To display a list of ignored files in a Git repository, you can use the following command:
git check-ignore -v *
Understanding Ignored Files
What are Ignored Files?
Ignored files are those that Git will not track or include in the versioning process. This feature is particularly important for components such as temporary files, compiled code, and logs. These files may change frequently and do not hold valuable historical significance, making it unnecessary to clutter the repository with them.
Common Use Cases for ignored files include:
- Build artifacts: Files produced by build systems like `dist`, `out`, or `bin`.
- Editor/user-specific files: Settings or files generated by code editors like IDE configuration files or archives (for example, `.idea` directories from JetBrains products).
- Logs and dump files: Any output generated during operations that might be useful for debugging but not for version tracking.
The Importance of `.gitignore`
The `.gitignore` file serves as a guide for Git on which files to ignore. It contains a list of rules and patterns that define which files are excluded from tracking. Properly configuring your `.gitignore` is essential to ensure that irrelevant files don't clutter your version control.
To create a `.gitignore` file, place it in the root directory of your Git repository. The basic structure includes patterns that match the files or directories you wish to ignore. Here’s a simple example of a `.gitignore` file:
# Ignore node modules
node_modules/
# Ignore log files
*.log
# Ignore IDE configuration
.idea/
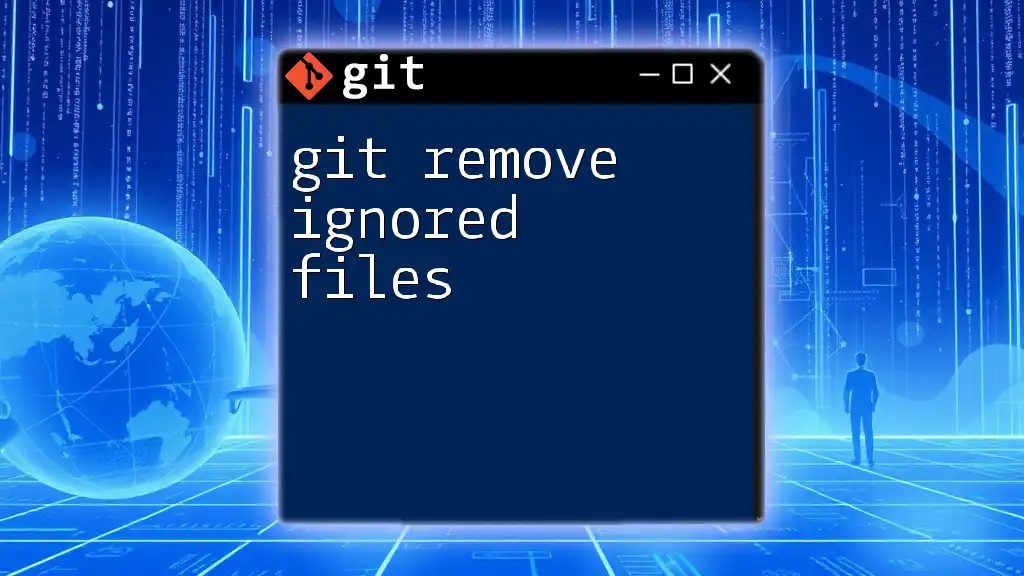
Viewing Ignored Files in Git
Using the `git check-ignore` Command
The `git check-ignore` command is a powerful tool that allows users to check whether specific files are ignored based on the rules defined in their `.gitignore`.
Basic Usage
git check-ignore -v <file>
Here, the `-v` flag provides a verbose output that shows you exactly which rule in the `.gitignore` is causing the file to be ignored.
Example
To check if a specific file, like `my-file.txt`, is ignored, you can use the command:
git check-ignore -v my-file.txt
If `my-file.txt` is ignored, the output will indicate the rule that applied to it.
Listing All Ignored Files
To see a comprehensive list of all files that are currently ignored in your repository, you can utilize the `git ls-files` command with relevant options.
git ls-files --ignored --exclude-standard
- `--ignored`: Tells Git to list ignored files.
- `--exclude-standard`: Ensures that standard ignore rules (from `.gitignore`, system-wide ignore, etc.) are also applied.
This command provides a straightforward way to pull an overview of all files being ignored in your repository.
Combining with Other Git Commands
Viewing ignored files can also be combined with other Git commands for effective management of your project.
Example: Showing Ignored Files and File Status
You can list the status of files along with which ones are ignored by using:
git status --ignored
This command displays the state of the repository, including tracked, untracked, and ignored files, allowing for a comprehensive view at a glance.
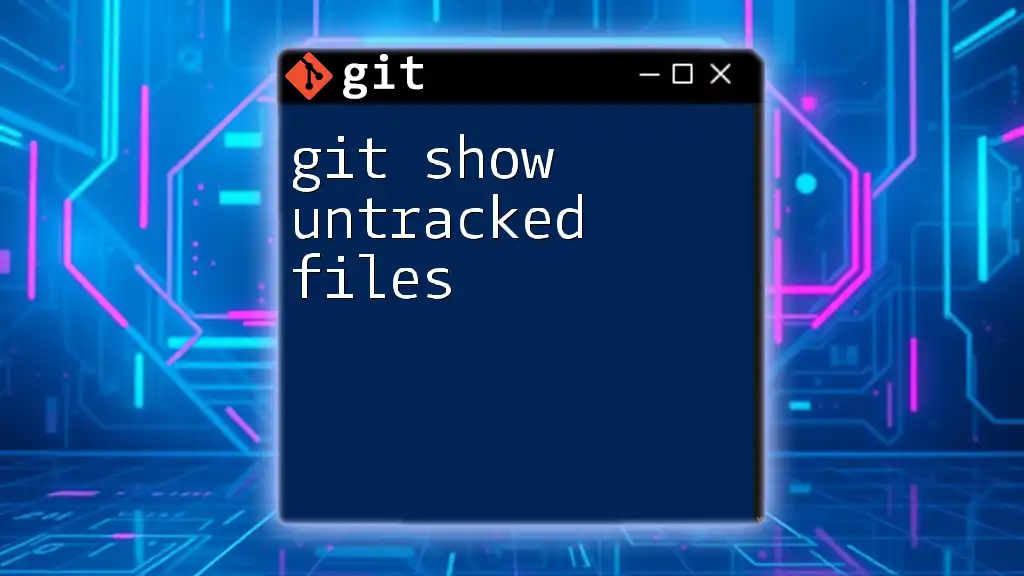
Troubleshooting Common Issues
Why Can't I See Ignored Files?
When users try to view ignored files and do not see what they expect, it can often stem from misunderstandings about Git's ignore rules. It's essential to ensure that the correct patterns are defined in `.gitignore`.
Common Mistakes in `.gitignore`
A frequent issue is incorrect syntax in the `.gitignore` file which can lead to files not being ignored as intended. Patterns in `.gitignore` must be properly formatted:
- Ensure no leading spaces exist.
- Check that wildcards and negation patterns (`!`) are correctly applied.
Ignored Files Not Showing As Expected
If ignored files are not displaying as anticipated, debugging the `.gitignore` content is key. You can search through the contents of your `.gitignore` and analyze which patterns might conflict or could be too broad.
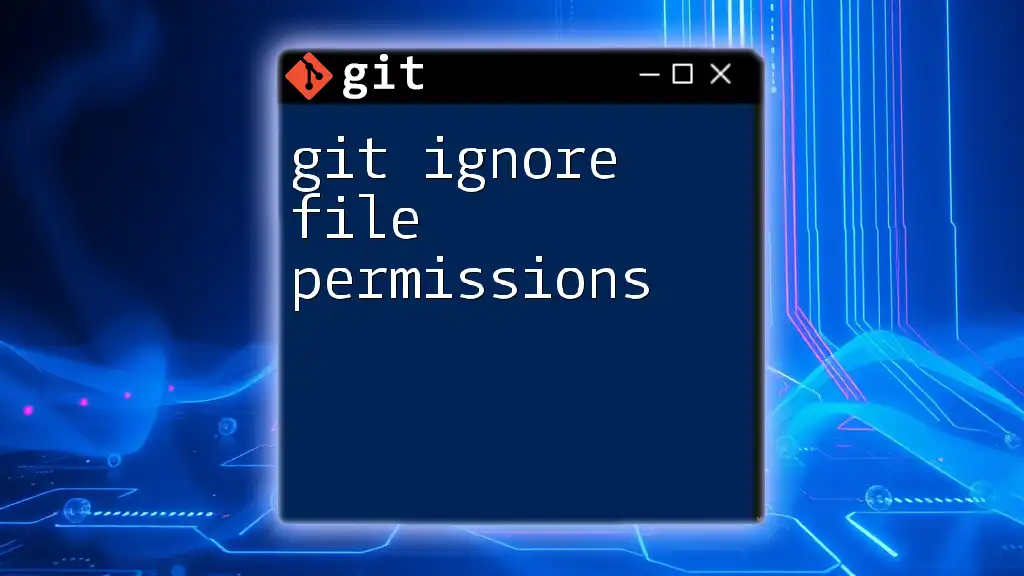
Best Practices for Managing Ignored Files
Maintaining an Effective `.gitignore`
To maintain a clean repository, consistently reviewing and updating your `.gitignore` is vital. Over time, the needs of your project may change, and files that were previously necessary may no longer need to be tracked.
Here are strategies to keep your `.gitignore` effective:
- Document new additions: Whenever new files or patterns are added, provide comments to clarify their purpose.
- Periodically evaluate what you’re ignoring: Check whether files in `.gitignore` are still relevant.
Regularly Reviewing Ignored Files
Why You Should Revisit Your Ignored Files: As a project evolves, it’s crucial to regularly assess ignored files. Files that were once seen as temporary or non-essential may suddenly become necessary, or vice versa.
Endeavor to revisit your `.gitignore` every few iterations of your project to ensure it continues to serve its intended purpose.
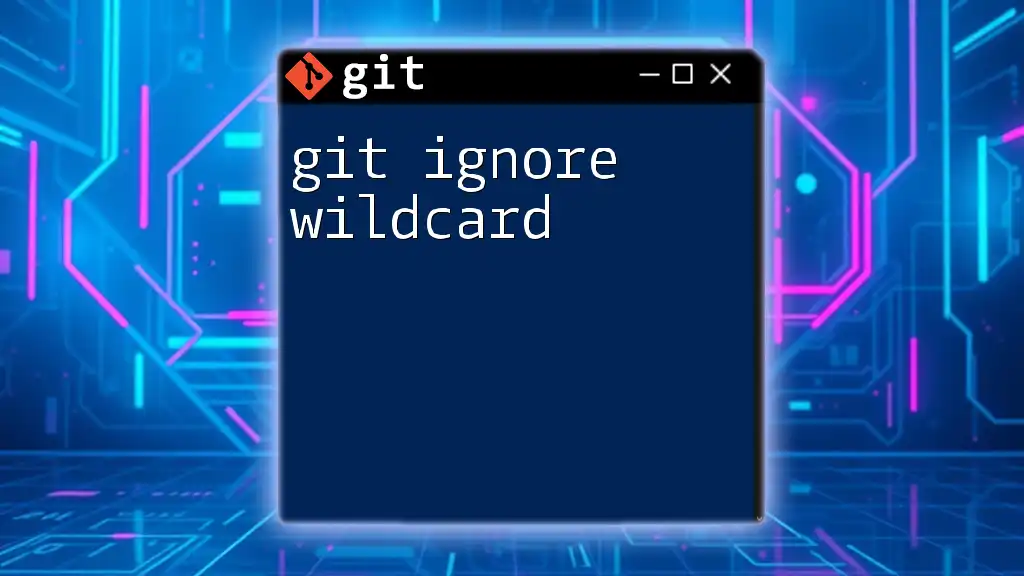
Conclusion
Understanding how to effectively use the `git show ignored files` commands empowers users to manage their repositories more efficiently. By integrating practices like maintaining a solid `.gitignore` file and leveraging commands like `git check-ignore` and `git ls-files`, you can ensure that your version control retains clarity and relevance.
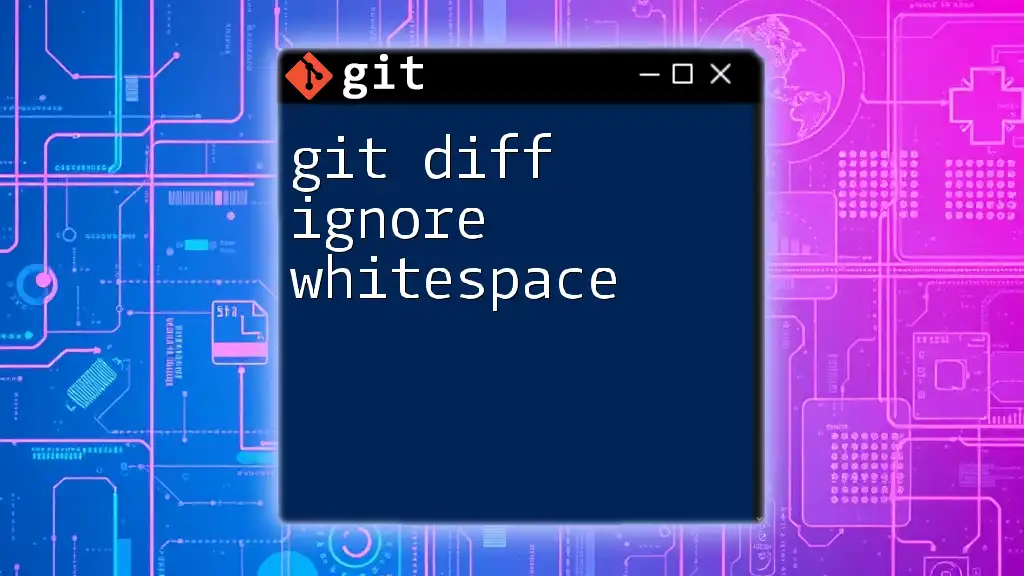
Further Reading and Resources
For those wishing to delve deeper into Git, consider exploring the official Git documentation, as well as reputable blogs and tutorials that elaborate on Git practices and advanced commands.