A Git cheat sheet provides a quick reference guide to essential Git commands for efficient version control management.
Here's a basic code snippet of commonly used Git commands:
# Initialize a new Git repository
git init
# Stage changes for commit
git add .
# Commit changes with a message
git commit -m "Your commit message here"
# Check the status of the repository
git status
# View the commit history
git log
# Push changes to the remote repository
git push origin main
# Pull changes from the remote repository
git pull origin main
Basic Git Commands
Setting Up Git
Installing Git
To start using Git, the first step is to install it on your system. The process differs slightly based on the operating system you are using:
For Windows:
- Download the installer from the official Git website.
- Follow the installation wizard, choosing the default options is usually sufficient.
For macOS:
- You can install Git through Homebrew with the command:
brew install git
For Linux:
- Use the package manager for your distribution. For example, on Ubuntu, you can run:
sudo apt-get install git
Configuring Git
After installation, it’s important to configure Git with your identity. This is how Git associates your commits with your name and email address. Run the following commands in your terminal:
git config --global user.name "Your Name"
git config --global user.email "youremail@example.com"
By using the `--global` flag, these settings will apply to all your repositories. To see your settings, use the command `git config --list`.
Creating a Repository
Initialize a Git Repository
Creating a new Git repository is simply done with the `git init` command. This command sets up a new `.git` directory, creating a new version control system for your project:
git init
Make sure to navigate to the project directory before running this command.
Basic Workflow
Cloning a Repository
If you need to collaborate on projects hosted on platforms like GitHub, you can clone an existing repository using the command:
git clone <repository-url>
This command copies all the content, history, and branches from the specified repository to your local machine. For example, if you're cloning a repository from GitHub, your command might look like this:
git clone https://github.com/username/repo.git
Checking Status
To see the current state of your repository, including changes that are staged for commit and any untracked files, you can use the command:
git status
This command is invaluable in assessing your next steps before proceeding with commits or changes.
Adding and Committing Changes
Staging Changes
Before you commit changes, you must stage them. This allows you to select which changes you want to include in your next commit. You can stage individual files or all changes:
To stage a specific file:
git add <file-name>
To stage all changes:
git add .
Understanding the staging area is key to managing your commits effectively.
Committing Changes
Once your changes are staged, you can commit them to the repository. It’s essential to provide a meaningful commit message to describe what you’ve done:
git commit -m "Your detailed commit message"
A succinct yet descriptive commit message helps others (and future you) understand the history of changes.
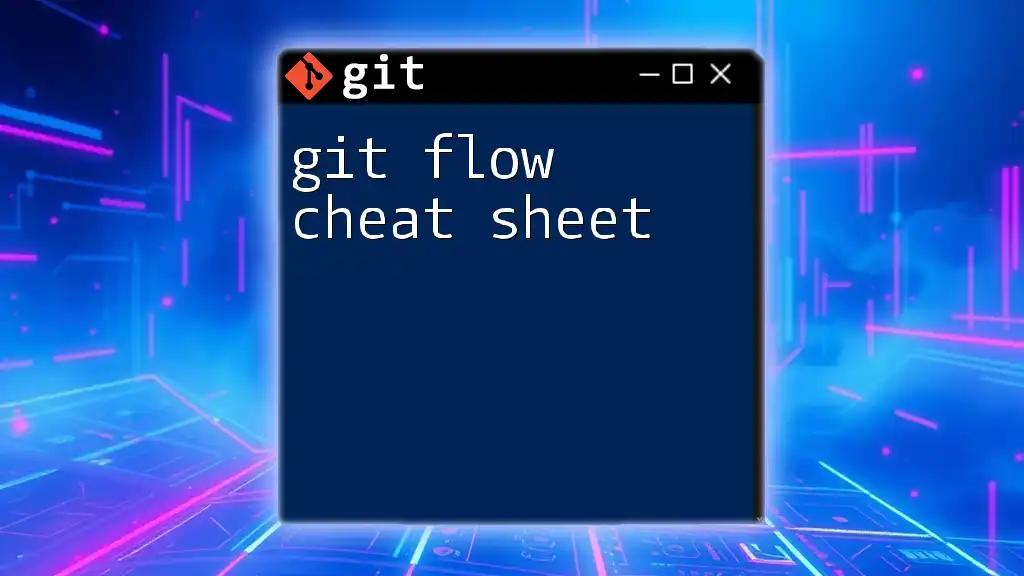
Branching and Merging
Understanding Branches
Creating a New Branch
Branches in Git allow you to work on different features or bug fixes without affecting the main project. You can create a new branch with:
git branch <branch-name>
Switching Branches
To switch between branches, use the command:
git checkout <branch-name>
Alternatively, Git 2.23 introduced the `git switch` command, which is more intuitive for switching branches:
git switch <branch-name>
Merging Branches
Merging Changes
After making changes in a branch, you may want to merge them back into the main branch. Switch to the branch you want to merge into (usually `main`) and run:
git merge <branch-name>
You will encounter either a fast-forward or a three-way merge depending on your commit history. Fast-forward merges occur when the branch you're merging into has no new commits. In contrast, three-way merges entail combining different commit histories and may lead to conflicts.
Resolving Merge Conflicts
When merging, if you modify the same part of a file in different branches, Git will alert you to a conflict that must be resolved. Open the conflicting file to find markers indicating the conflicting sections. Edit the file to resolve the conflict, then stage and commit the resolved changes.

Remote Repositories
Working with Remotes
Adding a Remote Repository
To link your local repository to a remote repository, use the `git remote add` command:
git remote add origin <repository-url>
Pushing Changes to a Remote
After committing your changes locally, you can push them to the remote repository using:
git push origin <branch-name>
This command uploads your local changes to the specified remote branch.
Fetching and Pulling Changes
To incorporate updates from the remote repository, use:
git fetch
This command downloads the changes but does not automatically merge them. To fetch and merge in one command, you can use:
git pull
It’s crucial to understand that `git fetch` keeps your local repository updated with all changes without breaking your workflow.
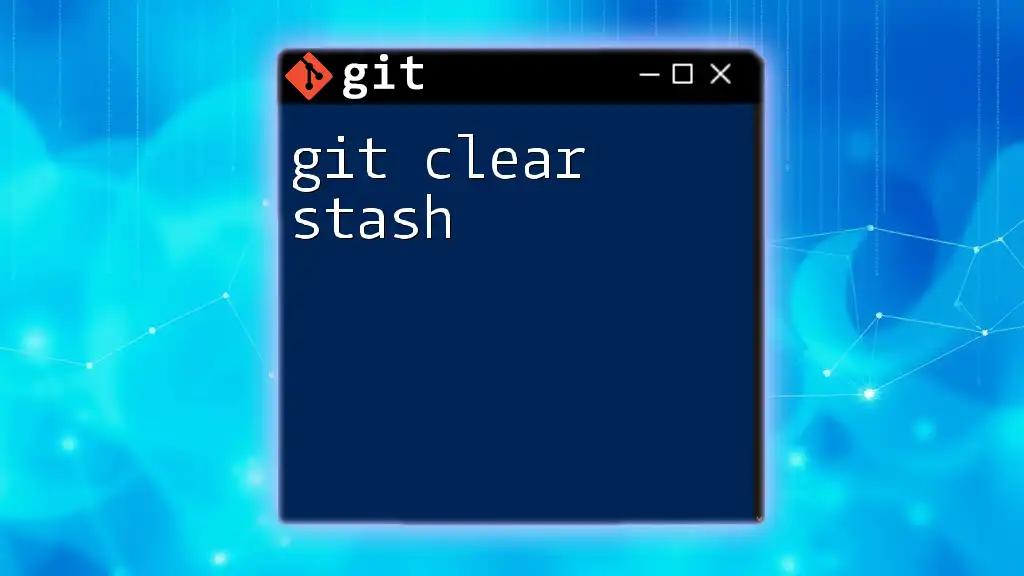
Stashing Changes
Saving Work-In-Progress
What is Stashing?
Sometimes, you might need to switch contexts and work on something else without committing incomplete work. Git allows you to stash your changes temporarily.
Using Stash Commands
To stash your changes, simply execute:
git stash
Later, you can bring back your stashed changes with:
git stash pop
This will apply the stashed changes to your working directory, allowing you to continue where you left off.
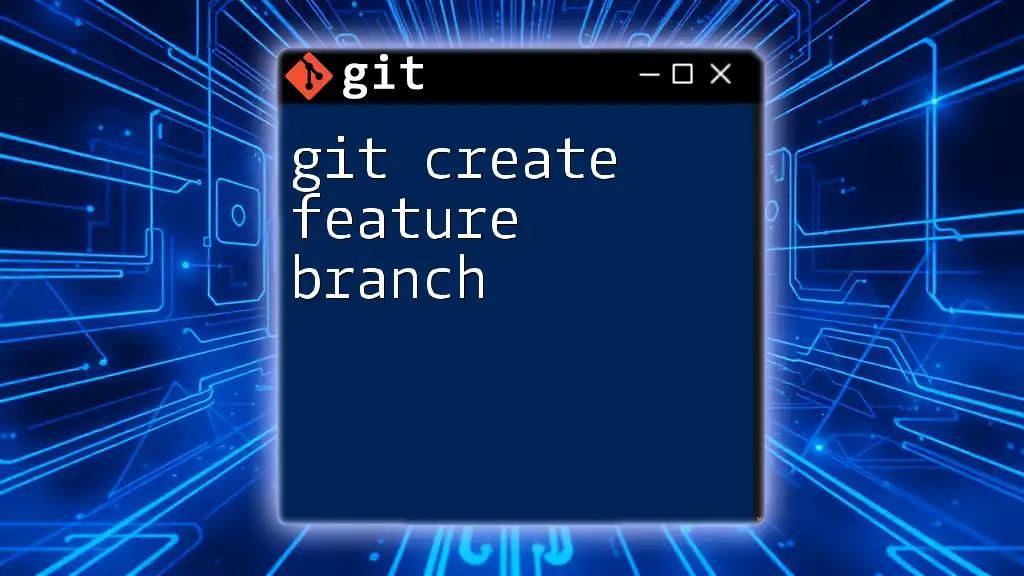
Inspecting the History
Viewing Commit Log
Viewing Commit History
Understanding the history of commits is essential for tracking changes. Use the command:
git log
You can further refine this with formatting options to show a concise view:
git log --oneline --graph
This displays a graphical representation of your commit history, making it easier to understand the structure of your project changes.
Checking Differences
Comparing Changes
To see what has changed between commits or files, the `git diff` command is invaluable. For example, to see differences in your working directory compared to the staged files:
git diff
You can also compare specific commits using their IDs:
git diff <commit-id>
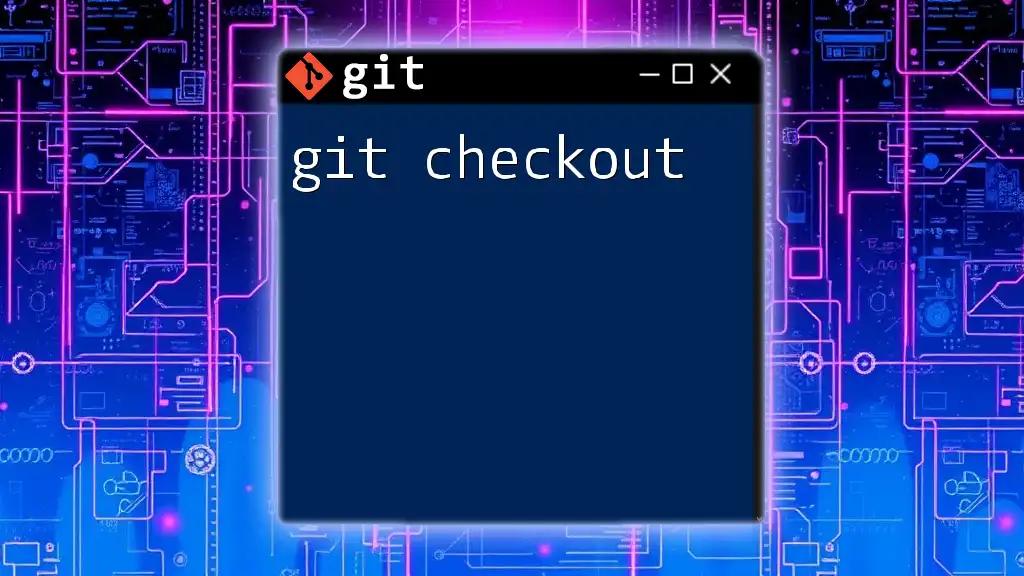
Cleanup and Maintenance
Removing Files
Removing Tracked Files
Occasionally, you may need to remove files from your repository. To do so safely, use:
git rm <file-name>
This removes the file from both your working directory and the staging area.
Removing Untracked Files
To delete files that aren’t being tracked by Git, run:
git clean -f
Be cautious with this command, as it will permanently delete untracked files.
Reverting Changes
Undoing a Commit
If you make a mistake in your commits, you can revert it using the command:
git revert <commit-id>
This command creates a new commit that undoes the changes made in the specified commit. It's a safe way to back out of a particular change without altering commit history.
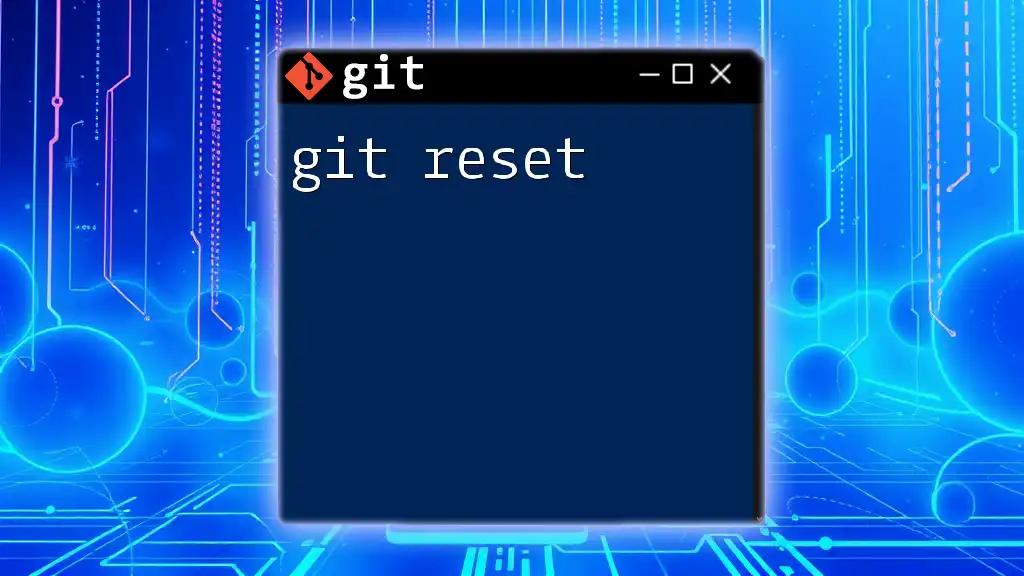
Conclusion
Git is a powerful tool for managing changes in projects, and understanding its commands can greatly enhance your productivity. Whether you're working on personal projects or collaborating with others, referring to a git cheat sheet will help you navigate through common tasks quickly. By familiarizing yourself with these commands and regularly practicing, you'll gain confidence in using Git for your development needs.
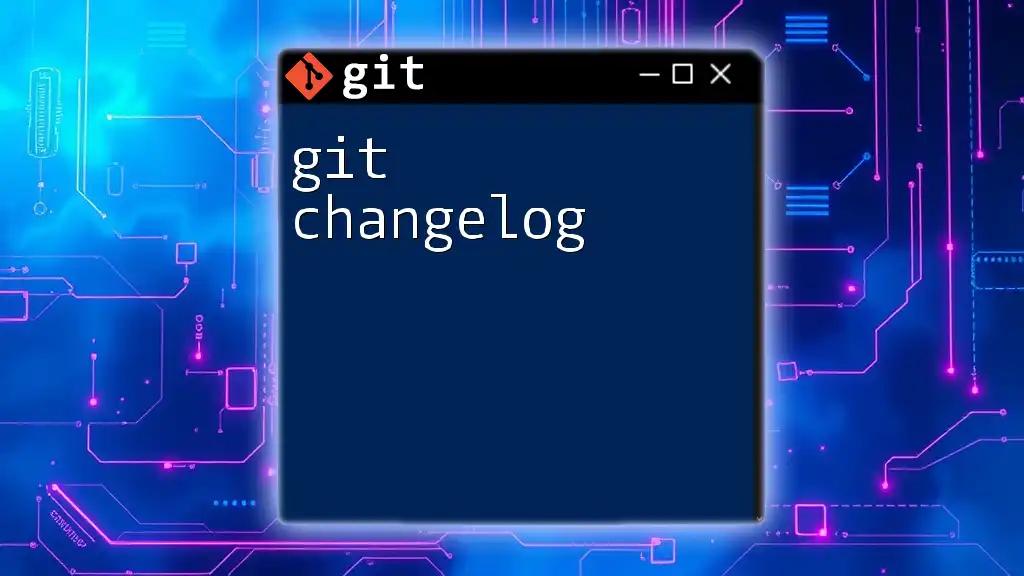
Additional Resources
For further reading and deeper understanding, consider exploring the [official Git documentation](https://git-scm.com/doc) and finding recommended tutorials and videos that cater to visual learners.
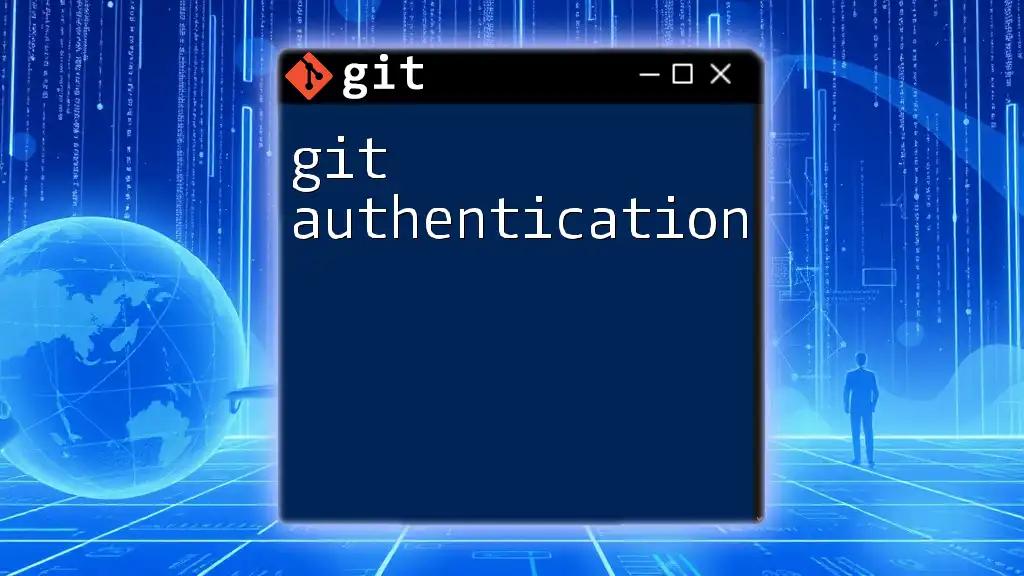
Call to Action
If you're eager to master Git and ensure a smooth workflow in your development endeavors, consider joining our course tailored to make learning Git efficient and enjoyable. Start mastering Git today!