To list all local branches in a Git repository, use the following command:
git branch
Understanding Git Branches
What are Git Branches?
In Git, a branch is essentially a lightweight movable pointer to a commit. Branching is a core concept in Git that allows developers to diverge from the main line of development, enabling parallel development and collaborative workflows. Each branch encapsulates changes and features, enabling developers to work in isolation until they are ready to merge their changes back into the main branch.
Why Use Local Branches?
Local branches provide immense flexibility when working on new features or bug fixes. They allow developers to isolate their work from the main codebase, facilitating experimentation and ensuring that the main project remains stable. By adopting a branching strategy, teams can collaborate more efficiently, making it easy for members to work on different aspects of a project simultaneously without conflicts.
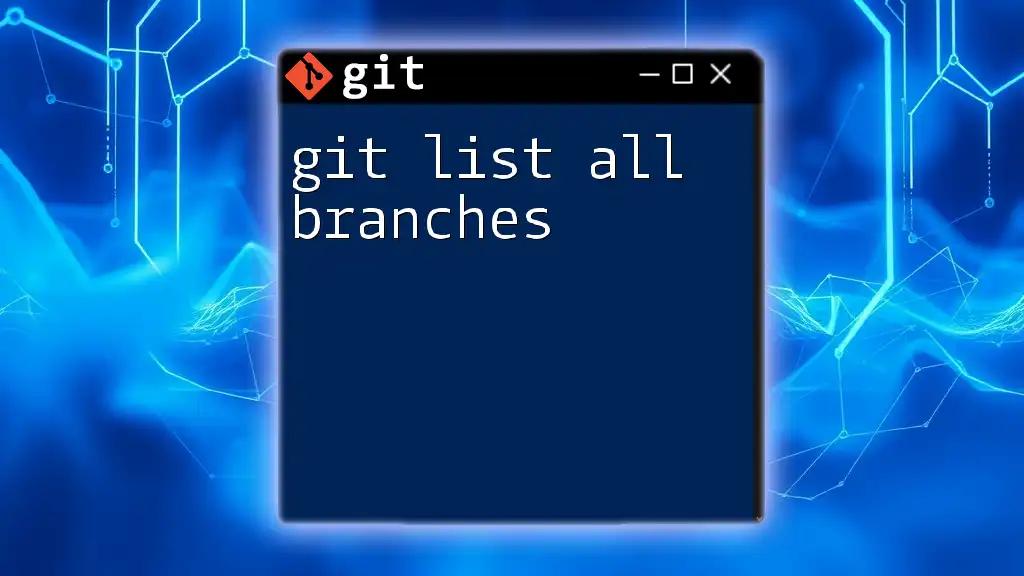
Listing Local Git Branches
The Git Command to List Local Branches
To list all local branches in your Git repository, you simply need to use the `git branch` command. This command displays all branches that exist in your local environment.
Example Usage
To view your local branches, use the following command:
git branch
Executing this command will output a list of local branches, highlighting the branch you are currently on with an asterisk (*).
Understanding the Output
The output of the `git branch` command will display all your local branches. The asterisk (*) indicates the active branch, denoting where you currently are in your development. For example:
* main
feature/login
feature/signup
In this example, you are currently on the `main` branch, with two additional local branches: `feature/login` and `feature/signup`.
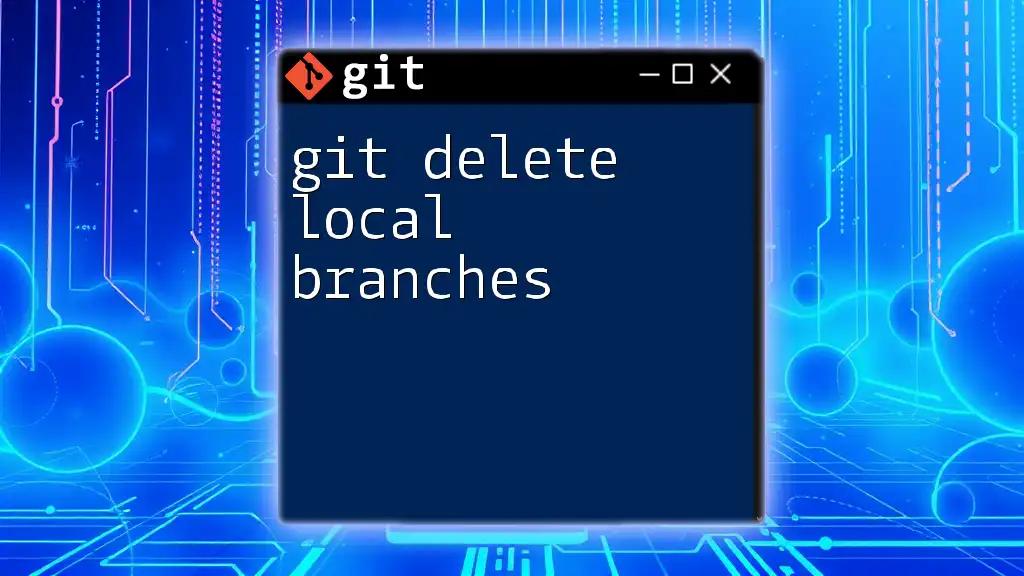
Additional Commands Related to Local Branches
Listing All Branches (Local and Remote)
If you want to see both your local and remote branches, you can use the command:
git branch -a
This command will display all branches, indicating remote branches with the prefix `remotes/origin/`. This is useful for getting a comprehensive view of all branches associated with your repository.
Filtering Branches
Listing Only Merged or Unmerged Branches
You can filter branches based on their merging status.
To list branches that have been merged into your currently active branch, use:
git branch --merged
Conversely, to list branches that have not been merged, use:
git branch --no-merged
These commands help you manage your branches effectively, allowing you to clean up merged branches or focus on unmerged work.
Listing Branches with Additional Information
Using `git show-branch`
For a more detailed look at branches and their commits, the `git show-branch` command can be particularly insightful:
git show-branch
This will present an overview of the branches and commits associated with each branch in a more digestible format.
Customizing Output
Formatting with `--format`
You can customize the output of the `git branch` command by using the `--format` option. This can be particularly useful if you want to include specific information about each branch.
For instance:
git branch --format='%(refname:short) %(committerdate:relative)'
This command will display each branch alongside the last commit date in a relative format (e.g., "2 weeks ago"), providing context for each branch's activity.
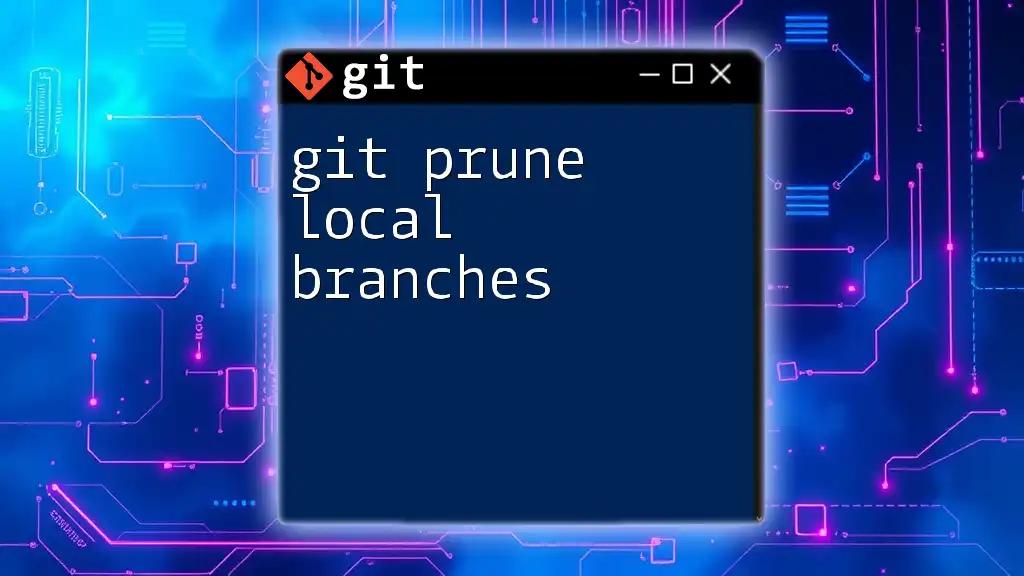
Managing Local Branches
Creating a New Local Branch
To create a new local branch, use the `git branch` command followed by your new branch name:
git branch feature/new-feature
This command will create a new branch called `feature/new-feature`, allowing you to work on this feature in isolation.
Deleting a Local Branch
When a local branch is no longer needed, you can delete it using:
git branch -d <branch_name>
For example, to delete a branch named `feature/old-feature`, you would run:
git branch -d feature/old-feature
Note that Git will not allow you to delete a branch that has unmerged changes unless you use the `-D` option to force deletion.
Renaming a Local Branch
If you need to rename an existing branch, you can do so with the following command:
git branch -m <old_name> <new_name>
For instance, to change `feature/old-feature` to `feature/renamed-feature`, you would execute:
git branch -m feature/old-feature feature/renamed-feature
This command keeps your commit history intact while allowing you to use a more appropriate branch name.
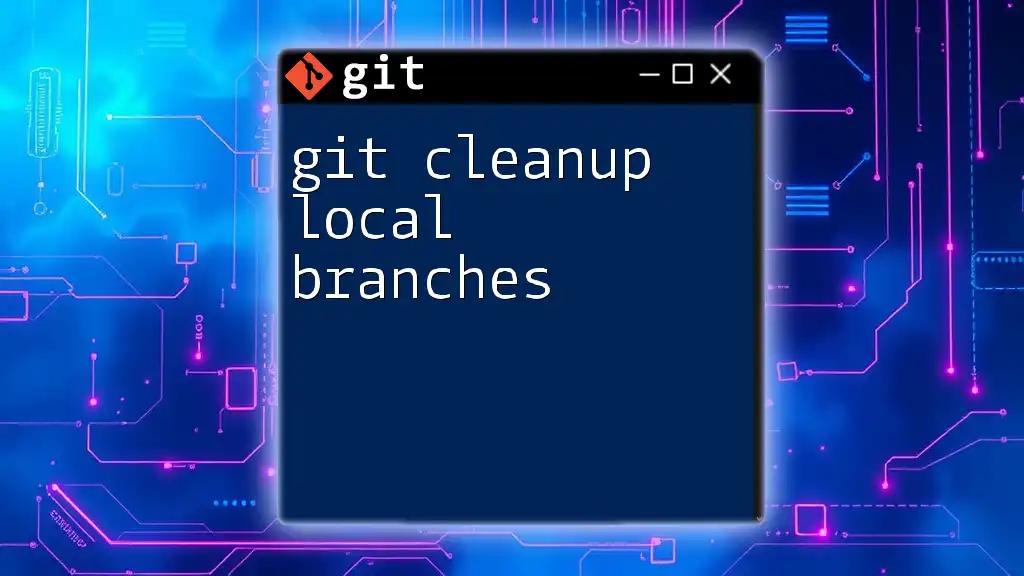
Best Practices for Managing Local Branches
Naming Conventions
Adopting a consistent naming convention for branches can significantly improve collaboration. Common practices include prefixing branch names with the type of work (e.g., `feature/`, `bugfix/`, or `hotfix/`) followed by a concise description of the task. For example, `feature/user-authentication` or `bugfix/navbar-crash` convey clear purposes and origins.
Regular Housekeeping
It’s crucial to routinely clean up your branches to avoid clutter and confusion. Regularly check for branches that have been merged and delete them using the `-d` command. To identify stale branches, you can utilize:
git branch --merged
Performing this maintenance ensures that your project stays organized and navigable.
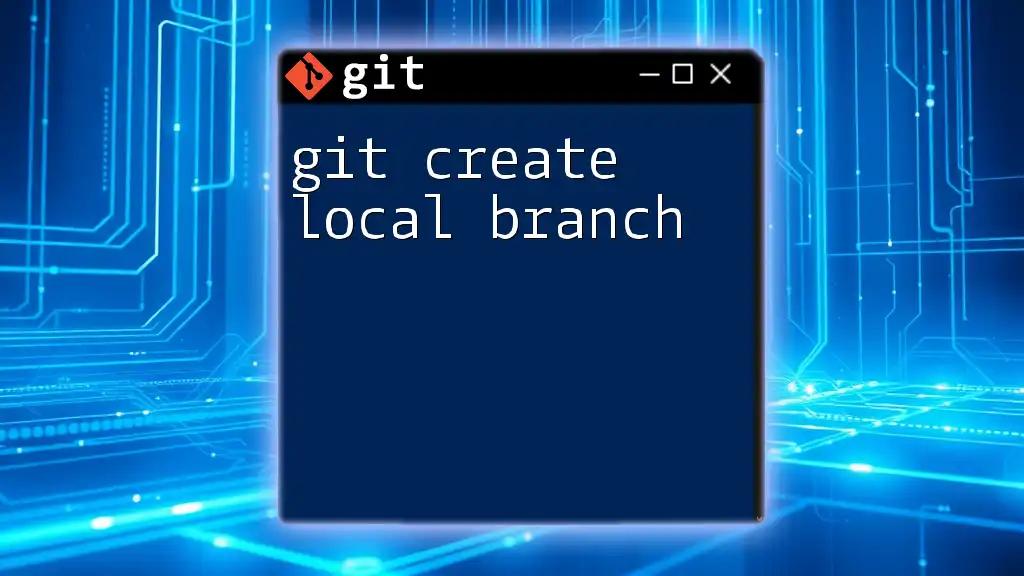
Conclusion
Recap of Key Points
In this article, we covered the essential commands associated with the git list of local branches. We explored how to create, delete, and rename branches while discussing commands to filter, format, and manage local and remote branches effectively.
Encouragement to Practice
As with any tool, practice makes perfect. I encourage you to experiment with the commands discussed, explore their options, and integrate them into your workflow. Mastery of local branch management will significantly enhance your productivity in Git.
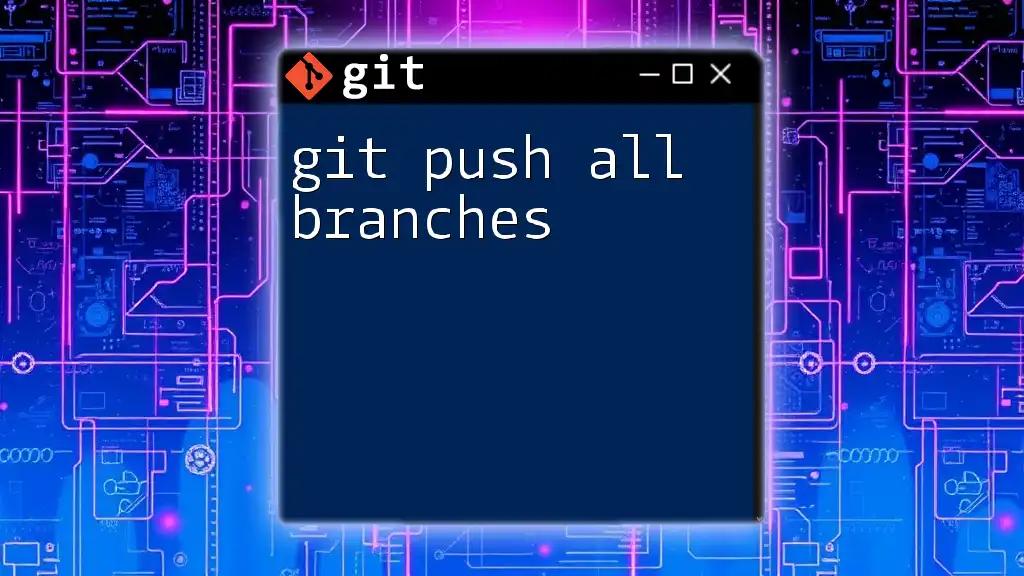
References
Recommended Tools and Resources
For further learning, explore the official Git documentation and community resources that dive deeper into advanced Git topics. This knowledge will serve as a solid foundation in your journey to becoming a Git expert.