To add a Git dependency in a Rust project using Cargo, you can specify the desired Git repository in your `Cargo.toml` file under the `[dependencies]` section as follows:
[dependencies]
your_dependency_name = { git = "https://github.com/username/repository.git" }
This enables you to include a library from a Git repository directly into your Rust project.
Understanding Dependencies in Rust
What are Dependencies?
In the world of software development, a dependency is essentially a piece of software that your project requires to function properly. When working on programming projects, especially in Rust, you'll often rely on libraries or packages created by others. These libraries contain pre-written code that helps you perform specific tasks without reinventing the wheel.
Understanding the distinction between different types of dependencies is crucial:
- Dependencies are required for your project to run.
- Dev-dependencies are necessary only during development and testing but not in production.
- Build-dependencies come into play during project compilation.
Why Use Cargo for Managing Dependencies?
Cargo, the Rust package manager, streamlines the process of managing these dependencies. It comes with several features that enhance productivity:
- Version management: Cargo automatically handles updates and compatibility issues.
- Simplicity: Installing and updating dependencies is as straightforward as updating a single file (Cargo.toml).
- Reproducibility: With Cargo.lock, you ensure that your build is consistent across all environments.
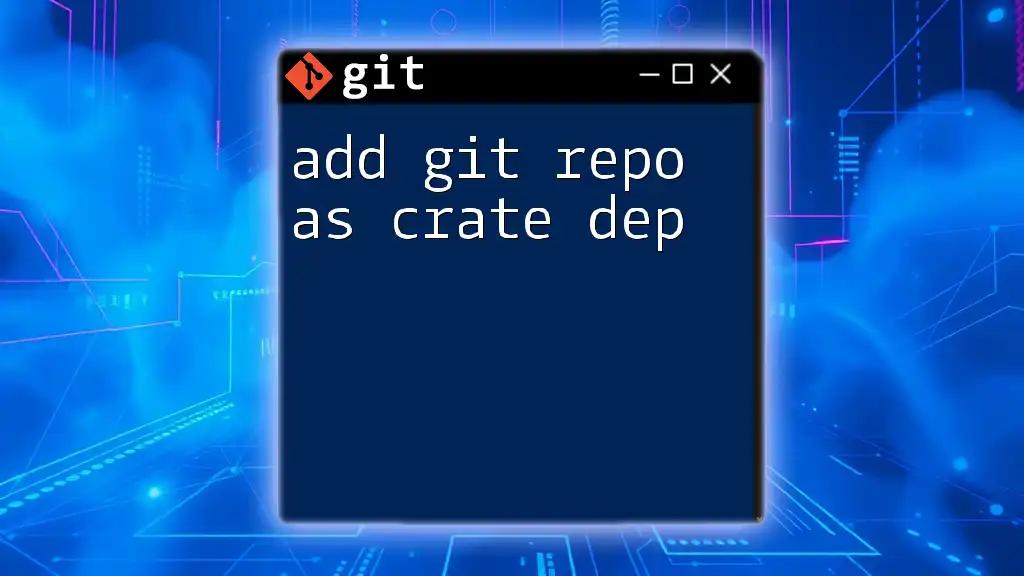
Setting Up Your Rust Environment
Installing Rust and Cargo
Before you can effectively use Cargo, you need to have Rust installed on your machine. The easiest way to do this is by using `rustup`, Rust's official installer. Here’s how:
- Open your terminal.
- Run the command:
curl --proto '=https' --tlsv1.2 -sSf https://sh.rustup.rs | sh
- Follow the on-screen instructions to complete the installation.
To confirm that both Rust and Cargo are successfully installed, you can check their versions:
rustc --version
cargo --version
Creating a New Rust Project
Once Rust and Cargo are installed, you can create a new Rust project with a single command:
cargo new my_project
This command generates a new directory named `my_project` containing a basic structure, including a sample `Cargo.toml` file. This file is where you'll define your dependencies.
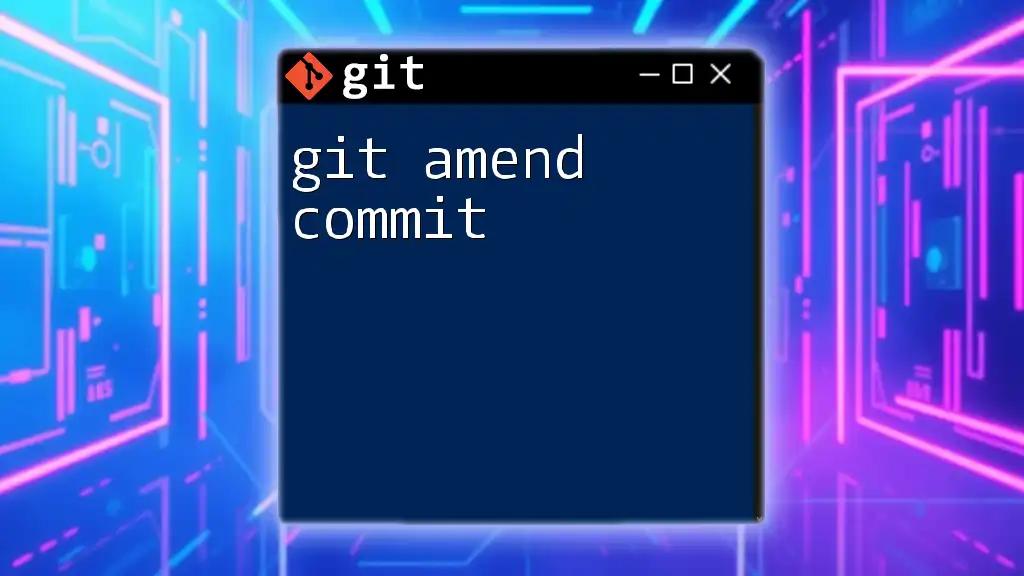
Adding Dependencies in Cargo
Understanding `Cargo.toml`
The `Cargo.toml` file is the cornerstone of dependency management in your Rust project. It includes the metadata for your project and specifies its dependencies. Here’s a brief overview of its major sections:
- [package]: Contains information about your package like name, version, and authors.
- [dependencies]: Where you'll list your project's runtime dependencies.
- [dev-dependencies]: For dependencies needed only in the development and testing phase.
How to Add a Dependency
Adding a dependency to your project is straightforward. In your `Cargo.toml` file, navigate to the `[dependencies]` section and include your desired package. For example, if you wanted to add the `serde` library, your file would look like this:
[dependencies]
serde = "1.0"
This command tells Cargo to fetch version 1.0 of the `serde` package.
Specifying Dependency Versions
Semantic Versioning Explained
Most libraries follow Semantic Versioning, which breaks down versions into three components: major, minor, and patch. Understanding this system is crucial for effectively managing your dependencies.
- Major version denotes incompatible changes.
- Minor version indicates backward-compatible additions.
- Patch version refers to backward-compatible bug fixes.
Examples of Version Specifiers
When adding dependencies, you can specify versions in different ways to accommodate your needs:
-
To support a range of versions, you could write:
[dependencies] serde = ">=1.0, <2.0"
-
Alternatively, using version ranges allows for flexibility:
[dependencies] serde = "^1.0"
The caret (`^`) symbol implies you want to allow updates that do not change the left-most non-zero digit.
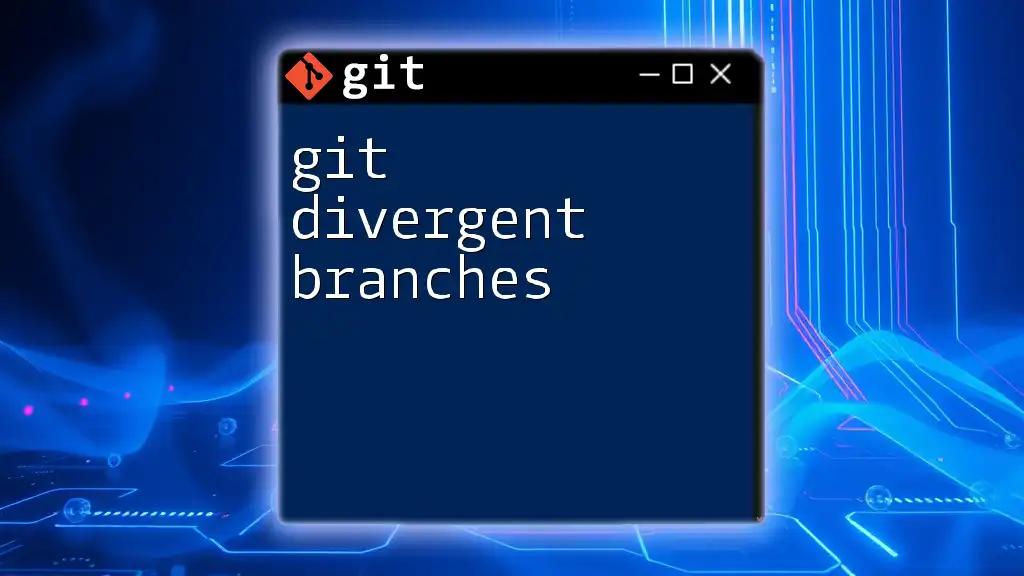
Managing Dependency Updates
Checking for Updates
To ensure you're using the latest compatible versions of your dependencies, you can run the `cargo update` command in your terminal. This command checks your `Cargo.toml` against the versions available in the registry and updates your `Cargo.lock` file accordingly:
cargo update
Locking Dependencies
The `Cargo.lock` file plays a crucial role in dependency management by locking the packages to specific versions that were used at the time the project was created or last updated. This ensures that anyone else who clones your project will install the exact same versions of the dependencies, leading to reproducible builds.
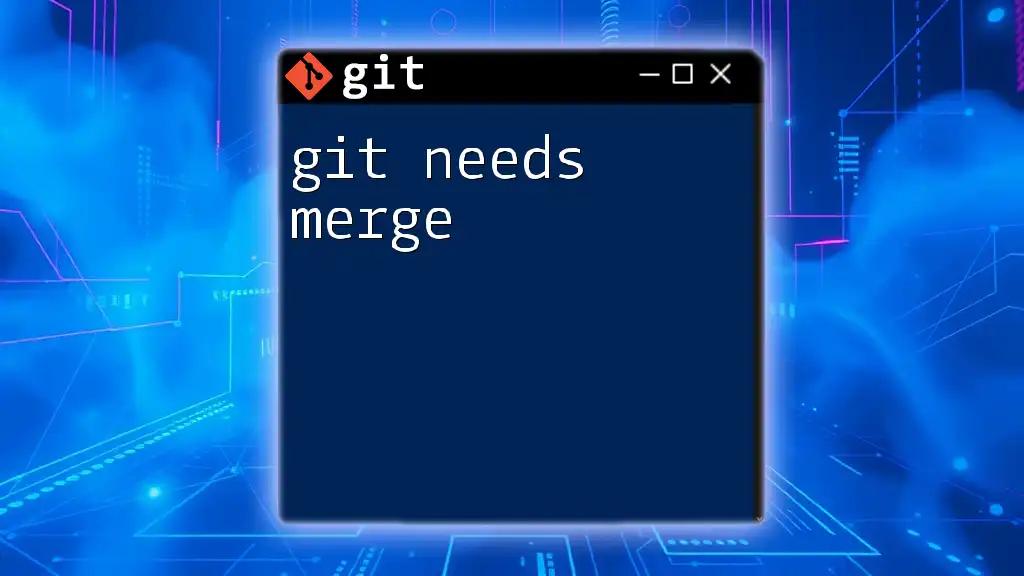
Best Practices for Managing Dependencies
Avoiding Bloat
A common pitfall in software projects is dependency bloat. It's essential to keep the number of dependencies to a minimum, as each one adds additional complexity and potential for conflicts. Regularly assess your dependencies and remove any that are unnecessary.
Regularly Updating Dependencies
Keeping your dependencies up to date is vital for security and performance. Regular updates also prevent you from falling too far behind, which can lead to a more significant effort to catch up later on. As a rule of thumb, update your dependencies every few weeks or at least before a major release of your project.
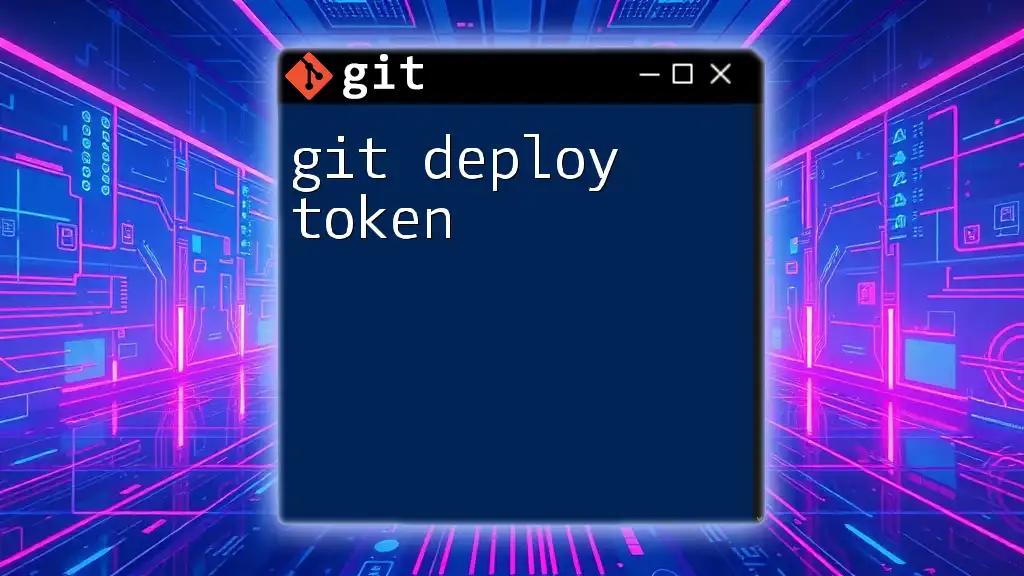
Troubleshooting Common Issues
Failed Dependency Resolution
During development, you may encounter errors indicating that Cargo is unable to resolve certain dependencies. These errors can stem from conflicts between specified versions or unavailability of the requested package. Pay close attention to the error messages, as they often provide guidance on which versions are incompatible.
Handling Version Conflicts
If multiple dependencies require differing versions of the same library, you'll need to resolve these conflicts manually. This can occur by inspecting your `Cargo.toml` file and adjusting the requested versions, or by modifying your dependency's requirements directly, if possible.
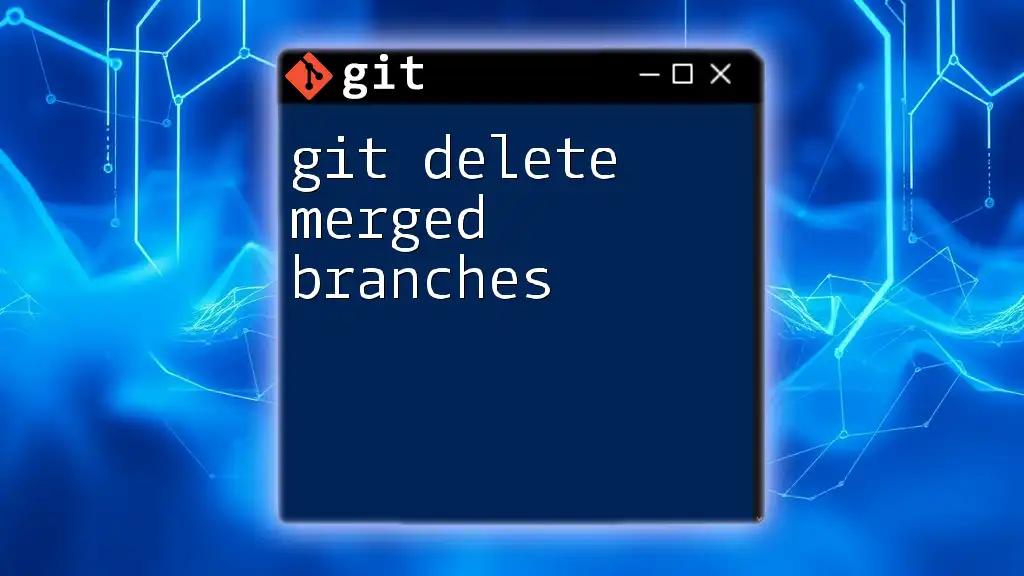
Conclusion
In summary, adding a Git dependency in Cargo involves understanding how to effectively manage your projects with the `Cargo.toml` file and the tools Cargo provides. By following best practices and staying vigilant about updates, you ensure that your Rust projects run smoothly and are well-maintained. Start applying the lessons from this guide, and you'll become proficient in using Git with Cargo in no time.
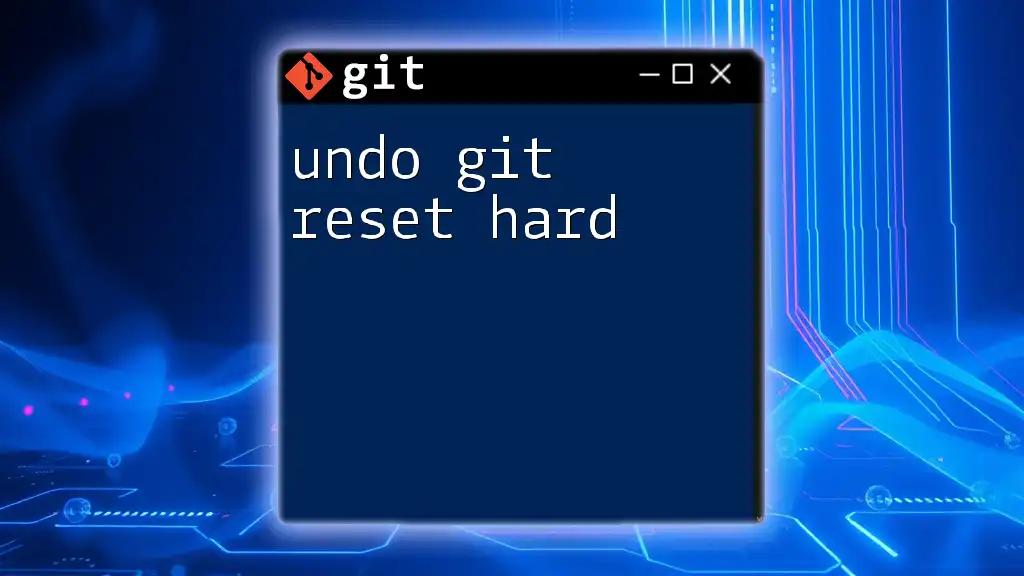
Additional Resources
For a deeper dive into Cargo and Rust, consult the official Rust documentation and the Cargo user guide. If you're looking for comprehensive learning materials, several books and tutorials that focus on Rust programming can provide more insights and advanced concepts.
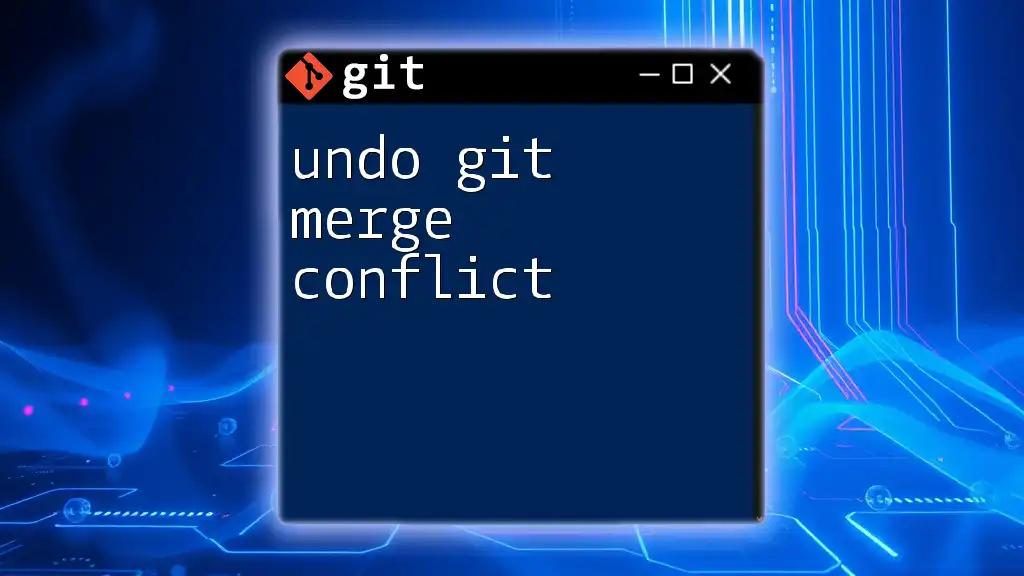
Call to Action
What are your experiences with adding dependencies in Rust projects? Share your thoughts or ask questions in the comments section! Stay tuned for more expert tutorials on Git and Cargo to help you further your programming journey.