Git API refers to the use of Git commands and functionalities through a programming interface, enabling developers to automate and integrate Git operations within their applications.
Here's a simple example of using the Git API to create a new branch:
git checkout -b new-feature-branch
Understanding Git API
What is Git API?
The Git API is an interface that allows developers to interact programmatically with Git repositories. It provides a way to access and manipulate data stored in version control systems like GitHub and GitLab. The Git API essentially acts as a bridge, enabling software applications to interact with Git through HTTP requests, allowing for seamless automation, integrations, and custom applications.
Why Use Git API?
Utilizing the Git API can significantly enhance a development workflow. Its advantages include:
- Automation: Automate repetitive tasks such as repository management, issue tracking, and release workflows.
- Integration: Integrate services and applications smoothly. For example, linking CI/CD tools with repositories.
- Flexibility: Build custom applications that cater specifically to your team's needs or workflow.
Using the Git API allows developers to perform actions without the need for command line interactions, which can be particularly useful in environments where automation is key.
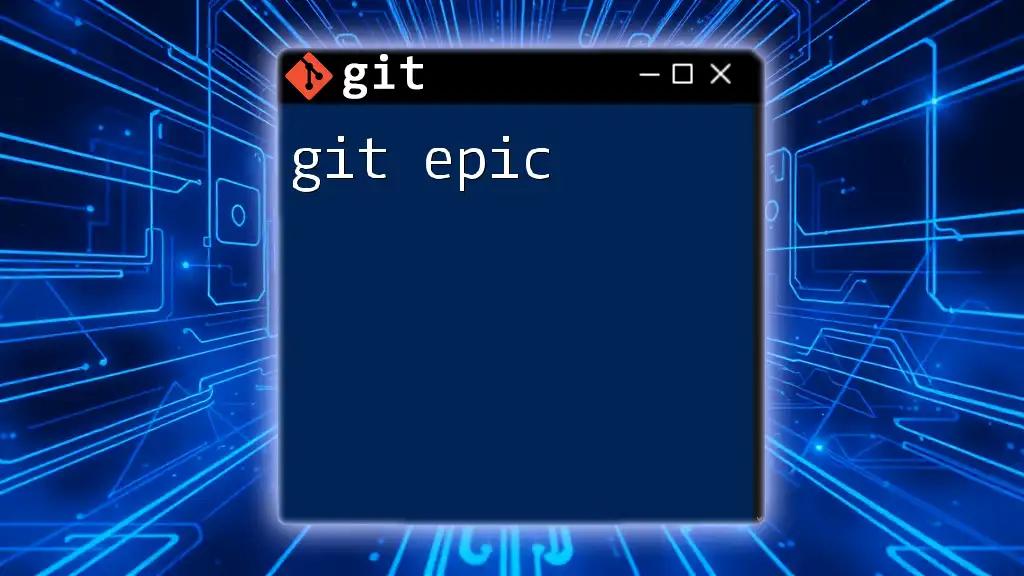
Key Concepts of Git API
RESTful Principles in Git API
The Git API often adheres to principles of REST (Representational State Transfer), which means it uses standard HTTP methods:
- GET: Retrieve data (e.g., fetching repository details).
- POST: Send data to create resources (e.g., adding a commit).
- PUT: Update existing resources (e.g., modifying a file).
- DELETE: Remove resources (e.g., deleting a repository).
Each endpoint in the Git API follows these principles, making it predictable and easier to work with.
Authentication and Authorization
For security, authentication is mandatory when accessing the Git API. The most common methods include:
- Basic Authentication: Involves sending your username and password with each request.
- OAuth: A more secure method that generates tokens for authorization without sharing credentials.
Example of authenticating with GitHub API using Basic Authentication in `curl`:
curl -u username:token https://api.github.com/user
In this command, `username` is your GitHub username, and `token` is your personal access token.
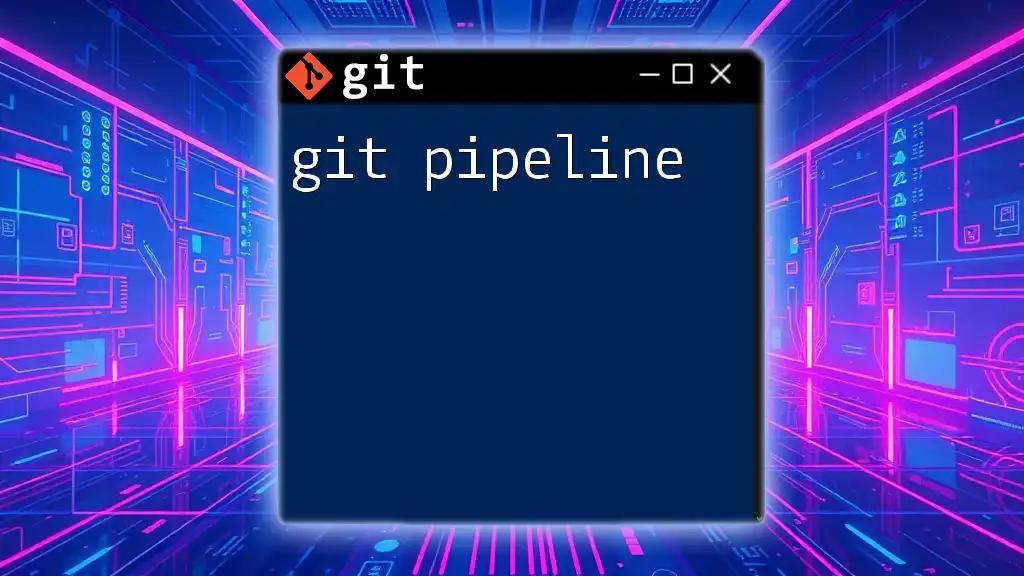
Working with Git API
Setting Up Your Environment
Before starting, ensure you have a GitHub or GitLab account with API access and obtain your access tokens. You can use tools like Postman or curl for testing API calls.
Essential Git API Commands
Repositories
One of the fundamental areas of interaction with the Git API is repository management.
- Retrieving Repository Information: To get details about a specific repository, use:
curl https://api.github.com/repos/username/repo-name
This retrieves essential information, such as the repository structure, owner details, and visibility status.
- Creating a New Repository: To create a new repository, send a `POST` request with a JSON body:
curl -X POST -H "Authorization: token YOUR_TOKEN" \
-H "Content-Type: application/json" \
-d '{"name": "new-repo", "private": false}' \
https://api.github.com/user/repos
This command creates a public repository named "new-repo."
- Deleting a Repository: To remove an existing repository, use:
curl -X DELETE -H "Authorization: token YOUR_TOKEN" \
https://api.github.com/repos/username/repo-name
This command permanently deletes the specified repository.
Commits
Working with commits is critical for maintaining project history.
- Fetching Commit History: Retrieve the commit log of a repository using:
curl https://api.github.com/repos/username/repo-name/commits
This returns a list of past commits with associated metadata.
- Creating a New Commit: Making a commit requires setting the context first—sending the actual data through the appropriate endpoint. Here's a simplified command:
curl -X POST -H "Authorization: token YOUR_TOKEN" \
-H "Content-Type: application/json" \
-d '{"message": "Commit message","content": "base64-encoded file content"}' \
https://api.github.com/repos/username/repo-name/contents/path/to/file
In this command, replace `"Commit message"` and the content appropriately.
- Updating Existing Commits: Although rewriting commit history is generally advised against, you can do so for the most recent commit using:
curl -X PATCH -H "Authorization: token YOUR_TOKEN" \
-H "Content-Type: application/json" \
-d '{"message": "Updated commit message"}' \
https://api.github.com/repos/username/repo-name/git/commits/commit_sha
Branches
Branch management is crucial for facilitating feature development.
- Listing Branches: To fetch all branches in a repository, use:
curl https://api.github.com/repos/username/repo-name/branches
- Creating and Deleting Branches: You can create a new branch based on a commit reference:
curl -X POST -H "Authorization: token YOUR_TOKEN" \
-H "Content-Type: application/json" \
-d '{"ref":"refs/heads/new-branch","sha":"commit_sha"}' \
https://api.github.com/repos/username/repo-name/git/refs
For deleting a branch, send a `DELETE` request:
curl -X DELETE -H "Authorization: token YOUR_TOKEN" \
https://api.github.com/repos/username/repo-name/git/refs/heads/branch_name
- Merging Branches: Merge a branch back into the main branch with:
curl -X POST -H "Authorization: token YOUR_TOKEN" \
-H "Content-Type: application/json" \
-d '{"base": "main", "head": "new-branch"}' \
https://api.github.com/repos/username/repo-name/merges
Error Handling in Git API
When making API requests, you may encounter various error responses. Common HTTP error codes include:
- 404 Not Found: You might be trying to access a resource that doesn’t exist.
- 403 Forbidden: It indicates you don’t have the necessary permissions to perform the action.
- 401 Unauthorized: Your authentication failed, often due to an invalid token.
Properly handle these errors by checking response codes and informing users or logging errors for debugging.
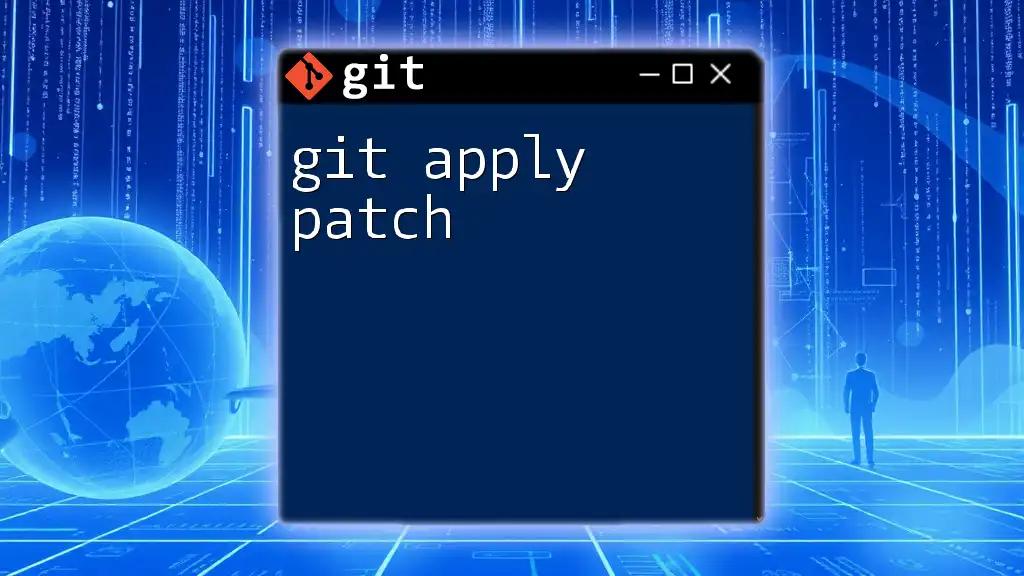
Integrating Git API with Applications
Case Study: Automating Deployment Processes
An exciting implementation of the Git API is automating deployment processes. Consider a scenario where each commit to the main branch triggers a deployment process:
- Set up a webhook on your repository to listen for push events.
- Upon receiving a push event, automatically fetch the latest commit details using the Git API.
- Trigger a CI/CD pipeline using the Git API to build and deploy your application seamlessly.
Building Custom Applications with Git API
Building your custom applications using the Git API can provide tailored features that meet your team's specific needs.
Example Project: Building a Git Dashboard
You can create a Git dashboard that displays real-time insights of repositories, such as:
- Open pull requests
- Recent issues
- Commit history
Utilizing frameworks such as Node.js or Python Flask, you can continuously poll the Git API and visualize data in a user-friendly manner.
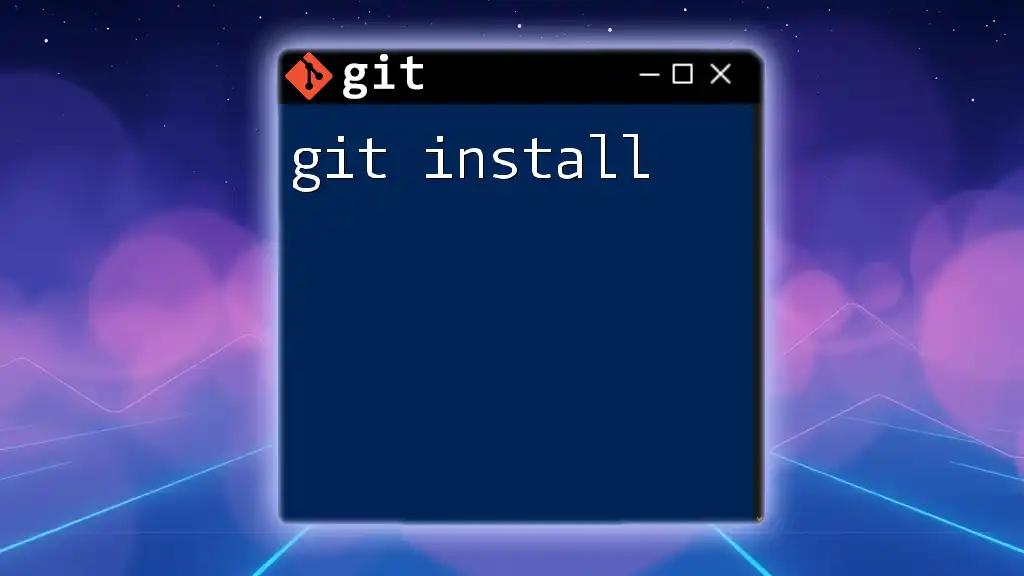
Best Practices for Using Git API
Optimization Techniques
To ensure optimal performance when using the Git API:
- Minimize API Calls: Reduce the number of requests by caching the results, especially for data that does not change frequently.
- Throttling Requests: Be aware of rate limits enforced by APIs. Implement retries with exponential backoff to avoid getting blocked.
- Batch Requests: Use batch endpoints provided by some APIs to reduce the number of calls.
Staying Updated
APIs often undergo changes and enhancements. Keep up with the official Git API documentation to stay informed about the latest features, deprecated functions, and best practices.
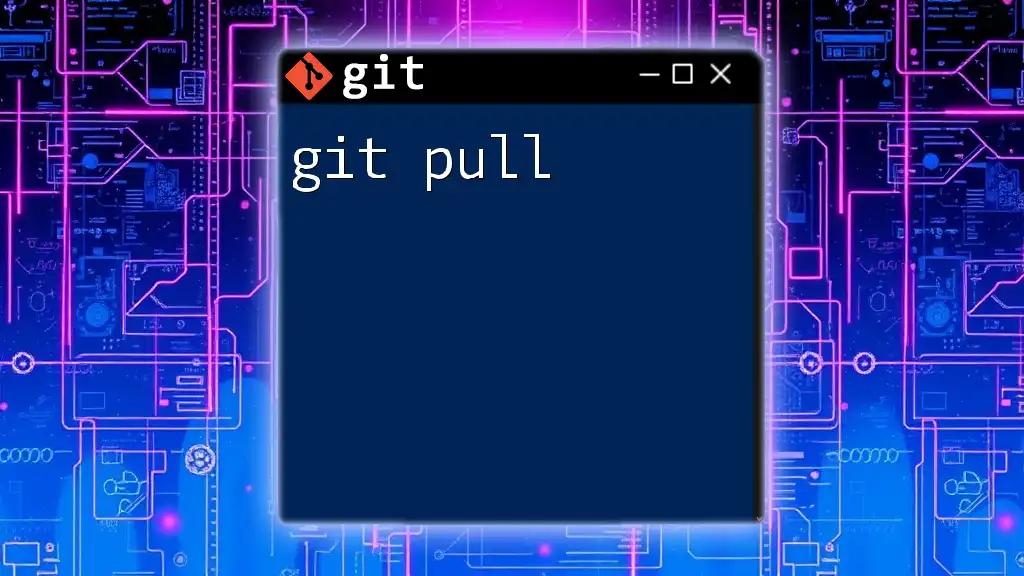
Conclusion
Mastering the Git API can significantly enhance your development processes by enabling automation, integrations, and custom applications. Whether you’re managing repositories or building custom tools, the possibilities are endless.
Additional Resources
For further learning, explore the following:
- [GitHub REST API Documentation](https://docs.github.com/en/rest)
- [GitLab API Documentation](https://docs.gitlab.com/ee/api/)
- Community forums and platforms like Stack Overflow for discussion and help.
In summary, dive into the Git API and discover how it can transform your development experience today!