The `git branch -b` command is used to create a new branch and switch to it immediately, allowing you to start working on it right away.
Here's how you can use it:
git checkout -b new-branch-name
Understanding Branches in Git
What is a Branch?
A branch in Git is essentially a pointer to a specific commit. It enables developers to work on different features, fix bugs, and isolate changes without affecting the main codebase. By creating a branch, you can make changes and experiment freely, ensuring that your main branch remains stable and clean.
Types of Branches
Branches in Git can be categorized into several types, each serving a distinct purpose:
-
Main Branch: This is often referred to as `main` or `master`. It represents the primary working version of your project and is the default base for all branches.
-
Feature Branches: These branches are used to develop new features. They provide a separate environment for feature development, ensuring that incomplete features don't disrupt the main code.
-
Hotfix Branches: Created for urgent fixes, these branches allow developers to address critical issues directly from the main branch. Once the fix is complete, the changes can be merged back into both the main branch and the relevant feature branches.
-
Release Branches: These branches serve as a staging area for preparing a production release. They provide a way to finalize versions while allowing for minor tweaks and last-minute fixes.
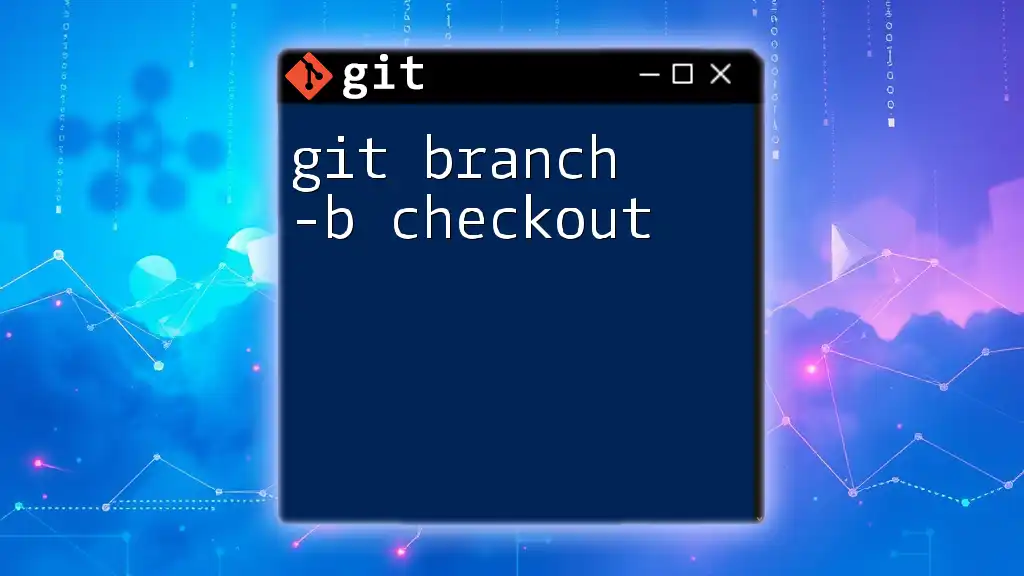
What is `git branch -b`?
Explanation of the Command
The command `git branch -b` is actually a common misconception. The correct usage to create and switch to a new branch in a single step is `git checkout -b [branch-name]`. This command is not just about branching; it’s about streamlining your workflow by eliminating the need for two separate commands.
Purpose of Using `git branch -b`
The main purpose of using `git checkout -b` is to create a new branch and immediately switch to it, thus saving time and effort. This command is especially useful in collaborative environments where developers need to branch off frequently to work on different features or fixes without interrupting others.
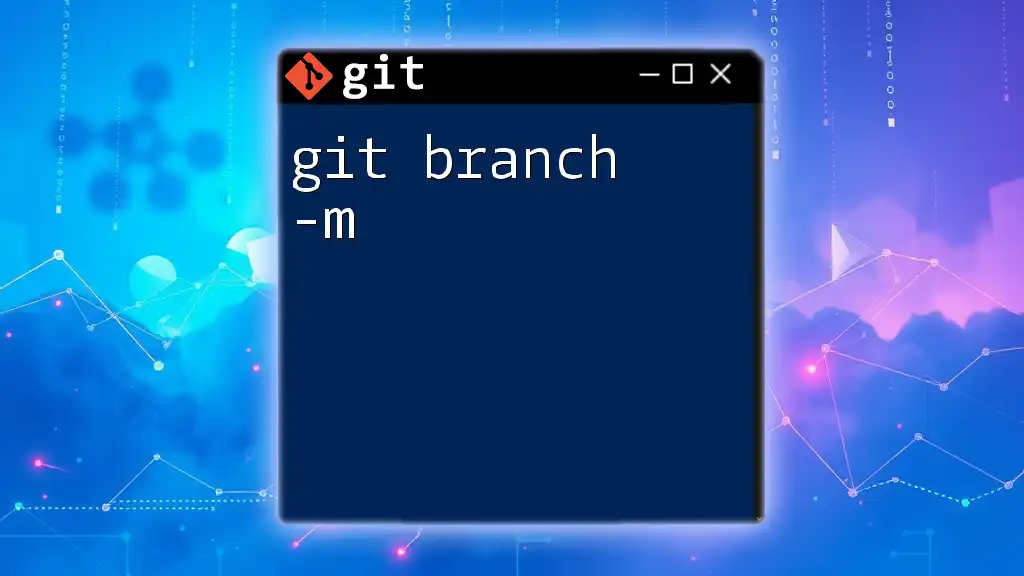
How to Use `git branch -b`
Basic Syntax
To create a new branch and switch to it, you would use the following command:
git checkout -b [branch-name]
Step-by-Step Guide
Step 1: Check Your Current Branch
Before creating a new branch, it's important to know your current branch. You can see this by running:
git branch
This lists all branches in your repository, highlighting the branch you are currently on.
Step 2: Create a New Branch
To create and switch to a new branch, simply run:
git checkout -b feature/new-feature
In this example, a new branch named `feature/new-feature` is created and checked out in a single command.
Step 3: Confirm the New Branch Creation
To verify that your new branch has been created and that you have successfully switched to it, run:
git branch
You should see the list of branches, with the new branch highlighted to indicate you are currently on it.
Common Use Cases
Creating and switching to a new branch is particularly useful when embarking on a new feature, fixing a bug, or experimenting with ideas without affecting the stable main branch. This method allows for parallel development in a collaborative setting, making it easier to manage features independently.
Example Scenario
Imagine you're developing a new project and need to implement user authentication. Instead of modifying the `main` branch, you create a separate branch to keep your changes isolated:
git checkout -b feature/user-authentication
As you work on this feature, you can make multiple commits, test other features, or even merge changes from other branches without risk to the stable codebase.
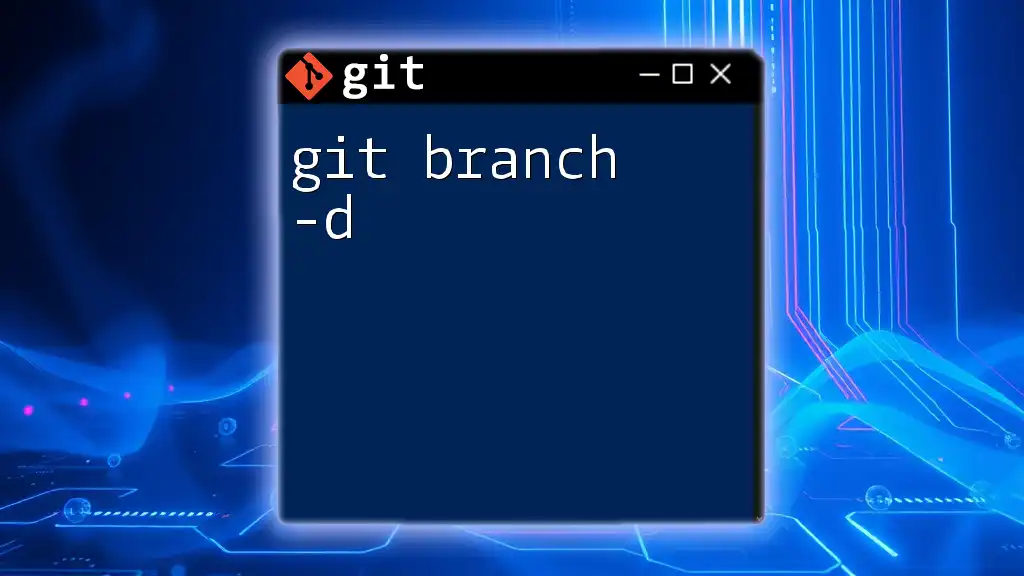
Best Practices for Branching
Naming Conventions
Consistent naming conventions for branches improve clarity and organization. Use prefixes like `feature/`, `bugfix/`, or `hotfix/` to categorize branches according to their purpose. For instance, naming a branch `feature/user-authentication` immediately communicates its purpose.
Regularly Deleting Merged Branches
Once branches have been merged back into the main branch, it's a good practice to delete them to keep your branch list clutter-free. To delete a branch after it has been merged, you can run:
git branch -d [branch-name]
This command ensures that your development environment remains manageable and up to date.
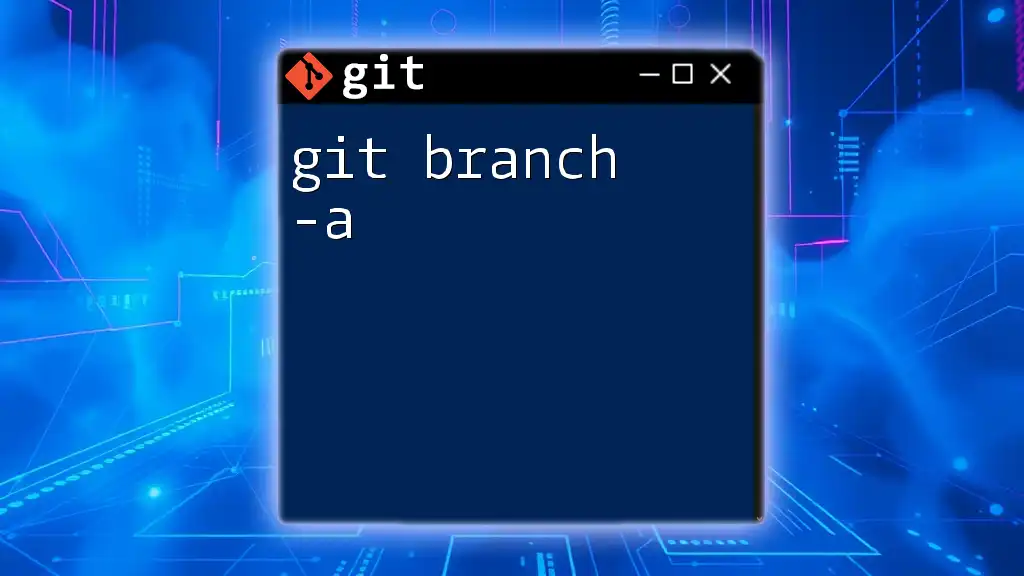
Troubleshooting Common Issues
Error Messages
You might encounter error messages when trying to create branches, such as:
fatal: A branch named '...' already exists.
This indicates that a branch with the specified name already exists. To resolve this, you may either choose a different name or delete the existing branch first.
Conflicts During Branch Creation
Handling conflicts when switching between branches can arise if there are uncommitted changes. Ensure your working directory is clean by either committing changes or stashing them before attempting to switch branches.
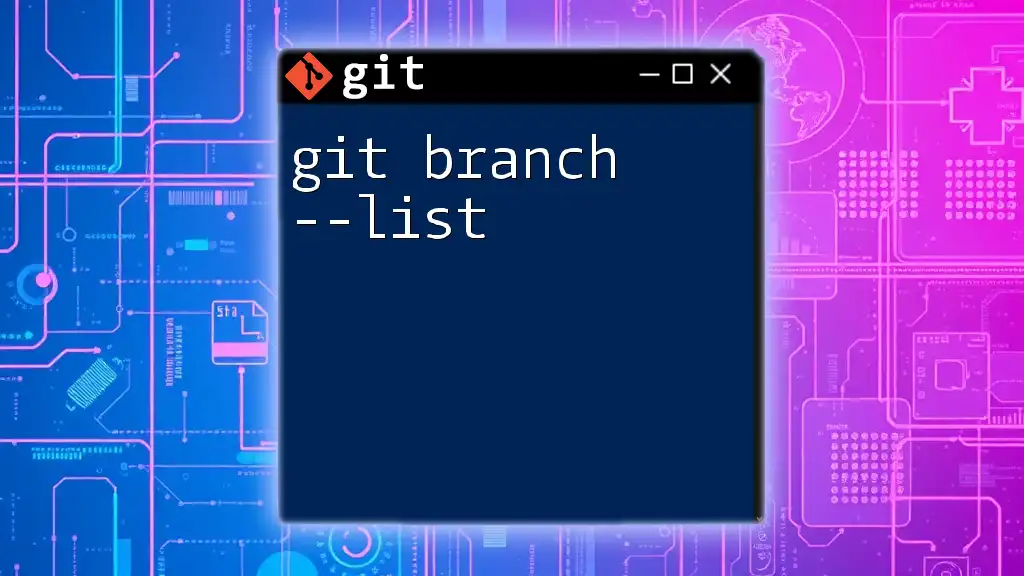
Conclusion
Mastering the `git checkout -b` command is essential for any developer looking to streamline their workflow and enhance their productivity in Git. By effectively utilizing this command, you can create separate branches for features, hotfixes, or experiments with ease. Remember to follow best practices in naming conventions and managing your branches to maintain an organized and efficient codebase.
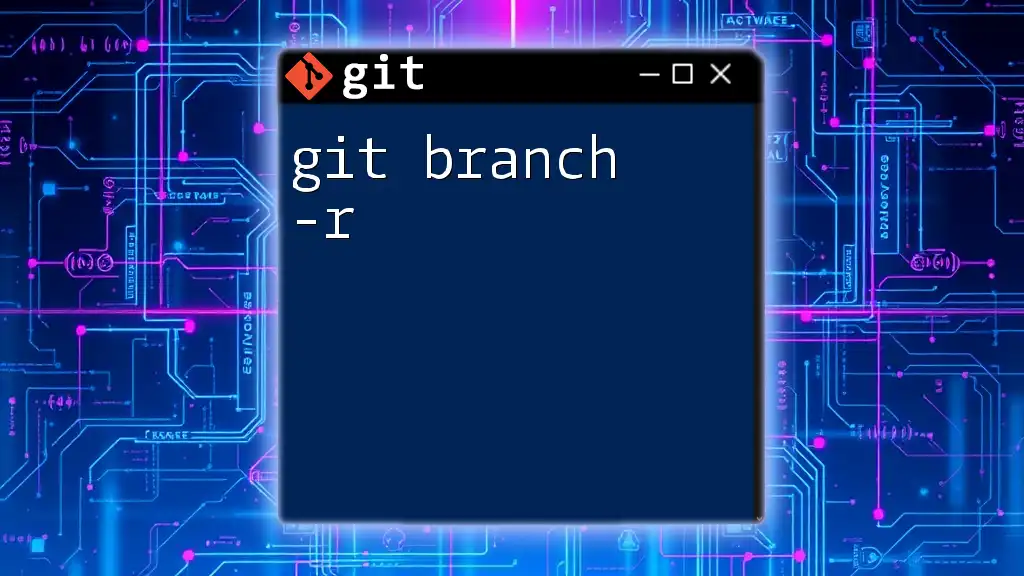
Additional Resources
For further information, consult the official Git documentation and explore additional tutorials and courses that delve deeper into Git branching strategies.