The `git track` command is used to set up a new branch to track a remote branch, enabling you to easily synchronize changes between your local and remote repositories.
Here's a code snippet demonstrating how to track a remote branch:
git checkout -b my-branch origin/remote-branch
What is Git Tracking?
Git tracking refers to the way Git monitors changes to files in your repository, enabling developers to maintain a historical record and collaborate efficiently. When you work with Git, every modification you make can be tracked, allowing you to understand the evolution of your codebase over time.
Importance of Git Tracking
Understanding git track and its associated commands is critical for effective version control. By tracking changes, you can easily see what modifications have been made, identify who made these changes, and revert to previous versions if needed. This capability is vital in both team environments and solo projects, enabling better collaboration and reducing the risk of lost work.
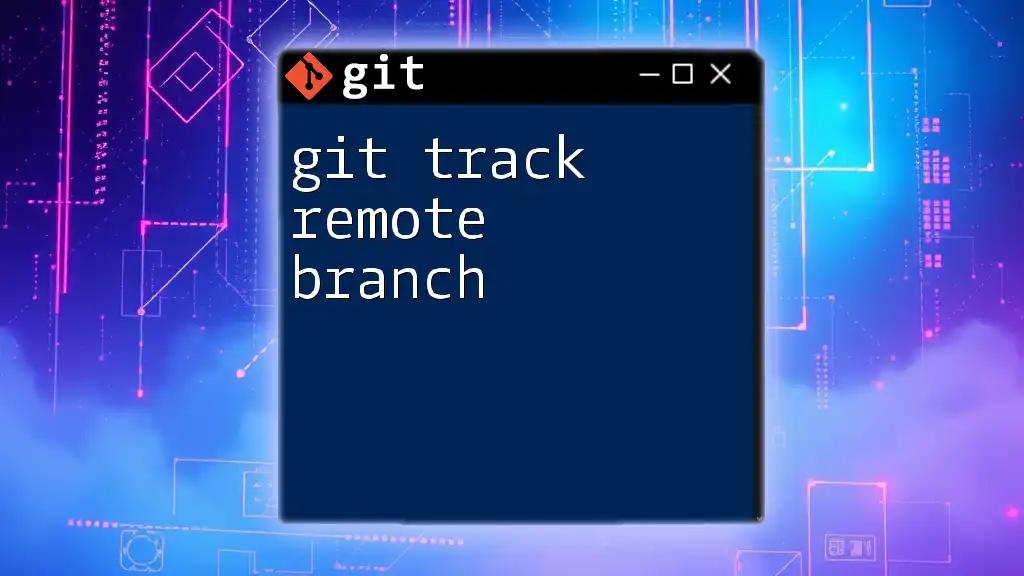
Understanding Git's Tracking Capabilities
The Basics of Tracking
In Git, files can be classified as either tracked or untracked.
- Untracked files are those that haven't been staged or committed to the repository. They might be new files created in your project directory but not included in version control yet.
- Tracked files, on the other hand, are files that Git is monitoring for changes. They can exist in three states: unmodified, modified, or staged. Understanding these states is crucial for effective use of Git.
Types of Tracking in Git
Untracked Files
Untracked files are those that Git does not recognize because they haven't been added to version control. For instance, when you create a new file in your project, you must explicitly tell Git to track it:
touch newfile.txt
git status
You'll see `newfile.txt` listed as an untracked file, indicating it's not yet included in version control.
Tracked Files
Tracked files exist in one of the three stages: unmodified, modified, and staged.
- Unmodified: These files have not changed since the last commit.
- Modified: Changes have been made to the file, but they haven't yet been staged.
- Staged: Changes that are ready to be committed.
Here's an example that showcases the transition between these states:
echo "Initial content" > myfile.txt
git add myfile.txt # myfile.txt is now staged
echo "More content" >> myfile.txt # myfile.txt is now modified
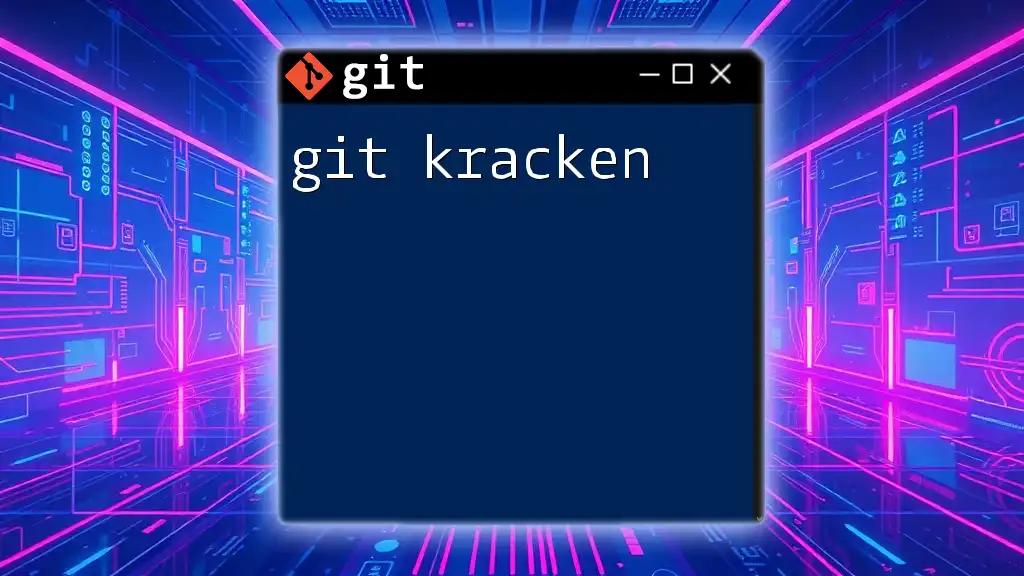
The `git track` Command Deep Dive
Overview of `git track`
While `git track` isn’t a direct command in Git, it embodies the concept of how Git monitors and handles changes to your project files. Understanding how to manage file tracking is crucial.
Common Git Commands for Tracking
`git add`
The `git add` command is essential for tracking changes you want to include in your next commit. It marks modified files to be added to the staging area, effectively telling Git, "I want to track these changes."
Example of using `git add`:
git add <file-name>
If you want to add all modified files at once, use:
git add .
`git status`
To see which files are tracked, untracked, staged, or modified, you can use the `git status` command. This command provides helpful information about the state of your working directory.
Example output might look like this:
On branch main
Your branch is up to date with 'origin/main'.
Changes not staged for commit:
(use "git add <file>..." to update what will be committed)
modified: myfile.txt
Untracked files:
(use "git add <file>..." to include in what will be committed)
newfile.txt
`git diff`
Before adding files to the staging area, you might want to review the changes you've made. The `git diff` command shows the differences between your modified files and the last committed version.
Example of `git diff`:
git diff
This will display all unstaged changes in your currently tracked files.
`git commit`
The `git commit` command finalizes the changes you've made and recorded in the staging area. You can add a message for the commit to describe what this change entails.
Example syntax for committing changes:
git commit -m "Your informative commit message"
This action effectively saves the state of tracked files in the repository.
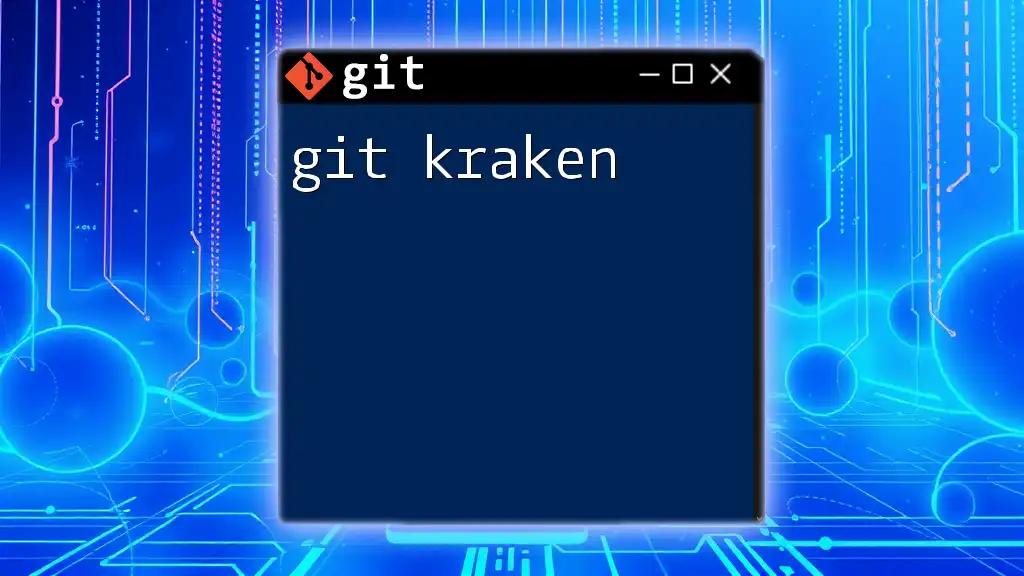
Tracking Changes in a Project
Starting a New Git Repository
To start tracking changes in a new project, you first need to initialize a Git repository. This process is straightforward:
git init
This command creates a new .git directory in your project folder, effectively enabling version control.
Adding and Committing a File
Once your repository is initialized, you can add files for tracking. Here’s a simple demonstration:
echo "Hello, Git!" > hello.txt
git add hello.txt
git commit -m "Add hello.txt"
This sequence creates a new file called `hello.txt`, stages it, and commits it with a descriptive message.
Modifying Tracked Files
Once you have tracked files, updating them and committing those changes is simple. Here’s how you can modify a tracked file:
echo "Hello, World!" >> hello.txt
git add hello.txt
git commit -m "Update hello.txt with greeting"
Here, you append content to `hello.txt`, stage that change, and commit it, tracking your project’s evolution.
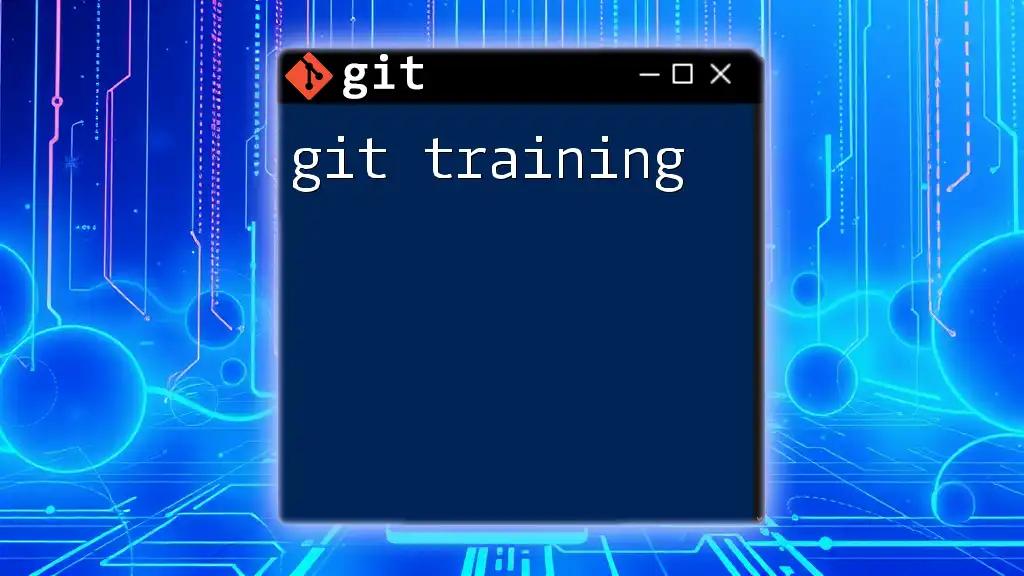
Best Practices for Tracking Changes in Git
Consistent Commit Messages
Maintaining a standardized approach to commit messages benefits anyone interacting with the repository. Aim for clarity and conciseness. For example:
- Good: "Fix button alignment issue on home page"
- Bad: "Fixed stuff"
Having clear commit messages makes it easier for team members (or your future self) to understand the project's timeline.
Regularly Checking Status
Adopting a habit of regularly checking your Git status with:
git status
can help you stay on top of file changes and ensure you're aware of what is tracked and what isn't, reducing the risk of missed commits or untracked files.
Using Branching Strategically
Taking advantage of Git's branching allows you to track changes separately from the main codebase, which is especially useful for feature development or bug fixes. To create and switch branches, use:
git checkout -b new-feature
This command simplifies your workflow by allowing you to isolate and manage changes without affecting the main branch.
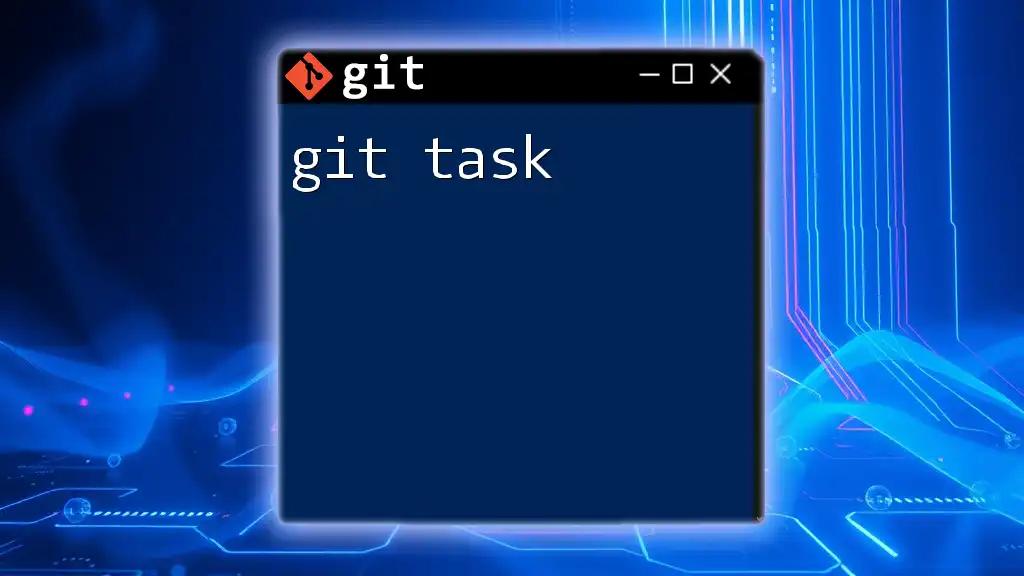
Troubleshooting Common Tracking Issues
Forgetting to `git add`
If you forget to add a new file and realize it only after committing, use `git status` to confirm. You can re-add the file and create a new commit as follows:
git add <file-name>
git commit -m "Add missing file"
Confusion Between Staged and Unstaged Changes
It's common to confuse staged and unstaged changes. Always double-check your status by running:
git status
This will give you a clear picture of which changes are staged for the next commit.
Handling Untracked Files
To manage untracked files gracefully, especially those you don't want to include in your repository (e.g., temporary files). Use a `.gitignore` file to specify files and directories Git should ignore.
Here’s a basic example of a `.gitignore` file:
node_modules/
*.log
This setup ensures that you’re only tracking relevant files and maintaining a clean repository.
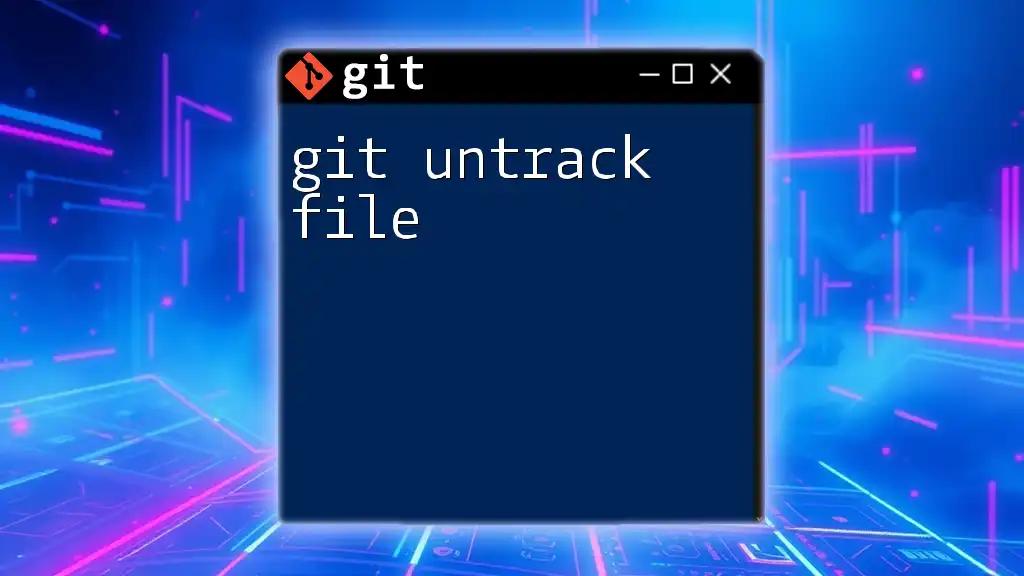
Recap of Git Tracking
Understanding git track and its associated commands is fundamental for any developer. Whether you are just beginning your journey with Git or looking to refine your skills, mastering tracking allows for smoother collaboration and better version control in your projects.
Call to Action
Now that you have a comprehensive understanding of Git tracking, it’s time to put these principles into practice. Experiment with `git add`, `git status`, and `git commit`, and dive deeper into the world of version control by exploring further resources. Your journey with Git has just begun—happy tracking!