To create a new Laravel application and initialize it as a Git repository, you can use the following commands:
composer create-project --prefer-dist laravel/laravel your-app-name && cd your-app-name && git init
What is Laravel?
Laravel is a powerful PHP framework designed for building modern web applications. It follows the MVC (Model-View-Controller) architectural pattern, which allows developers to build applications in a cleaner and more organized manner. The framework comes packed with features such as routing, middleware, templating, and Eloquent ORM, which makes it an excellent choice for both beginners and seasoned developers.
Key Features of Laravel
- Elegant Syntax: Laravel provides an intuitive and clean syntax, allowing for quicker development and easier readability.
- Built-in Authentication: Implements secure authentication features out of the box.
- Migration System: Keeps the database schema in sync and allows for easy updates and version control.
- Artisan Console: A powerful command-line tool that can handle repetitive tasks, reducing friction in development.
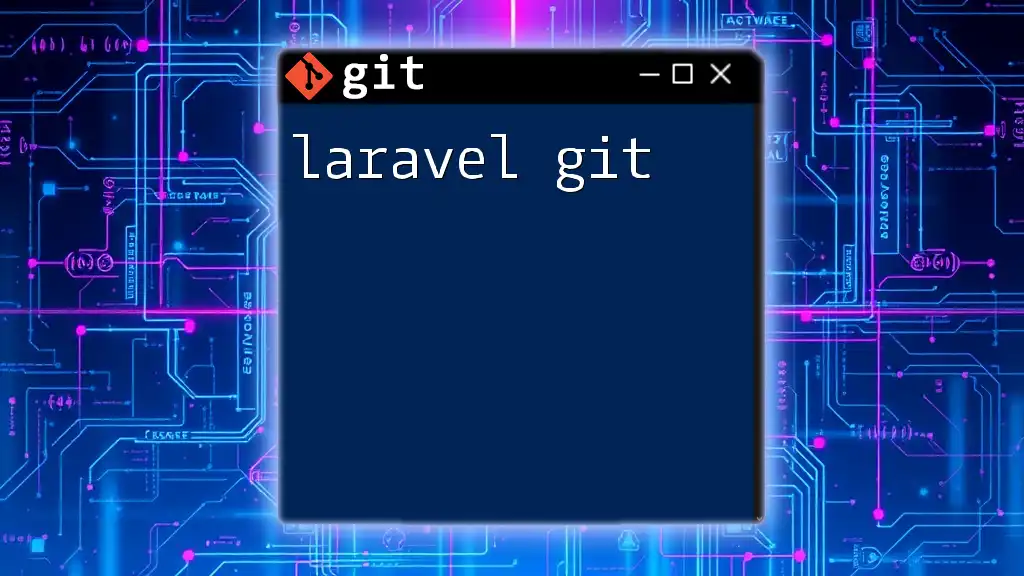
Setting Up Your Laravel Environment
Installing Composer
Composer is a dependency management tool for PHP that simplifies the installation and updating of libraries required for your Laravel application.
To install Composer on your machine, execute the following commands in your terminal:
curl -sS https://getcomposer.org/installer | php
mv composer.phar /usr/local/bin/composer
This downloads and installs Composer globally on your system, allowing you to use it from any directory.
Installing Laravel
Once Composer is installed, you can create a new Laravel project. We will use Composer to globally install the Laravel installer:
composer global require laravel/installer
With the installer ready, you can now create a new Laravel project using:
laravel new my-laravel-app
Creating a New Laravel Project
This command sets up a new directory named `my-laravel-app`, downloading all of Laravel's files and dependencies in one swift move.
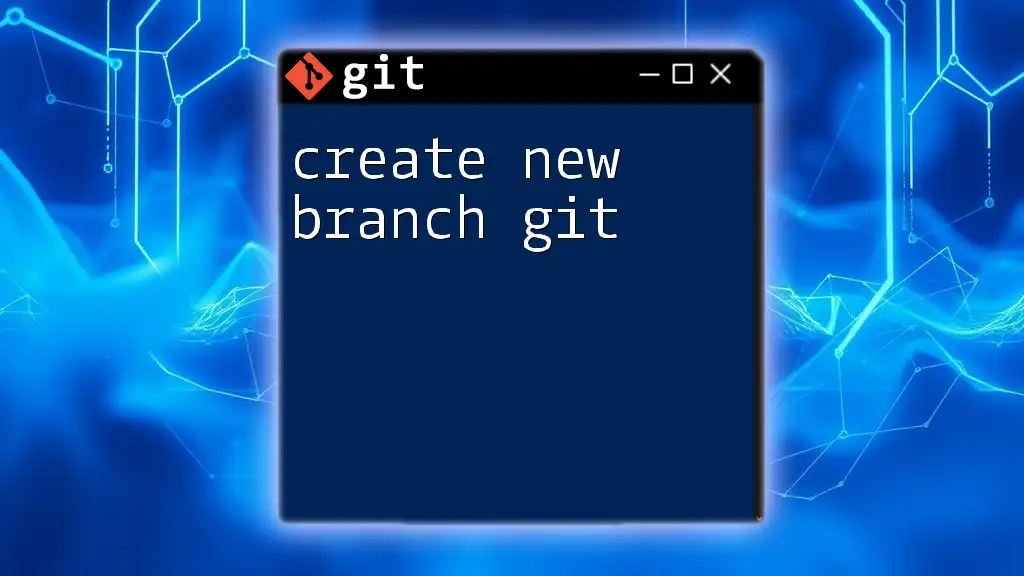
Initializing Git in Your Laravel Project
Navigating to Your Project Directory
Change your directory to the newly created Laravel project by running:
cd my-laravel-app
Initializing a Git Repository
A Git repository is where all your version history will be stored. To initialize a Git repository in your Laravel project, enter:
git init
This command creates a new `.git` directory that tracks your project files for version control.
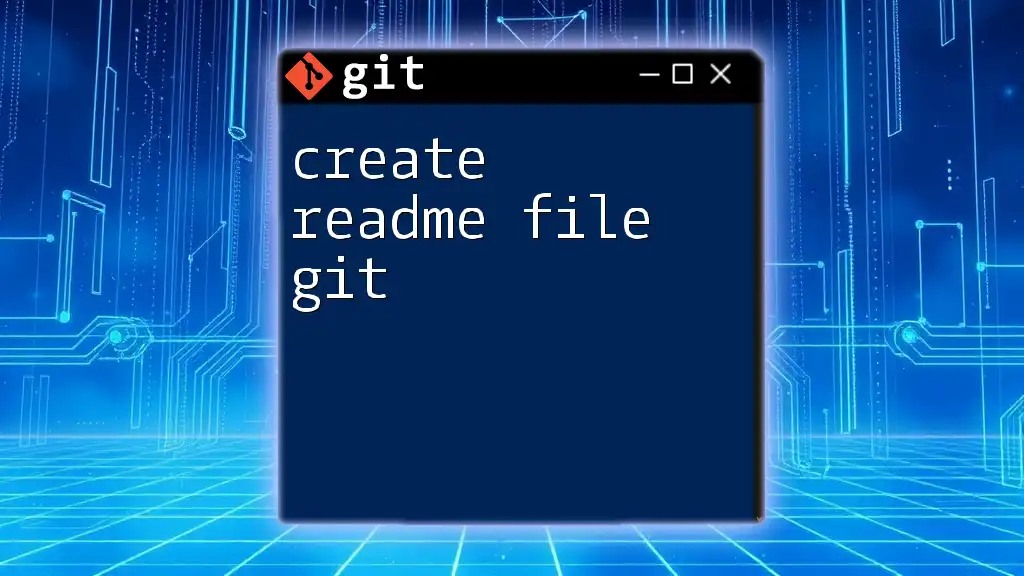
Understanding Git Structure with Laravel
Laravel Project File Structure
Understanding the Laravel file structure is crucial as it differentiates between the various components of your application. The primary directories include:
- app/: Contains the core application code.
- config/: Holds configuration files, facilitating easy modifications.
- database/: Contains your database migrations and seed files.
- resources/: Houses your views, raw assets like Sass, and JavaScript.
- routes/: Contains all route declarations for your application.
Ignoring Files with .gitignore
It's important to manage what files Git tracks to keep your repository clean. The `.gitignore` file instructs Git on which files and directories to ignore. Common files to exclude in Laravel projects include:
/vendor/
.env
node_modules/
Create a `.gitignore` file in your project's root and add these entries to prevent sensitive and unnecessary files from being pushed to your repository.
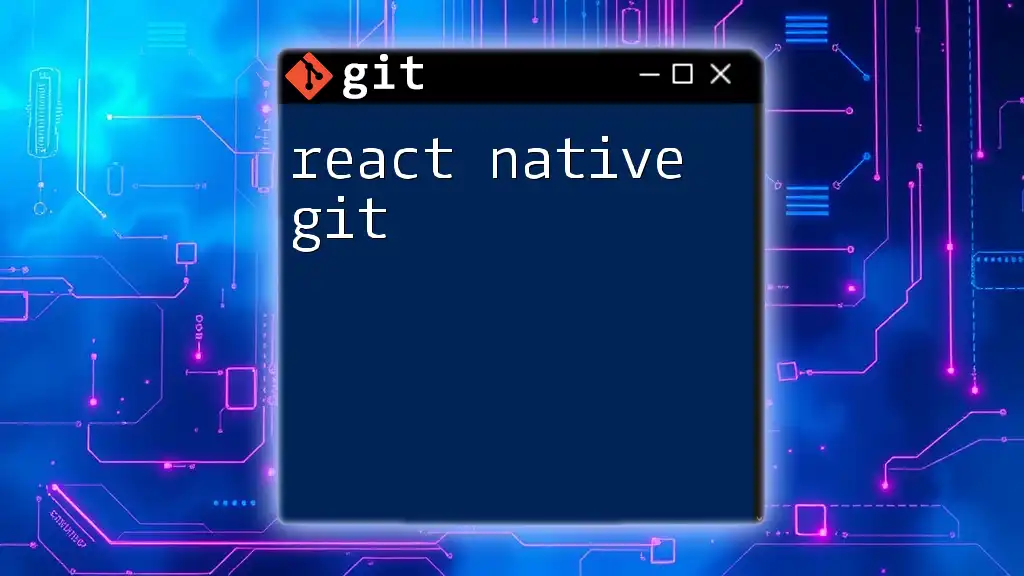
First Commit to Git
Staging Changes
Staging allows you to prepare files for a commit without including unnecessary changes. To stage all your files, use:
git add .
Committing Changes
Committing captures the state of your project at a particular point. To create a commit with a descriptive message, run:
git commit -m "Initial commit of my Laravel app"
Your message should be clear, providing context into what changes were made.
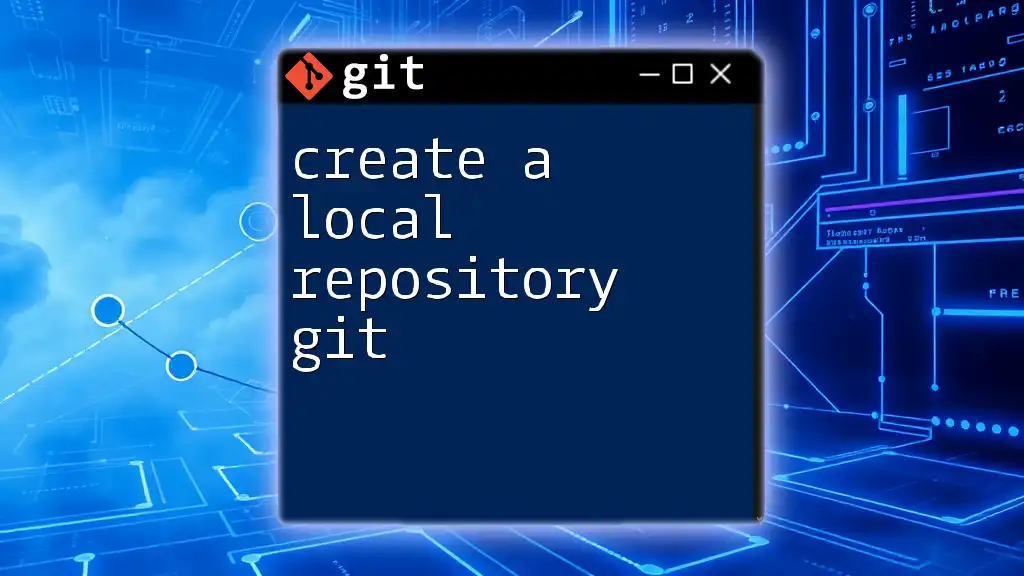
Creating a Remote Repository on GitHub
Setting Up GitHub Account
If you haven't done so already, create a free account at [GitHub](https://github.com/).
Creating a New GitHub Repository
Once logged in, click on the `New` repository button. Provide a unique repository name, ensure the "Initialize this repository with a README" option is not selected, and click `Create repository`. Note the URL provided; you'll need this to connect your local repository.
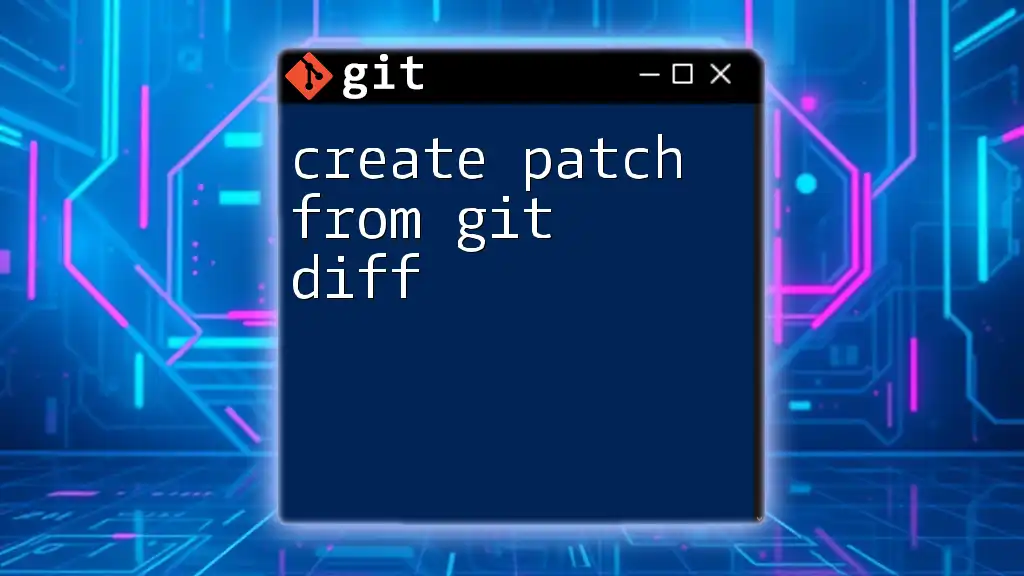
Pushing Your Laravel App to GitHub
Adding Remote Origin
Connecting your local repository to the remote GitHub repository is essential for collaboration and backup. Execute the following command, replacing the URL with your repository's URL:
git remote add origin https://github.com/username/my-laravel-app.git
Pushing Changes to GitHub
Now that your remote origin is set, push your local commits using:
git push -u origin master
The `-u` flag establishes a tracking connection for future pushes.
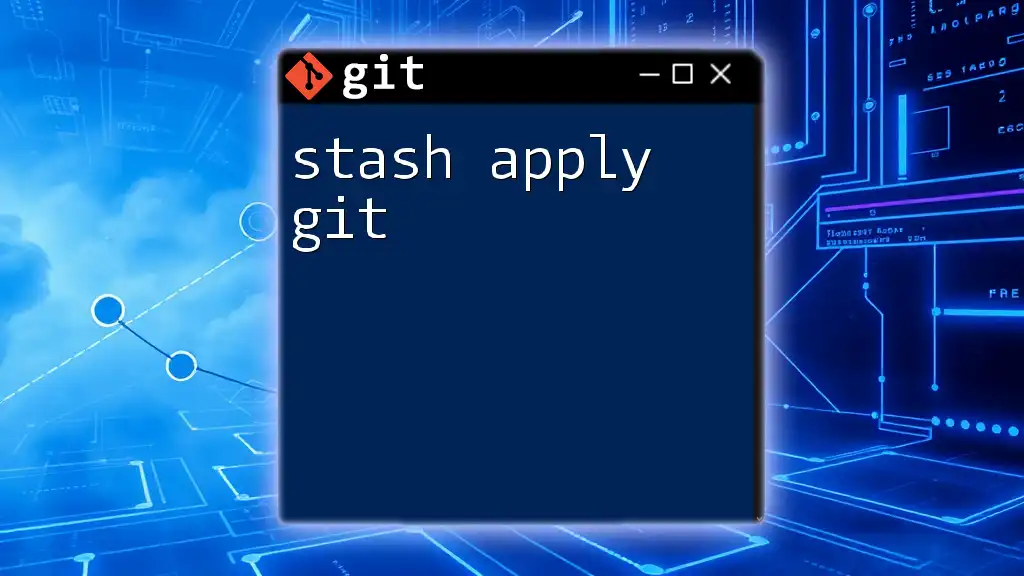
Cloning Your Laravel App
What is Cloning?
Cloning creates a copy of another repository on your machine, making it essential for collaboration. This command can be widely used when you or someone else wants to work on the same project from different locations.
How to Clone Your Laravel App
If you want to clone your newly pushed Laravel app from GitHub, you can use the following command:
git clone https://github.com/username/my-laravel-app.git
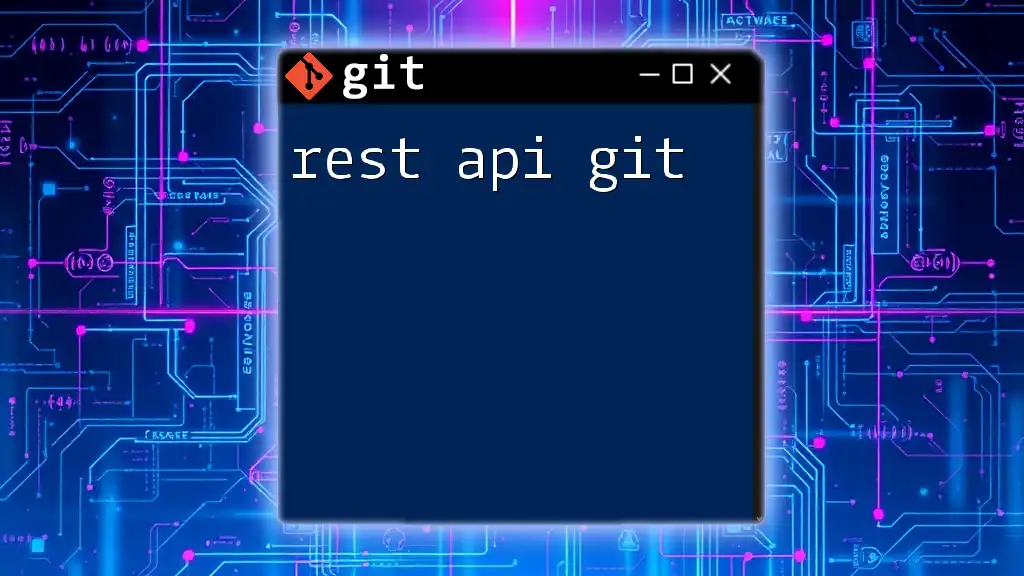
Best Practices for Version Control with Laravel and Git
Commit Often
Frequent commits prevent losing work and make it easy to identify when specific changes were made. Smaller, focused commits enhance clarity.
Writing Meaningful Commit Messages
Commit messages should articulate what changes have been made. A well-written message can be the difference between understanding the history of the project or getting lost in it.
Branching Strategies
Utilizing branches allows different features or fixes to be developed simultaneously. A popular approach is to create a branch for each feature or bug fix, which you can then merge back into the main codebase upon completion.
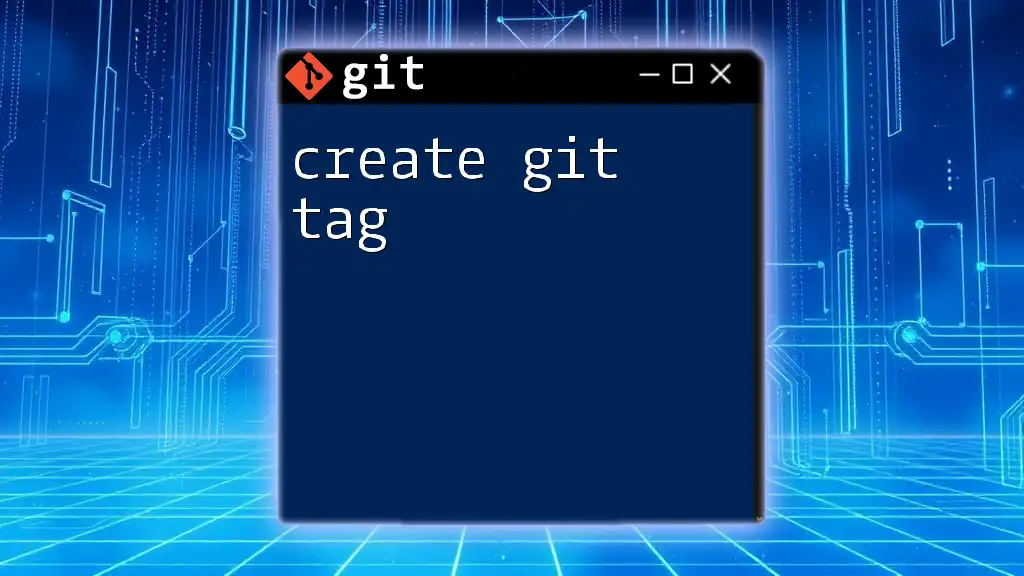
Conclusion
In this guide, we've gone through the steps to create a Laravel app to Git, from installation to committing code and pushing a repository to GitHub. Incorporating version control into your development workflow will not only enhance your productivity but also foster a collaborative working environment. Embrace Git in your Laravel projects, and you'll find yourself managing code with greater ease.
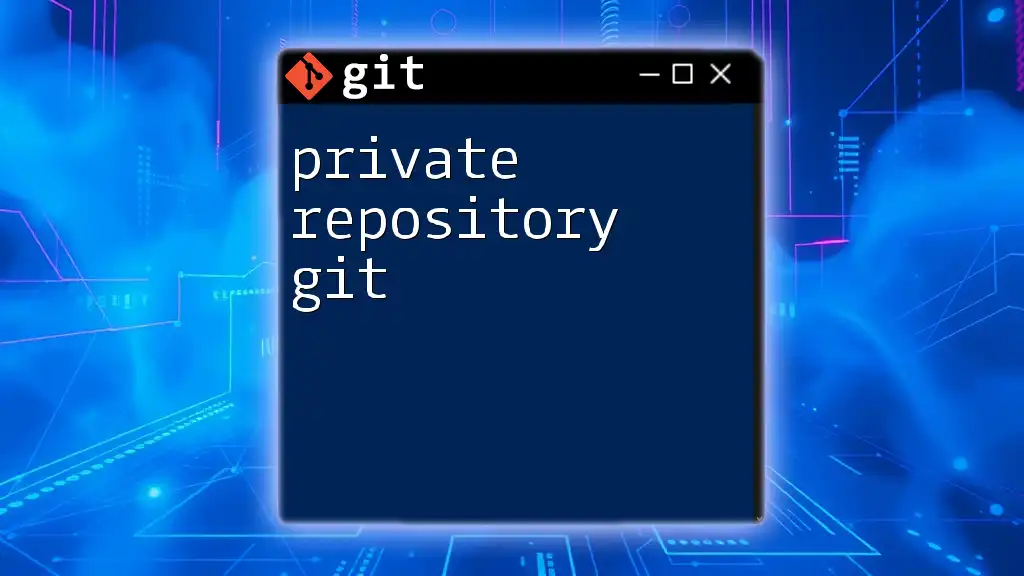
Additional Resources
Useful Links and References
- [Laravel Documentation](https://laravel.com/docs)
- [Git Documentation](https://git-scm.com/doc)
- [GitHub Guides](https://guides.github.com/)
Feel free to leverage these resources to deepen your understanding of Git and Laravel!