To delete local branches that no longer exist on the remote repository, you can use the following command:
git fetch -p && git branch --merged | grep -v '\*' | xargs -n 1 git branch -d
Understanding Local and Remote Branches
What are Local Branches?
Local branches in Git are essentially pointers to commits that exist in your local repository. They allow you to work on different features, fixes, or experiments without affecting the main codebase. Each local branch diverges from your main branch, which is often called `main` or `master`. This setup enables developers to manage various lines of development easily.
What are Remote Branches?
Remote branches are references to the state of your branches in a remote repository, like GitHub or GitLab. They help you track changes made by others and keep your repository synchronized when collaborating with a team. When you clone a repository, you create local copies of these remote branches, but your local copies are not directly linked—they must be pulled or fetched regularly to keep them updated.
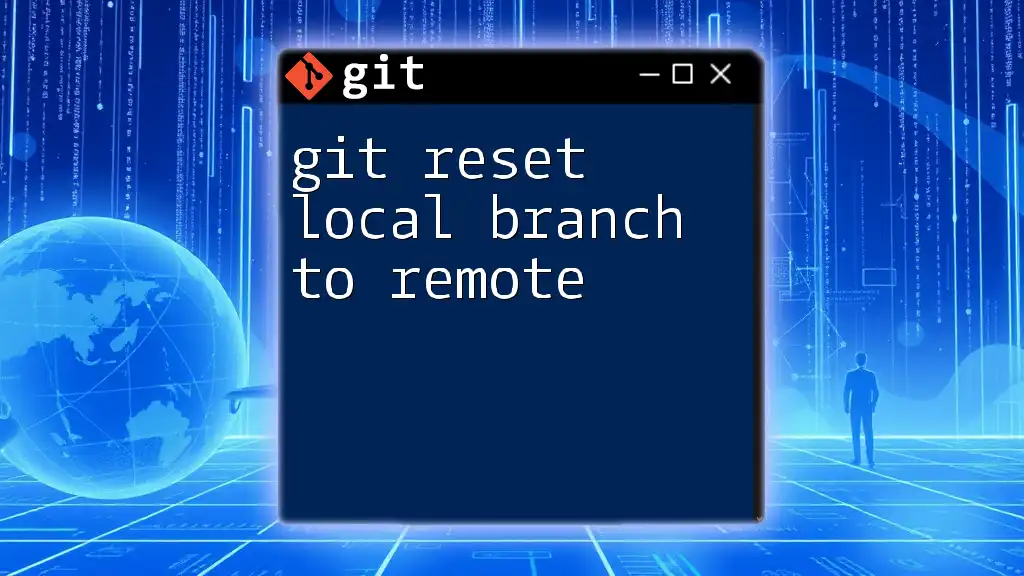
Why Delete Local Branches Not on Remote?
Cleaning up stale local branches is a crucial practice for several reasons.
First, it reduces clutter in your local repository, making it easier to navigate and work on branches. A cleaner workspace allows you and your team to focus on active developments, rather than being distracted by many irrelevant branches.
Second, having too many untracked local branches can lead to confusion about which branches are significant or currently in action. A tidy repository reflects an organized workflow and promotes better collaboration.
However, it’s essential to remember that deleting local branches should be performed with caution. Untracked branches might contain unpushed commits that you need, so always ensure you don’t lose any vital work before deleting.
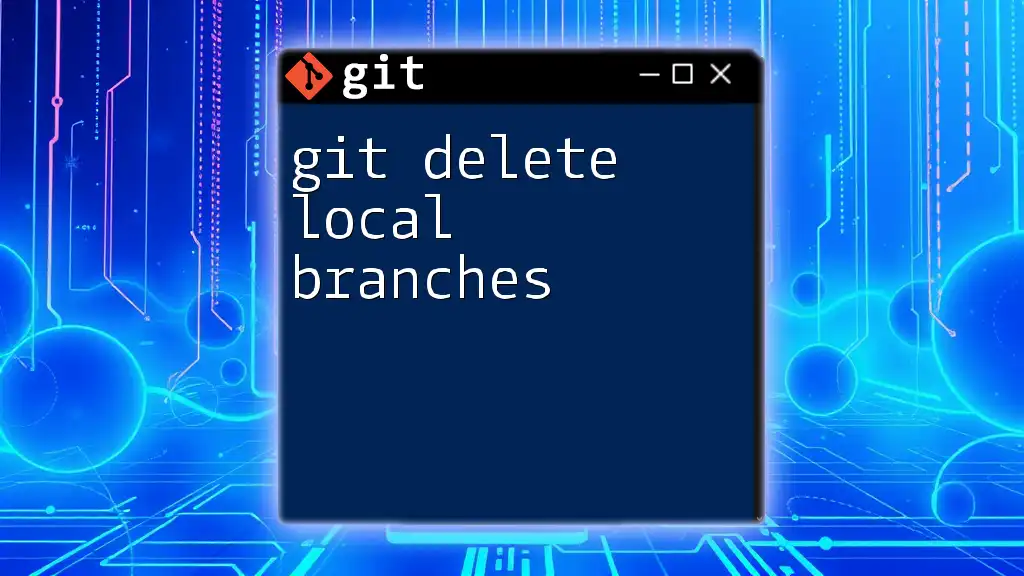
Checking Your Local Branches
Listing All Local Branches
To list all the local branches in your Git repository, you can use the following command:
git branch
This command will display the names of all local branches, highlighting the current branch you are on with an asterisk. Reviewing this list is the first step in determining which branches you can delete.
Checking Remote Branches
To view the remote branches, the command is slightly different:
git branch -r
This command will show you all the branches that exist on your remote repository. Understanding these branches is essential, as it allows you to identify which local branches are no longer associated with any remote counterpart.
Identifying Local Branches Not on Remote
Using Git Command to Compare
To identify which local branches do not have a corresponding remote branch, you can use the following command:
git branch --no-merged
This command lists all branches that have not been merged into the current branch, helping you spot candidates for deletion. It's worth noting that unmerged branches are often a sign of incomplete work, so take the time to investigate their current status.
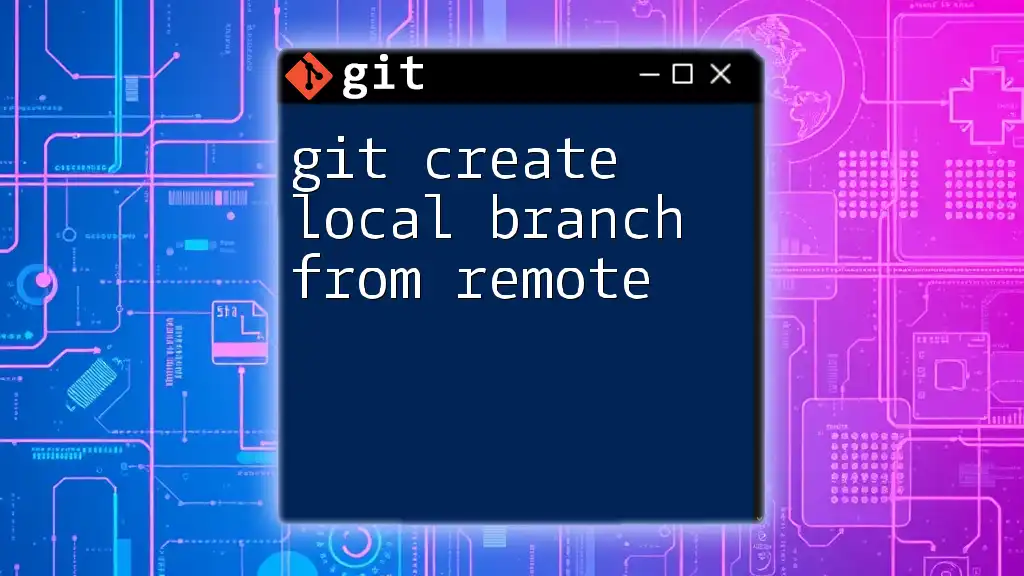
Deleting Local Branches Not on Remote
Precautions Before Deleting
Before you start deleting branches, it’s prudent to check if you have uncommitted work or important changes in those branches. You can create a backup of a branch by checking it out to a new branch:
git checkout -b backup-branch-name
This way, you ensure that you have a fallback in case you need to refer back to any code or changes present in the branch you are about to delete.
Deleting Single Local Branch
If you're ready to delete a local branch that you’ve confirmed is safe to remove, use the following command:
git branch -d branch-name
The `-d` flag stands for "delete," and it safely deletes the branch if it has been merged with your current branch. However, if the branch hasn't been merged and you wish to force-delete it, you can use:
git branch -D branch-name
This command removes the branch without considering merge status, which should be used with caution.
Deleting Multiple Local Branches
Using a Pattern to Delete
If you need to delete multiple branches matching a specific pattern, you can leverage a combination of shell commands:
git branch | grep 'pattern' | xargs git branch -d
Here, `grep` filters the branches to match your pattern, and `xargs` pipes the results into a delete command. This approach is efficient for cleaning up related branches after a feature development phase.
Batch Deletion of Untracked Branches
To delete multiple branches that have been merged, excluding, for example, your main branch, you can run:
git branch --merged | grep -v 'main' | xargs git branch -d
This command lists all branches that have been merged and uses `grep` to exclude any that you want to retain, thus ensuring you can clean up your branches effectively.
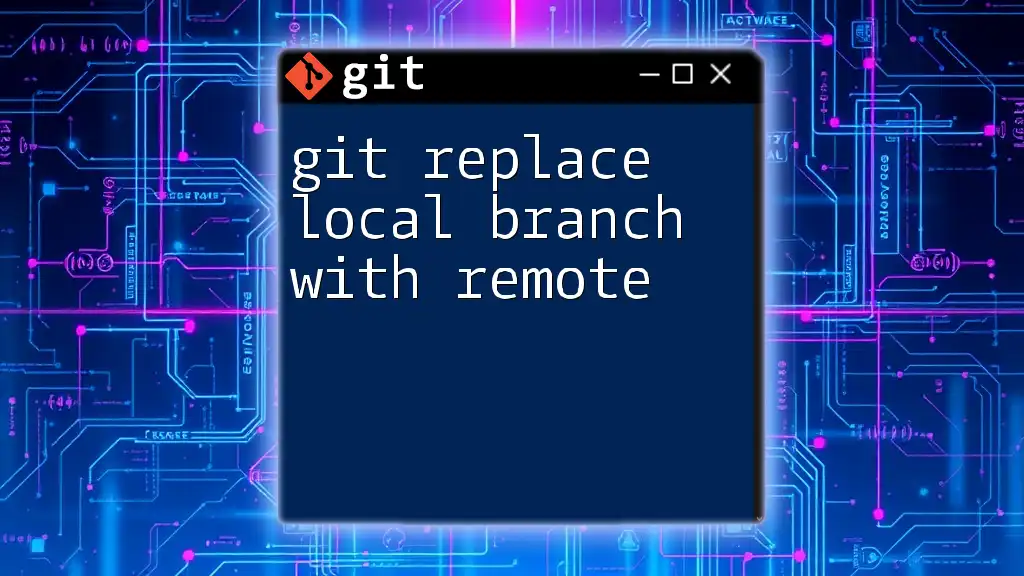
Best Practices for Managing Local Branches
To maintain a healthy and efficient Git workflow, consider these best practices:
- Conduct regular clean-up sessions by reviewing your branches periodically.
- Merging branches upon completion of a feature or fix helps ensure they don’t clutter your repository.
- Establish naming conventions for your branches, which can facilitate easier identification and management.
- You might also devise scripts or simply set reminders in your calendar to help automate or establish a routine for cleaning up.
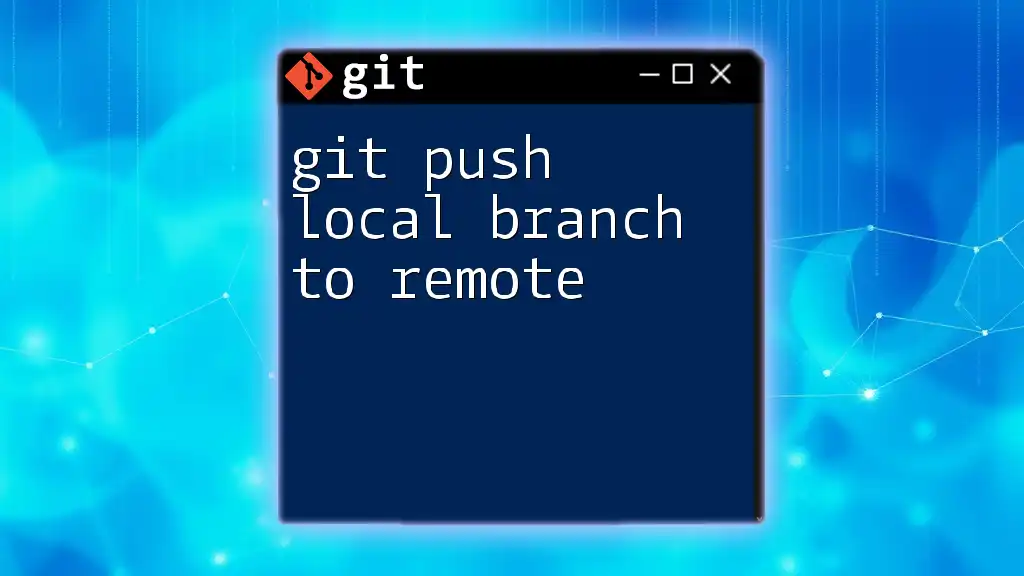
Troubleshooting Common Issues
Branch Cannot Be Deleted
If you try to delete a branch and receive an error, it might be due to the branch having unmerged changes. To resolve this, either merge the branch into your current branch or use the force delete option with:
git branch -D branch-name
Recovering Deleted Branches
In case you've accidentally deleted a branch and need to recover it, you can use the reflog feature:
git reflog
The reflog records updates to the tip of branches, including deletions. You can find the commit hash of the branch you deleted and recreate it by running:
git checkout -b branch-name hash
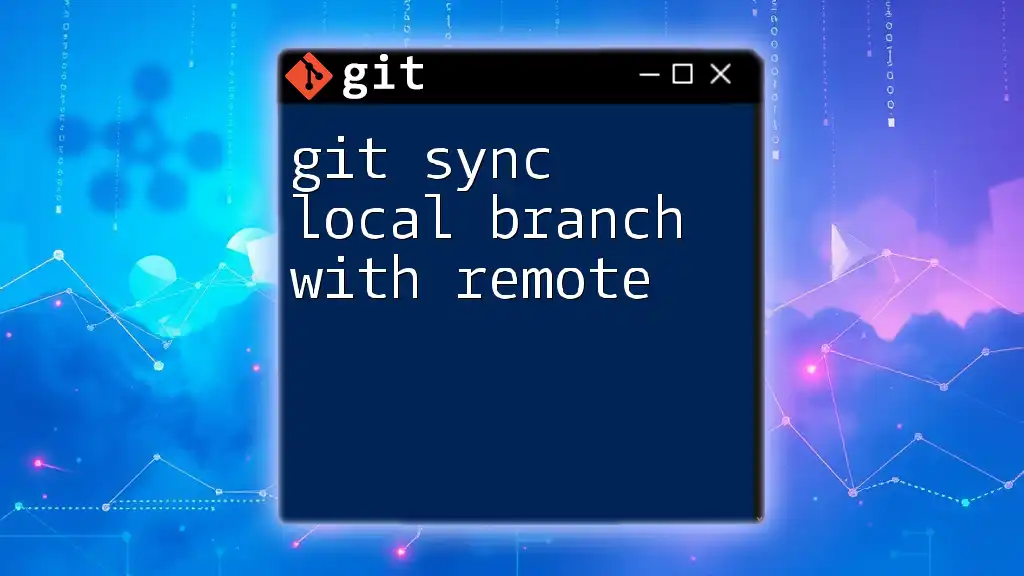
Conclusion
Efficiently managing your local branches is essential for maintaining a clean and organized Git repository. By learning how to delete local branches not on remote, you can streamline your development process and focus on what truly matters—your code. Adopting best practices and regularly cleaning up your workspace will foster better collaboration and productivity within your projects. Join our courses for more insights and mastery of Git commands!
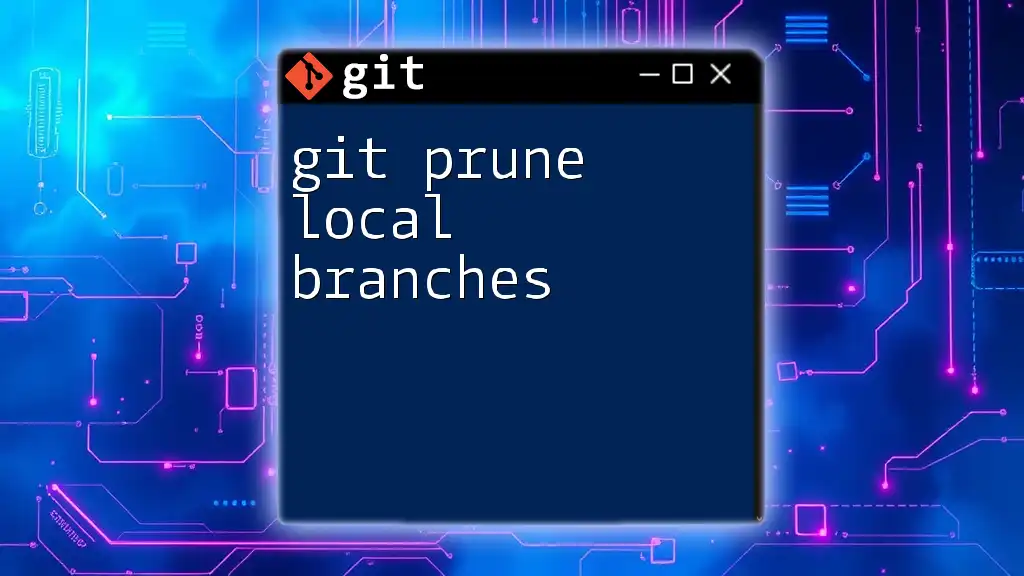
Additional Resources
For further reading and learning, consider exploring the official Git documentation, recommended books, and tutorials dedicated to Git branch management to enhance your skills.