The `git log -p` command displays the commit history along with the differences introduced in each commit, providing a patch-like view of changes.
git log -p
What is `git log`?
`git log` is a powerful command in Git that allows you to view the history of your project. It displays a chronological list of commits, serving as the main tool for tracking changes made to the codebase. Understanding this command is essential to effectively navigate through a project's history, find specific changes, and analyze how the code has evolved over time.
Common Uses of `git log`
The `git log` command can be utilized for various purposes:
- Viewing commit history: Developers can see the timeline of all changes made, allowing them to understand when and what changes were introduced.
- Finding specific commits or changes: By searching through the commit history, a developer can locate a particular change that may have caused an issue.
- Understanding the evolution of the project: It provides insights into decision-making processes, feature additions, and how the code has adapted over time.
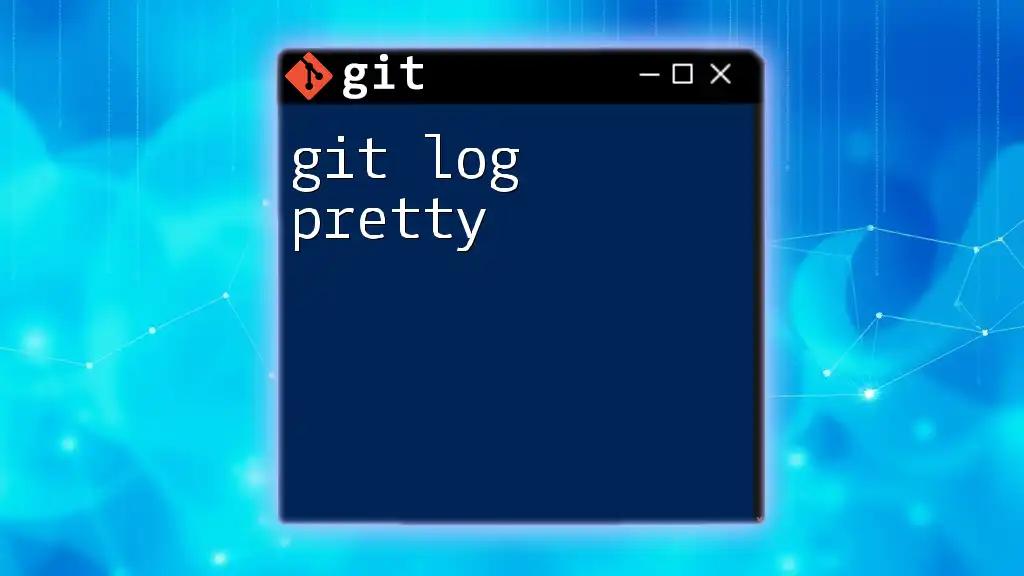
Understanding the `-p` Option
The `-p` option, short for "patch," enhances the output of `git log` by displaying the actual code changes made in each commit. This is crucial for understanding not just what changes were made, but how they impact the codebase.
Why Use `-p`?
Using `git log -p` allows developers to examine the diffs (differences) between commits, making it easier to comprehend changes at the line level. This is particularly useful for:
- Code reviews: It helps teams review changes before integrating them into the main branch.
- Debugging: Understanding changes that may have introduced bugs.
- Learning: Developers can learn from code changes made by others, understanding best practices and the rationale behind decisions.
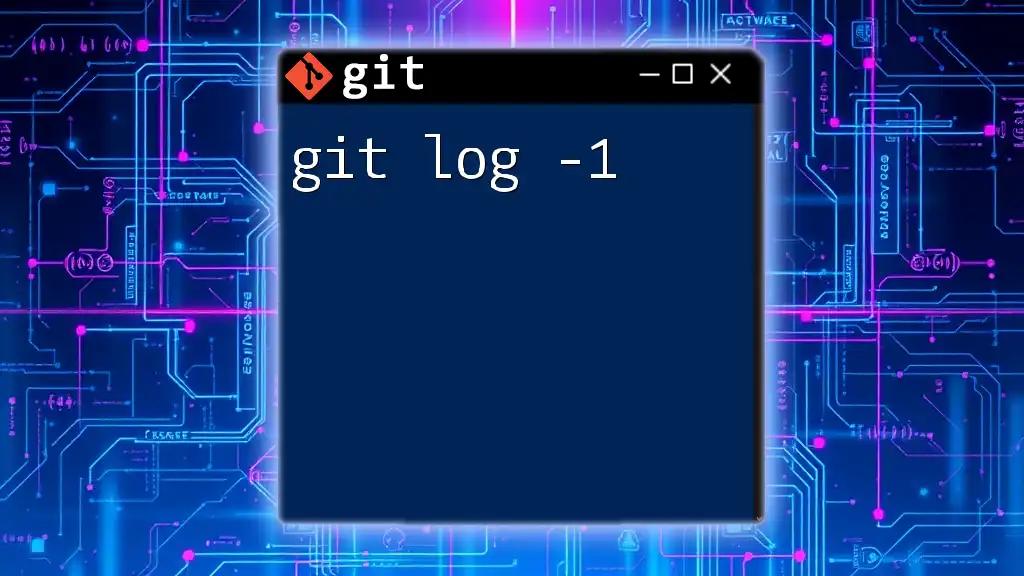
Basic Syntax of `git log -p`
The basic syntax for the command is straightforward. You simply type:
git log -p
This command will list all commit history along with the corresponding patches (diffs) for each commit. You can also tailor the output further with additional options to make it more relevant to your needs.
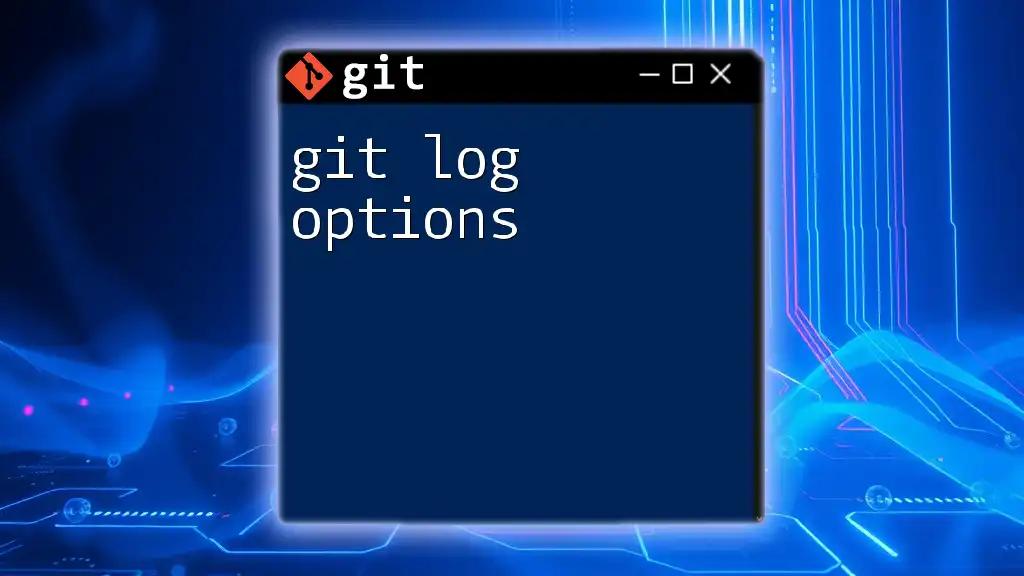
How to Use `git log -p`
Basic Usage
To run the command, just execute:
git log -p
Upon running this command, you can expect an output that includes information about each commit and the differences in the code that each commit represents. This output can be lengthy, depending on the number of commits.
Tailoring Your Output with Options
You can modify the output of `git log -p` for a more focused view. For instance:
- Using `--author` to filter by author:
git log -p --author="Author Name"
This command filters the results to only include commits made by a specified author.
- Utilizing `--since` and `--until` to focus on a specific timeframe:
git log -p --since="2023-01-01" --until="2023-12-31"
This option allows you to limit the log to commits made within a specific date range.
- Combining multiple options for better insights:
git log -p --author="Author Name" --since="2023-01-01"
By combining options, you can precisely view the history that matters most to you.
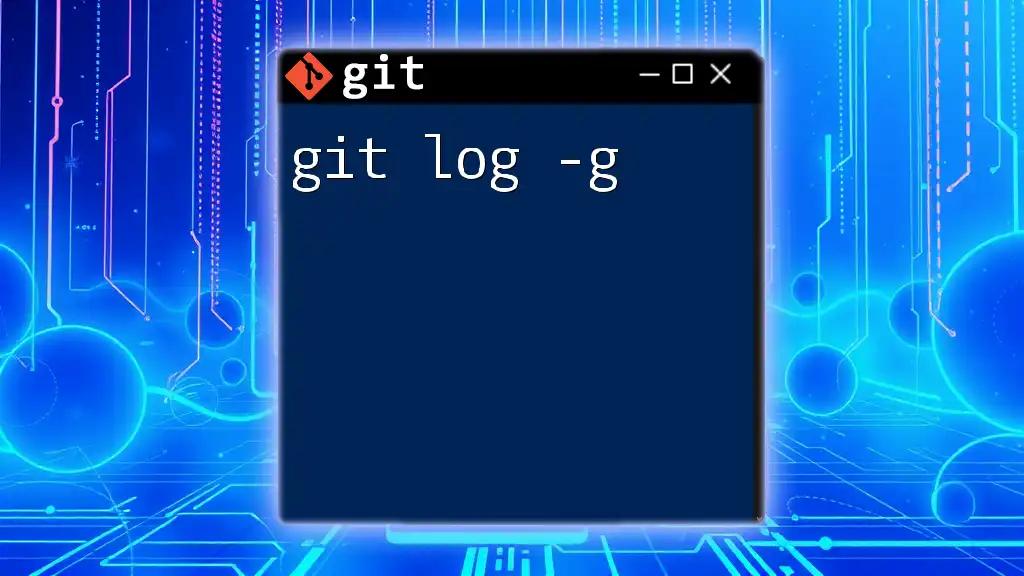
Interpreting the Output of `git log -p`
Breakdown of the Output
When you run `git log -p`, the output comprises several key components:
- Commit hash: This unique identifier for each commit allows you to reference specific changes.
- Author information: Displays who made the changes, providing context around the commit.
- Date and time of commit: Indicates when the changes were made.
- Commit message: A brief description of the changes helps in understanding the purpose of the commit.
- Diff of changes: This is where the real action happens, showing the actual lines added or removed from the codebase.
Understanding the Diff
The diffs displayed in the output show how the code has changed from one version to another.
- Lines marked with a `+` represent additions, illustrating what new code has been introduced.
- Lines marked with a `-` represent deletions, highlighting what has been removed.
This format helps in quickly understanding how the code has evolved and what impact those changes may have.
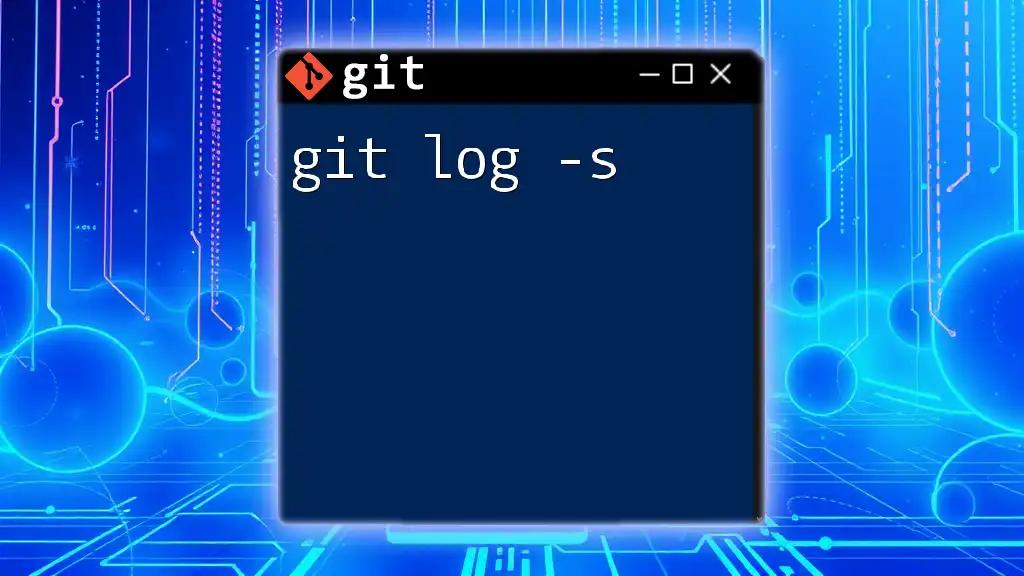
Advanced Usage Scenarios
Viewing Specific File Changes
If you're interested in understanding changes made to a specific file, you can limit the log to that file:
git log -p path/to/file.txt
This command shows only the commits that affected the specified file, giving you a more granular view of changes.
Comparing Between Branches
In scenarios where you need to compare changes across different branches, `git log -p` proves invaluable:
git log -p branch1..branch2
This will display the commits and changes that are in `branch2` but not in `branch1`, allowing you to see what has been added or modified in the newer branch.
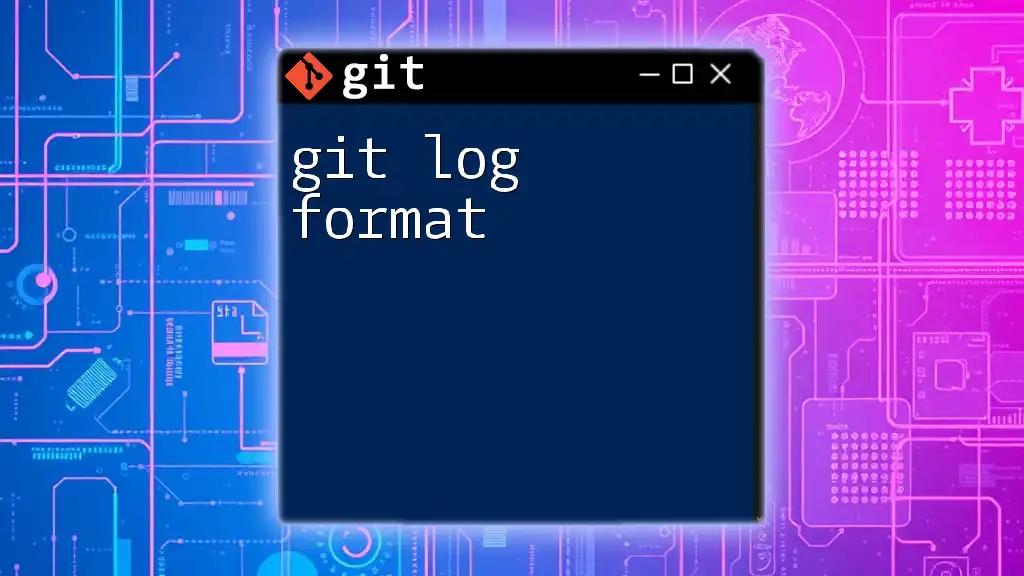
Best Practices for Using `git log -p`
To make the most out of `git log -p`, consider these best practices:
- Use `-p` when debugging: If you encounter an issue, `git log -p` helps trace back through the changes to spot what might be wrong.
- Integrate it into code reviews: Use this command to review changes thoroughly before merging them.
- Combine with other git commands: Pair it with commands like `git show` and `git diff` for a robust understanding of your project history and state.
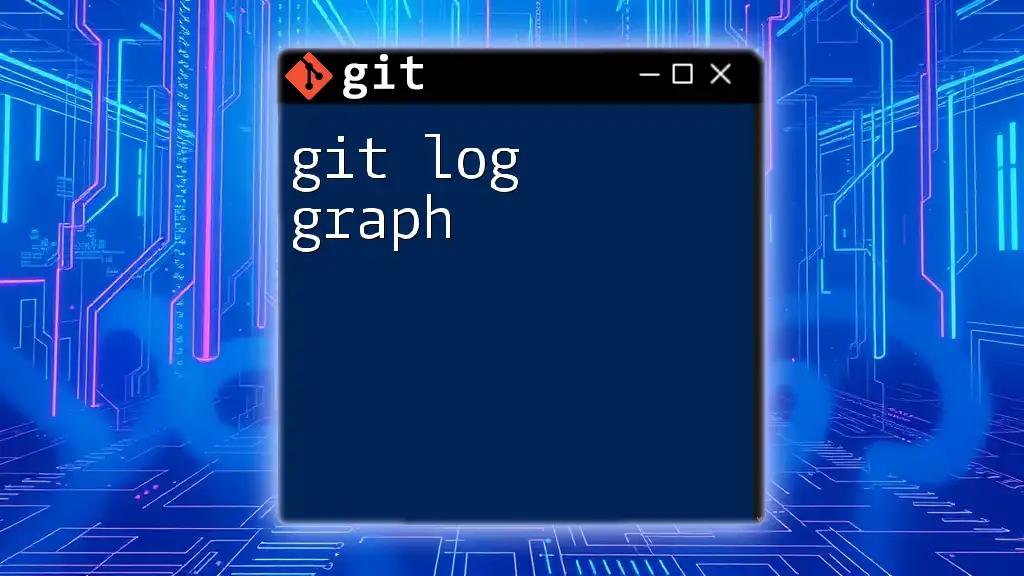
Conclusion
Understanding `git log -p` is fundamental for any developer looking to master Git. This command not only provides a clear picture of project history but also enhances your ability to review and comprehend changes at a detailed level. Practicing with `git log -p` will significantly improve your Git fluency and make you a more effective collaborator.
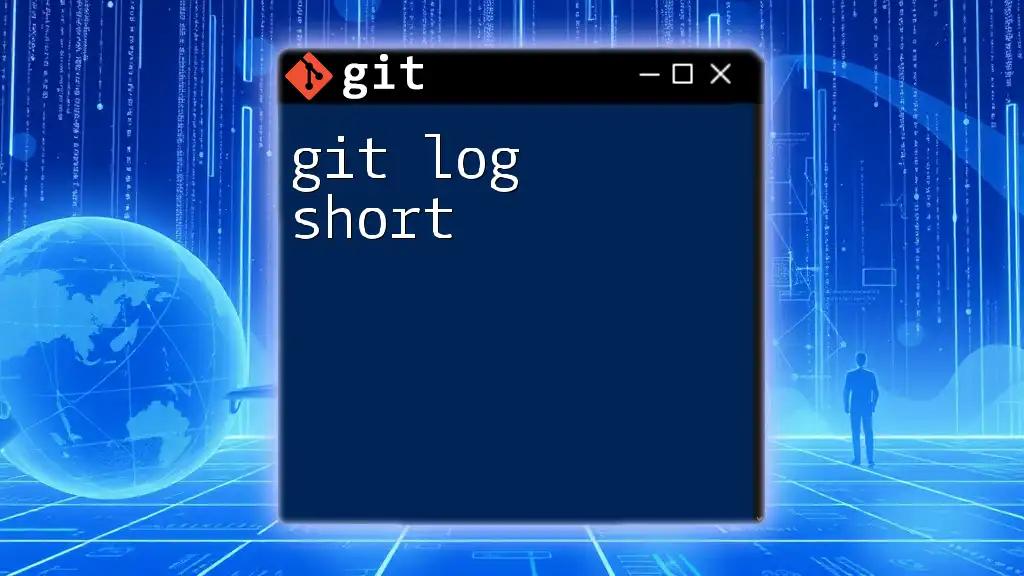
Additional Resources
While this article covers the essentials of `git log -p`, further exploration in the official Git documentation and community forums can significantly enrich your understanding. Consider diving into recommended tutorials and books on Git to elevate your skills even further.