The `git diff` command with the `--ignore-space-change` option allows you to view differences in your code while ignoring changes in whitespace, making it easier to focus on actual content changes. Here's how to use it:
git diff --ignore-space-change
Understanding Git Diff
What is Git Diff?
The `git diff` command is an essential tool in Git that allows you to see the differences between various commits, branches, or even your working directory. By comparing changes, developers can review modifications, understand code evolution, and prepare for merges. Without `git diff`, developers would struggle to visualize updates, making collaboration significantly more challenging.
Basic Syntax of Git Diff
Understanding the basic syntax of the `git diff` command is crucial. The command follows this structure:
git diff [options] [<commit>] [--] [<path>...]
- `options`: These are flags that modify the behavior of the command, such as ignoring whitespace.
- `<commit>`: This is where you specify one or more commits for comparison.
- `<path>`: Represents specific files or directories you want to examine.
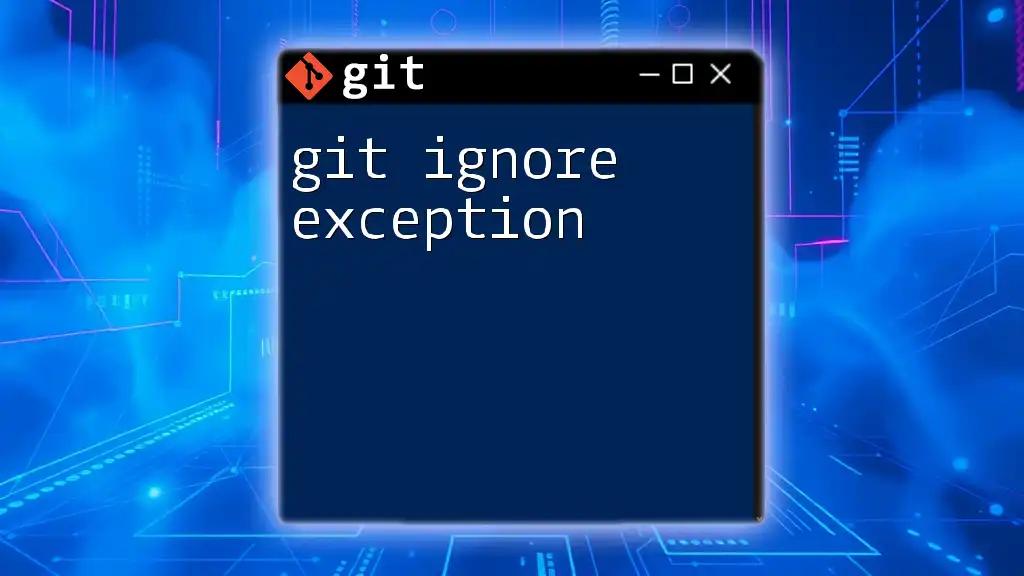
The Role of Whitespace in Git Diff
What is Whitespace?
Whitespace refers to any character that is used for spacing and formatting in your code, including spaces, tabs, and newlines. While it’s essential for readability and formatting, excessive or inconsistent whitespace can lead to confusion during code reviews, creating unnecessary diff changes that distract from significant updates.
Common Scenarios Where Whitespace Matters
In collaborative projects, different coding styles often emerge. For instance, one developer might use tabs for indentation, while another prefers spaces. When reviewing code changes or merging branches, inconsistencies in whitespace can result in lengthy and confusing diffs, making it difficult to discern the actual changes made. Ignoring these trivial changes becomes essential for clarity and efficiency.
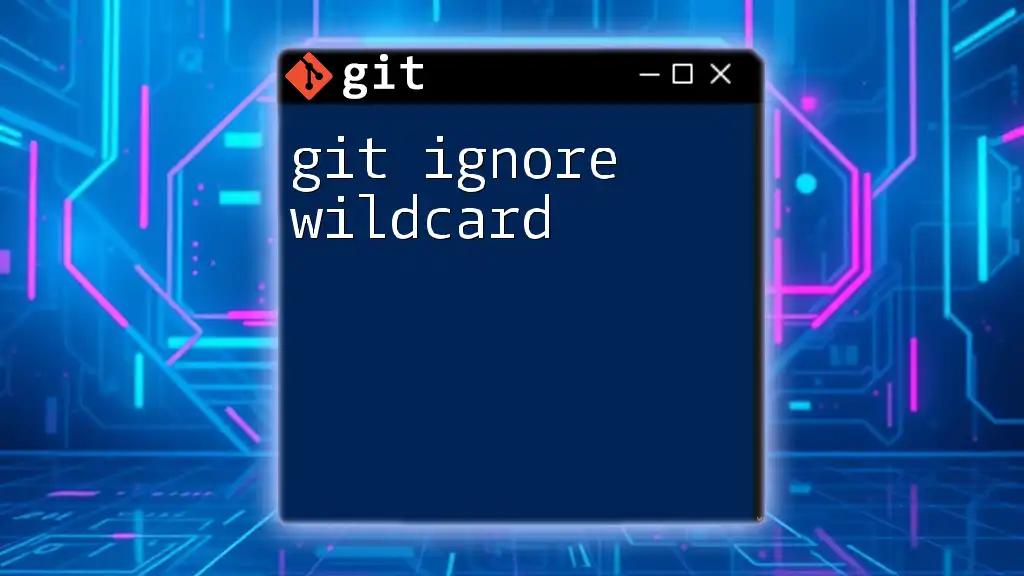
Ignoring Whitespace in Git Diff
Why Ignore Whitespace Changes?
Ignoring whitespace changes in Git can help focus on the core changes – the actual code logic. This approach is vital in several scenarios, especially when handling large patch files where formatting inconsistencies can bloat diffs, making it harder to review code accurately. By emphasizing real changes, developers can enhance productivity during code reviews and minimize the potential for conflict resolution headaches down the line.
Using the Ignore Whitespace Option
Git provides specific options to ignore whitespace changes while using `git diff`. Understanding and utilizing these options are key to effective code reviews:
- `-w`: This option tells Git to ignore all whitespace changes.
- `--ignore-all-space`: Similar to `-w`, this option ignores whitespace but has nuances in dealing with leading and trailing spaces.
Examples:
-
Ignoring Whitespace Globally To see differences without considering whitespace, you can run:
git diff -w
Explanation: This command outputs the differences between your working directory and the last committed version while completely ignoring whitespace changes.
-
Ignoring Whitespace During Specific Comparisons Want to compare the last commit with the one before it while ignoring whitespace for a particular file? Use:
git diff HEAD~1 HEAD -- path/to/file
Explanation: This command compares the last two commits and shows differences for a specific file, neglecting any whitespace modifications.
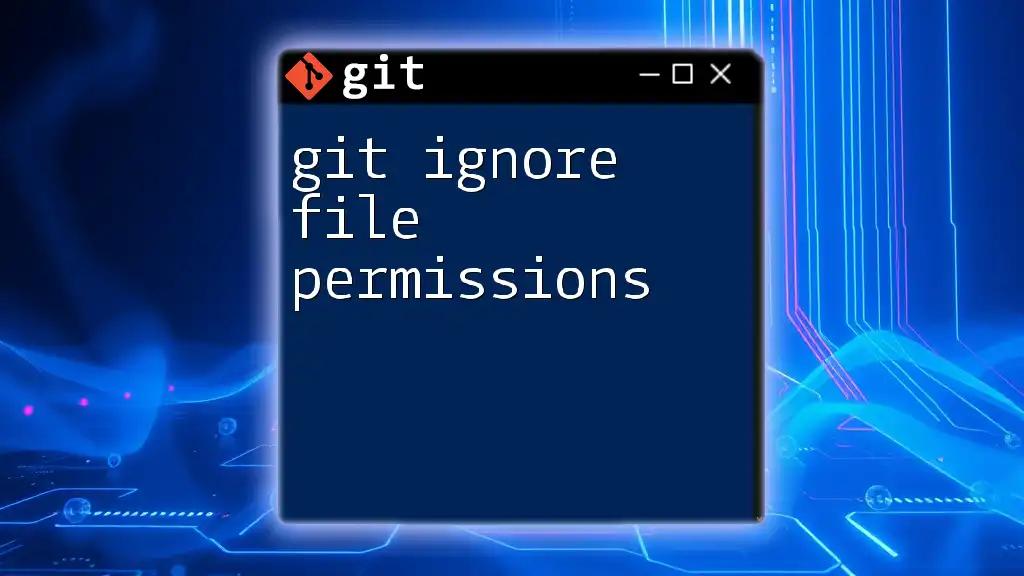
Practical Scenarios for Using Git Diff with Ignoring Whitespace
Reviewing Code Changes Before Merging
Before merging branches, it's essential to review the changes made. To prepare for a code review while ignoring whitespace changes, you can run:
git diff -w branch-to-merge
Explanation: This command allows you to see what changes will be brought in by the specific branch, focusing solely on substantive code changes rather than formatting variances, making the review process more efficient.
Collaboration Between Different Coding Styles
Development teams often comprise programmers with varied formatting preferences. To foster clearer communication and understanding of actual code changes, using `git diff` with whitespace ignoring options is highly beneficial. For example, by running:
git diff --ignore-all-space
you can quickly identify the core modifications in a mixed-format environment.
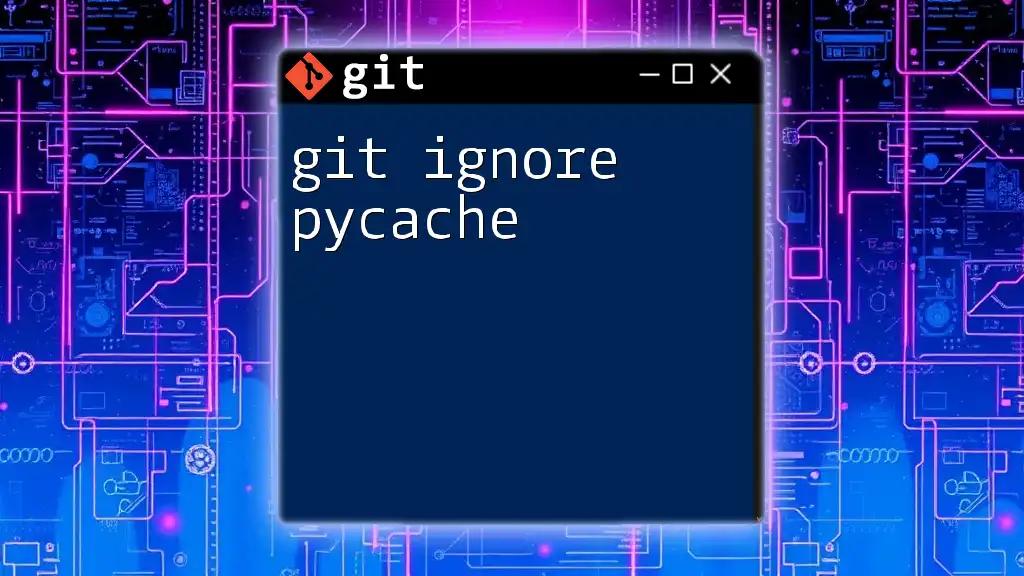
Advanced Usage of Git Diff Ignoring Whitespace
Combining with Other Options
To maximize the utility of the `git diff` command, certain options can be combined with the whitespace ignore feature. For instance, if you want to only see staged files while ignoring whitespace, you can use:
git diff -w --cached
Explanation: This command shows differences in the staged files while ignoring any whitespace changes, providing a clearer picture of what has been prepared for the next commit.
Another useful command is:
git diff -w --name-only
Explanation: This shows only the names of the files that have been modified, excluding any whitespace discrepancies, which is perfect for quick evaluations.
Configuring Git for Default Whitespace Handling
If you often find yourself needing to ignore whitespace changes, you can set up your global Git configuration. Modify your `.gitconfig` file to include:
[diff]
whitespace = fix,-indent-with-non-tab
Explanation: This setting ensures that your Git diffs will consistently ignore certain whitespace changes. It provides a cleaner history and helps maintain focus on crucial changes.
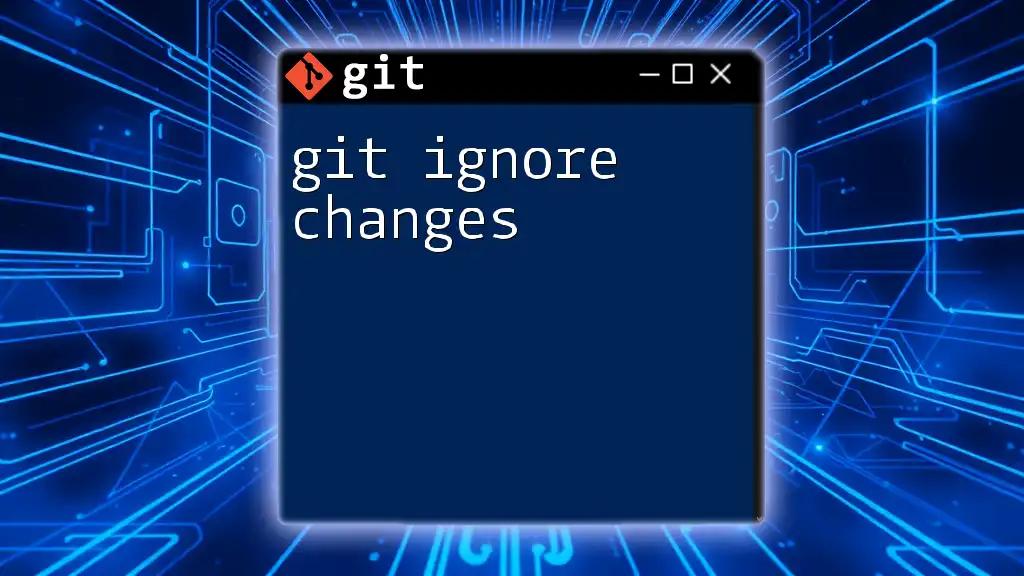
Conclusion
Recap of Key Points
Understanding how to use `git diff ignore whitespace` commands is essential for efficient code reviews and collaboration. By focusing on substantive changes rather than formatting discrepancies, developers can enhance productivity, foster clearer communication, and streamline the development process.
Next Steps for Learning More About Git
To deepen your knowledge of Git and improve your programming workflows, explore additional resources, courses, or articles. Each step will help you become more adept at using Git to its fullest potential and enhance your overall coding experience.
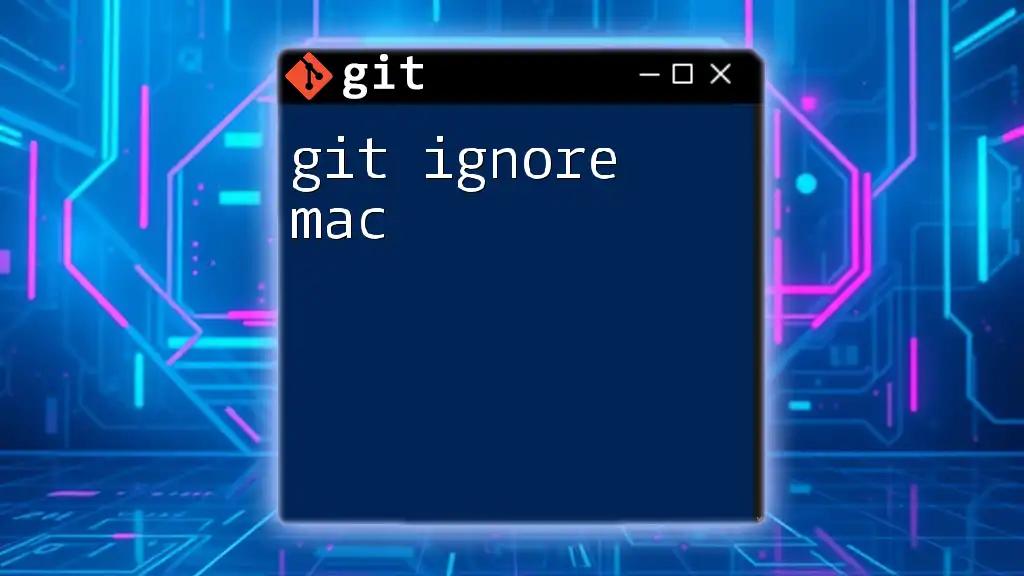
Frequently Asked Questions (FAQs)
What happens if I don't ignore whitespace changes?
Failing to ignore whitespace changes can lead to complex diffs that obscure the real modifications, complicating the review process and potentially causing confusion among team members.
Can I ignore only some whitespace?
While Git allows you to ignore all whitespace changes, more granular control is limited. The options primarily available focus on all whitespace. Custom scripts may be needed for specific scenarios.
How does ignoring whitespace affect merge conflicts?
Ignoring whitespace can minimize merge conflicts related to formatting differences, allowing developers to concentrate on substantive code changes and enhance the clarity of their revisions.