The `git diff` command is used to show the changes between commits, commit and working tree, etc., displaying the differences in a concise format.
git diff
Understanding Git Diff
What Does `git diff` Do?
The `git diff` command plays a crucial role in version control, acting as a comparison tool between different versions of your files within your Git repository. When executed, it showcases the differences between files, highlighting what has been added, modified, or deleted. The output typically consists of two distinct sections: lines prefixed by a minus sign (-) indicate deletions, while lines prefixed by a plus sign (+) signify additions. Understanding these symbols will help you quickly grasp the changes made during your coding sessions.
Common Scenarios for Using Git Diff
There are several practical scenarios where `git diff` becomes indispensable:
-
Comparing Uncommitted Changes: Use this to review what you've modified but haven't staged yet. This is useful for verifying that your code changes align with expectations before going live or committing them.
-
Comparing Changes Between Commits: When analyzing the evolution of your codebase, `git diff` can be utilized to compare specific commits. This can help you understand why certain changes were made, improving your understanding of the project's history.
-
Comparing Branches: To assess how different branches have diverged from one another, `git diff` lets you compare various branches. This aids in making informed decisions about merging or rebasing.
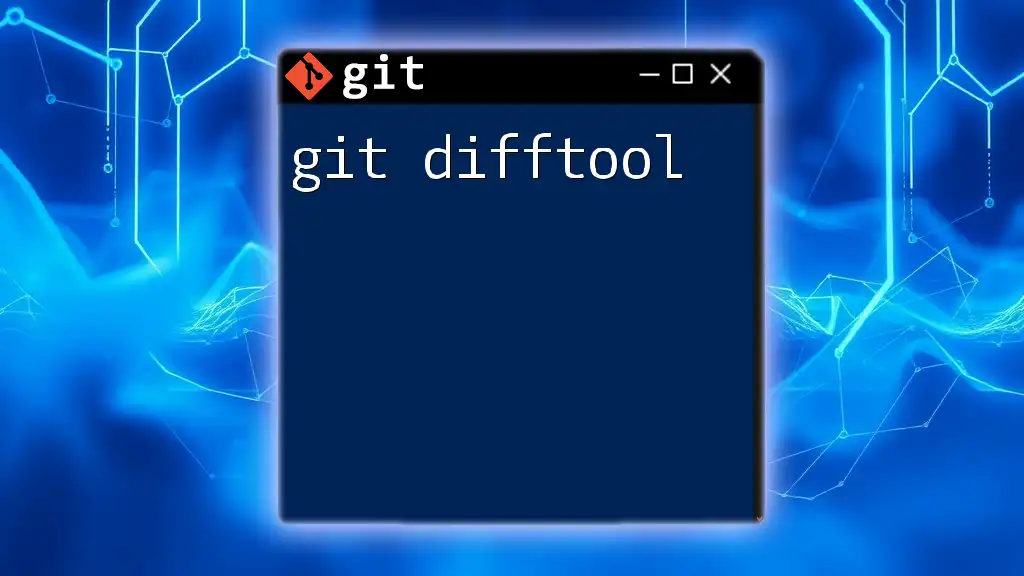
Online Tools for Git Diff
Overview of Online Git Diff Tools
Online tools that specialize in Git diff capabilities provide a simple and user-friendly experience. These tools are especially beneficial when you need to compare code snippets without the overhead of a full developer environment. The benefits include:
- Easy accessibility from any device with an internet connection.
- Intuitive interfaces that often provide graphical representations of differences.
- Compatibility with various file formats and the ability to manipulate files effortlessly.
Popular Online Git Diff Platforms
Some noteworthy online diff tools include:
-
GitHub's Diff Viewer: An integrated tool that allows developers to see changes between pull requests and commits directly on the platform.
-
GitLab’s Merge Request Diff Viewer: This platform provides a thorough comparison of changes in merge requests, significantly streamlining code review processes.
Comparing Local Files Using Online Tools
Here’s how to compare local files using these online platforms:
Step 1: Open your preferred online diff tool.
Step 2: Copy the content of your local files into the provided text areas.
Step 3: Click on the compare button to visualize the differences.
For example, consider the following two simple local files:
echo "Hello World!" > file1.txt
echo "Hello, Git World!" > file2.txt
When you input these into an online diff tool, the output will clearly show that "World!" was altered to "Git World!" and that a comma was added, making it easier to comprehend the changes at a glance.
Implementation of Git Diff in Code Repositories
Many online services, like GitHub and GitLab, offer built-in capabilities for visualizing diffs directly within your repositories. When you create pull requests or merge requests, these platforms automatically generate a detailed git diff, making it especially user-friendly for both technical and non-technical stakeholders.
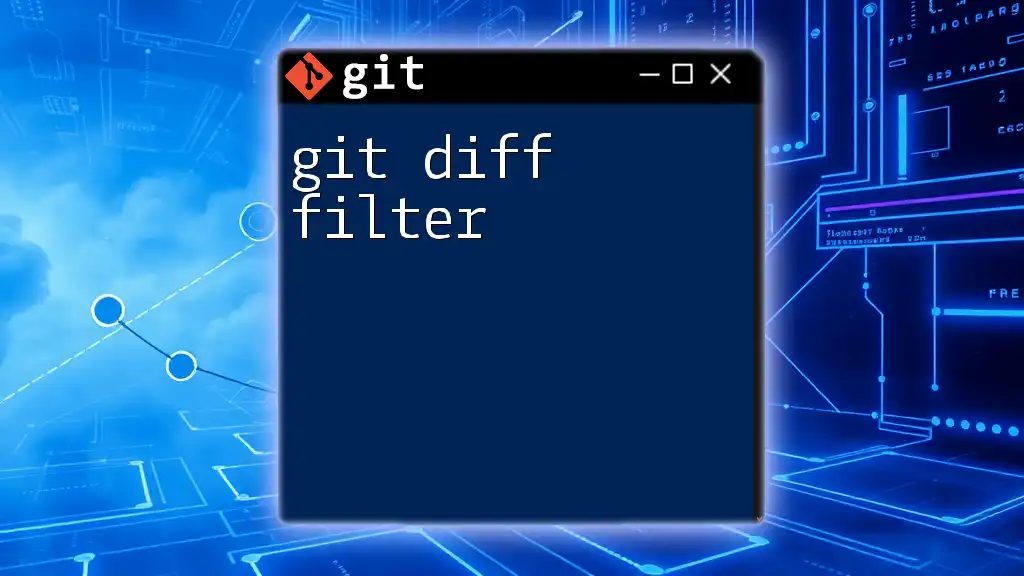
How to Use `git diff` in Your Workflow
Basic Syntax of `git diff`
The `git diff` command can be executed with various options based on your needs. The general syntax is:
git diff [options] [<commit>] [<commit>] [--] [<path>...]
Practical Examples
Here are some essential commands with explanations:
-
Comparing Working Directory to Staged Changes: If you want to see what changes you've made that are not yet staged, simply run:
git diff
This displays all untracked modifications, allowing you to verify your work before staging.
-
Comparing Staged Changes to the Last Commit: To inspect what you have staged for commit:
git diff --cached
This command helps confirm that you've tracked the right changes before committing them to the repository.
-
Comparing Two Commits: If you're interested in understanding what changes were made between two different commits, you can use:
git diff commit1 commit2
Replace `commit1` and `commit2` with the respective commit hashes. This is beneficial for retrospective code analysis.
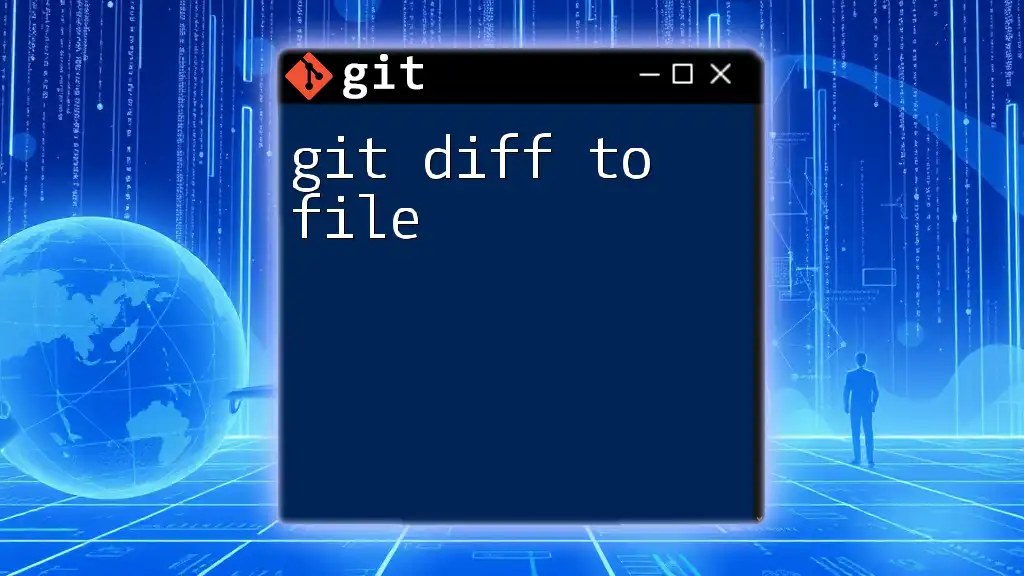
Visualizing Differences
Advantages of Visual Diff Options
While command-line tools are powerful, visual diff options can make it easier to grasp complex changes through graphical representations. This can simplify the review process, allowing for quicker and clearer communication among team members.
Recommended Tools
Some recommended tools for visualizing Git diffs include:
-
Gitk: A straightforward GUI that enables viewing your commit history and comparing changes.
-
SourceTree: A visually appealing Git client that simplifies the process of managing repositories and understanding diffs.
-
Visual Studio Code Extensions: Extensions like GitLens can augment your editing experience by showcasing inline diffs and history.
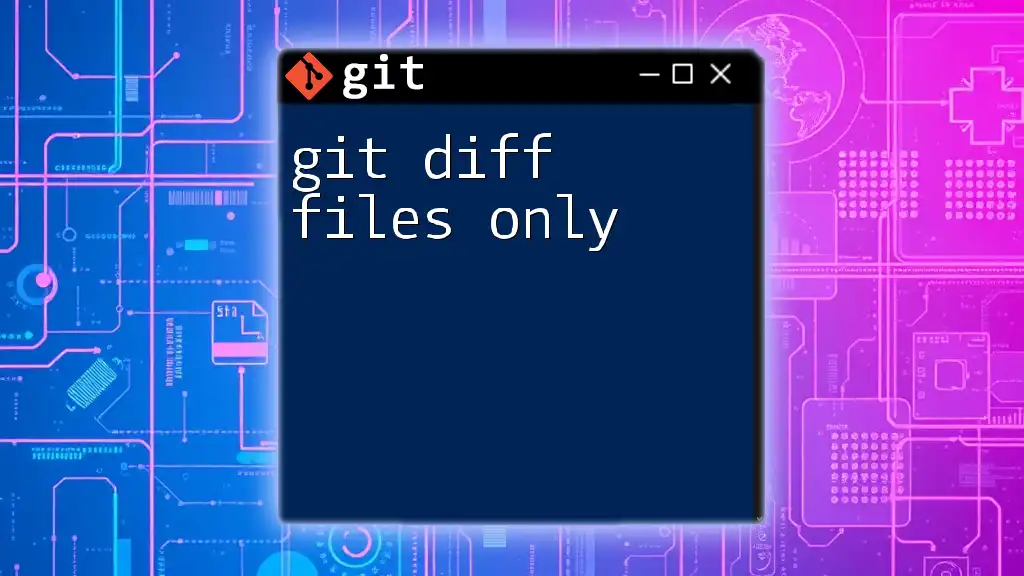
Advanced Git Diff Techniques
Using Options with `git diff`
The execution of `git diff` can be refined using various options that enhance its functionality. Here are some useful flags:
-
`--color`: This adds color coding to your output, making it visually easier to identify changes.
-
`--stat`: Displays a summary of the changes in a concise format instead of the full diff.
-
`--name-only`: Lists just the names of changed files without displaying the diffs, useful for a quick overview.
Leveraging `git difftool`
An alternative to `git diff` is `git difftool`, which allows you to use third-party diff tools for more enhanced visual comparisons. To invoke this command:
git difftool
This facilitates a more graphical approach to comparing differences, especially useful when dealing with larger or more complex changes across multiple files.
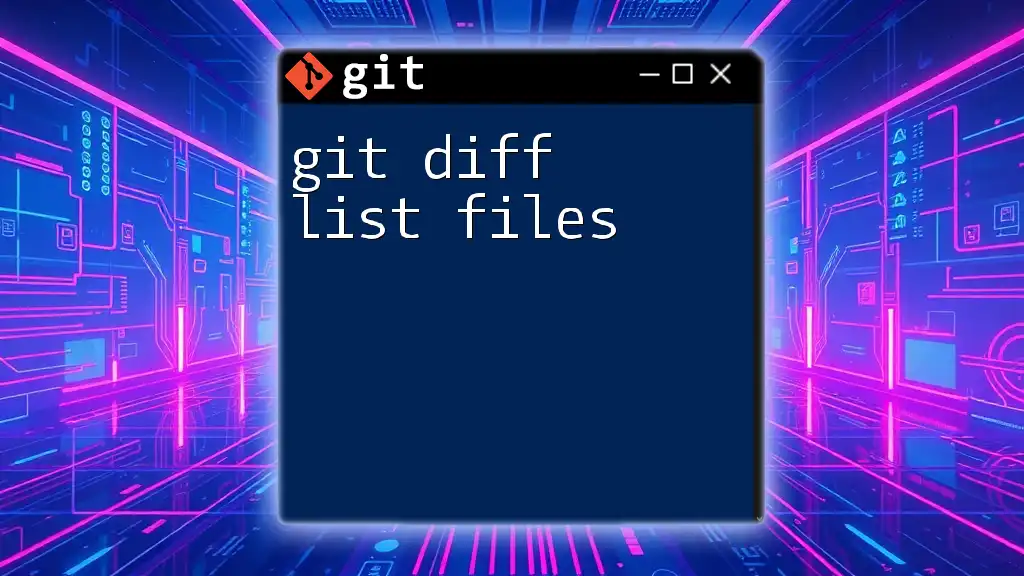
Conclusion
In summary, mastering the `git diff` command and utilizing online diff tools significantly enhances your efficiency and ability to track changes in your projects. Whether you are reviewing uncommitted changes or analyzing a complex commit history, an understanding of how to effectively use these resources is invaluable. Embracing these practices will make you a more effective collaborator and coder.
For those looking to deepen their knowledge, consider exploring additional resources on advanced Git commands and how they can optimize your workflow. Engage with the Git community and participatory forums to accelerate your learning curve and gain insights from experienced developers.