The `git tree` command is used to visualize the commit history in a tree-like structure, showing the branching paths of your project, and can be achieved using the `git log` command with specific formatting.
Here's an example of how to use it:
git log --graph --oneline --all
Understanding the Basics of Git Tree
What is a Git Tree?
A Git tree is a fundamental concept in Git that represents the state and structure of your project at a given moment. It organizes the different elements of your repository, including commits, branches, and tags, in a hierarchical manner. Understanding the Git tree is crucial for navigating your project's history and managing your code effectively.
Components of a Git Tree
Commits
Commits are snapshots of your project's changes. Each commit contains a unique identifier, a message describing the changes, and a pointer to the commit's parent. This means that commits create a path, forming a timeline of how your project has evolved.
Example of a simple commit:
git commit -m "Initial commit"
This command captures the current state of your project, creating a new commit in the Git tree.
Branches
Branches represent divergent paths in your project. You can think of a branch as a pointer to a specific commit. By default, your repository starts on the main branch, but you can create additional branches for features or bug fixes without altering the primary codebase.
To create a new branch, you can use:
git branch new-feature
This command adds a new branch called new-feature to your Git tree.
Tags
Tags are used to mark specific points in your Git history, often aligning with important milestones, like releases. Tags do not change, which makes them perfect for versioning your software.
To create a tag, you would typically use:
git tag v1.0
This command creates a new tag named v1.0 that you can later reference or use for release management.
Visual Representation of a Git Tree
The Concept of a Commit Graph
A commit graph is a visual way to represent your Git tree, showcasing how commits, branches, and merges are structured over time. Each commit appears as a node, with arrows indicating parent-child relationships. This visual representation helps you to quickly grasp the history and evolution of your project.
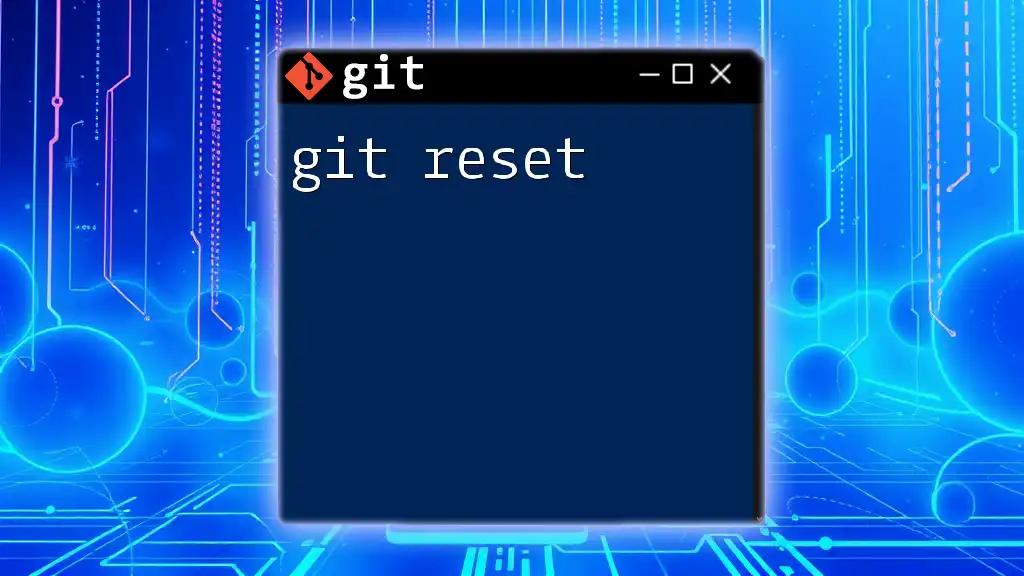
Working with the Git Tree
Viewing the Git Tree
Using `git log`
The `git log` command allows you to view the commit history in your repository. You can customize its output to show various details, making it easier to understand the changes made over time.
For example, you can use:
git log --oneline --graph --all
This command provides a condensed, graphical view of your commit history across all branches, making it simpler to track the project's evolution.
Using `git show`
To dive deeper into specific commits, the `git show` command displays the details of a particular commit, including the differences introduced in that step.
An example command is:
git show <commit-hash>
Replace `<commit-hash>` with the actual hash of the commit you want to inspect.
Navigating the Git Tree
Checking Out a Commit
The checkout command allows you to switch between different points in your Git tree. This is useful for viewing the state of your project at a specific commit.
You can check out a commit by running:
git checkout <commit-hash>
Be cautious, as this may put you into a detached HEAD state if you don’t check out a branch.
Switching Branches
Switching between branches is straightforward and essential for working in Git. The `git switch` command is the safest way to move between branches.
Use the following command to switch:
git switch new-feature
This command takes you to the new-feature branch, allowing you to work on it without affecting other branches.
Modifying the Git Tree
Adding New Commits
To capture new changes in your project, you must stage them and then commit. The process involves two main commands: `git add` and `git commit`.
For instance:
git add .
git commit -m "Add new feature implementation"
The first command stages all modified files, and the second command creates a new commit, adding those changes to the Git tree.
Merging and Rebasing
Merging and rebasing are two methods for integrating changes from one branch into another.
Merging combines changes from different branches while preserving the branch history. A common command to merge a branch into another is:
git merge feature-branch
Rebasing, on the other hand, rewrites commit history to integrate changes linearly, creating a cleaner history. You can rebase the current branch onto another branch using:
git rebase main
Utilizing either approach depends on your workflow preferences and project requirements.
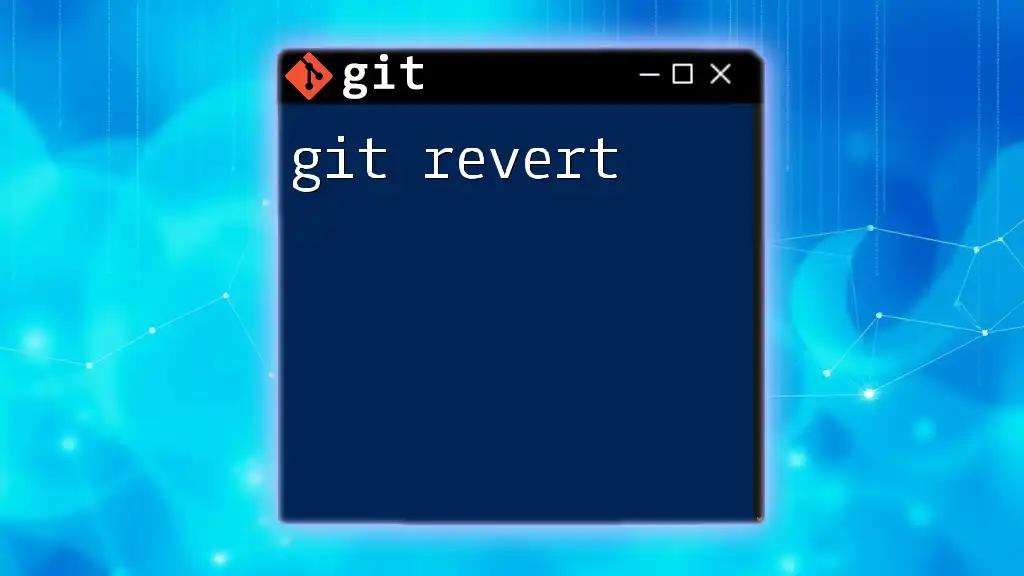
Advanced Git Tree Commands
Tree Object Representation
In Git, the content of your repository is represented as tree objects. These objects form the backbone of the Git tree and actually store the hierarchical structure of the files in your commits.
To view the details of a tree object, you may use:
git cat-file -p <tree-hash>
This command provides an internal view of the tree, revealing how files and directories are structured.
Using `git reflog`
The `git reflog` command tracks updates to the tips of branches, allowing you to see where references were at different times. This is particularly useful for recovering commits that may seem lost.
You can view your reflog with:
git reflog
This will show you a chronological list of actions performed in your repository, letting you recover previous commits even after branching or resetting actions.
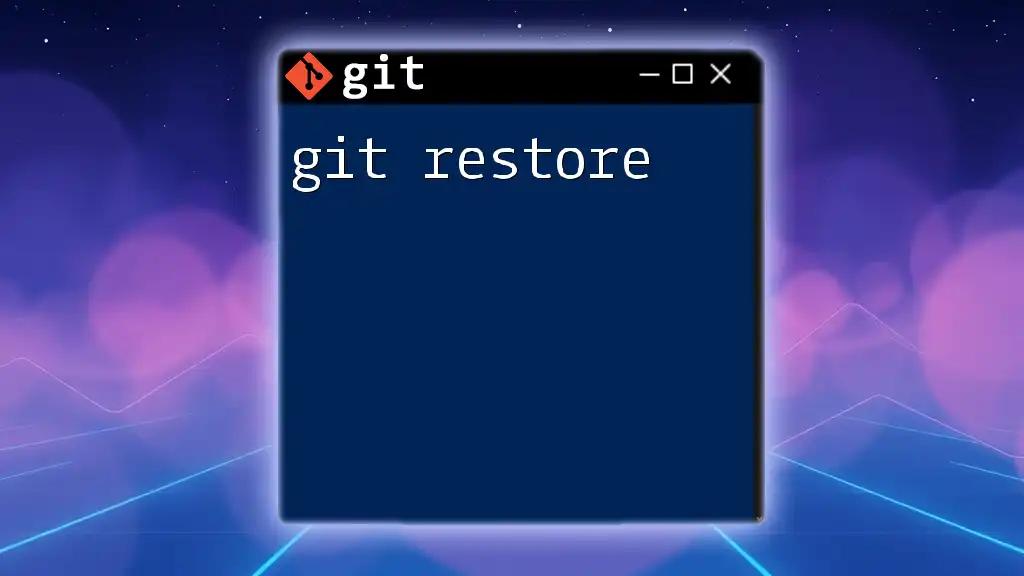
Best Practices for Managing the Git Tree
Structuring Branches
Creating a well-defined branch structure is crucial for effective collaboration. Consider using naming conventions such as feature/ for new features, bugfix/ for bug fixes, and release/ for finalized versions. This organization aids team members in navigating your branches easily.
Clean History Practices
Maintaining a clean history enhances your repository's readability. Squashing commits through interactive rebase minimizes clutter by combining multiple commits into one meaningful entry. This can be done with:
git rebase -i HEAD~N
Here, N represents the number of commits to review and potentially squash.
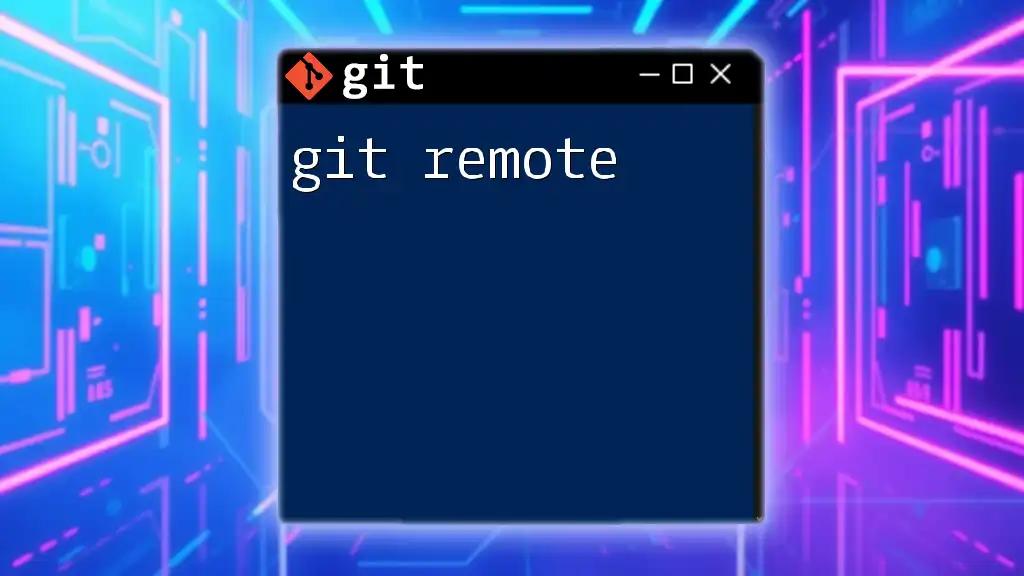
Common Git Tree Issues and Troubleshooting
Resolving Merge Conflicts
When merging changes, you might encounter merge conflicts if changes in two branches overlap. Git flags these conflicts, requiring you to manually resolve them. Open the affected files, look for conflict markers, and edit the files to resolve the differences.
After resolving, you can finalize the merge by staging and committing the changes.
Dealing with Detached HEAD State
A detached HEAD state occurs when you check out a specific commit instead of a branch. While you can inspect files, any new commits won’t attach to a branch. To avoid issues, either create a new branch from the detached state using:
git checkout -b new-branch
Or return to your previous branch with:
git checkout main
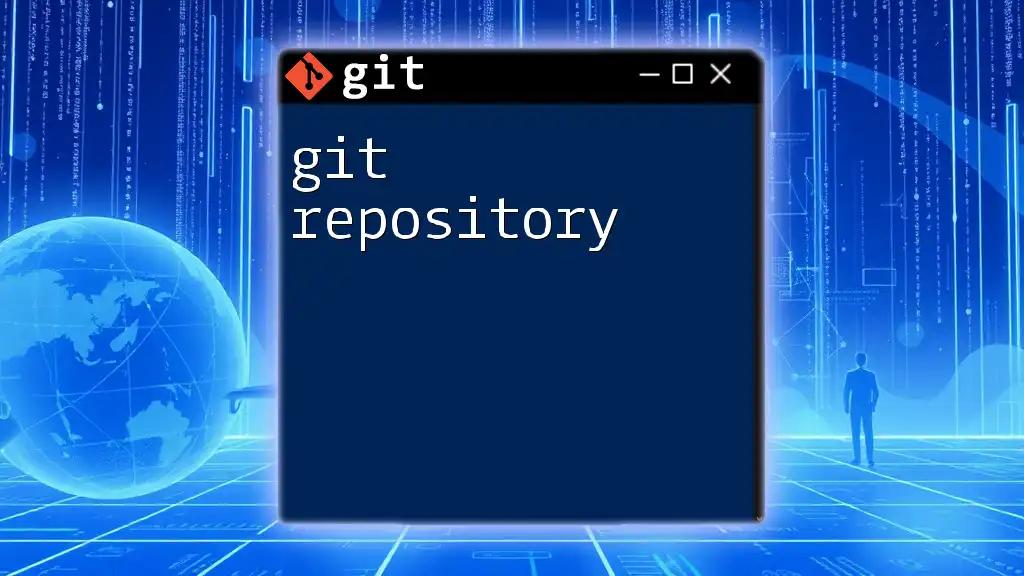
Conclusion
Understanding the Git tree is essential for effective code management and collaboration. By grasping its components and mastering commands, you'll be better equipped to navigate your repository's history and maintain an organized project structure. The more you practice and experiment with Git commands, the more confident you'll become in using this powerful tool for your development needs.
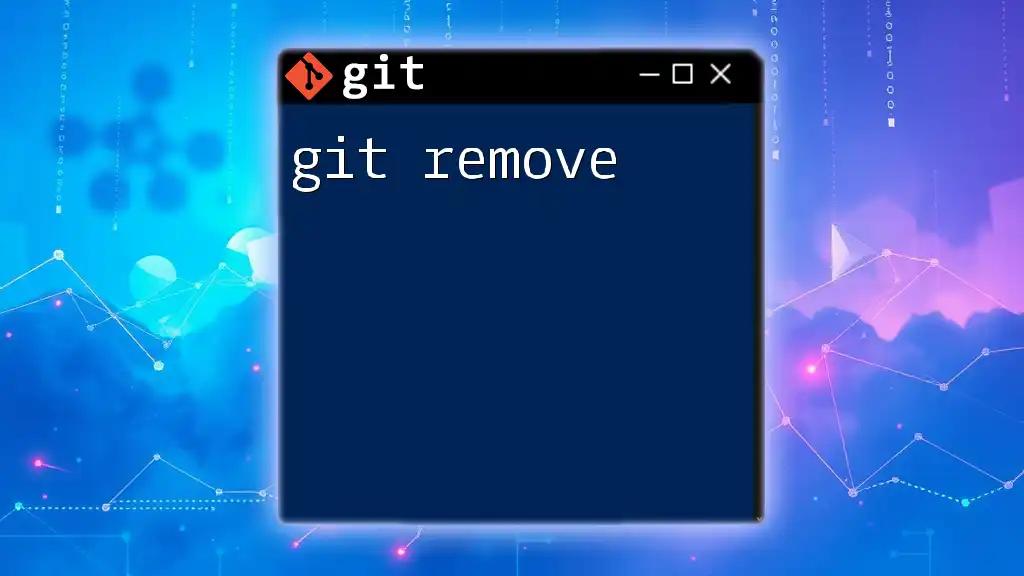
Additional Resources
For comprehensive learning, refer to the official Git documentation, where you'll find extensive tutorials and detailed command references. Engaging with community forums can also help deepen your knowledge and resolve specific issues in real-time.