The `git diff` command allows you to compare the differences between two branches, showing the changes that have been made in one branch compared to another.
git diff branchA..branchB
Understanding Git Branches
What are Git Branches?
In Git, a branch is essentially a pointer to a specific commit in your repository. It allows you to develop features, fix bugs, or experiment without affecting the main production codebase. Think of branches like different paths in a project; they enable you to work concurrently on various features.
Common Branching Strategies
Developers often adopt different branching strategies based on their team’s workflow and project requirements. Some popular strategies include:
- Feature Branching: Each feature is developed in its own branch, allowing teams to focus on one task at a time.
- Git Flow: A more structured approach that uses multiple branches for development, staging, and release.
Understanding the differences between these branches is crucial, especially when multiple team members contribute to the same project. This is where the git diff between branches becomes very valuable.
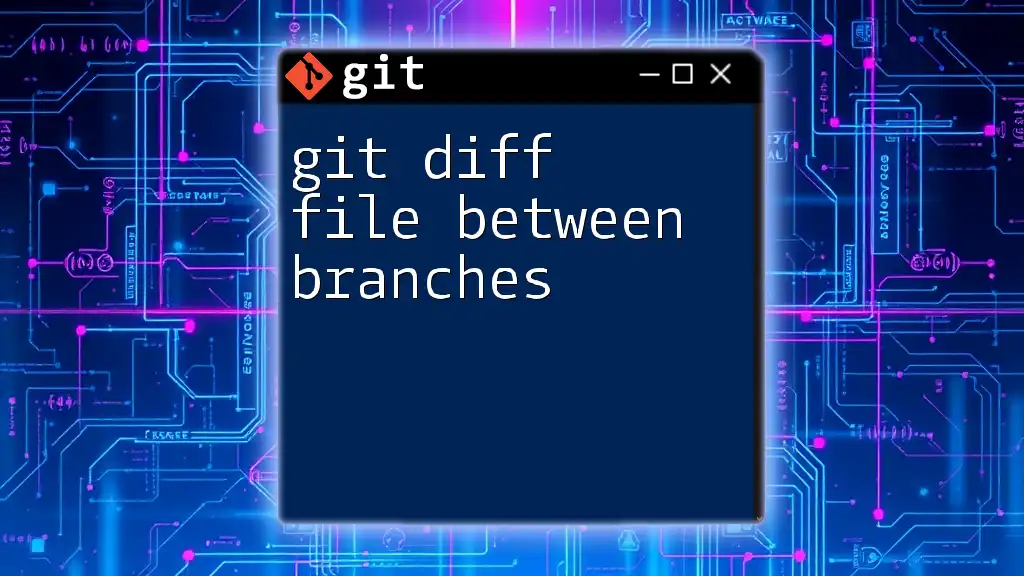
The Role of `git diff`
What Does `git diff` Do?
The `git diff` command is a powerful tool that shows the changes between commits, branches, files, and even the working directory. It allows developers to see what has changed between different points in the repository, providing vital insights before merging or making further adjustments.
Syntax and Basic Usage
The general syntax for the `git diff` command is as follows:
git diff <options> <commit1> <commit2>
Here, <commit1> and <commit2> can be specific branch names, tags, or commit hashes. This versatility makes `git diff` an essential command in your Git toolkit.
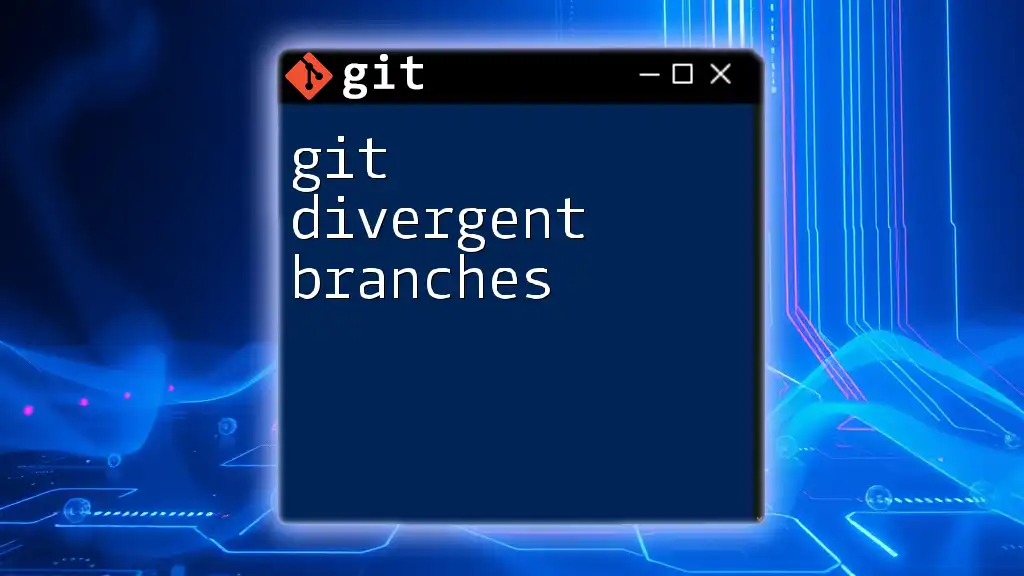
Comparing Branches with `git diff`
How to Compare Two Branches
To compare two branches directly using `git diff`, simply run the following command in your terminal:
git diff branch1 branch2
Replace branch1 and branch2 with the names of the branches you want to compare. The output will display the differences between the two, highlighting which lines have been added or removed in `branch2` compared to `branch1`.
Understanding the Output
The output of the `git diff` command has a specific structure that helps you interpret changes easily:
- Added lines are prefixed with a `+`, indicating lines present in the second branch that are not in the first.
- Removed lines display a `-`, showing what has been deleted in the second branch.
- Context lines are shown without any signs, providing you with a frame of reference.
Example: Comparing Feature and Main Branch
Imagine you're developing a new feature in a branch called feature-branch while the main code is in main. You can compare these two branches with:
git diff feature-branch main
This command allows you to identify any changes you've made in the `feature-branch` that are not yet integrated into `main`. Understanding these differences can help prevent conflicts when merging.
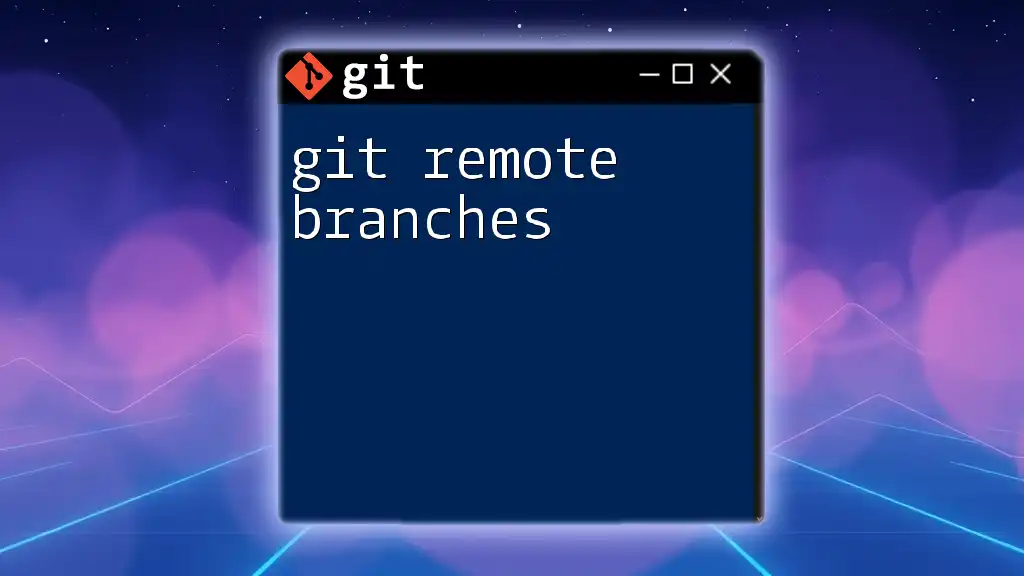
Options to Enhance `git diff`
Useful Flags and Their Applications
To maximize the utility of the `git diff` command, several options can be utilized:
- `--stat`: Displays a summary of changes, showing how many insertions and deletions occurred. You can run:
git diff --stat branch1 branch2
This concise overview is particularly useful for getting a quick snapshot of changes before diving into the detailed `git diff`.
- `--color`: Compels Git to use color coding, making it easier to distinguish between additions and removals. The command looks like this:
git diff --color branch1 branch2
- `--name-only` vs `--name-status`: Both options list the files that have changed, but they differ in their details. Use:
git diff --name-only branch1 branch2
To get just the file names, or:
git diff --name-status branch1 branch2
To see the status of each file, indicating whether it was added, modified, or deleted.
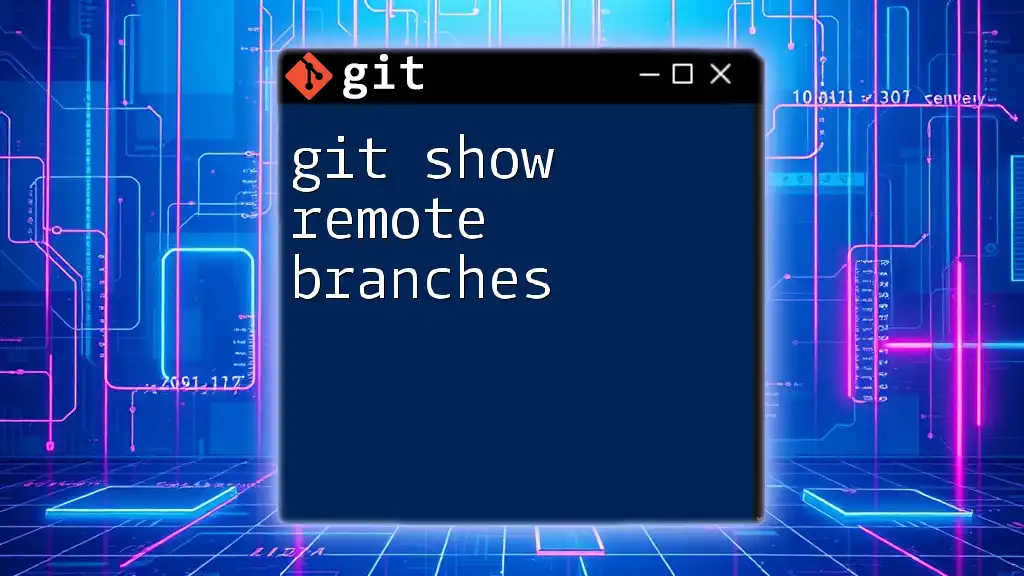
Visualizing Differences Graphically
Using Git GUI Tools
In addition to command-line tools, several Git GUI tools can help visualize differences between branches. Tools like GitKraken and Sourcetree provide a graphical representation of changes, making it easier to interpret differences without extensive command line interaction.
These tools can often display diffs in a side-by-side format, simplifying the review process, especially for visual learners.
Integrating VSCode for Git Diff
If you use Visual Studio Code, it has built-in support for viewing diffs. You can easily compare branches directly within the application. Simply right-click on the branch you want to compare and choose "Compare with..." to view the changes visually.
Additionally, there are extensions available that can further enhance your diff viewing experience, allowing you to customize how changes are displayed.
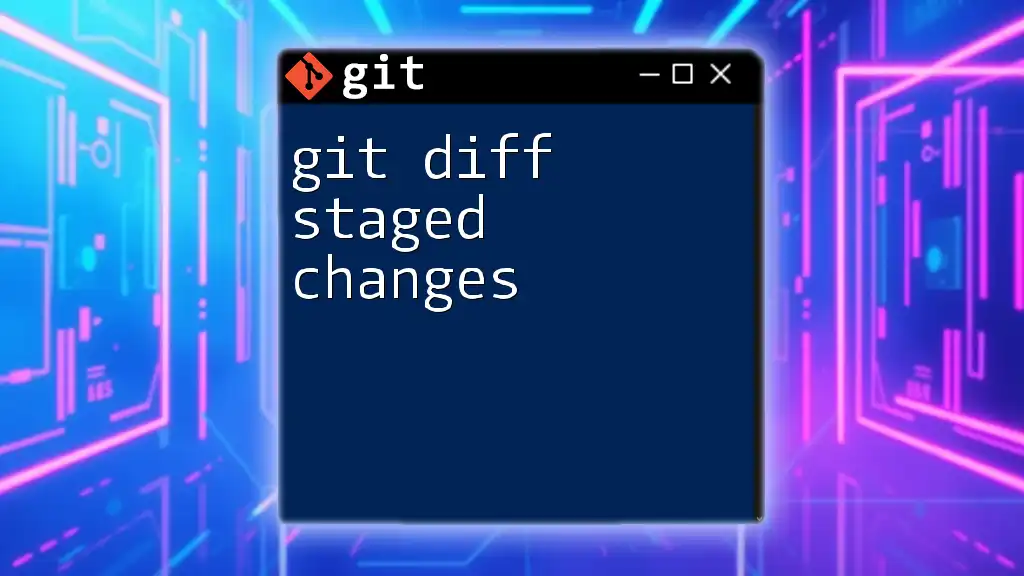
Best Practices for Using `git diff`
Regularly Compare Branches
It’s a good practice to frequently check for differences between branches during development. Not only does this help in identifying unmerged changes, but it also mitigates potential merge conflicts, ensuring smoother integration later on.
Incorporate Code Review with `git diff`
Utilizing `git diff` as part of your code review process can significantly enhance code quality. It allows team members to discuss and review changes before they are merged, fostering collaboration and ensuring that every change aligns with the project’s standards.
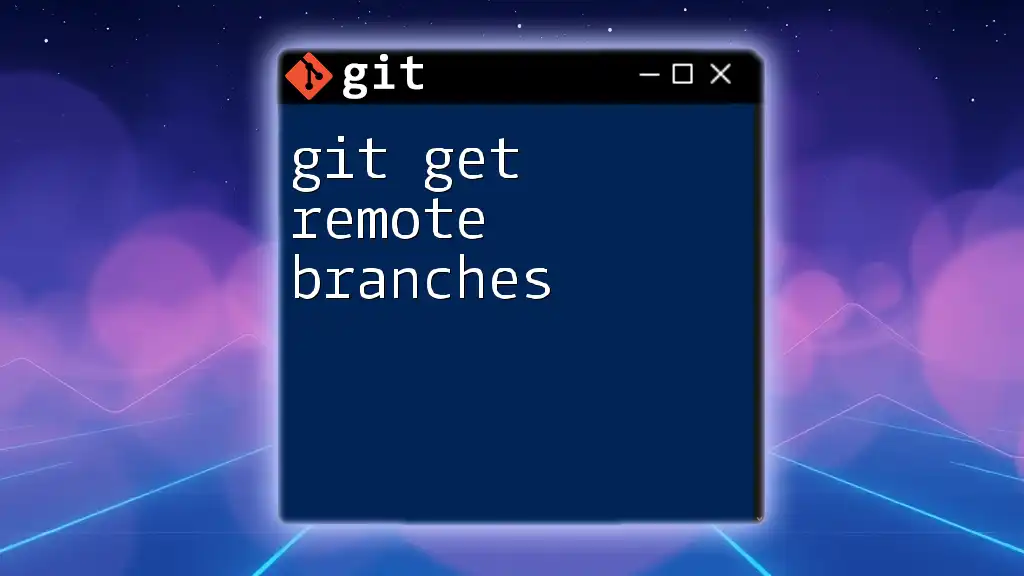
Conclusion
Understanding the nuances of `git diff between branches` is crucial for anyone involved in software development using Git. By mastering this command and knowing how to interpret its output, you empower yourself to work more effectively within team dynamics, prevent merge conflicts, and maintain high code quality. With practice, these skills will become an invaluable part of your development toolkit.
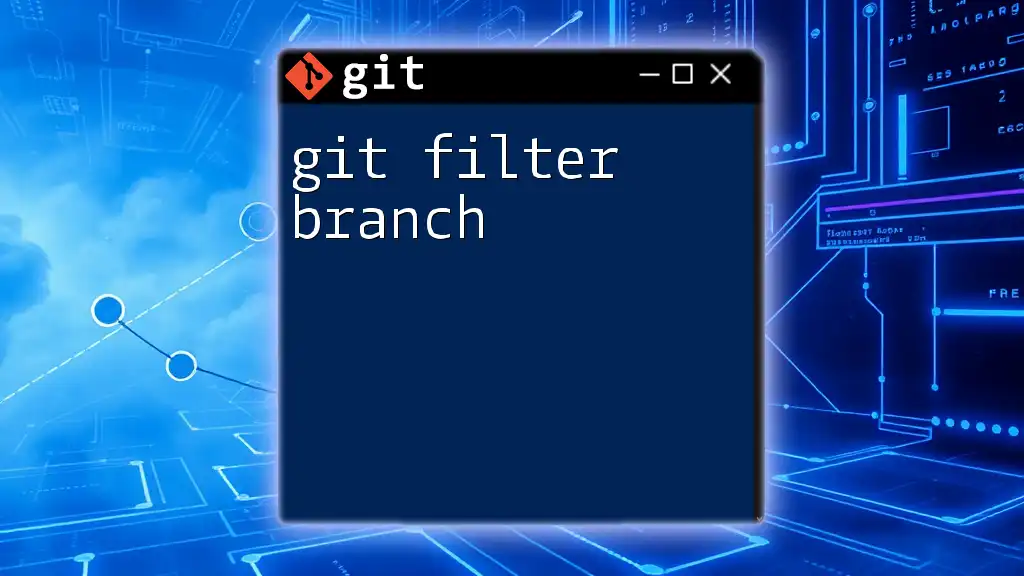
Additional Resources
For further learning, I recommend checking out the official Git documentation, which offers in-depth explanations of all Git commands, including `git diff`. Additionally, consider exploring online tutorials, courses, or even local meetups to deepen your understanding of Git and version control. Don't hesitate to reach out for assistance or clarification on any concepts!