To push a local branch to a remote repository in Git, use the command below, replacing `your-branch-name` with the name of your local branch and `origin` with the name of your remote repository if different.
git push origin your-branch-name
Understanding Git Branches
What is a Git Branch?
A Git branch is essentially a pointer to a specific commit within your project’s history. By default, when you create a new Git repository, you start on a branch called `main` (previously often referred to as `master`). Branches allow you to create isolated environments for features or fixes, which helps maintain a clean and organized workflow.
Real-world Analogy
Imagine a tree with branches; each branch represents a different path your project can take. You can work on various features or fixes independently without affecting the main trunk (or the primary branch of your project).
Types of Branches in Git
- Local Branches: These are branches that exist only in your local repository. You can create and modify these branches without impacting others.
- Remote Branches: These represent branches in remote repositories like GitHub or GitLab. They serve as a reference for the state of other people's work.
- Tracking Branches: These are local branches that have a direct correspondence with remote branches. They allow you to keep track of remote changes easily.
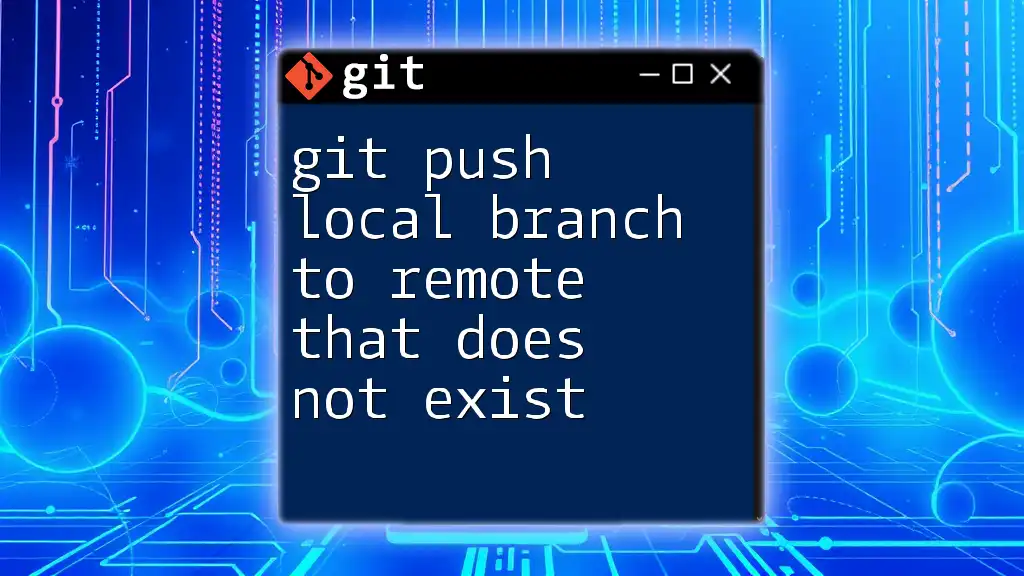
Setting up Your Local Repository
Initial Setup
To start working with Git, you need to create a local repository.
- Creating a Local Repository
You can create a new local repository using the following commands:
git init my-repo
cd my-repo
- This initializes a directory called `my-repo` and moves you into it!
Creating a New Branch Locally
Now that you have your local repository set up, you can create a new branch.
- How to Create a New Branch
Use the command below to create a new branch and switch to it:
git checkout -b my-feature
- Switching Between Branches
If you need to switch back to the main branch, the command is:
git checkout main
This functionality allows you to move freely between different parts of your project's development.
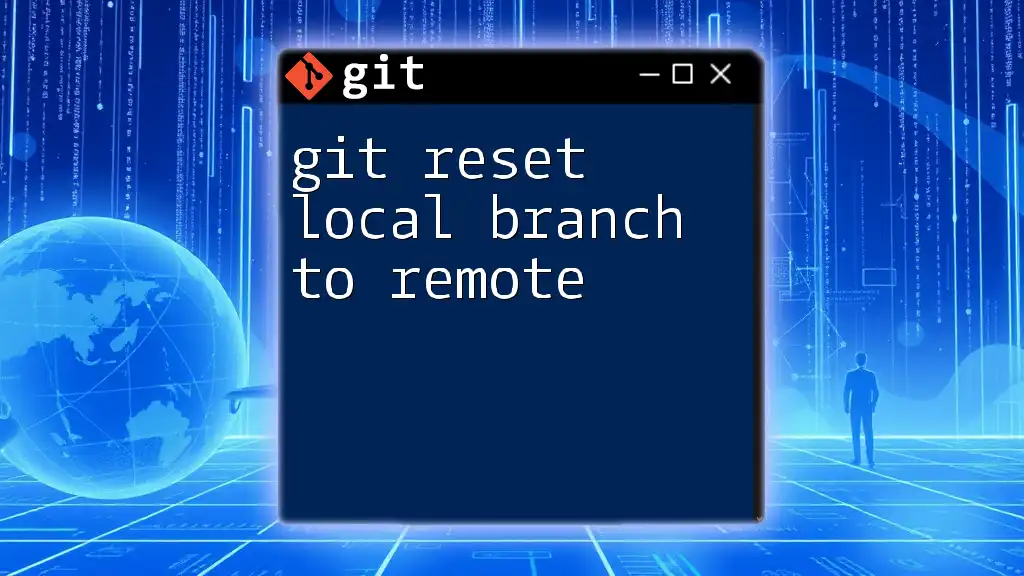
The Process of Pushing a Local Branch to Remote
Understanding Remote Repositories
Before you can push your local branch, it's essential to understand what a remote repository is. A remote repository is a version of your project hosted on the internet or another network. Common platforms include GitHub, GitLab, and Bitbucket.
Adding a Remote Repository
To push your changes, you first need to link your local repository to a remote one. You can do this by using:
git remote add origin https://github.com/username/my-repo.git
This command associates your local repository with the remote repository located at the specified URL.
Pushing a Local Branch
The `git push` Command
The `git push` command is used to send your committed changes to a remote repository. The basic syntax of the command is:
git push <remote> <branch>
Here, `<remote>` is usually `origin` (the default name for your remote repository), and `<branch>` is the name of the branch you want to push.
Code Snippet Example
For example, to push your local branch called `my-feature`, you would use:
git push origin my-feature
Explaining the Components
-
Origin: This refers to the default name Git uses for your remote repository. Think of it as a nickname you can use to refer to the repository on GitHub or GitLab.
-
my-feature: This is your local branch name. You are telling Git that you want to upload the changes from this specific branch to the remote repository.
Pushing New Branch to Remote
When you push a branch for the first time, it needs a connection between your local branch and the remote branch. This is established using the `-u` option, which creates a tracking relationship.
For the initial push, use:
git push -u origin my-feature
What happens here is that your local branch `my-feature` will be pushed to the remote repository and associated with a remote branch of the same name. This allows future pushes to be simplified, as you would simply use:
git push
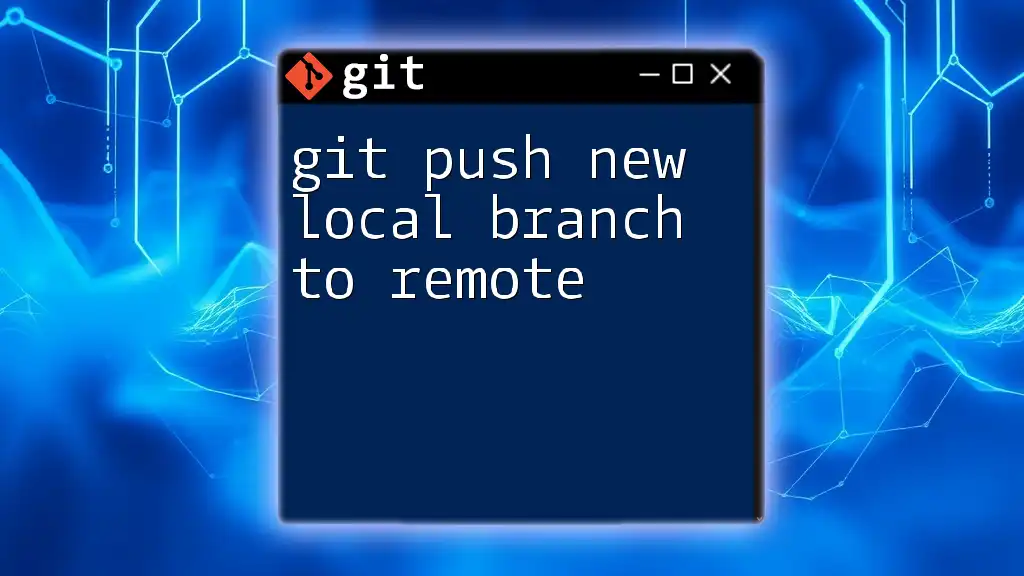
Handling Common Issues
Authentication Problems
One common hurdle when pushing to a remote repository is authentication issues. If you encounter problems, consider the following:
- Make sure you have the correct permissions to push to the repository.
- If using HTTPS, be aware that you might need to provide credentials. Setting up SSH keys might provide a smoother experience, eliminating the need for username/password prompts.
Push Failures
There are times when your push may fail. This can occur due to:
- Outdated Local Branch: If your local branch is behind the remote branch, Git will reject the push. You'll need to pull the latest changes first.
To resolve this, run:
git pull origin my-feature
- Merge any conflicts that arise, then attempt the push again.
Conflicts and Resolutions
Merge conflicts happen when two divergent changes are made to the same line in a file. If you encounter this, you will need to resolve the conflicts manually.
- Check which files have conflicts.
- Open the files, look for conflict markers (e.g., `<<<<<< HEAD`), and decide how to integrate the changes.
- After resolving conflicts, stage your changes with:
git add <file_name>
- Complete the merge with a commit:
git commit -m "Resolved merge conflict"
Finally, you can retry pushing your changes.
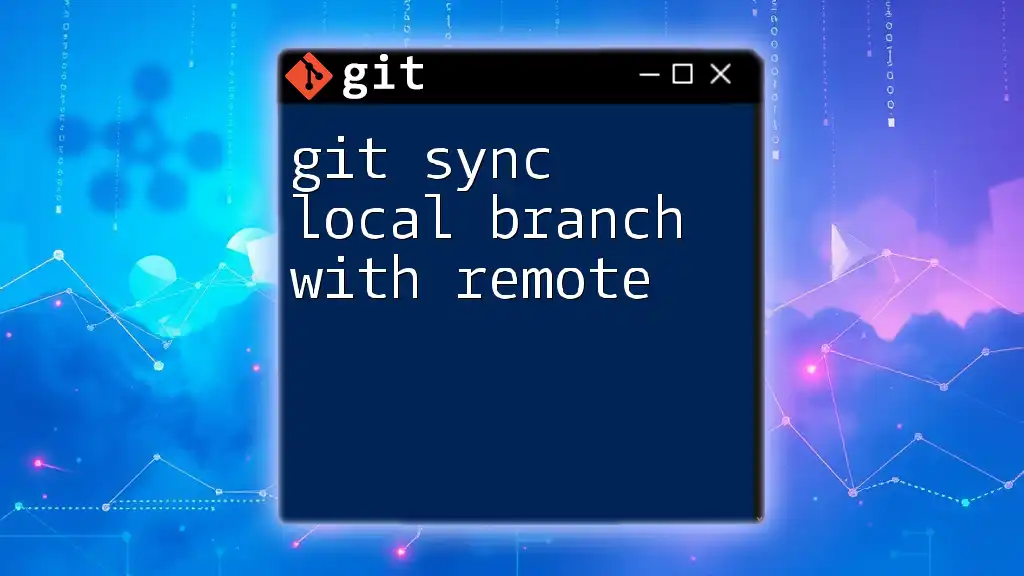
Best Practices for Using `git push`
-
Frequency of Pushing Changes: It's a good habit to push often. Smaller, frequent updates make it easier to track changes and reduce conflicts.
-
Commit Messages: Always use clear and descriptive commit messages. This will make it easier for you and others to understand the project's history.
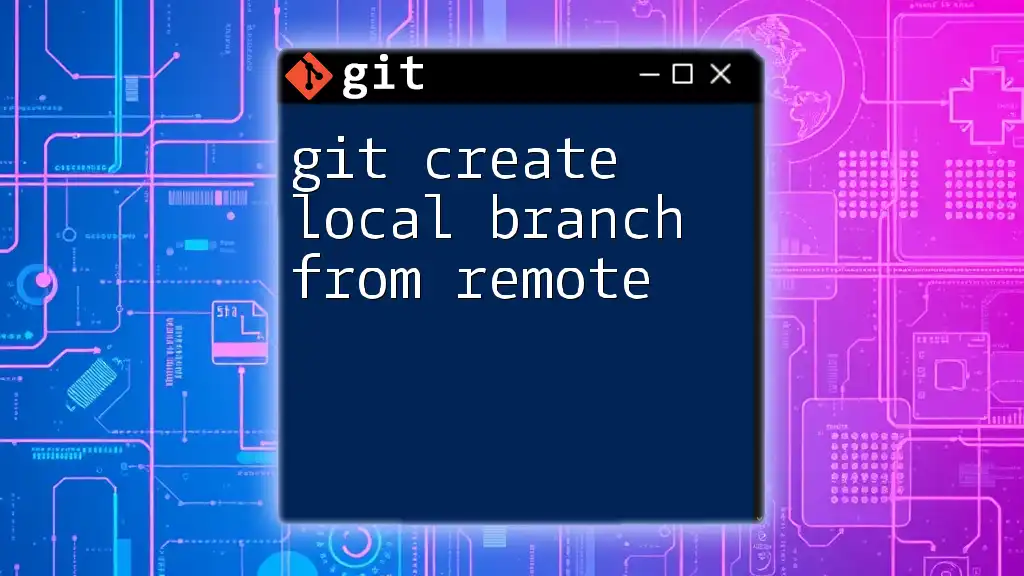
Conclusion
In summary, pushing a local branch to a remote repository is a critical aspect of efficient collaboration with Git. By mastering commands like `git push`, you ensure that your work is not just isolated to your computer but available to your team as well. The more you practice and integrate these commands into your routine, the more intuitive they will become.
Consider experimenting with different scenarios in your local repository, and don't hesitate to reach out to the community for feedback or assistance.
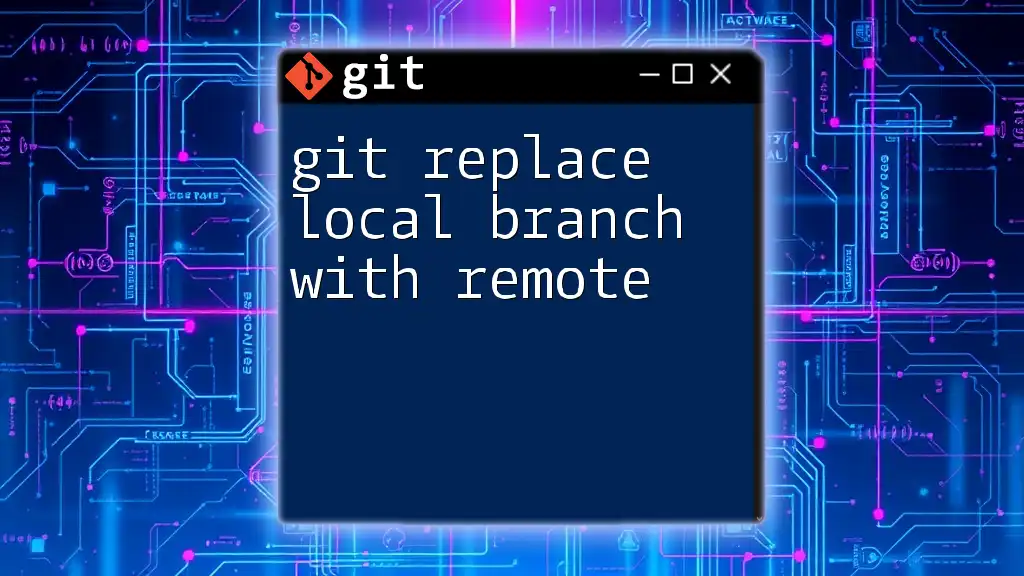
Additional Resources
Refer to the official Git documentation for in-depth insight and instructions. Consider taking online tutorials or courses to strengthen your understanding and application of Git in various scenarios. Engage with community forums to discuss challenges and solutions pertaining to Git.
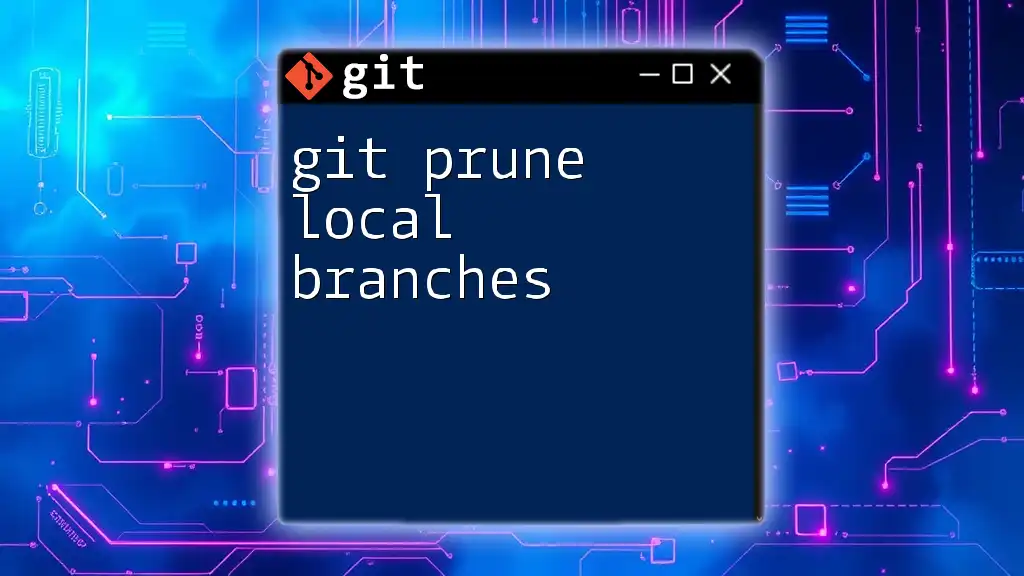
FAQ Section
Common Questions about Pushing in Git
- What if the remote branch doesn’t exist yet?
When pushing a branch for the first time, Git can automatically create a remote branch if it doesn't already exist, provided you include the `-u` option.
- How can I delete a remote branch?
To delete a remote branch, you can use:
git push origin --delete branch-name
- Can I push without a tracking branch?
Yes, but you need to specify the remote and branch explicitly each time unless you set up a tracking branch with the `-u` flag during your first push.