To delete all local branches in a Git repository except for the currently checked-out branch, you can use the following command:
git branch | grep -v "master" | xargs git branch -d
This command lists all branches, filters out the "master" branch (or any other branch you want to keep), and deletes the remaining ones.
Understanding Git Branches
What Are Git Branches?
In Git, branches are fundamental components used to isolate development work. They allow you to build features, fix bugs, or experiment without affecting the main codebase, typically referred to as the `main` or `master` branch. When you create a branch, you are essentially creating a snapshot of your project’s current state, providing you with a separate workspace to make changes.
Why Delete Local Branches?
Over time, as you work on multiple features or fixes, your local repository can become cluttered with branches that are no longer needed. Here are some reasons why you might consider deleting local branches:
- Finished Features or Merged Branches: Once a feature branch has been successfully merged into the main branch, it becomes obsolete and consuming space in your local environment.
- Reducing Clutter for Better Focus: A clean, organized branch list can significantly improve focus, making it easier to find the branches you actively work on.
- Avoiding Confusion with Obsolete Branches: Keeping unnecessary branches can lead to confusion, especially in collaborative environments where multiple team members work on various features.
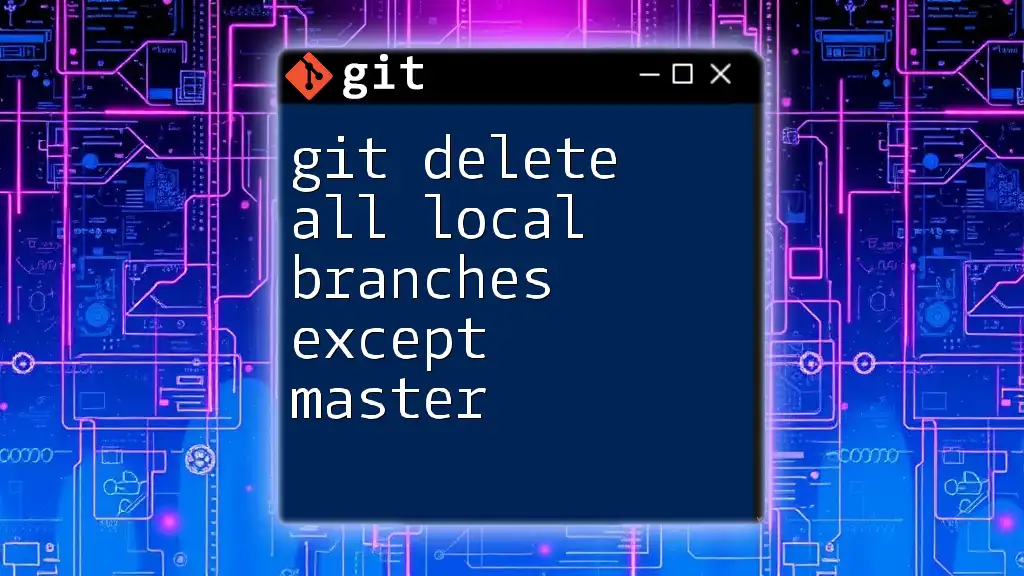
Precautions Before Deleting Local Branches
Ensure No Uncommitted Changes
Before proceeding with any deletions, it’s critical to check for uncommitted changes in your working directory. Uncommitted changes can lead to unintended loss of work. To check the status of your branch, use the command:
git status
Check Which Branch You Are On
It's also important to confirm the current branch you are working on, as you cannot delete the branch you’re currently in. You can easily check this by running:
git branch
The currently active branch will be highlighted with an asterisk (*).
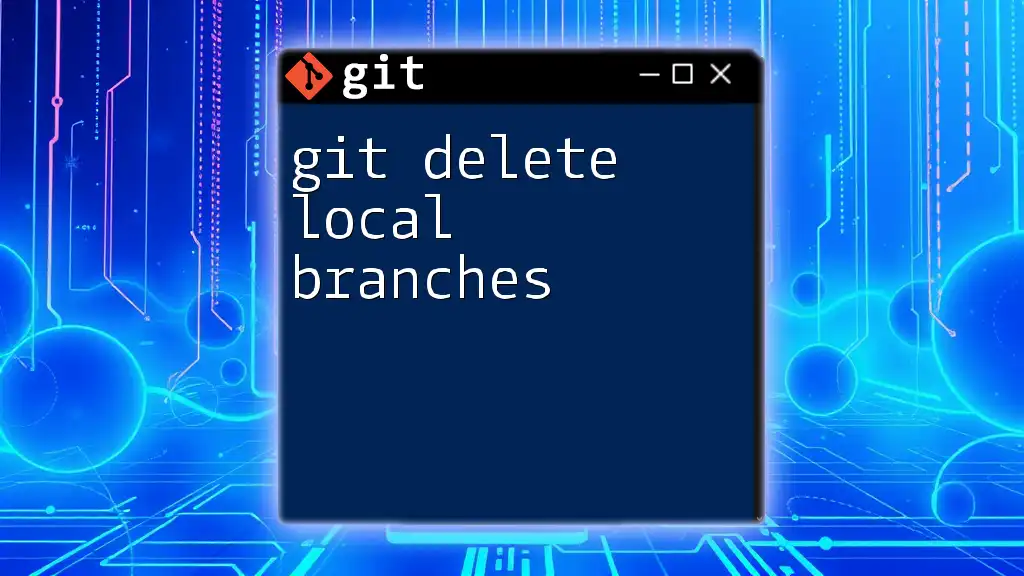
Basic Commands for Branch Management
Listing Local Branches
To display all local branches in your repository, you can use the following command:
git branch
This command provides a list of branches, allowing you to identify which ones you want to delete. It’s a good practice to formulate a plan for which branches you will remove, especially in team settings.
Merging or Deleting
Before deleting a branch, consider merging changes, if necessary. This ensures that any valuable code is retained. To merge branches, utilize the following command:
git merge <branch-name>
Be sure to replace `<branch-name>` with the name of the branch you wish to merge.
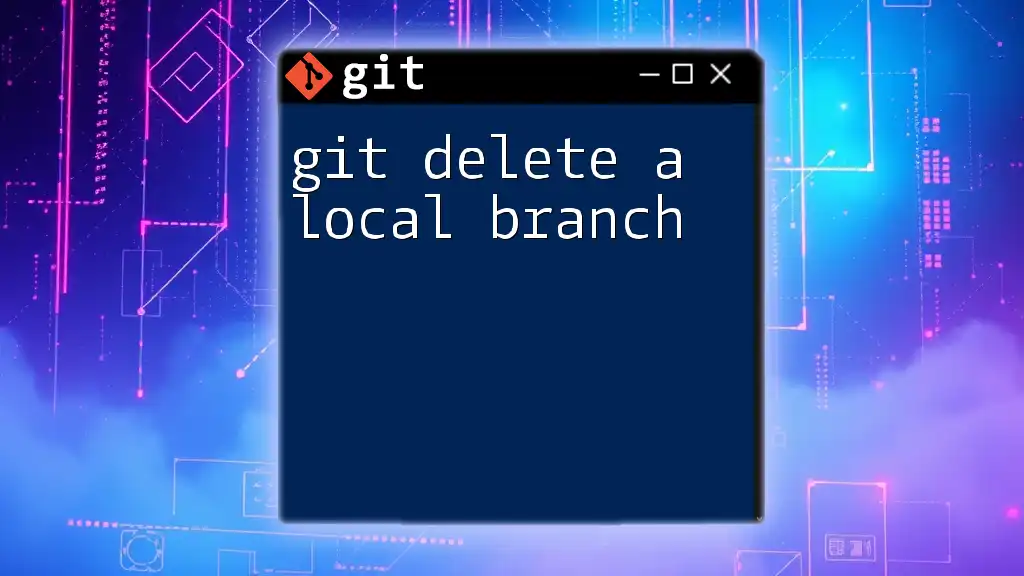
Deleting a Single Local Branch
How to Delete a Specific Branch
If you want to delete a specific local branch that you know is no longer needed, you can execute the delete command:
git branch -d <branch-name>
This command uses the `-d` flag, which safely deletes the branch, preventing you from deleting branches that have unmerged changes.
Force Deleting a Branch
In cases where you are certain you want to delete a branch regardless of its state, you can use the force delete command:
git branch -D <branch-name>
The `-D` flag forces deletion, even if the branch contains changes that haven't been merged.
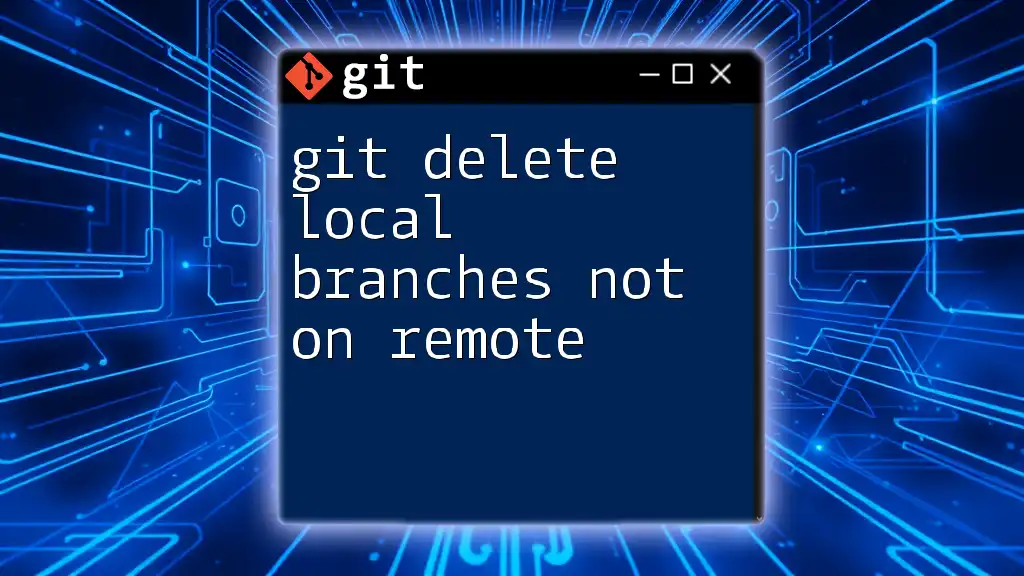
Deleting All Local Branches
Using Git Commands to Delete All Local Branches
When you're ready to declutter by deleting all local branches except for the one you are currently working on, you can use the following command:
git branch | grep -v "main" | xargs git branch -d
Here's a breakdown of how this command works:
- `git branch` lists all local branches.
- `grep -v "main"` filters out the main branch (assuming it’s called “main”); you can change this to whatever your main branch is named.
- `xargs git branch -d` takes the filtered list of branches and deletes them one by one.
Deleting All Local Branches Including the Current One
If you want to delete all local branches, including the current one, you can run:
git branch | xargs git branch -D
That said, approach this command with caution, as it will permanently delete all local branches.
Automating the Process with a Script
To streamline the process of deleting branches, particularly if you frequently clean up your workspace, consider creating a simple shell script. Here’s a sample script:
#!/bin/bash
git branch | grep -v "main" | xargs git branch -d
To use this script, simply save it in a `.sh` file, give it executable permissions, and run it whenever you need to perform a cleanup.
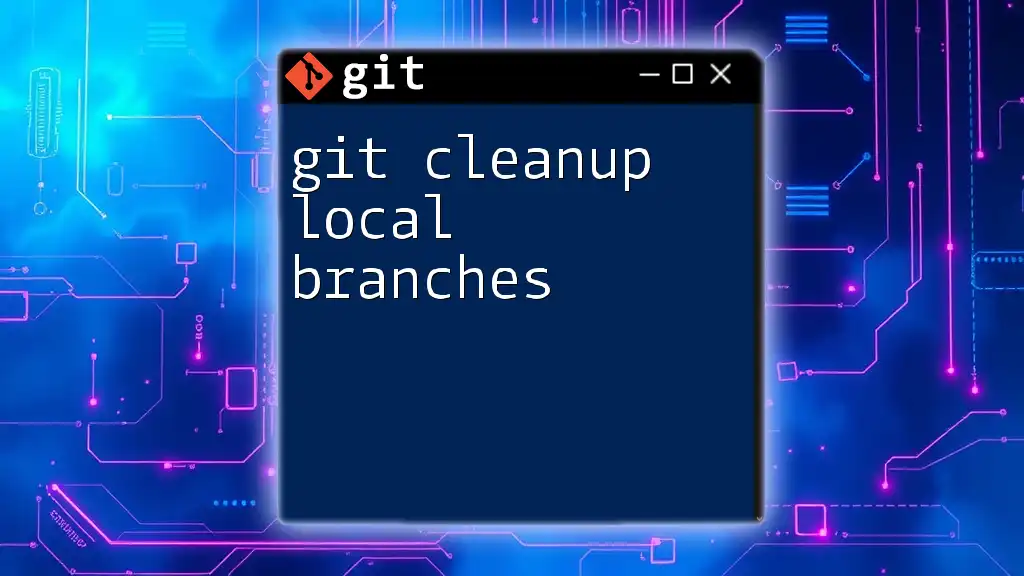
Best Practices for Managing Local Branches
Regular Maintenance
Implementing a routine for cleaning up unused branches can greatly improve your workflow. Schedule regular sessions to review and delete branches that are no longer relevant.
Naming Conventions
Establishing a consistent naming convention for your branches can simplify management. Opt for names that clearly convey the purpose of the branch, such as `feature/login`, `bugfix/ui-issue`, or `test/new-feature`.
Using Git Hooks
Additionally, you may consider utilizing Git hooks to automate cleanup processes. Hooks can execute scripts at key points in Git’s workflow, allowing for automatic branch deletions upon certain triggers, such as merging or pushing.
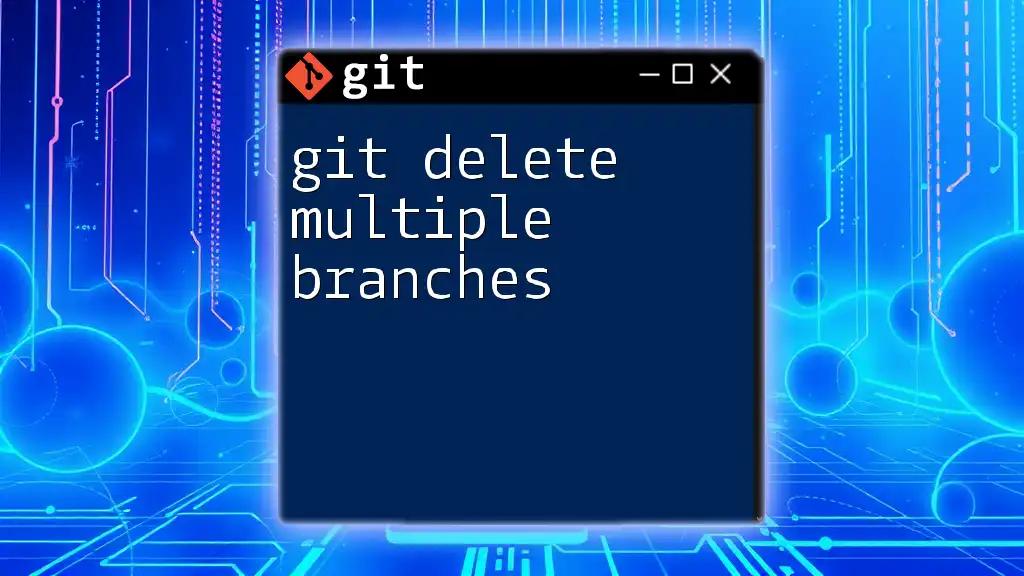
Conclusion
Managing local branches effectively is a crucial aspect of using Git. By regularly performing cleanups and deleting unnecessary branches, you can maintain a focused and organized workspace. By embracing best practices, you can ensure your Git environment supports your development goals efficiently. Explore further tutorials and resources to sharpen your Git skills and streamline your version control processes.