The `git pull -f` command forcibly updates your local branch with the latest changes from the remote repository, potentially discarding local changes that conflict.
Here's the code snippet in markdown format:
git pull -f origin main
Understanding Git
What is Git?
Git is a powerful version control system widely used in collaborative software development. It allows multiple developers to work on the same project simultaneously, enabling effective tracking and management of changes in the source code. Git stands out for its flexibility, speed, and robust branching and merging capabilities, making it an essential tool for modern development workflows.
Basic Git Commands
To effectively utilize Git, it's crucial to understand some fundamental commands. Core commands such as `clone`, `commit`, `push`, and `pull` form the basis of daily operations. Each command serves a particular role in managing repositories:
- Clone: Creates a copy of a remote repository on your local machine.
- Commit: Saves your changes in the local repository.
- Push: Uploads your local changes to a remote repository.
- Pull: Fetches and integrates changes from a remote repository into your local branch.
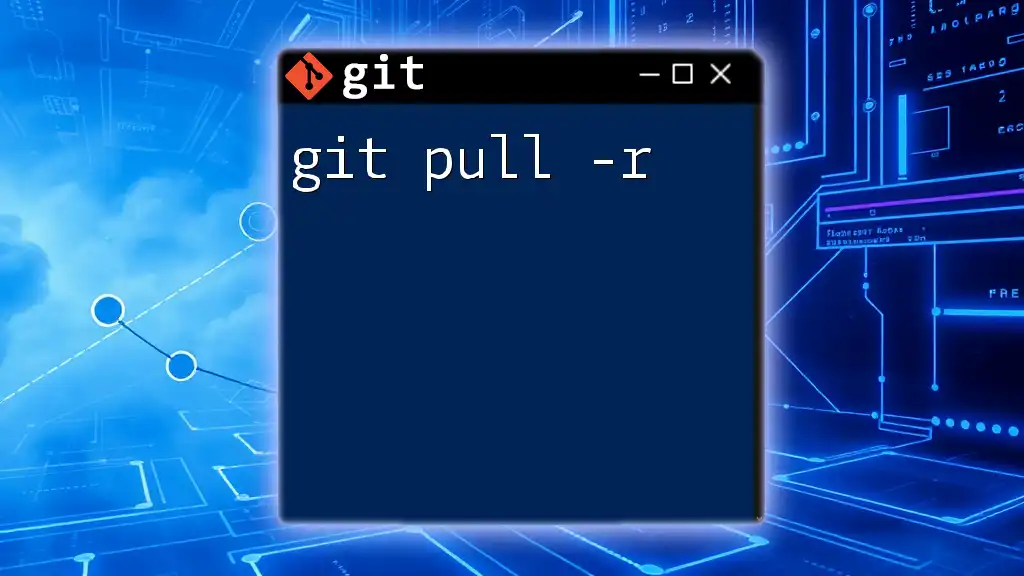
Introduction to `git pull`
What Does `git pull` Do?
At its core, `git pull` is a command that updates your local branch with changes from a remote repository. It automates the process of fetching the updates and merging them into your current working branch, ensuring that you have the latest code and are synchronized with your team.
It's important to note that `git pull` performs two steps:
- Fetch: Downloads new data from the specified remote repository.
- Merge: Integrates that data into your current branch.
Anatomy of the `git pull` Command
The full syntax for the `git pull` command is as follows:
git pull [<options>] [<repository> [<refspec>...]]
- <options>: Various flags (e.g., `--rebase`, `-f`, etc.).
- <repository>: The name of the remote repository (e.g., `origin`).
- <refspec>: The specific branch you want to pull changes from (e.g., `master`).
Understanding each component is essential to utilizing `git pull` effectively and ensuring that you’re only pulling updates that you need.
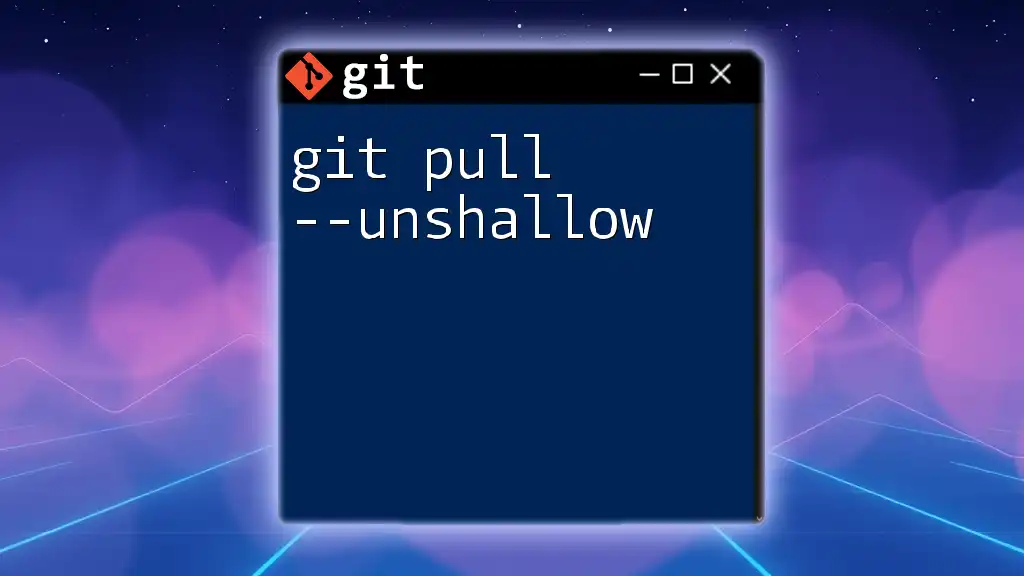
The Force Behind `git pull -f`
What is the `-f` Option?
The `-f` flag in `git pull -f`, shorthand for `--force`, instructs Git to override any local changes or conflicts that would normally prevent a successful merge. This option can be a double-edged sword; while it allows you to forcefully update your local repository, it can lead to the loss of crucial local modifications. Thus, using `-f` should always be approached with caution.
How `git pull -f` Differs from Standard `git pull`
Using `git pull -f` bypasses the usual protections against conflicts. When the current branch has diverged from the upstream branch (meaning your local changes conflict with what's on the remote), a standard `git pull` will typically result in an error message or provide you with a chance to resolve the conflict manually. However, with `git pull -f`, Git will automatically take the remote changes, effectively discarding your local changes in the process.
This makes `git pull -f` an appropriate choice in specific situations but carries inherent risks that users must be aware of.
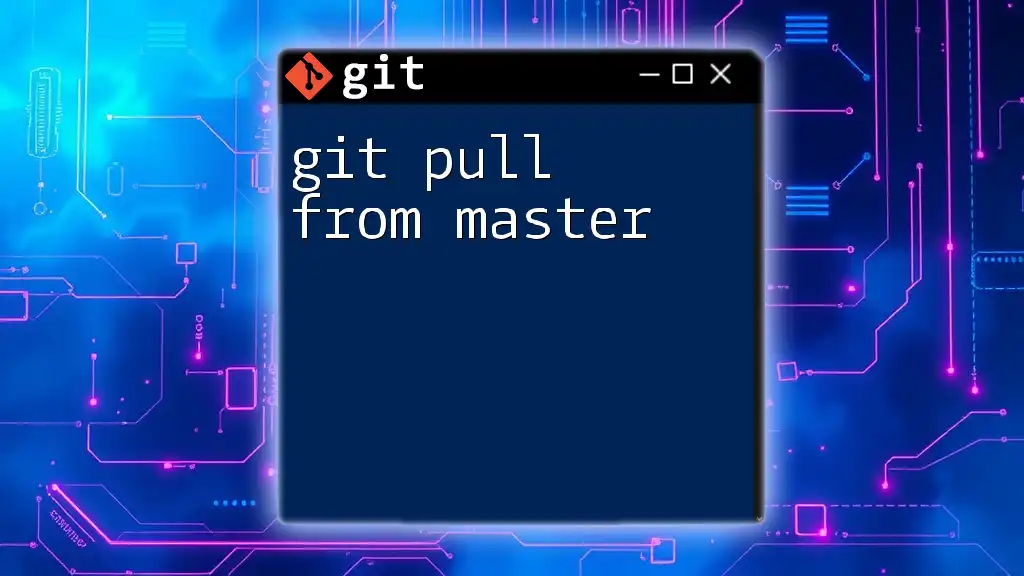
Practical Applications
When to Use `git pull -f`
There are several scenarios where using `git pull -f` is warranted:
- Recovering from Conflicts: If a merge went awry and you need the latest updates from the remote branch, forcing the pull can be a way to reset the local state.
- Cleaning Up Unwanted Changes: If you've made experimental changes that you no longer want to keep, a force pull will clear the slate and bring in the latest code from your team.
Important Note: Be aware that using this command will erase any uncommitted changes in your local copy!
Example Scenarios
Let’s consider a couple of practical examples to illustrate the use of `git pull -f`:
Example 1: You are working on a feature branch and need to incorporate the latest updates from your team. If your local branch has diverged from the remote, you can run:
git pull -f origin feature-branch
This command forces your local feature branch to align with the remote version, disregarding any conflicts that may arise from your local changes.
Example 2: If you find yourself needing to recover from a messy merge and just want to ensure your local copy matches the repository's master branch, you can use:
git pull -f origin master
This approach provides a clean slate, allowing you to start fresh with the latest codebase.
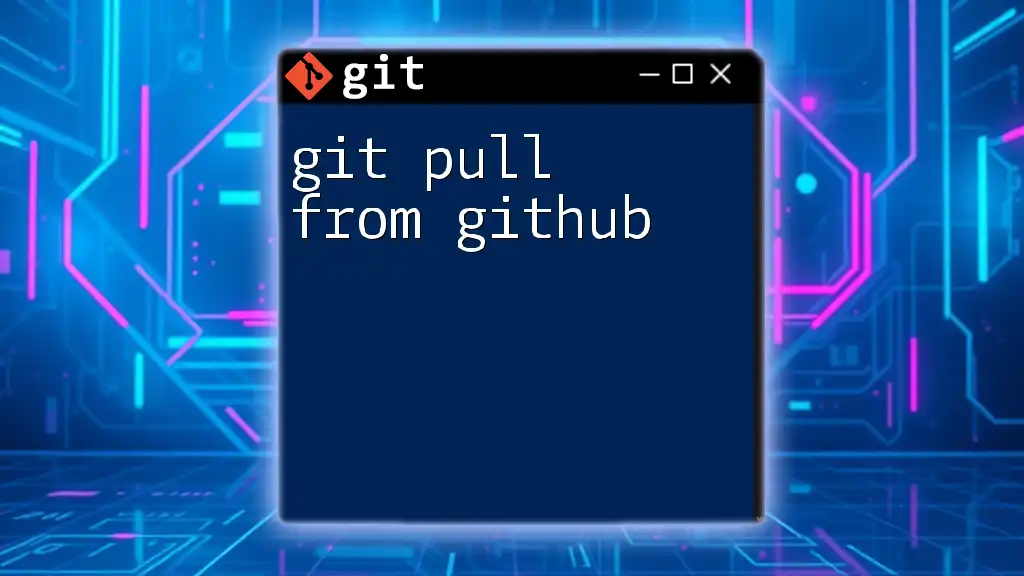
Risks and Precautions
Potential Consequences of Force Pulling
While `git pull -f` can be immensely useful, it comes with significant risks, primarily the potential loss of local changes and commits. If you haven't committed your work, these changes will vanish without a trace, which can lead to frustrations and setbacks.
Best Practices Before Using `git pull -f`
To minimize risks when considering a forced pull, adhere to the following best practices:
- Commit Local Changes: Always commit your changes before executing a forced pull.
- Use Git Stash: If you're unsure about your local changes, you can temporarily save them using:
git stash
This command saves your modifications and provides you an opportunity to pull the latest updates without losing anything.
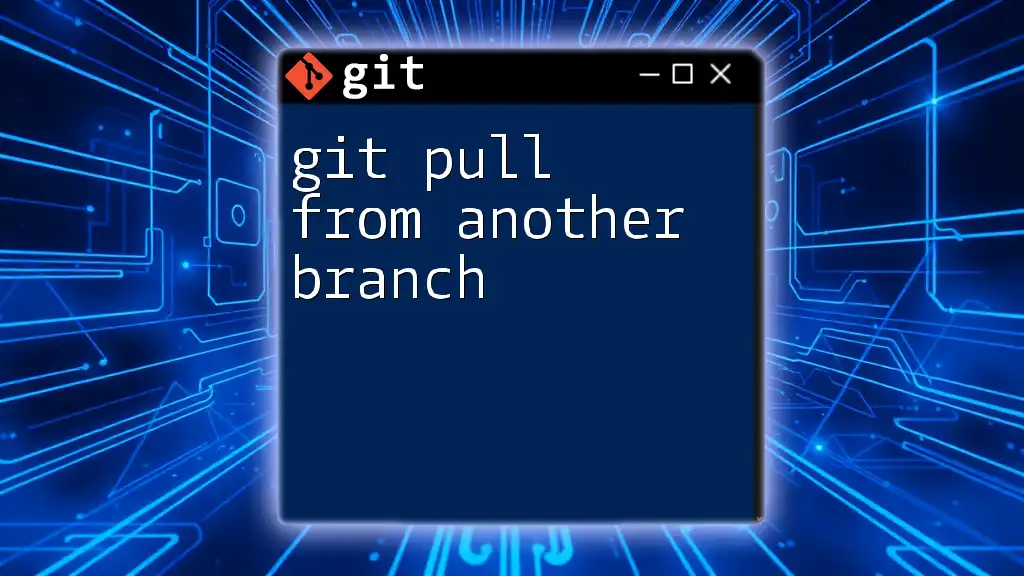
Conclusion
Summary of Key Points
The `git pull -f` command is a powerful tool for syncing your local repository with a remote version while ignoring local conflicts. This command can be a boon in specific contexts but carries a significant risk of losing local modifications.
Final Thoughts
Git's flexibility empowers developers to handle various situations efficiently, but it also demands a careful approach, especially when it involves forced commands. Mastering the nuances of `git pull -f` can greatly enhance your Git skills, making collaboration smooth and productive.
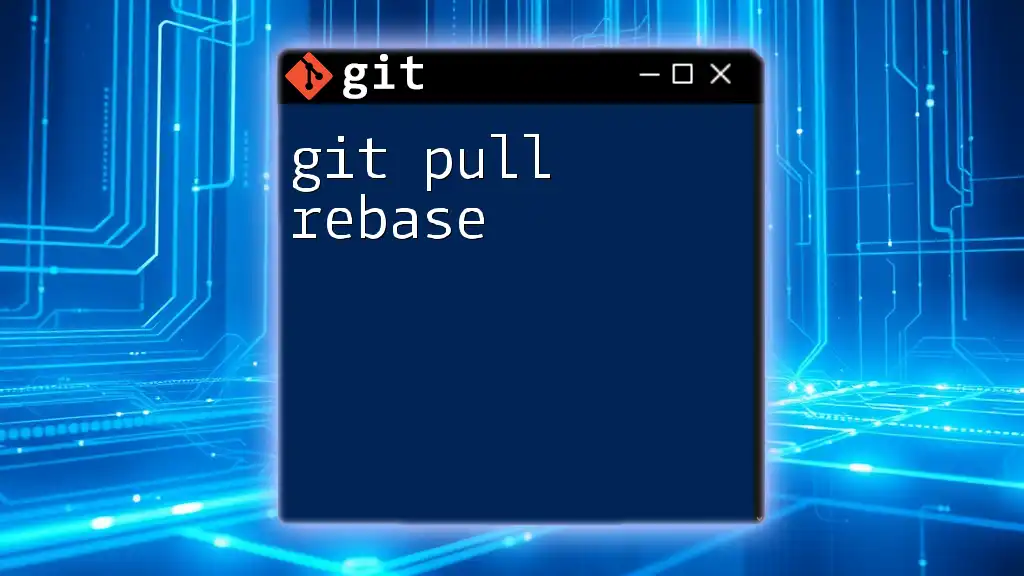
Further Resources
Recommended Readings
For a deeper understanding of Git commands, consider exploring the official [Git Documentation](https://git-scm.com/doc) and related literature that provides extensive insights into version control best practices.
Practice Exercises
To solidify your understanding of `git pull -f`, practice using it in a test repository where you can afford to lose changes. Experiment with different scenarios, such as merging branches and resolving conflicts, to build confidence in your Git abilities.
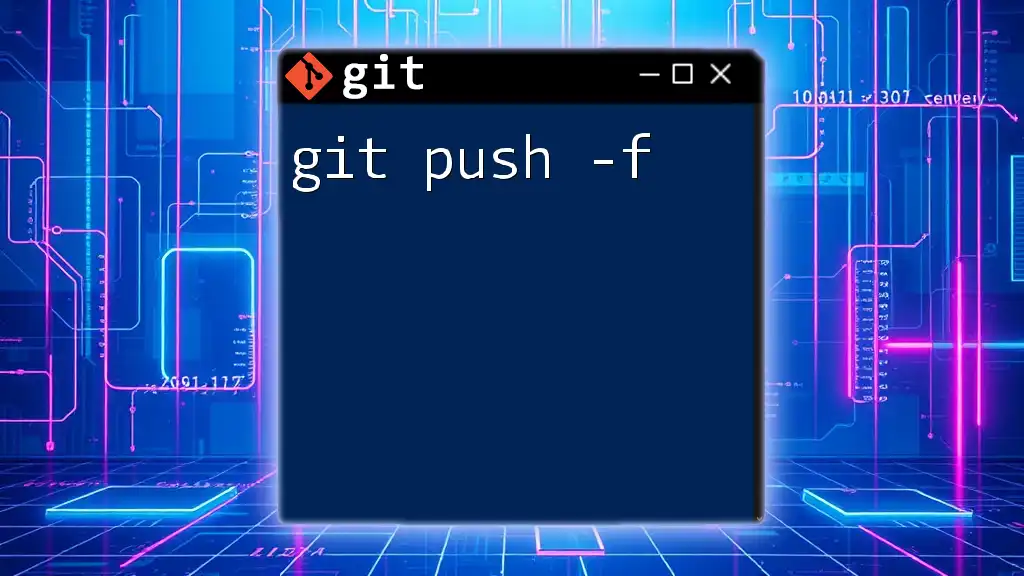
Frequently Asked Questions
Common Questions About `git pull -f`
Many users are wary of using the force option due to its consequences. Common concerns include losing local changes or inadvertently affecting collaborative work. Understanding its proper use can alleviate these worries.
Troubleshooting `git pull -f`
If you encounter issues when using `git pull -f`, such as merge conflicts or unexpected results, consider reviewing your Git history and local changes to identify potential problems before forcing a pull.