"Git LLVM refers to the integration of Git with the LLVM project, allowing developers to manage their LLVM-related code repositories efficiently using Git commands."
git clone https://github.com/llvm/llvm-project.git
What is LLVM?
Understanding LLVM Architecture
LLVM (Low-Level Virtual Machine) is a powerful suite of modular and reusable compiler and toolchain technologies. It provides a framework for developing compilers and supports various programming languages. The architecture of LLVM consists of multiple components, including:
- LLVM Core: It offers the infrastructure necessary for optimization and code generation.
- Intermediate Representation (IR): A language-agnostic representation that allows for various levels of optimization.
- LLVM Backends: These convert the IR into machine code tailored for specific hardware.
Key Features of LLVM
LLVM is notable for its high performance and flexibility. Key features worth mentioning include:
- Optimization: LLVM performs numerous optimizations that improve the execution speed and reduce resource consumption.
- Code Generation: It supports multiple architectures, making it suitable for cross-platform development.
- Modularity: Developers can utilize only the components they need, promoting reusability.
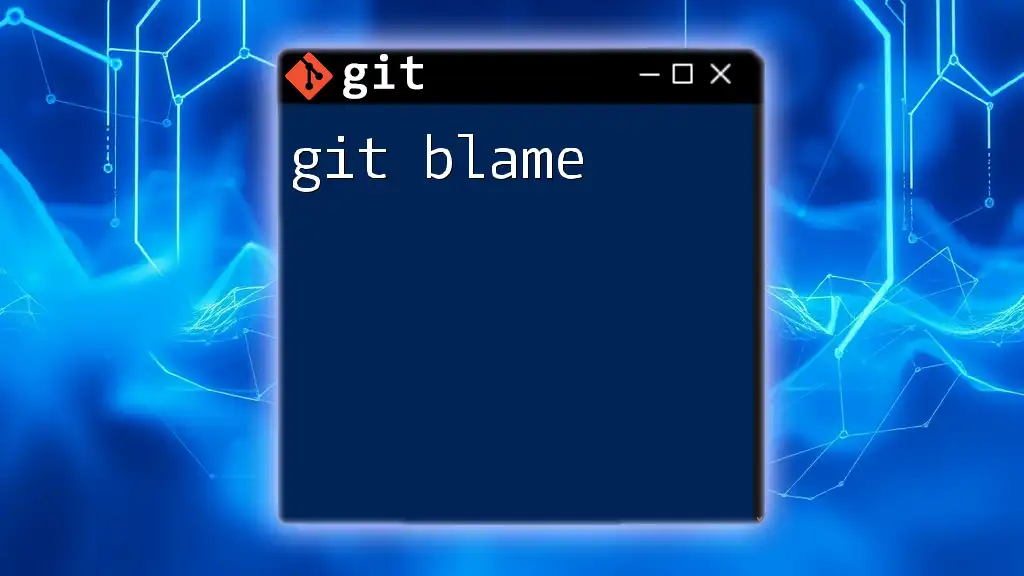
Why Use Git with LLVM?
Benefits of Version Control in LLVM Projects
Version control systems like Git are crucial in managing changes over time. By using Git with LLVM, you can effectively track modifications to the codebase, which is particularly important in extensive projects where multiple developers may contribute simultaneously. Version control aids in the recovery of previous versions, ensuring that you can revert to a stable state if needed.
Collaboration Among Developers
In collaborative projects, Git enhances teamwork by simplifying the process of sharing code. Developers can push their features or bug fixes to a central repository, which reduces conflicts and promotes an organized development workflow. Git’s branching feature allows developers to work on separate features without interfering with one another.
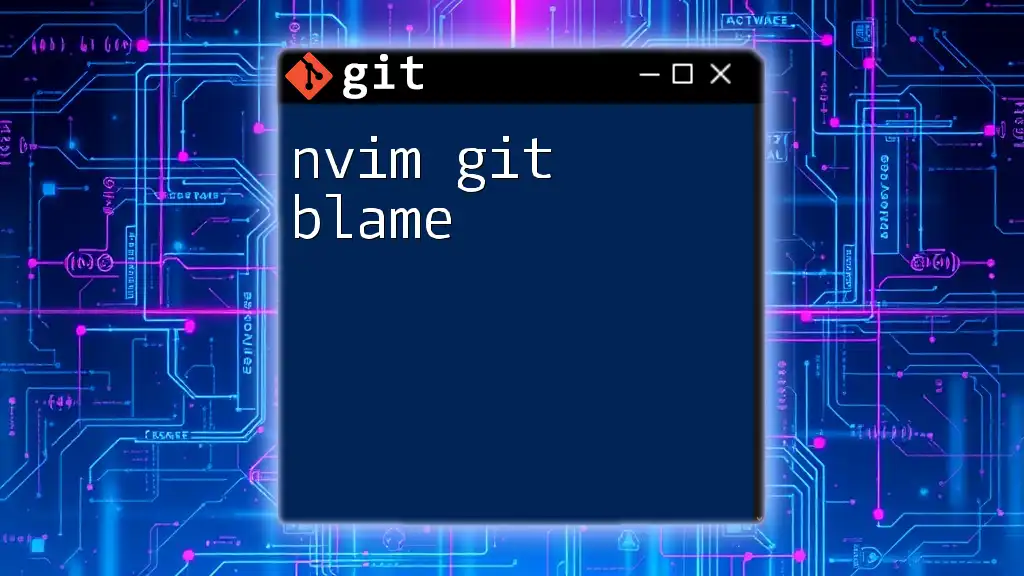
Setting Up Your Environment
Installing Git and LLVM
Before you begin using Git with LLVM, it's essential to have both tools installed on your system. Here’s how to install Git for various operating systems:
-
For Ubuntu:
sudo apt-get install git
-
For macOS:
brew install git
-
For Windows: Download and install Git from the official website.
After installing Git, you will need to install LLVM. You can follow the instructions provided by LLVM’s official documentation for platform-specific details.
Configuring Git for Your LLVM Project
Once Git is installed, it’s vital to configure your user information so that your commits are correctly attributed. You can set this up with the following commands:
git config --global user.name "Your Name"
git config --global user.email "you@example.com"
Configuring your identity ensures that your contributions are recorded accurately whenever you commit changes to your Git repository.
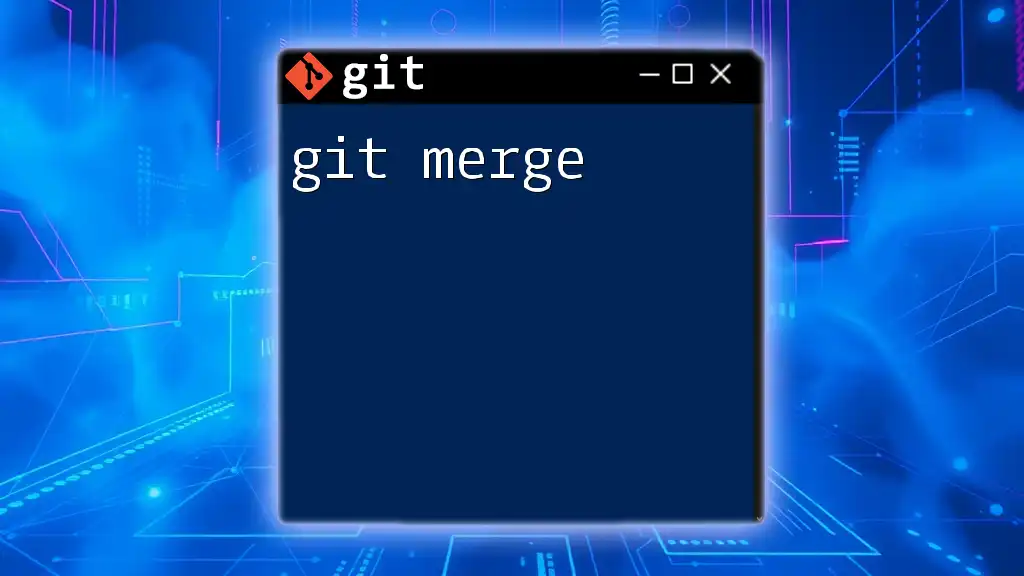
Creating a New LLVM Project with Git
Initializing a Git Repository
To start a new LLVM project, you first need to create a new directory and initialize a Git repository within it. Here’s how you can do this:
-
Create a new directory for your project:
mkdir my-llvm-project
-
Navigate into your project directory:
cd my-llvm-project
-
Initialize a new Git repository:
git init
Structuring Your LLVM Project
Organizing your LLVM project correctly can save time and effort. Here are best practices for structuring your project:
- src/: Place all your source files here.
- include/: For header files.
- tests/: Include unit tests in this folder.
- CMakeLists.txt: For managing build configurations using CMake.
Proper organization makes your project easier to understand and maintain, especially when working in teams.
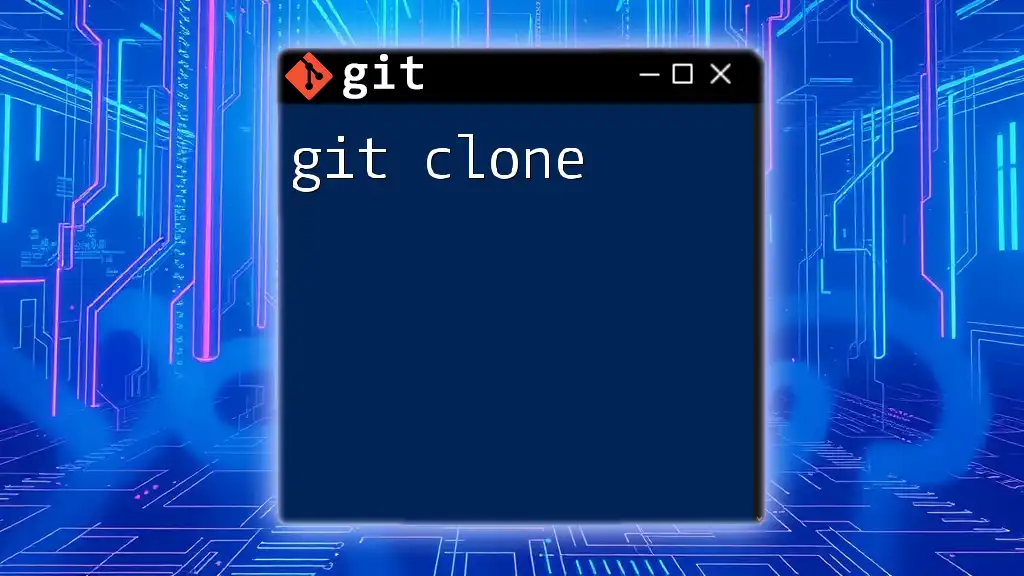
Common Git Commands for Managing LLVM Projects
Staging and Committing Changes
In Git, changes must be staged before being committed. Staging allows you to select which changes you want to include in a commit, providing flexibility. Here’s how to stage and commit your changes:
git add .
git commit -m "Initial commit of LLVM project"
In this command, `git add .` stages all changes in the directory, while `git commit -m "..."` creates a commit with a message describing the changes.
Branching Strategies
Branches allow you to diverge from the main line of development and continue to work independently. A common strategy is to maintain a `main` or `master` branch for stable, production-ready code, while creating separate branches for features, improvements, or experiments. Here’s how to create a new branch for a feature:
git checkout -b feature/new-optimizer
This command creates a new branch and switches you to it, allowing you to start working without affecting the main project.
Merging Changes
When the work on the feature branch is complete, you will want to merge it back into your main branch. This command will help you do that:
git merge feature/new-optimizer
If there are conflicts between branches, Git will prompt you to resolve them, ensuring that merges result in a clean, coherent codebase.
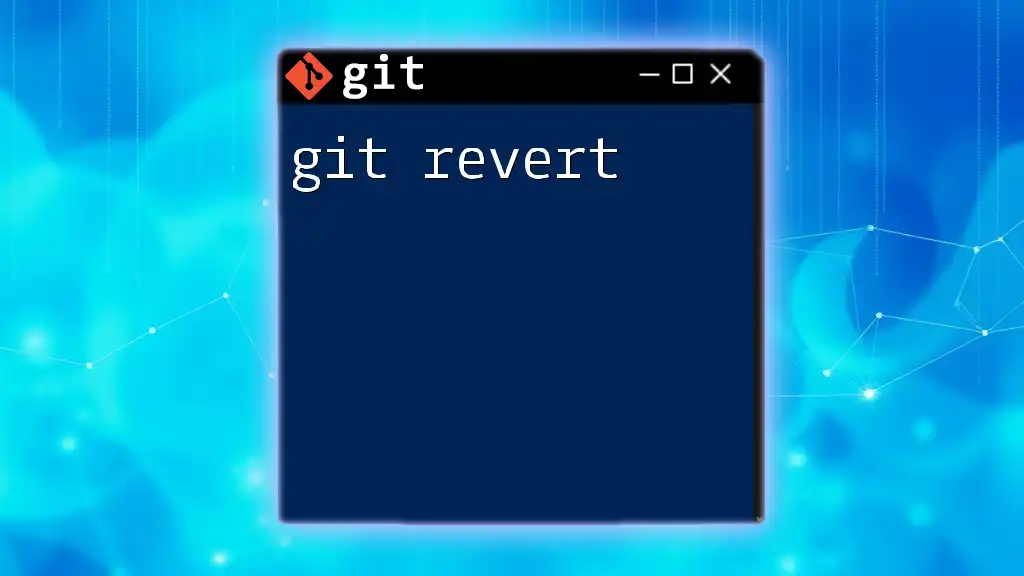
Collaboration with Git and LLVM
Cloning an Existing LLVM Repository
If you want to work on an existing LLVM project, you can clone its repository. For instance, to clone the official LLVM project, use the following command:
git clone https://github.com/llvm/llvm-project.git
This will create a local copy of the repository, including all its history and branches.
Working with Remote Repositories
Collaborating on remote repositories increases efficiency. Fundamental commands include pushing local changes to a remote repository and pulling changes from it:
git push origin main
git pull origin main
These commands ensure that your changes are synchronized with the central repository, keeping your team in sync.
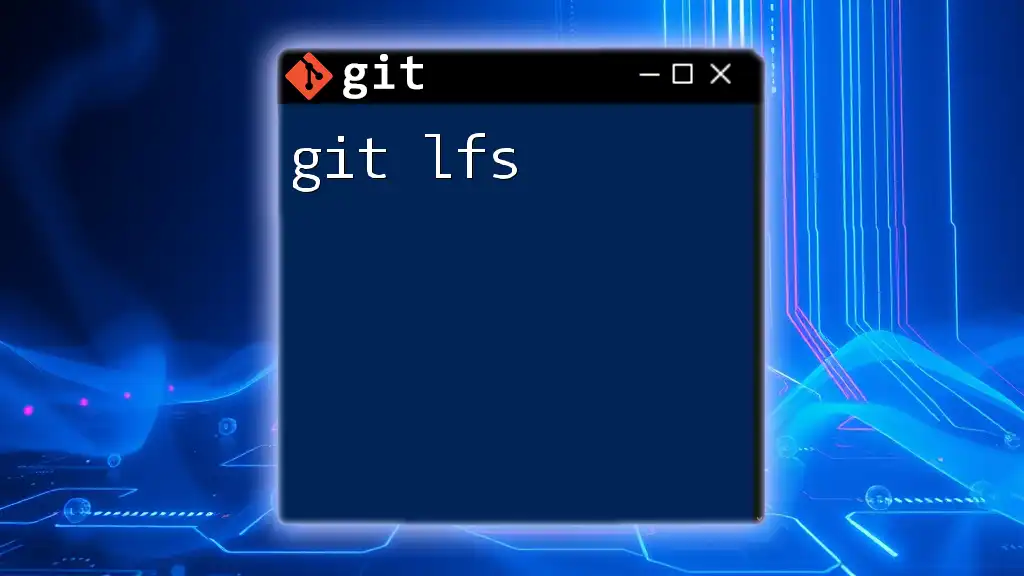
Using Git Hooks with LLVM Projects
Setting Up Pre-commit Hooks
Git hooks are scripts that automatically run on certain events in the Git lifecycle. A popular use is the pre-commit hook, which can enforce code quality by running tests or linters before allowing a commit.
To set up a pre-commit hook:
- Navigate to the `.git/hooks` directory.
- Create a new file named `pre-commit` and make it executable:
touch pre-commit chmod +x pre-commit
Example of a Simple Hook Script
Here’s a simple pre-commit hook script that runs tests:
#!/bin/sh
# Pre-commit hook script to run tests
make test
This script ensures that tests run before any commit is finalized, preventing potentially faulty code from being integrated into the codebase.
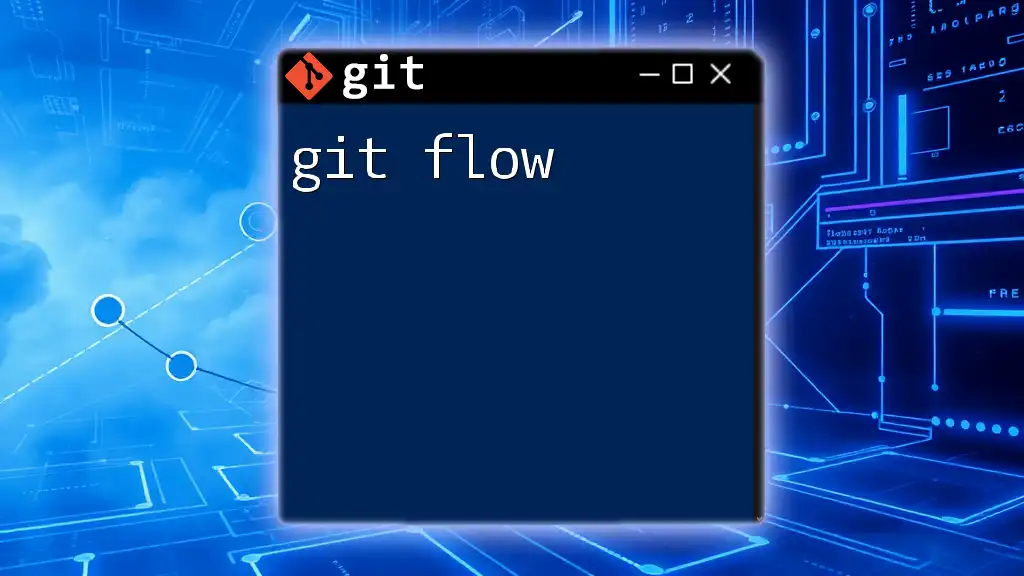
Best Practices for Git in LLVM Development
Commit Often, Commit Early
Frequent commits can capture incremental changes, which makes it easier to locate issues and understand project evolution. Strive to commit every small change that improves the code, rather than piling up large changes that might obscure the history.
Writing Meaningful Commit Messages
Clear, concise commit messages enhance understanding for yourself and your collaborators. Instead of vague messages like "fixed stuff," aim for specific descriptions, such as "Refactor optimizer to improve efficiency in phase X."
Using Tags for Release Management
Tags are essential for marking specific versions of your software, enabling straightforward retrieval of state at a given point:
git tag -a v1.0 -m "Release version 1.0"
Using tags allows you to easily access and manage versions, which is crucial for collaboration and software distribution.
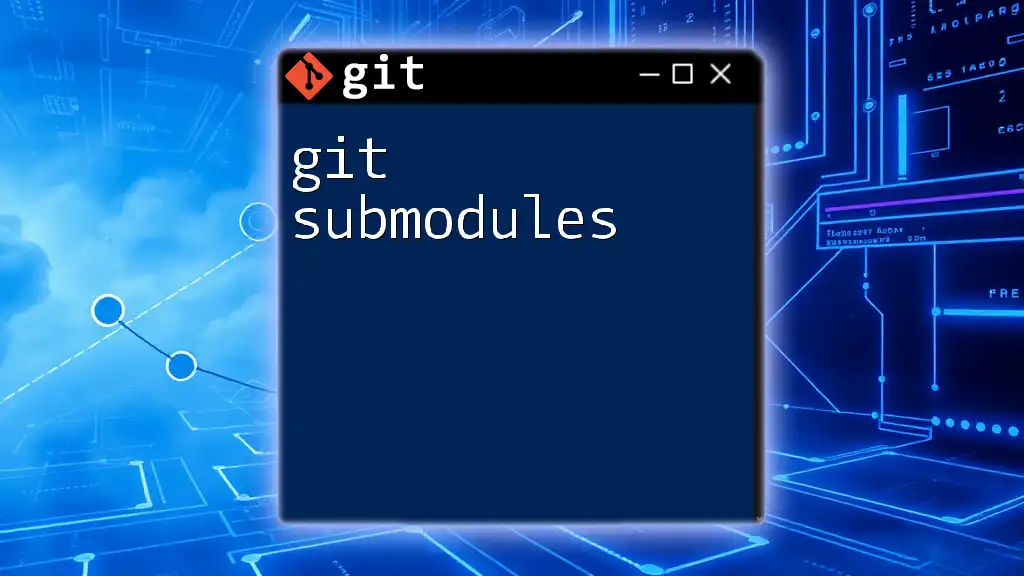
Troubleshooting Common Git Issues in LLVM Projects
Resolving Merge Conflicts
Merge conflicts arise when changes in different branches collide. If you find a merge conflict, Git will indicate which files need attention. You can manually edit the files to resolve conflicts, then stage and commit the resolved files.
Reverting Changes
If you need to undo changes, whether they are staged or committed, Git provides commands that allow you to revert back to a previous state. For instance, to revert the most recent commit:
git revert HEAD
This command creates a new commit that undoes the changes made in the last commit, allowing you to maintain the project history.
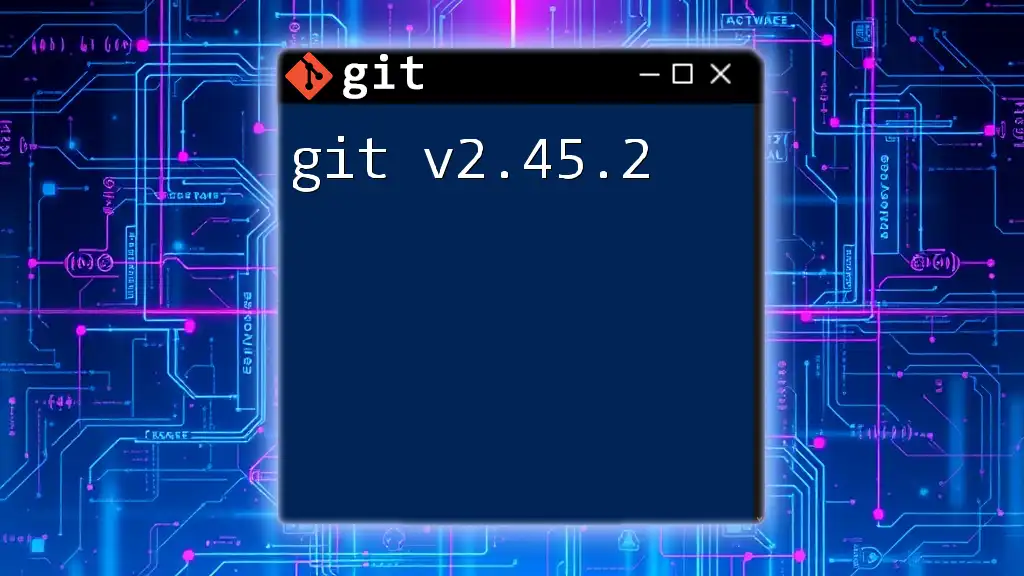
Conclusion
Combining Git with LLVM provides a powerful toolkit for managing code efficiently and collaboratively. Mastering Git commands enhances your ability to maintain version control, collaborate with others, and troubleshoot problems effectively. Leveraging these tools can significantly improve your development workflow, ensuring smoother project execution and better software quality.
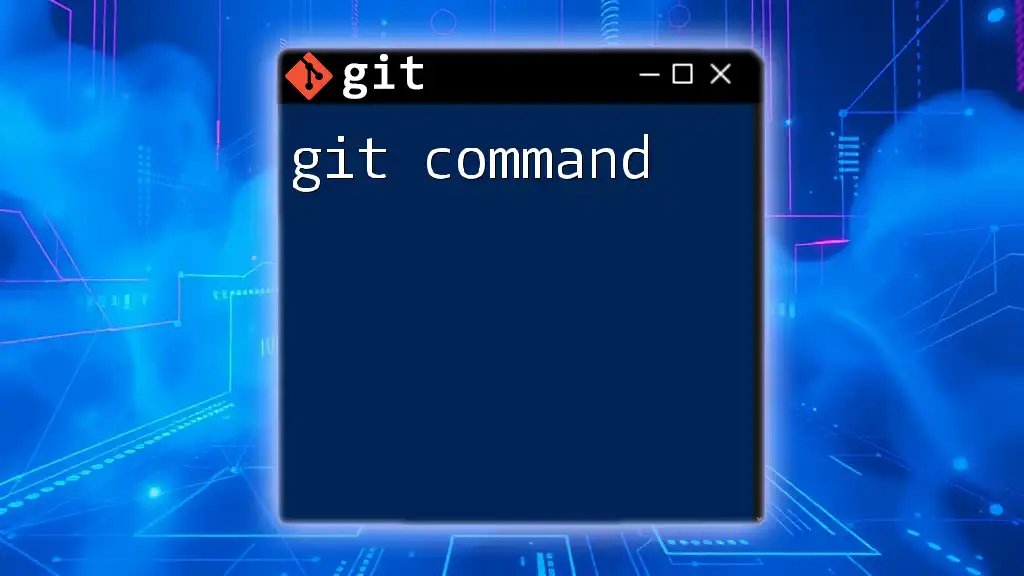
Additional Resources
To further enhance your understanding and skills, explore the official Git and LLVM documentation. Numerous tutorials and tools are available online to aid in mastering version control and development with LLVM, empowering you to take your projects to the next level.