To sync your local branch with the remote branch in Git, you can use the following command to fetch the latest changes and merge them into your current branch.
git pull origin <branch-name>
Replace `<branch-name>` with the name of the remote branch you want to sync with.
Understanding Local and Remote Branches
What Is a Local Branch?
A local branch is a version of a project that you can modify freely without affecting the central or shared codebase. Local branches are created on your own machine and enable you to work on features, fix bugs, and experiment with code without disrupting others' work. This environment encourages creativity and productivity since you can commit changes at your own pace.
What Is a Remote Branch?
A remote branch exists on a remote repository (like GitHub or GitLab) and serves as a central reference point for collaboration. Unlike local branches, remote branches are shared among team members, ensuring that everyone has access to the same codebase. When you push your changes, you update the remote branch, making your modifications visible to the entire team.
Key Differences Between Local and Remote Branches
While local branches are for personal development, remote branches facilitate collaboration. Local changes do not affect the remote branch until you push them, which is crucial to avoid unnecessary conflicts. Always ensure that your local branch is in sync with the corresponding remote branch before making significant changes.
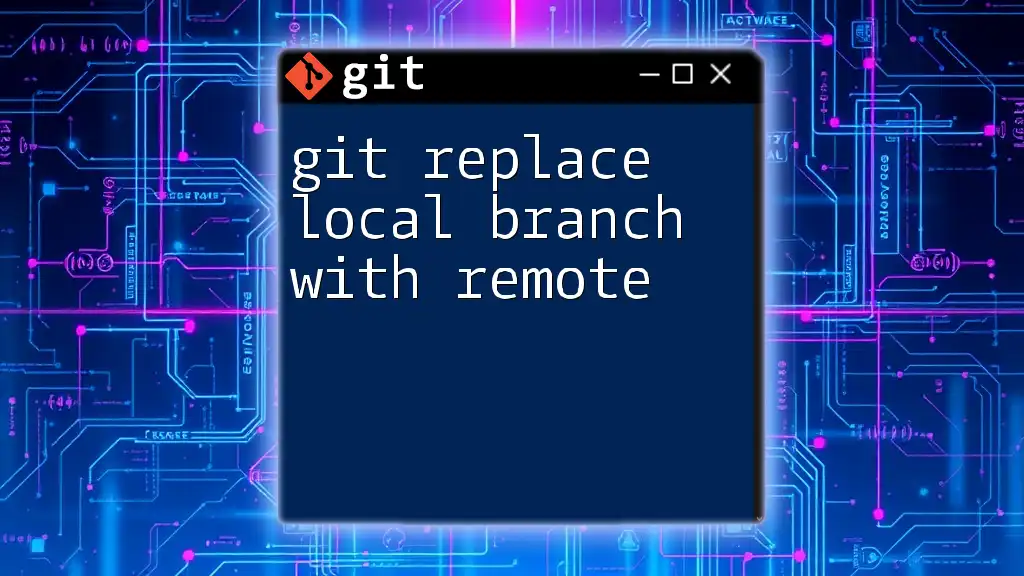
Preparing Your Environment
Setting Up Your Git Repository
Before you can sync your local branch with a remote repository, you need to set up a Git repository. This can be done easily by initializing a new Git project:
git init
This command creates a new subdirectory named `.git` that contains all the files necessary for version control.
Adding a Remote Repository
To sync your local branch with a remote, you must first establish a connection to that remote repository. You can do this using the `git remote add` command:
git remote add origin https://github.com/username/repository.git
In this command, `origin` is an alias for the remote repository. It simplifies referring to your main remote URL during future commands, such as pushing or fetching changes.
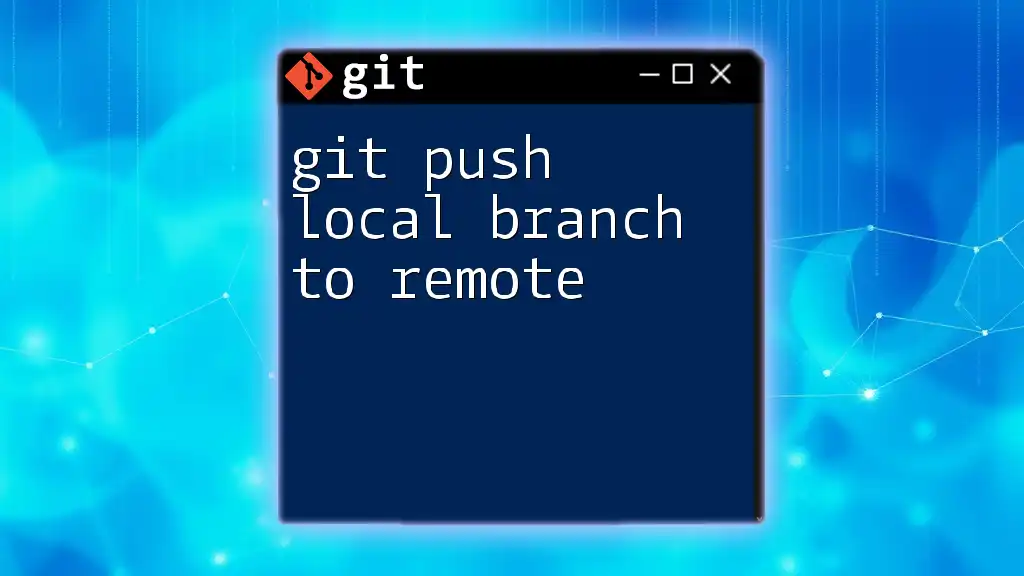
Syncing Your Local Branch with the Remote Branch
Fetching the Latest Changes from the Remote
Before syncing, it is crucial to gather the latest updates from the remote repository. This is accomplished with the `git fetch` command:
git fetch origin
The `git fetch` command retrieves the latest changes from the remote repository without merging them into your local branch. This is particularly useful for reviewing history before integrating any updates into your code.
Merging Changes Into Your Local Branch
Once you have fetched the latest changes, you need to merge them into your local branch. You can use the `git merge` command:
git merge origin/main
If there are conflicting changes between your local branch and the fetched changes, Git will notify you, allowing you to resolve these conflicts manually. Understanding the difference between fast-forward and three-way merges is vital here. A fast-forward merge occurs when there are no diverging changes, effectively moving the branch pointer forward. On the other hand, a three-way merge requires Git to combine multiple sources of changes, potentially leading to conflicts that need resolution.
Rebasing Your Local Branch
An alternative to merging is to use `git rebase`, which can result in a cleaner project history. Rebasing takes your local commits and replays them on top of the latest commits from the remote branch:
git rebase origin/main
This approach can streamline your commit history and make it easier to follow. However, it's essential to avoid rebasing public branches that other collaborators might rely on, as it can lead to confusion and inconsistency.
Pushing Your Local Changes to the Remote
Once your local branch is in sync with the remote branch and you have committed your desired changes, it’s time to push them to the remote repository:
git push origin main
If you attempt to push and receive an error message, it may be due to your local branch falling behind the remote. In such cases, you might need to fetch and merge the remote changes again or consider a `git pull` to automatically do both.
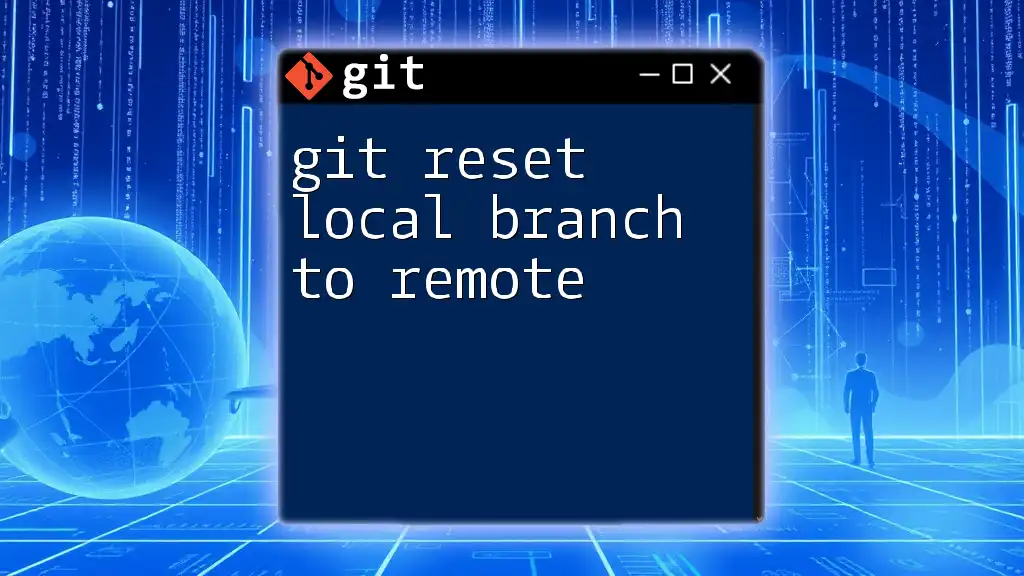
Troubleshooting Common Issues
Handling Merge Conflicts
Merge conflicts are an unavoidable part of collaborative development. When encountered, Git will mark the areas of conflict within the affected files. Here’s how to address conflicts:
- Open the file where the conflict occurred.
- Search for the conflict markers (`<<<<<<<`, `=======`, `>>>>>>>`) and resolve the differences manually.
- After resolving the conflicts, add the file using:
git add filename
- Finally, complete the merge process by committing the resolved changes:
git commit -m "Resolved merge conflict"
Dealing with Diverged Branches
When your local and remote branches have diverged, you’ll need to bring them back in sync. First, fetch the latest changes from the remote. If conflicts arise, resolve them as described earlier. After addressing any discrepancies, ensure that your commit history aligns correctly with the remote branch.
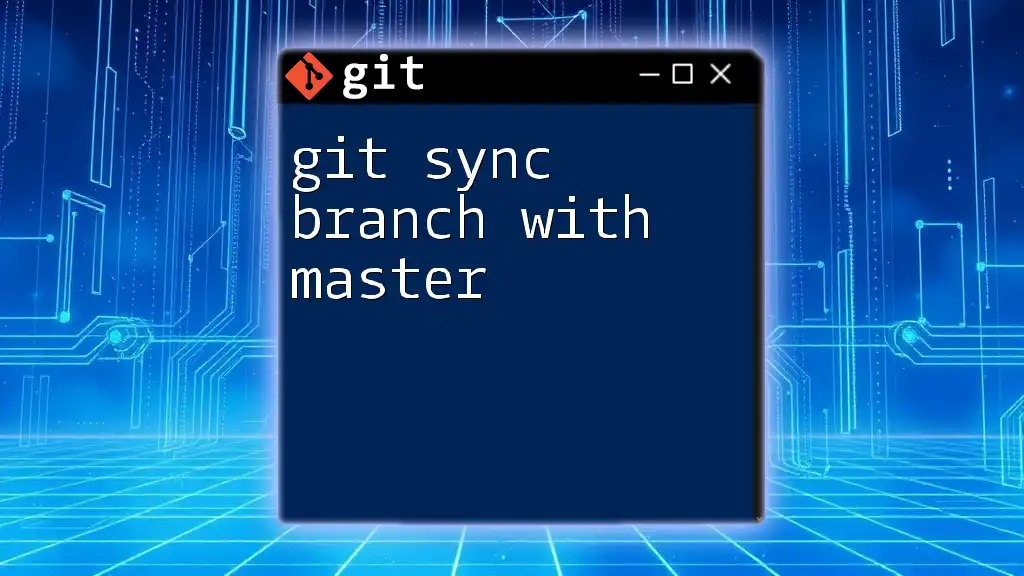
Best Practices for Syncing Branches
Pull Frequently
Make it a habit to pull frequently from the remote repository, reducing the chances of diverging branches. This habit ensures that the code you are working on is up to date, minimizing potential merge conflicts.
Consistent Commit Practices
When making changes, commit often and with clear, descriptive messages. This practice not only helps you keep track of your own changes but also aids other team members in understanding the evolution of the codebase.
Stashing Changes Before Syncing
If you have uncommitted changes on your local branch and want to sync with the remote, you can stash your changes temporarily:
git stash save "message"
This command saves your modifications, allowing you to pull or fetch updates without losing your work. Once you have synced your branches, retrieve your stashed changes with:
git stash pop
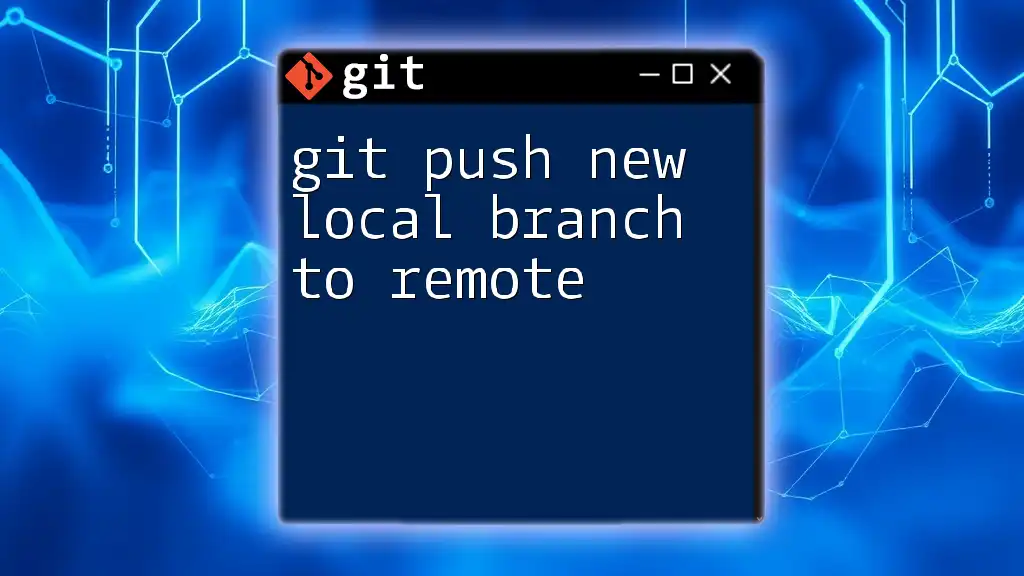
Conclusion
Maintaining an up-to-date local branch by syncing with remote repositories is a fundamental aspect of using Git effectively. By mastering commands such as `git fetch`, `git merge`, and `git rebase`, you can ensure your contributions are valuable and collaborative. Regular practice will help solidify these concepts, enabling smoother teamwork and more efficient development processes.
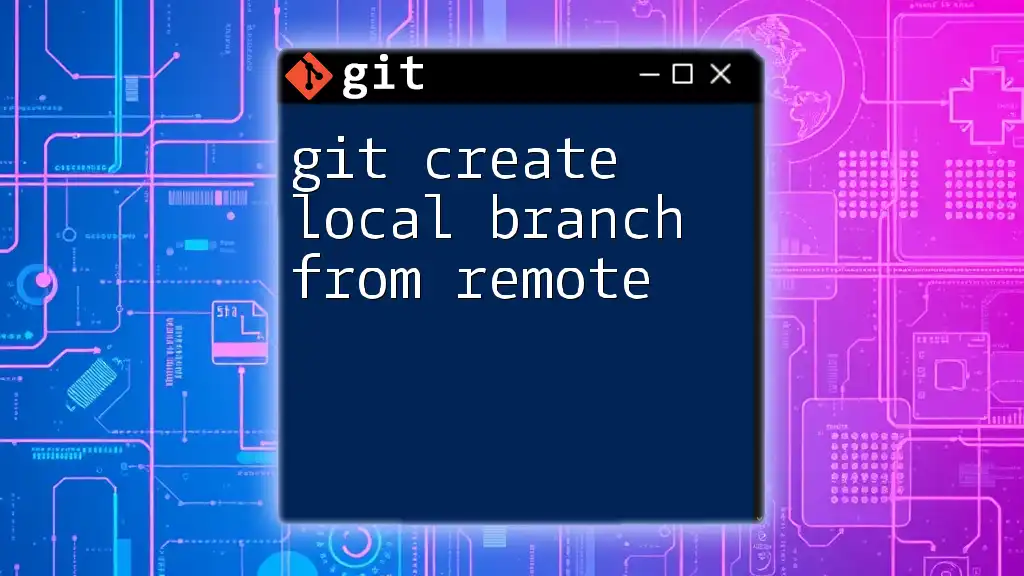
Additional Resources
For further learning, refer to the official Git documentation and various online tutorials to deepen your understanding of Git workflows and best practices. Engaging with these resources will enhance your ability to navigate challenges in collaborative environments confidently.