A Git snapshot captures the state of your entire repository at a specific point in time, allowing you to track changes, revert to previous versions, or collaborate effectively.
git commit -m "Snapshot of project at specific point in time"
What is a Git Snapshot?
A git snapshot is a point-in-time representation of your project at a specific moment. Unlike traditional version control systems that track changes as deltas (the differences between two versions), Git captures the entire state of your project through snapshots. Each commit in Git effectively creates a snapshot of your codebase.
This approach brings several advantages. Not only does it provide a complete history of your project, enabling easy rollbacks and comparisons, but it also offers a built-in backup mechanism. With each snapshot, you retain all files, making it straightforward to recover lost or altered code.
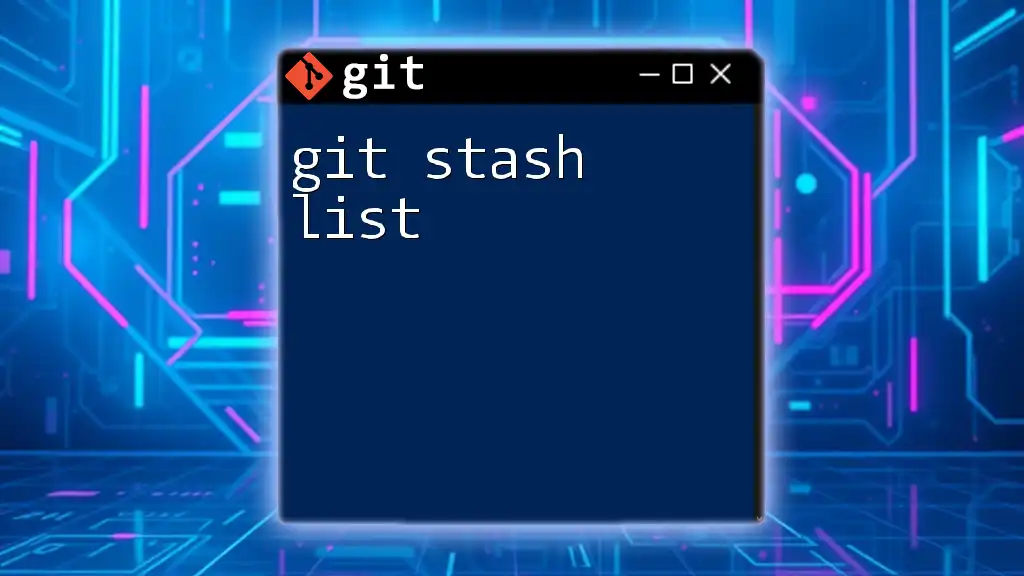
How Git Snapshots Work
Internal Mechanics of Snapshots
To truly understand git snapshots, it's important to grasp the underlying components of Git's object model. Git organizes data into three primary types of objects: blobs, trees, and commits.
-
Blobs store the content of the files in your repository. Each unique version of a file is represented by a blob object, which is identified by a unique hash.
-
Trees represent the directory structure of your project. They act as pointers that link tree objects with blobs, establishing relationships between files and folders.
-
Commits are the metadata associated with each snapshot. Each commit points to a tree object (representing the directory structure at that commit), and contains information such as the author, timestamp, and a reference to the previous commit.
The Git Object Model
When you create a commit, Git constructs a tree object that represents the state of all files. A commit can be seen as a pointer to this tree. Together, blobs, trees, and commits create a powerful structure that forms the foundation of Git's ability to track your project history.
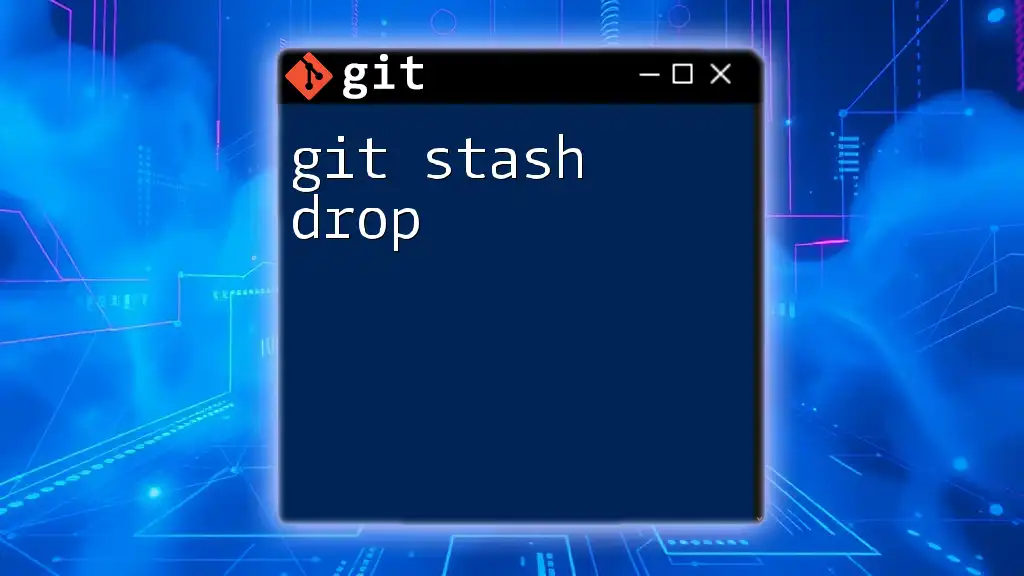
Creating Snapshots in Git
Making Your First Snapshot
Creating your first git snapshot is simple. The primary commands involved are `git add` and `git commit`. The following steps outline how to capture a snapshot of your project:
-
Use `git add` to stage changes. This command tells Git which modifications you want to include in your snapshot. For example:
git add .
-
Next, commit the staged changes to the repository with a meaningful message that describes the changes:
git commit -m "Initial snapshot of project."
Here, the commit captures your project's current state, storing it as a snapshot in the repository.
Understanding the Commit Command
When you run `git commit`, Git performs several critical operations:
- It creates a new commit object.
- It links this commit to the previous one, forming a chain of snapshots.
- It updates the branch pointer to refer to this new commit.
This process ensures that every change you make is chronologically recorded, allowing you to navigate your project's history with ease.
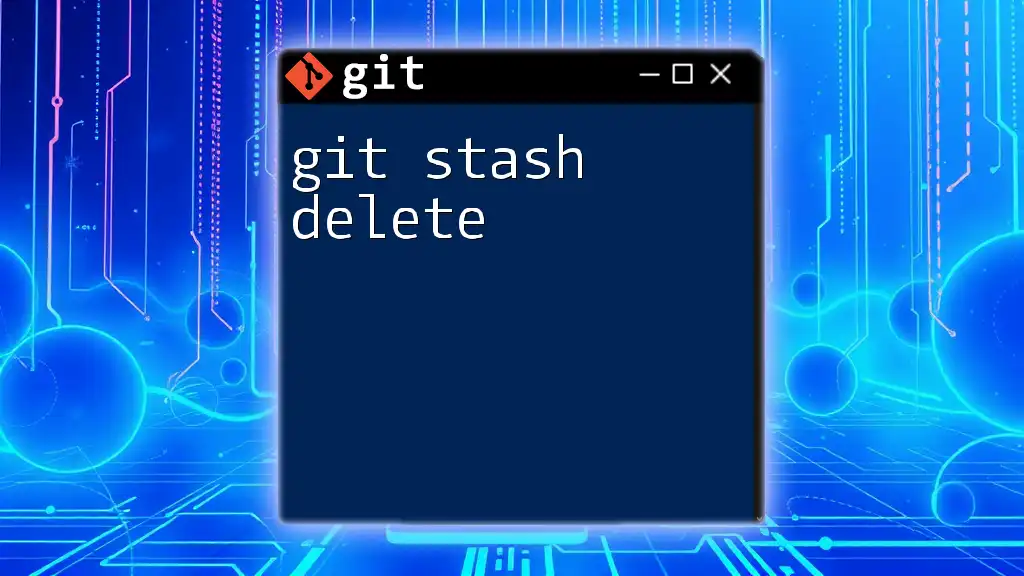
Viewing Your Snapshots
Using Git Log
To view the history of your snapshots, you can use the `git log` command. This command displays a chronological list of commits, showing the commit hash, author, date, and summary. A simplified version can be executed as follows:
git log --oneline
This format offers a quick overview, allowing you to identify relevant snapshots easily.
Exploring Snapshots with Git Show
To inspect a specific snapshot in detail, you can use the `git show` command followed by the commit hash. For example:
git show <commit_hash>
This command reveals the changes introduced in that commit, including modified files and their content changes, enabling you to analyze past work.
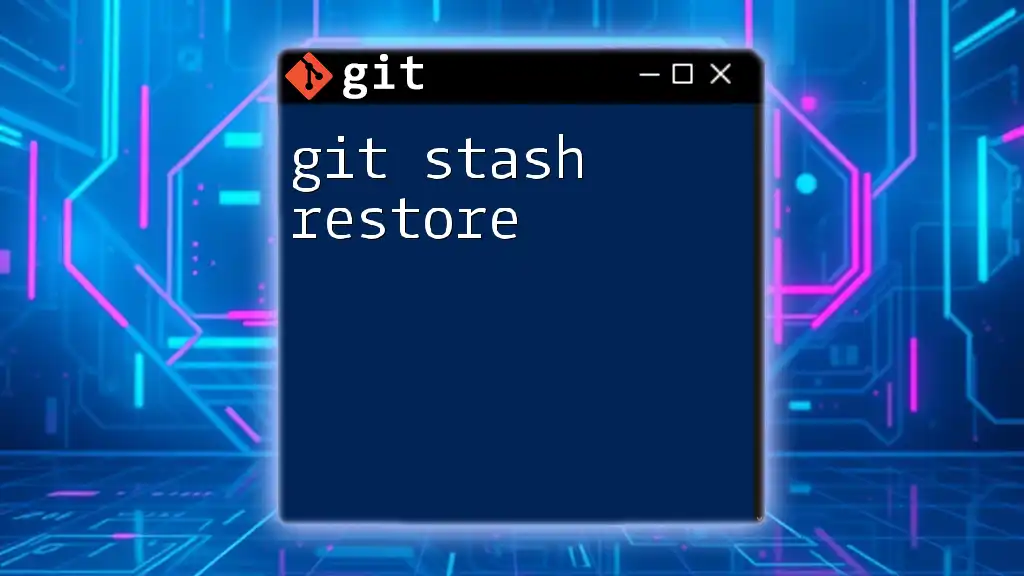
Managing Snapshots
Reverting to Past Snapshots
One of the powerful features of Git is the ability to revert back to previous snapshots. By using the `git checkout` command followed by a commit hash, you can switch to the state of the repository at that specific point:
git checkout <commit_hash>
This action allows you to work with or examine older versions of your project.
Creating Branches from Snapshots
Git allows you to create branches based on existing snapshots, making it easier to develop features or fixes in isolation. You can create a branch by using the following command:
git checkout -b new-feature <commit_hash>
This command creates a new branch named "new-feature" pointing to the specified commit, enabling parallel development without affecting the main branch.
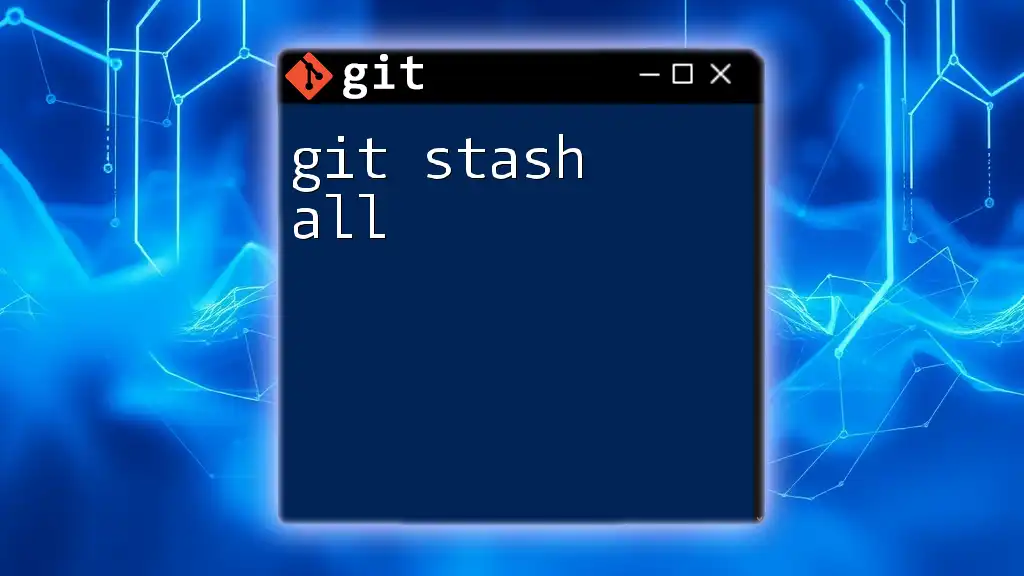
Best Practices for Using Git Snapshots
Commit Often, Commit Small
Adopting the habit of making small, frequent commits results in clearer snapshots and makes it easier to track progress and resolve issues. For instance, instead of committing a massive change at the end of a project phase, break it down into smaller, meaningful commits. This enhances clarity and simplifies the review process.
Meaningful Commit Messages
Writing effective commit messages is crucial. A good practice is to state what was changed and why. For example, instead of a vague message like "fixed stuff," a better message would be "Corrected layout issues in the homepage." Meaningful messages create context and understanding for future reference.
Keeping Your Snapshots Clean
To maintain a clean history of snapshots, consider using Git's advanced features. Commands like `git rebase` help in restructuring commits, while `git cherry-pick` allows you to apply specific changes from one branch to another. Keeping your commit history organized reduces confusion and aids collaboration, especially in team environments.
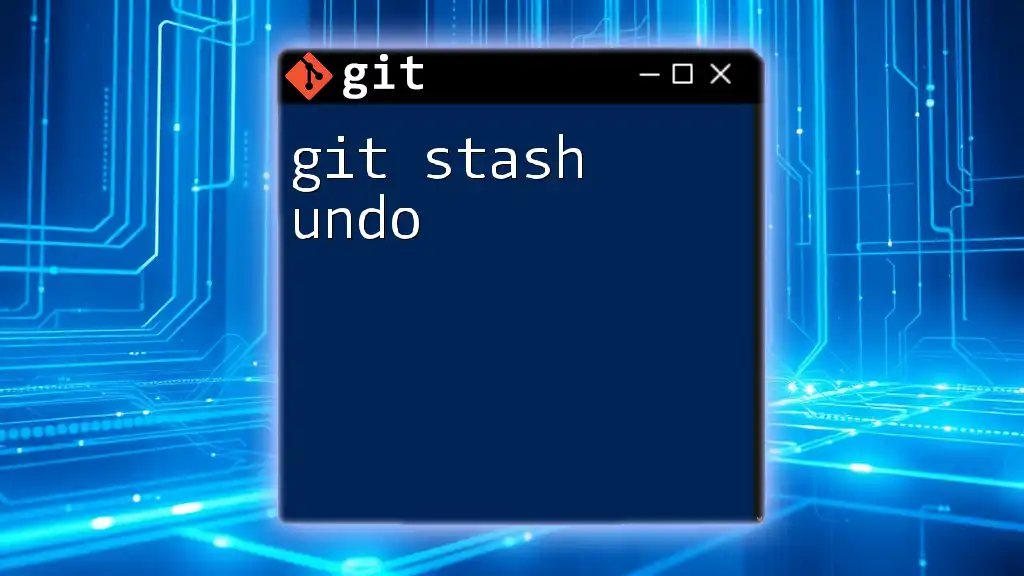
Conclusion
In summary, understanding git snapshots is essential for effective version control. Each snapshot captures a moment in your project's evolution, allowing you to review, revert, and branch as needed. By practicing good commit discipline, writing meaningful messages, and managing your snapshots wisely, you'll be better equipped to maintain the quality and integrity of your codebase.
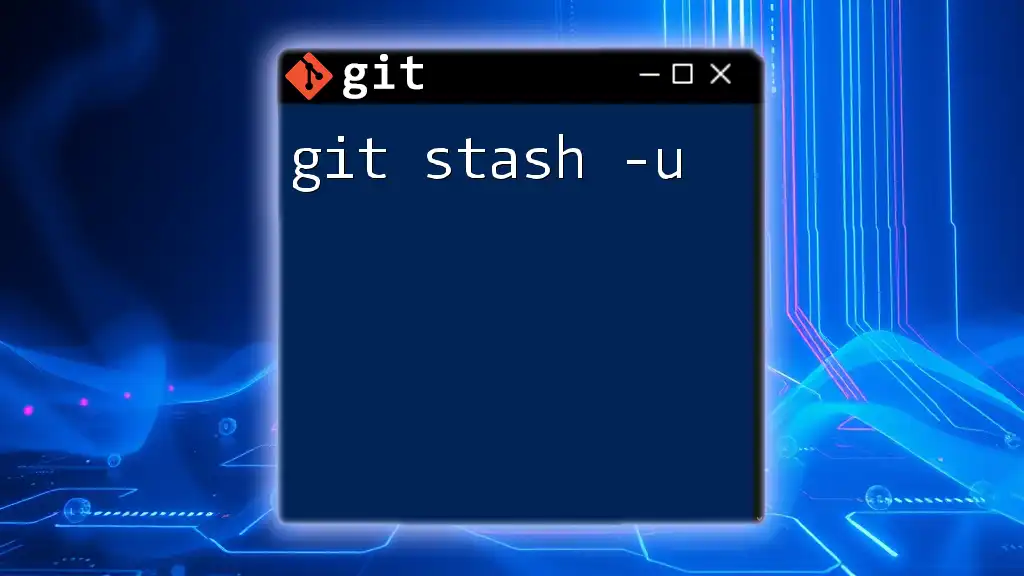
Additional Resources
For those eager to further their understanding of Git, various official documentation sites and tutorials are available online. These resources can provide deeper insights into advanced features and best practices.
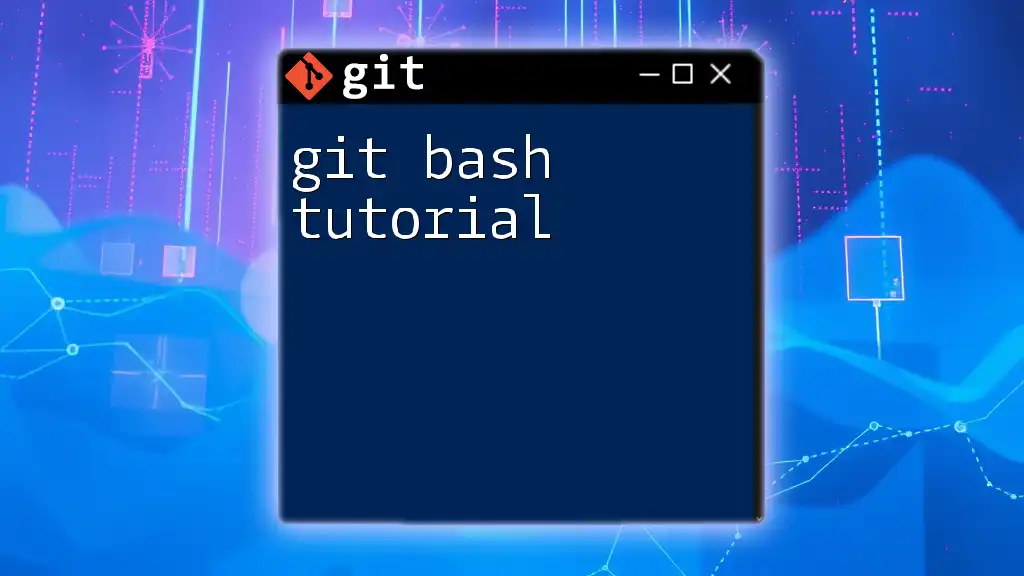
FAQ Section
Common questions often arise regarding git snapshots:
-
What happens to uncommitted changes? Uncommitted changes remain in your working directory and will not be included in the snapshot until you stage and commit them.
-
Can I recover deleted snapshots? As long as a commit isn't garbage collected, it can be recovered through Git's reflog feature, which tracks changes in your repository.
-
What are the risks of rewriting commit history? While it can be useful for cleaning up commits, rewriting history (e.g., through commands like `git rebase`) can lead to confusion, especially when collaborating, as others may have based their work on the original history. Always proceed with caution when altering commit history.