The `git diff` command shows the differences between various commits, the working tree, and the staging area, allowing you to see what has changed in your files.
Here's a code snippet demonstrating how to use `git diff`:
git diff
What is `git diff`?
`git diff` is a command used in Git, the popular version control system, to compare changes between various states of a file or multiple files. Understanding how to utilize `git diff` is crucial for any developer, as it allows one to assess modifications, identify issues, and manage code efficiently—ensuring that the development process remains organized and transparent.
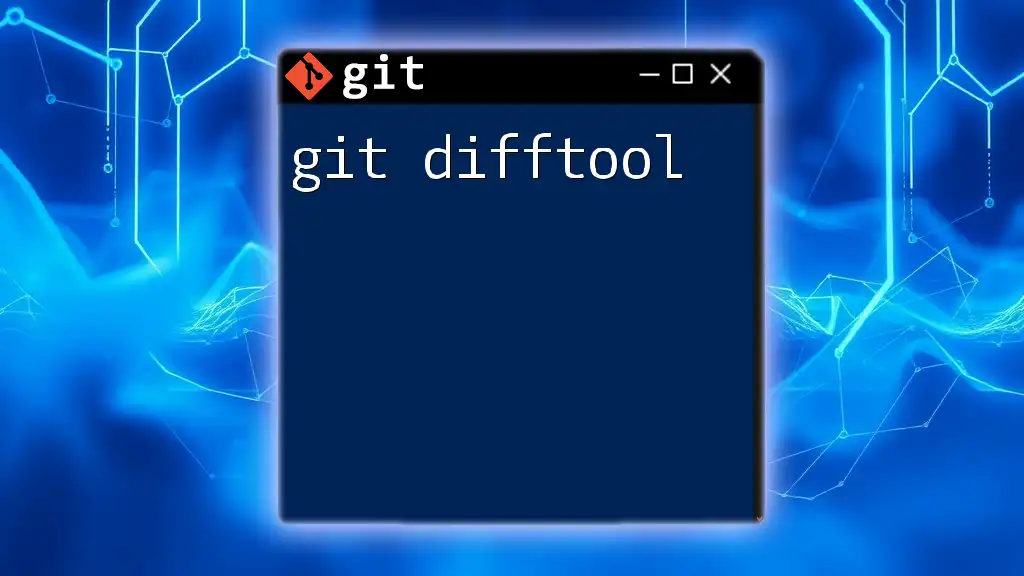
The Basics of `git diff`
The Structure of a `git diff` Output
When you run `git diff`, the output typically consists of several components that depict the differences between file versions. You'll see:
- Changed lines are highlighted, often with a + symbol for additions and a - symbol for deletions.
- File headers indicate which files are being compared.
- Context lines surrounding the changes provide helpful reference points, showing where the changes occur in relation to the rest of the file.
Basic Command Syntax
The fundamental syntax for using `git diff` is:
git diff [options] [<commit>] [--] [<path>...]
Each component functions as follows:
- options: Optional flags that modify the behavior of the command.
- <commit>: A specific commit to compare against.
- <path>: The path of the file or files you wish to check.
Examples of Basic Usage
Comparing working directory changes To see changes made to files since the last stage:
git diff
This command provides a comprehensive view of modifications that remain uncommitted.
Comparing staged changes To inspect changes that have been staged and are ready for the next commit:
git diff --cached
This helps ensure you're aware of what will be included in your next commit, aiding in maintaining clean commit histories.
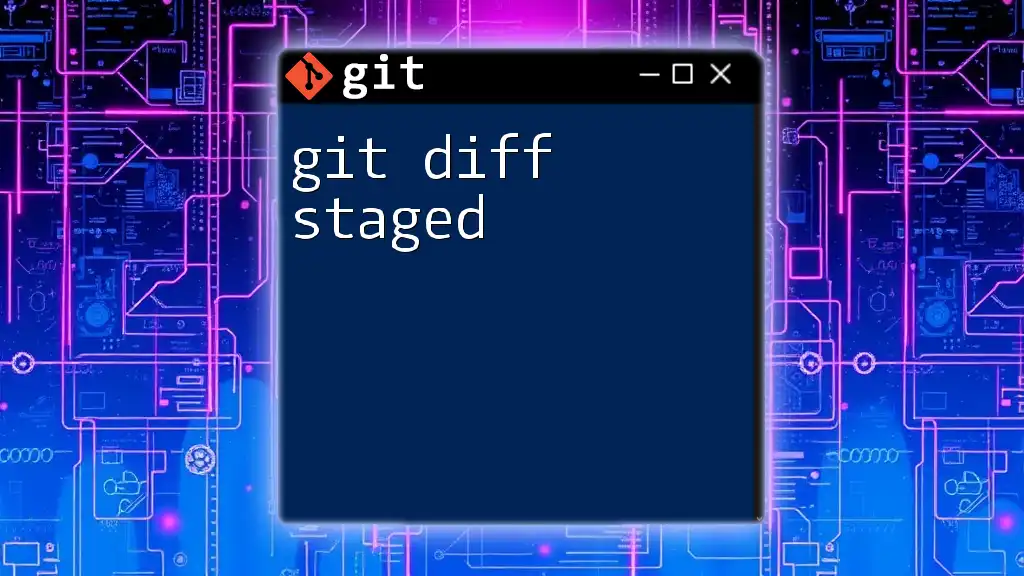
Advanced Usage of `git diff`
Comparing Commits
To compare two specific commits, the syntax is:
git diff <commit1> <commit2>
For instance, if you want to compare the most recent commit to the one before it, use:
git diff HEAD~1 HEAD
This command allows you to see the exact changes made during that most recent commit, providing insight into what modifications occurred.
Comparing Branches
You may want to see the differences between two branches. The command format here is:
git diff <branch1>..<branch2>
For example, to compare your `feature-branch` to the `main` branch:
git diff main..feature-branch
This gives you a detailed look at what has been added or removed in the feature branch compared to the main line of development.
Using Flags for Enhanced Output
Unified and Context Diffs
You can adjust the number of context lines in your diff output to gain better insight into the changes. For a more detailed look, use:
git diff -U3
This shows three lines of context around each change, helping you understand the modifications in relation to surrounding code.
Word Diffs
To drill down to more granular changes, such as viewing differences at the word level, you can run:
git diff --word-diff
This command highlights changes within lines, which may be especially useful for textual modifications.
Ignoring Whitespace
When working on projects where formatting changes might clutter the diff output, you can instruct Git to ignore these changes with:
git diff -w
Utilizing this flag can improve readability, enabling you to focus on substantial, logical changes.
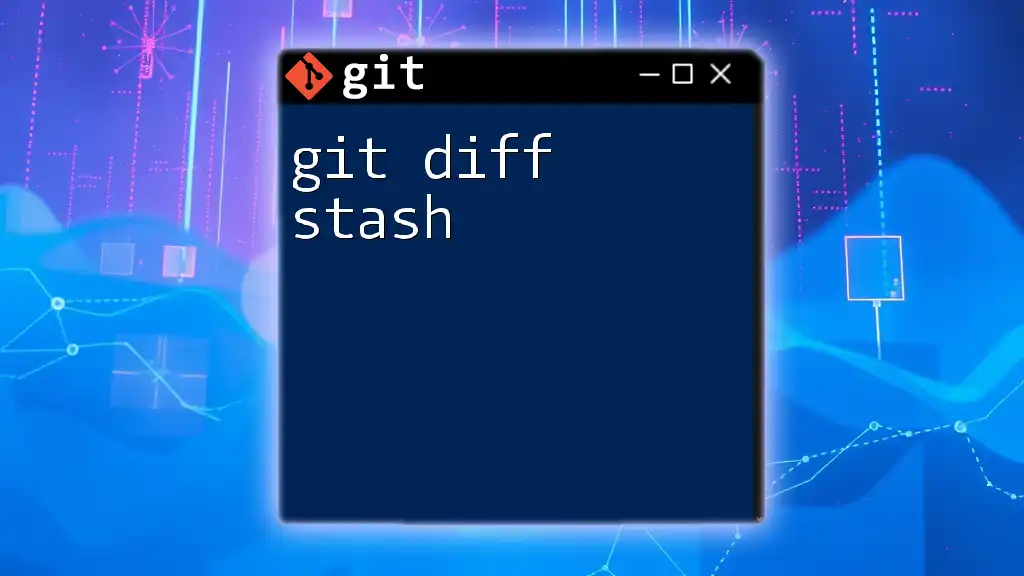
Visualizing Differences with Diff Tools
Integrating Diff Tools
Many developers prefer visual representations of differences for clarity. Git allows you to integrate various graphical diff tools. To initiate a diff using a configured tool, simply use:
git difftool
This command opens the selected external diff tool, offering an intuitive interface to review file differences visually and efficiently.
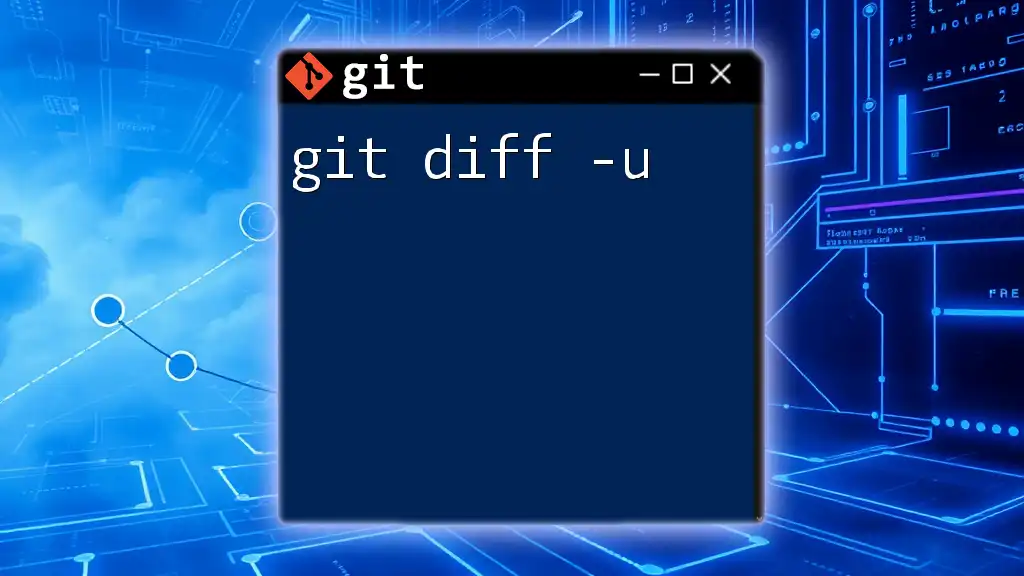
Common Scenarios and Use Cases
Reviewing Code Before Merging
One of the most critical uses of `git diff` is in code reviews before merging changes. It provides a means to verify that modifications are correct, consistent, and meet project standards, reducing the potential for introducing bugs.
Tracking Changes Over Time
Over the lifespan of any project, using `git diff` enables you to track how your codebase evolves. You can easily identify when features were added, issues were fixed, or styles were changed, thus giving insight into the development process.
Collaborating with Teams
In collaborative environments, `git diff` facilitates clear communication by allowing team members to see what others are working on. This transparency helps in coordinating efforts and ensuring that everyone is aligned on project objectives.
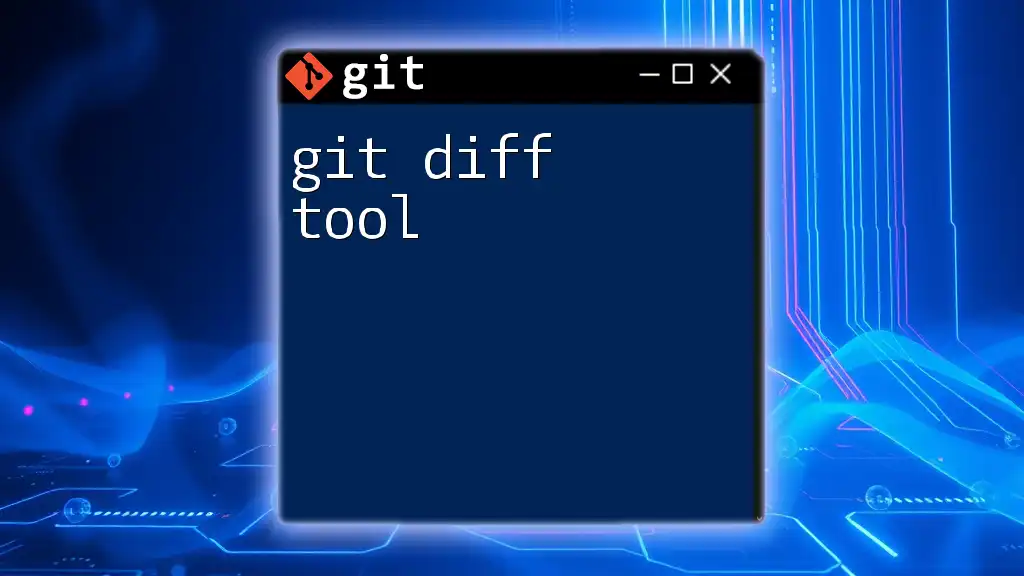
Conclusion
In conclusion, mastering the `git diff` command is an invaluable step for any developer engaging with Git. It provides a powerful means to understand and visualize changes, ensuring clean project management and collaboration. By regularly incorporating `git diff` into your workflow, you can enhance your coding practices and maintain a clear overview of your project's evolution.
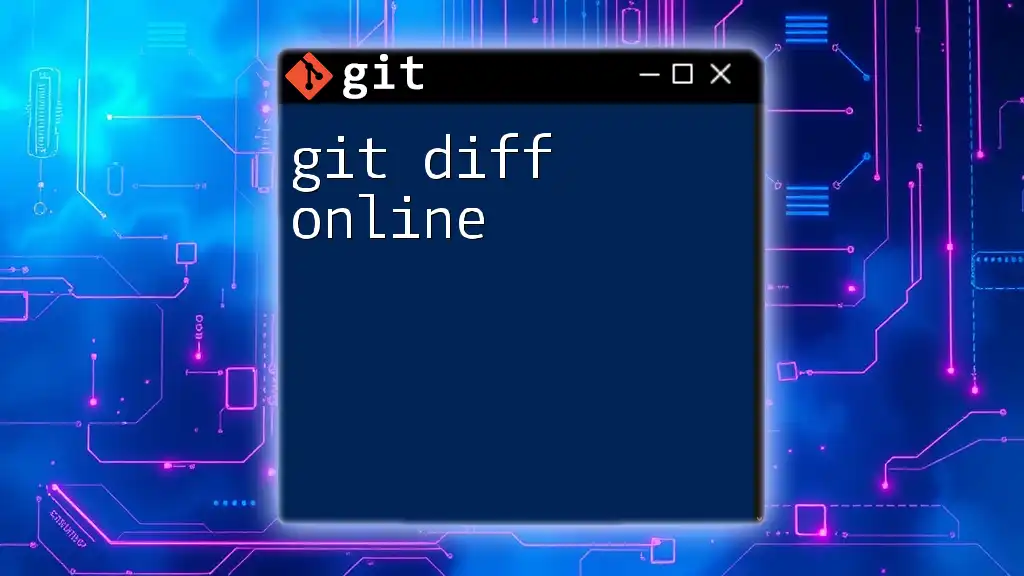
Further Reading and Resources
To expand your knowledge of `git diff` further, consider exploring the official Git documentation, as well as recommended tutorials that delve deeper into Git usage and best practices in the development landscape.
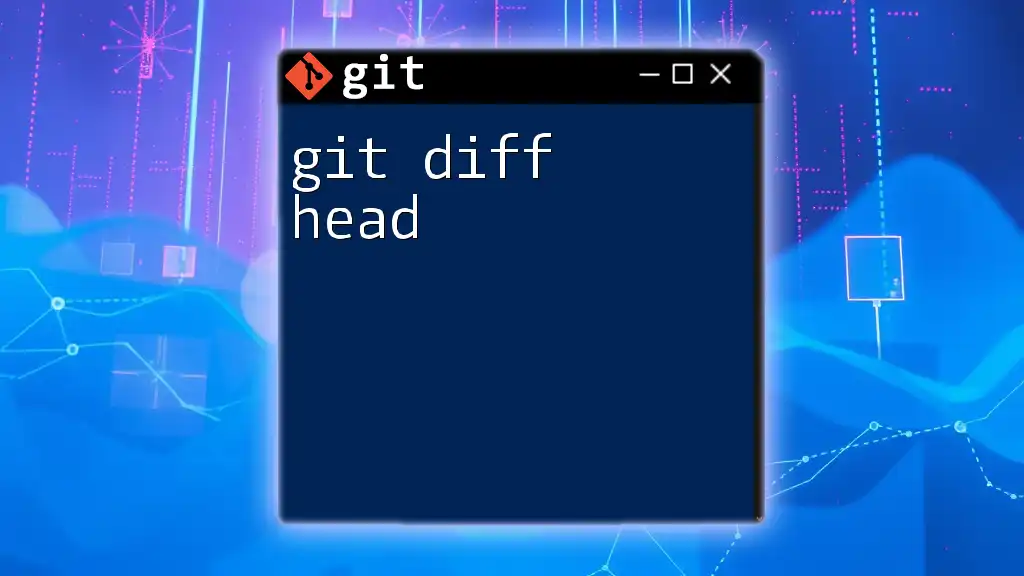
Call to Action
Feel free to share your experiences or questions concerning `git diff`. Engaging with others can help reinforce your understanding. Don't forget to subscribe to our newsletter for more Git-related tips and resources!