Git skills refer to the essential commands and techniques needed to effectively manage version control and collaborate on software projects using Git.
Here's a simple example of a git command to check the status of your repository:
git status
Core Git Concepts
Understanding Repositories
Local vs Remote Repositories
To grasp Git skills effectively, it's essential to understand the difference between local and remote repositories. A local repository is stored on your own machine, providing you with a workspace to manage your files and revisions. In contrast, a remote repository is a hosted version of your project, often located on platforms like GitHub, Bitbucket, or GitLab.
To create a local repository, you initiate it in a directory using the command:
git init
For working with existing projects, you can clone an entire remote repository using:
git clone [repository-url]
Branching and Merging
What are Branches?
Branches are a fundamental feature in Git that allow you to develop features, fix bugs, or experiment within isolated environments. This separation enables parallel development without affecting the main codebase. To create a new branch, you can use:
git branch [branch-name]
For example, if you’re working on a new feature called `feature-x`, you can create and switch to it like this:
git checkout -b feature-x
Merging Branches
Once your work is complete, you can integrate your changes back into the main branch (often called main or master) by merging. Use the following command:
git merge [branch-name]
However, merging doesn’t always go smoothly. Merge conflicts can occur if changes are made on both branches in the same area of the code. In such cases, Git will pause the process and highlight the conflicting files, allowing you to resolve the issues manually.
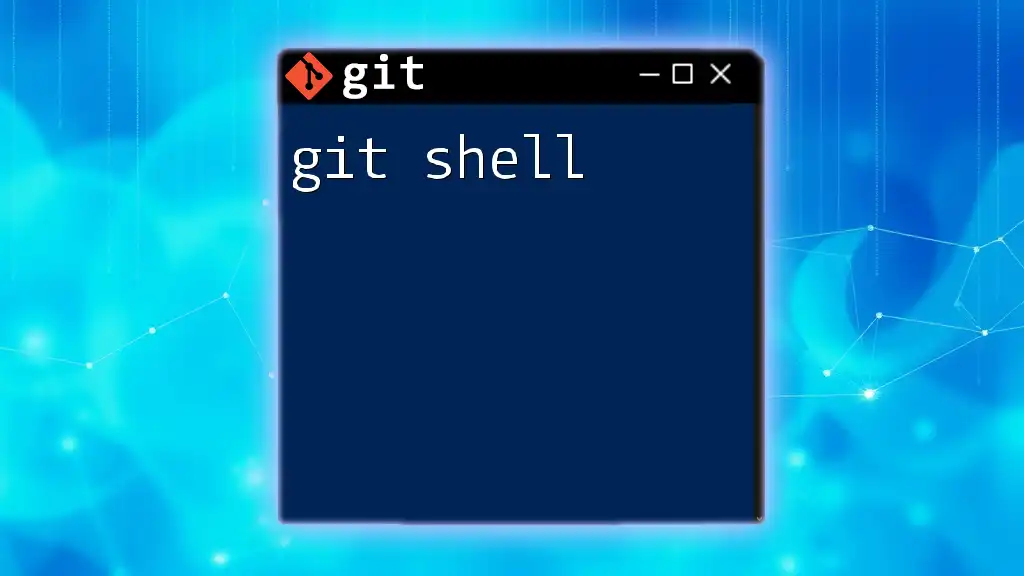
Essential Git Commands
Setting Up Git
Configuring User Information
Before diving deep into your Git skills, you must configure your user information. This ensures that all your commits are correctly attributed to you. Run the commands:
git config --global user.name "Your Name"
git config --global user.email "your.email@example.com"
Proper configuration helps maintain an organized and traceable history of contributions.
Basic Commands
Cloning a Repository
To work on an existing project, cloning is your first step. It downloads the project files and history from a remote repository to your local machine. You perform this action by executing:
git clone [repository-url]
Checking Status and Changes
Understanding `git status` and `git diff`
Using `git status` helps you understand the current state of your working directory, showing which files are staged, modified, or untracked. You can execute:
git status
If you want to see the actual changes made to your files, the `git diff` command allows you to view a line-by-line comparison between your working directory and the last commit.
Staging and Committing Changes
Staging Files
Before committing any changes, you must stage your files. This action prepares your selected changes to be included in the next commit. Use:
git add [file-name]
or to stage all changes:
git add .
Making Commits
After staging, you can commit your changes with an appropriate message that explains the context of the modifications:
git commit -m "Your commit message here"
Crafting meaningful commit messages is a vital Git skill; they help others understand the changes and improve project documentation.
Viewing Commit History
Exploring the Commit Log
You can view the history of your commits using the `git log` command, which provides a detailed record of every commit made in your repository:
git log
For a more concise view, you might use:
git log --oneline
This presents your commit history in a simplified format, aiding in quickly reviewing what has happened in your project.
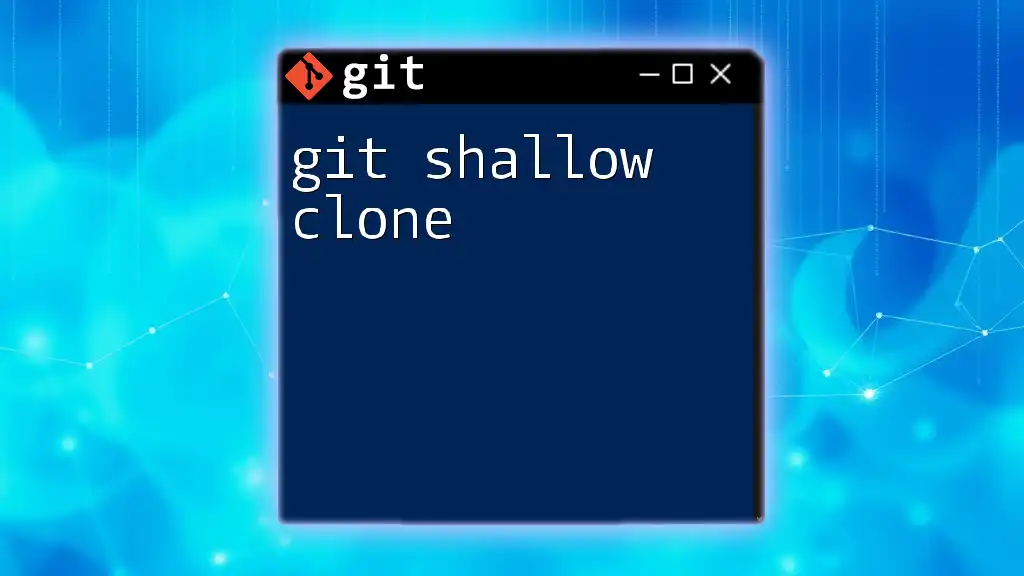
Advanced Git Skills
Conflict Resolution
Understanding Merge Conflicts
As you collaborate with others or even work independently on complex features, you may encounter merge conflicts. These occur when two branches have changes to the same line of a file and cannot be automatically merged by Git. To resolve conflicts, Git can leverage a merge tool. You can invoke it using:
git mergetool
This command opens a tool that assists you in resolving conflicts by providing a visual comparison of changes.
Using Rebase
What is Rebasing?
Rebasing is a powerful technique that allows you to integrate changes from one branch into another by moving the entire branch to a new base point. Unlike merging, which preserves all commit history, rebasing creates a linear project history. To rebase your current branch onto another branch, use:
git rebase [branch-name]
This process can help keep a clean and understandable history, which is advantageous when collaborating with teams.
Tags in Git
Creating and Using Tags
Tags are essential for marking specific points in your repository's history, often used to signify release versions. You can create a tag with the following command:
git tag -a v1.0 -m "Version 1.0"
This labels your current commit with a version identifier, making it easy to reference important milestones in the project.
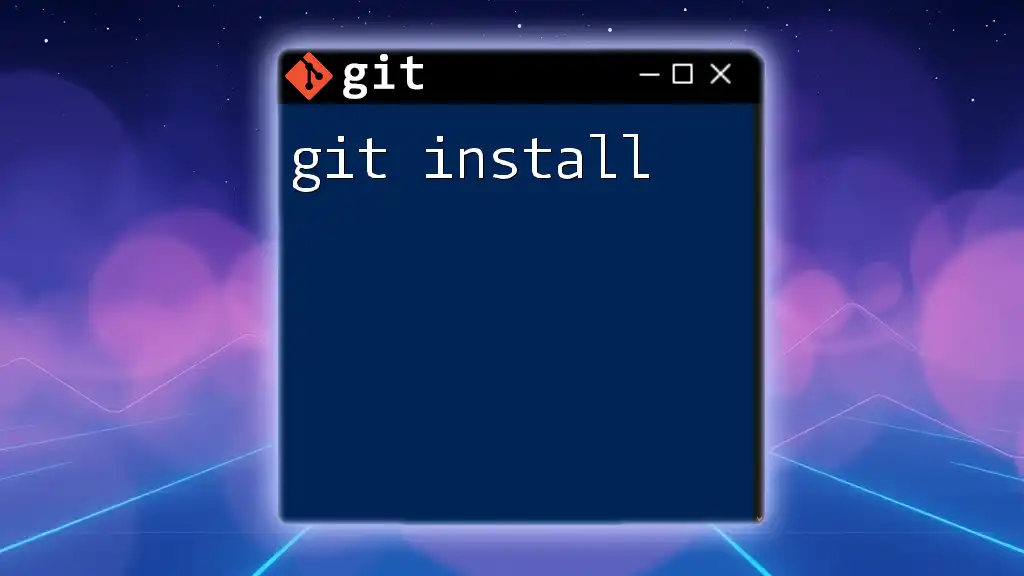
Best Practices for Version Control
Commit Best Practices
Writing Effective Commit Messages
A well-structured commit message comprises a short, informative subject line followed by a detailed explanatory body if necessary. This practice enhances communication within your team and simplifies code reviews.
Branching Strategies
Popular Branching Models
Utilizing structured branching strategies can significantly improve your workflow. The Git Flow model is suited for larger projects with versioned releases and well-defined roles. Conversely, GitHub Flow is more streamlined, focusing on simplicity, making it ideal for continuous deployment scenarios.
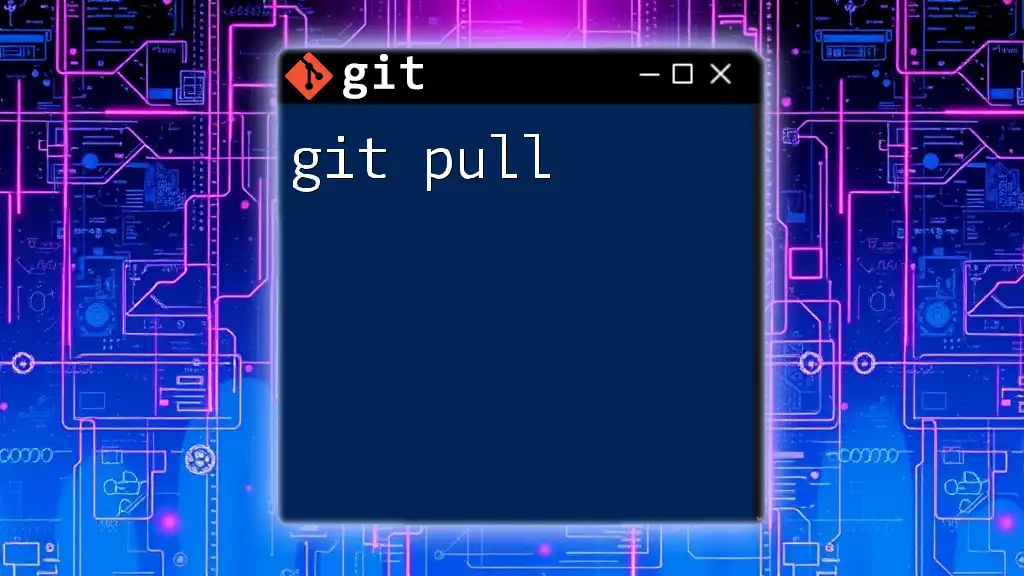
Collaborating with Others
Remote Collaboration
Adding Remote Repositories
Collaboration often involves connecting to a remote repository. You can add a remote by executing:
git remote add origin [repository-url]
This command links your local repository to the remote one, enabling seamless sharing of changes.
Pushing and Pulling Changes
Commands for Changing Synchronization
Once you’ve made your contributions locally, pushing those changes to a remote repository is essential. You can accomplish this by using:
git push
Conversely, to retrieve updates made by others, use:
git pull
These commands allow your local repository to stay synchronized with the evolving remote project.
Pull Requests and Code Reviews
The Importance of Pull Requests
When working collaboratively, requesting feedback on your changes through pull requests is essential. This process encourages code reviews, maintaining code quality while ensuring a collaborative mindset. Most platforms like GitHub and GitLab provide intuitive interfaces for creating and managing pull requests.
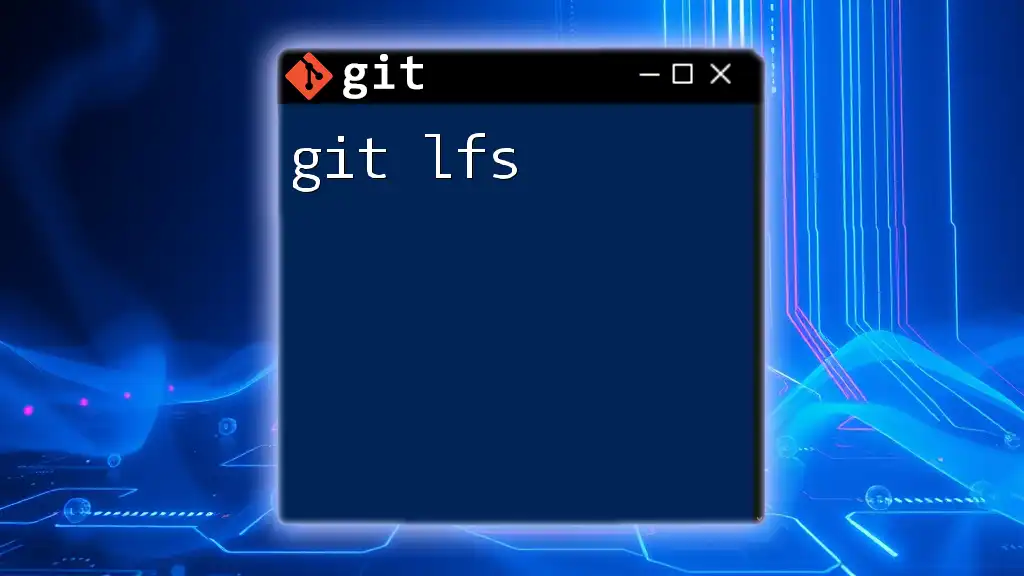
Conclusion
In conclusion, mastering Git skills is vital for any developer or team working on code projects. Continuous engagement with Git practices, community resources, and learning from peers will not only improve your expertise but also positively influence your software development workflow. Embrace the journey, expand your Git skills, and contribute effectively in your projects.
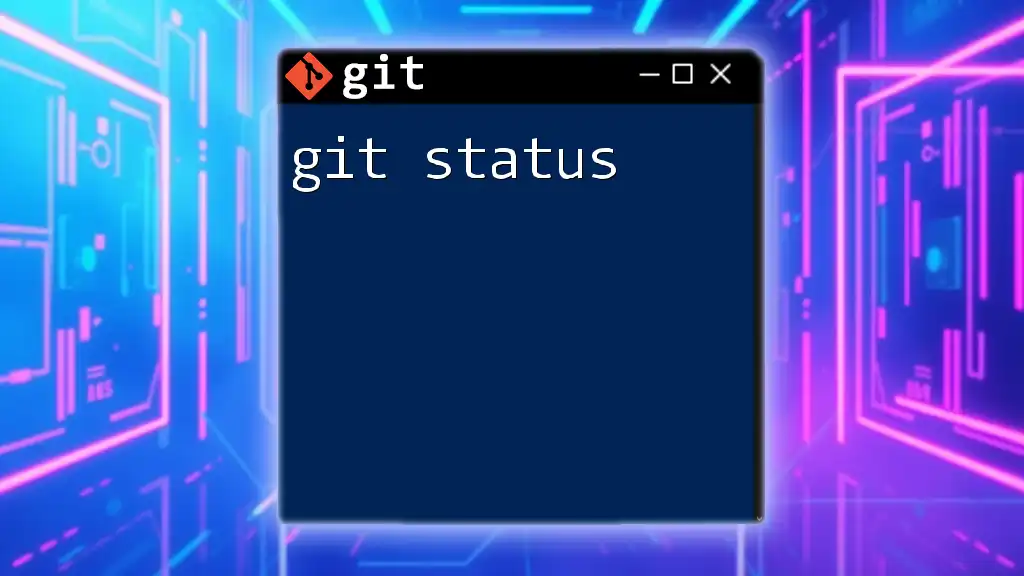
Additional Resources
To further enhance your Git skills, refer to online tutorials, official Git documentation, and recommended courses. Engaging with Git communities will also provide support and insights from both beginners and seasoned developers. Keep practicing and refining your skills, as the benefits of mastering Git are immeasurable in today's collaborative and fast-paced development environment.