.git", branch = "develop" }
Using a Tag: Alternatively, if you want a stable release, you can reference a tag instead:
my_crate = { git = "https://github.com/username/my_crate.git", tag = "v1.0.0" }
This allows you to pin your dependency to a specific version, providing predictability in your builds.
Cloning a Local Git Repository
If you're developing a crate or using one stored locally during development, you can set it as a dependency too. For example:
my_crate = { path = "../path_to_local_repo" }
This approach is beneficial when you are actively developing the crate and wish to test it simultaneously with your main project.
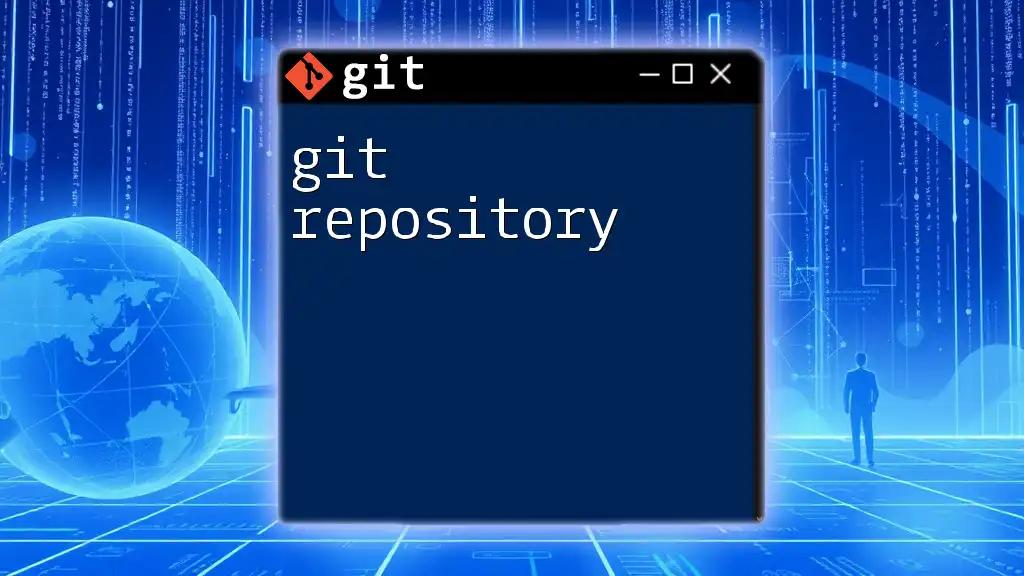
Best Practices for Using Git Dependencies
Keeping Dependencies Up-to-Date
Managing your dependencies effectively means regularly checking for updates. You can do this by utilizing:
-
Cargo’s Update Command: This command attempts to update your dependencies to the latest versions specified in `Cargo.toml`. Regularly running `cargo update` helps keep your application current.
-
Reviewing Upstream Changes: When using Git repositories, you should have a system to check changes in the upstream repositories, ensuring any breaking changes do not disrupt your workflow.
Managing Multiple Dependencies
When a project relies on multiple Git dependencies, you can add them all in the `Cargo.toml` file like so:
[dependencies]
first_crate = { git = "https://github.com/username/first_crate.git" }
second_crate = { git = "https://github.com/username/second_crate.git" }
Keeping your `Cargo.toml` well-organized is essential for maintainability. Use clear naming conventions and consistent formatting to enhance readability.
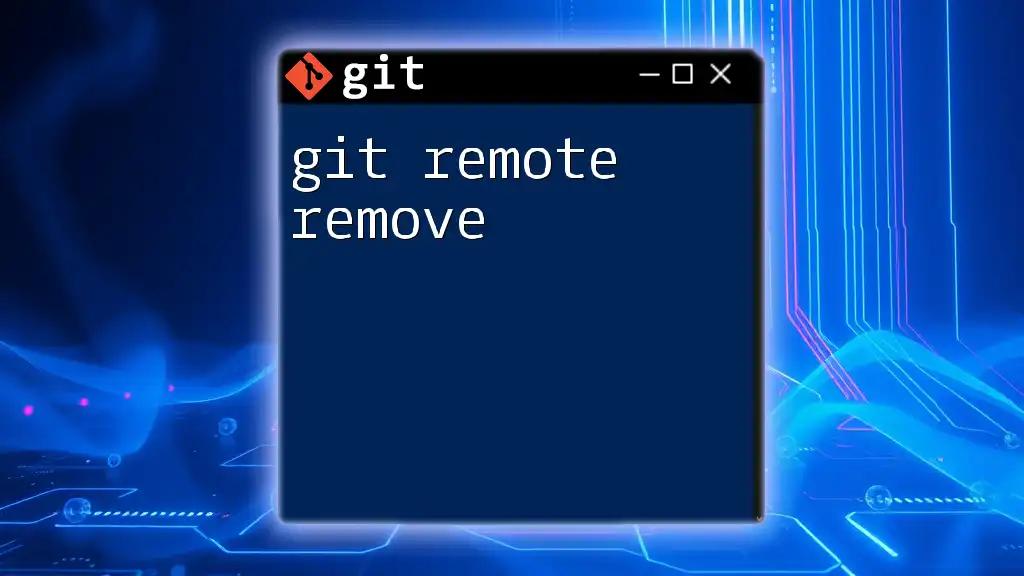
Common Issues and Troubleshooting
Dependency Resolution Problems
Occasionally, you might encounter issues when Cargo cannot resolve the specific Git dependency. Common troubleshooting steps include:
- Ensure the URL is correct and points to a valid Git repository.
- Check that your internet connection is active and stable, as dependency fetching happens over the network.
If you are using submodules, ensure they are updated so that all dependencies are resolved correctly.
Versioning Conflicts
Versioning conflicts may arise when multiple parts of your project or its dependencies require different versions of the same crate. To manage these:
-
Use Cargo’s features: Cargo allows you to specify features for your dependencies to avoid bloat and potential conflicts.
-
Explicit Versions: Be explicit in your `Cargo.toml` regarding which versions of dependencies you are using. Use compatible version syntax when specifying a Git dependency to indicate which versions you expect to work with.
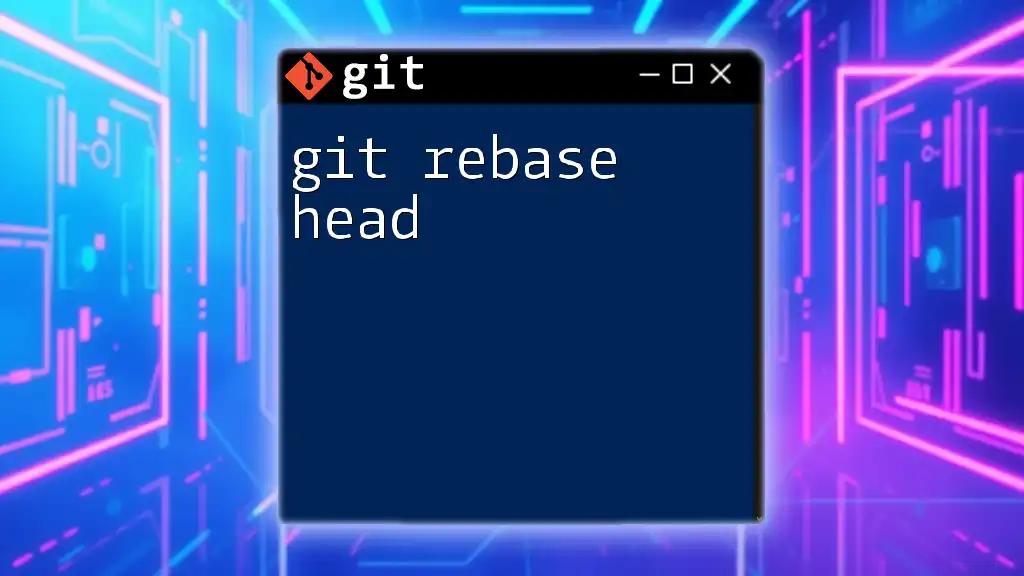
Conclusion
In summary, using Git repositories as dependencies in your Rust projects provides flexibility, access to the latest features, and better collaboration opportunities. By following the steps outlined in this guide, from setting up your project to handling dependency conflicts, you can effectively manage your Rust crates. Experimenting with adding Git dependencies will empower you to utilize and contribute to a vibrant ecosystem of Rust libraries.
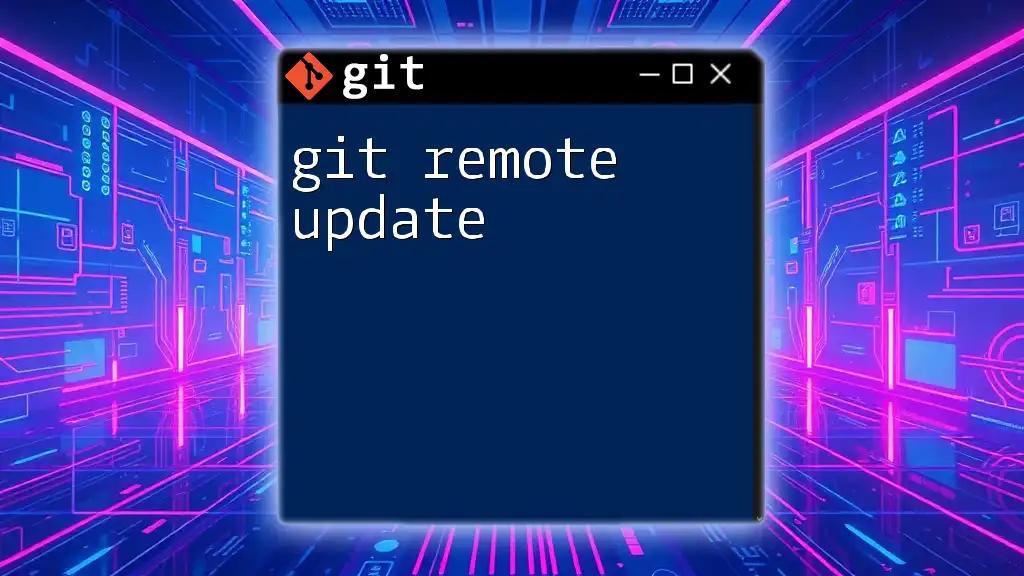
Additional Resources
To enhance your understanding and skills:
- Visit the official [Cargo documentation](https://doc.rust-lang.org/cargo/) for comprehensive guidelines.
- Explore tutorials focused on advanced dependency management in Rust applications.
- Seek avenues for learning more about the integration of Rust and Git, which can bolster your project management capabilities.
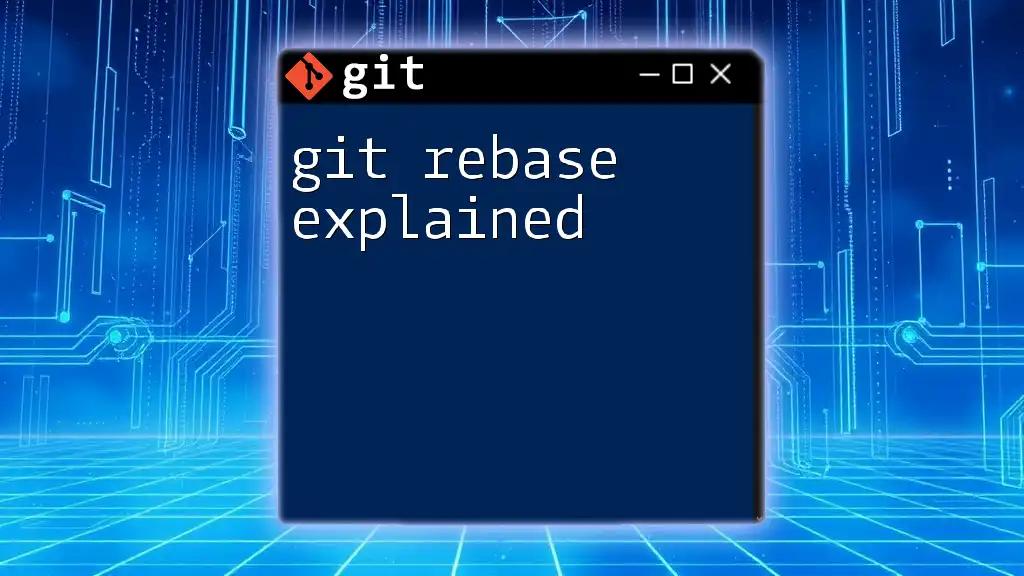
Call to Action
We invite you to begin your own projects and delve into the world of Rust development. Share your experiences and insights in the comments below; your contributions could help others in their journeys!