To efficiently clean up local branches in Git that have already been merged into your current branch, you can use the following command:
git branch --merged | grep -v "\*" | xargs -n 1 git branch -d
Understanding Local Branches
What Is a Local Branch?
A local branch in Git allows developers to work independently on features, fixes, or experiments without affecting the stable codebase in the main branch (often called `main` or `master`). Local branches exist in your local repository and can be created, modified, or deleted without impacting others until you push them to a remote repository.
Why Cleanup Local Branches?
Over time, your local repository can fill up with numerous branches, especially when working on multiple features or bug fixes. Cleaning up local branches is crucial for several reasons:
- Managing Repository Clutter: A multitude of branches can lead to confusion and difficulty in locating the active ones.
- Improving Workflow Efficiency: By removing unnecessary branches, you can streamline your workflow, allowing you to focus on current tasks.
- Preventing Confusion During Collaboration: When collaborating with team members, a clean branch structure avoids miscommunication and mistakes.
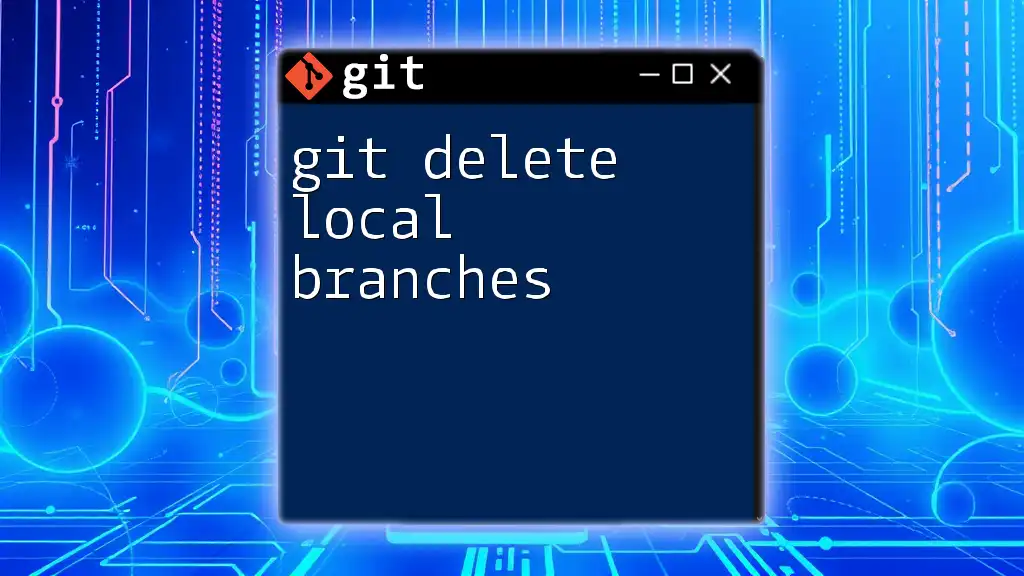
Identifying Local Branches
Listing Local Branches
To start managing your branches, you'll first need to see what you have. The command to list all local branches is simple:
git branch
This command displays all local branches in your repository. The current branch is indicated with an asterisk, making it easy to identify where you are working.
Identifying Merged and Unmerged Branches
It’s essential to know which branches have been merged into your current branch and which ones have not. Use the following commands for this purpose:
To list merged branches:
git branch --merged
To list unmerged branches:
git branch --no-merged
With these commands, you'll gain clarity about which branches can safely be deleted and which ones require further work.
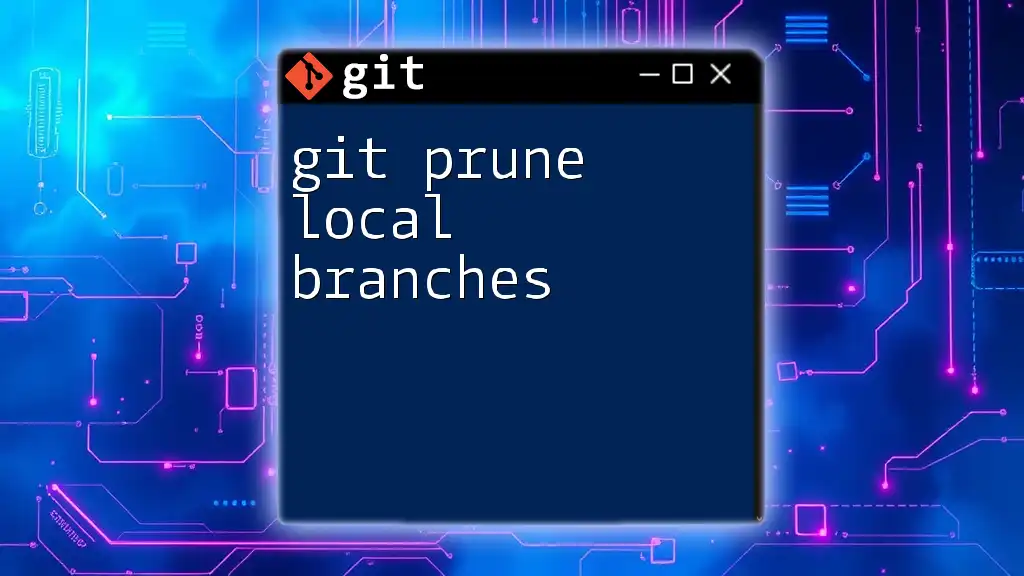
Cleaning Up Local Branches
Deleting Local Branches
Once you've identified branches no longer needed, the next step is deletion.
Safe Deletion
To safely delete a local branch that has already been merged into your current branch, use:
git branch -d <branch_name>
For example:
git branch -d feature-branch
This command removes the specified branch if it’s been merged, protecting your codebase from accidental loss.
Forced Deletion
In situations where a branch has not been merged but you still want to delete it, you can use the force option:
git branch -D <branch_name>
Example:
git branch -D feature-branch
Note: Be cautious with this command as it removes a branch regardless of its merge status, potentially resulting in lost work.
Deleting Multiple Local Branches
If you find yourself with multiple branches to delete, consider deleting them all at once. You can use a command that filters and deletes branches with a certain pattern:
git branch | grep <pattern> | xargs git branch -d
For instance, if you want to delete all branches starting with `feature/`, you could run:
git branch | grep 'feature/' | xargs git branch -d
This approach saves time and helps keep your repository tidy.
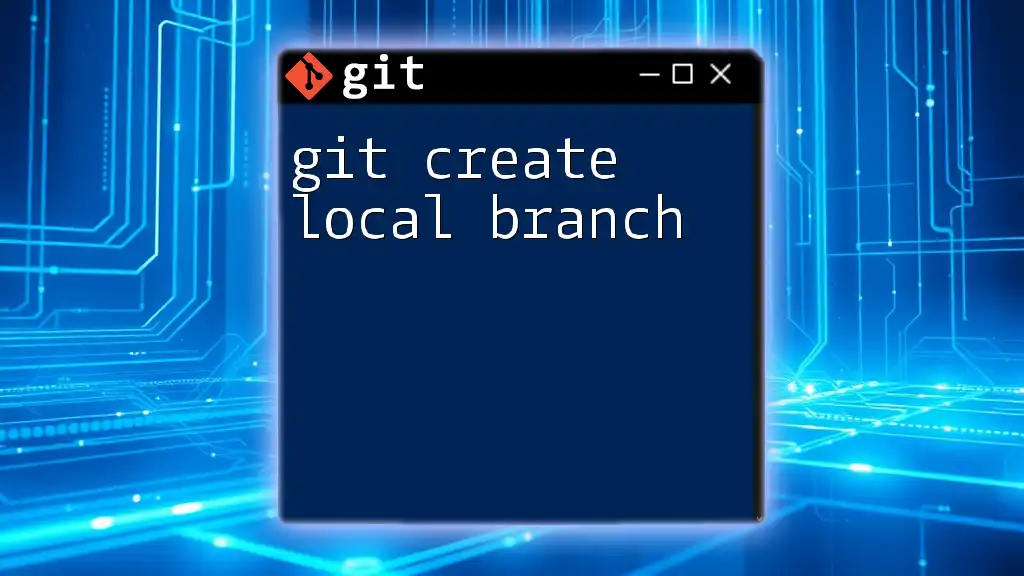
Tips for Effective Git Branch Management
Regular Cleanup Schedule
Consistency is key when managing branches. Establishing a regular cleanup schedule—be it weekly or bi-weekly—ensures that your repository remains organized and functional.
Establishing Naming Conventions
Using clear and descriptive branch names can significantly ease the management process. Examples include:
- `feature/login-page`
- `bugfix/cart-bug`
With meaningful names, both you and your collaborators will find it easier to understand the purpose of each branch at a glance.
Tagging Important Branches
Before you delete a branch that contains vital changes, consider using tags to mark significant development milestones. Tags serve as a snapshot of your project at a certain point in time, allowing you to reference them later if needed.
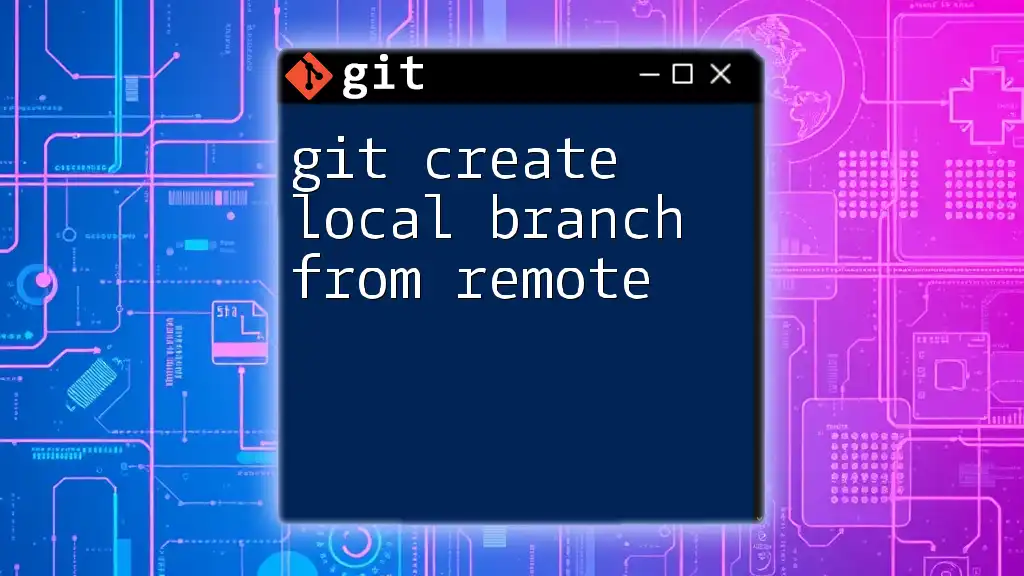
Automating Branch Cleanup
Using Git Aliases
One of the best ways to streamline your workflow is by creating Git aliases for commonly used commands. For example, if you frequently remove merged branches, you might consider adding an alias:
git config --global alias.cleanup '!git branch --merged | grep -v "main" | xargs git branch -d'
With this alias, you can simply run `git cleanup` to quickly remove merged branches, excluding your main branch, significantly reducing the repetitive tasks involved in branch management.
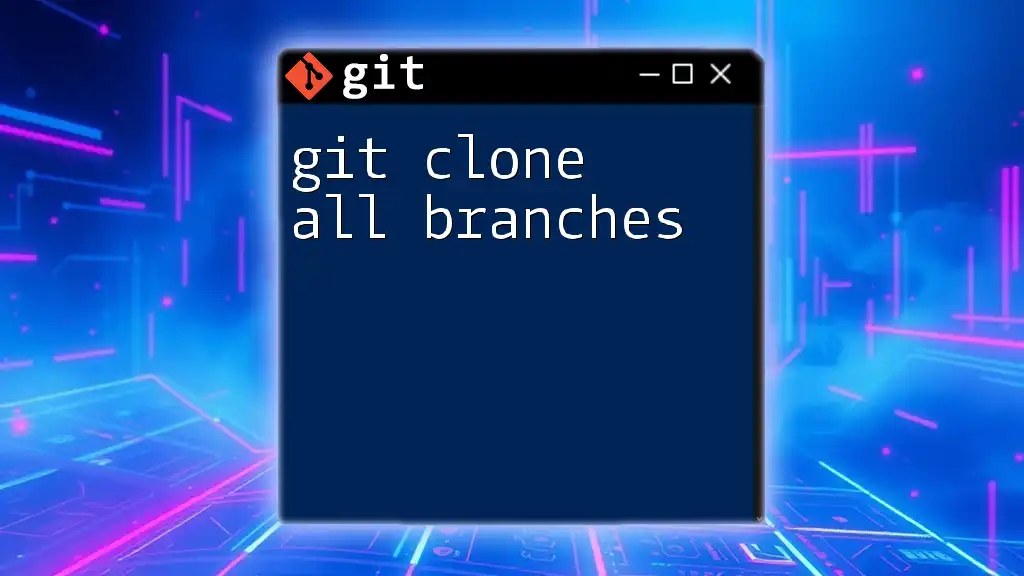
Conclusion
Cleaning up local branches is a critical practice for maintaining an efficient and organized Git repository. It minimizes confusion, enhances collaboration, and contributes significantly to workflow efficiency. Regularly assess and delete unnecessary branches to avoid clutter, and consider implementing strategies like naming conventions and tagging for better management.
By incorporating these practices into your routine, you can ensure that your local repository remains streamlined and functional, paving the way for effective collaboration and development progress.