Switching Git branches allows you to navigate between different lines of development in your project, and can be done using the command:
git checkout <branch-name>
Understanding Git Branches
What is a Git Branch?
A branch in Git represents an independent line of development. You can think of branches as different versions or "paths" of your repository. They allow multiple team members to work on features or fixes simultaneously without interfering with each other's work. Utilizing branches helps to keep your codebase organized and manageable, especially in projects that require parallel development efforts.
How Git Branches Work
In a typical Git workflow, there is a base branch (often called `main` or `master`), which contains the stable version of your code. When you create a new branch, Git essentially makes a copy of this base branch. Any changes you make on the new branch are isolated, meaning they won’t affect the main branch until you're ready to merge them back in. This structure allows for greater flexibility and safer experimentation.
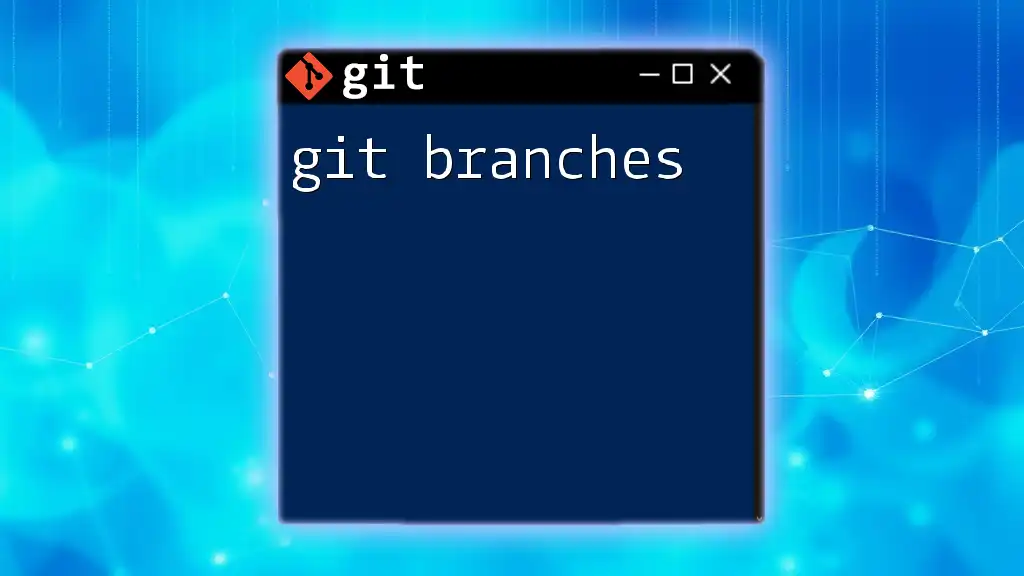
Commands for Switching Git Branches
Checking Current Branch
To identify which branch you are currently on, you can use the following command:
git branch
When executed, this command will list all branches in your repository and highlight the one you are currently working on with an asterisk (*). This is essential to keep track of your current focus and avoid confusion when working on multiple branches simultaneously.
Switching to an Existing Branch
To switch to an existing branch, you will use the following command:
git checkout <branch-name>
When you run this command, Git updates your working directory to match the specified branch. It's important to ensure that any changes you have made are either committed or stashed, as switching branches with uncommitted changes may lead to conflicts or complications. For example, if you're developing a feature on the `feature/new-login` branch and need to switch back to `main`, simply execute:
git checkout main
This action will load the state of the `main` branch, allowing you to continue work or review other changes.
Using Newer Commands: `git switch`
As Git continues to evolve, it has introduced more straightforward commands for common tasks. One such command for switching branches is `git switch`:
git switch <branch-name>
This command serves the same purpose as `git checkout` but is specifically designed to clarify your intention to switch branches. It's recommended to use `git switch` as it is more intuitive and helps to avoid confusion with other functionalities of `git checkout`, such as file operations.
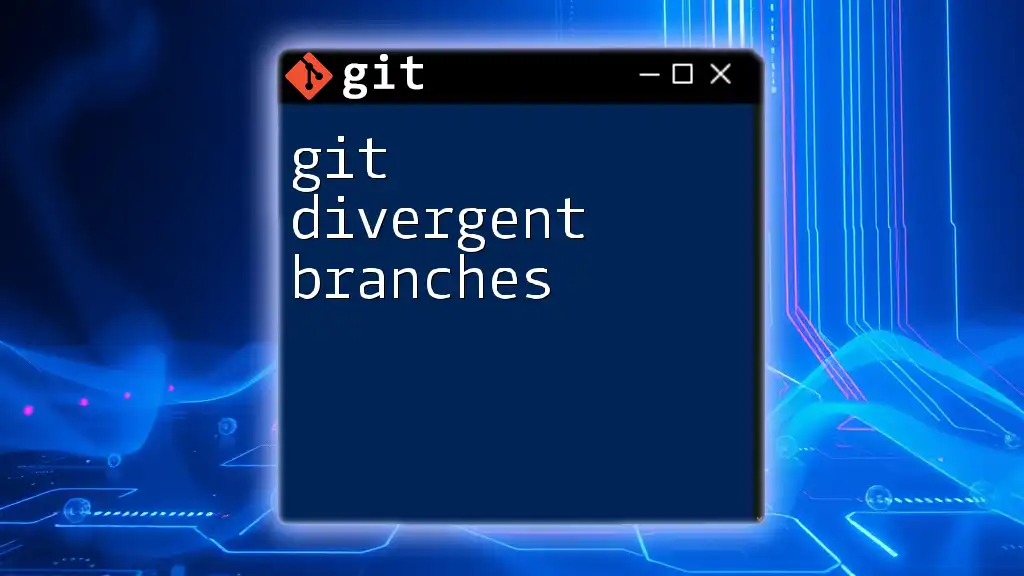
Creating and Switching to a New Branch
Creating a Branch and Switching in One Command
If you want to create a new branch and immediately switch to it, you can use the following command:
git checkout -b <new-branch-name>
This command accomplishes two tasks: it creates the specified branch and then checks it out immediately, allowing you to start working on it right away. For example, to create and switch to a branch for a new login feature, run:
git checkout -b feature/new-login
Best Practices for Naming Branches
When creating branches, it’s crucial to name them in a meaningful way. Clear naming conventions contribute to better collaboration and understanding among team members. Consider incorporating the following guidelines for branch naming:
- Use descriptive names: For example, `feature/add-user-authentication` clearly conveys what the branch aims to capture.
- Prefix branch names by type: For instance, use `feature/`, `bugfix/`, or `hotfix/` to categorize branches quickly.
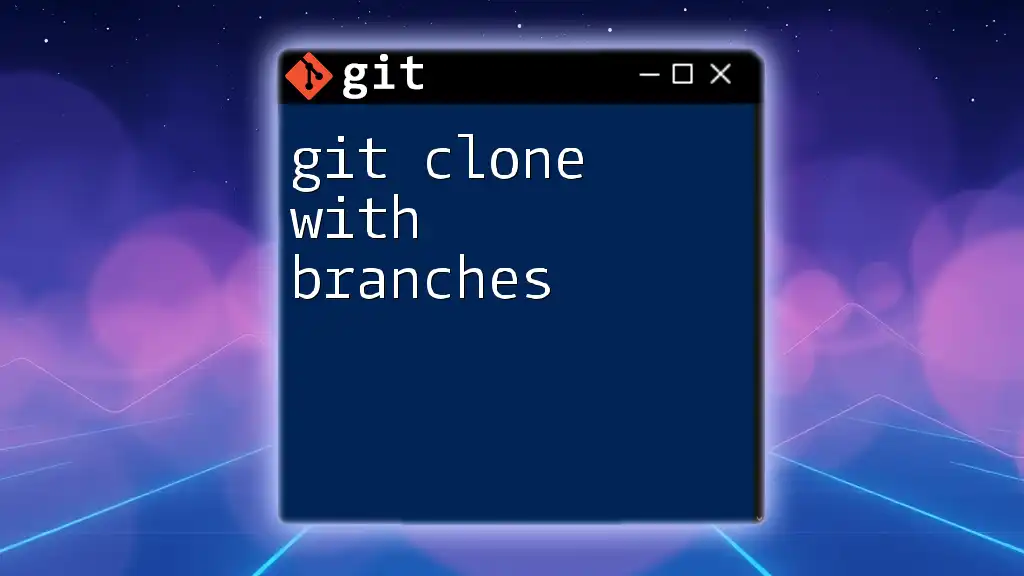
Resolving Potential Issues When Switching Branches
Uncommitted Changes
If you attempt to switch branches while there are uncommitted changes in your working directory, Git may block the operation to prevent potential data loss or conflicts. Before switching, it’s advisable to either:
-
Commit your changes: This can be done using:
git commit -m "Your commit message"
-
Stash your changes: If you're not ready to commit, you can save your uncommitted changes temporarily with:
git stash
After stashing, you can switch branches freely. To retrieve your stashed changes later, use:
git stash pop
Conflicts When Switching
Branch conflicts can arise if two branches have diverged and contain competing changes. To resolve conflicts, follow these steps:
- Switch to the branch you want to merge into.
- Merge the conflicting branch:
git merge <conflicting-branch-name>
- Manually resolve any conflicts in the affected files, commit those resolutions, and finalize the merge.
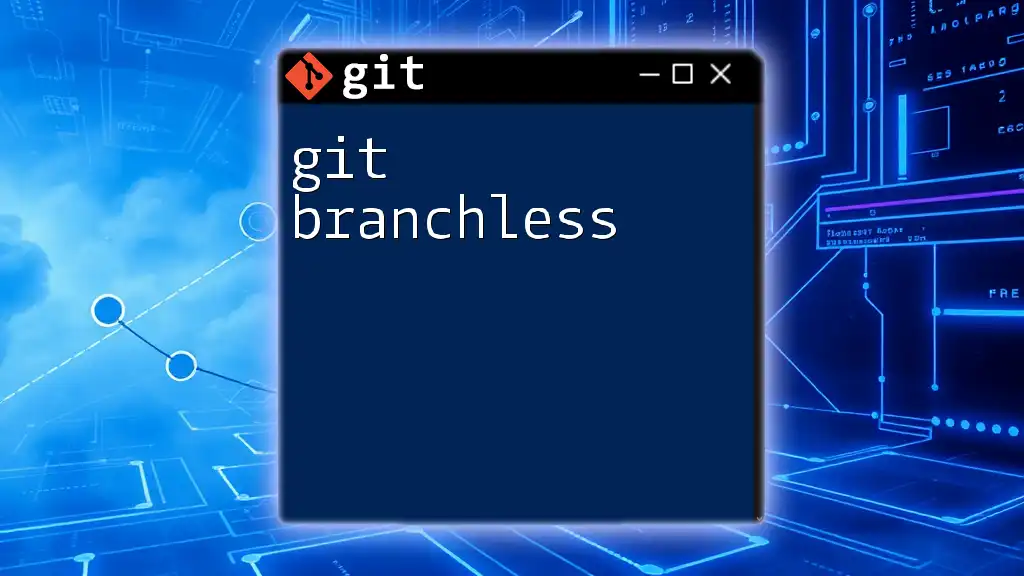
Additional Tips for Branch Management
Merging vs. Switching
It’s essential to differentiate between merging branches and switching branches. While switching is simply about changing your current focus, merging involves combining changes from one branch into another. Use merging when you want to incorporate updates from a feature branch back into your main branch or another relevant branch.
Deleting a Branch After Use
After you’ve successfully merged changes from a branch, it’s often a good practice to delete the branch to maintain a clean and organized repository. You can do this using:
git branch -d <branch-name>
The `-d` flag ensures that Git won't delete branches that have not been merged to prevent data loss. If you are sure you want to delete a branch regardless of its merge status, you can use `-D` instead, but do so with caution.
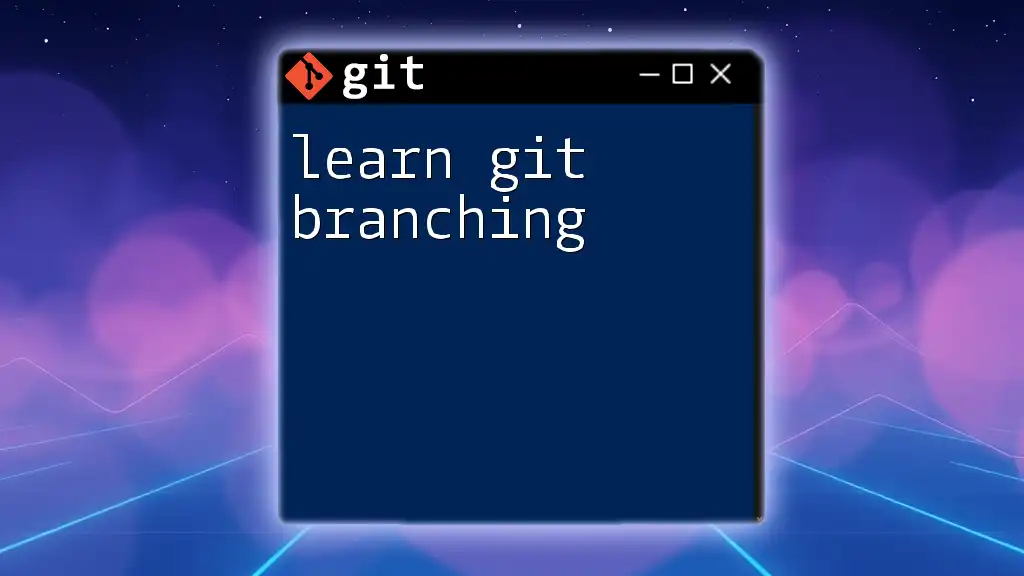
Conclusion
Mastering the process of switching Git branches is paramount for any developer looking to maintain a streamlined workflow. Regular practice will help you feel more comfortable navigating through branches, ensuring that your code remains organized and your collaborative efforts continue to flourish.
By implementing the discussed commands and best practices, you'll be well-equipped to manage branches effectively and contribute positively to any development project. As you continue your learning journey with Git, consider exploring further articles and tutorials that delve deeper into more advanced concepts and commands related to version control.
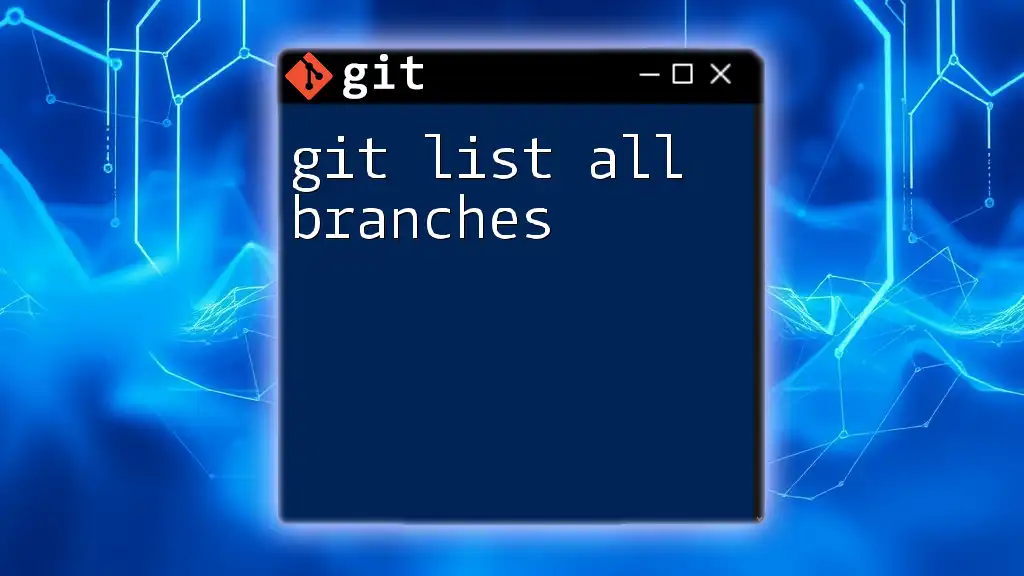
Additional Resources
For continued learning, explore the official Git documentation for comprehensive guidelines and extensive command lists. Additionally, consider utilizing graphical interfaces for Git, such as GitHub Desktop or SourceTree, to simplify the management of repos and branches visually. Please find suggested tutorials and resources to solidify your understanding of Git workflows, tips, and best practices.