To create a local Git repository, navigate to your desired directory in the terminal and use the command `git init` to initialize a new repository.
cd /path/to/your/directory
git init
Understanding Local Repositories
What is a Local Repository?
A local repository is a Git repository that resides on your own computer. It serves as a personal workspace where you can develop and test your projects without affecting the shared or remote versions. This environment is crucial for safe experimentation and learning. The structure encompasses three main components: your working directory, the staging area, and the local repository itself.
Key Components of a Local Repository
- Working Directory: This is the folder where you actively work on your files. It's everything you see and modify.
- Staging Area (Index): The staging area acts as an intermediary between the working directory and the local repository. Here, you prepare files for commit.
- Local Repository (History): This component holds the history of your project’s changes, allowing you to reference previous versions and manage your development effectively.
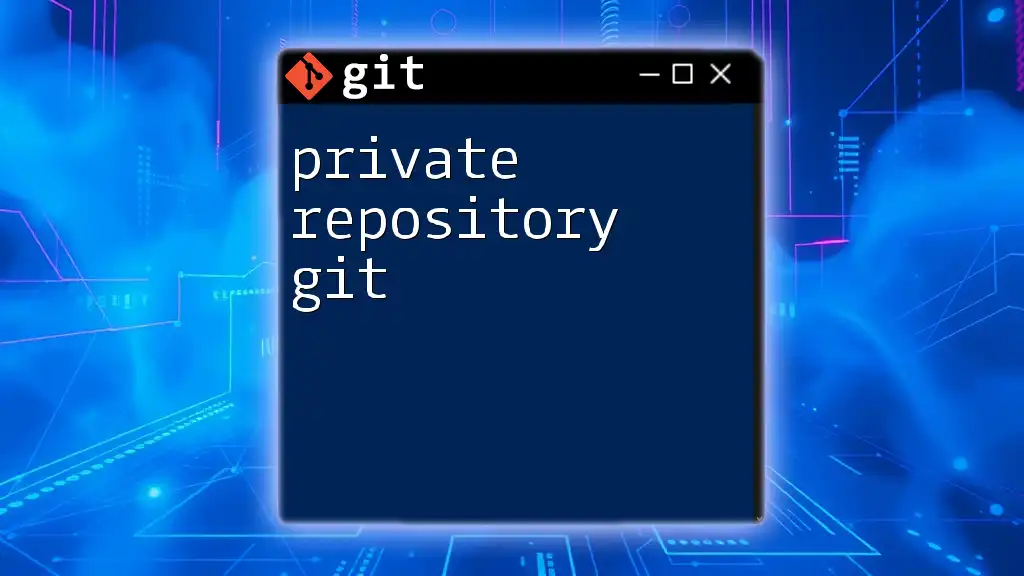
Setting Up Your First Local Repository
Prerequisites
Before you can start the process to create a local repository git, ensure that Git is installed on your system. Follow these steps based on your operating system:
- Windows: Download the installer from the [Git official website](https://git-scm.com/download/win) and run it.
- macOS: You can install Git via Homebrew. Open the terminal and type:
brew install git
- Linux: Install Git using the package manager for your distribution. For Ubuntu, use:
sudo apt-get install git
After installation, verify it by executing the following command in your terminal:
git --version
You should see the version number of Git, confirming it’s successfully installed. Familiarity with the command line will also aid you in executing Git commands smoothly.
Creating a Local Repository
To create your first local repository, you will use the `git init` command. This command initializes a new Git repository.
- First, create a new directory for your project:
Navigate into this directory:mkdir my-first-repo
cd my-first-repo
- Now, run the command to initialize the Git repository:
git init
This process creates a hidden `.git` folder in your project directory. This directory tracks all the changes, file versions, and history.
Understanding the Directory Structure
The .git folder is the core of your local repository. It contains essential components, such as:
- Objects: These are the latest versions of your files.
- Refs: This includes references to branches and tags.
- HEAD: This points to the current branch you're working on.
Understanding this structure is crucial, as it helps you navigate and manage your repository effectively.
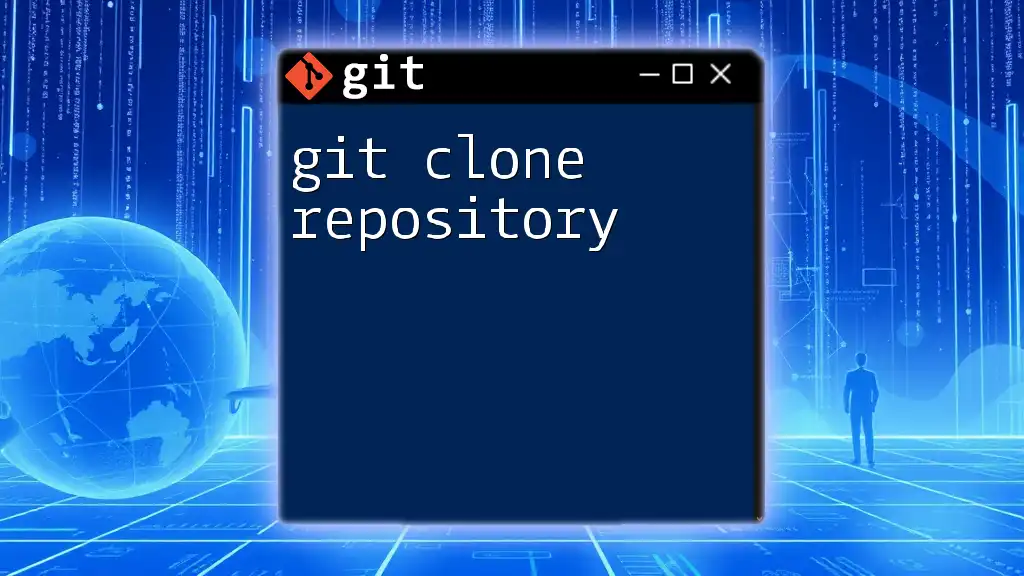
Adding Files to Your Local Repository
Creating Files
You can begin creating files to add to your repository. For instance, you might want to create a simple README file to start documenting your project:
echo "Hello, Git!" > README.md
This command creates a file named `README.md` that contains the text "Hello, Git!".
Tracking Changes
To track your changes, use the `git add` command. This command stages your files for the next commit:
git add README.md
This tells Git that you want to include `README.md` in the next commit. Remember, until you add a file to the staging area, Git does not track it.
Viewing the Status of Your Repository
To see which files are staged, modified, or untracked, utilize the `git status` command. This command provides a quick overview of your repository:
git status
You’ll see output indicating the current state, such as files that are staged for commit and those that are untracked.
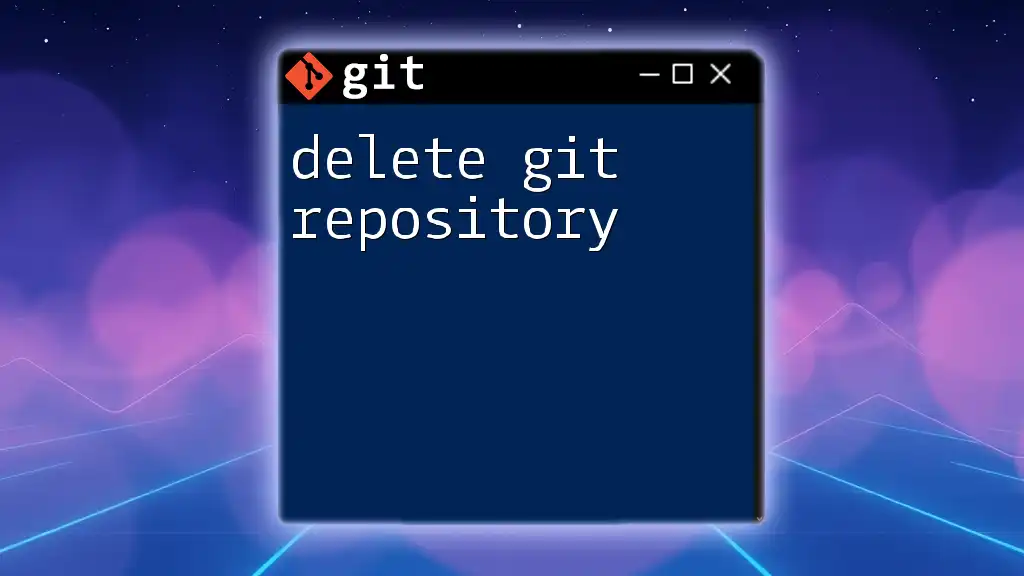
Committing Changes to Your Local Repository
Making Your First Commit
After staging your changes, you are ready to commit them to your local repository. Use the `git commit` command, which saves the staged changes along with a message describing what you’ve done:
git commit -m "Initial commit: Add README.md"
The `-m` flag allows you to provide a message directly in the command line, making it convenient and organized.
Reviewing Commit History
To check the history of your commits, run the `git log` command. This command displays a list of commits made in your repository:
git log
You will see detailed information about each commit, including the commit hash, author, date, and message, allowing you to track the evolution of your project.
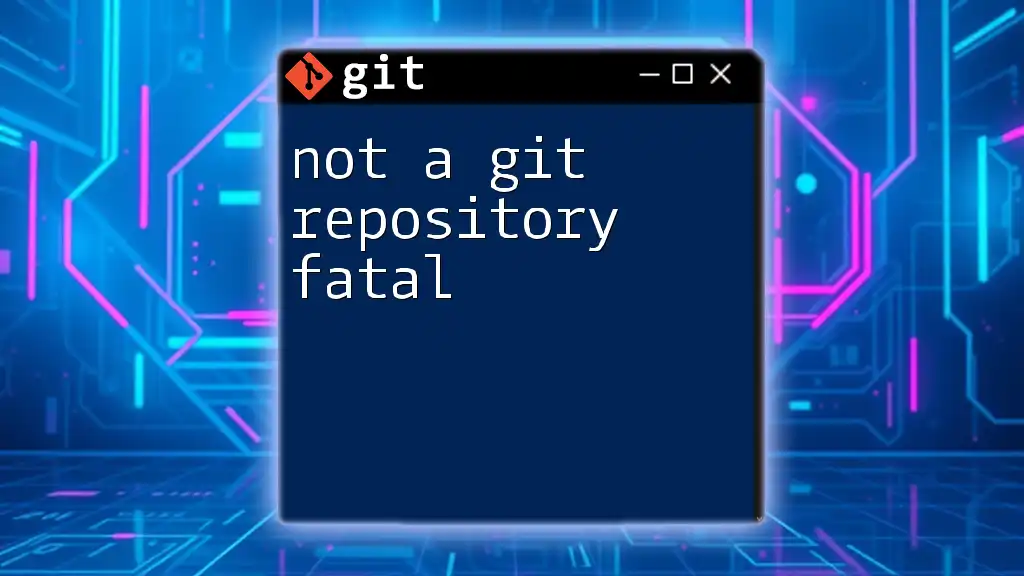
Working with Branches in Your Local Repository
Understanding Branching
Branches in Git enable you to work on different features or fixes independently. They allow you to experiment without destabilizing the main project.
Creating a New Branch
To create a new branch, use the `git branch` command followed by the branch name. After creating a branch, you can switch to it using the `git checkout` command:
git branch feature-branch
git checkout feature-branch
This creates a new branch called `feature-branch` and switches you to it, isolating your changes from the main branch (master/main).
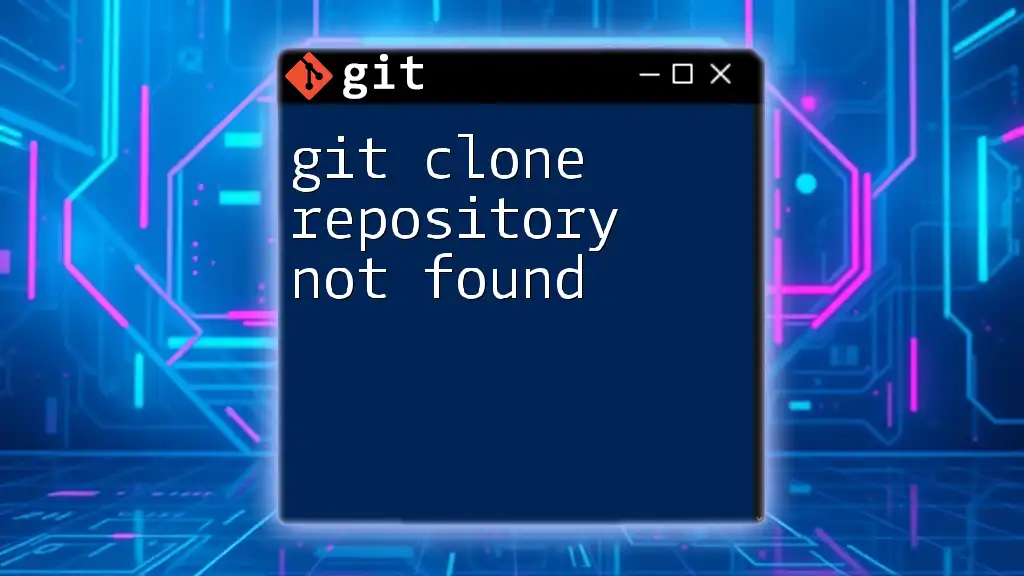
Best Practices for Local Repositories
Organizing Your Repositories
Maintaining an organized structure within your repositories is crucial. Proper naming conventions for both files and branches aid in clarity and understanding.
Frequent Commits
Committing regularly is important for tracking changes effectively. Each commit should serve a clear purpose, and you should write descriptive commit messages that summarize the changes made.
Using .gitignore
Creating a `.gitignore` file is a best practice for excluding files and directories that you do not want to track, such as logs or temporary files.
To create a `.gitignore`, simply add the names of files or directories you want to exclude:
echo "node_modules/" >> .gitignore
This command adds `node_modules/` to the `.gitignore`, ensuring that Git ignores it in future operations.
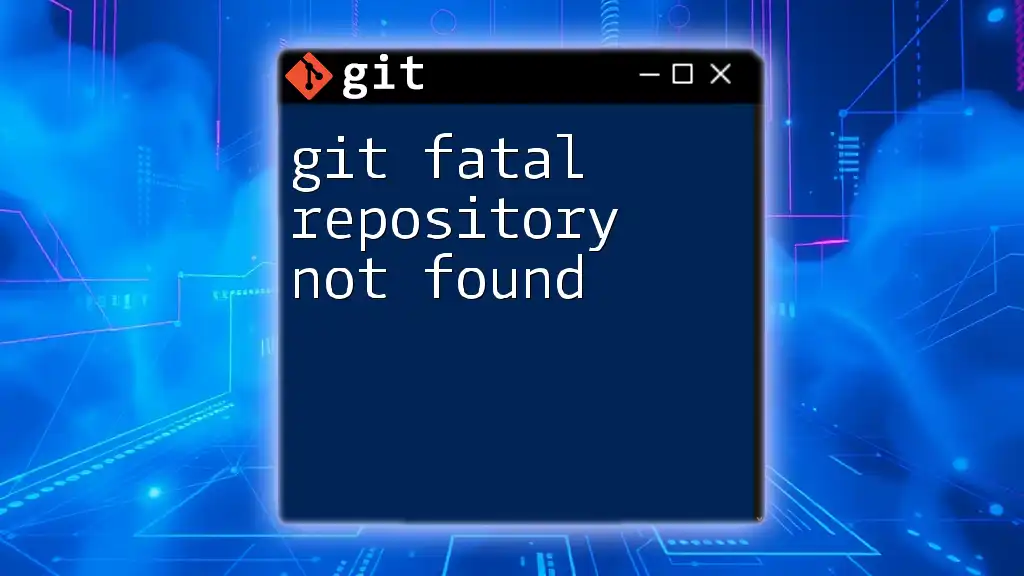
Troubleshooting Common Issues
Common Errors and Their Fixes
As you work with Git, you may encounter some errors:
- Misconfigured repository: Check if you've initialized the repo correctly with `git init`.
- Staging issues: If a file isn't adding as expected, use `git status` to see its state.
By practicing troubleshooting and understanding commands, you can effectively resolve these issues.
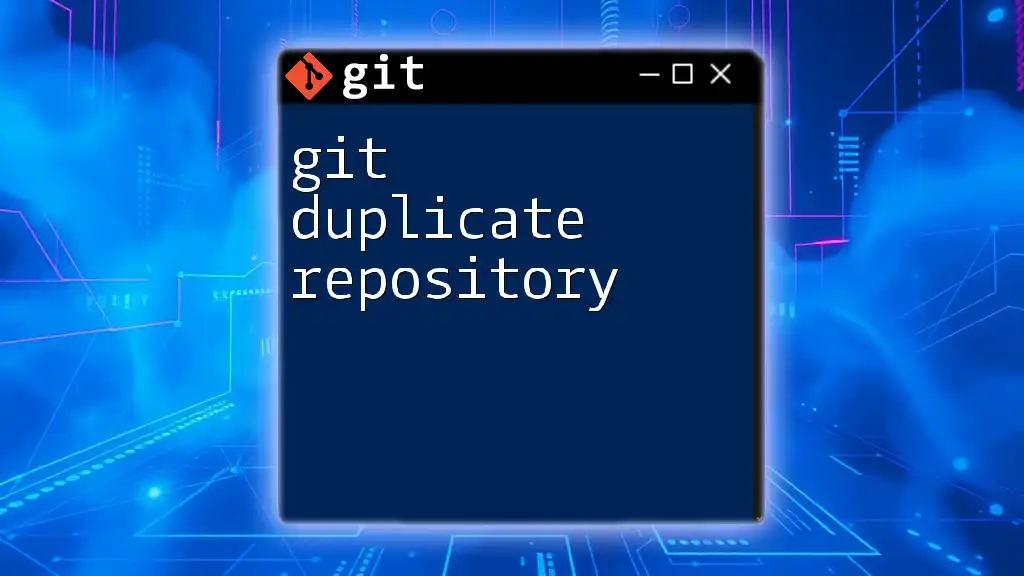
Conclusion
Creating a local repository in Git is a foundational skill for any developer. By following the outlined steps, you can confidently set up a local workspace, add and commit changes, and explore branching.
As you continue to practice and utilize these commands, you'll become proficient with Git, enhancing your software development process. Explore additional resources, documentation, and community forums for further learning and support.