To delete the most recent commit from your local Git repository, you can use the following command, which preserves changes in your working directory:
git reset HEAD~1
Understanding Git Commits
What is a Git Commit?
A Git commit serves as a snapshot of your project's files at a specific moment. It captures changes made to your codebase, allowing you to track modifications, revert to previous stages, and understand the evolution of your project. Each commit is accompanied by a unique identifier (hash) and metadata, which includes the author's information and a commit message that describes the changes made.
Why Delete a Commit?
While commits are essential for tracking your project's history, there are certain circumstances where you may need to delete a commit. Common scenarios include:
- Mistaken Commits: You accidentally committed unwanted changes.
- Refactoring or Clean-Up: You realize that some commits are redundant or irrelevant to the final product.
- Sensitive Information: You inadvertently included sensitive data in a commit message or file.
It’s crucial to understand that deleting a commit alters your project history. Depending on your collaborative workflow, this can significantly affect others' work, particularly when the commits are already pushed to a shared repository.
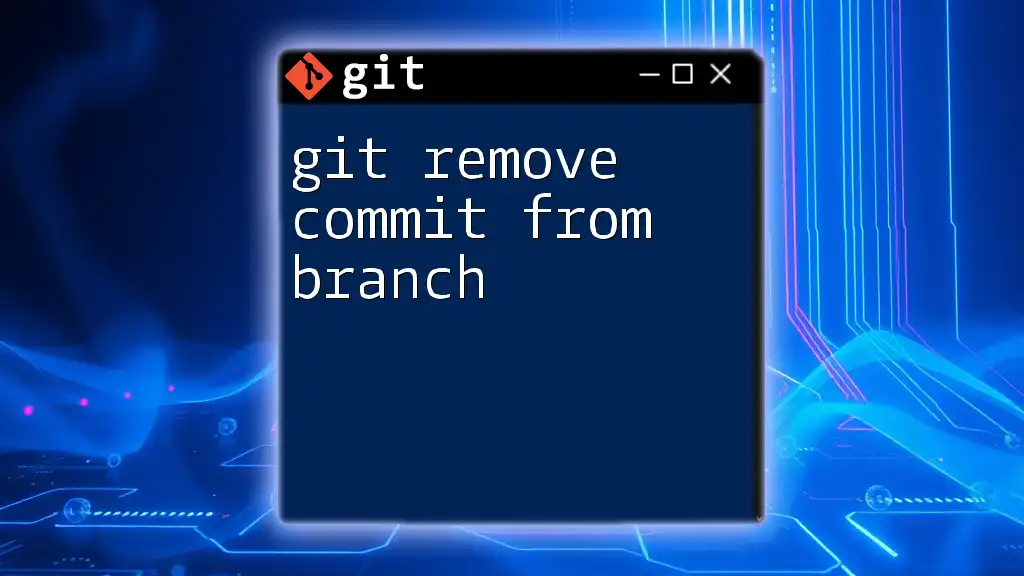
Methods to Delete a Commit From Local Repository
Deleting the Most Recent Commit
If you need to delete the most recent commit from your local repository, you can do this using the `git reset` command. This allows you to remove the latest commit while deciding what to do with the changes—keep them in your working directory or discard them entirely.
To delete the last commit, use the following command:
git reset --soft HEAD~1
Breaking Down the Command
- `git reset`: This command alters the current branch's commit history.
- `--soft`: This option keeps your changes in the working directory, allowing you to modify or recommit them as necessary.
- `HEAD~1`: This refers to the commit just before the most recent one. You can adjust this number to specify how many commits you want to remove.
Deleting Specific Commits
In some cases, you may want to delete commits that are not the most recent. This is often done using git rebase or git reset.
Using Git Rebase
Git rebase allows you to revise existing commit history. By using the interactive mode, you can choose which commits to keep, modify, or delete.
To start an interactive rebase, run:
git rebase -i HEAD~n
Replace `n` with the number of commits you want to review. This will open your default text editor with a list of commits.
In the editor, you will see something like this:
pick 1234567 Commit message 1
pick 89abcde Commit message 2
pick fedcba9 Commit message 3
To delete a commit, simply change `pick` to `drop` or delete the line corresponding to that commit. After saving and closing the editor, the specified commit(s) will be removed.
Using Git Reset
You can also use the `git reset` command in different modes to delete commits. The options include:
-
Soft Reset: Retain changes in the working directory for future commits.
git reset --soft <commit-hash>
-
Mixed Reset: Retain changes but reset staging area (default option).
git reset <commit-hash>
-
Hard Reset: Discard all changes, irreversibly deleting the commit and any associated modifications.
git reset --hard <commit-hash>
Each method has its use cases, so choose carefully based on your intentions with the changes.
Important Considerations Before Deleting Commits
Before proceeding with deleting any commit:
- Impact on Others: If you are collaborating with others, be aware that deleting commits that have been pushed to a remote repository can disrupt their workflow.
- Backups: Always consider creating a backup branch before deleting commits, ensuring you can restore any necessary work.
- Branch Merges: If you have already merged branches that contain the commits you are deleting, understand that this will create discrepancies that you may need to address in the future.
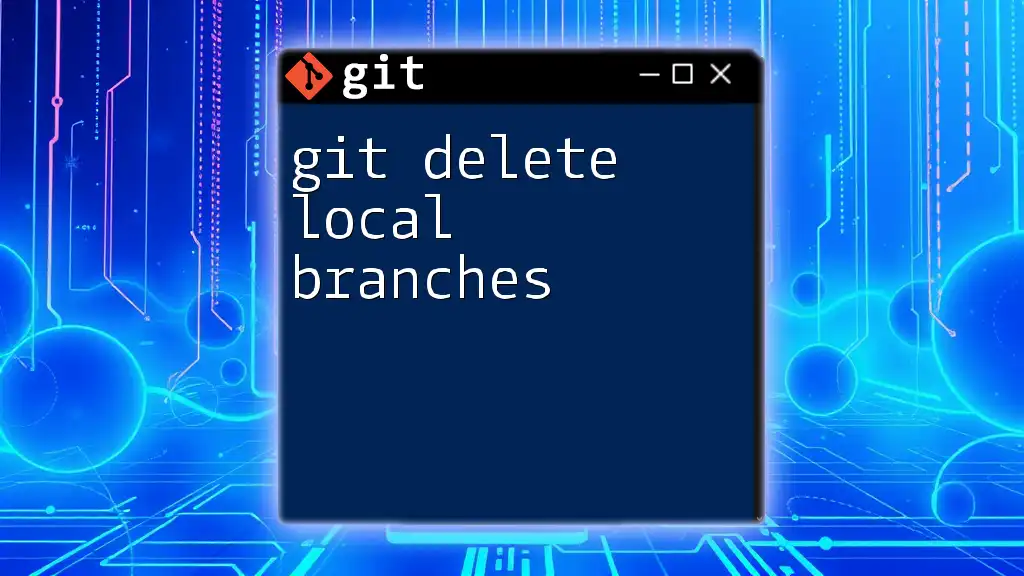
Practical Examples
Deleting a Commit from a Branch
Imagine you have a feature branch containing several commits that are in need of revision. Suppose you want to delete a couple of commits from your `feature-branch`. Here's how you can do it:
git checkout feature-branch
git rebase -i HEAD~3
This command will allow you to review the last three commits. In the interactive rebase interface that opens, you can choose to drop or modify specific commits.
Recovering a Deleted Commit
Mistakes can happen, and you may find that you want to restore a commit you previously deleted. Fortunately, Git has a built-in recovery tool called reflog. This enables you to find reference logs of all the changes made in your repository.
To view your reflog, run:
git reflog
You’ll see a list of recent actions, each associated with a unique identifier. Once you find the commit you want to restore, you can check it out by using:
git checkout HEAD@{n}
Replace `n` with the index of your desired commit in the reflog list.
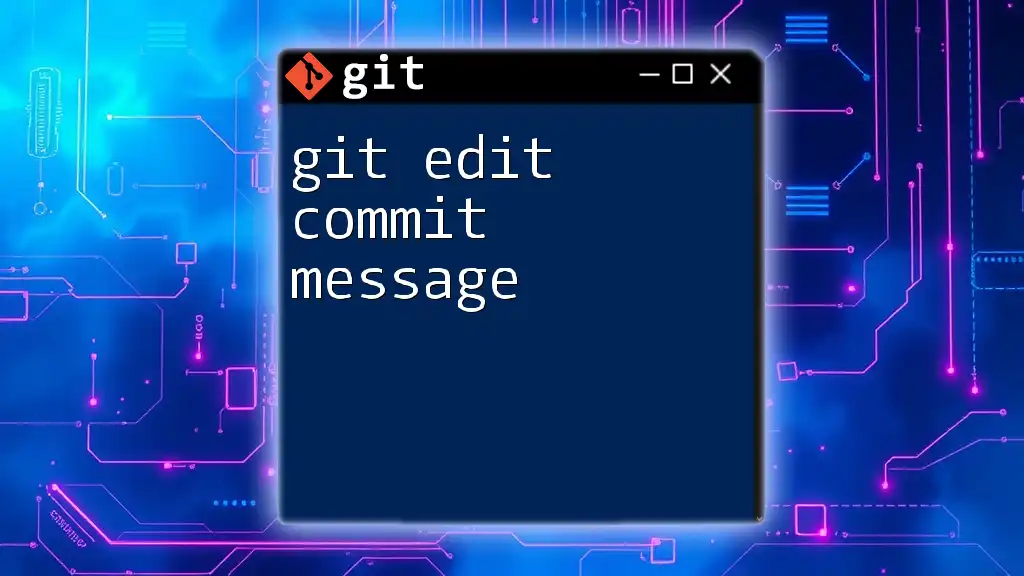
Conclusion
Best Practices for Managing Commits
Managing your commit history effectively can help prevent issues in the long run. Some best practices to consider include:
- Regularly Review Commit History: This allows you to catch unwanted commits before they become a problem.
- Use Descriptive Commit Messages: Clear messages help you and others understand a commit's purpose and facilitate easier navigation.
- Leverage Branches: By using branches for features or fixes, you can isolate changes and minimize complications in your main project history.
Resources for Further Learning
For those looking to deepen their understanding of Git, consider exploring the following resources:
- Git's official documentation is an excellent starting point for command syntax and advanced topics.
- Online platforms, such as Codecademy and freeCodeCamp, offer interactive Git tutorials.
- Books such as "Pro Git" provide in-depth knowledge about version control and best practices.
By mastering how to git delete commit from local, you're not only enhancing your personal repository management skills but also ensuring your team has a cleaner, more understandable version history.