The `git branch -r` command is used to list all remote branches in your Git repository.
git branch -r
Understanding Git Branches
What are Git Branches?
In Git, branches represent an independent line of development. Each branch allows you to develop features or fix bugs while keeping the master branch clean and stable. When you create a new branch, you make a copy of the code at a particular point in time, enabling you to work in isolation.
Local vs. Remote Branches
Git distinguishes between local and remote branches.
- Local branches are those you create and manage in your local repository. They allow you to work on new features or fixes without affecting the main project.
- Remote branches are branches stored in a remote repository, typically on a service like GitHub or GitLab. They reflect the state of the project on that remote server.
Understanding both is crucial for effective collaboration and version control in any software development project.
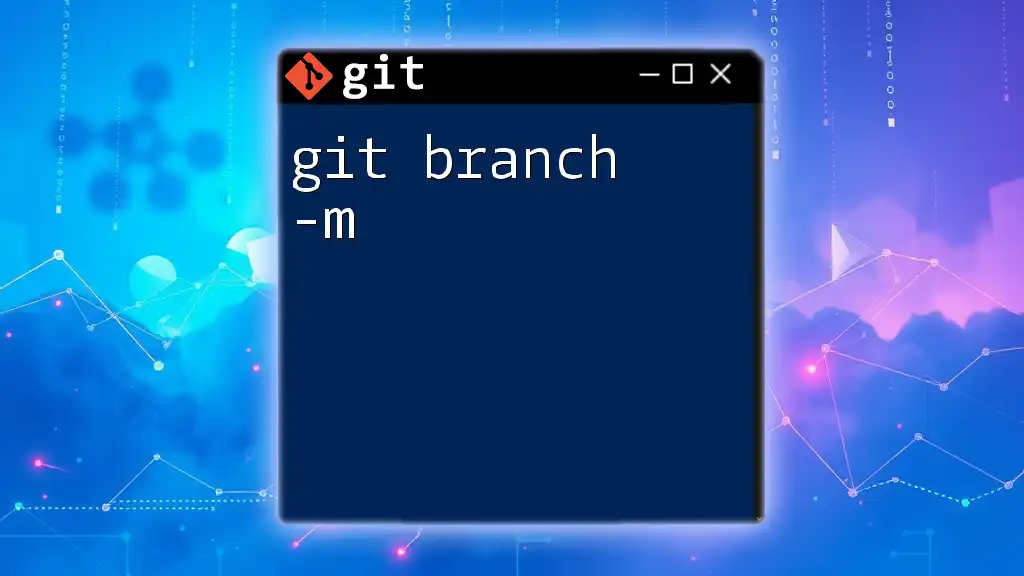
The `git branch` Command
Overview of the `git branch` Command
The `git branch` command is fundamental in Git, enabling you to create, delete, and manage branches. The basic structure is:
git branch [options] [branch-name]
Common options include:
- `-a`: List both local and remote branches.
- `-d`: Delete a branch.
- `-m`: Rename a branch.
Using `git branch -r`
The `-r` flag with the `git branch` command is specifically for listing all remote branches. This command allows developers to see the branches that exist in the remote repository without needing to check out each one individually.
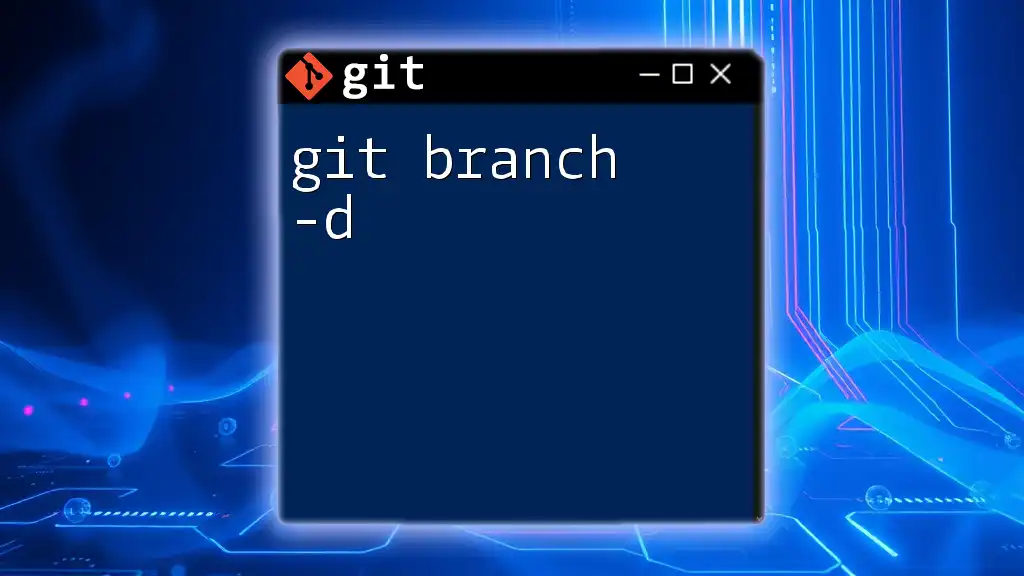
How to View Remote Branches
Basic Usage of `git branch -r`
To view all remote branches, you can execute the following command:
git branch -r
When you run this command, Git will output a list of branches that exist on the remote repository. This helps you see what others are working on and allows you to understand the branching framework of the project.
Example: Viewing Remote Branches
Here’s a step-by-step example of how to view remote branches:
git init
git remote add origin <repository-url>
git fetch
git branch -r
- `git init` creates a new local repository.
- `git remote add origin <repository-url>` connects your local repository to a remote repository.
- `git fetch` synchronizes your local repository with the remote repository by fetching all branches.
- `git branch -r` lists the remote branches.
Upon executing these commands, you will be presented with the names of remote branches, providing you a comprehensive insight into available branches on the remote.
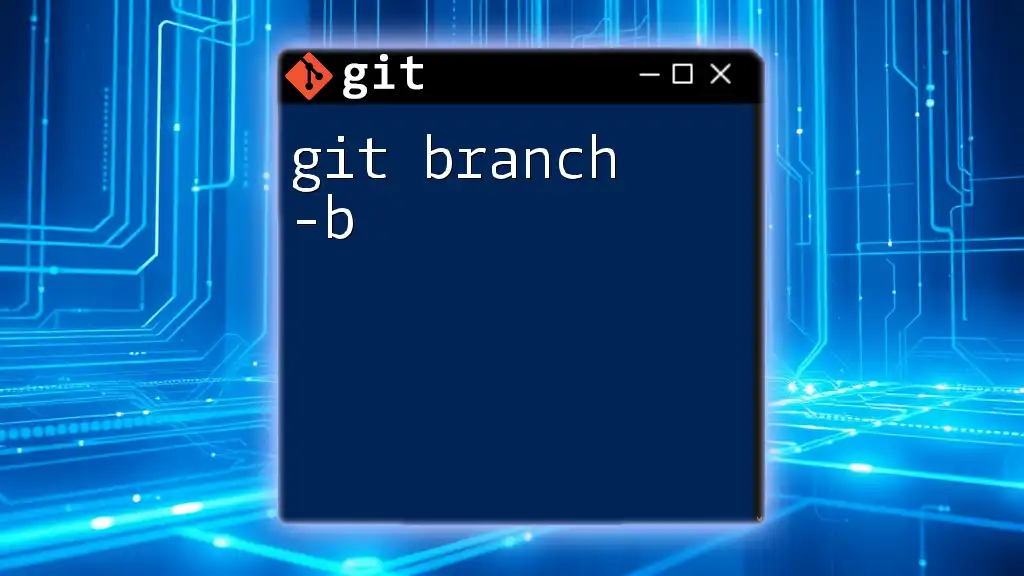
Understanding the Output
What the Output Tells You
The output of `git branch -r` typically looks like this:
origin/HEAD -> origin/main
origin/feature/login
origin/bugfix/issue-123
origin/release/v1.0
- Each line represents a remote branch.
- The `origin/HEAD` entry indicates the default branch at the remote, often pointing to the main production branch.
Identifying Default Remote Branches
The prefixes in the branch names indicate their remote origin. In the example above, `origin` is the name of the remote repository, and the branches follow as `feature/login`, `bugfix/issue-123`, and so forth. Understanding this structure can assist in navigating complex project repositories.
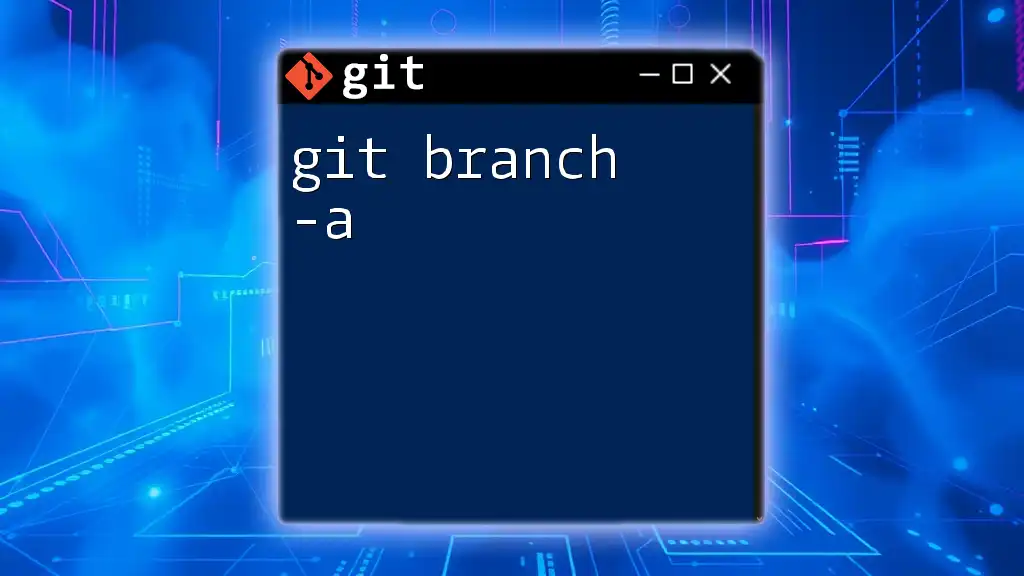
Filtering Remote Branches
Using Grep with `git branch -r`
When dealing with multiple remote branches, it can become overwhelming. You can use filtering to keep things manageable. For instance, if you want to find all branches that relate to a feature, you can combine the command with `grep`:
git branch -r | grep 'feature/'
This command will filter the list and display only those branches with "feature" in their names, allowing you to focus on relevant developments.
Advanced Filtering Techniques
You may further enhance your branch listing using other command-line tools that complement `git branch -r`. Utilize combinations with commands like `sort`, `uniq`, or even custom scripts to organize your output effectively.
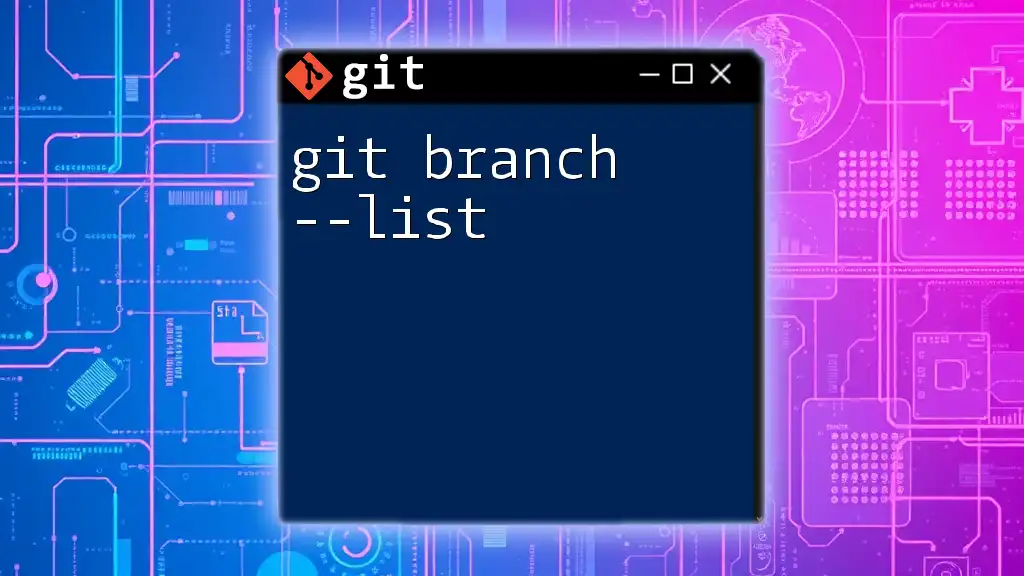
Common Use Cases for `git branch -r`
Checking Remote Branches Before Checkout
Before switching to a remote branch, it's crucial to check its status. This helps prevent conflicts and provides insight into ongoing developments. Always run `git branch -r` to ensure you’re aware of what's available.
Keeping Track of Branches in Collaborations
In collaborative development scenarios, being aware of remote branches helps streamline communications. It allows team members to see what others are working on, aligning efforts and avoiding duplicate work.
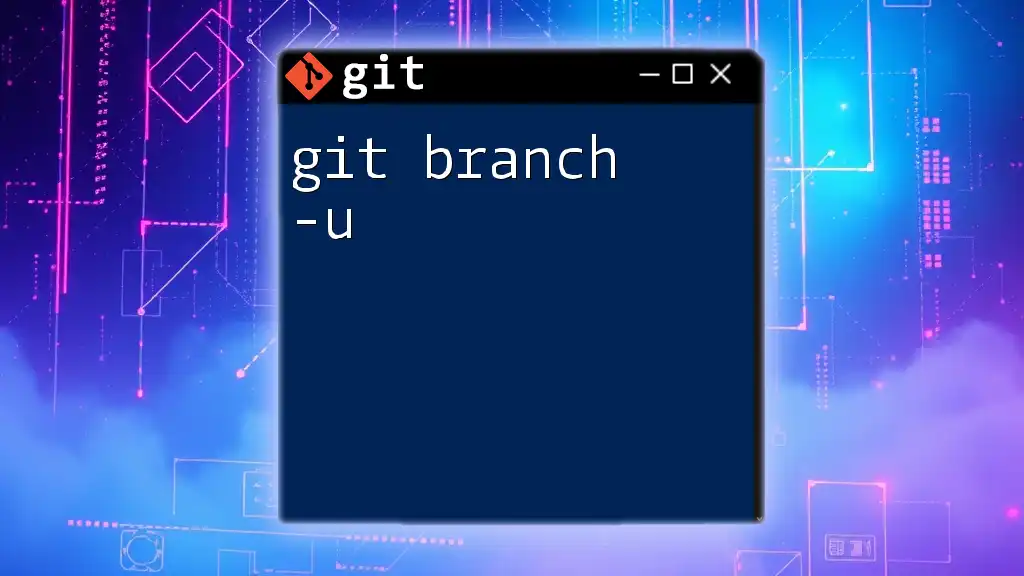
Best Practices
Regularly Fetching Updates
To ensure you have the latest information regarding remote branches, make it a habit to run:
git fetch
Performing this command frequently synchronizes your local repository with the remote, updating references so that `git branch -r` provides the most accurate views of remote branches.
Maintaining Clean Remote Branches
In a proactive approach to collaboration, it’s beneficial to regularly clean up stale branches on the remote. Communicate with your team about merging, deleting, or archiving outdated branches to keep the project repository organized and easy to navigate.
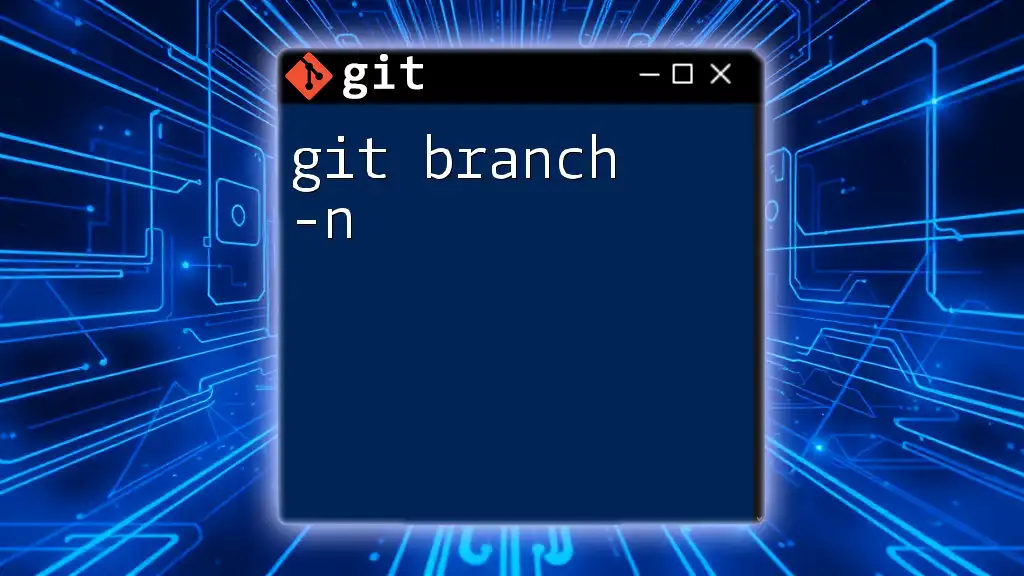
Conclusion
Understanding how to use `git branch -r` is essential for anyone working with Git, especially when collaborating in teams. This command is a powerful tool for gaining insight into remote branches and facilitating effective development practices. By incorporating it into your routine, you significantly enhance your Git workflow.
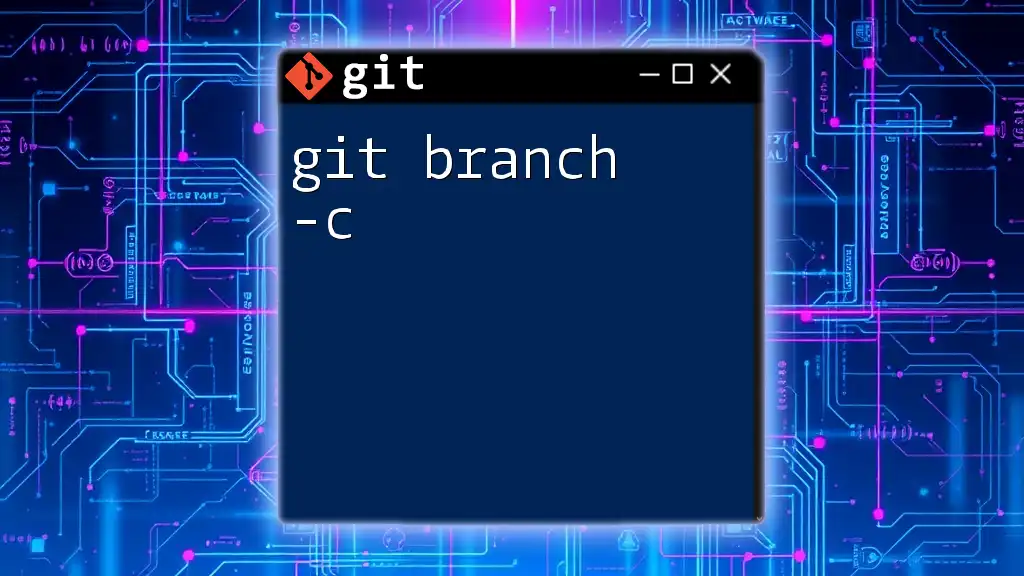
Additional Resources
Official Git Documentation
For further reading and in-depth explanations of all Git commands, visit the [official Git documentation](https://git-scm.com/doc).
Recommended Tutorials and Guides
Explore curated tutorials available through our platform for a more structured learning approach to mastering Git.
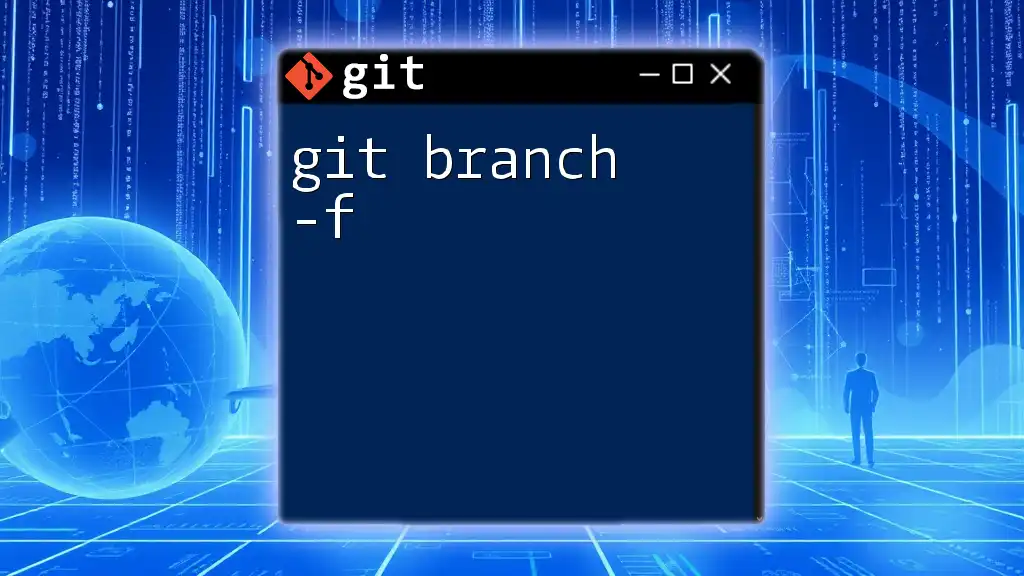
Call to Action
Start practicing with `git branch -r` in your projects today! Share your experiences and any questions in the comments below, and take your Git skills to the next level with our specialized courses.