The `git rebase -i --root` command allows you to interactively rebase all commits in a repository, starting from the very first commit, enabling you to edit, squash, or reorder them as needed.
git rebase -i --root
Understanding Git Rebase
Git rebase is a powerful command in version control, primarily used to integrate changes from one branch into another. It allows developers to streamline their project history by applying commits one after another, providing a cleaner, linear progression of changes. Unlike merging, which creates a new commit for the integration, rebasing rewrites commit history, making it look as if all changes were made sequentially.
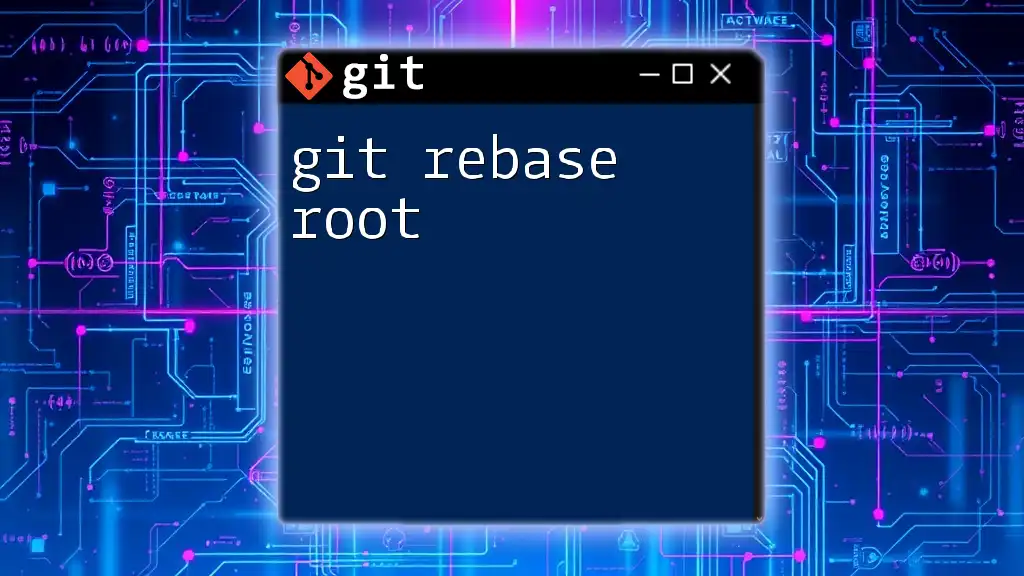
Understanding the `--root` Option
The `--root` option allows you to rebase starting from the very first commit—hence the name "root." This capability is invaluable when you need to modify the entire history of your project. Using `git rebase -i --root` gives you the flexibility to clean up the entire project history effectively. It's especially beneficial in projects where initial commits need adjustments or when you want a more coherent commit history.
When to Use `git rebase -i --root`
Utilize `git rebase -i --root` when:
- You want to rewrite the commit history from the very first commit.
- You need to clean up commit messages or consolidate multiple commits into one.
- Your project has diverged significantly, and you want to make it look cohesive by combining or modifying commits from the beginning.
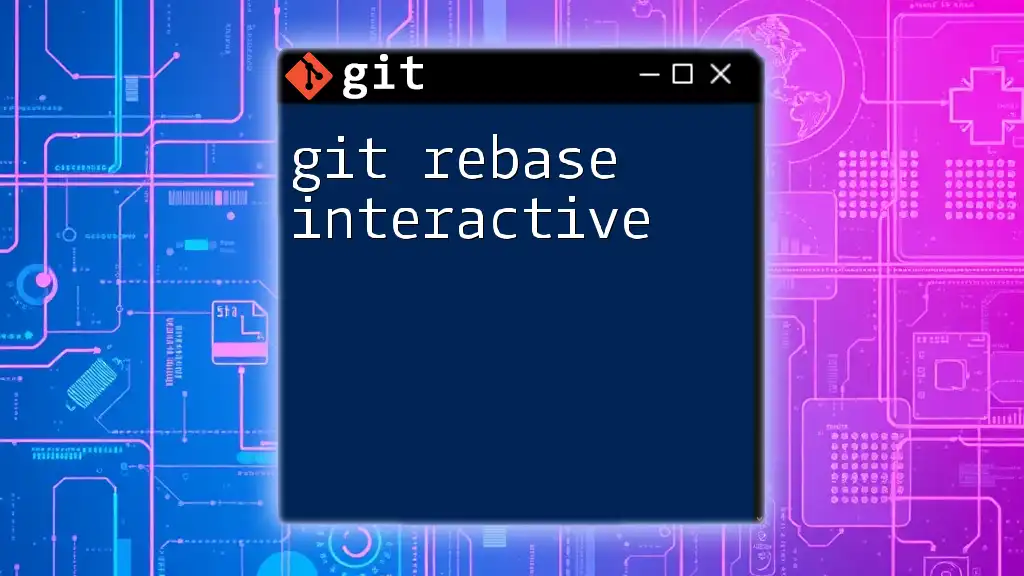
Setting Up Your Repository for Rebasing
To start rebasing interactively from the root, you'll first need to set up a sample Git repository for practice.
Initial Configuration
Begin by installing Git if you haven't already. After installation, open your terminal and configure your Git environment with these basic commands:
git config --global user.name "Your Name"
git config --global user.email "you@example.com"
Creating a Sample Repository
Next, create a sample repository:
mkdir sample-repo
cd sample-repo
git init
Committing Changes for Rebase
Now, create a few commits for demonstration. Here’s a simple sequence:
echo "Initial Commit" > file.txt
git add file.txt
git commit -m "Initial commit"
echo "Adding some content" >> file.txt
git commit -m "Add content"
echo "Updating content" >> file.txt
git commit -m "Update content"
You now have three commits in your repository to work with.
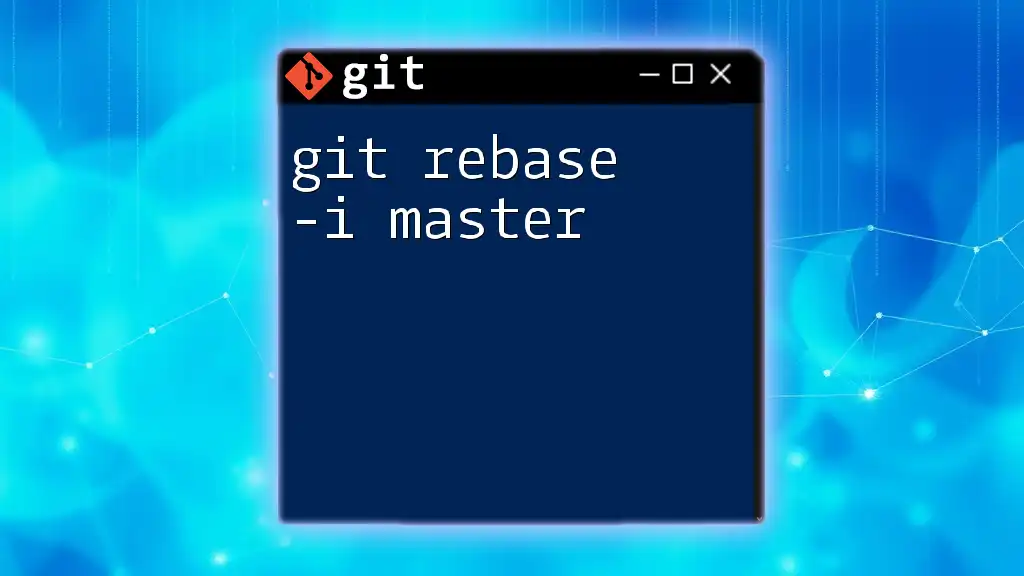
Performing an Interactive Rebase
What is Interactive Rebase?
Interactive rebase allows you to edit commits as they are replayed onto your branch. Unlike a standard rebase, which applies all commits without alteration, interactive rebasing provides total control over your project's commit history.
Running the Command
To initiate the interactive rebase from the root, execute:
git rebase -i --root
This command will open your default text editor, displaying a list of your commits.
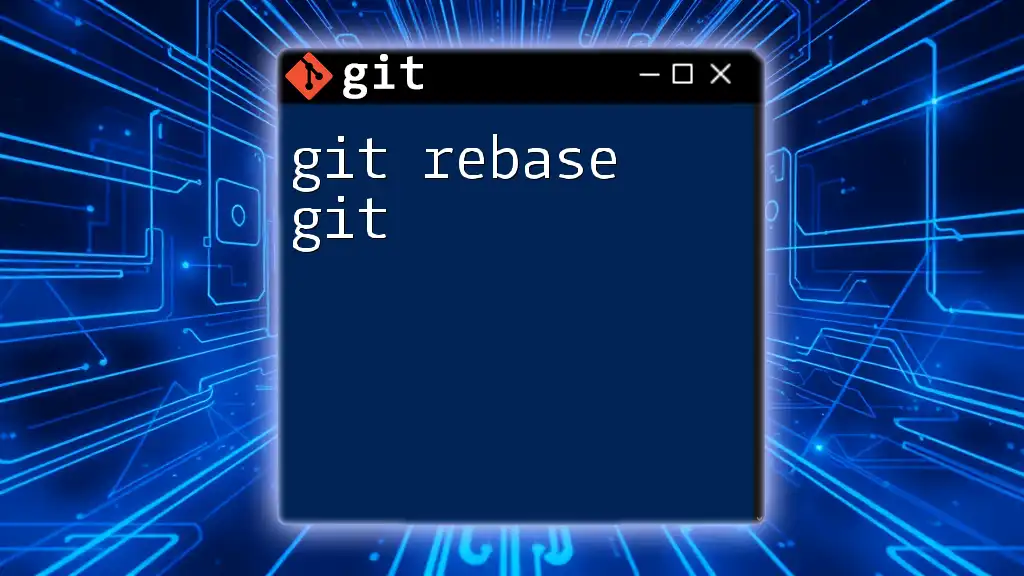
The Interactive Rebase Interface
Understanding the TODO List
When you enter the interactive rebase, you will see a TODO list that shows all your commits in chronological order. Each line contains the action (like `pick`) and the commit hash with its message.
Common Commands in the TODO List
- `pick`: Use the commit as it is.
- `squash`: Combine this commit with the previous one.
- `edit`: Edit this commit message or contents.
- `fixup`: Like `squash`, but discard the commit message.
Example of a TODO List
Here’s what a sample TODO list might look like:
pick 123456a Initial commit
squash 654321b Add detailed README
edit abcdef0 Fix typo in README
In this example, you can see how the second commit will be combined with the initial commit, and the third commit is marked for editing.
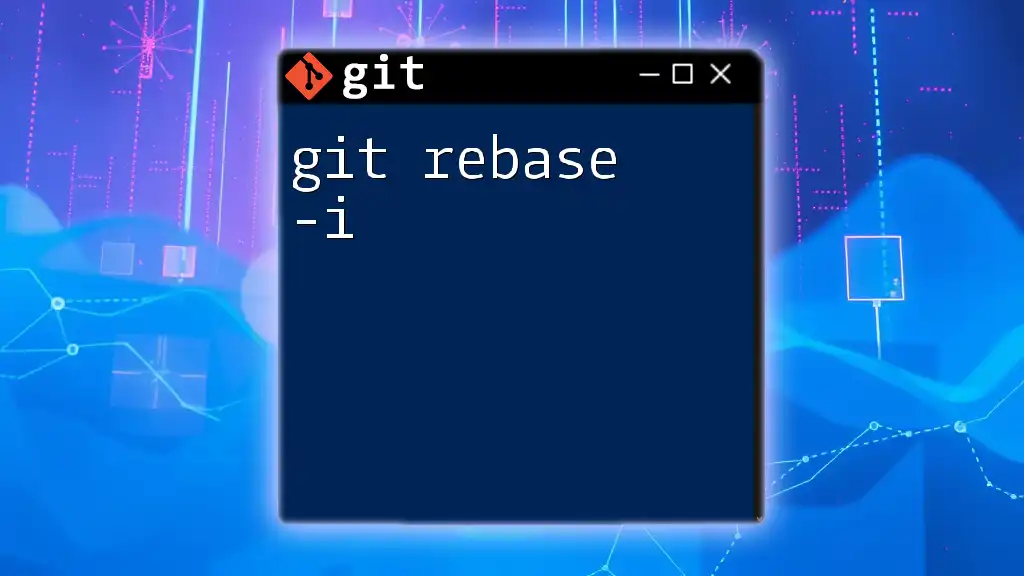
Modifying Commits During the Rebase
Changing Commit Messages
You can modify commit messages by changing `pick` to `edit` in the TODO list. When you save and exit, Git will pause the rebase process, allowing you to amend the commit. Run:
git commit --amend -m "Updated initial commit message"
Once done, continue the rebase with:
git rebase --continue
Squashing Commits
To squash commits, simply change `pick` to `squash` for the commits you want to merge. After saving, Git will prompt you to combine commit messages. You can choose which messages to keep or write a new one.
Dropping Commits
If you wish to drop a commit, change `pick` to `drop` in the TODO list. This will remove that specific commit from history, allowing you to simplify your project logs as needed.
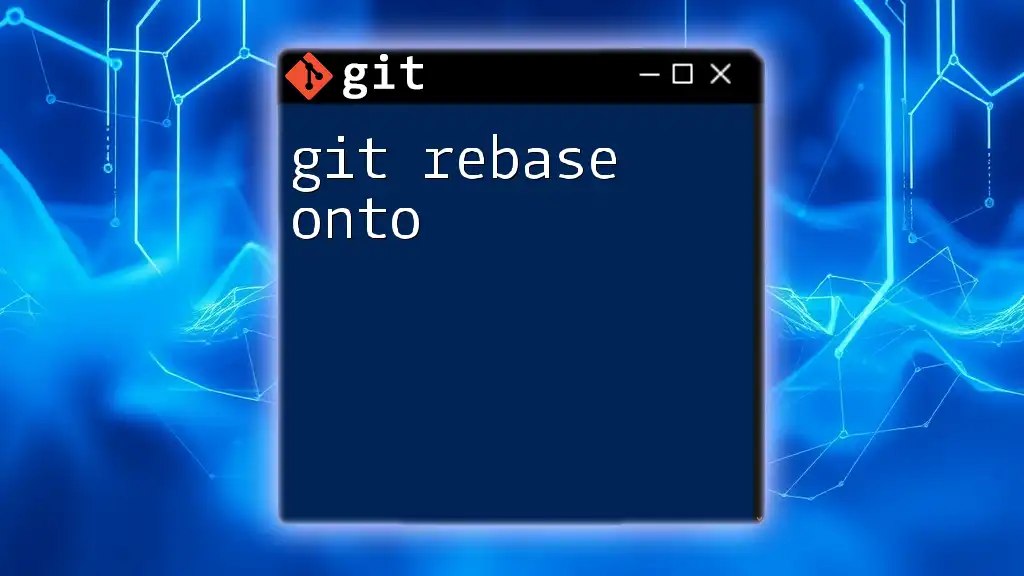
Resolving Conflicts During the Rebase
As with any rebasing operation, you may run into conflicts. If this occurs, Git will pause the process, notifying you of the conflicting files. Resolve any issues in those files, and then mark them as resolved with:
git add <conflicted-file>
You can then continue the rebase:
git rebase --continue
If you find that the rebase is causing more issues than it's worth, you can always abort and return to your pre-rebase state:
git rebase --abort
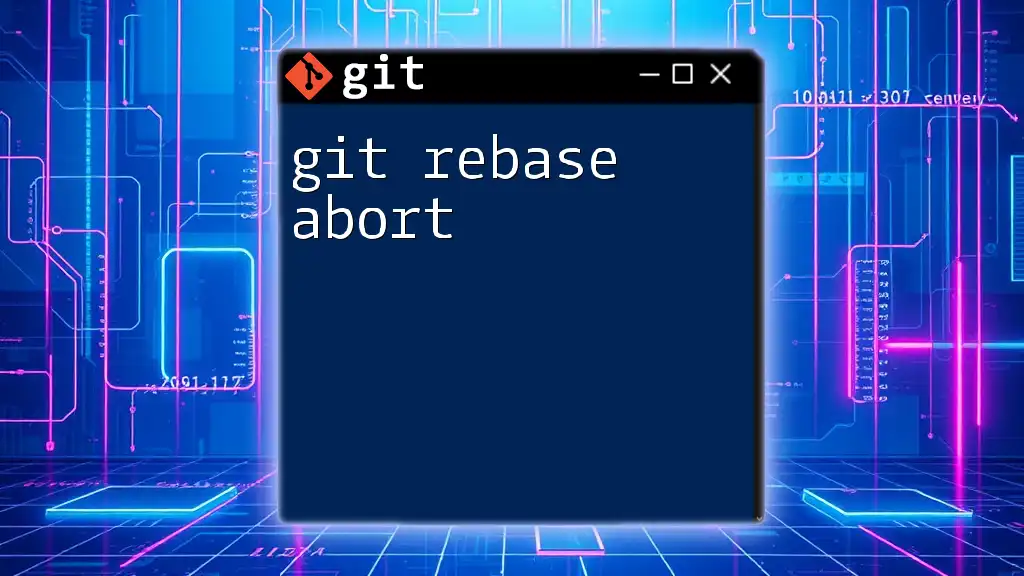
Finalizing the Rebase
After resolving conflicts and editing commit messages, it's crucial to finalize the rebase. Execute:
git rebase --continue
This command replays the remaining commits according to your configuration in the TODO list. Lastly, if your history has diverged from a remote repository, remember to push your changes:
git push origin <branch> --force
Note: Using `--force` can overwrite history on the remote, so ensure you're in sync with other collaborators.
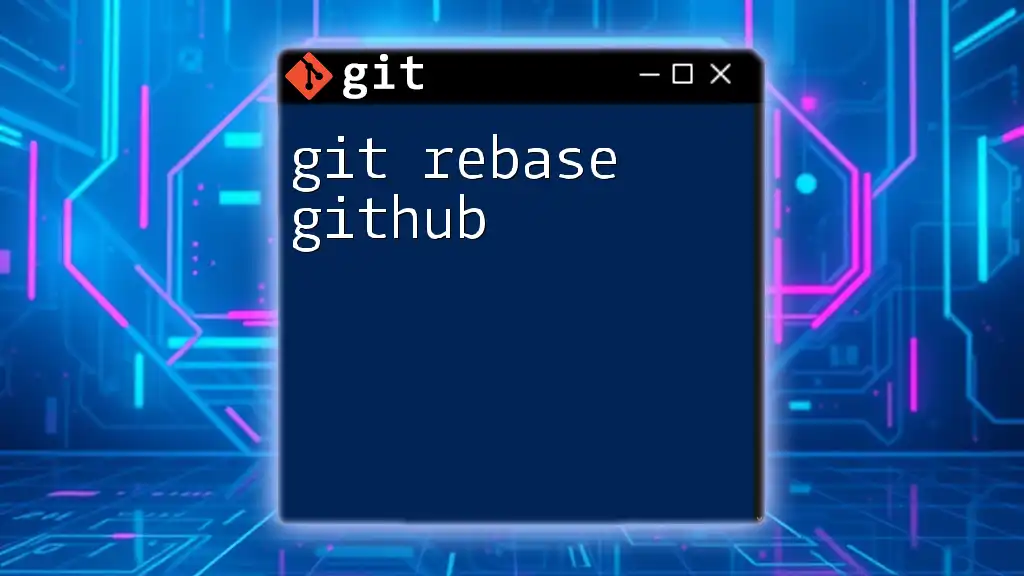
Best Practices for Using `git rebase -i --root`
When using `git rebase -i --root`, it’s essential to consider the following best practices:
- Use with Caution: Because rebasing rewrites history, make sure you fully understand the implications.
- Collaborate and Communicate: Ensure your team knows you are rewriting history to avoid confusion.
- Utilize for Cleanup: Use this technique primarily for cleaning up commit history on branches that are not shared.
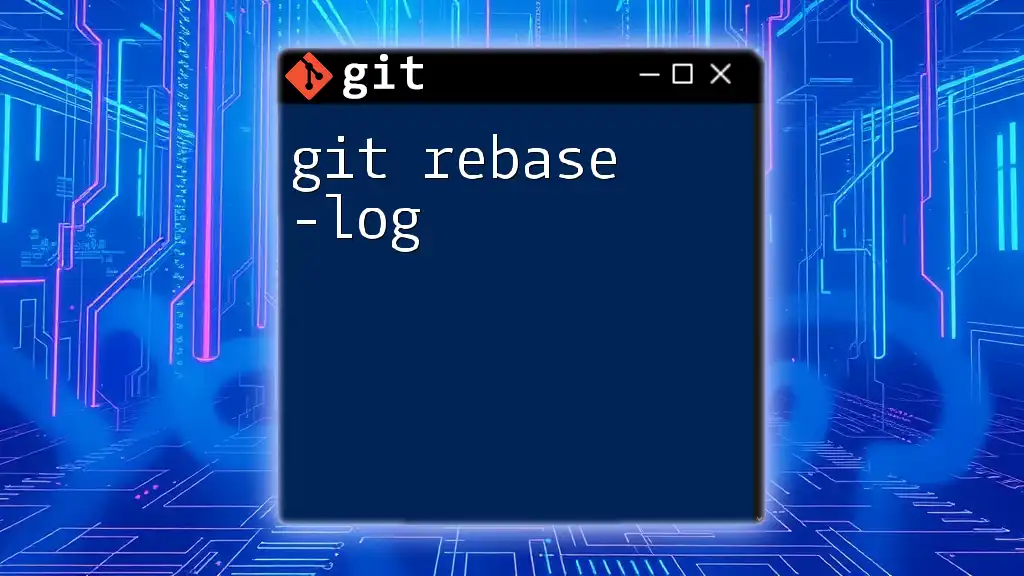
Conclusion
In conclusion, `git rebase -i --root` is a potent tool for developers looking to streamline their commit history from the beginning of their project. With the ability to squash, edit, and drop commits, you can create a clean and coherent project history that reflects your development process. Try using it in your next project and observe how it transforms your workflow!