To edit the last commit message in Git, use the following command, which will open your default text editor for you to modify the message:
git commit --amend -m "New commit message"
Understanding Git Commit Messages
A commit message is a crucial element in Git that describes the changes made in a specific version of your code. It's not just a formality; these messages serve as a historical record of your project, enabling collaborators (and future you) to understand the rationale behind code changes. Writing effective commit messages can simplify collaboration and code reviews substantially.
What Makes a Good Commit Message?
An effective commit message answers three important questions:
- What changes were made?
- Why were those changes made?
- How do those changes impact the project?
Examples:
- Effective: "Fix bug in user login handling for edge cases"
- Ineffective: "Updates"
The first message clearly outlines what was done, while the second lacks any meaningful information.
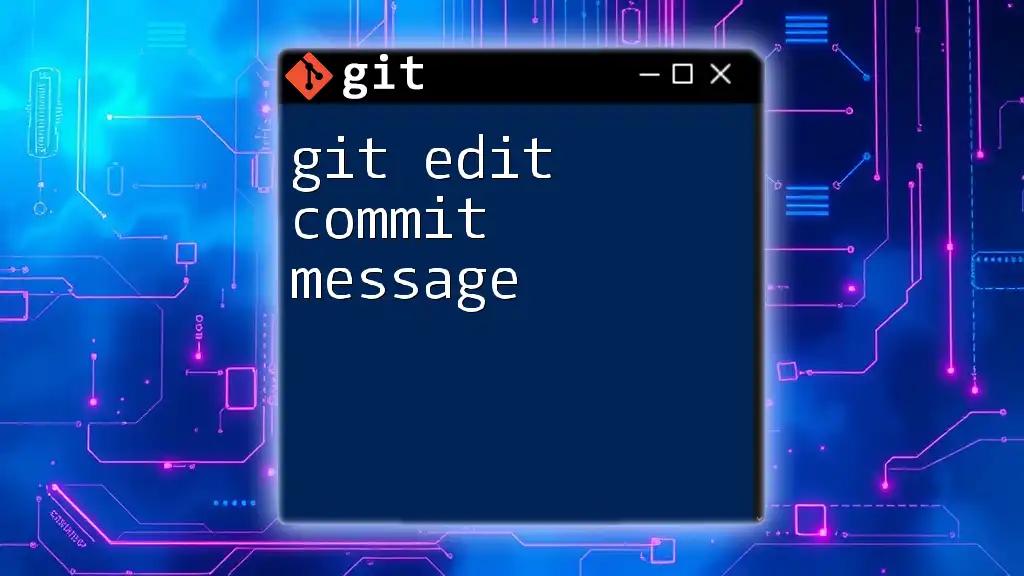
When to Edit the Last Commit Message
Sometimes, you may need to edit the last commit message to correct typos or provide clarity on changes. Here are some common scenarios that might prompt you to git edit last commit message:
- You realize you've made a typo in the existing message.
- The original message was vague and needs clarification.
- You forgot to include important context about the commit.
However, it's vital to consider how late is too late; if the commit has already been pushed to a shared repository, modifying the commit could create inconsistencies for others.
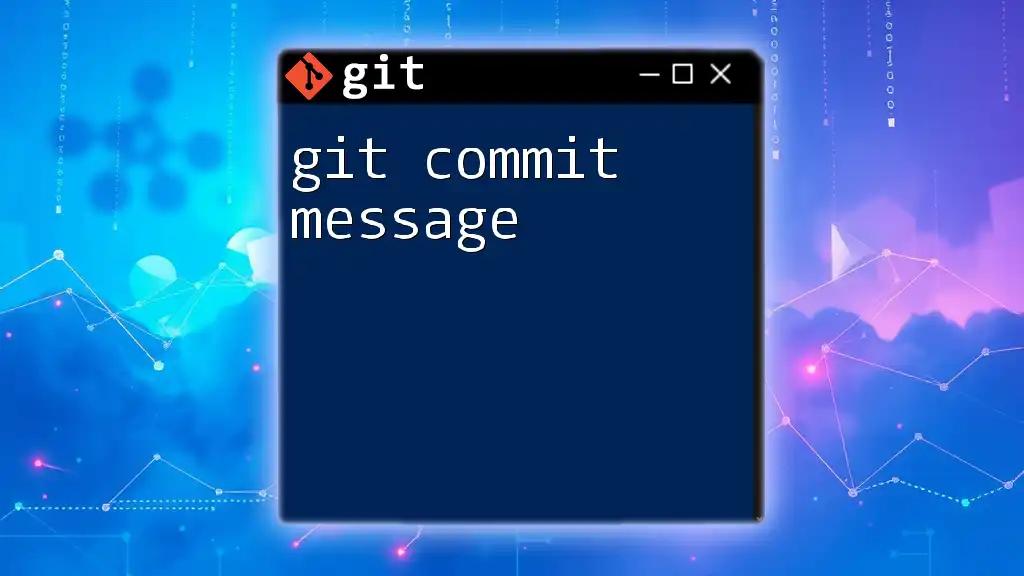
Editing the Last Commit Message Directly
Using the `git commit --amend` Command
One of the simplest ways to edit the last commit message is by using the `git commit --amend` command. This command allows you to modify the most recent commit.
Here is how to use it:
- Make sure you've saved any changes in your working directory.
- Execute the following command:
git commit --amend -m "Updated commit message"
The above command overrides the last commit message with your new input. Important: This action rewrites your Git history, which can lead to complications if this commit has already been shared with others.
Using Interactive Rebase
If you need to edit earlier commits, or if you want to review your commit history more comprehensively, using interactive rebase is the way to go.
What is Interactive Rebase? Interactive rebase allows you to rewrite commit history in a more sophisticated manner. You can reorder commits, squash them, or edit commit messages.
How to Use Interactive Rebase:
- Start the interactive rebase by executing:
git rebase -i HEAD~1
The `HEAD~1` tells Git to start rebase from the last commit. If you want to edit multiple commits, replace `1` with the number of commits you wish to modify.
-
An editor window will open listing your recent commits. You can change the word `pick` to `reword` next to the commit you wish to edit.
-
Save and exit the editor. Git will then prompt you to edit that particular commit message.
-
After editing, save again and exit.
This method is powerful, but similar to `amend`, you should be cautious if these commits have already been pushed to a shared repository.
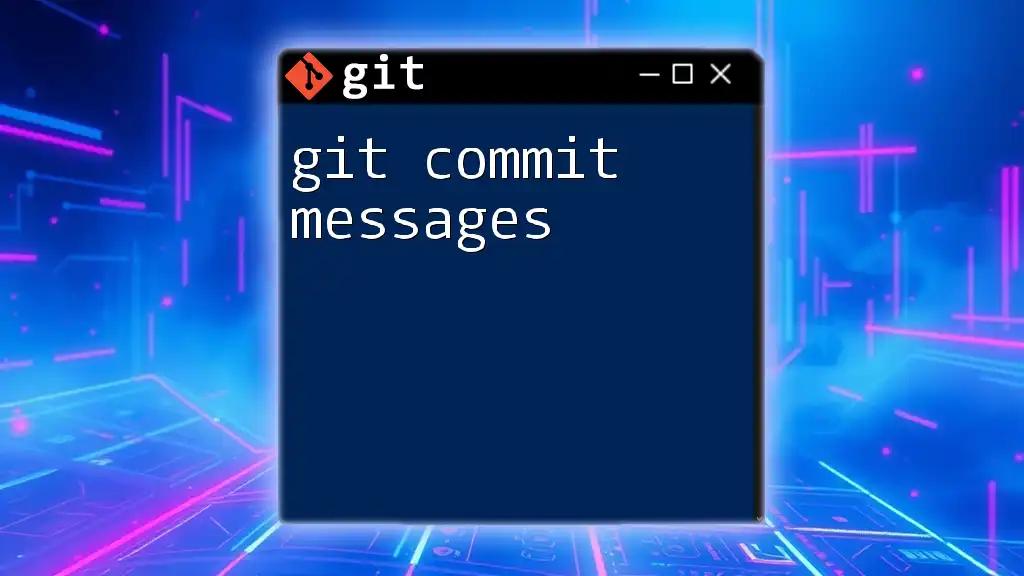
Considerations and Best Practices
What Happens to the Commit History?
When you edit a commit, you're essentially creating a new commit with a different hash. This means that any references to the old commit are now outdated, and anyone who has pulled that commit will have conflicts. Always inform your team before rewriting commit history.
Risks of Amending Shared Commits
If you amend a commit that has already been pushed to a shared repository, you may create what is known as a "divergent history." This can lead to confusion and complications when collaborators attempt to pull or push their changes. To mitigate this risk, it is best to only amend local commits that have not yet been shared.
Tips for Crafting Good Commit Messages After Editing
- Aim for clarity and conciseness.
- Start with a short summary (ideally under 50 characters), followed by a blank line and then a more detailed description if necessary.
- Use the imperative mood (e.g., "Fix bug" rather than "Fixed bug").
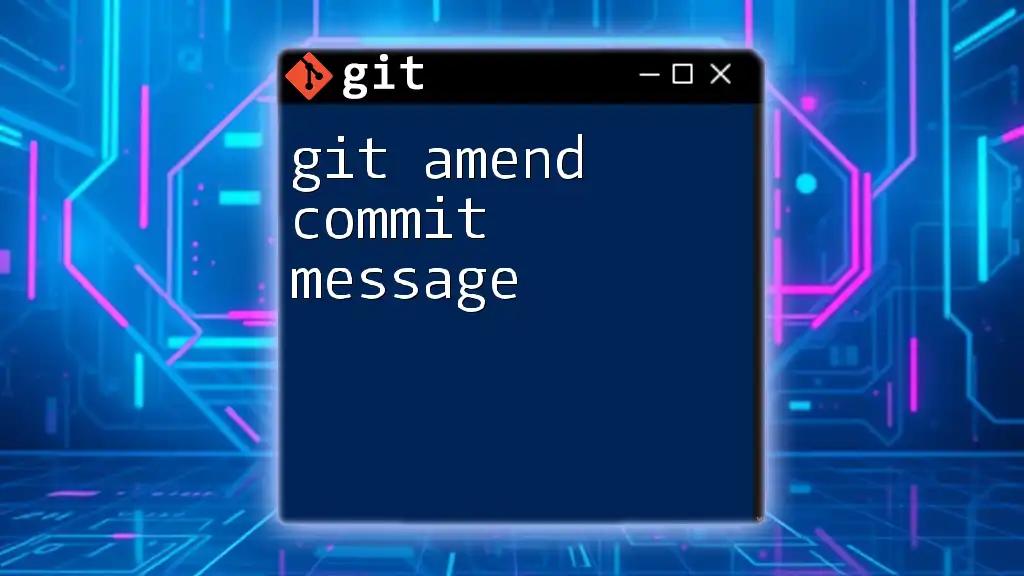
Common Mistakes and How to Avoid Them
- Forgetting to push after amending: Don’t forget that you'll need to force-push (`git push --force`) if you amend a shared commit.
- Amending a merge commit: Editing merge commits can lead to confusion, as it can impact the entire commit history.
- Editing the wrong commit: Take care to check your commit history before amending, especially when using interactive rebase or the `--amend` flag.
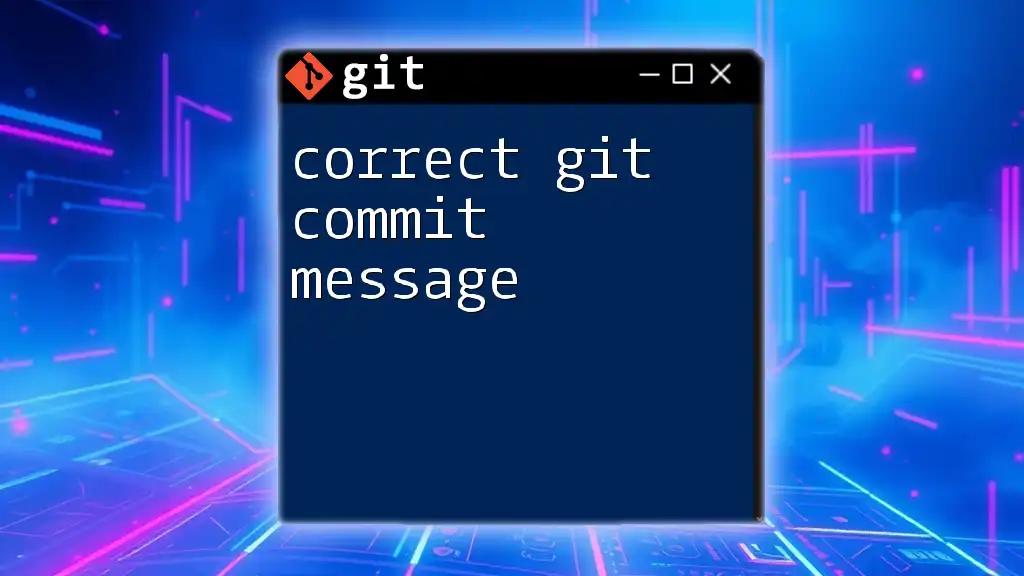
Troubleshooting
If something goes wrong after editing a commit message, you can revert back using the following command:
git reflog
This command shows you a log of your recent commands and actions. From there, you can identify the previous commit hash and reset to that commit using:
git reset --hard <commit_hash>
This allows you to return to your previous state if needed.
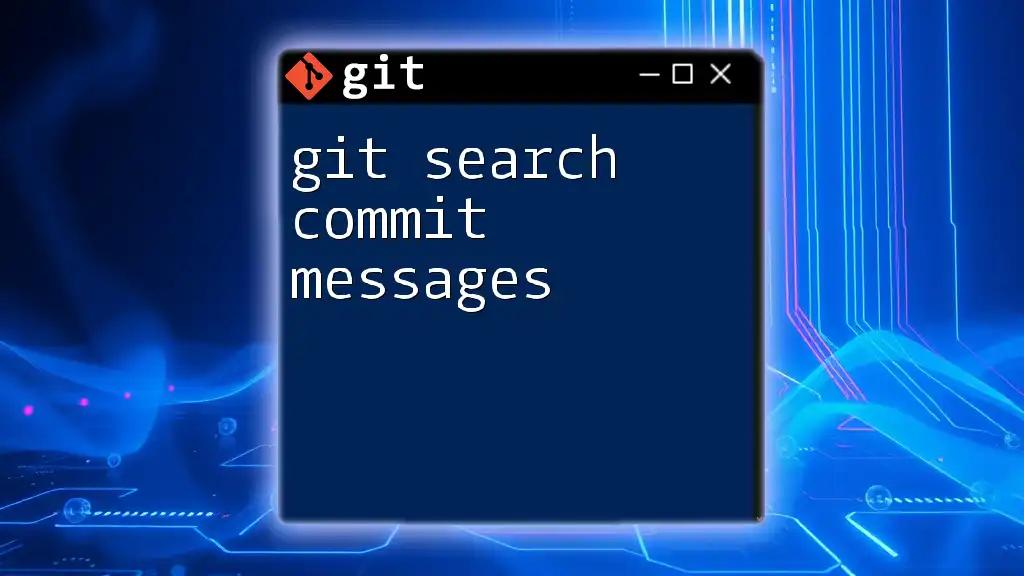
Conclusion
Understanding how to git edit last commit message is an essential skill for effective version control. Whether using `--amend` for quick fixes or interactive rebase for more in-depth edits, following best practices can help maintain the integrity of your commit history. The power of Git lies not only in code management but also in clear communication through commit messages. By taking the time to edit your messages thoughtfully, you enhance both collaboration and personal accountability in your projects.
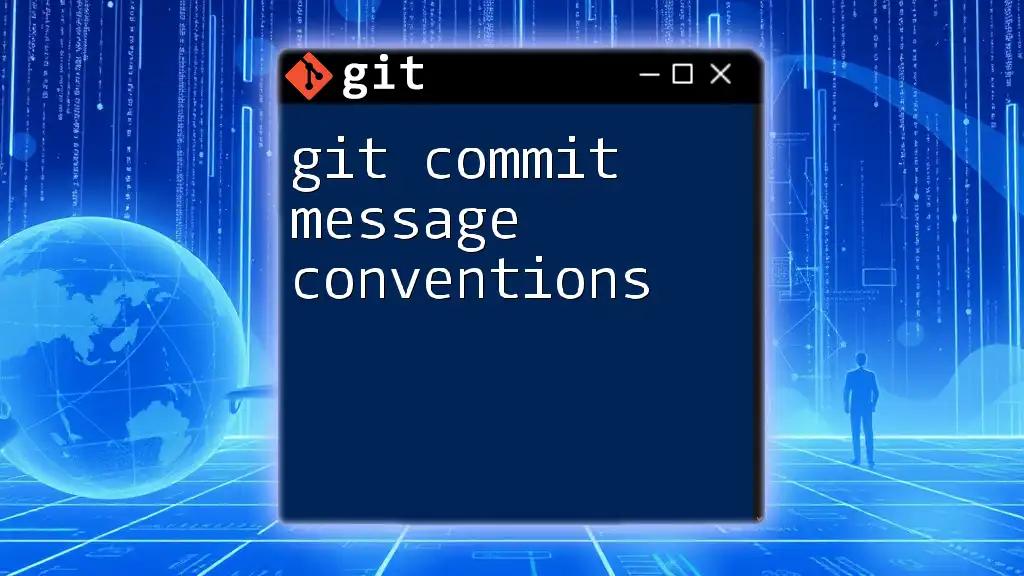
Additional Resources
For those interested in learning more about Git, consider exploring the [official Git documentation](https://git-scm.com/docs) or various online tutorials that can further solidify your understanding of this powerful tool.