The `git log` command displays a chronological list of commits in the current branch, along with details such as the commit hash, author, date, and message.
git log --oneline --graph --decorate
What is `git log`?
`git log` is a fundamental command in Git used to view the commit history of a repository. It allows developers to track changes and gain insights into the evolution of their projects. Understanding the full history is crucial for effective collaboration and maintaining awareness of how a project has developed over time.
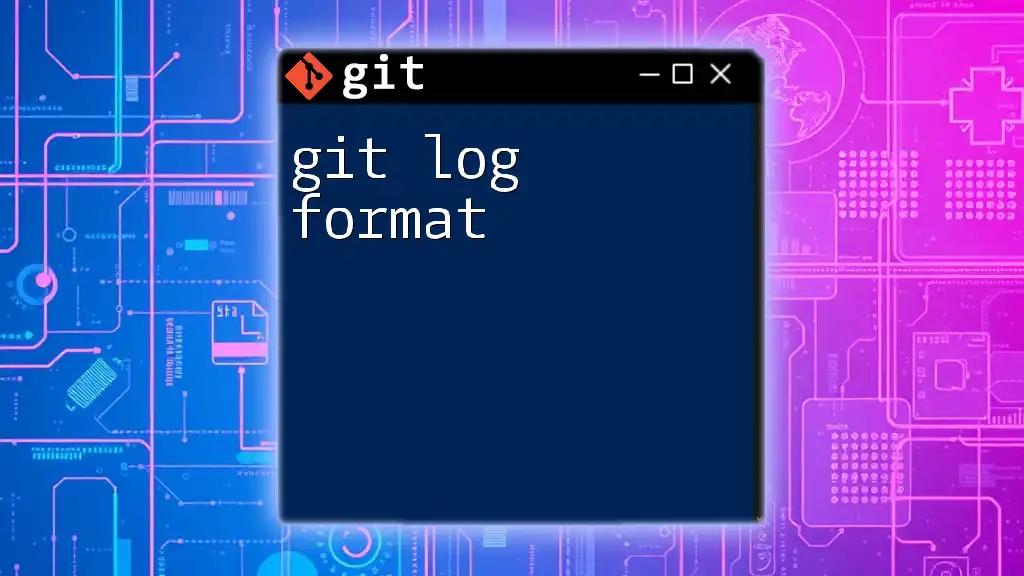
Why is `git log` useful?
The usefulness of `git log` stems from its ability to:
- Track project changes: Every time a commit is made, it records what changes were made, by whom, and when.
- Audit changes and collaboration: It provides a detailed history that can be referenced when collaborating with team members, ensuring everyone is aligned.
- Understand commit history: A clear understanding of the commit history helps in debugging, code reviews, and identifying when specific changes were introduced.
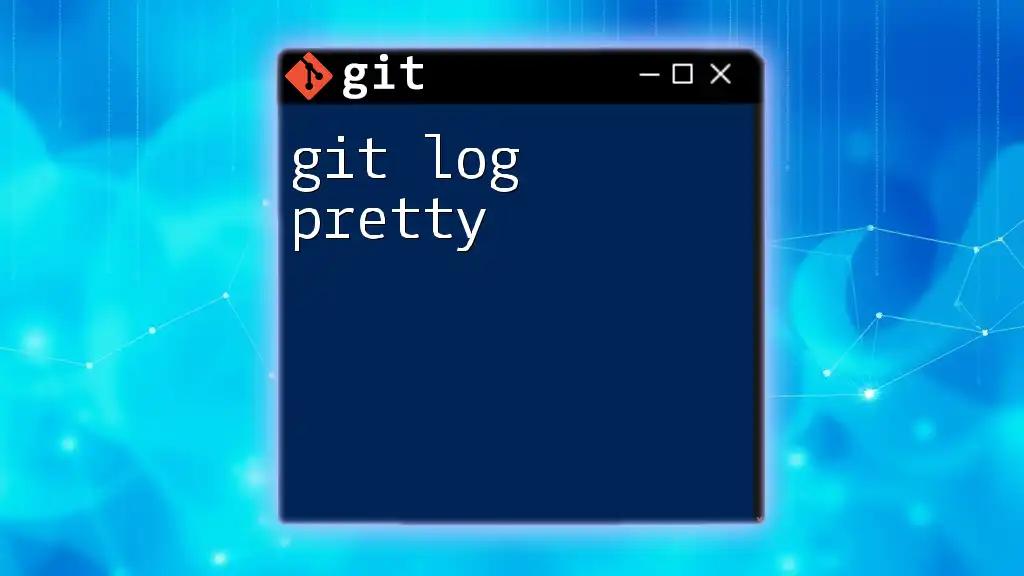
Basic Usage of `git log`
How to run `git log`
To execute the basic `git log` command, simply type:
git log
Upon running this command, you'll see a list of commits that includes the commit hash, author, date, and the commit message. Each entry provides context about the changes made, enabling you to trace the project's history easily.
Basic command options
Understanding the basic structure of the `git log` command and its options can significantly enhance how you interact with your project's history.
Using `--pretty`
By using the `--pretty` flag, you can change how the log output is formatted. For example:
git log --pretty=oneline
This command will display each commit on a single line, showing the commit hash and message. Other format options include:
- `--pretty=short`: Shows a condensed commit entry.
- `--pretty=format:"%h %s"`: Customizes the output to display the commit hash (`%h`) followed by the subject line of the commit message (`%s`).
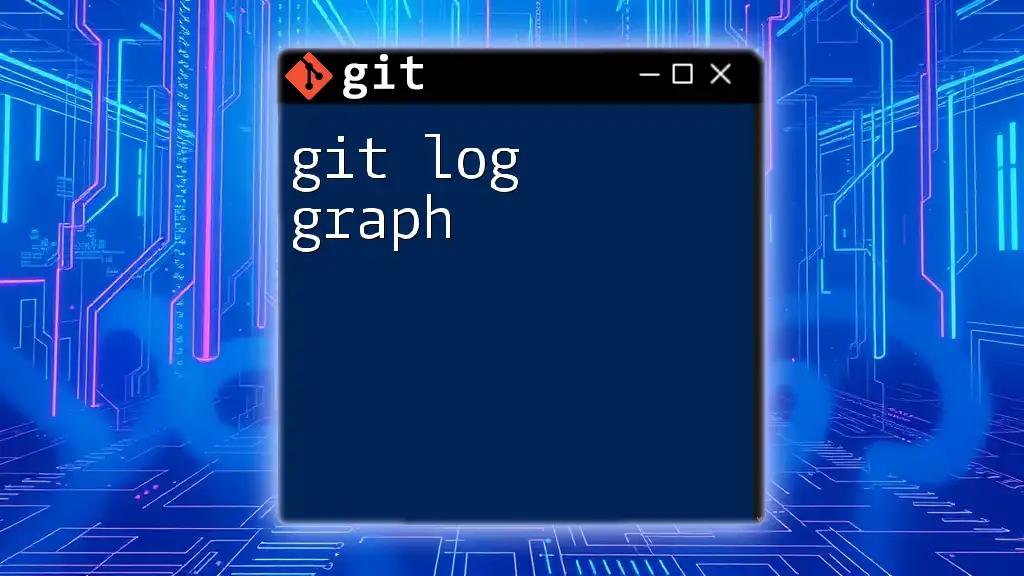
Customizing Output with `git log`
Formatting commits
You can reshape the appearance of the output with different formatting options. The `--oneline` flag provides a succinct view that is easy to read:
git log --oneline
This will list each commit's hash and message in a single line, making it simple to get an overview of the history.
Filtering commits
Filtering the log is essential when you need to zoom in on specific types of changes.
Using `--author`
To filter commits by author, you can use the `--author` flag. For example:
git log --author="John Doe"
This command will display only the commits made by the author named John Doe, providing a focused look at their contributions.
Committing date filters
Date filters can also be beneficial. Using `--since` and `--until`, you can target commits within specific date ranges:
git log --since="1 week ago"
git log --until="2023-01-01"
These commands allow you to examine changes made during a particular timeframe, helpful for project milestones or deadlines.
Displaying specific files
When you need to assess changes made to a specific file, `git log` can be used as follows:
git log -- path/to/file.txt
This command will show you the commit history affecting only the specified file, streamlining the review process for particular areas of the codebase.
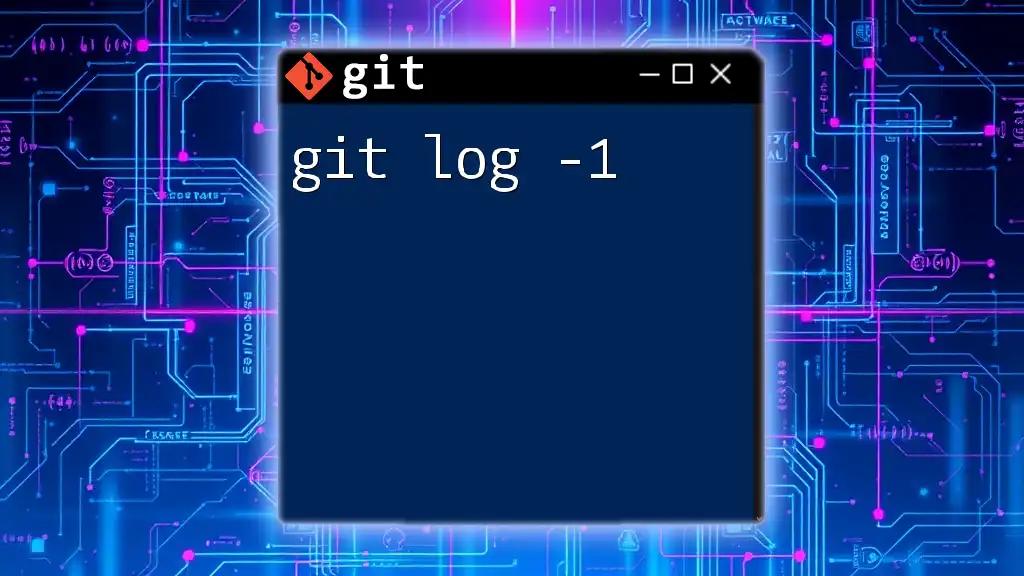
Advanced `git log` Options
Merging commits
In larger projects, it’s important to monitor merges. Using:
git log --merges
You can filter the log to show only commits representing merges. This is particularly useful for understanding integration points in collaborative workflows.
Graphical representation of commits
Visualizing the commit history can enhance comprehension. The `--graph` flag creates a visual representation of the commit graph:
git log --graph --oneline
This displays a visual tree structure alongside each commit message, illustrating branches and merges in an easily digestible format.
Combining multiple options
For those who want a more tailored view, you can combine options. For example:
git log --author="John Doe" --since="1 month ago" --graph
This command allows you to visualize John Doe’s commits over the last month, providing both author-focused and timeline-centric perspectives.
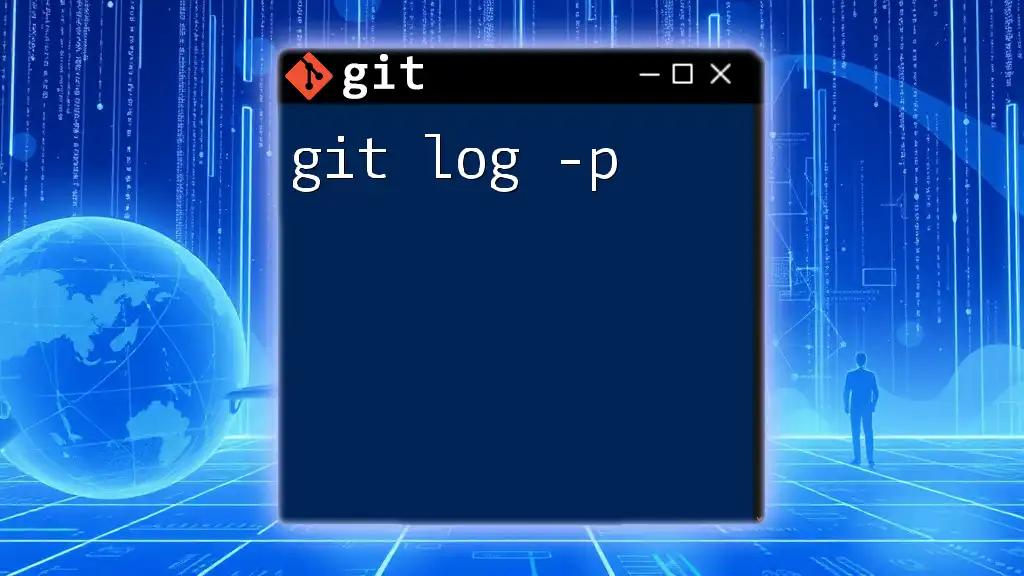
Practical Examples of `git log` in Real Projects
Using `git log` for code reviews
In code reviews, `git log` is an invaluable tool. You can rapidly navigate the history of changes made before a merge, allowing for a deeper understanding of the updates being proposed. This insight can lead to informed discussions and decision-making among team members.
Finding changes that introduced bugs
If a bug arises, `git log` can help trace the changes that introduced it. You can visualize the history to identify potentially problematic commits. Additionally, using `git blame` in conjunction with `git log` can provide further detail on who last modified specific lines of code:
git blame <file>
This highlights the most recent commits that affected the file, allowing you to identify changes that may have led to issues.
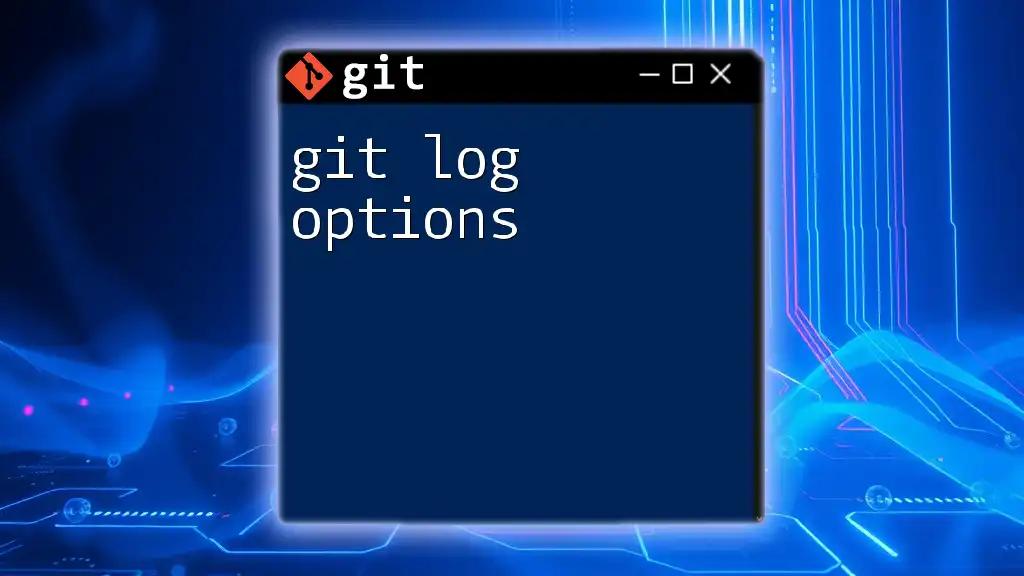
Conclusion
Recapping the key points, `git log` is a powerful command that serves as a cornerstone in understanding a project's history. Its comprehensive features allow users to filter, format, and visualize commit data effectively. By mastering the `git log`, you can significantly enhance your workflow, making version control much more efficient and transparent.
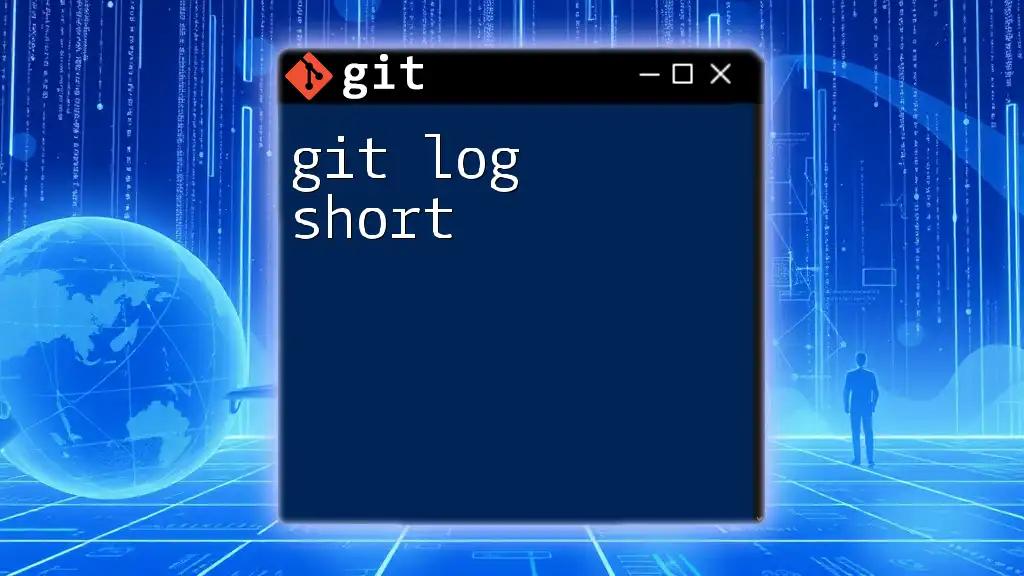
Further Resources and Learning
To extend your knowledge about `git log` and other Git functionalities, refer to the [official Git documentation](https://git-scm.com/doc). You may also explore various tutorials available online that delve into advanced Git commands. Practicing with `git log` in your projects will fortify your skills and expedite your command over version control systems.