To point a local Git repository to a remote repository, use the `git remote add` command followed by the desired remote name and the repository URL.
git remote add origin https://github.com/username/repo.git
Understanding Local and Remote Repositories
What is a Local Repository?
A local repository is a directory on your machine where you store your project files and the history of changes made to those files. This repository includes a special `.git` directory that tracks version control history, allowing you to see what changes have been made, revert to previous versions, and manage collaborative edits.
What is a Remote Repository?
A remote repository is a hosted location (typically on platforms like GitHub, GitLab, or Bitbucket) where teams can share their codebase. Remote repositories provide a centralized place where multiple developers can push and pull changes, collaborate efficiently, and back up their work.
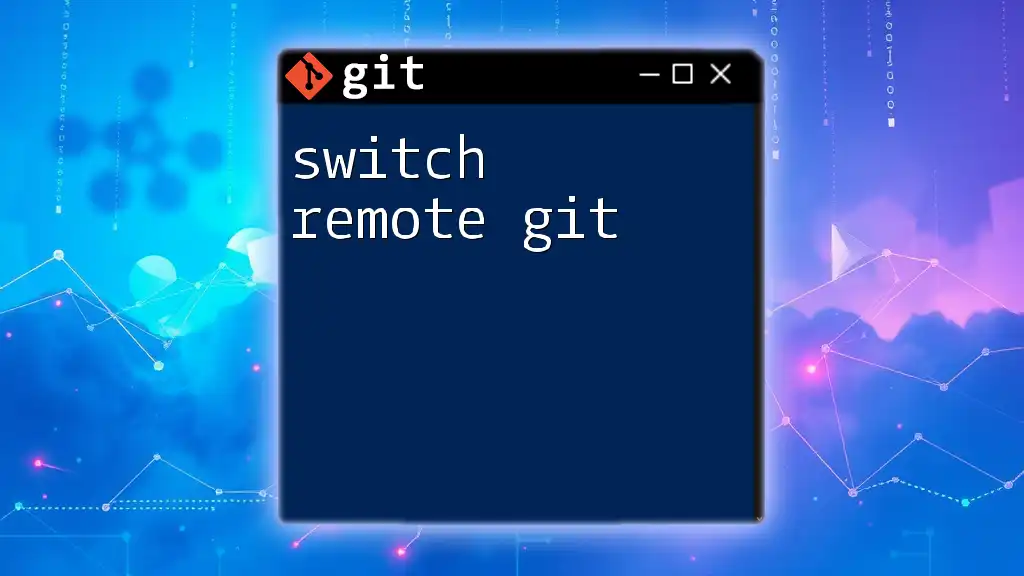
Setting Up Your Local Repository
Initializing a Local Git Repository
Before you can point your local repo to a remote Git source, you need to have a local repository set up. If you haven’t done this yet, initialize your repository with the following command:
git init
This command creates a new directory with `.git` set up, marking it as a Git version control environment.
Adding Files to Your Local Repository
To start tracking changes, you need to add files to your local repository. Use the command:
git add <filename>
You can also add multiple files or even all files in the directory by using:
git add .
Staging changes is crucial since it allows you to select which modifications will be included in your next commit.
Committing Changes Locally
Once you have added your files, the next step is to commit those changes:
git commit -m "Your commit message"
Your commit message should succinctly describe the changes made. Well-written commit messages are vital for maintaining a clear project history.
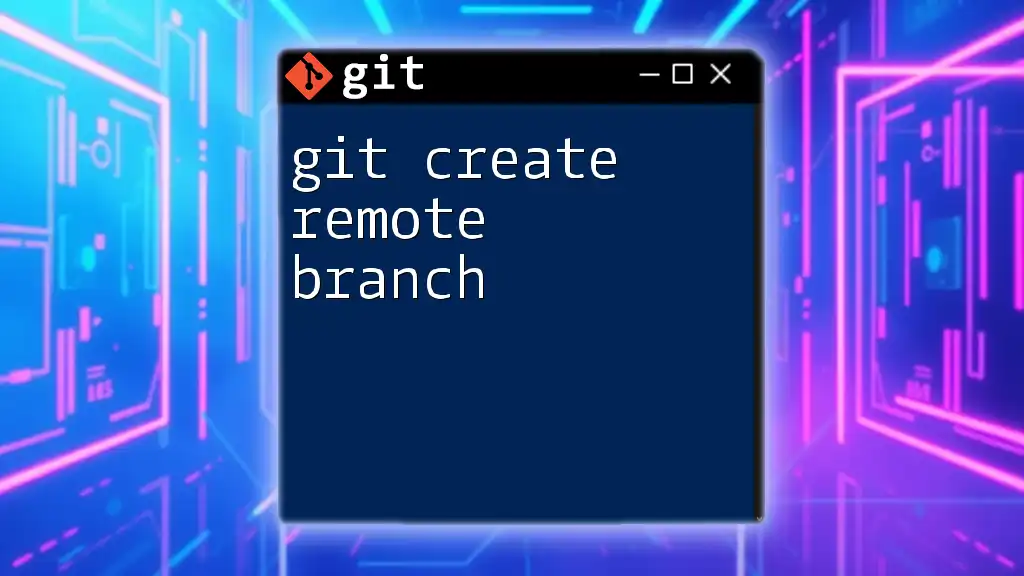
Understanding Remote Repositories
Why Use Remote Repositories?
Utilizing a remote repository encourages:
- Team collaboration: Multiple developers can contribute concurrently without overwriting each other's work.
- Backup and recovery: Your code is safely stored remotely, reducing the risk of data loss.
- Access from multiple devices: You can work on your project from different locations easily.
Common Remote Repository Services
Several platforms facilitate remote repositories, including:
- GitHub: Widely used and great for open-source projects.
- GitLab: Offers integrated CI/CD features.
- Bitbucket: Supports both Git and Mercurial and features a user-friendly interface.
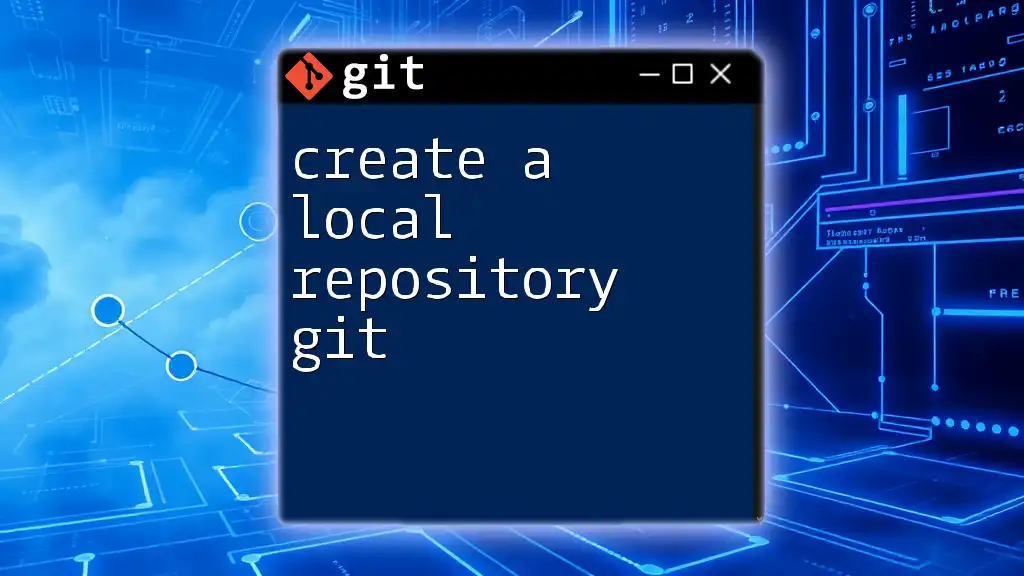
Pointing Your Local Repository to a Remote Repository
How to Create a Remote Repository
First, you’ll need a remote repository created on a platform such as GitHub. Here’s how:
- Go to GitHub and log in to your account.
- Click on the "New" button to create a new repository.
- Fill in the repository name and description, then click on "Create repository."
Connecting Your Local Repository to the Remote Repository
To point your local repo to a remote Git, you need to link your local repository with the remote one. This is done with the command:
git remote add origin <remote-repository-URL>
Here, `origin` is a conventional name given to your primary remote repository. It essentially acts as an alias for the actual remote URL, making it easier to manage.
Verifying the Remote Connection
To confirm that your local repository is successfully linked to the remote repository, use:
git remote -v
This command lists all remote connections, showing you the name (`origin`) and the corresponding URL. You should see the URL of the remote repository listed here.
Pushing Your Local Changes to the Remote Repository
First Push
To send your local commits to the remote repository, execute:
git push -u origin main
In this command, `-u` sets `origin main` as the default upstream branch. This configuration allows you to simply use `git push` for future updates without specifying both the remote and the branch every time.
Handling Possible Errors
Common Error Messages
While connecting your local repository to a remote, you might encounter errors such as:
- fatal: remote origin already exists: This means that a remote named `origin` has already been set up.
Solutions
If you need to change the existing remote URL instead, use:
git remote set-url origin <new-repo-URL>
This command reassigns the `origin` remote to a different URL.
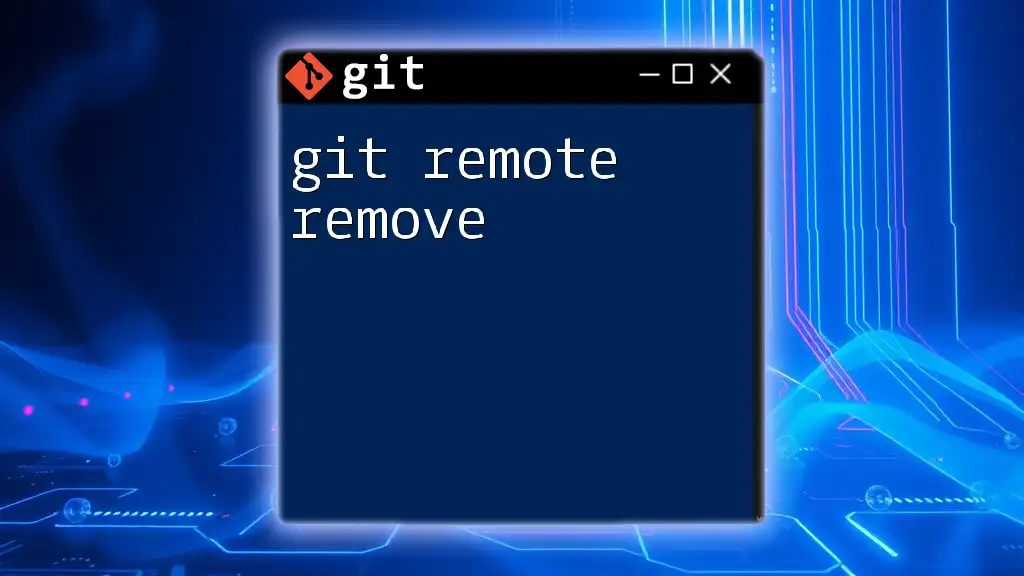
Best Practices for Remote Repository Management
Regularly Syncing Local and Remote Repositories
To avoid merge conflicts and keep your repositories up to date, regularly sync your local changes with the remote by using:
git pull
This command downloads changes from the remote repository to your local machine.
Branch Management Strategies
Branching allows you to work on features or fixes independently. Use:
git checkout -b <branch-name>
to create a new branch. Merging these branches back into the main branch helps maintain a clean project history.
Collaboration Tips
Managing pull requests effectively is crucial when collaborating. Always check for:
- Merge conflicts: These occur when changes in different branches conflict. Resolve them by manually editing files in conflict.
- Code reviews: Regularly review changes before they are merged to maintain code quality.
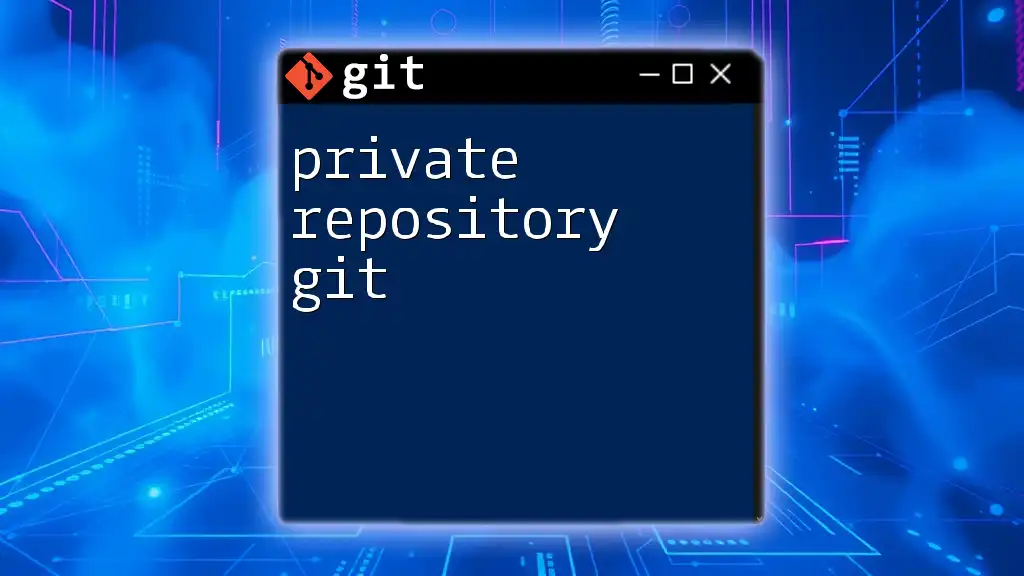
Conclusion
Learning how to point your local repo to remote Git is essential for modern development workflows. Not only does it facilitate collaboration, but it also ensures that your work is safely backed up. Practice these commands, and soon you’ll be managing Git repositories like a pro.
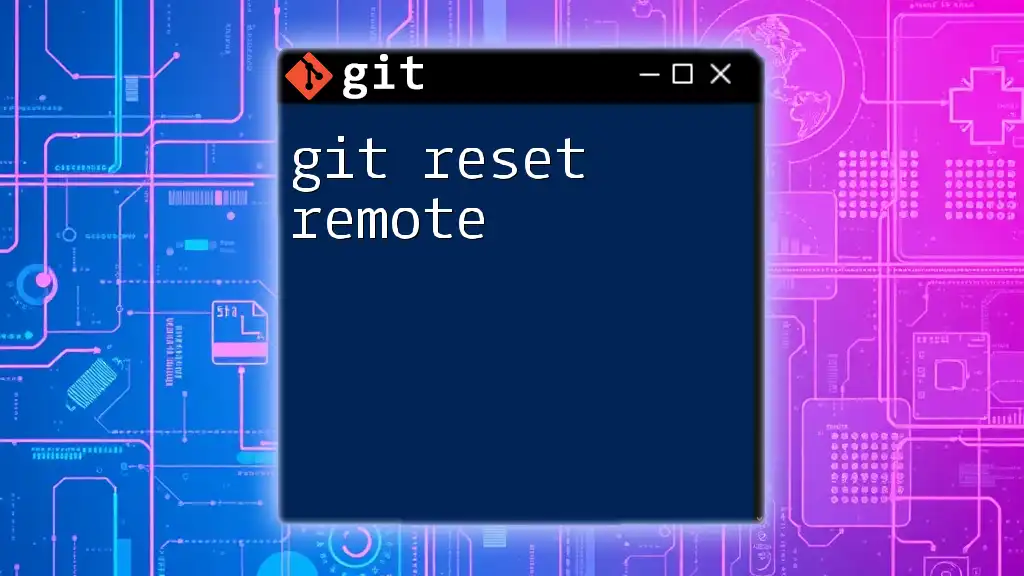
Additional Resources
For deeper understanding, refer to Git’s official documentation and explore tutorials that provide hands-on experiences with Git commands and workflows.
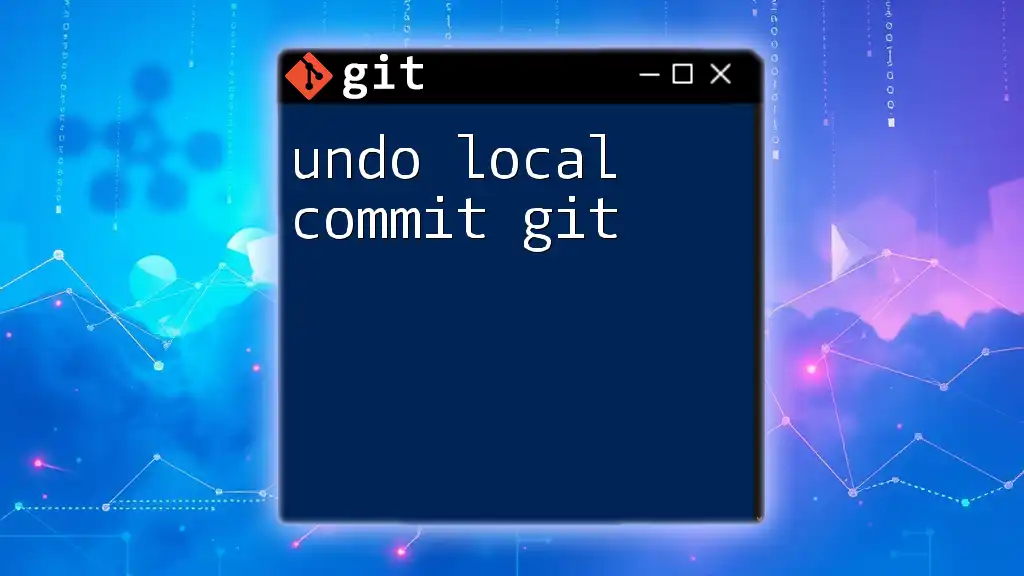
FAQs
How can I change my remote repository URL?
If you need to update your remote repository URL, you can use:
git remote set-url origin <new-repo-URL>
What if I only want to push specific branches?
You can push specific branches using the following syntax:
git push origin <branch-name>
This allows you to control exactly what gets pushed to the remote.