To retrieve a list of all branches in a Git repository, including both local and remote branches, you can use the following command:
git branch -a
Understanding Git Branches
What is a Git Branch?
A Git branch is a fundamental concept in version control that allows developers to diverge from the main line of development and isolate changes. It acts like a temporary workspace where you can work on different features or fixes without affecting the main project. Once the changes are ready, they can be merged back into the main branch. This functionality is crucial for collaborative work as it allows multiple people to work on different aspects of a project simultaneously without interfering with one another.
Types of Branches in Git
Local Branches
Local branches are those that exist on your local machine. You can create, modify, and delete these branches without impacting the remote repository. They are essential for your individual development workflow.
Remote Branches
Remote branches, on the other hand, exist in the remote repository (like GitHub or GitLab). These branches are tracked locally but require a different set of commands to manipulate. They represent branches that have been pushed to the remote repository.
Local vs Remote Branches
- Local branches are meant for active development.
- Remote branches serve as pointers to the state of branches in a remote repository.
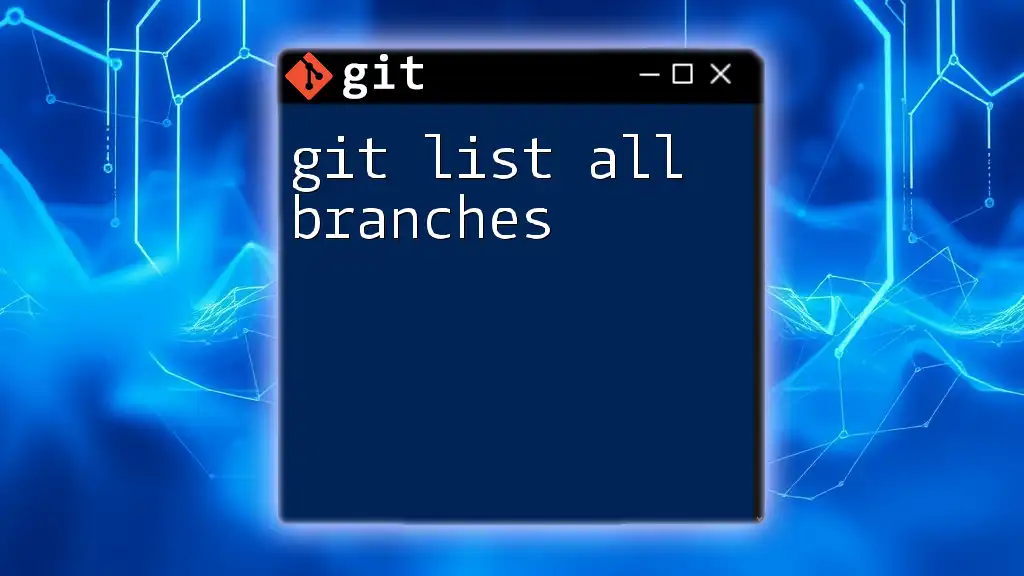
How to View All Branches in Git
Viewing Local Branches
To see all the branches that exist locally in your Git repository, you can use the command:
git branch
When you execute this command, it will list all the local branches in your repository. The currently active branch will be indicated by an asterisk (*) next to its name. This quick command is vital for a developer to keep track of which branch they are currently working on.
Viewing Remote Branches
If you need to see the branches that exist in the remote repository, you can use the following command:
git branch -r
This command displays all the remote branches without cluttering your output with local information. Knowing the names and states of remote branches can help you understand what your collaborators are working on and what gets pushed to the main repository.
Viewing All Branches (Local and Remote)
To view both local and remote branches at the same time, use:
git branch -a
This command is particularly useful when you need a comprehensive overview of both your work and your team's contributions. The output will distinguish between the local branches (listed normally) and remote branches (which are prefixed by `remotes/` and usually prints the remote's name).
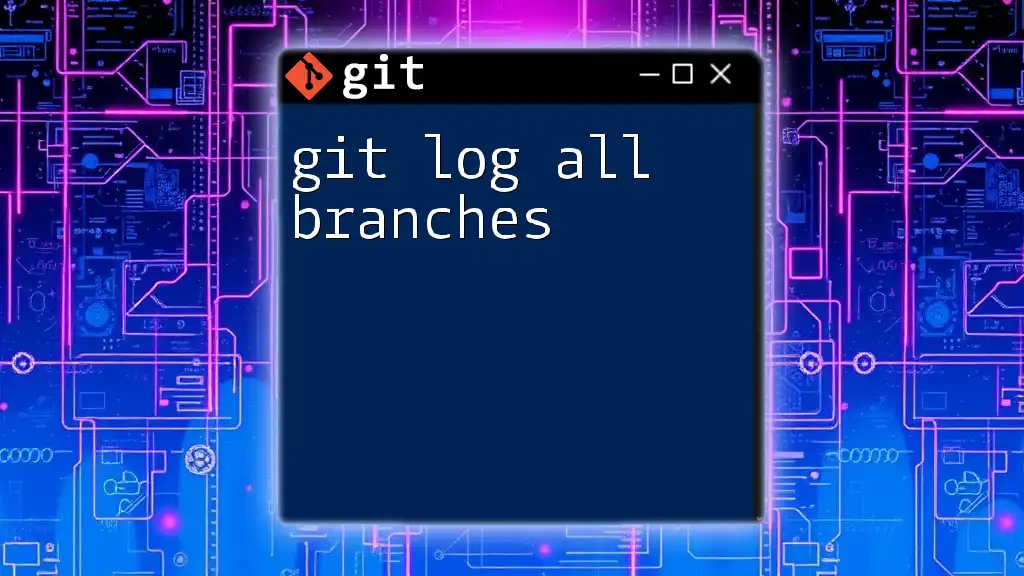
Understanding Branch Information
Interpreting the Branch List
When interpreting the output from `git branch -a`, it’s essential to understand that the branch names often follow conventions. For example, naming a branch after the feature it implements or the bug it fixes helps maintain organization within your repository. Additionally, remote-tracking branches will usually precede the branch name with the remote’s name, making it clear where these branches exist.
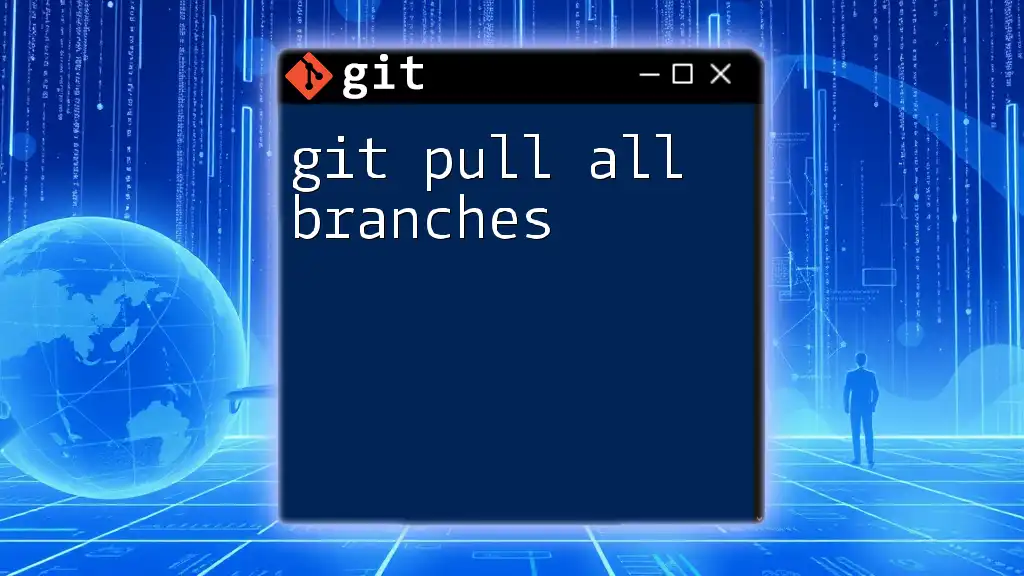
Practical Examples
Example: Viewing Branches in a Sample Repository
Let’s create a mock Git repository to demonstrate how to manage branches effectively. Start by initializing a new Git repository:
git init
Next, create a few branches:
git checkout -b feature-1
git checkout -b feature-2
git checkout main
git push origin feature-1
Now that you have your branches set up, using:
git branch -a
will display all your active branches, allowing you to easily verify that `feature-1` and `feature-2` have been created and their status.
Example: Dealing with Detached HEAD State
In some cases, you might find yourself in a detached HEAD state. This generally occurs when you check out a specific commit rather than a branch. To view branches while in this state, simply run the same branch commands. However, remember that any commits made in this state will not be attached to a branch unless you create a new branch from that point:
git checkout -b new-branch-name
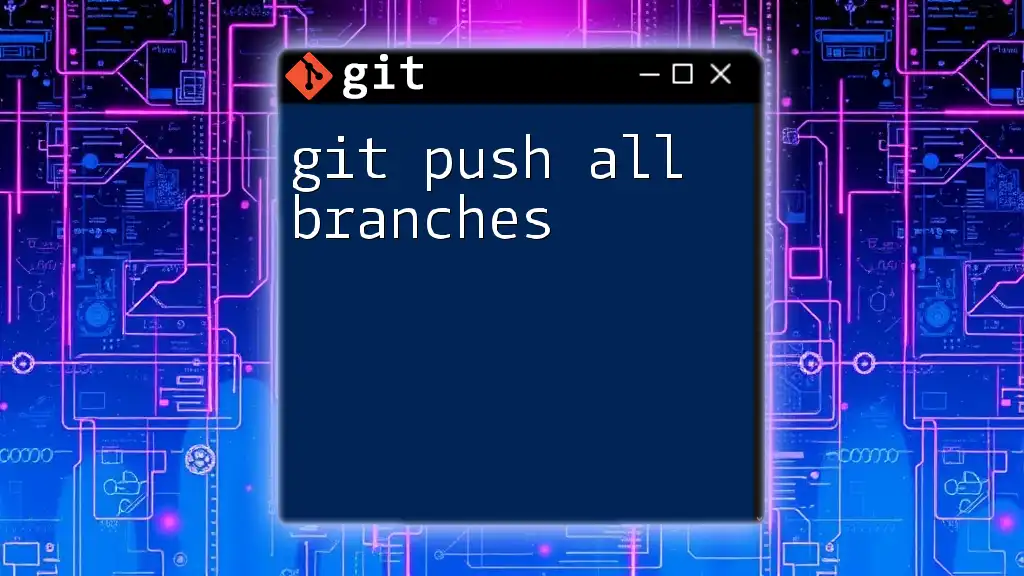
Additional Branch Management Commands
Deleting a Branch
If you want to clean up your repository by removing branches that are no longer needed, you can delete branches locally or remotely.
To delete a local branch, use:
git branch -d branch_name
For a remote branch, you can eliminate it using:
git push origin --delete branch_name
These commands help you maintain a tidy workflow by ensuring that only relevant branches are present.
Renaming a Branch
If you ever need to rename a local branch for clarity, simply run:
git branch -m old_branch_name new_branch_name
Renaming is straightforward and can help keep your branch names meaningful as the project evolves.
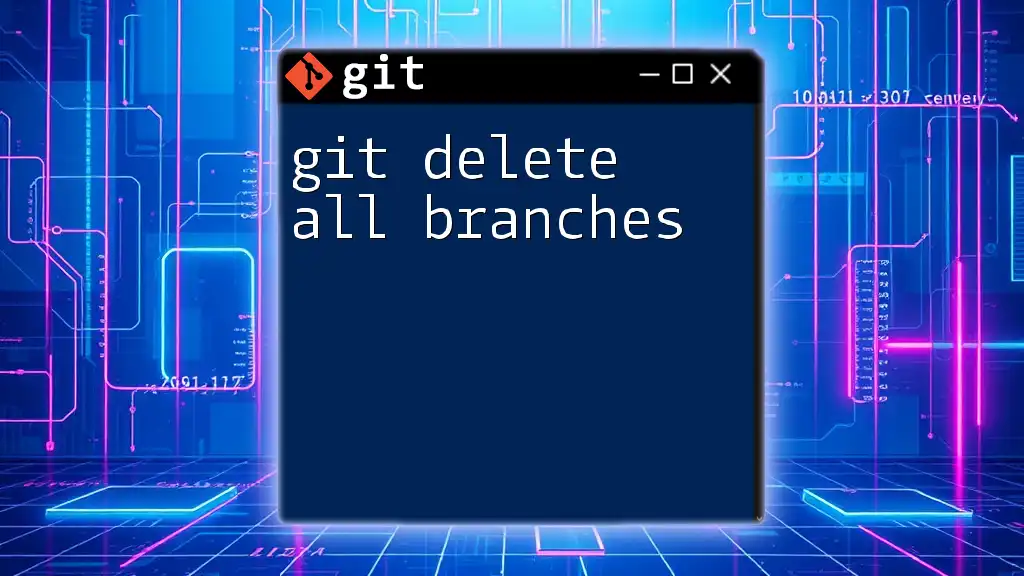
Conclusion
Understanding how to git get all branches is a vital skill for any developer working with Git. Whether for collaborating with a team or managing a personal project, the ability to view, create, and manipulate branches enhances productivity and organization. Practice the commands discussed in this guide to strengthen your branching prowess, and don’t hesitate to explore more advanced branching techniques such as rebasing and merging to optimize your Git workflow.