The `git log` command allows you to view commit history, and you can search for specific commits using keywords, author names, or even dates.
Here's a code snippet to search for commits containing the keyword "fix":
git log --grep="fix"
Understanding `git log`
What is `git log`?
`git log` is a powerful command that allows developers to explore the history of commits in a Git repository. Each commit contains essential information, including the author's name, timestamp, and a descriptive message about the changes made. Understanding this command is crucial for efficient version control, enabling you to trace the evolution of your project and identify specific changes when needed.
Default Behavior of `git log`
When you run `git log` without any options, it displays a chronological list of all commits made to the repository. The default output includes details like the commit SHA-1 hash, author, date, and commit message—all presented in a standard format. This standard view, while informative, can quickly become overwhelming for larger projects.
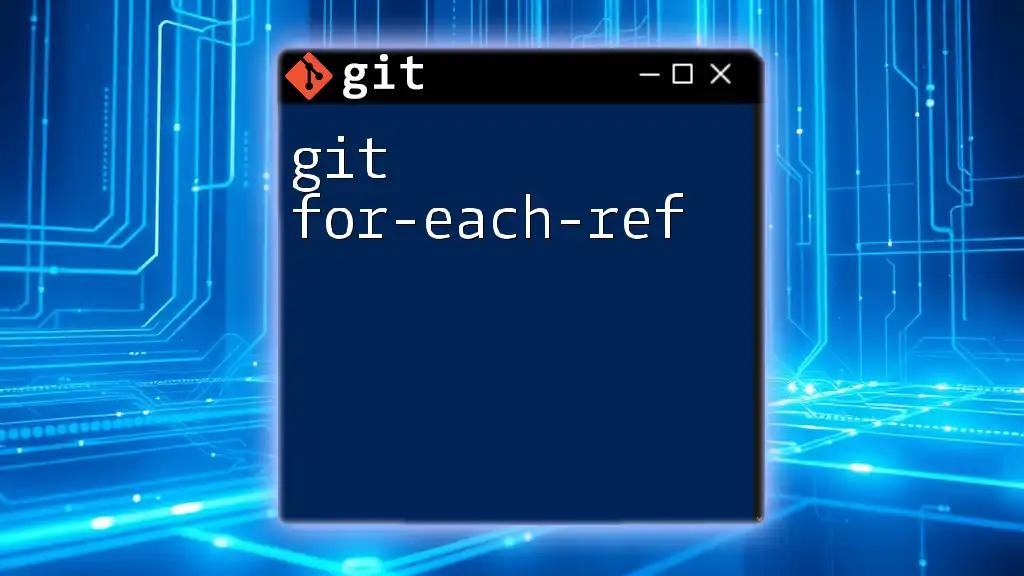
Searching with `git log`
Why Search Commits?
Finding specific commits or changes can be a time-consuming process if you don't utilize search features. Searching through your commit history allows you to quickly locate relevant changes, track down bugs, or review project evolution. This not only saves time but also enhances productivity, as you can focus on specific areas of interest without sifting through every commit.
Basic Search Commands
Searching by Commit Message
Using the `--grep` option, you can filter commits by keywords in the commit message. This is particularly useful for identifying bug fixes or feature additions based on your convention in commit messages.
git log --grep="fix"
This command will return all commits containing the word "fix," allowing you to quickly access all relevant changes. Make sure to use specific keywords to refine your search.
Searching by Author
To find commits made by a specific contributor, use the `--author` option. This is helpful for team-oriented projects where tracking contributions is essential.
git log --author="John Doe"
This command will display all commits authored by "John Doe," making it easy to review contributions or gather insights regarding a specific developer's work.
Advanced Search Techniques
Searching by Date
Narrowing your search to a specific timeframe can significantly enhance efficiency. You can filter commits by specifying a date range using the `--since` and `--until` options.
git log --since="2023-01-01" --until="2023-10-01"
This command shows all commits made between January 1, 2023, and October 1, 2023. Make sure to format dates in a way that Git recognizes; popular formats include "YYYY-MM-DD," "yesterday," or "2 weeks ago."
Combining Search Options
When you have multiple criteria in mind, combining options can refine your search further. For example, to find all commits by a specific author within a certain timeframe, you can combine the options like this:
git log --author="John Doe" --since="2023-01-01"
This command will list all commits by "John Doe" since January 1, 2023, providing a precise view of their contributions during that period.
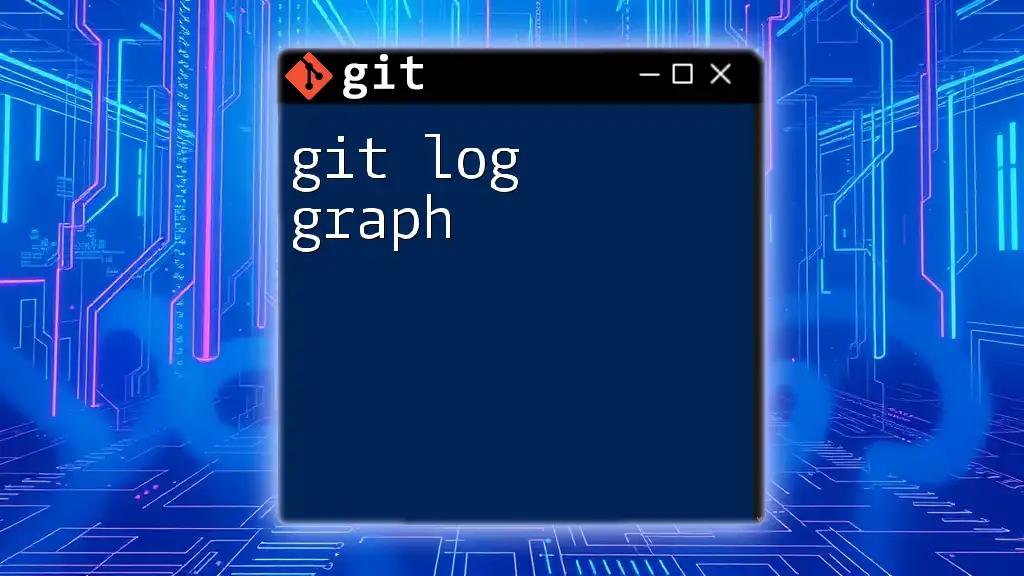
Enhanced Output Formats
Customizing Git Log Output
Improving the readability of your commit output can make a significant difference in efficiency. You can alter the format using options that tailor the display to fit your needs.
One Line Format
For a clean and concise view, you can use the `--oneline` option. This presents each commit on a single line, displaying only the commit hash and message.
git log --oneline
While this format is beneficial for a quick overview, it may lack context regarding specific authors or commit times.
Detailed Format
If you require more detailed information, you can customize output further using the `--pretty` option. This allows you to specify what fields to display.
git log --pretty=format:"%h - %an, %ar : %s"
In this format:
- `%h`: abbreviated commit hash
- `%an`: author name
- `%ar`: author date in relative format (e.g., "2 weeks ago")
- `%s`: commit message
This format strikes a balance between brevity and detail, offering enough information for most users without overwhelming them.
Tree View and Graphical Representation
Displaying the Commit Graph
Visualizing the commit history can elucidate complex relationships between branches. The `--graph` flag helps you visualize the commit history in a tree-like structure.
git log --graph --oneline
This command displays the commit history alongside a visual representation of branching and merging. Understanding the project's flow can help you grasp how changes evolved over time.
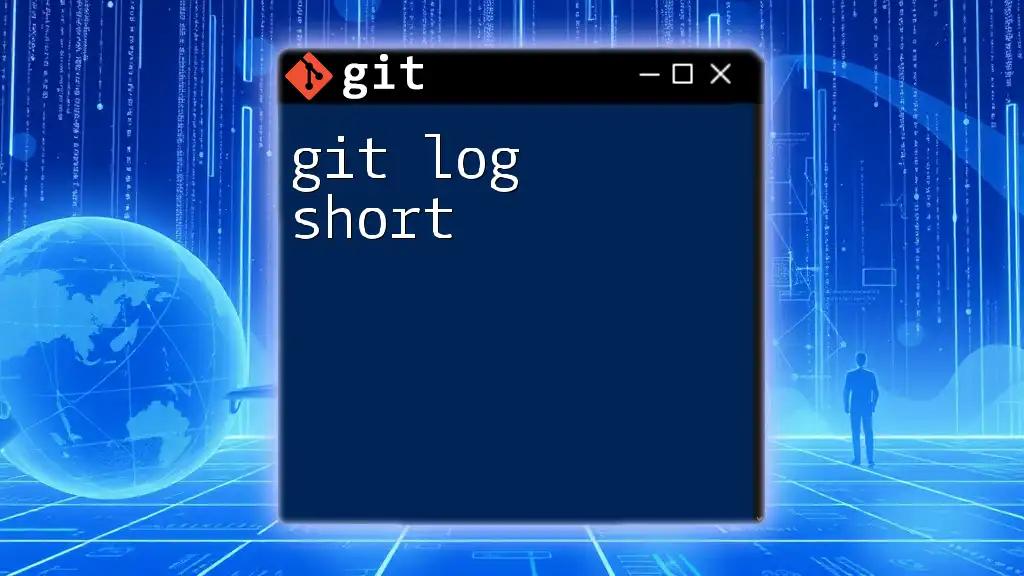
Searching Within File Changes
Using `git log` with Path Specification
It's often useful to examine commits that affected specific files, especially in larger projects. You can specify a file path to filter the results.
git log -- path/to/file
Running this command reveals all commits that modified the specified file, allowing you to gain insights without needing to sift through the entire commit history.
Searching for Changes with `--patch` or `-p`
If you're interested in seeing the actual changes made in each commit, you can add the `-p` or `--patch` option to your command.
git log -p
This command shows the commit history alongside the detailed diffs for each change. This is particularly helpful for code reviews and understanding the impact of changes on your codebase.
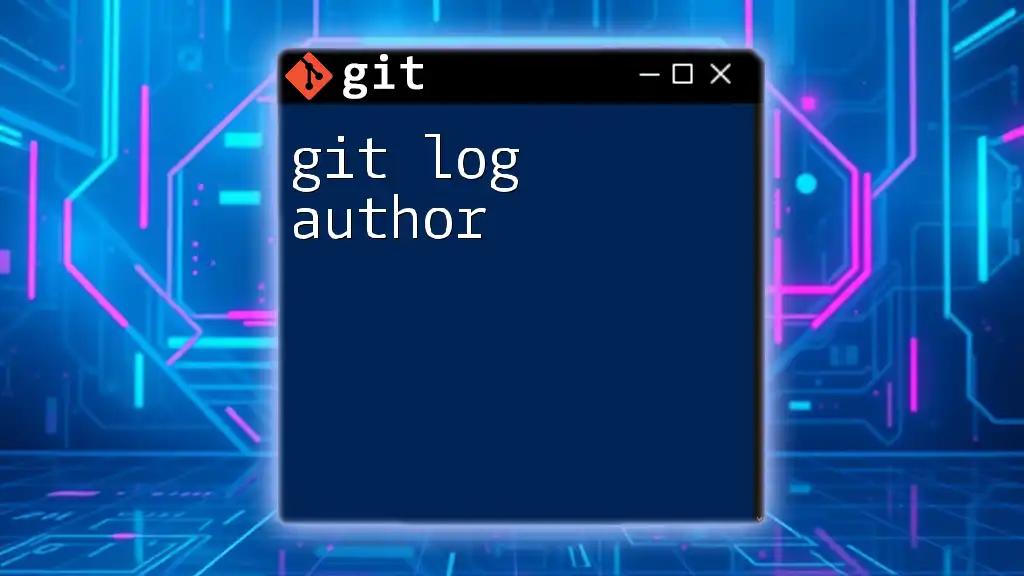
Practical Tips for Effective Searching
Best Practices for Using `git log`
Regularly Commit Changes: Committing changes often not only provides a valuable history but also creates more meaningful search results. Frequent commits encourage developers to document their progress accurately.
Meaningful Commit Messages: Always write descriptive commit messages. Clear, informative messages facilitate easier searching, so consider adopting conventions that clarify the purpose of the changes.
Keep Your History Clean: Utilize techniques like rebasing to streamline your commit history. A clean history is easier to navigate and understand, making both `git log search` and interpretation less daunting.
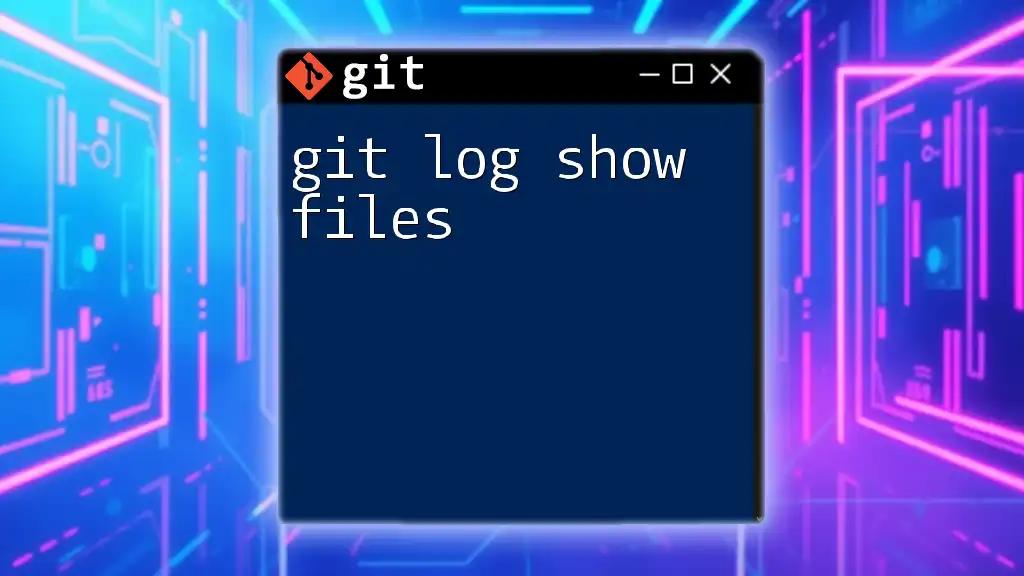
Common Errors and Troubleshooting
Common Mistakes in Searching
Sometimes users misunderstand the options available or misformulate their commands. Double-checking your command syntax can often prevent frustration. Always refer to the documentation if you're unsure.
Troubleshooting Search Queries
When commands don’t return expected results, consider refining your search parameters or checking your branch context. Ensure that you're in the correct repository or branch when executing your queries.
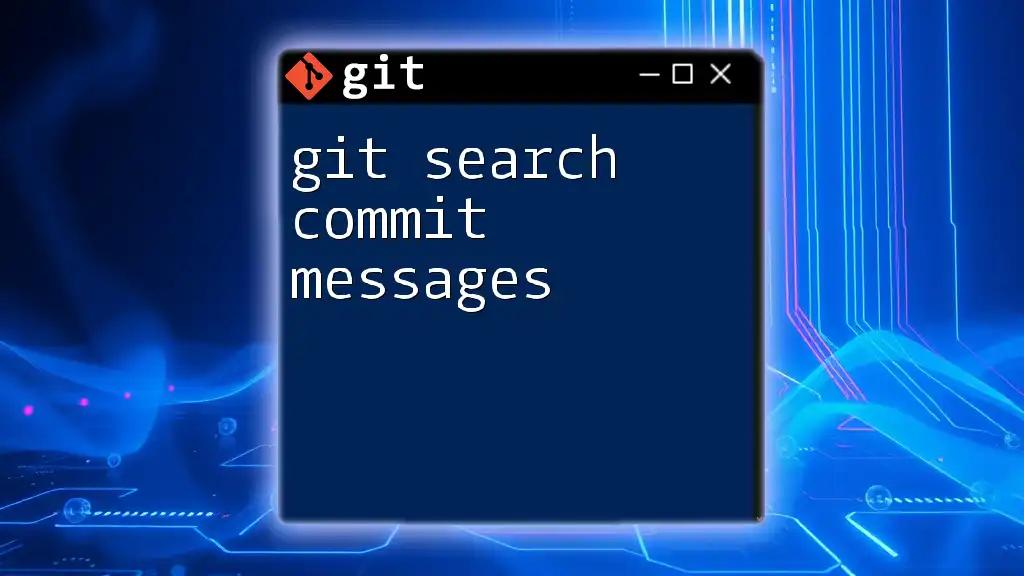
Conclusion
Mastering the `git log search` capabilities transforms how you interact with your repository. With techniques ranging from basic message searches to advanced filtering by author or date, you can efficiently navigate your commit history. By understanding how to customize the output and explore changes made to specific files, you amplify your productivity and enhance your grasp of the project’s evolution. Embrace these practices to harness the full potential of Git in your version control process.