The Git REST API allows developers to interact with Git repositories over HTTP, enabling operations like fetching, pushing, and modifying repository data programmatically.
curl -H "Authorization: token YOUR_ACCESS_TOKEN" https://api.github.com/repos/OWNER/REPO/git/refs/heads
What is the Git REST API?
The Git REST API allows developers to interact with Git repositories through a set of RESTful web services. It provides a programmatic way to perform common Git operations without needing to use the command line interface (CLI). REST (Representational State Transfer) is an architectural style that uses a simple set of stateless operations to interact with resources, which in this case are the various entities in a Git repository.
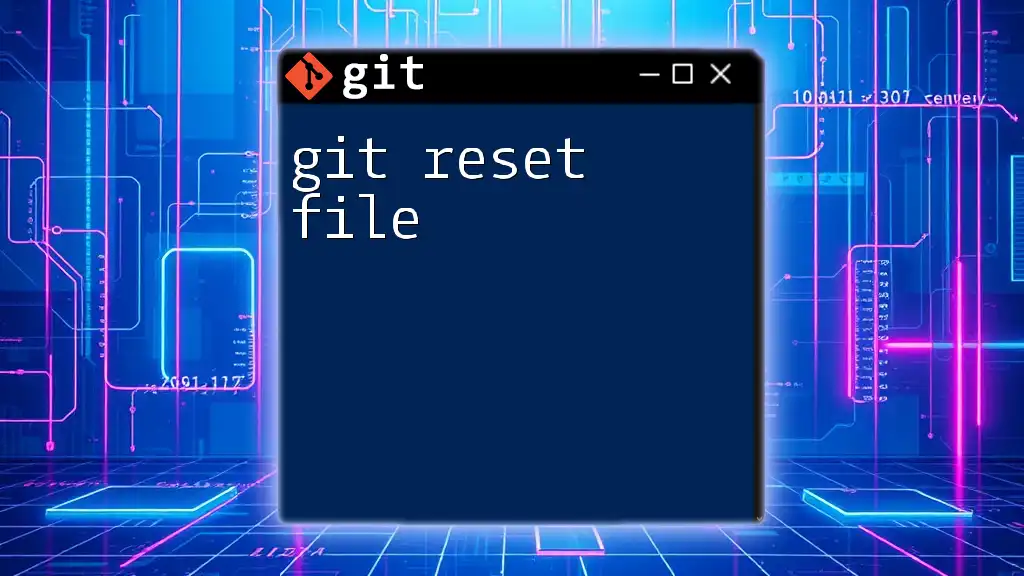
Why Use Git REST API?
Using the Git REST API has several advantages over traditional command-line interactions. Here are a few key reasons why developers opt for it:
- Automation: It enables automation of Git operations within scripts or applications, reducing the need for manual intervention.
- Integration: You can easily integrate Git functionalities into other software applications, enhancing productivity.
- Scalability: The API allows for scalability in managing repositories, especially when working with large teams or multiple projects.
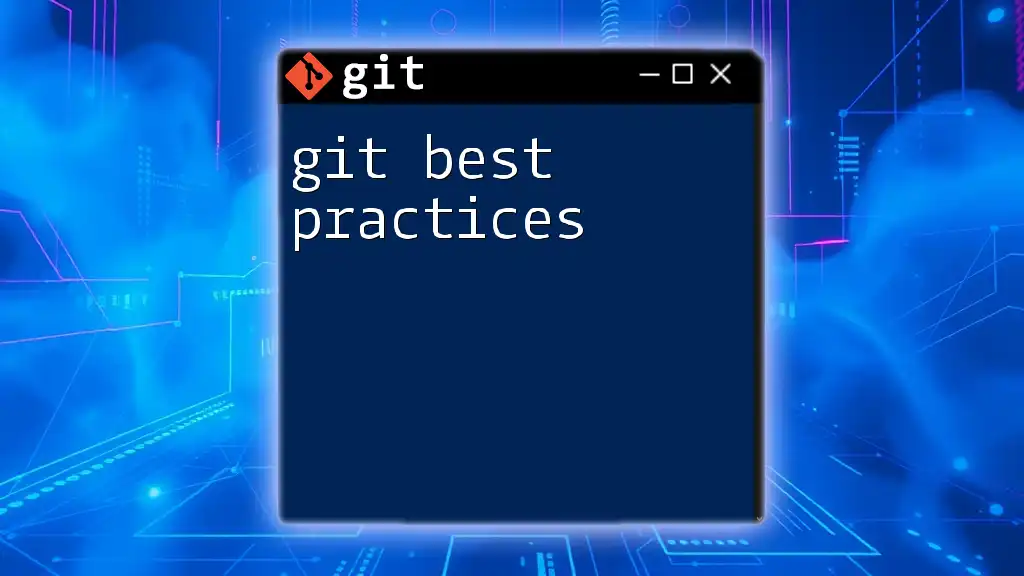
Understanding Git REST API Basics
Core Principles of REST APIs
To maximize the utility of the Git REST API, it’s essential to understand the core principles that govern RESTful APIs:
- Resources and Endpoints: In the context of the Git REST API, each entity (like repositories, commits, branches) is treated as a resource and is accessible via specific endpoints.
- HTTP Methods: API operations are typically performed using standard HTTP methods:
- GET: Retrieve data from the server.
- POST: Create a new resource.
- PUT: Update an existing resource completely.
- PATCH: Update part of a resource.
- DELETE: Remove a resource.
- Status Codes: Understanding response status codes (such as 200 for success, 404 for not found, or 401 for unauthorized) is crucial for developing effective API interactions.
Common Use Cases for Git REST API
Some of the common scenarios for using the Git REST API include:
- Integrating Git with other tools: For example, integrating with CI/CD tools for automated deployments.
- Building custom Git clients: Create specialized tools that suit specific workflows or requirements by leveraging the API to manage repositories.
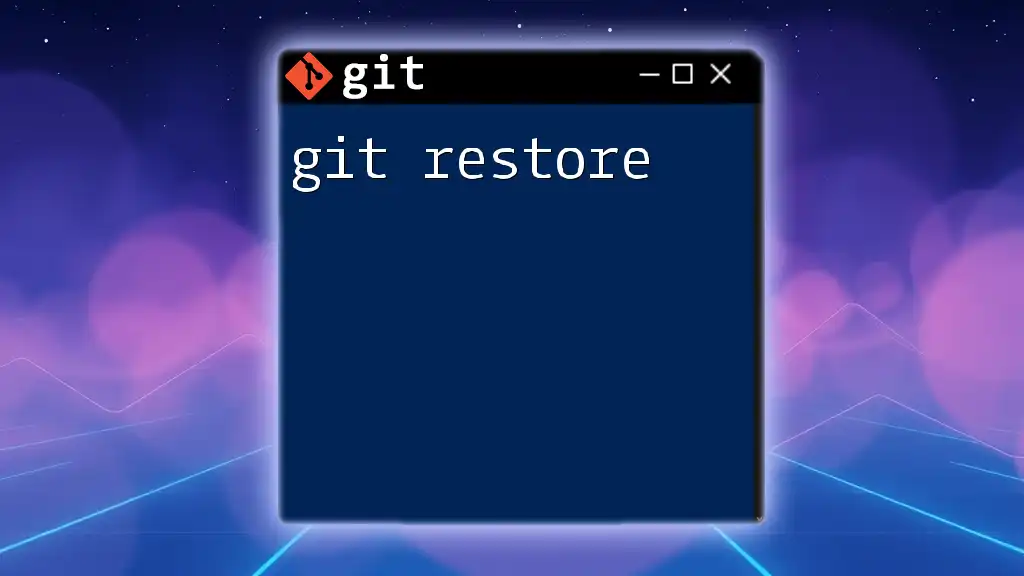
Getting Started with Git REST API
Authentication Methods
The first step to using Git REST API is authentication. The two primary methods are:
- Personal Access Tokens: A simple way to authenticate over HTTPS that is easy to create and use.
- OAuth Tokens: A more secure method for web applications but requires additional configuration.
To generate a Personal Access Token, follow these steps:
- Go to your Git hosting service (like GitHub or GitLab).
- Navigate to Developer settings.
- Choose Personal access tokens, and generate a new token with the required scopes.
Here’s an example code snippet to authenticate a request using a Personal Access Token:
curl -H "Authorization: token YOUR_PERSONAL_ACCESS_TOKEN" https://api.github.com/user
Basic Setup and Tools
To interact with the Git REST API, you can use tools like Postman for a user-friendly interface, or curl if you prefer the command line:
curl -H "Authorization: token YOUR_PERSONAL_ACCESS_TOKEN" https://api.github.com/users/YOUR_USERNAME
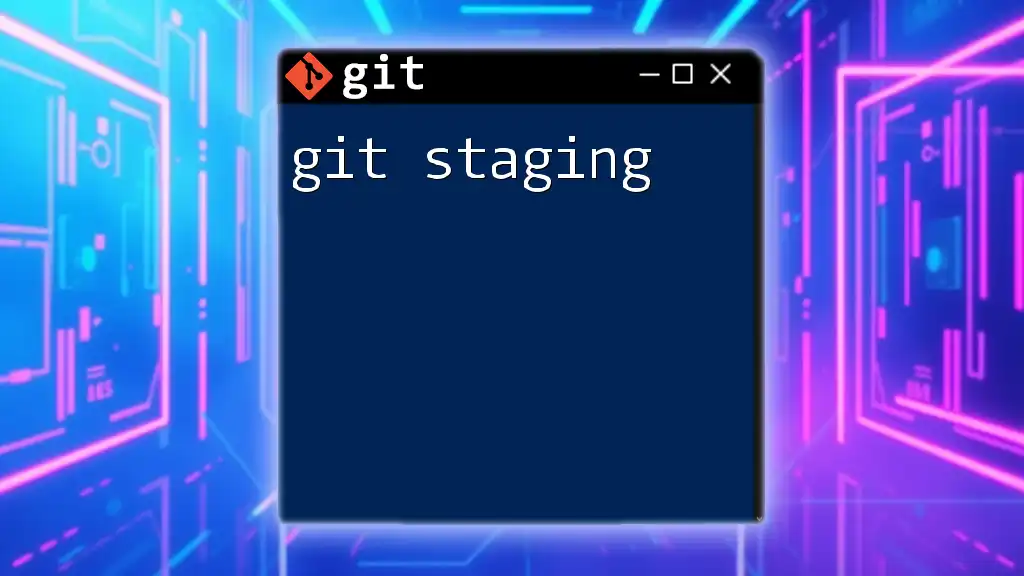
Exploring Git REST API Endpoints
Repository Endpoints
The Git REST API provides various endpoints to perform operations on repositories:
Creating a Repository
To create a new repository, you can send a POST request like this:
curl -X POST -H "Authorization: token YOUR_PERSONAL_ACCESS_TOKEN" \
-H "Content-Type: application/json" \
-d '{"name":"new-repo","private":false}' \
https://api.github.com/user/repos
Fetching Repository Information
You can retrieve details about a specific repository using the GET method:
curl -H "Authorization: token YOUR_PERSONAL_ACCESS_TOKEN" \
https://api.github.com/repos/YOUR_USERNAME/new-repo
Commit Endpoints
Understanding commits is essential to manipulating repositories:
Creating a Commit
To create a commit, you first need to add files to your repository:
curl -X POST -H "Authorization: token YOUR_PERSONAL_ACCESS_TOKEN" \
-H "Content-Type: application/json" \
-d '{"message":"Initial commit", "tree":"COMMIT_SHA", "parents":["PARENT_COMMIT_SHA"]}' \
https://api.github.com/repos/YOUR_USERNAME/new-repo/git/commits
Fetching Committed Changes
To retrieve the commit history, you can execute:
curl -H "Authorization: token YOUR_PERSONAL_ACCESS_TOKEN" \
https://api.github.com/repos/YOUR_USERNAME/new-repo/commits
Branching and Tagging
Branches and tags are crucial in managing different code versions:
Creating a Branch
To create a new branch using the Git REST API:
curl -X POST -H "Authorization: token YOUR_PERSONAL_ACCESS_TOKEN" \
-H "Content-Type: application/json" \
-d '{"ref":"refs/heads/new-branch","sha":"COMMIT_SHA"}' \
https://api.github.com/repos/YOUR_USERNAME/new-repo/git/refs
Tagging a Release
You can easily tag a release with a request like:
curl -X POST -H "Authorization: token YOUR_PERSONAL_ACCESS_TOKEN" \
-H "Content-Type: application/json" \
-d '{"tag":"v1.0.0","message":"Release v1.0.0","object":"COMMIT_SHA","type":"commit"}' \
https://api.github.com/repos/YOUR_USERNAME/new-repo/git/tags
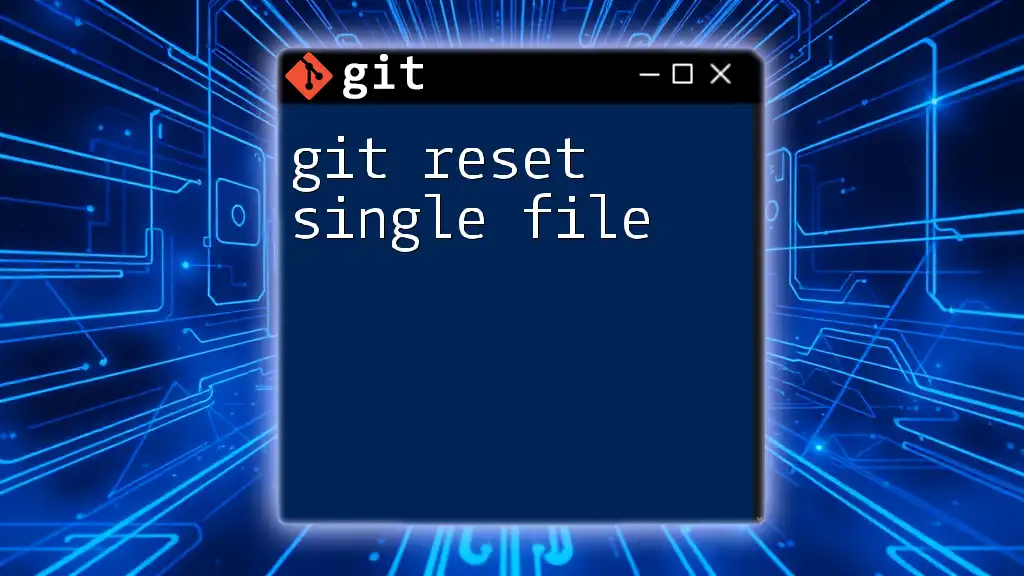
Advanced Git REST API Features
Handling Webhooks
Webhooks are powerful features that allow you to receive real-time updates from your Git repositories. This can be invaluable for applications that require immediate notification of changes or events.
Setting up a webhook involves registering a URL with your Git service that will be triggered on specified events, such as pushes or pull requests.
Working with Pull Requests
Pull requests are central to collaborative development:
Creating a Pull Request
Use the following example to create a pull request:
curl -X POST -H "Authorization: token YOUR_PERSONAL_ACCESS_TOKEN" \
-H "Content-Type: application/json" \
-d '{"title":"New Feature","head":"feature-branch","base":"main"}' \
https://api.github.com/repos/YOUR_USERNAME/new-repo/pulls
Reviewing and Merging Pull Requests
To review open pull requests, you can fetch them with:
curl -H "Authorization: token YOUR_PERSONAL_ACCESS_TOKEN" \
https://api.github.com/repos/YOUR_USERNAME/new-repo/pulls
Merging a pull request can be done using:
curl -PUT -H "Authorization: token YOUR_PERSONAL_ACCESS_TOKEN" \
-d '{"commit_message":"Merging pull request"}' \
https://api.github.com/repos/YOUR_USERNAME/new-repo/pulls/PULL_REQUEST_NUMBER/merge
Using the Git REST API for CI/CD
Integrating Git operations into your CI/CD pipeline can massively simplify deployment processes. Tools like Jenkins and GitHub Actions can use the Git REST API to automatically push changes, run tests, and manage deployments based on the state of your repository.
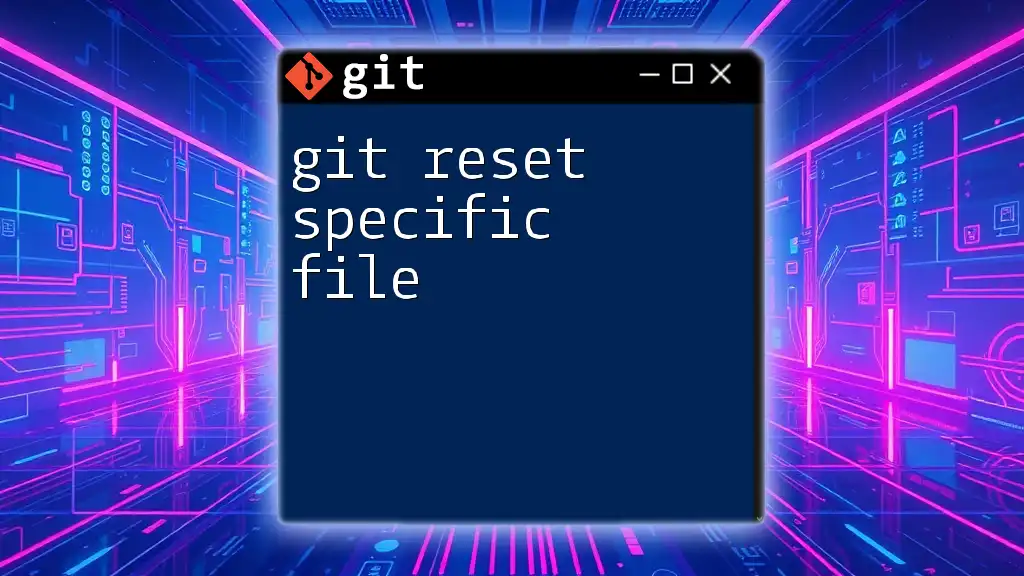
Error Handling & Debugging
Common Errors and Their Solutions
Some frequent issues include authentication errors (401), not found (404), or rate limit exceeded (403). Understanding these errors can help in troubleshooting.
Best Practices for Debugging API Calls
When debugging API interactions, it's beneficial to log request and response details. Using a tool like Postman can help visualize these interactions effectively.
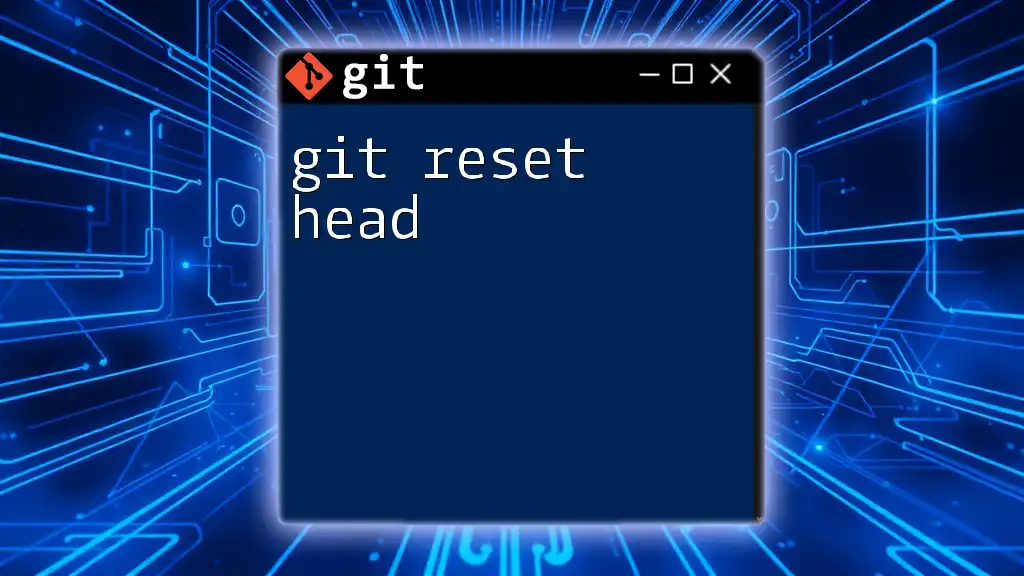
Tips for Effective Use of Git REST API
Rate Limiting and Throttling Considerations
Be aware of the rate limits imposed by the Git service you are using. This is usually specified in the API documentation. Implementing a back-off strategy can be useful when approaching these limits.
Optimizing API Calls for Performance
To enhance performance, consider batching requests where possible. Instead of making multiple API calls individually, you can often make a single call that retrieves or modifies multiple resources.
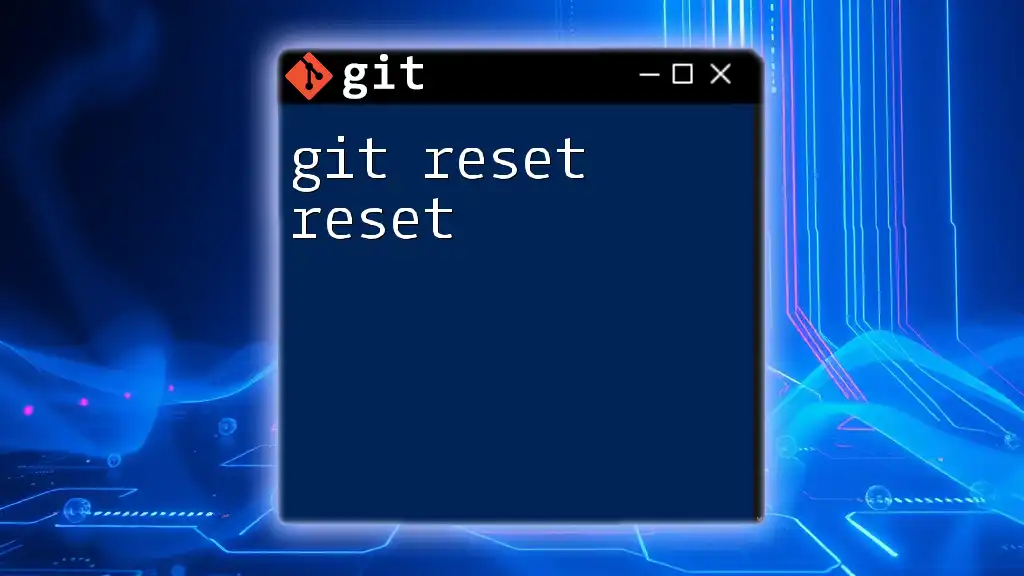
Resources for Learning More
Official Documentation
For detailed information, always refer to the official documentation of the Git REST API you are working with (like GitHub, GitLab, etc.), as it contains comprehensive information about available endpoints, parameters, and more.
Community and Forums
Engaging with communities on platforms like Stack Overflow or GitHub discussions can provide insights and additional help when you're tackling specific issues or need best practices from experienced developers.
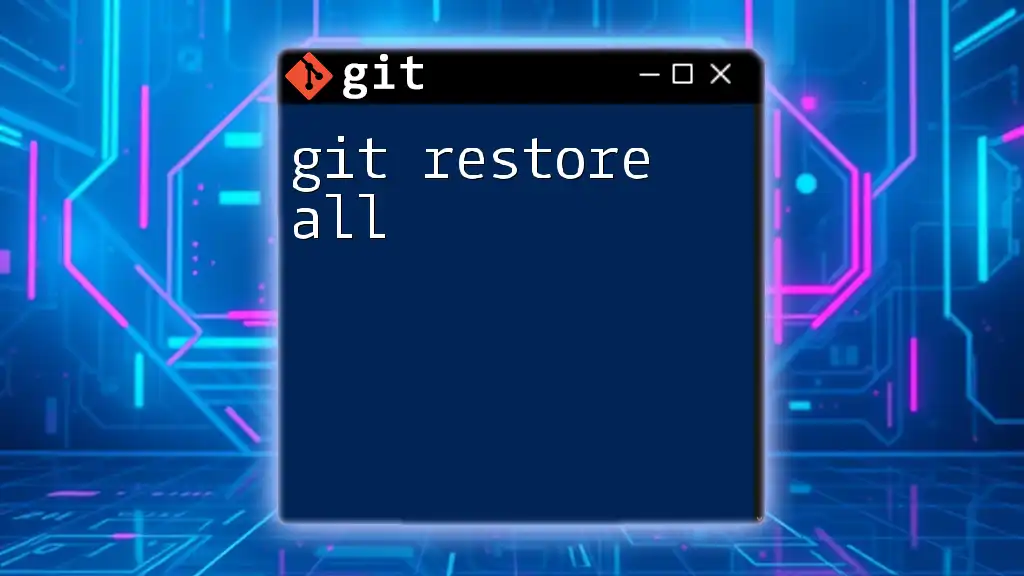
Conclusion
The Git REST API is a powerful tool that allows developers to interact programmatically with Git repositories. By understanding its capabilities and best practices, you can enhance your development workflows, automate tasks, and gain all the benefits of using Git without diving deep into command-line syntax.
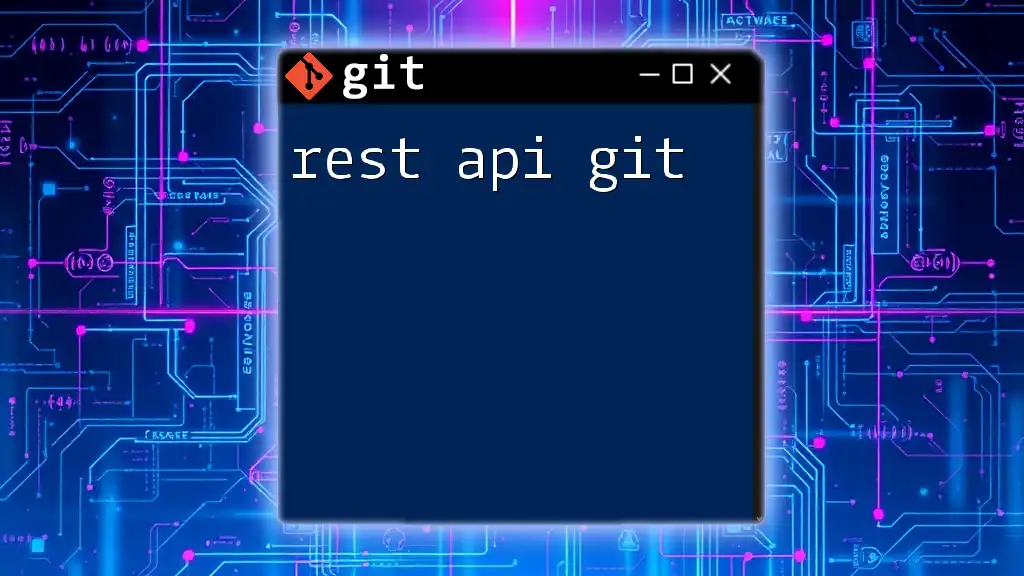
Call to Action
For those eager to learn more, we invite you to join us for upcoming tutorials and guides that will deepen your understanding of Git and its API. Whether you're a beginner or an experienced developer, there’s always more to discover!